
SD card example with the file system (complete). Created for FTF2014
Dependencies: SDFileSystem mbed
Fork of FRDMK64_SDCard by
main.cpp
00001 #include "mbed.h" 00002 #include "SDFileSystem.h" 00003 00004 SDFileSystem sd(PTE3, PTE1, PTE2, PTE4, "sd"); // MOSI, MISO, SCK, CS 00005 Serial pc(USBTX, USBRX); 00006 FILE *fp; 00007 00008 int file_copy(const char *src, const char *dst) 00009 { 00010 int retval = 0; 00011 int ch; 00012 00013 FILE *fpsrc = fopen(src, "r"); // src file 00014 FILE *fpdst = fopen(dst, "w"); // dest file 00015 00016 while (1) { // Copy src to dest 00017 ch = fgetc(fpsrc); // until src EOF read. 00018 if (ch == EOF) break; 00019 fputc(ch, fpdst); 00020 } 00021 fclose(fpsrc); 00022 fclose(fpdst); 00023 00024 fpdst = fopen(dst, "r"); // Reopen dest to insure 00025 if (fpdst == NULL) { // that it was created. 00026 retval = -1; // Return error. 00027 } else { 00028 fclose(fpdst); 00029 retval = 0; // Return success. 00030 } 00031 return retval; 00032 } 00033 00034 uint32_t do_list(const char *fsrc) 00035 { 00036 DIR *d = opendir(fsrc); 00037 struct dirent *p; 00038 uint32_t counter = 0; 00039 00040 while ((p = readdir(d)) != NULL) { 00041 counter++; 00042 printf("%s\n", p->d_name); 00043 } 00044 closedir(d); 00045 return counter; 00046 } 00047 00048 // bool is_folder(const char *fdir) 00049 // { 00050 // DIR *dir = opendir(fdir); 00051 // if (dir) { 00052 // closedir(dir); 00053 // } 00054 // return (dir != NULL); 00055 00056 // } 00057 00058 // bool is_file(const char *ffile) 00059 // { 00060 // FILE *fp = fopen(ffile, "r"); 00061 // if (fp) { 00062 // fclose(fp); 00063 // } 00064 // return (fp != NULL); 00065 // } 00066 00067 void do_remove(const char *fsrc) 00068 { 00069 DIR *d = opendir(fsrc); 00070 struct dirent *p; 00071 char path[30] = {0}; 00072 while((p = readdir(d)) != NULL) { 00073 strcpy(path, fsrc); 00074 strcat(path, "/"); 00075 strcat(path, p->d_name); 00076 remove(path); 00077 } 00078 closedir(d); 00079 remove(fsrc); 00080 } 00081 00082 int main() 00083 { 00084 pc.printf("Initializing \n"); 00085 wait(2); 00086 00087 do_remove("/sd/test1"); /* clean up from the previous Lab 5 if was executed */ 00088 if (do_list("/sd") == 0) { 00089 printf("No files/directories on the sd card."); 00090 } 00091 00092 printf("\nCreating two folders. \n"); 00093 mkdir("/sd/test1", 0777); 00094 mkdir("/sd/test2", 0777); 00095 00096 fp = fopen("/sd/test1/1.txt", "w"); 00097 if (fp == NULL) { 00098 pc.printf("Unable to write the file \n"); 00099 } else { 00100 fprintf(fp, "1.txt in test 1"); 00101 fclose(fp); 00102 } 00103 00104 fp = fopen("/sd/test2/2.txt", "w"); 00105 if (fp == NULL) { 00106 pc.printf("Unable to write the file \n"); 00107 } else { 00108 fprintf(fp, "2.txt in test 2"); 00109 fclose(fp); 00110 } 00111 00112 printf("\nList all directories/files /sd.\n"); 00113 do_list("/sd"); 00114 00115 printf("\nList all files within /sd/test1.\n"); 00116 do_list("/sd/test1"); 00117 00118 00119 printf("\nList all files within /sd/test2.\n"); 00120 do_list("/sd/test2"); 00121 00122 int status = file_copy("/sd/test2/2.txt", "/sd/test1/2_copy.txt"); 00123 if (status == -1) { 00124 printf("Error, file was not copied.\n"); 00125 } 00126 printf("Removing test2 folder and 2.txt file inside."); 00127 remove("/sd/test2/2.txt"); 00128 remove("/sd/test2"); 00129 00130 printf("\nList all directories/files /sd.\n"); 00131 do_list("/sd"); 00132 00133 printf("\nList all files within /sd/test1.\n"); 00134 do_list("/sd/test1"); 00135 00136 printf("\nEnd of complete Lab 5. \n"); 00137 }
Generated on Thu Jul 21 2022 06:13:38 by
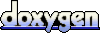