
NanoService device game controller for NSPong.
Dependencies: Beep C12832_lcd EthernetInterface MMA7660 mbed-rtos mbed nsdl_lib
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "C12832_lcd.h" 00004 #include "nsdl_support.h" 00005 #include "dbg.h" 00006 00007 // Include resources 00008 #include "accelerometer.h" 00009 #include "joystick.h" 00010 #include "buzzer.h" 00011 #include "led.h" 00012 #include "lcd.h" 00013 00014 static C12832_LCD lcd; 00015 Serial pc(USBTX, USBRX); // tx, rx 00016 00017 // **************************************************************************** 00018 // Configuration section 00019 00020 // Ethernet configuration 00021 /* Define this to enable DHCP, otherwise manual address configuration is used */ 00022 //#define DHCP 00023 00024 /* Manual IP configurations, if DHCP not defined */ 00025 #define IP "192.168.1.108" 00026 #define MASK "255.255.255.0" 00027 #define GW "" 00028 00029 // NSP configuration 00030 /* Change this IP address to that of your NanoService Platform installation */ 00031 static const char* NSP_ADDRESS = "192.168.1.101"; /* demo NSP address */ 00032 static const int NSP_PORT = 5683; 00033 char endpoint_name[16] = "mbed-"; 00034 uint8_t ep_type[] = {"mbed_device"}; 00035 uint8_t lifetime_ptr[] = {"1200"}; 00036 00037 // **************************************************************************** 00038 // Ethernet initialization 00039 00040 EthernetInterface eth; 00041 00042 static void ethernet_init() 00043 { 00044 char mbed_uid[33]; // for creating unique name for the board 00045 00046 /* Initialize network */ 00047 #ifdef DHCP 00048 NSDL_DEBUG("DHCP in use\r\n"); 00049 eth.init(); 00050 #else 00051 eth.init(IP, MASK, GW); 00052 #endif 00053 if(eth.connect(30000) == 0) 00054 pc.printf("Connect OK\n\r"); 00055 00056 mbed_interface_uid(mbed_uid); 00057 mbed_uid[32] = '\0'; 00058 strncat(endpoint_name, mbed_uid + 27, 15 - strlen(endpoint_name)); 00059 00060 lcd.locate(0,11); 00061 lcd.printf("IP:%s", eth.getIPAddress()); 00062 00063 NSDL_DEBUG("IP Address:%s ", eth.getIPAddress()); 00064 } 00065 00066 // **************************************************************************** 00067 // NSP initialization 00068 00069 UDPSocket server; 00070 Endpoint nsp; 00071 00072 static void nsp_init() 00073 { 00074 server.init(); 00075 server.bind(NSP_PORT); 00076 00077 nsp.set_address(NSP_ADDRESS, NSP_PORT); 00078 00079 NSDL_DEBUG("name: %s", endpoint_name); 00080 NSDL_DEBUG("NSP=%s - port %d\n", NSP_ADDRESS, NSP_PORT); 00081 00082 lcd.locate(0,22); 00083 lcd.printf("EP name:%s\n", endpoint_name); 00084 } 00085 00086 // **************************************************************************** 00087 // Resource creation 00088 00089 static int create_resources() 00090 { 00091 sn_nsdl_resource_info_s *resource_ptr = NULL; 00092 sn_nsdl_ep_parameters_s *endpoint_ptr = NULL; 00093 00094 NSDL_DEBUG("Creating resources"); 00095 00096 /* Create resources */ 00097 resource_ptr = (sn_nsdl_resource_info_s*)nsdl_alloc(sizeof(sn_nsdl_resource_info_s)); 00098 if(!resource_ptr) 00099 return 0; 00100 memset(resource_ptr, 0, sizeof(sn_nsdl_resource_info_s)); 00101 00102 resource_ptr->resource_parameters_ptr = (sn_nsdl_resource_parameters_s*)nsdl_alloc(sizeof(sn_nsdl_resource_parameters_s)); 00103 if(!resource_ptr->resource_parameters_ptr) 00104 { 00105 nsdl_free(resource_ptr); 00106 return 0; 00107 } 00108 memset(resource_ptr->resource_parameters_ptr, 0, sizeof(sn_nsdl_resource_parameters_s)); 00109 00110 // Dynamic resources 00111 create_accelerometer_resource(resource_ptr); 00112 create_joystick_resource(resource_ptr); 00113 create_buzz_resource(resource_ptr); 00114 create_led_resource(resource_ptr); 00115 create_lcd_resource(resource_ptr); 00116 00117 /* Register with NSP */ 00118 endpoint_ptr = nsdl_init_register_endpoint(endpoint_ptr, (uint8_t*)endpoint_name, ep_type, lifetime_ptr); 00119 if(sn_nsdl_register_endpoint(endpoint_ptr) != 0) 00120 pc.printf("NSP registering failed\r\n"); 00121 else 00122 pc.printf("NSP registering OK\r\n"); 00123 nsdl_clean_register_endpoint(&endpoint_ptr); 00124 00125 nsdl_free(resource_ptr->resource_parameters_ptr); 00126 nsdl_free(resource_ptr); 00127 return 1; 00128 } 00129 00130 // **************************************************************************** 00131 // Program entry point 00132 00133 int main() 00134 { 00135 lcd.cls(); 00136 lcd.locate(0,0); 00137 lcd.printf("NS Game Controller Demo\n"); 00138 00139 // Initialize Ethernet interface first 00140 ethernet_init(); 00141 00142 // Initialize NSP node 00143 nsp_init(); 00144 00145 // Initialize NSDL stack 00146 nsdl_init(); 00147 00148 // Create NSDL resources 00149 create_resources(); 00150 00151 // Run the NSDL event loop (never returns) 00152 nsdl_event_loop(); 00153 }
Generated on Wed Jul 13 2022 01:21:07 by
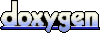