
RT1050 GUI demo using emWin library
Embed:
(wiki syntax)
Show/hide line numbers
GRAPH.h
00001 /********************************************************************* 00002 * SEGGER Microcontroller GmbH & Co. KG * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2016 SEGGER Microcontroller GmbH & Co. KG * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.38 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed to NXP Semiconductors USA, Inc. whose 00019 registered office is situated at 411 E. Plumeria Drive, San Jose, 00020 CA 95134, USA solely for the purposes of creating libraries for 00021 NXPs M0, M3/M4 and ARM7/9 processor-based devices, sublicensed and 00022 distributed under the terms and conditions of the NXP End User License 00023 Agreement. 00024 Full source code is available at: www.segger.com 00025 00026 We appreciate your understanding and fairness. 00027 ---------------------------------------------------------------------- 00028 Licensing information 00029 00030 Licensor: SEGGER Microcontroller Systems LLC 00031 Licensed to: NXP Semiconductors, 1109 McKay Dr, M/S 76, San Jose, CA 95131, USA 00032 Licensed SEGGER software: emWin 00033 License number: GUI-00186 00034 License model: emWin License Agreement, dated August 20th 2011 00035 Licensed product: - 00036 Licensed platform: NXP's ARM 7/9, Cortex-M0,M3,M4 00037 Licensed number of seats: - 00038 ---------------------------------------------------------------------- 00039 File : GRAPH.h 00040 Purpose : GRAPH include 00041 --------------------END-OF-HEADER------------------------------------- 00042 */ 00043 00044 #ifndef GRAPH_H 00045 #define GRAPH_H 00046 00047 #include "WM.h" 00048 #include "DIALOG_Intern.h" /* Req. for Create indirect data structure */ 00049 #include "WIDGET.h" 00050 00051 #if GUI_WINSUPPORT 00052 00053 #if defined(__cplusplus) 00054 extern "C" { /* Make sure we have C-declarations in C++ programs */ 00055 #endif 00056 00057 /********************************************************************* 00058 * 00059 * Defines 00060 * 00061 ********************************************************************** 00062 */ 00063 #define GRAPH_CI_BK 0 00064 #define GRAPH_CI_BORDER 1 00065 #define GRAPH_CI_FRAME 2 00066 #define GRAPH_CI_GRID 3 00067 00068 #define GRAPH_SCALE_CF_HORIZONTAL (0 << 0) 00069 #define GRAPH_SCALE_CF_VERTICAL (1 << 0) 00070 00071 #define GRAPH_SCALE_SF_HORIZONTAL GRAPH_SCALE_CF_HORIZONTAL 00072 #define GRAPH_SCALE_SF_VERTICAL GRAPH_SCALE_CF_VERTICAL 00073 00074 #define GRAPH_DRAW_FIRST 0 00075 #define GRAPH_DRAW_AFTER_BORDER 1 00076 #define GRAPH_DRAW_LAST 2 00077 00078 #define GRAPH_ALIGN_RIGHT (0 << 0) 00079 #define GRAPH_ALIGN_LEFT (1 << 0) 00080 00081 // 00082 // Creation flags (ExFlags) 00083 // 00084 #define GRAPH_CF_GRID_FIXED_X (1 << 0) 00085 #define GRAPH_CF_AVOID_SCROLLBAR_H (1 << 1) 00086 #define GRAPH_CF_AVOID_SCROLLBAR_V (1 << 2) 00087 00088 // 00089 // Status flags 00090 // 00091 #define GRAPH_SF_AVOID_SCROLLBAR_H GRAPH_CF_AVOID_SCROLLBAR_H 00092 #define GRAPH_SF_AVOID_SCROLLBAR_V GRAPH_CF_AVOID_SCROLLBAR_V 00093 00094 /********************************************************************* 00095 * 00096 * Public Types 00097 * 00098 ********************************************************************** 00099 */ 00100 typedef WM_HMEM GRAPH_Handle; 00101 typedef WM_HMEM GRAPH_DATA_Handle; 00102 typedef WM_HMEM GRAPH_SCALE_Handle; 00103 00104 /********************************************************************* 00105 * 00106 * Create functions 00107 * 00108 ********************************************************************** 00109 */ 00110 00111 GRAPH_Handle GRAPH_CreateEx (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int ExFlags, int Id); 00112 GRAPH_Handle GRAPH_CreateUser (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int ExFlags, int Id, int NumExtraBytes); 00113 GRAPH_Handle GRAPH_CreateIndirect(const GUI_WIDGET_CREATE_INFO * pCreateInfo, WM_HWIN hWinParent, int x0, int y0, WM_CALLBACK * cb); 00114 00115 GRAPH_DATA_Handle GRAPH_DATA_XY_Create(GUI_COLOR Color, unsigned MaxNumItems, const GUI_POINT * pData, unsigned NumItems); 00116 GRAPH_DATA_Handle GRAPH_DATA_YT_Create(GUI_COLOR Color, unsigned MaxNumItems, const I16 * pData, unsigned NumItems); 00117 GRAPH_SCALE_Handle GRAPH_SCALE_Create (int Pos, int TextAlign, unsigned Flags, unsigned TickDist); 00118 00119 /********************************************************************* 00120 * 00121 * The callback ... 00122 * 00123 * Do not call it directly ! It is only to be used from within an 00124 * overwritten callback. 00125 */ 00126 void GRAPH_Callback(WM_MESSAGE * pMsg); 00127 00128 /********************************************************************* 00129 * 00130 * Member functions 00131 * 00132 ********************************************************************** 00133 */ 00134 void GRAPH_AttachData (GRAPH_Handle hObj, GRAPH_DATA_Handle hData); 00135 void GRAPH_AttachScale (GRAPH_Handle hObj, GRAPH_SCALE_Handle hScale); 00136 void GRAPH_DetachData (GRAPH_Handle hObj, GRAPH_DATA_Handle hData); 00137 void GRAPH_DetachScale (GRAPH_Handle hObj, GRAPH_SCALE_Handle hScale); 00138 I32 GRAPH_GetScrollValue (GRAPH_Handle hObj, U8 Coord); 00139 int GRAPH_GetUserData (GRAPH_Handle hObj, void * pDest, int NumBytes); 00140 void GRAPH_SetAutoScrollbar (GRAPH_Handle hObj, U8 Coord, U8 OnOff); 00141 void GRAPH_SetBorder (GRAPH_Handle hObj, unsigned BorderL, unsigned BorderT, unsigned BorderR, unsigned BorderB); 00142 GUI_COLOR GRAPH_SetColor (GRAPH_Handle hObj, GUI_COLOR Color, unsigned Index); 00143 unsigned GRAPH_SetGridFixedX (GRAPH_Handle hObj, unsigned OnOff); 00144 unsigned GRAPH_SetGridOffY (GRAPH_Handle hObj, unsigned Value); 00145 unsigned GRAPH_SetGridVis (GRAPH_Handle hObj, unsigned OnOff); 00146 unsigned GRAPH_SetGridDistX (GRAPH_Handle hObj, unsigned Value); 00147 unsigned GRAPH_SetGridDistY (GRAPH_Handle hObj, unsigned Value); 00148 U8 GRAPH_SetLineStyleH (GRAPH_Handle hObj, U8 Value); 00149 U8 GRAPH_SetLineStyleV (GRAPH_Handle hObj, U8 Value); 00150 void GRAPH_SetLineStyle (GRAPH_Handle hObj, U8 Value); 00151 void GRAPH_SetScrollValue (GRAPH_Handle hObj, U8 Coord, U32 Value); 00152 unsigned GRAPH_SetVSizeX (GRAPH_Handle hObj, unsigned Value); 00153 unsigned GRAPH_SetVSizeY (GRAPH_Handle hObj, unsigned Value); 00154 int GRAPH_SetUserData (GRAPH_Handle hObj, const void * pSrc, int NumBytes); 00155 void GRAPH_SetUserDraw (GRAPH_Handle hObj, void (* pOwnerDraw)(WM_HWIN, int)); 00156 00157 void GRAPH_DATA_YT_AddValue (GRAPH_DATA_Handle hDataObj, I16 Value); 00158 void GRAPH_DATA_YT_Clear (GRAPH_DATA_Handle hDataObj); 00159 void GRAPH_DATA_YT_Delete (GRAPH_DATA_Handle hDataObj); 00160 int GRAPH_DATA_YT_GetValue (GRAPH_DATA_Handle hDataObj, I16 * pValue, U32 Index); 00161 00162 void GRAPH_DATA_YT_SetAlign (GRAPH_DATA_Handle hDataObj, int Align); 00163 void GRAPH_DATA_YT_SetOffY (GRAPH_DATA_Handle hDataObj, int Off); 00164 void GRAPH_DATA_YT_MirrorX (GRAPH_DATA_Handle hDataObj, int OnOff); 00165 00166 void GRAPH_DATA_XY_AddPoint (GRAPH_DATA_Handle hDataObj, GUI_POINT * pPoint); 00167 void GRAPH_DATA_XY_Clear (GRAPH_DATA_Handle hDataObj); 00168 void GRAPH_DATA_XY_Delete (GRAPH_DATA_Handle hDataObj); 00169 unsigned GRAPH_DATA_XY_GetLineVis (GRAPH_DATA_Handle hDataObj); 00170 int GRAPH_DATA_XY_GetPoint (GRAPH_DATA_Handle hDataObj, GUI_POINT * pPoint, U32 Index); 00171 unsigned GRAPH_DATA_XY_GetPointVis (GRAPH_DATA_Handle hDataObj); 00172 void GRAPH_DATA_XY_SetLineStyle (GRAPH_DATA_Handle hDataObj, U8 LineStyle); 00173 unsigned GRAPH_DATA_XY_SetLineVis (GRAPH_DATA_Handle hDataObj, unsigned OnOff); 00174 void GRAPH_DATA_XY_SetOffX (GRAPH_DATA_Handle hDataObj, int Off); 00175 void GRAPH_DATA_XY_SetOffY (GRAPH_DATA_Handle hDataObj, int Off); 00176 void GRAPH_DATA_XY_SetPenSize (GRAPH_DATA_Handle hDataObj, U8 PenSize); 00177 void GRAPH_DATA_XY_SetPointSize (GRAPH_DATA_Handle hDataObj, unsigned PointSize); 00178 unsigned GRAPH_DATA_XY_SetPointVis (GRAPH_DATA_Handle hDataObj, unsigned OnOff); 00179 void GRAPH_DATA_XY_SetOwnerDraw (GRAPH_DATA_Handle hDataObj, WIDGET_DRAW_ITEM_FUNC * pOwnerDraw); 00180 00181 void GRAPH_SCALE_Delete (GRAPH_SCALE_Handle hScaleObj); 00182 float GRAPH_SCALE_SetFactor (GRAPH_SCALE_Handle hScaleObj, float Factor); 00183 const GUI_FONT * GRAPH_SCALE_SetFont (GRAPH_SCALE_Handle hScaleObj, const GUI_FONT * pFont); 00184 int GRAPH_SCALE_SetNumDecs (GRAPH_SCALE_Handle hScaleObj, int NumDecs); 00185 int GRAPH_SCALE_SetOff (GRAPH_SCALE_Handle hScaleObj, int Off); 00186 int GRAPH_SCALE_SetPos (GRAPH_SCALE_Handle hScaleObj, int Pos); 00187 GUI_COLOR GRAPH_SCALE_SetTextColor(GRAPH_SCALE_Handle hScaleObj, GUI_COLOR Color); 00188 unsigned GRAPH_SCALE_SetTickDist (GRAPH_SCALE_Handle hScaleObj, unsigned Value); 00189 00190 #if defined(__cplusplus) 00191 } 00192 #endif 00193 00194 #endif // GUI_WINSUPPORT 00195 #endif // GRAPH_H 00196 00197 /*************************** End of file ****************************/
Generated on Tue Jul 12 2022 19:43:35 by
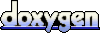