
update with altimeter, swimfile.txt endleg.txt, etc see changes_13sep.txt also reset_PI()
Dependencies: mbed MODSERIAL FATFileSystem
MbedLogger.cpp
00001 #include "MbedLogger.hpp" 00002 #include "StaticDefs.hpp" 00003 #include <stdarg.h> 00004 00005 //Timer t; //used to test time to create packet //timing debug 00006 00007 MbedLogger::MbedLogger(string file_system_input_string) { 00008 _file_system_string = file_system_input_string; // how to make the LOG000.csv a variable string? 00009 _full_file_path_string = _file_system_string + "LOG000.csv"; //use multiple logs in the future? (after file size is too large) 00010 _full_diagfile_path_string = _file_system_string + "DIAG000.txt"; 00011 _file_number = 0; 00012 _file_transmission = true; 00013 _confirmed_packet_number = 0; //must set this to zero 00014 _transmit_counter = 0; 00015 _file_transmission_state = -1; 00016 _total_number_of_packets = 0; 00017 _mbed_transmit_loop = false; 00018 _received_filename = ""; 00019 00020 _log_file_line_counter = 0; //used to set timer in finite state machine based on size of log 00021 00022 //heading string is 254 bytes long, FIXED LENGTH 00023 _heading_string = "ZStateStr,St#,TimeSec,Timesec-diff,DepthCmd,DepthM,PitchCmd,PitchDeg,RudderPWM,RudderCmdDeg,HeadDeg,bceCmd,bce_mm,battCmd,batt_mm,PitchRateDegSec,depth_rate_mps,SystemAmps,SystemVolts,AltChRd,Int_PSI\n"; 00024 _heading_string2 = "Statenum,TimeSec-diff,DepthM,PitchDeg,RollDeg,HeadDeg,VertSpeed, Altim\n"; 00025 _diag_heading_string = "Diagnostics file header \n"; 00026 00027 _fsm_transmit_complete = false; 00028 00029 _end_transmit_packet = false; 00030 _default_timestamp_time = 1518467832; 00031 00032 _end_sequence_transmission = false; 00033 _time_set =0; 00034 } 00035 00036 //this function has to be called for the time to function correctly 00037 void MbedLogger::setLogTime(long int setting_time) { 00038 00039 if ( setting_time == -1) { set_time(_default_timestamp_time); } 00040 else { set_time(setting_time); _default_timestamp_time = setting_time; } 00041 xbee().printf("\n%s log time set.\n\r", _file_system_string.c_str()); 00042 // set_time(time_stamp); // Set RTC time to Mon, 12 FEB 2018 15:37 00043 _time_set =1; 00044 } 00045 00046 //in the future create the ability to set the start time 00047 time_t MbedLogger::getSystemTime() { 00048 time_t seconds = time(NULL); // Time as seconds since January 1, 1970 00049 00050 return seconds; 00051 } 00052 void MbedLogger::recordData_short(int current_state) { 00053 int data_log_time = mbedLogger().getSystemTime(); //read the system timer to get unix timestamp 00054 int start_time = 1518467832; 00055 if(_time_set) { start_time = _default_timestamp_time;} 00056 00057 _data_log[0] = depthLoop().getPosition(); //depth reading (filtered depth) 00058 00059 _data_log[1] = pitchLoop().getPosition(); //pitch reading (filtered pitch) 00060 00061 _data_log[2] = imu().getRoll(); 00062 _data_log[3] = headingLoop().getPosition(); //heading reading (filtered heading) 00063 _data_log[4] = depthLoop().getVelocity(); //vertical speed 00064 // _data_log[5] = sensors().getAltimeterReading_m(); // Altimeter Channel value in meters 00065 _data_log[5] = altimLoop().getPosition(); // AltimLoop runs smoothed values of the sensor readings. 00066 00067 fprintf(_fp, "%02d,%05d,%5.1f,%5.1f,%5.1f,%5.1f,%5.1f,%5.1f\n", 00068 current_state, data_log_time-start_time, //note offset relative to start 00069 _data_log[0],_data_log[1],_data_log[2],_data_log[3], _data_log[4],_data_log[5]); 00070 00071 } 00072 void MbedLogger::recordData_long(int current_state) { 00073 int data_log_time = mbedLogger().getSystemTime(); //read the system timer to get unix timestamp 00074 int start_time = 1518467832; 00075 if(_time_set) { start_time = _default_timestamp_time;} 00076 _data_log[0] = depthLoop().getCommand(); //depth command 00077 _data_log[1] = depthLoop().getPosition(); //depth reading (filtered depth) 00078 _data_log[2] = pitchLoop().getCommand(); //pitch command 00079 _data_log[3] = pitchLoop().getPosition(); //pitch reading (filtered pitch) 00080 _data_log[4] = rudder().getSetPosition_pwm(); //rudder command PWM 00081 _data_log[5] = rudder().getSetPosition_deg(); //rudder command DEG 00082 _data_log[6] = headingLoop().getPosition(); //heading reading (filtered heading) 00083 00084 _data_log[7] = bce().getSetPosition_mm(); //BCE command 00085 _data_log[8] = bce().getPosition_mm(); //BCE reading 00086 _data_log[9] = batt().getSetPosition_mm(); //Batt command 00087 _data_log[10] = batt().getPosition_mm(); //Batt reading 00088 _data_log[11] = pitchLoop().getVelocity(); // pitchRate_degs (degrees per second) 00089 _data_log[12] = depthLoop().getVelocity(); // depthRate_fps (feet per second) 00090 _data_log[13] = sensors().getCurrentInput(); // i_in 00091 _data_log[14] = sensors().getVoltageInput(); // v_in 00092 // _data_log[15] = sensors().getAltimeterReading_m(); // Altimeter Channel in meters 00093 _data_log[15] = altimLoop().getPosition(); // AltimLoop runs smoothed values of the sensor readings. 00094 _data_log[16] = sensors().getInternalPressurePSI(); // int_press_PSI 00095 00096 string string_state; string_state= "UNKNOWN"; //default just in case. 00097 00098 if (current_state == SIT_IDLE) 00099 string_state = "SIT_IDLE"; 00100 else if (current_state == FIND_NEUTRAL) 00101 string_state = "FIND_NEUTRAL"; 00102 else if (current_state == DIVE) 00103 string_state = "DIVE"; 00104 else if (current_state == RISE) 00105 string_state = "RISE"; 00106 else if (current_state == FLOAT_LEVEL) 00107 string_state = "FLOAT_LEVEL"; 00108 else if (current_state == FLOAT_BROADCAST) 00109 string_state = "FLOAT_BROADCAST"; 00110 else if (current_state == EMERGENCY_CLIMB) 00111 string_state = "EMERGENCY_CLIMB"; 00112 else if (current_state == MULTI_DIVE) 00113 string_state = "MULTI_DIVE"; 00114 else if (current_state == MULTI_RISE) 00115 string_state = "MULTI_RISE"; 00116 else if (current_state == KEYBOARD) 00117 string_state = "KEYBOARD"; 00118 else if (current_state == CHECK_TUNING) 00119 string_state = "CHECK_TUNING"; 00120 else if (current_state == POSITION_DIVE) 00121 string_state = "POSITION_DIVE"; 00122 else if (current_state == POSITION_RISE) 00123 string_state = "POSITION_RISE"; 00124 else if (current_state == TX_MBED_LOG) 00125 string_state = "TX_MBED_LOG"; 00126 else if (current_state == RX_SEQUENCE) 00127 string_state = "RECEIVE_SEQUENCE"; 00128 else if (current_state == LEG_POSITION_DIVE) 00129 string_state = "LEG_POS_DIVE"; 00130 else if (current_state == LEG_POSITION_RISE) 00131 string_state = "LEG_POS_RISE"; 00132 else if (current_state == FB_EXIT) 00133 string_state = "FB_EXIT"; 00134 else if (current_state == START_SWIM) 00135 string_state = "START_SWIM"; 00136 else if (current_state == FLYING_IDLE) 00137 string_state = "FLYING_IDLE"; 00138 else if (current_state == ENDLEG_WAIT) 00139 string_state = "ENDLEG_WAIT"; 00140 00141 fprintf(_fp, "Z%17s,%02d,%10d,%05d,%5.1f,%5.1f,%6.1f,%6.1f,%4.0f,%4.0f,%6.1f,%5.1f,%6.1f,%5.1f,%6.1f,%6.1f,%6.1f,%6.3f,%6.2f,%5.0f,%6.2f\n", 00142 string_state.c_str(),current_state, data_log_time,data_log_time-start_time, _data_log[0],_data_log[1],_data_log[2],_data_log[3],_data_log[4], 00143 _data_log[5],_data_log[6],_data_log[7],_data_log[8],_data_log[9],_data_log[10],_data_log[11],_data_log[12],_data_log[13], 00144 _data_log[14],_data_log[15], _data_log[16]); 00145 00146 00147 } 00148 void MbedLogger::recordData(int current_state) { 00149 int data_log_time = mbedLogger().getSystemTime(); //read the system timer to get unix timestamp 00150 int start_time = 1518467832; 00151 if(_time_set) { start_time = _default_timestamp_time;} 00152 _data_log[0] = depthLoop().getCommand(); //depth command 00153 _data_log[1] = depthLoop().getPosition(); //depth reading (filtered depth) 00154 _data_log[2] = pitchLoop().getCommand(); //pitch command 00155 _data_log[3] = pitchLoop().getPosition(); //pitch reading (filtered pitch) 00156 _data_log[4] = rudder().getSetPosition_pwm(); //rudder command PWM 00157 _data_log[5] = rudder().getSetPosition_deg(); //rudder command DEG 00158 _data_log[6] = headingLoop().getPosition(); //heading reading (filtered heading) 00159 00160 _data_log[7] = bce().getSetPosition_mm(); //BCE command 00161 _data_log[8] = bce().getPosition_mm(); //BCE reading 00162 _data_log[9] = batt().getSetPosition_mm(); //Batt command 00163 _data_log[10] = batt().getPosition_mm(); //Batt reading 00164 _data_log[11] = pitchLoop().getVelocity(); // pitchRate_degs (degrees per second) 00165 _data_log[12] = depthLoop().getVelocity(); // depthRate_fps (feet per second) 00166 00167 _data_log[13] = sensors().getCurrentInput(); // i_in 00168 _data_log[14] = sensors().getVoltageInput(); // v_in 00169 _data_log[15] = sensors().getAltimeterChannelReadings(); // Altimeter Channel Readings 00170 _data_log[16] = sensors().getInternalPressurePSI(); // int_press_PSI 00171 00172 //BCE_p,i,d,freq,deadband 00173 _data_log[17] = bce().getControllerP(); 00174 _data_log[18] = bce().getControllerI(); 00175 _data_log[19] = bce().getControllerD(); 00176 00177 _data_log[20] = batt().getControllerP(); 00178 _data_log[21] = batt().getControllerI(); 00179 _data_log[22] = batt().getControllerD(); 00180 00181 _data_log[23] = depthLoop().getControllerP(); 00182 _data_log[24] = depthLoop().getControllerI(); 00183 _data_log[25] = depthLoop().getControllerD(); 00184 _data_log[26] = depthLoop().getFilterFrequency(); 00185 _data_log[27] = depthLoop().getDeadband(); 00186 00187 _data_log[28] = pitchLoop().getControllerP(); 00188 _data_log[29] = pitchLoop().getControllerI(); 00189 _data_log[30] = pitchLoop().getControllerD(); 00190 00191 _data_log[31] = headingLoop().getControllerP(); 00192 _data_log[32] = headingLoop().getControllerI(); 00193 _data_log[33] = headingLoop().getControllerD(); 00194 _data_log[34] = headingLoop().getFilterFrequency(); 00195 _data_log[35] = headingLoop().getDeadband(); 00196 00197 string string_state; string_state= "UNKNOWN"; //default just in case. 00198 00199 if (current_state == SIT_IDLE) 00200 string_state = "SIT_IDLE"; 00201 else if (current_state == FIND_NEUTRAL) 00202 string_state = "FIND_NEUTRAL"; 00203 else if (current_state == DIVE) 00204 string_state = "DIVE"; 00205 else if (current_state == RISE) 00206 string_state = "RISE"; 00207 else if (current_state == FLOAT_LEVEL) 00208 string_state = "FLOAT_LEVEL"; 00209 else if (current_state == FLOAT_BROADCAST) 00210 string_state = "FLOAT_BROADCAST"; 00211 else if (current_state == EMERGENCY_CLIMB) 00212 string_state = "EMERGENCY_CLIMB"; 00213 else if (current_state == MULTI_DIVE) 00214 string_state = "MULTI_DIVE"; 00215 else if (current_state == MULTI_RISE) 00216 string_state = "MULTI_RISE"; 00217 else if (current_state == KEYBOARD) 00218 string_state = "KEYBOARD"; 00219 else if (current_state == CHECK_TUNING) 00220 string_state = "CHECK_TUNING"; 00221 else if (current_state == POSITION_DIVE) 00222 string_state = "POSITION_DIVE"; 00223 else if (current_state == POSITION_RISE) 00224 string_state = "POSITION_RISE"; 00225 else if (current_state == TX_MBED_LOG) 00226 string_state = "TX_MBED_LOG"; 00227 else if (current_state == RX_SEQUENCE) 00228 string_state = "RECEIVE_SEQUENCE"; 00229 else if (current_state == LEG_POSITION_DIVE) 00230 string_state = "LEG_POS_DIVE"; 00231 else if (current_state == LEG_POSITION_RISE) 00232 string_state = "LEG_POS_RISE"; 00233 else if (current_state == FB_EXIT) 00234 string_state = "FB_EXIT"; 00235 else if (current_state == START_SWIM) 00236 string_state = "START_SWIM"; 00237 else if (current_state == FLYING_IDLE) 00238 string_state = "FLYING_IDLE"; 00239 else if (current_state == ENDLEG_WAIT) 00240 string_state = "ENDLEG_WAIT"; 00241 00242 string blank_space = ""; //to get consistent spacing in the file (had a nonsense char w/o this) 00243 00244 //below this format is used for data transmission, each packet needs to be 254 characters long (not counting newline char) 00245 00246 //verified that this generates the correct line length of 254 using SOLELY an mbed 08/16/2018 00247 fprintf(_fp, "%17s,%.2d,%10d,%5.1f,%5.1f,%6.1f,%6.1f,%4.0f,%4.0f,%6.1f,%5.1f,%6.1f,%5.1f,%6.1f,%6.1f,%6.1f,%6.3f,%6.2f,%5.0f,%6.2f,%5.3f,%5.3f,%5.3f,%5.3f,%5.3f,%5.3f,%6.2f,%5.3f,%5.3f,%4.1f,%4.1f,%5.3f,%5.3f,%5.3f,%5.3f,%5.3f,%5.3f,%4.1f,%4.1f\n", 00248 string_state.c_str(),current_state, 00249 data_log_time-start_time, //note offset relative to start 00250 _data_log[0],_data_log[1],_data_log[2],_data_log[3],_data_log[4],_data_log[5],_data_log[6],_data_log[7],_data_log[8],_data_log[9],_data_log[10],_data_log[11],_data_log[12],_data_log[13],_data_log[14],_data_log[15], 00251 _data_log[16],_data_log[17],_data_log[18],_data_log[19],_data_log[20],_data_log[21],_data_log[22],_data_log[23],_data_log[24],_data_log[25],_data_log[26],_data_log[27],_data_log[28],_data_log[29],_data_log[30], 00252 _data_log[31],_data_log[32],_data_log[33],_data_log[34],_data_log[35]); 00253 00254 //each line in the file is 160 characters long text-wise, check this with a file read 00255 } 00256 00257 void MbedLogger::printMbedDirectory() { 00258 DIR *dir; 00259 struct dirent *dp; //dirent.h is the format of directory entries 00260 int log_found =0,loop=0; 00261 long int temp=0; 00262 00263 //char * char_pointer; 00264 char * numstart; 00265 //char *numstop; 00266 00267 xbee().printf("\n\rPrinting out the directory of device %s\n\r", _file_system_string.c_str()); 00268 00269 if ( NULL == (dir = opendir( _file_system_string.c_str() )) ) { 00270 xbee().printf("MBED directory could not be opened\r\n"); 00271 } 00272 else 00273 { 00274 while ( NULL != (dp = readdir( dir )) ) 00275 { 00276 if(strncmp(dp->d_name,"LOG",3)==0) 00277 { 00278 log_found=1; 00279 /*numstart = dp->d_name; //Look for third character (do safety) 00280 numstop = strchr(dp->d_name,'.'); 00281 if(numstart!=NULL&&numstop!=NULL) 00282 { 00283 temp=numstop-numstart; 00284 } 00285 else 00286 log_found=0; //Something is not right. Ignore 00287 */ 00288 numstart=dp->d_name+3; 00289 temp=strtol(numstart,NULL,10); //add in check to see if this is null (start logs at one) 00290 } 00291 else 00292 log_found=0; 00293 xbee().printf( "%d. %s (log file: %d, %d)\r\n", loop, dp->d_name,log_found,temp); 00294 00295 loop++; 00296 } 00297 } 00298 } 00299 00300 //prints current log file to the screen (terminal) 00301 void MbedLogger::printCurrentLogFile() { 00302 //open the file for reading 00303 // string file_name_string = _file_system_string + "LOG000.csv"; 00304 string file_name_string = _file_system_string + configFileIO().logFilesStruct.logFileName; // "DIAG000.txt"; 00305 //string file_name_string = _file_system_string + "LOG000.csv"; // how to pass in log file name as a string? 00306 00307 _log_file_line_counter = 0; 00308 00309 _fp = fopen(file_name_string.c_str(), "r"); 00310 00311 char buffer[500]; 00312 00313 //read the file line-by-line and print that to the screen 00314 xbee().printf("\n\rCURRENT MBED LOG FILE /local/Log%03d.csv:\n\n\r",_file_number); 00315 while (!feof(_fp)) { 00316 // read in the line and make sure it was successful 00317 if (fgets(buffer,500,_fp) != NULL) { //stops at new line 00318 xbee().printf("%s\r",buffer); 00319 _log_file_line_counter++; 00320 } 00321 } 00322 00323 //fgets stops when either (n-1) characters are read, the newline character is read, 00324 // or the end-of-file is reached 00325 00326 //close the file 00327 closeLogFile(); 00328 xbee().printf("\n\rLog file closed. Lines in log file: %d.\n\r", _log_file_line_counter); 00329 } 00330 00331 void MbedLogger::blastData() { 00332 xbee().printf("blastData FUNCTION\n\r"); 00333 00334 //OPEN FILE FOR READING 00335 00336 //string file_name_string = _file_system_string + "LOG000.csv"; 00337 string file_name_string = _file_system_string + configFileIO().logFilesStruct.logFileName; // "DIAG000.txt"; 00338 //string file_name_string = _file_system_string + "LOG000.csv"; // how to pass in log file name as a string? 00339 00340 _fp = fopen(file_name_string.c_str(), "r"); 00341 00342 /******************************/ 00343 while(1) { 00344 wait(0.05); 00345 if (!feof(_fp)) { //check for end of file 00346 //based on the internal packet number of the class //createDataPacket //transmit data packet 00347 transmitPacketNumber(_packet_number); 00348 xbee().printf("\r"); // for proper spacing 00349 00350 _packet_number++; 00351 } 00352 else { 00353 _packet_number = 0; //reset packet number 00354 break; 00355 } 00356 } 00357 /******************************/ 00358 00359 //CLOSE THE FILE 00360 closeLogFile(); 00361 xbee().printf("\n\rblastData: Log file closed. %d.\n\r"); 00362 } 00363 00364 void MbedLogger::createDataPacket() { 00365 // packet is 7565 0001 FFFF EEEE CC DATA DATA DATA ... CRC1 CRC2 00366 00367 //CLEAR: Removes all elements from the vector (which are destroyed), leaving the container with a size of 0. 00368 _data_packet.clear(); 00369 00370 //DATA PACKET HEADER 00371 _data_packet.push_back(117); //0x75 00372 _data_packet.push_back(101); //0x65 00373 00374 _data_packet.push_back(_packet_number/256); //current packet number in 0x#### form 00375 _data_packet.push_back(_packet_number%256); //current packet number in 0x#### form 00376 00377 _data_packet.push_back(_total_number_of_packets/256); //total number of packets, 0x#### form 00378 _data_packet.push_back(_total_number_of_packets%256); //total number of packets, 0x#### form 00379 00380 _data_packet.push_back(_current_line_length); 00381 00382 //xbee().printf("DEBUG: Current line buffer: %s\n\r", _line_buffer); //debug 00383 00384 //DATA FROM LINE READ (read string character by chracter) 00385 for (int i = 0; i < _current_line_length; i++) { 00386 _data_packet.push_back(_line_buffer[i]); 00387 } 00388 00389 //CRC CALCULATIONS BELOW 00390 //character version of calculation screws up on null character 0x00, scrapped and using vector of integers 00391 00392 int crc_one = calcCrcOne(); 00393 int crc_two = calcCrcTwo(); 00394 00395 //place the crc bytes into the data packet that is transmitted 00396 _data_packet.push_back(crc_one); 00397 _data_packet.push_back(crc_two); 00398 } 00399 00400 //new 6/27/2018 00401 void MbedLogger::createDataPacket(char line_buffer_sent[], int line_length_sent) { 00402 // packet is 7565 0001 FFFF EEEE CC DATA DATA DATA ... CRC1 CRC2 00403 00404 //CLEAR: Removes all elements from the vector (which are destroyed), leaving the container with a size of 0. 00405 _data_packet.clear(); 00406 00407 //DATA PACKET HEADER 00408 _data_packet.push_back(117); //0x75 00409 _data_packet.push_back(101); //0x65 00410 00411 _data_packet.push_back(_packet_number/256); //current packet number in 0x#### form 00412 _data_packet.push_back(_packet_number%256); //current packet number in 0x#### form 00413 00414 _data_packet.push_back(_total_number_of_packets/256); //total number of packets, 0x#### form 00415 _data_packet.push_back(_total_number_of_packets%256); //total number of packets, 0x#### form 00416 00417 _data_packet.push_back(line_length_sent); 00418 00419 //xbee().printf("DEBUG: Current line buffer: %s\n\r", _line_buffer); //debug 00420 00421 //DATA FROM LINE READ (read string character by chracter) 00422 for (int i = 0; i < line_length_sent; i++) { 00423 _data_packet.push_back(line_buffer_sent[i]); 00424 } 00425 00426 //CRC CALCULATIONS BELOW 00427 //character version of calculation screws up on null character 0x00, scrapped and using vector of integers 00428 00429 int crc_one = calcCrcOne(); 00430 int crc_two = calcCrcTwo(); 00431 00432 //place the crc bytes into the data packet that is transmitted 00433 _data_packet.push_back(crc_one); 00434 _data_packet.push_back(crc_two); 00435 00436 //xbee().printf("debug createDataPacket(char line_buffer_sent[], int line_length_sent)\n\r"); 00437 } 00438 00439 //should i save it as a string and transmit the string? next iteration maybe 00440 00441 void MbedLogger::transmitDataPacket() { 00442 //WRITE the data (in bytes) to the serial port 00443 for (_it=_data_packet.begin(); _it < _data_packet.end(); _it++) { 00444 xbee().putc(*_it); //send integers over serial port one byte at a time 00445 } 00446 } 00447 00448 // test to see if transmitDataPacket is working slower than you think. 00449 00450 // REACH ONE CHAR AT A TIME (EACH ITERATION OF STATE MACHINE...) 00451 00452 00453 void MbedLogger::continuouslyTransmitDataNoTimer() { 00454 // string file_name_string = _file_system_string + "LOG000.csv"; 00455 string file_name_string = _file_system_string + configFileIO().logFilesStruct.logFileName; // "DIAG000.txt"; 00456 //string file_name_string = _file_system_string + "LOG000.csv"; // how to pass in log file name as a string? 00457 00458 _fp = fopen(file_name_string.c_str(), "r"); 00459 00460 static int packet_num = 0; 00461 00462 while(!feof(_fp)) { 00463 00464 _packet_number = packet_num; 00465 00466 readPacketInSeries(); //not using this but for testing purposes... 00467 00468 // // createDataPacket requires _packet_number, _total_number_of_packets, _current_line_length (data packet size) 00469 // // uses char _line_buffer[256] variable to hold characters read from the file 00470 // // packs this into a vector for transmission 00471 00472 createDataPacket(); 00473 00474 transmitDataPacket(); 00475 00476 packet_num++; 00477 00478 led3() = !led3(); 00479 00480 //check for incoming request 00481 } 00482 00483 closeLogFile(); //close log file if open 00484 led4() = !led4(); 00485 } 00486 00487 void MbedLogger::fsmTransmitData() { //using the finite state machine 00488 if ( !feof(_fp) and (!_fsm_transmit_complete) ) { //check for end of file 00489 //based on the internal packet number of the class //createDataPacket //transmit data packet 00490 transmitPacketNumber(_packet_number); 00491 00492 xbee().printf("\r"); // for proper spacing 00493 00494 _packet_number++; 00495 00496 led2() = !led2(); 00497 } 00498 else { 00499 _packet_number = 0; //reset packet number 00500 00501 _fsm_transmit_complete = true; 00502 00503 led3() = !led3(); 00504 } 00505 } 00506 00507 //transmitting log file with fixed length of characters to receiver program 00508 void MbedLogger::transmitPacketNumber(int line_number) { 00509 int line_size = 254; //length of lines in the log file, EVERY LINE MUST BE THE SAME LENGTH 00510 00511 fseek(_fp,(line_size+1)*line_number,SEEK_SET); //fseek must use the +1 to get the newline character 00512 00513 //write over the internal _line_buffer //start from the beginning and go to this position 00514 fread(_line_buffer, 1, line_size, _fp); //read the line that is exactly 160 characters long 00515 00516 //xbee().printf("Debug (transmitPacketNumber): line_buffer <<%s>> (line size: %d)\n\r", line_buffer,line_size); 00517 00518 // createDataPacket requires _packet_number, _total_number_of_packets, _current_line_length (data packet size) 00519 // uses char _line_buffer[256] variable to hold characters read from the file 00520 // packs this into a vector for transmission 00521 00522 //change the internal member variable for packet number, reorg this later 00523 _packet_number = line_number; 00524 00525 createDataPacket(_line_buffer, line_size); //create the data packet from the _line_buffer (char array) 00526 00527 transmitDataPacket(); //transmit the assembled packet 00528 } 00529 00530 00531 //receive correct data packet from python, immediately send a transmit packet from the MBED 00532 void MbedLogger::transmitOnePacket() { 00533 xbee().printf("transmitOnePacket\n"); 00534 00535 static int transmit_state = HEADER_117; //state in switch statement 00536 //int incoming_byte = -1; //reset each time a character is read 00537 int requested_packet_number = -1; //reset each time a character is read 00538 static int input_packet[4]; //changed from char in previous iteration 03/28/2018 00539 static int transmit_crc_one = 0; //hold crc values until they're reset with calculations 00540 static int transmit_crc_two = 0; 00541 00542 int incoming_byte[6]; 00543 00544 static int bytes_received = 0; 00545 00546 int req_packet_number = -1; 00547 00548 while (1) { 00549 incoming_byte[bytes_received] = xbee().getc(); 00550 xbee().printf("<%d> ", incoming_byte[bytes_received]); 00551 00552 bytes_received++; 00553 00554 if (bytes_received > 5) { 00555 00556 req_packet_number = incoming_byte[2] * 256 + incoming_byte[3]; 00557 00558 xbee().printf("req_packet_number = %d\n\r", req_packet_number); 00559 00560 bytes_received = 0; 00561 break; 00562 } 00563 } 00564 00565 setTransmitPacketNumber(0); 00566 00567 //open the file 00568 // string file_name_string = _file_system_string + "LOG000.csv"; 00569 string file_name_string = _file_system_string + configFileIO().logFilesStruct.logFileName; // "DIAG000.txt"; 00570 //string file_name_string = _file_system_string + "LOG000.csv"; // how to pass in log file name as a string? 00571 _fp = fopen(file_name_string.c_str(), "r"); 00572 00573 //receive correct checksum, immediately send this packet 00574 transmitPacketNumber(req_packet_number); 00575 00576 //fclose(_fp); 00577 closeLogFile(); 00578 } 00579 00580 void MbedLogger::transmitMultiplePackets() { 00581 xbee().printf("transmitMultiplePackets\n"); 00582 00583 //static int transmit_state = HEADER_117; //state in switch statement 00584 //int incoming_byte = -1; //reset each time a character is read 00585 //int requested_packet_number = -1; //reset each time a character is read 00586 static int input_packet; //changed from char in previous iteration 03/28/2018 00587 static int transmit_crc_one = 0; //hold crc values until they're reset with calculations 00588 static int transmit_crc_two = 0; 00589 00590 int current_byte = -1; 00591 00592 static int bytes_received = 0; 00593 00594 int req_packet_number = -1; 00595 00596 //GET TOTAL NUMBER OF PACKETS! 00597 getNumberOfPacketsInCurrentLog(); 00598 //GET TOTAL NUMBER OF PACKETS! 00599 00600 //open the file 00601 // string file_name_string = _file_system_string + "LOG000.csv"; 00602 string file_name_string = _file_system_string + configFileIO().logFilesStruct.logFileName; // "DIAG000.txt"; 00603 //string file_name_string = _file_system_string + "LOG000.csv"; // how to pass in log file name as a string? 00604 _fp = fopen(file_name_string.c_str(), "r"); 00605 00606 //DEFAULT STATE 00607 static int current_state = HEADER_117; 00608 00609 bool active_loop = true; 00610 00611 while (active_loop) { 00612 //INCOMING BYTE 00613 current_byte = xbee().getc(); 00614 00615 //provide the next byte / state 00616 00617 switch (current_state) { 00618 case HEADER_117: 00619 //xbee().printf("HEADING 117\n\r"); 00620 if (current_byte == 0x75) { 00621 current_state = HEADER_101; 00622 } 00623 00624 00625 else if (current_byte == 0x10) { 00626 current_state = END_TX_1; 00627 } 00628 break; 00629 case HEADER_101: 00630 //xbee().printf("HEADING 101\n\r"); 00631 if (current_byte == 0x65) { 00632 current_state = PACKET_NO_1; 00633 } 00634 break; 00635 case PACKET_NO_1: 00636 //xbee().printf("PACKET_NO_1\n\r"); 00637 input_packet = current_byte * 256; 00638 00639 current_state = PACKET_NO_2; 00640 //xbee().printf("PACKET # 1 current byte %d\n\r", current_byte); 00641 break; 00642 00643 case PACKET_NO_2: 00644 //xbee().printf("PACKET_NO_2\n\r"); 00645 input_packet = input_packet + current_byte; 00646 00647 current_state = PACKET_CRC_ONE; 00648 //xbee().printf("PACKET # 2 current byte %d (req packet num: %d)\n\r", current_byte, input_packet); 00649 break; 00650 00651 case PACKET_CRC_ONE: 00652 //xbee().printf("PACKET_CRC_ONE\n\r"); 00653 current_state = PACKET_CRC_TWO; 00654 break; 00655 00656 case PACKET_CRC_TWO: 00657 //xbee().printf("PACKET_CRC_TWO\n\r"); 00658 current_state = HEADER_117; 00659 transmitPacketNumber(input_packet); 00660 break; 00661 00662 case END_TX_1: 00663 //xbee().printf("END_TX_1\n\r"); 00664 current_state = END_TX_2; 00665 break; 00666 case END_TX_2: 00667 //xbee().printf("END_TX_2\n\r"); 00668 current_state = HEADER_117; 00669 active_loop = false; 00670 break; 00671 00672 default: 00673 //reset state 00674 //xbee().printf("DEFAULT. HEADER_117\n\r"); 00675 current_state = HEADER_117; //reset here 00676 break; 00677 00678 } 00679 } 00680 00681 //CLOSE THE FILE 00682 closeLogFile(); 00683 xbee().printf("08/05/2018 CLOSE THE LOG FILE\n\r"); 00684 00685 //RESET THE STATE 00686 //current_state = HEADER_117; 00687 } 00688 00689 void MbedLogger::checkForPythonTransmitRequest() { 00690 //xbee().printf("checkForPythonTransmitRequest\n"); 00691 00692 if ( xbee().readable() ) { 00693 00694 //PC READABLE DOES NOT WORK HERE?! 00695 00696 led2() = !led2(); 00697 led3() = !led3(); 00698 00699 xbee().printf("########################\n"); 00700 //xbee().printf("%d", xbee().getc()); 00701 } 00702 00703 // static int transmit_state = HEADER_117; //state in switch statement 00704 // //int incoming_byte = -1; //reset each time a character is read 00705 // int requested_packet_number = -1; //reset each time a character is read 00706 // static int input_packet[4]; //changed from char in previous iteration 03/28/2018 00707 // static int transmit_crc_one = 0; //hold crc values until they're reset with calculations 00708 // static int transmit_crc_two = 0; 00709 // 00710 // static int incoming_byte[6]; 00711 // 00712 // static int bytes_received = 0; 00713 // 00714 // int req_packet_number = -1; 00715 // 00716 // if (xbee().readable()) { 00717 // incoming_byte[bytes_received] = xbee().getc(); 00718 // xbee().printf("<%d> ", incoming_byte[bytes_received]); 00719 // 00720 // bytes_received++; 00721 // 00722 // if (bytes_received > 5) { 00723 // 00724 // req_packet_number = incoming_byte[2] * 256 + incoming_byte[3]; 00725 // 00726 // xbee().printf("req_packet_number = %d\n\r", req_packet_number); 00727 // 00728 // //reset 00729 // bytes_received = 0; 00730 // } 00731 // } 00732 // 00733 // //setTransmitPacketNumber(0); 00734 // 00735 // /* OPEN THE FILE */ 00736 // string file_name_string = _file_system_string + "LOG000.csv"; 00737 //_fp = fopen(file_name_string.c_str(), "r"); 00738 00739 /* RECEIVE CORRECT CHECKSUM, SEND PACKET */ 00740 //transmitPacketNumber(req_packet_number); 00741 00742 //fclose(_fp); 00743 00744 // switch(transmit_state) { 00745 // case HEADER_117: 00746 // led3() = !led3(); 00747 // 00748 // //continue processing 00749 // if (incoming_byte == 117){ //"u" 00750 // transmit_state = HEADER_101; 00751 // } 00752 // //did not receive byte 1 00753 // else { 00754 // transmit_state = HEADER_117; //go back to checking the first packet 00755 // } 00756 // break; 00757 // 00758 // case HEADER_101: 00759 // if(incoming_byte == 101) { //"e" 00760 // transmit_state = TRANSMIT_PACKET_1; 00761 // //xbee().printf(" U E \n\r"); 00762 // } 00763 // else { 00764 // transmit_state = HEADER_117; 00765 // } 00766 // break; 00767 // 00768 // case TRANSMIT_PACKET_1: 00769 // if (incoming_byte >= 0) { 00770 // input_packet[0] = 117; 00771 // input_packet[1] = 101; 00772 // input_packet[2] = incoming_byte; 00773 // 00774 // transmit_state = TRANSMIT_PACKET_2; 00775 // //_reply_byte3 = incoming_byte; 00776 // 00777 // led1() = !led1(); 00778 // //xbee().printf(" T P \n\r"); 00779 // //xbee().printf("DEBUG: Transmit Packet %d\n\r", incoming_byte); 00780 // } 00781 // break; 00782 // 00783 // case TRANSMIT_PACKET_2: 00784 // 00785 // if (incoming_byte >= 0) { 00786 // input_packet[3] = incoming_byte; 00787 // //_reply_byte4 = incoming_byte; 00788 // } 00789 // transmit_state = PACKET_CRC_ONE; 00790 // 00791 // break; 00792 // 00793 // case (PACKET_CRC_ONE): 00794 // transmit_crc_one = calcCrcOneArray(input_packet, 4); //calc CRC 1 from the input packet (size 4) 00795 // 00796 // if (incoming_byte == transmit_crc_one) { 00797 // transmit_state = PACKET_CRC_TWO; 00798 // } 00799 // 00800 // else 00801 // transmit_state = HEADER_117; 00802 // //or state remains the same? 00803 // 00804 // break; 00805 // 00806 // case (PACKET_CRC_TWO): 00807 // transmit_state = HEADER_117; 00808 // transmit_crc_two = calcCrcTwoArray(input_packet, 4); //calc CRC 2 from the input packet (size 4) 00809 // 00810 // //check if CRC TWO is correct (then send full packet) 00811 // if (incoming_byte == transmit_crc_two) { 00812 // requested_packet_number = input_packet[2] * 256 + input_packet[3]; //compute the numbers 0 through 65535 with the two bytes 00813 // 00814 // //receive correct checksum, immediately send this packet 00815 // transmitPacketNumber(requested_packet_number); 00816 // 00817 // //fseek(_fp, 0, SEEK_END); //reset _fp (this was causing errors with the other function) 00818 // led3() = !led3(); 00819 // 00820 // } //end of checksum (incoming_byte) if statement 00821 // 00822 // break; 00823 // } //switch statement complete 00824 // } //while statement complete 00825 } 00826 00827 int MbedLogger::readTransmitPacket() { 00828 _file_transmission = true; 00829 _file_transmission_state = 0; 00830 00831 //first check if you're receiving data, then read four bytes 00832 00833 int transmit_state = 0; //for state machine 00834 00835 int incoming_byte = -1; 00836 00837 int requested_packet_number = -1; 00838 00839 static int inside_while_loop = 1; 00840 00841 while (inside_while_loop) { 00842 if (xbee().readable()) { //don't rely on pc readable being open all the time 00843 00844 incoming_byte = xbee().getc(); 00845 00846 switch(transmit_state) { 00847 00848 case (HEADER_117): 00849 //continue processing 00850 if (incoming_byte == 117){ 00851 transmit_state = HEADER_101; 00852 } 00853 //end transmission 00854 else if (incoming_byte == 16) { 00855 transmit_state = END_TRANSMISSION; 00856 } 00857 else { 00858 transmit_state = HEADER_117; 00859 } 00860 break; 00861 00862 case (HEADER_101): 00863 if(incoming_byte == 101) { 00864 transmit_state = TRANSMIT_PACKET_1; 00865 } 00866 break; 00867 00868 case (TRANSMIT_PACKET_1): 00869 if (incoming_byte >= 0) { 00870 transmit_state = TRANSMIT_PACKET_2; 00871 _reply_byte3 = incoming_byte; 00872 } 00873 break; 00874 00875 case (TRANSMIT_PACKET_2): 00876 if (incoming_byte >= 0) { 00877 _reply_byte4 = incoming_byte; 00878 } 00879 00880 requested_packet_number = _reply_byte3 * 256 + _reply_byte4; //compute the numbers 0 through 65535 with the two bytes 00881 00882 if (requested_packet_number != _previous_reply_byte) { //CHANGE THE NAME TO SOMETHING NOT BYTE! 00883 //MUST SET THE PACKET NUMBER 00884 _packet_number = requested_packet_number; 00885 00886 readPacketInSeries(); 00887 createDataPacket(); 00888 _previous_reply_byte = requested_packet_number; //RECORD THIS BYTE to prevent new packets being created 00889 } 00890 00891 //continuously transmit current packet until it tells you to do the next packet (response from Python program) 00892 transmitDataPacket(); 00893 00894 //TRANSMIT_PACKET_2 00895 inside_while_loop = 0; //exit the while loop with this 00896 xbee().printf("(TRANSMIT_PACKET_2)reached inside_while_loop = 0\n\r"); //DEBUG 00897 break; 00898 } //end of switch statement 00899 } //end of while loop 00900 00901 // else { 00902 // //xbee().printf("pc not readable \n\r"); 00903 // } 00904 // 00905 } 00906 00907 //once you're outside of the while loop 00908 inside_while_loop = true; //for next iteration 00909 00910 xbee().printf("DEBUG: (readTransmitPacket) Outside of while loop\n\r"); 00911 return false; 00912 } 00913 00914 bool MbedLogger::endTransmitPacket() { 00915 00916 int incoming_byte; 00917 00918 while (xbee().readable()) { 00919 incoming_byte = xbee().getc(); //get first byte 00920 00921 if (incoming_byte == 16) { 00922 incoming_byte = xbee().getc(); //get second byte 00923 return true; 00924 } 00925 //quick and dirty 00926 00927 } 00928 00929 return false; 00930 } 00931 00932 void MbedLogger::reOpenLineReader() { 00933 //open a new one 00934 // string file_name_string = _file_system_string + "LOG000.csv"; 00935 string file_name_string = _file_system_string + configFileIO().logFilesStruct.logFileName; // "DIAG000.txt"; 00936 //string file_name_string = _file_system_string + "LOG000.csv"; // how to pass in log file name as a string? 00937 00938 _fp = fopen(file_name_string.c_str(), "r"); //open the log file to read 00939 00940 //check if this actually worked... 00941 if (!_fp) { 00942 xbee().printf("ERROR: Log file could not be opened\n\r"); 00943 } 00944 else { 00945 xbee().printf("Current Log file (%s) was opened.\n\r", configFileIO().logFilesStruct.logFileName); 00946 } 00947 } 00948 00949 bool MbedLogger::openLineReader() { 00950 // string file_name_string = _file_system_string + "LOG000.csv"; 00951 string file_name_string = _file_system_string + configFileIO().logFilesStruct.logFileName; // "DIAG000.txt"; 00952 //string file_name_string = _file_system_string + "LOG000.csv"; // how to pass in log file name as a string? 00953 00954 00955 _fp = fopen(file_name_string.c_str(), "r"); //open the log file to read 00956 00957 //check if this actually worked... 00958 if (!_fp) { 00959 //xbee().printf("ERROR: Log file could not be opened\n\r"); 00960 return false; 00961 } 00962 else { 00963 //xbee().printf("Current Log file (LOG000.csv) was opened.\n\r"); 00964 return true; 00965 } 00966 } 00967 00968 //VERIFIED THIS IS WORKING 00969 void MbedLogger::readPacketInSeries(){ 00970 memset(&_line_buffer[0], 0, sizeof(_line_buffer)); //clear buffer each time, start at the address, fill with zeroes, go to the end of the char array 00971 00972 //read the current line in the file 00973 fgets(_line_buffer, 256, _fp); //reads the line of characters until you reach a newline character 00974 00975 //RECORD THE STRING LENGTH 00976 _current_line_length = strlen(_line_buffer); 00977 } 00978 00979 int MbedLogger::getNumberOfPacketsInCurrentLog() { 00980 //takes less than a second to complete, verified 7/24/2018 00981 00982 //open the file 00983 // string file_name_string = _file_system_string + "LOG000.csv"; 00984 string file_name_string = _file_system_string + configFileIO().logFilesStruct.logFileName; // "DIAG000.txt"; 00985 //string file_name_string = _file_system_string + "LOG000.csv"; // how to pass in log file name as a string? 00986 00987 _fp = fopen(file_name_string.c_str(), "r"); 00988 00989 fseek(_fp, 0L, SEEK_END); 00990 00991 size_t size = ftell(_fp); 00992 00993 //move the FILE pointer back to the start 00994 fseek(_fp, 0, SEEK_SET); // SEEK_SET is the beginning of file 00995 00996 _total_number_of_packets = size/254; 00997 00998 //CLOSE THE FILE 00999 closeLogFile(); 01000 01001 return _total_number_of_packets; 01002 } 01003 01004 void MbedLogger::endTransmissionCloseFile() { 01005 // if the file pointer is null, the file was not opened in the first place 01006 if (!_fp) { 01007 xbee().printf("\n endTransmissionCloseFile: FILE WAS NOT OPENED!\n\r"); 01008 } 01009 else { 01010 xbee().printf("\n endTransmissionCloseFile: FILE FOUND AND CLOSED!\n\r"); 01011 closeLogFile(); 01012 } 01013 01014 _file_transmission = false; 01015 } 01016 01017 void MbedLogger::openWriteFile() { 01018 xbee().printf("Opening file for reception.\n\r"); 01019 01020 // string file_name_string = _file_system_string + "LOG000.csv"; 01021 string file_name_string = _file_system_string + configFileIO().logFilesStruct.logFileName; // "DIAG000.txt"; 01022 //string file_name_string = _file_system_string + "LOG000.csv"; // how to pass in log file name as a string? 01023 01024 01025 _fp = fopen(file_name_string.c_str(), "w"); 01026 } 01027 01028 01029 //weird bug noticed on 5/25/2018 where if you're not sending data the function is not completing 01030 01031 01032 01033 01034 // function checks for incoming data (receiver function) from a Python program that transmits a file 01035 // current limit is a file that has 255 lines of data 01036 bool MbedLogger::checkForIncomingData() { 01037 int receive_packet_number; 01038 int receive_total_number_packets; 01039 int receive_packet_size; 01040 01041 bool data_transmission_complete = false; 01042 01043 int incoming_byte; 01044 01045 char char_buffer[256] = {}; //create empty buffer 01046 01047 //starting state 01048 int process_state = HEADER_117; 01049 01050 //variables for processing data below 01051 int checksum_one = -1; 01052 int checksum_two = -1; 01053 01054 int i = 5; 01055 int serial_timeout = 0; 01056 01057 while (xbee().readable() && !data_transmission_complete) { 01058 incoming_byte = xbee().getc(); //getc returns an unsigned char cast to an int 01059 //xbee().printf("DEBUG: State 0\n\r"); 01060 01061 switch(process_state) { 01062 case HEADER_117: 01063 //continue processing 01064 if (incoming_byte == 117){ 01065 process_state = HEADER_101; 01066 //xbee().printf("DEBUG: Case 117\n\r"); 01067 } 01068 //end transmission 01069 else if (incoming_byte == 16) { 01070 process_state = END_TRANSMISSION; 01071 //xbee().printf("DEBUG: State 16 (END_TRANSMISSION)\n\r"); 01072 } 01073 else { 01074 process_state = HEADER_117; // ??? 01075 //xbee().printf("DEBUG: State Header 117\n\r"); 01076 } 01077 break; 01078 01079 case HEADER_101: 01080 if(incoming_byte == 101) { 01081 process_state = PACKET_NUM; 01082 //xbee().printf("DEBUG: Case 101\n\r"); 01083 } 01084 break; 01085 01086 case PACKET_NUM: 01087 receive_packet_number = incoming_byte; 01088 process_state = TOTAL_NUM_PACKETS; 01089 //xbee().printf("DEBUG: Case PACKET_NUM\n\r"); 01090 break; 01091 01092 case TOTAL_NUM_PACKETS: 01093 receive_total_number_packets = incoming_byte; 01094 process_state = PACKET_SIZE; 01095 //xbee().printf("DEBUG: Case TOTAL_NUM_PACKETS\n\r"); 01096 break; 01097 01098 case PACKET_SIZE: 01099 receive_packet_size = incoming_byte; 01100 //xbee().printf("DEBUG: Case PACKET_SIZE\n\r"); 01101 01102 //write the header stuff to it 01103 char_buffer[0] = 117; 01104 char_buffer[1] = 101; 01105 char_buffer[2] = receive_packet_number; 01106 char_buffer[3] = receive_total_number_packets; 01107 char_buffer[4] = receive_packet_size; 01108 01109 // tests confirmed that packet number is zero, number of packets is 12, packet size is 12 01110 //xbee().printf("char_buffer 2/3/4: %d %d %d\n\r", receive_packet_number,receive_total_number_packets,receive_packet_size); 01111 01112 //process packet data, future version will append for larger data sizes, 0xFFFF 01113 01114 // IF YOU GET AN INTERRUPTED DATA STREAM YOU NEED TO BREAK OUT OF THE LOOP 01115 i = 5; 01116 serial_timeout = 0; 01117 01118 while (true) { 01119 if (xbee().readable()) { 01120 char_buffer[i] = xbee().getc(); //read all of the data packets 01121 i++; 01122 01123 serial_timeout = 0; //reset the timeout 01124 } 01125 else { 01126 serial_timeout++; 01127 } 01128 01129 // When full data packet is received... 01130 if (i >= receive_packet_size+5) { //cannot do this properly with a for loop 01131 //get checksum bytes 01132 checksum_one = xbee().getc(); 01133 checksum_two = xbee().getc(); 01134 01135 //calculate the CRC from the header and data bytes using _data_packet (vector) 01136 //found out calculating crc with string was dropping empty or null spaces 01137 _data_packet.clear(); //clear it just in case 01138 01139 for (int a = 0; a < receive_packet_size+5; a++) { 01140 _data_packet.push_back(char_buffer[a]); //push the character array into the buffer 01141 } 01142 01143 //calculate the CRC using the vector (strings will cut off null characters) 01144 int calc_crc_one = calcCrcOne(); 01145 int calc_crc_two = calcCrcTwo(); 01146 01147 //xbee().printf("DEBUG: calc crc 1: %d, crc 2: %d\n\r", calc_crc_one, calc_crc_two); 01148 01149 // first confirm that the checksum is correct 01150 if ((calc_crc_one == checksum_one) and (calc_crc_two == checksum_two)) { 01151 01152 //xbee().printf("DEBUG: checksums are good!\n\r"); 01153 01154 //xbee().printf("receive_packet_number %d and _confirmed_packet_number %d\n\r", receive_packet_number, _confirmed_packet_number); //debug 01155 01156 // // CHECKSUM CORRECT & get the filename from the first packet (check that you receive first packet) 01157 // if (receive_packet_number == 0) { 01158 // char temp_char[receive_packet_size+1]; //temp array for memcpy 01159 // memset(&temp_char[0], 0, sizeof(temp_char)); //clear full array (or get random characters) 01160 // strncpy(temp_char, char_buffer + 5 /* Offset */, receive_packet_size*sizeof(char) /* Length */); //memcpy introduced random characters 01161 // 01162 // //xbee().printf("SEQUENCE: <<%s>>\n\r", temp_char]) // ERASE 01163 // 01164 // //have to terminate the string with '\0' 01165 // temp_char[receive_packet_size] = '\0'; 01166 // 01167 // //xbee().printf("\n\rDEBUG: ------ Filename? <<%s>>\n\r", temp_char); 01168 // //_received_filename = temp_char; 01169 // 01170 // //xbee().printf("\n\rDEBUG: _received_filename <<%s>>\n\r", _received_filename); 01171 // 01172 // //open a file for writing 01173 // //openReceiveFile(_received_filename); 01174 // 01175 // //send a reply to Python transmit program 01176 // sendReply(); 01177 // 01178 // led3() = 1; 01179 // 01180 // // even if correct CRC, counter prevents the program from writing the same packet twice 01181 // _confirmed_packet_number++; 01182 // } 01183 01184 // check if the packet that you're receiving (receive_packet_number) has been received already... 01185 01186 //CHECKSUM CORRECT & packet numbers that are 1 through N packets 01187 if (receive_packet_number == _confirmed_packet_number){ 01188 //save the data (char buffer) to the file if both checksums work... 01189 01190 // when a packet is received (successfully) send a reply 01191 //sendReply(); 01192 01193 // write correct data to file 01194 fprintf(_fp, "%s", char_buffer+5); 01195 01196 // even if correct CRC, counter prevents the program from writing the same packet twice 01197 _confirmed_packet_number++; 01198 } 01199 01200 //clear the variables 01201 checksum_one = -1; 01202 checksum_two = -1; 01203 } 01204 01205 process_state = HEADER_117; 01206 01207 break; 01208 } 01209 01210 // //counter breaks out of the loop if no data received 01211 // if (serial_timeout >= 10000) { 01212 // //xbee().printf("break serial_timeout %d\n\r", serial_timeout); 01213 // break; 01214 // } 01215 } 01216 break; 01217 01218 case END_TRANSMISSION: 01219 if (xbee().getc() == 16) { 01220 //xbee().printf("DEBUG: END_TRANSMISSION REACHED: 1. \n\r"); 01221 01222 if (xbee().getc() == 16) { 01223 //xbee().printf("DEBUG: END_TRANSMISSION REACHED: 2. \n\r"); 01224 01225 _end_sequence_transmission = true; 01226 //endReceiveData(); 01227 } 01228 } 01229 01230 //xbee().printf("DEBUG: END_TRANSMISSION REACHED: 5. \n\r"); 01231 01232 //process_state = HEADER_117; //don't do this unless the check is wrong 01233 //xbee().printf("END_TRANSMISSION process_state is %d\n\r", process_state); //should be 5 (debug) 02/06/2018 01234 data_transmission_complete = true; 01235 break; 01236 }//END OF SWITCH 01237 01238 if (data_transmission_complete) { 01239 //xbee().printf("DEBUG: checkForIncomingData data_transmission_complete \n\r"); 01240 break; //out of while loop 01241 } 01242 } // while loop 01243 01244 led3() = !led3(); 01245 01246 if (data_transmission_complete) 01247 return false; //tell state machine class that this is done (not transmitting, false) 01248 else { 01249 return true; 01250 } 01251 } 01252 01253 int MbedLogger::sendReply() { 01254 //being explicit in what's being transmitted 01255 01256 //change this method to be more explicit later 01257 01258 //integer vector _data_packet is used here, cleared fist just in case 01259 01260 _data_packet.clear(); //same data packet for transmission 01261 _data_packet.push_back(117); 01262 _data_packet.push_back(101); 01263 01264 //_confirmed_packet_number comes from the packet number that is sent from the Python program 01265 _data_packet.push_back(_confirmed_packet_number / 256); //packet number only changed when confirmed 01266 _data_packet.push_back(_confirmed_packet_number % 256); //split into first and second byte 01267 01268 //compute checksums 01269 01270 int receiver_crc_one = calcCrcOne(); 01271 int receiver_crc_two = calcCrcTwo(); 01272 01273 _data_packet.push_back(receiver_crc_one); 01274 _data_packet.push_back(receiver_crc_two); 01275 01276 //transmit this packet 01277 for (_it=_data_packet.begin(); _it < _data_packet.end(); _it++) { 01278 xbee().putc(*_it); //send integers over serial port one byte at a time 01279 } 01280 01281 //change process methodology later... 01282 01283 return _confirmed_packet_number; 01284 } 01285 01286 void MbedLogger::endReceiveData() { //DLE character * 4 ==> 10 10 10 10 01287 closeLogFile(); //close the file here 01288 xbee().printf("endReceiveData closed the file and ended transmission\n\r"); 01289 } 01290 01291 //calculate the crc with an integer array 01292 int MbedLogger::calcCrcOneArray(int *input_array, int array_length) { 01293 //can't initialize the table in the constructor in c++ 01294 int crc_table [256] = {0, 49345, 49537, 320, 49921, 960, 640, 49729, 50689, 1728, 1920, 51009, 1280, 50625, 50305, 1088, 52225, 3264, 3456, 52545, 3840, 53185, 52865, 3648, 2560, 51905, 52097, 2880, 51457, 2496, 2176, 51265, 55297, 6336, 6528, 55617, 6912, 56257, 55937, 6720, 7680, 57025, 57217, 8000, 56577, 7616, 7296, 56385, 5120, 54465, 54657, 5440, 55041, 6080, 5760, 54849, 53761, 4800, 4992, 54081, 4352, 53697, 53377, 4160, 61441, 12480, 12672, 61761, 13056, 62401, 62081, 12864, 13824, 63169, 63361, 14144, 62721, 13760, 13440, 62529, 15360, 64705, 64897, 15680, 65281, 16320, 16000, 65089, 64001, 15040, 15232, 64321, 14592, 63937, 63617, 14400, 10240, 59585, 59777, 10560, 60161, 11200, 10880, 59969, 60929, 11968, 12160, 61249, 11520, 60865, 60545, 11328, 58369, 9408, 9600, 58689, 9984, 59329, 59009, 9792, 8704, 58049, 58241, 9024, 57601, 8640, 8320, 57409, 40961, 24768, 24960, 41281, 25344, 41921, 41601, 25152, 26112, 42689, 42881, 26432, 42241, 26048, 25728, 42049, 27648, 44225, 44417, 27968, 44801, 28608, 28288, 44609, 43521, 27328, 27520, 43841, 26880, 43457, 43137, 26688, 30720, 47297, 47489, 31040, 47873, 31680, 31360, 47681, 48641, 32448, 32640, 48961, 32000, 48577, 48257, 31808, 46081, 29888, 30080, 46401, 30464, 47041, 46721, 30272, 29184, 45761, 45953, 29504, 45313, 29120, 28800, 45121, 20480, 37057, 37249, 20800, 37633, 21440, 21120, 37441, 38401, 22208, 22400, 38721, 21760, 38337, 38017, 21568, 39937, 23744, 23936, 40257, 24320, 40897, 40577, 24128, 23040, 39617, 39809, 23360, 39169, 22976, 22656, 38977, 34817, 18624, 18816, 35137, 19200, 35777, 35457, 19008, 19968, 36545, 36737, 20288, 36097, 19904, 19584, 35905, 17408, 33985, 34177, 17728, 34561, 18368, 18048, 34369, 33281, 17088, 17280, 33601, 16640, 33217, 32897, 16448}; 01295 int crc = 0; 01296 for (int z = 0; z < array_length; z++) { 01297 crc = (crc_table[(input_array[z] ^ crc) & 0xff] ^ (crc >> 8)) & 0xFFFF; 01298 } 01299 return crc / 256; //second-to-last byte 01300 } 01301 01302 int MbedLogger::calcCrcTwoArray(int *input_array, int array_length) { 01303 //can't initialize the table in the constructor in c++ 01304 int crc_table [256] = {0, 49345, 49537, 320, 49921, 960, 640, 49729, 50689, 1728, 1920, 51009, 1280, 50625, 50305, 1088, 52225, 3264, 3456, 52545, 3840, 53185, 52865, 3648, 2560, 51905, 52097, 2880, 51457, 2496, 2176, 51265, 55297, 6336, 6528, 55617, 6912, 56257, 55937, 6720, 7680, 57025, 57217, 8000, 56577, 7616, 7296, 56385, 5120, 54465, 54657, 5440, 55041, 6080, 5760, 54849, 53761, 4800, 4992, 54081, 4352, 53697, 53377, 4160, 61441, 12480, 12672, 61761, 13056, 62401, 62081, 12864, 13824, 63169, 63361, 14144, 62721, 13760, 13440, 62529, 15360, 64705, 64897, 15680, 65281, 16320, 16000, 65089, 64001, 15040, 15232, 64321, 14592, 63937, 63617, 14400, 10240, 59585, 59777, 10560, 60161, 11200, 10880, 59969, 60929, 11968, 12160, 61249, 11520, 60865, 60545, 11328, 58369, 9408, 9600, 58689, 9984, 59329, 59009, 9792, 8704, 58049, 58241, 9024, 57601, 8640, 8320, 57409, 40961, 24768, 24960, 41281, 25344, 41921, 41601, 25152, 26112, 42689, 42881, 26432, 42241, 26048, 25728, 42049, 27648, 44225, 44417, 27968, 44801, 28608, 28288, 44609, 43521, 27328, 27520, 43841, 26880, 43457, 43137, 26688, 30720, 47297, 47489, 31040, 47873, 31680, 31360, 47681, 48641, 32448, 32640, 48961, 32000, 48577, 48257, 31808, 46081, 29888, 30080, 46401, 30464, 47041, 46721, 30272, 29184, 45761, 45953, 29504, 45313, 29120, 28800, 45121, 20480, 37057, 37249, 20800, 37633, 21440, 21120, 37441, 38401, 22208, 22400, 38721, 21760, 38337, 38017, 21568, 39937, 23744, 23936, 40257, 24320, 40897, 40577, 24128, 23040, 39617, 39809, 23360, 39169, 22976, 22656, 38977, 34817, 18624, 18816, 35137, 19200, 35777, 35457, 19008, 19968, 36545, 36737, 20288, 36097, 19904, 19584, 35905, 17408, 33985, 34177, 17728, 34561, 18368, 18048, 34369, 33281, 17088, 17280, 33601, 16640, 33217, 32897, 16448}; 01305 int crc = 0; 01306 for (int z = 0; z < array_length; z++) { 01307 crc = (crc_table[(input_array[z] ^ crc) & 0xff] ^ (crc >> 8)) & 0xFFFF; 01308 } 01309 return crc % 256; //second-to-last byte 01310 } 01311 01312 int MbedLogger::calcCrcOne() { 01313 //can't initialize the table in the constructor in c++ 01314 int crc_table [256] = {0, 49345, 49537, 320, 49921, 960, 640, 49729, 50689, 1728, 1920, 51009, 1280, 50625, 50305, 1088, 52225, 3264, 3456, 52545, 3840, 53185, 52865, 3648, 2560, 51905, 52097, 2880, 51457, 2496, 2176, 51265, 55297, 6336, 6528, 55617, 6912, 56257, 55937, 6720, 7680, 57025, 57217, 8000, 56577, 7616, 7296, 56385, 5120, 54465, 54657, 5440, 55041, 6080, 5760, 54849, 53761, 4800, 4992, 54081, 4352, 53697, 53377, 4160, 61441, 12480, 12672, 61761, 13056, 62401, 62081, 12864, 13824, 63169, 63361, 14144, 62721, 13760, 13440, 62529, 15360, 64705, 64897, 15680, 65281, 16320, 16000, 65089, 64001, 15040, 15232, 64321, 14592, 63937, 63617, 14400, 10240, 59585, 59777, 10560, 60161, 11200, 10880, 59969, 60929, 11968, 12160, 61249, 11520, 60865, 60545, 11328, 58369, 9408, 9600, 58689, 9984, 59329, 59009, 9792, 8704, 58049, 58241, 9024, 57601, 8640, 8320, 57409, 40961, 24768, 24960, 41281, 25344, 41921, 41601, 25152, 26112, 42689, 42881, 26432, 42241, 26048, 25728, 42049, 27648, 44225, 44417, 27968, 44801, 28608, 28288, 44609, 43521, 27328, 27520, 43841, 26880, 43457, 43137, 26688, 30720, 47297, 47489, 31040, 47873, 31680, 31360, 47681, 48641, 32448, 32640, 48961, 32000, 48577, 48257, 31808, 46081, 29888, 30080, 46401, 30464, 47041, 46721, 30272, 29184, 45761, 45953, 29504, 45313, 29120, 28800, 45121, 20480, 37057, 37249, 20800, 37633, 21440, 21120, 37441, 38401, 22208, 22400, 38721, 21760, 38337, 38017, 21568, 39937, 23744, 23936, 40257, 24320, 40897, 40577, 24128, 23040, 39617, 39809, 23360, 39169, 22976, 22656, 38977, 34817, 18624, 18816, 35137, 19200, 35777, 35457, 19008, 19968, 36545, 36737, 20288, 36097, 19904, 19584, 35905, 17408, 33985, 34177, 17728, 34561, 18368, 18048, 34369, 33281, 17088, 17280, 33601, 16640, 33217, 32897, 16448}; 01315 01316 int crc = 0; 01317 for (_it=_data_packet.begin(); _it < _data_packet.end(); _it++) 01318 crc = (crc_table[(*_it ^ crc) & 0xff] ^ (crc >> 8)) & 0xFFFF; 01319 01320 return crc / 256; //second-to-last byte 01321 } 01322 01323 int MbedLogger::calcCrcTwo() { 01324 int crc_table [256] = {0, 49345, 49537, 320, 49921, 960, 640, 49729, 50689, 1728, 1920, 51009, 1280, 50625, 50305, 1088, 52225, 3264, 3456, 52545, 3840, 53185, 52865, 3648, 2560, 51905, 52097, 2880, 51457, 2496, 2176, 51265, 55297, 6336, 6528, 55617, 6912, 56257, 55937, 6720, 7680, 57025, 57217, 8000, 56577, 7616, 7296, 56385, 5120, 54465, 54657, 5440, 55041, 6080, 5760, 54849, 53761, 4800, 4992, 54081, 4352, 53697, 53377, 4160, 61441, 12480, 12672, 61761, 13056, 62401, 62081, 12864, 13824, 63169, 63361, 14144, 62721, 13760, 13440, 62529, 15360, 64705, 64897, 15680, 65281, 16320, 16000, 65089, 64001, 15040, 15232, 64321, 14592, 63937, 63617, 14400, 10240, 59585, 59777, 10560, 60161, 11200, 10880, 59969, 60929, 11968, 12160, 61249, 11520, 60865, 60545, 11328, 58369, 9408, 9600, 58689, 9984, 59329, 59009, 9792, 8704, 58049, 58241, 9024, 57601, 8640, 8320, 57409, 40961, 24768, 24960, 41281, 25344, 41921, 41601, 25152, 26112, 42689, 42881, 26432, 42241, 26048, 25728, 42049, 27648, 44225, 44417, 27968, 44801, 28608, 28288, 44609, 43521, 27328, 27520, 43841, 26880, 43457, 43137, 26688, 30720, 47297, 47489, 31040, 47873, 31680, 31360, 47681, 48641, 32448, 32640, 48961, 32000, 48577, 48257, 31808, 46081, 29888, 30080, 46401, 30464, 47041, 46721, 30272, 29184, 45761, 45953, 29504, 45313, 29120, 28800, 45121, 20480, 37057, 37249, 20800, 37633, 21440, 21120, 37441, 38401, 22208, 22400, 38721, 21760, 38337, 38017, 21568, 39937, 23744, 23936, 40257, 24320, 40897, 40577, 24128, 23040, 39617, 39809, 23360, 39169, 22976, 22656, 38977, 34817, 18624, 18816, 35137, 19200, 35777, 35457, 19008, 19968, 36545, 36737, 20288, 36097, 19904, 19584, 35905, 17408, 33985, 34177, 17728, 34561, 18368, 18048, 34369, 33281, 17088, 17280, 33601, 16640, 33217, 32897, 16448}; 01325 01326 int crc = 0; 01327 for (_it=_data_packet.begin(); _it < _data_packet.end(); _it++) 01328 crc = (crc_table[(*_it ^ crc) & 0xff] ^ (crc >> 8)) & 0xFFFF; 01329 01330 //xbee().printf("DEBUG: calcCrcTwo string length: %d crc: %d\n\r", input_array.length(), crc % 256); 01331 01332 return crc % 256; //last byte 01333 } 01334 01335 void MbedLogger::resetReplyPacket() { 01336 _confirmed_packet_number = 0; 01337 } 01338 01339 void MbedLogger::openNewMissionFile() { 01340 xbee().printf("Opening Mission file (sequence.txt) for reception.\n\r"); 01341 string filename_string = _file_system_string + "sequence.txt"; 01342 01343 xbee().printf("openNewMissionFile: %s\n\r", filename_string.c_str()); 01344 01345 _fp = fopen(filename_string.c_str(), "w"); 01346 } 01347 01348 void MbedLogger::openReceiveFile(string filename) { 01349 string filename_string = _file_system_string + filename; //example "sequence.txt" 01350 01351 _fp = fopen(filename_string.c_str(), "w"); //open a file for writing 01352 } 01353 01354 void MbedLogger::setDataCounter(int input_counter) { 01355 _transmit_counter = input_counter; 01356 } 01357 01358 void MbedLogger::closeIncompleteFile() { 01359 fprintf(_fp, "TRANSMISSION INTERRUPTED!"); //write this warning to the file 01360 closeLogFile(); //close file 01361 } 01362 01363 void MbedLogger::appendLogFile(int current_state, int option) { 01364 //option one means write to file 01365 01366 if (option >= 1) { 01367 if (!_fp) { //if not present 01368 _fp = fopen(_full_file_path_string.c_str(), "a"); 01369 } 01370 01371 //record data using the recordData function (takes in the state integer) 01372 if(option ==2) {recordData_long(current_state);} 01373 recordData_short(current_state); 01374 } 01375 01376 else { //option==0 just close log file 01377 closeLogFile(); 01378 } 01379 } 01380 void MbedLogger::appendDiagFile(char *printf_string, int flushclose) { 01381 // 01382 01383 int start_time = 1518467832; 01384 if(_time_set) { start_time = _default_timestamp_time;} 01385 //in the future create the ability to set the start time 01386 01387 int time_now = mbedLogger().getSystemTime(); 01388 if (!_fp2) { //if not present 01389 _fp2 = fopen(_full_diagfile_path_string.c_str(), "a"); 01390 } 01391 int del_time = time_now - start_time; 01392 //record data using the recordData function (takes in the state integer) 01393 fprintf(_fp2, "time=%d seconds ", del_time); 01394 fprintf(_fp2, printf_string); 01395 if(flushclose == 1) { fflush(_fp2); } 01396 if(flushclose == 0) {fclose(_fp2); _fp2 = NULL; } 01397 } 01398 01399 // initialize and close the file 01400 // log file freezes at 0x0000006c 01401 void MbedLogger::initializeLogFile() { 01402 string file_name_string = _file_system_string + configFileIO().logFilesStruct.logFileName; // "DIAG000.txt"; 01403 //string file_name_string = _file_system_string + "LOG000.csv"; // how to pass in log file name as a string? 01404 _full_file_path_string = file_name_string; 01405 char buf[256]; 01406 xbee().printf("%s file system init\n\r", _file_system_string.c_str()); 01407 sprintf(buf, "%s mbedlogger():initializelogfile: file system init\n\r", _file_system_string.c_str()); 01408 appendDiagFile(buf,1); 01409 //try to open this file... 01410 _fp = fopen(file_name_string.c_str(), "r"); 01411 01412 //if the file is empty, create this. 01413 if (!_fp) { 01414 _fp = fopen(file_name_string.c_str(), "w"); //write,print,close 01415 //fprintf(_fp,"state_string,state_ID,timer,depth_cmd,depth_ft,pitch_cmd,pitch_deg,bce_cmd,bce_mm,batt_cmd,batt_mm,pitchRate_degs,depthRate_fps\nempty log file!\n"); 01416 fprintf(_fp,_heading_string.c_str()); 01417 fprintf(_fp,_heading_string2.c_str()); 01418 closeLogFile(); 01419 } 01420 else 01421 closeLogFile(); //close the opened read file 01422 } 01423 void MbedLogger::initializeDiagFile(int print_diag) { 01424 //string file_name_string = _file_system_string + "DIAG000.txt"; // how to pass in log file name as a string? 01425 string file_name_string = _file_system_string + configFileIO().logFilesStruct.diagFileName; // "DIAG000.txt"; 01426 _full_diagfile_path_string = file_name_string; 01427 char buf[256]; 01428 xbee().printf("%s file system init\n\r", _file_system_string.c_str()); 01429 sprintf(buf, "mbedlogger():Initializediagfile input variable diagfilename: %s file system init\n\r", file_name_string.c_str()); 01430 if(print_diag == 1) {appendDiagFile(buf,3);} //configFileIO().logFilesStruct.diagFileName 01431 //try to open this file... 01432 if( _fp2 == NULL) { _fp2 = fopen(file_name_string.c_str(), "r"); } 01433 01434 //if the file is empty, create this. 01435 if (!_fp2) { 01436 _fp2 = fopen(file_name_string.c_str(), "w"); //write,print,close 01437 //fprintf(_fp,"state_string,state_ID,timer,depth_cmd,depth_ft,pitch_cmd,pitch_deg,bce_cmd,bce_mm,batt_cmd,batt_mm,pitchRate_degs,depthRate_fps\nempty log file!\n"); 01438 fprintf(_fp2,_diag_heading_string.c_str()); 01439 closeDiagFile(); 01440 } 01441 else 01442 closeDiagFile(); //close the opened read file 01443 } 01444 01445 int MbedLogger::fileTransmitState() { 01446 return _file_transmission_state; 01447 } 01448 01449 int MbedLogger::calcCrcOneString (string input_string) { 01450 int crc_table [256] = {0, 49345, 49537, 320, 49921, 960, 640, 49729, 50689, 1728, 1920, 51009, 1280, 50625, 50305, 1088, 52225, 3264, 3456, 52545, 3840, 53185, 52865, 3648, 2560, 51905, 52097, 2880, 51457, 2496, 2176, 51265, 55297, 6336, 6528, 55617, 6912, 56257, 55937, 6720, 7680, 57025, 57217, 8000, 56577, 7616, 7296, 56385, 5120, 54465, 54657, 5440, 55041, 6080, 5760, 54849, 53761, 4800, 4992, 54081, 4352, 53697, 53377, 4160, 61441, 12480, 12672, 61761, 13056, 62401, 62081, 12864, 13824, 63169, 63361, 14144, 62721, 13760, 13440, 62529, 15360, 64705, 64897, 15680, 65281, 16320, 16000, 65089, 64001, 15040, 15232, 64321, 14592, 63937, 63617, 14400, 10240, 59585, 59777, 10560, 60161, 11200, 10880, 59969, 60929, 11968, 12160, 61249, 11520, 60865, 60545, 11328, 58369, 9408, 9600, 58689, 9984, 59329, 59009, 9792, 8704, 58049, 58241, 9024, 57601, 8640, 8320, 57409, 40961, 24768, 24960, 41281, 25344, 41921, 41601, 25152, 26112, 42689, 42881, 26432, 42241, 26048, 25728, 42049, 27648, 44225, 44417, 27968, 44801, 28608, 28288, 44609, 43521, 27328, 27520, 43841, 26880, 43457, 43137, 26688, 30720, 47297, 47489, 31040, 47873, 31680, 31360, 47681, 48641, 32448, 32640, 48961, 32000, 48577, 48257, 31808, 46081, 29888, 30080, 46401, 30464, 47041, 46721, 30272, 29184, 45761, 45953, 29504, 45313, 29120, 28800, 45121, 20480, 37057, 37249, 20800, 37633, 21440, 21120, 37441, 38401, 22208, 22400, 38721, 21760, 38337, 38017, 21568, 39937, 23744, 23936, 40257, 24320, 40897, 40577, 24128, 23040, 39617, 39809, 23360, 39169, 22976, 22656, 38977, 34817, 18624, 18816, 35137, 19200, 35777, 35457, 19008, 19968, 36545, 36737, 20288, 36097, 19904, 19584, 35905, 17408, 33985, 34177, 17728, 34561, 18368, 18048, 34369, 33281, 17088, 17280, 33601, 16640, 33217, 32897, 16448}; 01451 01452 int crc = 0; 01453 for (unsigned int i = 0; i < input_string.length(); i++) { 01454 //convert each character to an integer 01455 int character_to_integer = input_string[i]; //correct 01456 01457 crc = (crc_table[(character_to_integer ^ crc) & 0xff] ^ (crc >> 8)) & 0xFFFF; 01458 } 01459 01460 //xbee().printf("DEBUG: calcCrcOne string length: %d crc: %d\n\r", input_string.length(), crc/256); 01461 01462 return crc / 256; //second-to-last byte 01463 } 01464 01465 int MbedLogger::calcCrcTwoString (string input_string) { 01466 int crc_table [256] = {0, 49345, 49537, 320, 49921, 960, 640, 49729, 50689, 1728, 1920, 51009, 1280, 50625, 50305, 1088, 52225, 3264, 3456, 52545, 3840, 53185, 52865, 3648, 2560, 51905, 52097, 2880, 51457, 2496, 2176, 51265, 55297, 6336, 6528, 55617, 6912, 56257, 55937, 6720, 7680, 57025, 57217, 8000, 56577, 7616, 7296, 56385, 5120, 54465, 54657, 5440, 55041, 6080, 5760, 54849, 53761, 4800, 4992, 54081, 4352, 53697, 53377, 4160, 61441, 12480, 12672, 61761, 13056, 62401, 62081, 12864, 13824, 63169, 63361, 14144, 62721, 13760, 13440, 62529, 15360, 64705, 64897, 15680, 65281, 16320, 16000, 65089, 64001, 15040, 15232, 64321, 14592, 63937, 63617, 14400, 10240, 59585, 59777, 10560, 60161, 11200, 10880, 59969, 60929, 11968, 12160, 61249, 11520, 60865, 60545, 11328, 58369, 9408, 9600, 58689, 9984, 59329, 59009, 9792, 8704, 58049, 58241, 9024, 57601, 8640, 8320, 57409, 40961, 24768, 24960, 41281, 25344, 41921, 41601, 25152, 26112, 42689, 42881, 26432, 42241, 26048, 25728, 42049, 27648, 44225, 44417, 27968, 44801, 28608, 28288, 44609, 43521, 27328, 27520, 43841, 26880, 43457, 43137, 26688, 30720, 47297, 47489, 31040, 47873, 31680, 31360, 47681, 48641, 32448, 32640, 48961, 32000, 48577, 48257, 31808, 46081, 29888, 30080, 46401, 30464, 47041, 46721, 30272, 29184, 45761, 45953, 29504, 45313, 29120, 28800, 45121, 20480, 37057, 37249, 20800, 37633, 21440, 21120, 37441, 38401, 22208, 22400, 38721, 21760, 38337, 38017, 21568, 39937, 23744, 23936, 40257, 24320, 40897, 40577, 24128, 23040, 39617, 39809, 23360, 39169, 22976, 22656, 38977, 34817, 18624, 18816, 35137, 19200, 35777, 35457, 19008, 19968, 36545, 36737, 20288, 36097, 19904, 19584, 35905, 17408, 33985, 34177, 17728, 34561, 18368, 18048, 34369, 33281, 17088, 17280, 33601, 16640, 33217, 32897, 16448}; 01467 01468 int crc = 0; 01469 for (unsigned int i = 0; i < input_string.length(); i++) { 01470 //convert each character to an integer 01471 int character_to_integer = input_string[i]; //correct 01472 01473 crc = (crc_table[(character_to_integer ^ crc) & 0xff] ^ (crc >> 8)) & 0xFFFF; 01474 } 01475 01476 //xbee().printf("DEBUG: calcCrcTwo string length: %d crc: %d\n\r", input_string.length(), crc % 256); 01477 01478 return crc % 256; //last byte 01479 } 01480 01481 void MbedLogger::createEmptyLog() { 01482 // string file_name_string = _file_system_string + "LOG000.csv"; 01483 string file_name_string = _file_system_string + configFileIO().logFilesStruct.logFileName; // "DIAG000.txt"; 01484 //string file_name_string = _file_system_string + "LOG000.csv"; // how to pass in log file name as a string? 01485 string empty_log = "EMPTY LOG"; 01486 01487 _fp = fopen(file_name_string.c_str(), "w"); 01488 01489 fprintf(_fp, "%.25s\n",empty_log.c_str()); //just write this string to the log (processing needs a file size that is not zero) 01490 closeLogFile(); 01491 } 01492 01493 int MbedLogger::getFileSize(string filename) { 01494 // fixed the const char * errror: 01495 // https://stackoverflow.com/questions/347949/how-to-convert-a-stdstring-to-const-char-or-char 01496 const char * char_filename = filename.c_str(); // Returns a pointer to an array that contains a null-terminated sequence of characters (i.e., a C-string) representing the current value of the string object. 01497 //http://www.cplusplus.com/reference/string/string/c_str/ 01498 01499 _fp = fopen(filename.c_str(), "rb"); //open the file for reading as a binary file 01500 01501 fseek(_fp, 0, SEEK_END); //SEEK_END is a constant in cstdio (end of the file) 01502 unsigned int file_size = ftell(_fp); //For binary streams, this is the number of bytes from the beginning of the file. 01503 fseek(_fp, 0, SEEK_SET); //SEEK_SET is hte beginning of the file, not sure this is necessary 01504 01505 xbee().printf("%s file size is %d bytes.\n\r", filename.c_str(), file_size); 01506 01507 closeLogFile(); //can probably just close the file pointer and not worry about position 01508 01509 return file_size; 01510 } 01511 01512 int MbedLogger::debugFileState() { 01513 xbee().printf("What is _fp right now? %p\n\r", _fp); //pointer notation 01514 01515 if (_fp) 01516 return 1; //file pointer does exist 01517 else 01518 return 0; //file pointer does not exist 01519 } 01520 01521 void MbedLogger::specifyFileForTransmit(string input_string) { 01522 xbee().printf("specifyFileForTransmit\n\r"); 01523 01524 string file_string = _file_system_string + input_string; 01525 01526 xbee().printf("file_string is <%s>\n\r", file_string.c_str()); 01527 01528 //open this file to read 01529 _fp = fopen(file_string.c_str(), "r"); 01530 01531 //transmit that file 01532 //transmitDataWithTicker(); //replaced ticker 01533 01534 _file_transmission = true; 01535 } 01536 01537 void MbedLogger::transmitFileFromDirectory( int file_number ) { 01538 file_number = file_number + 1; //to get the correct number 01539 01540 DIR *dir; 01541 struct dirent *dp; //dirent.h is the format of directory entries 01542 int log_found =0, loop=1; //start file numbers at 1 01543 long int temp=0; 01544 01545 // char * char_pointer; 01546 // char * numstart, *numstop; 01547 01548 if ( NULL == (dir = opendir( _file_system_string.c_str() )) ) { 01549 xbee().printf("MBED directory could not be opened\r\n"); 01550 } 01551 else 01552 { 01553 while ( NULL != (dp = readdir( dir )) ) 01554 { 01555 xbee().printf( "%d. %s (log file: %d, %d)\r\n", loop, dp->d_name,log_found,temp); 01556 01557 //process current file if it matches the file number 01558 if (file_number == loop) { 01559 char * current_file_name = dp->d_name; //pointer to this char array 01560 01561 specifyFileForTransmit(current_file_name); 01562 01563 break; 01564 } 01565 01566 loop++; 01567 } 01568 } 01569 } 01570 01571 void MbedLogger::accessMbedDirectory() { 01572 printMbedDirectory(); 01573 01574 xbee().printf("Type in the number of the file you want to transmit.\n\r"); 01575 01576 char message[42]; 01577 01578 xbee().scanf("%41s", message); 01579 01580 xbee().printf("Input received!\n\r"); 01581 01582 //check if char array is an integer 01583 char* conversion_pointer; 01584 long converted = strtol(message, &conversion_pointer, 10); 01585 01586 if (*conversion_pointer) { 01587 //conversion failed because the input was not a number 01588 xbee().printf("NOT A VALID FILE NUMBER!\n\r"); 01589 } 01590 else { 01591 //conversion worked! 01592 xbee().printf("You chose file number: %d\n\r", converted); 01593 01594 // transmit the file 01595 transmitFileFromDirectory(converted); 01596 } 01597 } 01598 01599 void MbedLogger::closeLogFile() { 01600 led4() = 1; 01601 01602 if (_fp == NULL){ 01603 xbee().printf("MbedLogger: (%s) LOG FILE WAS ALREADY CLOSED!\n\r", _file_system_string.c_str()); 01604 } 01605 01606 else { 01607 xbee().printf("MbedLogger: (%s) CLOSING LOG FILE!\n\r", _file_system_string.c_str()); 01608 01609 //close file 01610 fclose(_fp); 01611 01612 _fp = NULL; //set pointer to zero 01613 } 01614 } 01615 void MbedLogger::closeDiagFile() { // closes he present diag file on _fp2 01616 led4() = 1; 01617 01618 if (_fp2 == NULL){ 01619 xbee().printf("MbedLogger: (%s) DIAG FILE WAS ALREADY CLOSED!\n\r", _full_diagfile_path_string.c_str()); 01620 } 01621 01622 else { 01623 xbee().printf("MbedLogger: (%s) CLOSING LOG FILE!\n\r", _full_diagfile_path_string.c_str()); 01624 01625 //close file 01626 fclose(_fp2); 01627 01628 _fp2 = NULL; //set pointer to zero 01629 } 01630 } 01631 01632 void MbedLogger::activateReceivePacket() { 01633 _mbed_receive_loop = true; 01634 } 01635 01636 void MbedLogger::receiveSequenceFile() { 01637 //restart each time 01638 _end_sequence_transmission = false; 01639 01640 openNewMissionFile(); 01641 01642 //zero will be reserved for the file name, future 01643 _confirmed_packet_number = 1; //in sendReply() function that transmits a reply for incoming data 01644 01645 // int current_packet_number = 1; 01646 // int last_packet_number = -1; 01647 // int break_transmission = 0; 01648 01649 int counter = 0; 01650 01651 while(1) { 01652 wait(0.25); //runs at 4 Hz 01653 01654 checkForIncomingData(); 01655 01656 //xbee().printf("\n\rDEBUG: _confirmed_packet_number%d\n\r", _confirmed_packet_number); 01657 01658 counter++; 01659 01660 sendReply(); //bad name, should call it send request or something 01661 01662 if (_end_sequence_transmission) 01663 break; 01664 } 01665 01666 closeLogFile(); 01667 } 01668 01669 void MbedLogger::receiveMissionDataWithFSM() { 01670 checkForIncomingData(); 01671 01672 // Example: send reply 75 65 00 00 01673 // Example: send reply 75 65 00 01 01674 } 01675 01676 void MbedLogger::receiveMissionDataWithTicker() { 01677 openNewMissionFile(); //sequence.txt file opened 01678 01679 _mbed_receive_ticker.attach(callback(this, &MbedLogger::activateReceivePacket), 0.5); 01680 01681 xbee().printf("\n\r02/09/2018 MbedLogger receiveMissionData Beginning to receive sequence data...\n\r"); 01682 01683 resetReplyPacket(); //reset the reply packet 01684 01685 //idea for stopping this if data not being received 01686 int current_packet_number = 0; 01687 int last_packet_number = -1; 01688 int break_transmission = 0; 01689 01690 while(1) { 01691 //runs at 10 hz 01692 if (_mbed_receive_loop) { 01693 if (!checkForIncomingData()) { // run this until it finishes 01694 //when you complete data reception, this will become false 01695 xbee().printf("\n\rMbedLogger: Data RECEPTION complete.\n\r"); 01696 _mbed_receive_ticker.detach(); 01697 break; 01698 } 01699 else { 01700 01701 // SEND REPLY 75 65 00 00 01702 // SEND REPLY 75 65 00 01 01703 01704 //check if you keep getting the same thing 01705 current_packet_number = sendReply(); 01706 01707 //xbee().printf("DEBUG: current packet number %d (last packet number %d) \n\r", current_packet_number, last_packet_number); //debug 01708 01709 //let this count up a few times before it exits 01710 if (current_packet_number == last_packet_number) { 01711 break_transmission++; 01712 01713 //break transmission after 50 failed attempts (was 100) 01714 if (break_transmission >= 50) { 01715 closeIncompleteFile(); //close the file 01716 _mbed_receive_ticker.detach(); 01717 xbee().printf("MbedLogger: TRANSMISSION INTERRUPTED!\n\r"); 01718 break; 01719 } 01720 } 01721 else 01722 last_packet_number = current_packet_number; 01723 } 01724 _mbed_receive_loop = false; // wait until the loop rate timer fires again 01725 } 01726 } 01727 } 01728 01729 //only do this for the MBED because of the limited file size 01730 //write one line to the file (open to write, this will erase all other data) and close it. 01731 void MbedLogger::eraseFile() { 01732 _fp = fopen(_full_file_path_string.c_str(), "w"); // LOG000.csv 01733 01734 fprintf(_fp,_heading_string.c_str()); 01735 01736 closeLogFile(); 01737 } 01738 01739 void MbedLogger::intCreateDataPacket(int data_buffer[],int payload_length) { 01740 // packet is 7565 0001 FFFF EEEE CC DATA DATA DATA ... CRC1 CRC2 01741 01742 //check here https://www.rapidtables.com/convert/number/hex-to-decimal.html?x=01c0 01743 // ieee 754: http://www6.uniovi.es/~antonio/uned/ieee754/IEEE-754hex32.html 01744 01745 //CLEAR: Removes all elements from the vector (which are destroyed), leaving the container with a size of 0. 01746 _data_packet.clear(); 01747 01748 //DATA PACKET HEADER 01749 _data_packet.push_back(117); //0x75 01750 _data_packet.push_back(101); //0x65 01751 01752 _data_packet.push_back(_packet_number/256); //current packet number in 0x#### form 01753 _data_packet.push_back(_packet_number%256); //current packet number in 0x#### form 01754 01755 _data_packet.push_back(_total_number_of_packets/256); //total number of packets, 0x#### form 01756 _data_packet.push_back(_total_number_of_packets%256); //total number of packets, 0x#### form 01757 01758 _data_packet.push_back(_current_line_length); 01759 01760 //DATA FROM INTEGER ARRAY (read the array) 01761 for (int i = 0; i < payload_length; i++) { 01762 _data_packet.push_back(data_buffer[i]); 01763 } 01764 01765 //CRC CALCULATIONS BELOW, character version of calculation screws up on null character 0x00, scrapped and using vector of integers 01766 int crc_one = calcCrcOne(); 01767 int crc_two = calcCrcTwo(); 01768 01769 //place the crc bytes into the data packet that is transmitted 01770 _data_packet.push_back(crc_one); 01771 _data_packet.push_back(crc_two); 01772 } 01773 01774 void MbedLogger::setTransmitPacketNumber(int packet_number) { 01775 _transmit_packet_num = packet_number; 01776 01777 //also needed to reset a boolean flag on the transmit 01778 _fsm_transmit_complete = false; 01779 01780 _end_transmit_packet = false; 01781 } 01782 01783 int MbedLogger::currentPacketNumber() { 01784 return _packet_number; 01785 }
Generated on Wed Jul 13 2022 15:28:17 by
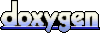