
RT1050 GUI demo using emWin library
Embed:
(wiki syntax)
Show/hide line numbers
BUTTON.h
00001 /********************************************************************* 00002 * SEGGER Microcontroller GmbH & Co. KG * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2016 SEGGER Microcontroller GmbH & Co. KG * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.38 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed to NXP Semiconductors USA, Inc. whose 00019 registered office is situated at 411 E. Plumeria Drive, San Jose, 00020 CA 95134, USA solely for the purposes of creating libraries for 00021 NXPs M0, M3/M4 and ARM7/9 processor-based devices, sublicensed and 00022 distributed under the terms and conditions of the NXP End User License 00023 Agreement. 00024 Full source code is available at: www.segger.com 00025 00026 We appreciate your understanding and fairness. 00027 ---------------------------------------------------------------------- 00028 Licensing information 00029 00030 Licensor: SEGGER Microcontroller Systems LLC 00031 Licensed to: NXP Semiconductors, 1109 McKay Dr, M/S 76, San Jose, CA 95131, USA 00032 Licensed SEGGER software: emWin 00033 License number: GUI-00186 00034 License model: emWin License Agreement, dated August 20th 2011 00035 Licensed product: - 00036 Licensed platform: NXP's ARM 7/9, Cortex-M0,M3,M4 00037 Licensed number of seats: - 00038 ---------------------------------------------------------------------- 00039 File : BUTTON.h 00040 Purpose : BUTTON public header file (API) 00041 --------------------END-OF-HEADER------------------------------------- 00042 */ 00043 00044 #ifndef BUTTON_H 00045 #define BUTTON_H 00046 00047 #include "WM.h" 00048 #include "DIALOG_Intern.h" /* Req. for Create indirect data structure */ 00049 #include "WIDGET.h" 00050 00051 #if GUI_WINSUPPORT 00052 00053 #if defined(__cplusplus) 00054 extern "C" { /* Make sure we have C-declarations in C++ programs */ 00055 #endif 00056 00057 /********************************************************************* 00058 * 00059 * Create flags 00060 */ 00061 /* For compatibility only ! */ 00062 #define BUTTON_CF_HIDE WM_CF_HIDE 00063 #define BUTTON_CF_SHOW WM_CF_SHOW 00064 #define BUTTON_CF_MEMDEV WM_CF_MEMDEV 00065 00066 /********************************************************************* 00067 * 00068 * Color indices 00069 */ 00070 #define BUTTON_CI_UNPRESSED 0 00071 #define BUTTON_CI_PRESSED 1 00072 #define BUTTON_CI_DISABLED 2 00073 00074 /********************************************************************* 00075 * 00076 * Bitmap indices 00077 */ 00078 #define BUTTON_BI_UNPRESSED 0 00079 #define BUTTON_BI_PRESSED 1 00080 #define BUTTON_BI_DISABLED 2 00081 00082 /********************************************************************* 00083 * 00084 * States 00085 */ 00086 #define BUTTON_STATE_FOCUS WIDGET_STATE_FOCUS 00087 #define BUTTON_STATE_PRESSED WIDGET_STATE_USER0 00088 00089 /********************************************************************* 00090 * 00091 * Skinning property indices 00092 */ 00093 #define BUTTON_SKINFLEX_PI_PRESSED 0 00094 #define BUTTON_SKINFLEX_PI_FOCUSSED 1 00095 #define BUTTON_SKINFLEX_PI_ENABLED 2 00096 #define BUTTON_SKINFLEX_PI_DISABLED 3 00097 00098 /********************************************************************* 00099 * 00100 * Types 00101 * 00102 ********************************************************************** 00103 */ 00104 typedef WM_HMEM BUTTON_Handle; 00105 00106 typedef struct { 00107 GUI_COLOR aColorFrame[3]; 00108 GUI_COLOR aColorUpper[2]; 00109 GUI_COLOR aColorLower[2]; 00110 int Radius; 00111 } BUTTON_SKINFLEX_PROPS; 00112 00113 /********************************************************************* 00114 * 00115 * Create function(s) 00116 00117 Note: the parameters to a create function may vary. 00118 Some widgets may have multiple create functions 00119 */ 00120 00121 BUTTON_Handle BUTTON_Create (int x0, int y0, int xSize, int ySize, int ID, int Flags); 00122 BUTTON_Handle BUTTON_CreateAsChild (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int Id, int Flags); 00123 BUTTON_Handle BUTTON_CreateEx (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int ExFlags, int Id); 00124 BUTTON_Handle BUTTON_CreateUser (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int ExFlags, int Id, int NumExtraBytes); 00125 BUTTON_Handle BUTTON_CreateIndirect(const GUI_WIDGET_CREATE_INFO * pCreateInfo, WM_HWIN hWinParent, int x0, int y0, WM_CALLBACK * cb); 00126 00127 /********************************************************************* 00128 * 00129 * Managing default values 00130 * 00131 ********************************************************************** 00132 */ 00133 GUI_COLOR BUTTON_GetDefaultBkColor (unsigned Index); 00134 const GUI_FONT * BUTTON_GetDefaultFont (void); 00135 int BUTTON_GetDefaultTextAlign (void); 00136 GUI_COLOR BUTTON_GetDefaultTextColor (unsigned Index); 00137 void BUTTON_SetDefaultBkColor (GUI_COLOR Color, unsigned Index); 00138 GUI_COLOR BUTTON_SetDefaultFocusColor(GUI_COLOR Color); 00139 void BUTTON_SetDefaultFont (const GUI_FONT * pFont); 00140 void BUTTON_SetDefaultTextAlign (int Align); 00141 void BUTTON_SetDefaultTextColor (GUI_COLOR Color, unsigned Index); 00142 00143 /********************************************************************* 00144 * 00145 * The callback ... 00146 * 00147 * Do not call it directly ! It is only to be used from within an 00148 * overwritten callback. 00149 */ 00150 void BUTTON_Callback(WM_MESSAGE *pMsg); 00151 00152 /********************************************************************* 00153 * 00154 * Member functions 00155 * 00156 ********************************************************************** 00157 */ 00158 GUI_COLOR BUTTON_GetBkColor (BUTTON_Handle hObj, unsigned int Index); 00159 const GUI_BITMAP * BUTTON_GetBitmap(BUTTON_Handle hObj,unsigned int Index); 00160 const GUI_FONT * BUTTON_GetFont (BUTTON_Handle hObj); 00161 GUI_COLOR BUTTON_GetFrameColor (BUTTON_Handle hObj); 00162 WIDGET * BUTTON_GetpWidget (BUTTON_Handle hObj); 00163 void BUTTON_GetText (BUTTON_Handle hObj, char * pBuffer, int MaxLen); 00164 GUI_COLOR BUTTON_GetTextColor (BUTTON_Handle hObj, unsigned int Index); 00165 int BUTTON_GetTextAlign (BUTTON_Handle hObj); 00166 int BUTTON_GetUserData (BUTTON_Handle hObj, void * pDest, int NumBytes); 00167 unsigned BUTTON_IsPressed (BUTTON_Handle hObj); 00168 void BUTTON_SetBitmap (BUTTON_Handle hObj, unsigned int Index, const GUI_BITMAP * pBitmap); 00169 void BUTTON_SetBitmapEx (BUTTON_Handle hObj, unsigned int Index, const GUI_BITMAP * pBitmap, int x, int y); 00170 void BUTTON_SetBkColor (BUTTON_Handle hObj, unsigned int Index, GUI_COLOR Color); 00171 void BUTTON_SetBMP (BUTTON_Handle hObj, unsigned int Index, const void * pBitmap); 00172 void BUTTON_SetBMPEx (BUTTON_Handle hObj, unsigned int Index, const void * pBitmap, int x, int y); 00173 void BUTTON_SetFont (BUTTON_Handle hObj, const GUI_FONT * pfont); 00174 void BUTTON_SetFrameColor (BUTTON_Handle hObj, GUI_COLOR Color); 00175 void BUTTON_SetState (BUTTON_Handle hObj, int State); /* Not to be doc. */ 00176 void BUTTON_SetPressed (BUTTON_Handle hObj, int State); 00177 GUI_COLOR BUTTON_SetFocusColor (BUTTON_Handle hObj, GUI_COLOR Color); 00178 void BUTTON_SetFocussable (BUTTON_Handle hObj, int State); 00179 void BUTTON_SetStreamedBitmap (BUTTON_Handle hObj, unsigned int Index, const GUI_BITMAP_STREAM * pBitmap); 00180 void BUTTON_SetStreamedBitmapEx(BUTTON_Handle hObj, unsigned int Index, const GUI_BITMAP_STREAM * pBitmap, int x, int y); 00181 int BUTTON_SetText (BUTTON_Handle hObj, const char* s); 00182 void BUTTON_SetTextAlign (BUTTON_Handle hObj, int Align); 00183 void BUTTON_SetTextColor (BUTTON_Handle hObj, unsigned int Index, GUI_COLOR Color); 00184 void BUTTON_SetTextOffset (BUTTON_Handle hObj, int xPos, int yPos); 00185 void BUTTON_SetSelfDrawEx (BUTTON_Handle hObj, unsigned int Index, GUI_DRAW_SELF_CB * pDraw, int x, int y); /* Not to be doc. */ 00186 void BUTTON_SetSelfDraw (BUTTON_Handle hObj, unsigned int Index, GUI_DRAW_SELF_CB * pDraw); /* Not to be doc. */ 00187 void BUTTON_SetReactOnLevel (void); 00188 void BUTTON_SetReactOnTouch (void); 00189 int BUTTON_SetUserData (BUTTON_Handle hObj, const void * pSrc, int NumBytes); 00190 00191 /********************************************************************* 00192 * 00193 * Member functions: Skinning 00194 * 00195 ********************************************************************** 00196 */ 00197 void BUTTON_GetSkinFlexProps (BUTTON_SKINFLEX_PROPS * pProps, int Index); 00198 void BUTTON_SetSkinClassic (BUTTON_Handle hObj); 00199 void BUTTON_SetSkin (BUTTON_Handle hObj, WIDGET_DRAW_ITEM_FUNC * pfDrawSkin); 00200 int BUTTON_DrawSkinFlex (const WIDGET_ITEM_DRAW_INFO * pDrawItemInfo); 00201 void BUTTON_SetSkinFlexProps (const BUTTON_SKINFLEX_PROPS * pProps, int Index); 00202 void BUTTON_SetDefaultSkinClassic(void); 00203 WIDGET_DRAW_ITEM_FUNC * BUTTON_SetDefaultSkin(WIDGET_DRAW_ITEM_FUNC * pfDrawSkin); 00204 00205 #define BUTTON_SKIN_FLEX BUTTON_DrawSkinFlex 00206 00207 #if defined(__cplusplus) 00208 } 00209 #endif 00210 00211 #endif // GUI_WINSUPPORT 00212 #endif // BUTTON_H 00213 00214 /*************************** End of file ****************************/
Generated on Tue Jul 12 2022 19:43:35 by
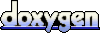