
RT1050 GUI demo using emWin library
Embed:
(wiki syntax)
Show/hide line numbers
GUI_Debug.h
00001 /********************************************************************* 00002 * SEGGER Microcontroller GmbH & Co. KG * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2016 SEGGER Microcontroller GmbH & Co. KG * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.38 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed to NXP Semiconductors USA, Inc. whose 00019 registered office is situated at 411 E. Plumeria Drive, San Jose, 00020 CA 95134, USA solely for the purposes of creating libraries for 00021 NXPs M0, M3/M4 and ARM7/9 processor-based devices, sublicensed and 00022 distributed under the terms and conditions of the NXP End User License 00023 Agreement. 00024 Full source code is available at: www.segger.com 00025 00026 We appreciate your understanding and fairness. 00027 ---------------------------------------------------------------------- 00028 Licensing information 00029 00030 Licensor: SEGGER Microcontroller Systems LLC 00031 Licensed to: NXP Semiconductors, 1109 McKay Dr, M/S 76, San Jose, CA 95131, USA 00032 Licensed SEGGER software: emWin 00033 License number: GUI-00186 00034 License model: emWin License Agreement, dated August 20th 2011 00035 Licensed product: - 00036 Licensed platform: NXP's ARM 7/9, Cortex-M0,M3,M4 00037 Licensed number of seats: - 00038 ---------------------------------------------------------------------- 00039 File : GUI_Debug.h 00040 Purpose : Debug macros 00041 ---------------------------------------------------------------------- 00042 Debug macros for logging 00043 00044 In the GUI Simulation, all output is transferred into the log window. 00045 */ 00046 00047 #ifndef GUI_DEBUG_H 00048 #define GUI_DEBUG_H 00049 00050 #include <stddef.h> 00051 00052 #include "GUI.h" 00053 00054 #define GUI_DEBUG_LEVEL_NOCHECK 0 /* No run time checks are performed */ 00055 #define GUI_DEBUG_LEVEL_CHECK_PARA 1 /* Parameter checks are performed to avoid crashes */ 00056 #define GUI_DEBUG_LEVEL_CHECK_ALL 2 /* Parameter checks and consistency checks are performed */ 00057 #define GUI_DEBUG_LEVEL_LOG_ERRORS 3 /* Errors are recorded */ 00058 #define GUI_DEBUG_LEVEL_LOG_WARNINGS 4 /* Errors & Warnings are recorded */ 00059 #define GUI_DEBUG_LEVEL_LOG_ALL 5 /* Errors, Warnings and Messages are recorded. */ 00060 00061 #ifndef GUI_DEBUG_LEVEL 00062 #ifdef WIN32 00063 #define GUI_DEBUG_LEVEL GUI_DEBUG_LEVEL_LOG_WARNINGS /* Simulation should log all warnings */ 00064 #else 00065 #define GUI_DEBUG_LEVEL GUI_DEBUG_LEVEL_NOCHECK /* For most targets, min. size is important */ 00066 #endif 00067 #endif 00068 00069 #define GUI_LOCK_H(hMem) GUI_ALLOC_LockH(hMem) 00070 #define GUI_UNLOCK_H(pMem) GUI_ALLOC_UnlockH((void **)&pMem) 00071 00072 /******************************************************************* 00073 * 00074 * Commandline 00075 * 00076 ******************************************************************** 00077 */ 00078 00079 #ifdef WIN32 00080 #define GUI_DEBUG_GETCMDLINE() SIM_GetCmdLine() 00081 #else 00082 #define GUI_DEBUG_GETCMDLINE() 0 00083 #endif 00084 00085 /******************************************************************* 00086 * 00087 * Error macros 00088 * 00089 ******************************************************************** 00090 */ 00091 00092 /* Make sure the macros are actually defined */ 00093 00094 #if GUI_DEBUG_LEVEL >= GUI_DEBUG_LEVEL_LOG_ERRORS 00095 #define GUI_DEBUG_ERROROUT(s) GUI_ErrorOut(s) 00096 #define GUI_DEBUG_ERROROUT1(s,p0) GUI_ErrorOut1(s,p0) 00097 #define GUI_DEBUG_ERROROUT2(s,p0,p1) GUI_ErrorOut2(s,p0,p1) 00098 #define GUI_DEBUG_ERROROUT3(s,p0,p1,p2) GUI_ErrorOut3(s,p0,p1,p2) 00099 #define GUI_DEBUG_ERROROUT4(s,p0,p1,p2,p3) GUI_ErrorOut4(s,p0,p1,p2,p3) 00100 #define GUI_DEBUG_ERROROUT_IF(exp,s) { if (exp) GUI_DEBUG_ERROROUT(s); } 00101 #define GUI_DEBUG_ERROROUT1_IF(exp,s,p0) { if (exp) GUI_DEBUG_ERROROUT1(s,p0); } 00102 #define GUI_DEBUG_ERROROUT2_IF(exp,s,p0,p1) { if (exp) GUI_DEBUG_ERROROUT2(s,p0,p1); } 00103 #define GUI_DEBUG_ERROROUT3_IF(exp,s,p0,p1,p2) { if (exp) GUI_DEBUG_ERROROUT3(s,p0,p1,p2); } 00104 #define GUI_DEBUG_ERROROUT4_IF(exp,s,p0,p1,p2,p3) { if (exp) GUI_DEBUG_ERROROUT4(s,p0,p1,p2,p3); } 00105 #else 00106 #define GUI_DEBUG_ERROROUT(s) 00107 #define GUI_DEBUG_ERROROUT1(s,p0) 00108 #define GUI_DEBUG_ERROROUT2(s,p0,p1) 00109 #define GUI_DEBUG_ERROROUT3(s,p0,p1,p2) 00110 #define GUI_DEBUG_ERROROUT4(s,p0,p1,p2,p3) 00111 #define GUI_DEBUG_ERROROUT_IF(exp,s) 00112 #define GUI_DEBUG_ERROROUT1_IF(exp,s,p0) 00113 #define GUI_DEBUG_ERROROUT2_IF(exp,s,p0,p1) 00114 #define GUI_DEBUG_ERROROUT3_IF(exp,s,p0,p1,p2) 00115 #define GUI_DEBUG_ERROROUT4_IF(exp,s,p0,p1,p2,p3) 00116 #endif 00117 00118 /******************************************************************* 00119 * 00120 * Warning macros 00121 * 00122 ******************************************************************** 00123 */ 00124 00125 /* Make sure the macros are actually defined */ 00126 00127 #if GUI_DEBUG_LEVEL >= GUI_DEBUG_LEVEL_LOG_WARNINGS 00128 #define GUI_DEBUG_WARN(s) GUI_Warn(s) 00129 #define GUI_DEBUG_WARN1(s,p0) GUI_Warn1(s,p0) 00130 #define GUI_DEBUG_WARN2(s,p0,p1) GUI_Warn2(s,p0,p1) 00131 #define GUI_DEBUG_WARN3(s,p0,p1,p2) GUI_Warn3(s,p0,p1,p2) 00132 #define GUI_DEBUG_WARN4(s,p0,p1,p2,p3) GUI_Warn4(s,p0,p1,p2,p3) 00133 #define GUI_DEBUG_WARN_IF(exp,s) { if (exp) GUI_DEBUG_WARN(s); } 00134 #define GUI_DEBUG_WARN1_IF(exp,s,p0) { if (exp) GUI_DEBUG_WARN1(s,p0); } 00135 #define GUI_DEBUG_WARN2_IF(exp,s,p0,p1) { if (exp) GUI_DEBUG_WARN2(s,p0,p1); } 00136 #define GUI_DEBUG_WARN3_IF(exp,s,p0,p1,p2) { if (exp) GUI_DEBUG_WARN3(s,p0,p1,p2); } 00137 #define GUI_DEBUG_WARN4_IF(exp,s,p0,p1,p2,p3) { if (exp) GUI_DEBUG_WARN4(s,p0,p1,p2,p3); } 00138 #else 00139 #define GUI_DEBUG_WARN(s) 00140 #define GUI_DEBUG_WARN1(s,p0) 00141 #define GUI_DEBUG_WARN2(s,p0,p1) 00142 #define GUI_DEBUG_WARN3(s,p0,p1,p2) 00143 #define GUI_DEBUG_WARN4(s,p0,p1,p2,p3) 00144 #define GUI_DEBUG_WARN_IF(exp,s) 00145 #define GUI_DEBUG_WARN1_IF(exp,s,p0) 00146 #define GUI_DEBUG_WARN2_IF(exp,s,p0,p1) 00147 #define GUI_DEBUG_WARN3_IF(exp,s,p0,p1,p2) 00148 #define GUI_DEBUG_WARN4_IF(exp,s,p0,p1,p2,p3) 00149 #endif 00150 00151 /******************************************************************* 00152 * 00153 * Logging macros 00154 * 00155 ******************************************************************** 00156 */ 00157 /* Make sure the macros are actually defined */ 00158 00159 #if GUI_DEBUG_LEVEL >= GUI_DEBUG_LEVEL_LOG_ALL 00160 #define GUI_DEBUG_LOG(s) GUI_Log(s) 00161 #define GUI_DEBUG_LOG1(s,p0) GUI_Log1(s,p0) 00162 #define GUI_DEBUG_LOG2(s,p0,p1) GUI_Log2(s,p0,p1) 00163 #define GUI_DEBUG_LOG3(s,p0,p1,p2) GUI_Log3(s,p0,p1,p2) 00164 #define GUI_DEBUG_LOG4(s,p0,p1,p2,p3) GUI_Log4(s,p0,p1,p2,p3) 00165 #define GUI_DEBUG_LOG_IF(exp,s) { if (exp) GUI_DEBUG_LOG(s); } 00166 #define GUI_DEBUG_LOG1_IF(exp,s,p0) { if (exp) GUI_DEBUG_LOG1(s,p0); } 00167 #define GUI_DEBUG_LOG2_IF(exp,s,p0,p1) { if (exp) GUI_DEBUG_LOG2(s,p0,p1); } 00168 #define GUI_DEBUG_LOG3_IF(exp,s,p0,p1,p2) { if (exp) GUI_DEBUG_LOG3(s,p0,p1,p2); } 00169 #define GUI_DEBUG_LOG4_IF(exp,s,p0,p1,p2,p3) { if (exp) GUI_DEBUG_LOG4(s,p0,p1,p2,p3); } 00170 #else 00171 #define GUI_DEBUG_LOG(s) 00172 #define GUI_DEBUG_LOG1(s,p0) 00173 #define GUI_DEBUG_LOG2(s,p0,p1) 00174 #define GUI_DEBUG_LOG3(s,p0,p1,p2) 00175 #define GUI_DEBUG_LOG4(s,p0,p1,p2,p3) 00176 #define GUI_DEBUG_LOG_IF(exp,s) 00177 #define GUI_DEBUG_LOG1_IF(exp,s,p0) 00178 #define GUI_DEBUG_LOG2_IF(exp,s,p0,p1) 00179 #define GUI_DEBUG_LOG3_IF(exp,s,p0,p1,p2) 00180 #define GUI_DEBUG_LOG4_IF(exp,s,p0,p1,p2,p3) 00181 #endif 00182 00183 /******************************************************************* 00184 * 00185 * Asserts 00186 * 00187 ******************************************************************** 00188 */ 00189 #if GUI_DEBUG_LEVEL >= GUI_DEBUG_LEVEL_LOG_ERRORS 00190 #define GUI_DEBUG_ASSERT(exp) { if (!exp) GUI_DEBUG_ERROROUT(#exp); } 00191 #else 00192 #define GUI_DEBUG_ASSERT(exp) 00193 #endif 00194 00195 #endif /* LCD_H */ 00196 00197 00198 00199 00200 /*************************** End of file ****************************/
Generated on Tue Jul 12 2022 19:43:36 by
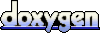