
Simple Mbed Cloud client application using features of K64 & K66 including Ethernet and SD Card
Fork of mbed-cloud-example_K64_K66 by
main.cpp
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2018 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #include "mbed.h" 00020 #include "simple-mbed-cloud-client.h" 00021 #include "FATFileSystem.h" 00022 00023 // An event queue is a very useful structure to debounce information between contexts (e.g. ISR and normal threads) 00024 // This is great because things such as network operations are illegal in ISR, so updating a resource in a button's fall() function is not allowed 00025 EventQueue eventQueue; 00026 Thread thread1; 00027 00028 // Default block device 00029 BlockDevice* bd = BlockDevice::get_default_instance(); 00030 FATFileSystem fs("sd", bd); 00031 00032 // Default network interface object 00033 NetworkInterface *net; 00034 00035 InterruptIn sw2(SW2); 00036 DigitalOut led2(LED2); 00037 00038 // Declaring pointers for access to Mbed Cloud Client resources outside of main() 00039 MbedCloudClientResource *button_res; 00040 MbedCloudClientResource *pattern_res; 00041 00042 static bool button_pressed = false; 00043 static int button_count = 0; 00044 00045 void button_press() { 00046 button_pressed = true; 00047 ++button_count; 00048 button_res->set_value(button_count); 00049 } 00050 00051 /** 00052 * PUT handler 00053 * @param resource The resource that triggered the callback 00054 * @param newValue Updated value for the resource 00055 */ 00056 void pattern_updated(MbedCloudClientResource *resource, m2m::String newValue) { 00057 printf("PUT received, new value: %s\n", newValue.c_str()); 00058 } 00059 00060 /** 00061 * POST handler 00062 * @param resource The resource that triggered the callback 00063 * @param buffer If a body was passed to the POST function, this contains the data. 00064 * Note that the buffer is deallocated after leaving this function, so copy it if you need it longer. 00065 * @param size Size of the body 00066 */ 00067 void blink_callback(MbedCloudClientResource *resource, const uint8_t *buffer, uint16_t size) { 00068 printf("POST received. Going to blink LED pattern: %s\n", pattern_res->get_value().c_str()); 00069 00070 static DigitalOut augmentedLed(LED1); // LED that is used for blinking the pattern 00071 00072 // Parse the pattern string, and toggle the LED in that pattern 00073 string s = std::string(pattern_res->get_value().c_str()); 00074 size_t i = 0; 00075 size_t pos = s.find(':'); 00076 while (pos != string::npos) { 00077 wait_ms(atoi(s.substr(i, pos - i).c_str())); 00078 augmentedLed = !augmentedLed; 00079 00080 i = ++pos; 00081 pos = s.find(':', pos); 00082 00083 if (pos == string::npos) { 00084 wait_ms(atoi(s.substr(i, s.length()).c_str())); 00085 augmentedLed = !augmentedLed; 00086 } 00087 } 00088 } 00089 00090 /** 00091 * Notification callback handler 00092 * @param resource The resource that triggered the callback 00093 * @param status The delivery status of the notification 00094 */ 00095 void button_callback(MbedCloudClientResource *resource, const NoticationDeliveryStatus status) { 00096 printf("Button notification, status %s (%d)\n", MbedCloudClientResource::delivery_status_to_string(status), status); 00097 } 00098 00099 /** 00100 * Registration callback handler 00101 * @param endpoint Information about the registered endpoint such as the name (so you can find it back in portal) 00102 */ 00103 void registered(const ConnectorClientEndpointInfo *endpoint) { 00104 printf("Connected to Mbed Cloud. Endpoint Name: %s\n", endpoint->internal_endpoint_name.c_str()); 00105 } 00106 00107 int main(void) { 00108 printf("Starting Simple Mbed Cloud Client example\n"); 00109 printf("Connecting to the network using Ethernet...\n"); 00110 00111 // Connect to the internet (DHCP is expected to be on) 00112 net = NetworkInterface::get_default_instance(); 00113 00114 nsapi_error_t status = net->connect(); 00115 00116 if (status != NSAPI_ERROR_OK) { 00117 printf("Connecting to the network failed %d!\n", status); 00118 return -1; 00119 } 00120 00121 printf("Connected to the network successfully. IP address: %s\n", net->get_ip_address()); 00122 00123 // SimpleMbedCloudClient handles registering over LwM2M to Mbed Cloud 00124 SimpleMbedCloudClient client(net, bd, &fs); 00125 int client_status = client.init(); 00126 if (client_status != 0) { 00127 printf("Initializing Mbed Cloud Client failed (%d)\n", client_status); 00128 return -1; 00129 } 00130 00131 // Creating resources, which can be written or read from the cloud 00132 button_res = client.create_resource("3200/0/5501", "button_count"); 00133 button_res->set_value(0); 00134 button_res->methods(M2MMethod::GET); 00135 button_res->observable(true); 00136 button_res->attach_notification_callback(button_callback); 00137 00138 pattern_res = client.create_resource("3201/0/5853", "blink_pattern"); 00139 pattern_res->set_value("500:500:500:500:500:500:500:500"); 00140 pattern_res->methods(M2MMethod::GET | M2MMethod::PUT); 00141 pattern_res->attach_put_callback(pattern_updated); 00142 00143 MbedCloudClientResource *blink_res = client.create_resource("3201/0/5850", "blink_action"); 00144 blink_res->methods(M2MMethod::POST); 00145 blink_res->attach_post_callback(blink_callback); 00146 00147 printf("Initialized Mbed Cloud Client. Registering...\n"); 00148 00149 // Callback that fires when registering is complete 00150 client.on_registered(®istered); 00151 00152 // Register with Mbed Cloud 00153 client.register_and_connect(); 00154 00155 // Setup the button 00156 sw2.mode(PullUp); 00157 00158 // The button fall handler is placed in the event queue so it will run in 00159 // thread context instead of ISR context, which allows safely updating the cloud resource 00160 sw2.fall(eventQueue.event(&button_press)); 00161 button_count = 0; 00162 00163 // Start the event queue in a separate thread so the main thread continues 00164 thread1.start(callback(&eventQueue, &EventQueue::dispatch_forever)); 00165 00166 while(1) 00167 { 00168 wait_ms(100); 00169 00170 if (button_pressed) { 00171 button_pressed = false; 00172 printf("button clicked %d times\r\n", button_count); 00173 } 00174 00175 } 00176 }
Generated on Thu Jul 14 2022 01:39:19 by
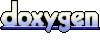