
RT1050 GUI demo using emWin library
Embed:
(wiki syntax)
Show/hide line numbers
EDIT.h
00001 /********************************************************************* 00002 * SEGGER Microcontroller GmbH & Co. KG * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2016 SEGGER Microcontroller GmbH & Co. KG * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.38 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed to NXP Semiconductors USA, Inc. whose 00019 registered office is situated at 411 E. Plumeria Drive, San Jose, 00020 CA 95134, USA solely for the purposes of creating libraries for 00021 NXPs M0, M3/M4 and ARM7/9 processor-based devices, sublicensed and 00022 distributed under the terms and conditions of the NXP End User License 00023 Agreement. 00024 Full source code is available at: www.segger.com 00025 00026 We appreciate your understanding and fairness. 00027 ---------------------------------------------------------------------- 00028 Licensing information 00029 00030 Licensor: SEGGER Microcontroller Systems LLC 00031 Licensed to: NXP Semiconductors, 1109 McKay Dr, M/S 76, San Jose, CA 95131, USA 00032 Licensed SEGGER software: emWin 00033 License number: GUI-00186 00034 License model: emWin License Agreement, dated August 20th 2011 00035 Licensed product: - 00036 Licensed platform: NXP's ARM 7/9, Cortex-M0,M3,M4 00037 Licensed number of seats: - 00038 ---------------------------------------------------------------------- 00039 File : EDIT.h 00040 Purpose : EDIT include 00041 --------------------END-OF-HEADER------------------------------------- 00042 */ 00043 00044 #ifndef EDIT_H 00045 #define EDIT_H 00046 00047 #include "WM.h" 00048 #include "DIALOG_Intern.h" // Required for Create indirect data structure 00049 00050 #if GUI_WINSUPPORT 00051 00052 #if defined(__cplusplus) 00053 extern "C" { // Make sure we have C-declarations in C++ programs 00054 #endif 00055 00056 /********************************************************************* 00057 * 00058 * Defaults for configuration switches 00059 * 00060 * The following are defaults for config switches which affect the 00061 * interface specified in this module 00062 * 00063 ********************************************************************** 00064 */ 00065 /********************************************************************* 00066 * 00067 * Defines 00068 * 00069 ********************************************************************** 00070 */ 00071 // 00072 // Create / Status flags 00073 // 00074 #define EDIT_CF_LEFT GUI_TA_LEFT 00075 #define EDIT_CF_RIGHT GUI_TA_RIGHT 00076 #define EDIT_CF_HCENTER GUI_TA_HCENTER 00077 #define EDIT_CF_VCENTER GUI_TA_VCENTER 00078 #define EDIT_CF_TOP GUI_TA_TOP 00079 #define EDIT_CF_BOTTOM GUI_TA_BOTTOM 00080 00081 // 00082 // Color indices 00083 // 00084 #define EDIT_CI_DISABLED 0 00085 #define EDIT_CI_ENABLED 1 00086 #define EDIT_CI_CURSOR 2 00087 00088 // 00089 // Signed or normal mode 00090 // 00091 #define GUI_EDIT_NORMAL (0 << 0) 00092 #define GUI_EDIT_SIGNED (1 << 0) 00093 #define GUI_EDIT_SUPPRESS_LEADING_ZEROES (1 << 1) 00094 00095 // 00096 // Cursor coloring 00097 // 00098 #define GUI_EDIT_SHOWCURSOR (1 << 2) 00099 #define GUI_EDIT_CUSTCOLORMODE (1 << 3) 00100 00101 // 00102 // Edit modes 00103 // 00104 #define GUI_EDIT_MODE_INSERT 0 00105 #define GUI_EDIT_MODE_OVERWRITE 1 00106 00107 // 00108 // Compatibility macros 00109 // 00110 #define EDIT_CI_DISABELD EDIT_CI_DISABLED 00111 #define EDIT_CI_ENABELD EDIT_CI_ENABLED 00112 00113 /********************************************************************* 00114 * 00115 * Types 00116 * 00117 ********************************************************************** 00118 */ 00119 typedef WM_HMEM EDIT_Handle; 00120 typedef void tEDIT_AddKeyEx (EDIT_Handle hObj, int Key); 00121 typedef void tEDIT_UpdateBuffer(EDIT_Handle hObj); 00122 00123 /********************************************************************* 00124 * 00125 * Create functions 00126 */ 00127 EDIT_Handle EDIT_Create (int x0, int y0, int xSize, int ySize, int Id, int MaxLen, int Flags); 00128 EDIT_Handle EDIT_CreateAsChild (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int Id, int Flags, int MaxLen); 00129 EDIT_Handle EDIT_CreateEx (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int ExFlags, int Id, int MaxLen); 00130 EDIT_Handle EDIT_CreateUser (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int ExFlags, int Id, int MaxLen, int NumExtraBytes); 00131 EDIT_Handle EDIT_CreateIndirect(const GUI_WIDGET_CREATE_INFO * pCreateInfo, WM_HWIN hWinParent, int x0, int y0, WM_CALLBACK * cb); 00132 00133 /********************************************************************* 00134 * 00135 * The callback ... 00136 * 00137 * Do not call it directly ! It is only to be used from within an 00138 * overwritten callback. 00139 */ 00140 void EDIT_Callback(WM_MESSAGE * pMsg); 00141 00142 /********************************************************************* 00143 * 00144 * Managing default values 00145 * 00146 ********************************************************************** 00147 */ 00148 void EDIT_SetDefaultBkColor (unsigned int Index, GUI_COLOR Color); 00149 void EDIT_SetDefaultFont (const GUI_FONT * pFont); 00150 void EDIT_SetDefaultTextAlign(int Align); 00151 void EDIT_SetDefaultTextColor(unsigned int Index, GUI_COLOR Color); 00152 00153 /********************************************************************* 00154 * 00155 * Individual member functions 00156 */ 00157 // 00158 // Query preferences 00159 // 00160 GUI_COLOR EDIT_GetDefaultBkColor(unsigned int Index); 00161 const GUI_FONT * EDIT_GetDefaultFont(void); 00162 int EDIT_GetDefaultTextAlign(void); 00163 GUI_COLOR EDIT_GetDefaultTextColor(unsigned int Index); 00164 // 00165 // Methods changing properties 00166 // 00167 void EDIT_AddKey (EDIT_Handle hObj, int Key); 00168 void EDIT_EnableBlink (EDIT_Handle hObj, int Period, int OnOff); 00169 GUI_COLOR EDIT_GetBkColor (EDIT_Handle hObj, unsigned int Index); 00170 void EDIT_SetBkColor (EDIT_Handle hObj, unsigned int Index, GUI_COLOR color); 00171 void EDIT_SetCursorAtChar (EDIT_Handle hObj, int Pos); 00172 void EDIT_SetCursorAtPixel (EDIT_Handle hObj, int xPos); 00173 void EDIT_SetFocussable (EDIT_Handle hObj, int State); 00174 void EDIT_SetFont (EDIT_Handle hObj, const GUI_FONT * pFont); 00175 int EDIT_SetInsertMode (EDIT_Handle hObj, int OnOff); 00176 void EDIT_SetMaxLen (EDIT_Handle hObj, int MaxLen); 00177 void EDIT_SetpfAddKeyEx (EDIT_Handle hObj, tEDIT_AddKeyEx * pfAddKeyEx); 00178 void EDIT_SetpfUpdateBuffer(EDIT_Handle hObj, tEDIT_UpdateBuffer * pfUpdateBuffer); 00179 void EDIT_SetText (EDIT_Handle hObj, const char * s); 00180 void EDIT_SetTextAlign (EDIT_Handle hObj, int Align); 00181 GUI_COLOR EDIT_GetTextColor(EDIT_Handle hObj, unsigned int Index); 00182 void EDIT_SetTextColor (EDIT_Handle hObj, unsigned int Index, GUI_COLOR Color); 00183 void EDIT_SetSel (EDIT_Handle hObj, int FirstChar, int LastChar); 00184 int EDIT_SetUserData (EDIT_Handle hObj, const void * pSrc, int NumBytes); 00185 int EDIT_EnableInversion (EDIT_Handle hObj, int OnOff); 00186 // 00187 // Get/Set user input 00188 // 00189 int EDIT_GetCursorCharPos (EDIT_Handle hObj); 00190 void EDIT_GetCursorPixelPos (EDIT_Handle hObj, int * pxPos, int * pyPos); 00191 float EDIT_GetFloatValue (EDIT_Handle hObj); 00192 const GUI_FONT * EDIT_GetFont(EDIT_Handle hObj); 00193 int EDIT_GetNumChars (EDIT_Handle hObj); 00194 void EDIT_GetText (EDIT_Handle hObj, char * sDest, int MaxLen); 00195 I32 EDIT_GetValue (EDIT_Handle hObj); 00196 void EDIT_SetFloatValue (EDIT_Handle hObj, float Value); 00197 int EDIT_GetUserData (EDIT_Handle hObj, void * pDest, int NumBytes); 00198 void EDIT_SetValue (EDIT_Handle hObj, I32 Value); 00199 00200 /********************************************************************* 00201 * 00202 * Routines for editing values 00203 * 00204 ********************************************************************** 00205 */ 00206 void EDIT_SetHexMode (EDIT_Handle hEdit, U32 Value, U32 Min, U32 Max); 00207 void EDIT_SetBinMode (EDIT_Handle hEdit, U32 Value, U32 Min, U32 Max); 00208 void EDIT_SetDecMode (EDIT_Handle hEdit, I32 Value, I32 Min, I32 Max, int Shift, U8 Flags); 00209 void EDIT_SetFloatMode(EDIT_Handle hEdit, float Value, float Min, float Max, int Shift, U8 Flags); 00210 void EDIT_SetTextMode (EDIT_Handle hEdit); 00211 void EDIT_SetUlongMode(EDIT_Handle hEdit, U32 Value, U32 Min, U32 Max); 00212 00213 U32 GUI_EditHex (U32 Value, U32 Min, U32 Max, int Len, int xSize); 00214 U32 GUI_EditBin (U32 Value, U32 Min, U32 Max, int Len, int xSize); 00215 I32 GUI_EditDec (I32 Value, I32 Min, I32 Max, int Len, int xSize, int Shift, U8 Flags); 00216 float GUI_EditFloat (float Value, float Min, float Max, int Len, int xSize, int Shift, U8 Flags); 00217 void GUI_EditString (char * pString, int Len, int xSize); 00218 00219 #if defined(__cplusplus) 00220 } 00221 #endif 00222 00223 #endif // GUI_WINSUPPORT 00224 #endif // EDIT_H 00225 00226 /*************************** End of file ****************************/
Generated on Tue Jul 12 2022 19:43:35 by
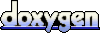