
NanoService device game controller for NSPong.
Dependencies: Beep C12832_lcd EthernetInterface MMA7660 mbed-rtos mbed nsdl_lib
lcd.cpp
00001 // Lcd resource implementation 00002 00003 #include "mbed.h" 00004 #include "nsdl_support.h" 00005 #include "C12832_lcd.h" 00006 #include "lcd.h" 00007 00008 #define LCD_RES_ID "lcd" 00009 00010 extern Serial pc; 00011 static C12832_LCD lcd; 00012 // Data from PUT request 00013 static char received_cmd[20]; 00014 static char player_in_selection[5]; 00015 00016 /* Only PUT method allowed */ 00017 static uint8_t lcd_resource_cb(sn_coap_hdr_s *received_coap_ptr, sn_nsdl_addr_s *address, sn_proto_info_s * proto) 00018 { 00019 sn_coap_hdr_s *coap_res_ptr = 0; 00020 00021 memcpy(received_cmd, (char *)received_coap_ptr->payload_ptr, received_coap_ptr->payload_len); 00022 received_cmd[received_coap_ptr->payload_len] = '\0'; 00023 sprintf(player_in_selection, "%s", received_cmd); 00024 00025 lcd.cls(); 00026 lcd.locate(0,0); 00027 00028 if (!strcmp(player_in_selection, "1")) { 00029 lcd.printf("You are player 1\nYour paddle is on the left\nGood luck and have fun!"); 00030 } 00031 else if (!strcmp(player_in_selection, "2")) { 00032 lcd.printf("You are player 2\nYour paddle is on the right\nGood luck and have fun!"); 00033 } 00034 else if(!strcmp(player_in_selection, "info")) { 00035 lcd.printf("You have been registered\nPress joystick to start!"); 00036 } 00037 00038 coap_res_ptr = sn_coap_build_response(received_coap_ptr, COAP_MSG_CODE_RESPONSE_CHANGED); 00039 sn_nsdl_send_coap_message(address, coap_res_ptr); 00040 sn_coap_parser_release_allocated_coap_msg_mem(coap_res_ptr); 00041 return 0; 00042 } 00043 00044 int create_lcd_resource(sn_nsdl_resource_info_s *resource_ptr) 00045 { 00046 nsdl_create_dynamic_resource(resource_ptr, sizeof(LCD_RES_ID)-1, (uint8_t*)LCD_RES_ID, 0, 0, 0, &lcd_resource_cb, SN_GRS_PUT_ALLOWED); 00047 return 0; 00048 }
Generated on Wed Jul 13 2022 01:21:07 by
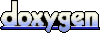