
RT1050 GUI demo using emWin library
Embed:
(wiki syntax)
Show/hide line numbers
GUI_BMP_Private.h
00001 /********************************************************************* 00002 * SEGGER Microcontroller GmbH & Co. KG * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2016 SEGGER Microcontroller GmbH & Co. KG * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.38 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed to NXP Semiconductors USA, Inc. whose 00019 registered office is situated at 411 E. Plumeria Drive, San Jose, 00020 CA 95134, USA solely for the purposes of creating libraries for 00021 NXPs M0, M3/M4 and ARM7/9 processor-based devices, sublicensed and 00022 distributed under the terms and conditions of the NXP End User License 00023 Agreement. 00024 Full source code is available at: www.segger.com 00025 00026 We appreciate your understanding and fairness. 00027 ---------------------------------------------------------------------- 00028 Licensing information 00029 00030 Licensor: SEGGER Microcontroller Systems LLC 00031 Licensed to: NXP Semiconductors, 1109 McKay Dr, M/S 76, San Jose, CA 95131, USA 00032 Licensed SEGGER software: emWin 00033 License number: GUI-00186 00034 License model: emWin License Agreement, dated August 20th 2011 00035 Licensed product: - 00036 Licensed platform: NXP's ARM 7/9, Cortex-M0,M3,M4 00037 Licensed number of seats: - 00038 ---------------------------------------------------------------------- 00039 File : GUI_BMP_Private.h 00040 Purpose : Private header file for GUI_BMP... functions 00041 ---------------------------END-OF-HEADER------------------------------ 00042 */ 00043 00044 #ifndef GUI_BMP_PRIVATE_H 00045 #define GUI_BMP_PRIVATE_H 00046 00047 #include "GUI_Private.h" 00048 00049 /********************************************************************* 00050 * 00051 * Defines 00052 * 00053 ********************************************************************** 00054 */ 00055 #define BI_RGB 0 00056 #define BI_RLE8 1 00057 #define BI_RLE4 2 00058 #define BI_BITFIELDS 3 00059 00060 /********************************************************************* 00061 * 00062 * Types 00063 * 00064 ********************************************************************** 00065 */ 00066 // 00067 // Default parameter structure for reading data from memory 00068 // 00069 typedef struct { 00070 const U8 * pFileData; 00071 } GUI_BMP_PARAM; 00072 00073 // 00074 // Context structure for getting stdio input 00075 // 00076 typedef struct { 00077 GUI_GET_DATA_FUNC * pfGetData; // Function pointer 00078 U32 Off; // Data pointer 00079 void * pParam; // Parameter pointer passed to function 00080 } GUI_BMP_CONTEXT; 00081 00082 // 00083 // Parameter structure for passing several required variables to the 00084 // functions _DrawLine_RGB() and _DrawLine_ARGB() (in GUI_BMP_EnableAlpha.c). 00085 // 00086 typedef struct { 00087 const U8 * pSrc; // Pointer to data 00088 I32 xSrc; // Used to read data 00089 int ySrc; // Used to read data 00090 I32 xSize; 00091 U32 BytesPerPixel; 00092 tLCDDEV_Color2Index * pfColor2Index; 00093 tLCDDEV_Index2Color * pfIndex2Color; // Used to manage bitfield conversion 00094 LCD_API_NEXT_PIXEL * pNextPixel_API; 00095 int x0; // Used to draw data 00096 int y0; // Used to draw data 00097 int x1; // Used to draw data 00098 int y1; // Used to draw data 00099 } GUI_DRAWLINE_INFO; 00100 00101 /********************************************************************* 00102 * 00103 * Interface 00104 * 00105 ********************************************************************** 00106 */ 00107 int GUI_BMP__GetData (void * p, const U8 ** ppData, unsigned NumBytesReq, U32 Off); 00108 int GUI_BMP__Init (GUI_BMP_CONTEXT * pContext, I32 * pWidth, I32 * pHeight, U16 * pBitCount, int * pNumColors, int * pCompression); 00109 int GUI_BMP__ReadData (GUI_BMP_CONTEXT * pContext, int NumBytes, const U8 ** ppData, unsigned StartOfFile); 00110 int GUI_BMP__ReadPalette(GUI_BMP_CONTEXT * pContext, int NumColors); 00111 00112 #endif /* GUI_BMP_PRIVATE_H */ 00113 00114 /*************************** End of file ****************************/
Generated on Tue Jul 12 2022 19:43:36 by
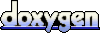