
Example using semaphore in ISR
Dependencies: IHM_V2
main.cpp
00001 // Title : mbed-os-isr-semaphore 00002 // Author: Jacques-Olivier Klein - IUT de CACHAN 00003 // Date: 2018-02-10 - rev. 2019-01-06 00004 00005 #include "mbed.h" 00006 #include "IHM.h" 00007 00008 IHM ihm; 00009 00010 DigitalOut L0 (PB_3) ; // led L0 00011 DigitalOut L1 (PA_7) ; // led L1 00012 00013 InterruptIn BP0_Interrupt(PA_9); 00014 00015 void thread_Semaphore(); 00016 void isrBP0 (); 00017 00018 Thread thread_waiting_for_semaphore (osPriorityNormal,OS_STACK_SIZE );; 00019 Semaphore mysemaphore(0); 00020 00021 int main(void) 00022 { ihm.LCD_clear(); 00023 ihm.LCD_printf("irq-semaphore-%s %s",__DATE__,__TIME__); 00024 printf("mbed-os-irq-semaphore-%s %s\n\r",__DATE__,__TIME__); 00025 printf("DEFAULT_STACK_SIZE:%d\n\r", OS_STACK_SIZE); 00026 00027 BP0_Interrupt.mode(PullUp); 00028 thread_waiting_for_semaphore.start(thread_Semaphore); 00029 BP0_Interrupt.fall(&isrBP0); 00030 while(1){ 00031 L0=!L0; 00032 printf("* [pid-%d]Main \n\r",osThreadGetId()); 00033 wait(0.200); 00034 } 00035 } 00036 00037 void isrBP0 (){ 00038 mysemaphore.release(); 00039 } 00040 00041 void thread_Semaphore(){ 00042 while(1){ 00043 mysemaphore.wait(); 00044 L1=!L1; 00045 printf(" * [pid-%d]Semaphore \n\r",osThreadGetId()); 00046 } 00047 } 00048
Generated on Thu Jul 14 2022 18:00:17 by
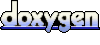