
Peer to Peer example
Dependencies: libmDot-dev-mbed2-deprecated mbed-rtos mbed
Fork of mDot_LoRa_PEER_TO_PEER by
main.cpp
00001 #include "mbed.h" 00002 #include "mDot.h" 00003 #include "mDotEvent.h" 00004 #include "MTSLog.h" 00005 #include <string> 00006 #include <vector> 00007 #include <algorithm> 00008 00009 // This example will setup a peer to peer node, copy program to two dots 00010 // Uplink packets will be sent every 5 seconds, however communication is half-duplex 00011 // An mDot cannot receive while transmitting 00012 // Received packets will be sent to the Serial port on pins 2 and 3 00013 // baud rate for serial ports is set to 115200 00014 00015 00016 Serial _serial(XBEE_DOUT, XBEE_DIN); 00017 Serial debug(USBTX, USBRX); 00018 00019 static uint8_t config_network_addr[] = { 0x01, 0x02, 0x03, 0x04 }; 00020 static uint8_t config_network_nskey[] = { 0x01, 0x02, 0x03, 0x04, 0x01, 0x02, 0x03, 0x04, 0x01, 0x02, 0x03, 0x04, 0x01, 0x02, 0x03, 0x04 }; 00021 static uint8_t config_network_dskey[] = { 0x01, 0x02, 0x03, 0x04, 0x01, 0x02, 0x03, 0x04, 0x01, 0x02, 0x03, 0x04, 0x01, 0x02, 0x03, 0x04 }; 00022 00023 // Custom event handler for receiving Class C packets 00024 00025 class RadioEvent : public mDotEvent 00026 { 00027 00028 00029 public: 00030 RadioEvent() {} 00031 00032 virtual ~RadioEvent() {} 00033 00034 /*! 00035 * MAC layer event callback prototype. 00036 * 00037 * \param [IN] flags Bit field indicating the MAC events occurred 00038 * \param [IN] info Details about MAC events occurred 00039 */ 00040 virtual void MacEvent(LoRaMacEventFlags* flags, LoRaMacEventInfo* info) { 00041 00042 if (mts::MTSLog::getLogLevel() == mts::MTSLog::TRACE_LEVEL) { 00043 std::string msg = "OK"; 00044 switch (info->Status) { 00045 case LORAMAC_EVENT_INFO_STATUS_ERROR: 00046 msg = "ERROR"; 00047 break; 00048 case LORAMAC_EVENT_INFO_STATUS_TX_TIMEOUT: 00049 msg = "TX_TIMEOUT"; 00050 break; 00051 case LORAMAC_EVENT_INFO_STATUS_RX_TIMEOUT: 00052 msg = "RX_TIMEOUT"; 00053 break; 00054 case LORAMAC_EVENT_INFO_STATUS_RX_ERROR: 00055 msg = "RX_ERROR"; 00056 break; 00057 case LORAMAC_EVENT_INFO_STATUS_JOIN_FAIL: 00058 msg = "JOIN_FAIL"; 00059 break; 00060 case LORAMAC_EVENT_INFO_STATUS_DOWNLINK_FAIL: 00061 msg = "DOWNLINK_FAIL"; 00062 break; 00063 case LORAMAC_EVENT_INFO_STATUS_ADDRESS_FAIL: 00064 msg = "ADDRESS_FAIL"; 00065 break; 00066 case LORAMAC_EVENT_INFO_STATUS_MIC_FAIL: 00067 msg = "MIC_FAIL"; 00068 break; 00069 default: 00070 break; 00071 } 00072 logTrace("Event: %s", msg.c_str()); 00073 00074 logTrace("Flags Tx: %d Rx: %d RxData: %d RxSlot: %d LinkCheck: %d JoinAccept: %d", 00075 flags->Bits.Tx, flags->Bits.Rx, flags->Bits.RxData, flags->Bits.RxSlot, flags->Bits.LinkCheck, flags->Bits.JoinAccept); 00076 logTrace("Info: Status: %d ACK: %d Retries: %d TxDR: %d RxPort: %d RxSize: %d RSSI: %d SNR: %d Energy: %d Margin: %d Gateways: %d", 00077 info->Status, info->TxAckReceived, info->TxNbRetries, info->TxDatarate, info->RxPort, info->RxBufferSize, 00078 info->RxRssi, info->RxSnr, info->Energy, info->DemodMargin, info->NbGateways); 00079 } 00080 00081 if (flags->Bits.Rx) { 00082 00083 logDebug("Rx %d bytes", info->RxBufferSize); 00084 if (info->RxBufferSize > 0) { 00085 00086 for (int i = 0; i < info->RxBufferSize; i++) { 00087 _serial.putc(info->RxBuffer[i]); 00088 } 00089 } 00090 } 00091 } 00092 }; 00093 00094 00095 int main() 00096 { 00097 int32_t ret; 00098 mDot* dot; 00099 std::vector<uint8_t> data; 00100 std::string data_str = "hello!"; 00101 00102 RadioEvent events; 00103 00104 debug.baud(115200); 00105 _serial.baud(115200); 00106 00107 // get a mDot handle 00108 dot = mDot::getInstance(); 00109 00110 // Set custom events handler for receiving Class C packets 00111 dot->setEvents(&events); 00112 00113 // print library version information 00114 logInfo("version: %s", dot->getId().c_str()); 00115 00116 //******************************************* 00117 // configuration 00118 //******************************************* 00119 // reset to default config so we know what state we're in 00120 dot->resetConfig(); 00121 00122 dot->setLogLevel(mts::MTSLog::TRACE_LEVEL); 00123 00124 // set up the mDot with our network information: Network Address and Session Keys must match on each device 00125 // these can all be saved in NVM so they don't need to be set every time - see mDot::saveConfig() 00126 std::vector<uint8_t> temp; 00127 00128 for (int i = 0; i < 4; i++) { 00129 temp.push_back(config_network_addr[i]); 00130 } 00131 00132 logInfo("setting network addr"); 00133 if ((ret = dot->setNetworkAddress(temp)) != mDot::MDOT_OK) { 00134 logError("failed to set network name %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00135 } 00136 00137 temp.clear(); 00138 for (int i = 0; i < 16; i++) { 00139 temp.push_back(config_network_nskey[i]); 00140 } 00141 00142 logInfo("setting network password"); 00143 if ((ret = dot->setNetworkSessionKey(temp)) != mDot::MDOT_OK) { 00144 logError("failed to set network password %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00145 } 00146 00147 temp.clear(); 00148 for (int i = 0; i < 16; i++) { 00149 temp.push_back(config_network_dskey[i]); 00150 } 00151 00152 logInfo("setting network password"); 00153 if ((ret = dot->setDataSessionKey(temp)) != mDot::MDOT_OK) { 00154 logError("failed to set network password %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00155 } 00156 00157 logInfo("setting TX frequency"); 00158 if ((ret = dot->setTxFrequency(915500000)) != mDot::MDOT_OK) { 00159 logError("failed to set TX frequency %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00160 } 00161 00162 // a higher spreading factor allows for longer range but lower throughput 00163 // in the 915 (US) frequency band, DR8-DR13 00164 logInfo("setting TX spreading factor"); 00165 if ((ret = dot->setTxDataRate(mDot::DR13)) != mDot::MDOT_OK) { 00166 logError("failed to set TX datarate %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00167 } 00168 00169 // set join mode to AUTO_OTA so the mDot doesn't have to rejoin after sleeping 00170 logInfo("setting join mode to AUTO_OTA"); 00171 if ((ret = dot->setJoinMode(mDot::PEER_TO_PEER)) != mDot::MDOT_OK) { 00172 logError("failed to set join mode %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00173 } 00174 00175 // save this configuration to the mDot's NVM 00176 logInfo("saving config"); 00177 if (! dot->saveConfig()) { 00178 logError("failed to save configuration"); 00179 } 00180 00181 //******************************************* 00182 // end of configuration 00183 //******************************************* 00184 00185 // format data for sending to the gateway 00186 for (std::string::iterator it = data_str.begin(); it != data_str.end(); it++) 00187 data.push_back((uint8_t) *it); 00188 00189 while(true) { 00190 // send the data 00191 if ((ret = dot->send(data)) != mDot::MDOT_OK) { 00192 logError("failed to send %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00193 } else { 00194 logInfo("successfully sent data to peer"); 00195 } 00196 00197 wait(5.0); 00198 } 00199 00200 return 0; 00201 }
Generated on Tue Jul 12 2022 23:51:37 by
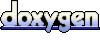