
RT1050 GUI demo using emWin library
Embed:
(wiki syntax)
Show/hide line numbers
GUIDRV_IST3088_Private.h
00001 /********************************************************************* 00002 * SEGGER Microcontroller GmbH & Co. KG * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2016 SEGGER Microcontroller GmbH & Co. KG * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.38 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed to NXP Semiconductors USA, Inc. whose 00019 registered office is situated at 411 E. Plumeria Drive, San Jose, 00020 CA 95134, USA solely for the purposes of creating libraries for 00021 NXPs M0, M3/M4 and ARM7/9 processor-based devices, sublicensed and 00022 distributed under the terms and conditions of the NXP End User License 00023 Agreement. 00024 Full source code is available at: www.segger.com 00025 00026 We appreciate your understanding and fairness. 00027 ---------------------------------------------------------------------- 00028 Licensing information 00029 00030 Licensor: SEGGER Microcontroller Systems LLC 00031 Licensed to: NXP Semiconductors, 1109 McKay Dr, M/S 76, San Jose, CA 95131, USA 00032 Licensed SEGGER software: emWin 00033 License number: GUI-00186 00034 License model: emWin License Agreement, dated August 20th 2011 00035 Licensed product: - 00036 Licensed platform: NXP's ARM 7/9, Cortex-M0,M3,M4 00037 Licensed number of seats: - 00038 ---------------------------------------------------------------------- 00039 File : GUIDRV_IST3088_Private.h 00040 Purpose : Interface definition for GUIDRV_IST3088 driver 00041 ---------------------------END-OF-HEADER------------------------------ 00042 */ 00043 00044 #include "GUIDRV_IST3088.h" 00045 #include "GUIDRV_NoOpt_1_8.h" 00046 00047 #ifndef GUIDRV_IST3088_PRIVATE_H 00048 #define GUIDRV_IST3088_PRIVATE_H 00049 00050 #if defined(__cplusplus) 00051 extern "C" { /* Make sure we have C-declarations in C++ programs */ 00052 #endif 00053 00054 // 00055 // Use unique context identified 00056 // 00057 #define DRIVER_CONTEXT DRIVER_CONTEXT_IST3088 00058 00059 /********************************************************************* 00060 * 00061 * Types 00062 * 00063 ********************************************************************** 00064 */ 00065 typedef struct DRIVER_CONTEXT DRIVER_CONTEXT; 00066 00067 /********************************************************************* 00068 * 00069 * MANAGE_VRAM_CONTEXT 00070 */ 00071 typedef struct { 00072 // 00073 // Function pointers for writing to display controller 00074 // 00075 void (* pfManagePixel) (DRIVER_CONTEXT * pContext, int * px, int * py); 00076 void (* pfManageRect) (DRIVER_CONTEXT * pContext, int * px0, int * py0, int * px1, int * py1); 00077 void (* pfAddDirtyPixelPhys)(DRIVER_CONTEXT * pContext, int x, int y); 00078 void (* pfAddDirtyRectPhys) (DRIVER_CONTEXT * pContext, int x0, int y0, int x1, int y1); 00079 void (* pfAddDirtyPixel) (DRIVER_CONTEXT * pContext, int x, int y); 00080 void (* pfAddDirtyRect) (DRIVER_CONTEXT * pContext, int x0, int y0, int x1, int y1); 00081 LCD_PIXELINDEX (* pfGetPixelIndexPhys)(DRIVER_CONTEXT * pContext, int x, int y); 00082 void (* pfSetPixelIndexPhys)(DRIVER_CONTEXT * pContext, int x, int y, LCD_PIXELINDEX ColorIndex); 00083 void (* pfXorPixelIndexPhys)(DRIVER_CONTEXT * pContext, int x, int y); 00084 LCD_PIXELINDEX (* pfGetPixelIndex) (DRIVER_CONTEXT * pContext, int x, int y); 00085 void (* pfSetPixelIndex) (DRIVER_CONTEXT * pContext, int x, int y, LCD_PIXELINDEX ColorIndex); 00086 void (* pfXorPixelIndex) (DRIVER_CONTEXT * pContext, int x, int y); 00087 void (* pfFlush) (DRIVER_CONTEXT * pContext); 00088 } MANAGE_VMEM_API; 00089 00090 /********************************************************************* 00091 * 00092 * DRIVER_CONTEXT 00093 */ 00094 struct DRIVER_CONTEXT { 00095 // 00096 // Common data 00097 // 00098 int xSize, ySize; 00099 int vxSize, vySize; 00100 int Orientation; 00101 // 00102 // Cache management 00103 // 00104 GUI_RECT rDirty; 00105 // 00106 // Accelerators for calculation 00107 // 00108 int ShortsPerLine; 00109 int BitsPerPixel; 00110 // 00111 // VRAM 00112 // 00113 U16 * pVMEM; 00114 // 00115 // Pointer to driver internal initialization routine 00116 // 00117 void (* pfInit) (GUI_DEVICE * pDevice); 00118 void (* pfCheck)(GUI_DEVICE * pDevice); 00119 // 00120 // API-Tables 00121 // 00122 MANAGE_VMEM_API ManageVMEM_API; // Memory management 00123 GUI_PORT_API HW_API; // Hardware routines 00124 }; 00125 00126 /********************************************************************* 00127 * 00128 * LOG2PHYS_xxx 00129 */ 00130 #define LOG2PHYS_X ( x ) 00131 #define LOG2PHYS_X_OX (pContext->xSize - x - 1) 00132 #define LOG2PHYS_X_OY ( x ) 00133 #define LOG2PHYS_X_OXY (pContext->xSize - x - 1) 00134 #define LOG2PHYS_X_OS ( y ) 00135 #define LOG2PHYS_X_OSX (pContext->ySize - y - 1) 00136 #define LOG2PHYS_X_OSY ( y ) 00137 #define LOG2PHYS_X_OSXY (pContext->ySize - y - 1) 00138 00139 #define LOG2PHYS_Y ( y ) 00140 #define LOG2PHYS_Y_OX ( y ) 00141 #define LOG2PHYS_Y_OY (pContext->ySize - y - 1) 00142 #define LOG2PHYS_Y_OXY (pContext->ySize - y - 1) 00143 #define LOG2PHYS_Y_OS ( x ) 00144 #define LOG2PHYS_Y_OSX ( x ) 00145 #define LOG2PHYS_Y_OSY (pContext->xSize - x - 1) 00146 #define LOG2PHYS_Y_OSXY (pContext->xSize - x - 1) 00147 00148 /********************************************************************* 00149 * 00150 * _SetPixelIndex_##EXT 00151 */ 00152 #define DEFINE_SETPIXELINDEX(EXT, X_PHYS, Y_PHYS) \ 00153 static void _SetPixelIndex_##EXT(GUI_DEVICE * pDevice, int x, int y, LCD_PIXELINDEX PixelIndex) { \ 00154 DRIVER_CONTEXT * pContext; \ 00155 \ 00156 pContext = (DRIVER_CONTEXT *)pDevice->u.pContext; \ 00157 _SetPixelIndex(pContext, X_PHYS, Y_PHYS, PixelIndex); \ 00158 } 00159 00160 /********************************************************************* 00161 * 00162 * _GetPixelIndex_##EXT 00163 */ 00164 #define DEFINE_GETPIXELINDEX(EXT, X_PHYS, Y_PHYS) \ 00165 static LCD_PIXELINDEX _GetPixelIndex_##EXT(GUI_DEVICE * pDevice, int x, int y) { \ 00166 DRIVER_CONTEXT * pContext; \ 00167 LCD_PIXELINDEX PixelIndex; \ 00168 \ 00169 pContext = (DRIVER_CONTEXT *)pDevice->u.pContext; \ 00170 PixelIndex = _GetPixelIndex(pContext, X_PHYS, Y_PHYS); \ 00171 return PixelIndex; \ 00172 } 00173 00174 /********************************************************************* 00175 * 00176 * _GetDevProp_##EXT 00177 */ 00178 #define DEFINE_GETDEVPROP(EXT, MX, MY, SWAP) \ 00179 static I32 _GetDevProp_##EXT(GUI_DEVICE * pDevice, int Index) { \ 00180 switch (Index) { \ 00181 case LCD_DEVCAP_MIRROR_X: return MX; \ 00182 case LCD_DEVCAP_MIRROR_Y: return MY; \ 00183 case LCD_DEVCAP_SWAP_XY: return SWAP; \ 00184 } \ 00185 return _GetDevProp(pDevice, Index); \ 00186 } 00187 00188 /********************************************************************* 00189 * 00190 * DEFINE_FUNCTIONS 00191 */ 00192 #define DEFINE_FUNCTIONS(EXT, X_PHYS, Y_PHYS, MX, MY, SWAP) \ 00193 DEFINE_SETPIXELINDEX(EXT, X_PHYS, Y_PHYS) \ 00194 DEFINE_GETPIXELINDEX(EXT, X_PHYS, Y_PHYS) \ 00195 DEFINE_GETDEVPROP(EXT, MX, MY, SWAP) \ 00196 DEFINE_GUI_DEVICE_API(EXT) 00197 00198 /********************************************************************* 00199 * 00200 * Private functions 00201 * 00202 ********************************************************************** 00203 */ 00204 void (*GUIDRV__IST3088_GetDevFunc(GUI_DEVICE ** ppDevice, int Index))(void); 00205 void GUIDRV__IST3088_SetOrg (GUI_DEVICE * pDevice, int x, int y); 00206 I32 GUIDRV__IST3088_GetDevProp(GUI_DEVICE * pDevice, int Index); 00207 void GUIDRV__IST3088_GetRect (GUI_DEVICE * pDevice, LCD_RECT * pRect); 00208 00209 #if defined(__cplusplus) 00210 } 00211 #endif 00212 00213 #endif 00214 00215 /*************************** End of file ****************************/
Generated on Tue Jul 12 2022 19:43:36 by
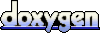