
RT1050 GUI demo using emWin library
Embed:
(wiki syntax)
Show/hide line numbers
GUIDRV_FlexColor_Private.h
00001 /********************************************************************* 00002 * SEGGER Microcontroller GmbH & Co. KG * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2016 SEGGER Microcontroller GmbH & Co. KG * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.38 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed to NXP Semiconductors USA, Inc. whose 00019 registered office is situated at 411 E. Plumeria Drive, San Jose, 00020 CA 95134, USA solely for the purposes of creating libraries for 00021 NXPs M0, M3/M4 and ARM7/9 processor-based devices, sublicensed and 00022 distributed under the terms and conditions of the NXP End User License 00023 Agreement. 00024 Full source code is available at: www.segger.com 00025 00026 We appreciate your understanding and fairness. 00027 ---------------------------------------------------------------------- 00028 Licensing information 00029 00030 Licensor: SEGGER Microcontroller Systems LLC 00031 Licensed to: NXP Semiconductors, 1109 McKay Dr, M/S 76, San Jose, CA 95131, USA 00032 Licensed SEGGER software: emWin 00033 License number: GUI-00186 00034 License model: emWin License Agreement, dated August 20th 2011 00035 Licensed product: - 00036 Licensed platform: NXP's ARM 7/9, Cortex-M0,M3,M4 00037 Licensed number of seats: - 00038 ---------------------------------------------------------------------- 00039 File : GUIDRV_FlexColor_Private.h 00040 Purpose : Private declarations for GUIDRV_FlexColor driver 00041 ---------------------------END-OF-HEADER------------------------------ 00042 */ 00043 00044 #include "GUIDRV_FlexColor.h" 00045 00046 #ifndef GUIDRV_FLEXCOLOR_PRIVATE_H 00047 #define GUIDRV_FLEXCOLOR_PRIVATE_H 00048 00049 #if defined(__cplusplus) 00050 extern "C" { /* Make sure we have C-declarations in C++ programs */ 00051 #endif 00052 00053 /********************************************************************* 00054 * 00055 * Defines 00056 * 00057 ********************************************************************** 00058 */ 00059 #define LCD_WRITE_BUFFER_SIZE 500 00060 00061 #define FLEXCOLOR_CF_MANAGE_ORIENTATION (1 << 0) 00062 #define FLEXCOLOR_CF_RAM_ADDR_SET (1 << 1) 00063 #define FLEXCOLOR_CF_SET_CURSOR (1 << 2) 00064 00065 #define FLEXCOLOR_CF_DEFAULT FLEXCOLOR_CF_RAM_ADDR_SET 00066 00067 #define FLEXCOLOR_MAX_NUM_DUMMY_READS 5 00068 #define FLEXCOLOR_NUM_DUMMY_READS 1 00069 00070 #define PUSH_RECT 0 00071 #define POP_RECT 1 00072 00073 // 00074 // Use unique context identified 00075 // 00076 #define DRIVER_CONTEXT DRIVER_CONTEXT_FLEXCOLOR 00077 00078 /********************************************************************* 00079 * 00080 * Types 00081 * 00082 ********************************************************************** 00083 */ 00084 00085 typedef struct DRIVER_CONTEXT DRIVER_CONTEXT; 00086 00087 /********************************************************************* 00088 * 00089 * DRIVER_CONTEXT 00090 */ 00091 struct DRIVER_CONTEXT { 00092 // 00093 // Data 00094 // 00095 int xSize, ySize; // Display size 00096 int vxSize, vySize; // Virtual display size 00097 int x0, y0, x1, y1; // Current rectangle 00098 int NumDummyReads; // Number of required dummy reads 00099 U16 RegEntryMode; // Can be used for storing additional configuration bits for 'EntryMode' register which is modified by the driver 00100 U16 Flags; 00101 LCD_PIXELINDEX IndexMask; 00102 int FirstSEG, FirstCOM; 00103 int Orientation; 00104 int BitsPerPixel; 00105 int Shift; 00106 GUI_DEVICE * pDevice; 00107 const GUI_DEVICE_API * pMemdev_API; 00108 // 00109 // Cache 00110 // 00111 void * pVRAM; 00112 int CacheLocked; 00113 int CacheStat; 00114 int xPos, yPos; 00115 U32 Addr; 00116 GUI_RECT CacheRect; 00117 // 00118 // Buffers 00119 // 00120 void * pWriteBuffer; 00121 void * pLineBuffer; 00122 U8 aPair_8 [3 + FLEXCOLOR_MAX_NUM_DUMMY_READS]; 00123 U16 aPair_16[3 + FLEXCOLOR_MAX_NUM_DUMMY_READS]; 00124 // 00125 // Functions for writing single items (data, cmd) regardless of the interface and getting the status 00126 // 00127 U16 (* pfReadReg) (DRIVER_CONTEXT * _pContext); 00128 void (* pfSetReg) (DRIVER_CONTEXT * _pContext, U16 _Data); 00129 void (* pfWritePara) (DRIVER_CONTEXT * _pContext, U16 _Data); 00130 void (* pfSetInterface)(DRIVER_CONTEXT * _pContext, int _BusWidth); 00131 // 00132 // Setting read mode, required for RA8870 00133 // 00134 void (* pfSetReadMode)(DRIVER_CONTEXT * _pContext, int OnOff, int SetCursor); 00135 // 00136 // Cache related function pointers 00137 // 00138 void (* pfSendCacheRect)(DRIVER_CONTEXT * _pContext, int _x0, int _y0, int _x1, int _y1); 00139 U32 (* pfReadData) (DRIVER_CONTEXT * _pContext); 00140 void (* pfWriteData) (DRIVER_CONTEXT * _pContext, U32 _PixelIndex); 00141 // 00142 // Controller specific routines 00143 // 00144 void (* pfSetRect) (DRIVER_CONTEXT * _pContext, int _x0, int _y0, int _x1, int _y1); 00145 void (* pfSetPhysRAMAddr) (DRIVER_CONTEXT * _pContext, int _x, int _y); 00146 void (* pfSetOrientation) (DRIVER_CONTEXT * _pContext); 00147 U16 (* pfReadPixel_16bpp_B16)(DRIVER_CONTEXT * _pContext); 00148 U16 (* pfReadPixel_16bpp_B8) (DRIVER_CONTEXT * _pContext); 00149 U32 (* pfReadPixel_18bpp_B9) (DRIVER_CONTEXT * _pContext); 00150 U32 (* pfReadPixel_18bpp_B18)(DRIVER_CONTEXT * _pContext); 00151 U32 (* pfReadPixel_32bpp_B8) (DRIVER_CONTEXT * _pContext); 00152 void (* pfReadRect_16bpp_B16) (GUI_DEVICE * _pDevice, int _x0, int _y0, int _x1, int _y1, U16 * _pBuffer); 00153 void (* pfReadRect_16bpp_B8) (GUI_DEVICE * _pDevice, int _x0, int _y0, int _x1, int _y1, U16 * _pBuffer); 00154 void (* pfReadRect_18bpp_B9) (GUI_DEVICE * _pDevice, int _x0, int _y0, int _x1, int _y1, U32 * _pBuffer); 00155 void (* pfReadRect_18bpp_B18) (GUI_DEVICE * _pDevice, int _x0, int _y0, int _x1, int _y1, U32 * _pBuffer); 00156 void (* pfReadRect_32bpp_B8) (GUI_DEVICE * _pDevice, int _x0, int _y0, int _x1, int _y1, U32 * _pBuffer); 00157 // 00158 // Custom read functions 00159 // 00160 U16 (* pfReadPixelCust_16bpp) (int LayerIndex); 00161 U32 (* pfReadPixelCust_18bpp) (int LayerIndex); 00162 void(* pfReadMPixelCust_16bpp)(int LayerIndex, U16 * pBuffer, U32 NumPixels); 00163 void(* pfReadMPixelCust_18bpp)(int LayerIndex, U32 * pBuffer, U32 NumPixels); 00164 // 00165 // Mode dependent drawing functions 00166 // 00167 void (* pfDrawBitmap )(GUI_DEVICE * _pDevice, int _x0, int _y0, int _xsize, int _ysize, int _BitsPerPixel, int _BytesPerLine, const U8 * _pData, int _Diff, const LCD_PIXELINDEX * _pTrans); 00168 void (* pfFillRect )(GUI_DEVICE * _pDevice, int _x0, int _y0, int _x1, int _y1); 00169 LCD_PIXELINDEX(* pfGetPixelIndex)(GUI_DEVICE * _pDevice, int _x, int _y); 00170 void (* pfSetPixelIndex)(GUI_DEVICE * _pDevice, int _x, int _y, LCD_PIXELINDEX _ColorIndex); 00171 int (* pfControlCache )(GUI_DEVICE * _pDevice, int _Cmd); 00172 void (* pfRefresh )(GUI_DEVICE * _pDevice); 00173 // 00174 // Controller dependent function pointers 00175 // 00176 void (* pfReadRect)(void); 00177 // 00178 // Orientation 00179 // 00180 int (* pfLog2PhysX)(DRIVER_CONTEXT * _pContext, int _x, int _y); 00181 int (* pfLog2PhysY)(DRIVER_CONTEXT * _pContext, int _x, int _y); 00182 // 00183 // Function pointer for setting up pfLog2Phys<X,Y> 00184 // 00185 void (* pfSetLog2Phys)(DRIVER_CONTEXT * _pContext); 00186 // 00187 // Hardware routines 00188 // 00189 GUI_PORT_API HW_API; 00190 }; 00191 00192 /********************************************************************* 00193 * 00194 * Private functions 00195 * 00196 ********************************************************************** 00197 */ 00198 void GUIDRV_FlexColor__InitOnce (GUI_DEVICE * pDevice); 00199 int GUIDRV_FlexColor__ControlCache (GUI_DEVICE * pDevice, int Cmd); 00200 void GUIDRV_FlexColor__Refresh (GUI_DEVICE * pDevice); 00201 00202 void GUIDRV_FlexColor__AddCacheRect (DRIVER_CONTEXT * pContext); 00203 void GUIDRV_FlexColor__ManageRect (DRIVER_CONTEXT * pContext, int Cmd); 00204 void GUIDRV_FlexColor__SetCacheAddr (DRIVER_CONTEXT * pContext, int x, int y); 00205 void GUIDRV_FlexColor__SetCacheRect (DRIVER_CONTEXT * pContext, int x0, int y0, int x1, int y1); 00206 void GUIDRV_FlexColor__SetLog2Phys (DRIVER_CONTEXT * pContext); 00207 void GUIDRV_FlexColor__SetSubRect (DRIVER_CONTEXT * pContext, int x0, int y0, int x1, int y1); 00208 void GUIDRV_FlexColor__ClearCacheRect (DRIVER_CONTEXT * pContext); 00209 00210 LCD_PIXELINDEX GUIDRV_FlexColor__GetPixelIndexCache (GUI_DEVICE * pDevice, int x, int y); 00211 void GUIDRV_FlexColor__SetPixelIndexCache (GUI_DEVICE * pDevice, int x, int y, LCD_PIXELINDEX PixelIndex); 00212 LCD_PIXELINDEX GUIDRV_FlexColor__GetPixelIndexNoCache(GUI_DEVICE * pDevice, int x, int y); 00213 void GUIDRV_FlexColor__SetPixelIndexNoCache(GUI_DEVICE * pDevice, int x, int y, LCD_PIXELINDEX PixelIndex); 00214 00215 void GUIDRV_FlexColor__SetFunc66712(GUI_DEVICE * pDevice, U16 AndMask_SetAddrRAM); 00216 00217 /********************************************************************* 00218 * 00219 * Simulation (Segger internal use only) 00220 * 00221 ********************************************************************** 00222 */ 00223 #if defined(WIN32) && defined(LCD_SIMCONTROLLER) 00224 00225 extern GUI_PORT_API SIM_FlexColor_HW_API; 00226 00227 void SIM_FlexColor_Config (GUI_DEVICE * pDevice, int Orientation, int xSize, int ySize, int FirstSEG, int FirstCOM, int BitsPerPixel, int NumDummyReads); 00228 void SIM_FlexColor_SetBus8 (GUI_DEVICE * pDevice); 00229 void SIM_FlexColor_SetBus9 (GUI_DEVICE * pDevice); 00230 void SIM_FlexColor_SetBus16 (GUI_DEVICE * pDevice); 00231 void SIM_FlexColor_SetBus32 (GUI_DEVICE * pDevice, int Shift); 00232 void SIM_FlexColor_SetBus8_24 (GUI_DEVICE * pDevice); 00233 void SIM_FlexColor_SetFunc66702(GUI_DEVICE * pDevice); 00234 void SIM_FlexColor_SetFunc66708(GUI_DEVICE * pDevice); 00235 void SIM_FlexColor_SetFunc66709(GUI_DEVICE * pDevice); 00236 void SIM_FlexColor_SetFunc66712(GUI_DEVICE * pDevice); 00237 void SIM_FlexColor_SetFunc66714(GUI_DEVICE * pDevice); 00238 void SIM_FlexColor_SetFunc66715(GUI_DEVICE * pDevice); 00239 void SIM_FlexColor_SetFunc66718(GUI_DEVICE * pDevice); 00240 void SIM_FlexColor_SetFunc66719(GUI_DEVICE * pDevice); 00241 void SIM_FlexColor_SetFunc66720(GUI_DEVICE * pDevice); 00242 void SIM_FlexColor_SetFunc66721(GUI_DEVICE * pDevice); 00243 void SIM_FlexColor_SetFunc66722(GUI_DEVICE * pDevice); 00244 void SIM_FlexColor_SetFunc66723(GUI_DEVICE * pDevice); 00245 void SIM_FlexColor_SetFunc66772(GUI_DEVICE * pDevice); 00246 00247 #endif 00248 00249 #if defined(__cplusplus) 00250 } 00251 #endif 00252 00253 #endif /* GUIDRV_FLEXCOLOR_PRIVATE_H */ 00254 00255 /*************************** End of file ****************************/
Generated on Tue Jul 12 2022 19:43:36 by
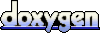