Bleeding edge development version of the xDot library for mbed 5. This version of the library is not guaranteed to be stable or well tested and should not be used in production or deployment scenarios.
Dependents: Dot-Examples Dot-AT-Firmware Dot-Examples TEST_FF1705 ... more
mDot.h
00001 // TODO: add license header 00002 00003 #ifndef MDOT_H 00004 #define MDOT_H 00005 00006 #include "mbed.h" 00007 #include "rtos.h" 00008 #include "Mote.h" 00009 #include <vector> 00010 #include <map> 00011 #include <string> 00012 00013 class mDotEvent; 00014 class LoRaConfig; 00015 00016 00017 class mDot { 00018 friend class mDotEvent; 00019 00020 private: 00021 00022 mDot(lora::ChannelPlan* plan); 00023 ~mDot(); 00024 00025 void initLora(); 00026 00027 void setLastError(const std::string& str); 00028 00029 static bool validateBaudRate(const uint32_t& baud); 00030 static bool validateFrequencySubBand(const uint8_t& band); 00031 bool validateDataRate(const uint8_t& dr); 00032 00033 int32_t joinBase(const uint32_t& retries); 00034 int32_t sendBase(const std::vector<uint8_t>& data, const bool& confirmed = false, const bool& blocking = true, const bool& highBw = false); 00035 void waitForPacket(); 00036 void waitForLinkCheck(); 00037 00038 00039 MBED_DEPRECATED("Will be removed in dotlib 3.3.0") 00040 void setActivityLedState(const uint8_t& state); 00041 00042 MBED_DEPRECATED("Will be removed in dotlib 3.3.0") 00043 uint8_t getActivityLedState(); 00044 00045 MBED_DEPRECATED("Will be removed in dotlib 3.3.0") 00046 void blinkActivityLed(void) { 00047 if (_activity_led) { 00048 int val = _activity_led->read(); 00049 _activity_led->write(!val); 00050 } 00051 } 00052 00053 mDot(const mDot&); 00054 mDot& operator=(const mDot&); 00055 00056 uint32_t RTC_ReadBackupRegister(uint32_t RTC_BKP_DR); 00057 void RTC_WriteBackupRegister(uint32_t RTC_BKP_DR, uint32_t Data); 00058 00059 void wakeup(); 00060 00061 void RTC_DisableWakeupTimer(); 00062 void RTC_EnableWakeupTimer(); 00063 00064 void enterStopMode(const uint32_t& interval, const uint8_t& wakeup_mode = RTC_ALARM); 00065 void enterStandbyMode(const uint32_t& interval, const uint8_t& wakeup_mode = RTC_ALARM); 00066 00067 static mDot* _instance; 00068 00069 lora::Mote* _mote; 00070 LoRaConfig* _config; 00071 lora::Settings _settings; 00072 mDotEvent* _events; 00073 00074 std::string _last_error; 00075 static const uint32_t _baud_rates[]; 00076 uint8_t _activity_led_state; //deprecated will be removed 00077 Ticker _tick; 00078 DigitalOut* _activity_led; //deprecated will be removed 00079 bool _activity_led_enable; //deprecated will be removed 00080 PinName _activity_led_pin; //deprecated will be removed 00081 bool _activity_led_external; //deprecated will be removed 00082 uint8_t _linkFailCount; 00083 uint8_t _class; 00084 InterruptIn* _wakeup; 00085 PinName _wakeup_pin; 00086 00087 typedef enum { 00088 OFF, 00089 ON, 00090 BLINK, 00091 } state; 00092 00093 public: 00094 00095 #if defined(TARGET_MTS_MDOT_F411RE) 00096 typedef enum { 00097 FM_APPEND = (1 << 0), 00098 FM_TRUNC = (1 << 1), 00099 FM_CREAT = (1 << 2), 00100 FM_RDONLY = (1 << 3), 00101 FM_WRONLY = (1 << 4), 00102 FM_RDWR = (FM_RDONLY | FM_WRONLY), 00103 FM_DIRECT = (1 << 5) 00104 } FileMode; 00105 #endif /* TARGET_MTS_MDOT_F411RE */ 00106 00107 typedef enum { 00108 MDOT_OK = 0, 00109 MDOT_INVALID_PARAM = -1, 00110 MDOT_TX_ERROR = -2, 00111 MDOT_RX_ERROR = -3, 00112 MDOT_JOIN_ERROR = -4, 00113 MDOT_TIMEOUT = -5, 00114 MDOT_NOT_JOINED = -6, 00115 MDOT_ENCRYPTION_DISABLED = -7, 00116 MDOT_NO_FREE_CHAN = -8, 00117 MDOT_TEST_MODE = -9, 00118 MDOT_NO_ENABLED_CHAN = -10, 00119 MDOT_AGGREGATED_DUTY_CYCLE = -11, 00120 MDOT_MAX_PAYLOAD_EXCEEDED = -12, 00121 MDOT_LBT_CHANNEL_BUSY = -13, 00122 MDOT_NOT_IDLE = -14, 00123 MDOT_ERROR = -1024, 00124 } mdot_ret_code; 00125 00126 enum JoinMode { 00127 MANUAL = 0, 00128 OTA, 00129 AUTO_OTA, 00130 PEER_TO_PEER 00131 }; 00132 00133 enum Mode { 00134 COMMAND_MODE, 00135 SERIAL_MODE 00136 }; 00137 00138 enum RX_Output { 00139 HEXADECIMAL, 00140 BINARY, 00141 EXTENDED 00142 }; 00143 00144 enum DataRates { 00145 DR0, 00146 DR1, 00147 DR2, 00148 DR3, 00149 DR4, 00150 DR5, 00151 DR6, 00152 DR7, 00153 DR8, 00154 DR9, 00155 DR10, 00156 DR11, 00157 DR12, 00158 DR13, 00159 DR14, 00160 DR15 00161 }; 00162 00163 enum FrequencySubBands { 00164 FSB_ALL, 00165 FSB_1, 00166 FSB_2, 00167 FSB_3, 00168 FSB_4, 00169 FSB_5, 00170 FSB_6, 00171 FSB_7, 00172 FSB_8 00173 }; 00174 00175 enum JoinByteOrder { 00176 LSB, 00177 MSB 00178 }; 00179 00180 enum wakeup_mode { 00181 RTC_ALARM, 00182 INTERRUPT, 00183 RTC_ALARM_OR_INTERRUPT 00184 }; 00185 00186 enum UserBackupRegs { 00187 UBR0, 00188 UBR1, 00189 UBR2, 00190 UBR3, 00191 UBR4, 00192 UBR5, 00193 UBR6, 00194 UBR7, 00195 UBR8, 00196 UBR9 00197 #if defined (TARGET_XDOT_L151CC) 00198 ,UBR10, 00199 UBR11, 00200 UBR12, 00201 UBR13, 00202 UBR14, 00203 UBR15, 00204 UBR16, 00205 UBR17, 00206 UBR18, 00207 UBR19, 00208 UBR20, 00209 UBR21 00210 #endif /* TARGET_XDOT_L151CC */ 00211 }; 00212 00213 #if defined(TARGET_MTS_MDOT_F411RE) 00214 typedef struct { 00215 int16_t fd; 00216 char name[33]; 00217 uint32_t size; 00218 } mdot_file; 00219 #endif /* TARGET_MTS_MDOT_F411RE */ 00220 00221 typedef struct { 00222 uint32_t Up; 00223 uint32_t Down; 00224 uint32_t Joins; 00225 uint32_t JoinFails; 00226 uint32_t MissedAcks; 00227 uint32_t CRCErrors; 00228 } mdot_stats; 00229 00230 typedef struct { 00231 int16_t last; 00232 int16_t min; 00233 int16_t max; 00234 int16_t avg; 00235 } rssi_stats; 00236 00237 typedef struct { 00238 int16_t last; 00239 int16_t min; 00240 int16_t max; 00241 int16_t avg; 00242 } snr_stats; 00243 00244 typedef struct { 00245 bool status; 00246 uint8_t dBm; 00247 uint32_t gateways; 00248 std::vector<uint8_t> payload; 00249 } link_check; 00250 00251 typedef struct { 00252 int32_t status; 00253 int16_t rssi; 00254 int16_t snr; 00255 } ping_response; 00256 00257 static std::string JoinModeStr(uint8_t mode); 00258 static std::string ModeStr(uint8_t mode); 00259 static std::string RxOutputStr(uint8_t format); 00260 static std::string DataRateStr(uint8_t rate); 00261 static std::string FrequencyBandStr(uint8_t band); 00262 static std::string FrequencySubBandStr(uint8_t band); 00263 00264 #if defined(TARGET_MTS_MDOT_F411RE) 00265 uint32_t UserRegisters[10]; 00266 #else 00267 uint32_t UserRegisters[22]; 00268 #endif /* TARGET_MTS_MDOT_F411RE */ 00269 00270 /** 00271 * Get a handle to the singleton object 00272 * @param plan the channel plan to use 00273 * @returns pointer to mDot object 00274 */ 00275 static mDot* getInstance(lora::ChannelPlan* plan); 00276 00277 /** 00278 * Can only be used after a dot has 00279 * configured with a plan 00280 * @returns pointer to mDot object 00281 */ 00282 static mDot* getInstance(); 00283 00284 void setEvents(mDotEvent* events); 00285 00286 /** 00287 * 00288 * Get library version information 00289 * @returns string containing library version information 00290 */ 00291 std::string getId(); 00292 00293 /** 00294 * Get MTS LoRa version information 00295 * @returns string containing MTS LoRa version information 00296 */ 00297 std::string getMtsLoraId(); 00298 00299 /** 00300 * Perform a soft reset of the system 00301 */ 00302 void resetCpu(); 00303 00304 /** 00305 * Reset config to factory default 00306 */ 00307 void resetConfig(); 00308 00309 /** 00310 * Save config data to non volatile memory 00311 * @returns true if success, false if failure 00312 */ 00313 bool saveConfig(); 00314 00315 /** 00316 * Set the log level for the library 00317 * options are: 00318 * NONE_LEVEL - logging is off at this level 00319 * FATAL_LEVEL - only critical errors will be reported 00320 * ERROR_LEVEL 00321 * WARNING_LEVEL 00322 * INFO_LEVEL 00323 * DEBUG_LEVEL 00324 * TRACE_LEVEL - every detail will be reported 00325 * @param level the level to log at 00326 * @returns MDOT_OK if success 00327 */ 00328 int32_t setLogLevel(const uint8_t& level); 00329 00330 /** 00331 * Get the current log level for the library 00332 * @returns current log level 00333 */ 00334 uint8_t getLogLevel(); 00335 00336 /** 00337 * Seed pseudo RNG in LoRaMac layer, uses random value from radio RSSI reading by default 00338 * @param seed for RNG 00339 */ 00340 void seedRandom(uint32_t seed); 00341 00342 00343 uint8_t setChannelPlan(lora::ChannelPlan* plan); 00344 00345 lora::Settings* getSettings(); 00346 00347 MBED_DEPRECATED("Will be removed in dotlib 3.3.0") 00348 void setActivityLedEnable(const bool& enable); 00349 00350 MBED_DEPRECATED("Will be removed in dotlib 3.3.0") 00351 bool getActivityLedEnable(); 00352 00353 MBED_DEPRECATED("Will be removed in dotlib 3.3.0") 00354 void setActivityLedPin(const PinName& pin); 00355 00356 MBED_DEPRECATED("Will be removed in dotlib 3.3.0") 00357 void setActivityLedPin(DigitalOut* pin); 00358 00359 MBED_DEPRECATED("Will be removed in dotlib 3.3.0") 00360 PinName getActivityLedPin(); 00361 00362 /** 00363 * Returns boolean indicative of start-up from standby mode 00364 * @returns true if dot woke from standby 00365 */ 00366 bool getStandbyFlag(); 00367 00368 std::vector<uint16_t> getChannelMask(); 00369 00370 int32_t setChannelMask(uint8_t offset, uint16_t mask); 00371 00372 /** 00373 * Add a channel 00374 * @returns MDOT_OK 00375 */ 00376 int32_t addChannel(uint8_t index, uint32_t frequency, uint8_t datarateRange); 00377 00378 /** 00379 * Add a downlink channel 00380 * @returns MDOT_OK 00381 */ 00382 int32_t addDownlinkChannel(uint8_t index, uint32_t frequency); 00383 00384 /** 00385 * Get list of channel frequencies currently in use 00386 * @returns vector of channels currently in use 00387 */ 00388 std::vector<uint32_t> getChannels(); 00389 00390 /** 00391 * Get list of downlink channel frequencies currently in use 00392 * @returns vector of channels currently in use 00393 */ 00394 std::vector<uint32_t> getDownlinkChannels(); 00395 00396 /** 00397 * Get list of channel datarate ranges currently in use 00398 * @returns vector of datarate ranges currently in use 00399 */ 00400 std::vector<uint8_t> getChannelRanges(); 00401 00402 /** 00403 * Get list of channel frequencies in config file to be used as session defaults 00404 * @returns vector of channels in config file 00405 */ 00406 std::vector<uint32_t> getConfigChannels(); 00407 00408 /** 00409 * Get list of channel datarate ranges in config file to be used as session defaults 00410 * @returns vector of datarate ranges in config file 00411 */ 00412 std::vector<uint8_t> getConfigChannelRanges(); 00413 00414 /** 00415 * Get default frequency band 00416 * @returns frequency band the device was manufactured for 00417 */ 00418 uint8_t getDefaultFrequencyBand(); 00419 00420 /** 00421 * Set frequency sub band 00422 * only applicable if frequency band is set for United States (FB_915) 00423 * sub band 0 will allow the radio to use all 64 channels 00424 * sub band 1 - 8 will allow the radio to use the 8 channels in that sub band 00425 * for use with Conduit gateway and MTAC_LORA, use sub bands 1 - 8, not sub band 0 00426 * @param band the sub band to use (0 - 8) 00427 * @returns MDOT_OK if success 00428 */ 00429 int32_t setFrequencySubBand(const uint8_t& band); 00430 00431 /** 00432 * Get frequency sub band 00433 * @returns frequency sub band currently in use 00434 */ 00435 uint8_t getFrequencySubBand(); 00436 00437 /** 00438 * Get frequency band 00439 * @returns frequency band (channel plan) currently in use 00440 */ 00441 uint8_t getFrequencyBand(); 00442 00443 /** 00444 * Get channel plan name 00445 * @returns name of channel plan currently in use 00446 */ 00447 std::string getChannelPlanName(); 00448 00449 /** 00450 * Get the datarate currently in use within the MAC layer 00451 * returns 0-15 00452 */ 00453 uint8_t getSessionDataRate(); 00454 00455 00456 /** 00457 * Get the current max EIRP used in the channel plan 00458 * May be changed by the network server 00459 * returns 0-36 00460 */ 00461 uint8_t getSessionMaxEIRP(); 00462 00463 /** 00464 * Set the current max EIRP used in the channel plan 00465 * May be changed by the network server 00466 * accepts 0-36 00467 */ 00468 void setSessionMaxEIRP(uint8_t max); 00469 00470 /** 00471 * Get the current downlink dwell time used in the channel plan 00472 * May be changed by the network server 00473 * returns 0-1 00474 */ 00475 uint8_t getSessionDownlinkDwelltime(); 00476 00477 /** 00478 * Set the current downlink dwell time used in the channel plan 00479 * May be changed by the network server 00480 * accepts 0-1 00481 */ 00482 void setSessionDownlinkDwelltime(uint8_t dwell); 00483 00484 /** 00485 * Get the current uplink dwell time used in the channel plan 00486 * May be changed by the network server 00487 * returns 0-1 00488 */ 00489 uint8_t getSessionUplinkDwelltime(); 00490 00491 /** 00492 * Set the current uplink dwell time used in the channel plan 00493 * May be changed by the network server 00494 * accepts 0-1 00495 */ 00496 void setSessionUplinkDwelltime(uint8_t dwell); 00497 00498 /** 00499 * Set the current downlink dwell time used in the channel plan 00500 * May be changed by the network server 00501 * accepts 0-1 00502 */ 00503 uint32_t getListenBeforeTalkTime(uint8_t ms); 00504 00505 /** 00506 * Set the current downlink dwell time used in the channel plan 00507 * May be changed by the network server 00508 * accepts 0-1 00509 */ 00510 void setListenBeforeTalkTime(uint32_t ms); 00511 00512 /** 00513 * Set public network mode 00514 * 0:PRIVATE_MTS, 1:PUBLIC_LORAWAN, 2:PRIVATE_LORAWAN 00515 * PRIVATE_MTS - Sync Word 0x12, US/AU Downlink frequencies per Frequency Sub Band 00516 * PUBLIC_LORAWAN - Sync Word 0x34 00517 * PRIVATE_LORAWAN - Sync Word 0x12 00518 * 00519 * The default Join Delay is 5 seconds 00520 * The default Join Delay for PRIVATE_MTS was 1 second in the previous release 00521 * The Join Delay must be changed independently of Public Network setting 00522 * 00523 * @see lora::NetworkType 00524 * @returns MDOT_OK if success 00525 */ 00526 int32_t setPublicNetwork(const uint8_t& val); 00527 00528 /** 00529 * Get public network mode 00530 * 00531 * The default Join Delay is 5 seconds 00532 * The default Join Delay for PRIVATE_MTS was 1 second in the previous release 00533 * The Join Delay must be changed independently of Public Network setting 00534 * 00535 * @see lora:NetworkType 00536 * @returns 0:PRIVATE_MTS, 1:PUBLIC_LORAWAN, 2:PRIVATE_LORAWAN 00537 */ 00538 uint8_t getPublicNetwork(); 00539 00540 /** 00541 * Get the device ID 00542 * @returns vector containing the device ID (size 8) 00543 */ 00544 std::vector<uint8_t> getDeviceId(); 00545 00546 /** 00547 * Get the device port to be used for lora application data (1-223) 00548 * @returns port 00549 */ 00550 uint8_t getAppPort(); 00551 00552 /** 00553 * Set the device port to be used for lora application data (1-223) 00554 * @returns MDOT_OK if success 00555 */ 00556 int32_t setAppPort(uint8_t port); 00557 00558 /** 00559 * Set the device class A, B or C 00560 * @returns MDOT_OK if success 00561 */ 00562 int32_t setClass(std::string newClass); 00563 00564 /** 00565 * Get the device class A, B or C 00566 * @returns MDOT_OK if success 00567 */ 00568 std::string getClass(); 00569 00570 /** 00571 * Get the max packet length with current settings 00572 * @returns max packet length 00573 */ 00574 uint8_t getMaxPacketLength(); 00575 00576 /** 00577 * Set network address 00578 * for use with MANUAL network join mode, will be assigned in OTA & AUTO_OTA modes 00579 * @param addr a vector of 4 bytes 00580 * @returns MDOT_OK if success 00581 */ 00582 int32_t setNetworkAddress(const std::vector<uint8_t>& addr); 00583 00584 /** 00585 * Get network address 00586 * @returns vector containing network address (size 4) 00587 */ 00588 std::vector<uint8_t> getNetworkAddress(); 00589 00590 /** 00591 * Set network session key 00592 * for use with MANUAL network join mode, will be assigned in OTA & AUTO_OTA modes 00593 * @param key a vector of 16 bytes 00594 * @returns MDOT_OK if success 00595 */ 00596 int32_t setNetworkSessionKey(const std::vector<uint8_t>& key); 00597 00598 /** 00599 * Get network session key 00600 * @returns vector containing network session key (size 16) 00601 */ 00602 std::vector<uint8_t> getNetworkSessionKey(); 00603 00604 /** 00605 * Set data session key 00606 * for use with MANUAL network join mode, will be assigned in OTA & AUTO_OTA modes 00607 * @param key a vector of 16 bytes 00608 * @returns MDOT_OK if success 00609 */ 00610 int32_t setDataSessionKey(const std::vector<uint8_t>& key); 00611 00612 /** 00613 * Get data session key 00614 * @returns vector containing data session key (size 16) 00615 */ 00616 std::vector<uint8_t> getDataSessionKey(); 00617 00618 /** 00619 * Set network name 00620 * for use with OTA & AUTO_OTA network join modes 00621 * generates network ID (crc64 of name) automatically 00622 * @param name a string of of at least 8 bytes and no more than 128 bytes 00623 * @return MDOT_OK if success 00624 */ 00625 int32_t setNetworkName(const std::string& name); 00626 00627 /** 00628 * Get network name 00629 * @return string containing network name (size 8 to 128) 00630 */ 00631 std::string getNetworkName(); 00632 00633 /** 00634 * Set network ID 00635 * for use with OTA & AUTO_OTA network join modes 00636 * setting network ID via this function sets network name to empty 00637 * @param id a vector of 8 bytes 00638 * @returns MDOT_OK if success 00639 */ 00640 int32_t setNetworkId(const std::vector<uint8_t>& id); 00641 00642 /** 00643 * Get network ID 00644 * @returns vector containing network ID (size 8) 00645 */ 00646 std::vector<uint8_t> getNetworkId(); 00647 00648 /** 00649 * Set network passphrase 00650 * for use with OTA & AUTO_OTA network join modes 00651 * generates network key (cmac of passphrase) automatically 00652 * @param name a string of of at least 8 bytes and no more than 128 bytes 00653 * @return MDOT_OK if success 00654 */ 00655 int32_t setNetworkPassphrase(const std::string& passphrase); 00656 00657 /** 00658 * Get network passphrase 00659 * @return string containing network passphrase (size 8 to 128) 00660 */ 00661 std::string getNetworkPassphrase(); 00662 00663 /** 00664 * Set network key 00665 * for use with OTA & AUTO_OTA network join modes 00666 * setting network key via this function sets network passphrase to empty 00667 * @param id a vector of 16 bytes 00668 * @returns MDOT_OK if success 00669 */ 00670 int32_t setNetworkKey(const std::vector<uint8_t>& id); 00671 00672 /** 00673 * Get network key 00674 * @returns a vector containing network key (size 16) 00675 */ 00676 std::vector<uint8_t> getNetworkKey(); 00677 00678 /** 00679 * Set lorawan application EUI 00680 * equivalent to setNetworkId 00681 * @param eui application EUI (size 8) 00682 */ 00683 int32_t setAppEUI(const uint8_t* eui); 00684 00685 /** 00686 * Get lorawan application EUI 00687 * equivalent to getNetworkId 00688 * @returns vector containing application EUI (size 8) 00689 */ 00690 const uint8_t* getAppEUI(); 00691 00692 /** 00693 * Set lorawan application key 00694 * equivalent to setNetworkKey 00695 * @param eui application key (size 16) 00696 */ 00697 int32_t setAppKey(const uint8_t* key); 00698 00699 /** 00700 * Set lorawan application key 00701 * equivalent to getNetworkKey 00702 * @returns eui application key (size 16) 00703 */ 00704 const uint8_t* getAppKey(); 00705 00706 /** 00707 * Add a multicast session address and keys 00708 * Downlink counter is set to 0 00709 * Up to 3 MULTICAST_SESSIONS can be set 00710 */ 00711 int32_t setMulticastSession(uint8_t index, uint32_t addr, const uint8_t* nsk, const uint8_t* dsk); 00712 00713 /** 00714 * Set a multicast session counter 00715 * Up to 3 MULTICAST_SESSIONS can be set 00716 */ 00717 int32_t setMulticastDownlinkCounter(uint8_t index, uint32_t count); 00718 00719 /** 00720 * Set join byte order 00721 * @param order 0:LSB 1:MSB 00722 */ 00723 uint32_t setJoinByteOrder(uint8_t order); 00724 00725 /** 00726 * Get join byte order 00727 * @returns byte order to use in joins 0:LSB 1:MSB 00728 */ 00729 uint8_t getJoinByteOrder(); 00730 00731 /** 00732 * Attempt to join network 00733 * each attempt will be made with a random datarate up to the configured datarate 00734 * JoinRequest backoff between tries is enforced to 1% for 1st hour, 0.1% for 1-10 hours and 0.01% after 10 hours 00735 * Check getNextTxMs() for time until next join attempt can be made 00736 * @returns MDOT_OK if success 00737 */ 00738 int32_t joinNetwork(); 00739 00740 /** 00741 * Attempts to join network once 00742 * @returns MDOT_OK if success 00743 */ 00744 int32_t joinNetworkOnce(); 00745 00746 /** 00747 * Resets current network session, essentially disconnecting from the network 00748 * has no effect for MANUAL network join mode 00749 */ 00750 void resetNetworkSession(); 00751 00752 /** 00753 * Restore saved network session from flash 00754 * has no effect for MANUAL network join mode 00755 */ 00756 void restoreNetworkSession(); 00757 00758 /** 00759 * Save current network session to flash 00760 * has no effect for MANUAL network join mode 00761 */ 00762 void saveNetworkSession(); 00763 00764 /** 00765 * Set number of times joining will retry each sub-band before changing 00766 * to the next subband in US915 and AU915 00767 * @param retries must be between 0 - 255 00768 * @returns MDOT_OK if success 00769 */ 00770 int32_t setJoinRetries(const uint8_t& retries); 00771 00772 /** 00773 * Get number of times joining will retry each sub-band 00774 * @returns join retries (0 - 255) 00775 */ 00776 uint8_t getJoinRetries(); 00777 00778 /** 00779 * Set network join mode 00780 * MANUAL: set network address and session keys manually 00781 * OTA: User sets network name and passphrase, then attempts to join 00782 * AUTO_OTA: same as OTA, but network sessions can be saved and restored 00783 * @param mode MANUAL, OTA, or AUTO_OTA 00784 * @returns MDOT_OK if success 00785 */ 00786 int32_t setJoinMode(const uint8_t& mode); 00787 00788 /** 00789 * Get network join mode 00790 * @returns MANUAL, OTA, or AUTO_OTA 00791 */ 00792 uint8_t getJoinMode(); 00793 00794 /** 00795 * Get network join status 00796 * @returns true if currently joined to network 00797 */ 00798 bool getNetworkJoinStatus(); 00799 00800 /** 00801 * Do a network link check 00802 * application data may be returned in response to a network link check command 00803 * @returns link_check structure containing success, dBm above noise floor, gateways in range, and packet payload 00804 */ 00805 link_check networkLinkCheck(); 00806 00807 /** 00808 * Set network link check count to perform automatic link checks every count packets 00809 * only applicable if ACKs are disabled 00810 * @param count must be between 0 - 255 00811 * @returns MDOT_OK if success 00812 */ 00813 int32_t setLinkCheckCount(const uint8_t& count); 00814 00815 /** 00816 * Get network link check count 00817 * @returns count (0 - 255) 00818 */ 00819 uint8_t getLinkCheckCount(); 00820 00821 /** 00822 * Set network link check threshold, number of link check failures or missed acks to tolerate 00823 * before considering network connection lost 00824 * @pararm count must be between 0 - 255 00825 * @returns MDOT_OK if success 00826 */ 00827 int32_t setLinkCheckThreshold(const uint8_t& count); 00828 00829 /** 00830 * Get network link check threshold 00831 * @returns threshold (0 - 255) 00832 */ 00833 uint8_t getLinkCheckThreshold(); 00834 00835 /** 00836 * Get/set number of failed link checks in the current session 00837 * @returns count (0 - 255) 00838 */ 00839 uint8_t getLinkFailCount(); 00840 int32_t setLinkFailCount(uint8_t count); 00841 00842 /** 00843 * Set UpLinkCounter number of packets sent to the gateway during this network session (sequence number) 00844 * @returns MDOT_OK 00845 */ 00846 int32_t setUpLinkCounter(uint32_t count); 00847 00848 /** 00849 * Get UpLinkCounter 00850 * @returns number of packets sent to the gateway during this network session (sequence number) 00851 */ 00852 uint32_t getUpLinkCounter(); 00853 00854 /** 00855 * Set UpLinkCounter number of packets sent by the gateway during this network session (sequence number) 00856 * @returns MDOT_OK 00857 */ 00858 int32_t setDownLinkCounter(uint32_t count); 00859 00860 /** 00861 * Get DownLinkCounter 00862 * @returns number of packets sent by the gateway during this network session (sequence number) 00863 */ 00864 uint32_t getDownLinkCounter(); 00865 00866 /** 00867 * Enable/disable AES encryption 00868 * AES encryption must be enabled for use with Conduit gateway and MTAC_LORA card 00869 * @param on true for AES encryption to be enabled 00870 * @returns MDOT_OK if success 00871 */ 00872 int32_t setAesEncryption(const bool& on); 00873 00874 /** 00875 * Get AES encryption 00876 * @returns true if AES encryption is enabled 00877 */ 00878 bool getAesEncryption(); 00879 00880 /** 00881 * Get RSSI stats 00882 * @returns rssi_stats struct containing last, min, max, and avg RSSI in dB 00883 */ 00884 rssi_stats getRssiStats(); 00885 00886 /** 00887 * Get SNR stats 00888 * @returns snr_stats struct containing last, min, max, and avg SNR in cB 00889 */ 00890 snr_stats getSnrStats(); 00891 00892 /** 00893 * Get ms until next free channel 00894 * only applicable for European models, US models return 0 00895 * @returns time (ms) until a channel is free to use for transmitting 00896 */ 00897 uint32_t getNextTxMs(); 00898 00899 /** 00900 * Get join delay in seconds 00901 * Defaults to 5 seconds 00902 * Must match join delay setting of the network server 00903 * 00904 * The default Join Delay is 5 seconds 00905 * The default Join Delay for PRIVATE_MTS was 1 second in the previous release 00906 * 00907 * @returns number of seconds before join accept message is expected 00908 */ 00909 uint8_t getJoinDelay(); 00910 00911 /** 00912 * Set join delay in seconds 00913 * Defaults to 5 seconds 00914 * Must match join delay setting of the network server 00915 * 00916 * The default Join Delay is 5 seconds 00917 * The default Join Delay for PRIVATE_MTS was 1 second in the previous release 00918 * 00919 * @param delay number of seconds before join accept message is expected 00920 * @return MDOT_OK if success 00921 */ 00922 uint32_t setJoinDelay(uint8_t delay); 00923 00924 /** 00925 * Get join Rx1 datarate offset 00926 * defaults to 0 00927 * @returns offset 00928 */ 00929 uint8_t getJoinRx1DataRateOffset(); 00930 00931 /** 00932 * Set join Rx1 datarate offset 00933 * @param offset for datarate 00934 * @return MDOT_OK if success 00935 */ 00936 uint32_t setJoinRx1DataRateOffset(uint8_t offset); 00937 00938 /** 00939 * Get join Rx2 datarate 00940 * defaults to US:DR8, AU:DR8, EU:DR0 00941 * @returns datarate 00942 */ 00943 uint8_t getJoinRx2DataRate(); 00944 00945 /** 00946 * Set join Rx2 datarate 00947 * @param datarate 00948 * @return MDOT_OK if success 00949 */ 00950 uint32_t setJoinRx2DataRate(uint8_t datarate); 00951 00952 /** 00953 * Get join Rx2 frequency 00954 * defaults US:923.3, AU:923.3, EU:869.525 00955 * @returns frequency 00956 */ 00957 uint32_t getJoinRx2Frequency(); 00958 00959 /** 00960 * Set join Rx2 frequency 00961 * @param frequency 00962 * @return MDOT_OK if success 00963 */ 00964 uint32_t setJoinRx2Frequency(uint32_t frequency); 00965 00966 /** 00967 * Get rx delay in seconds 00968 * Defaults to 1 second 00969 * @returns number of seconds before response message is expected 00970 */ 00971 uint8_t getRxDelay(); 00972 00973 /** 00974 * Set rx delay in seconds 00975 * Defaults to 1 second 00976 * @param delay number of seconds before response message is expected 00977 * @return MDOT_OK if success 00978 */ 00979 uint32_t setRxDelay(uint8_t delay); 00980 00981 /** 00982 * Get preserve session to save network session info through reset or power down in AUTO_OTA mode 00983 * Defaults to off 00984 * @returns true if enabled 00985 */ 00986 bool getPreserveSession(); 00987 00988 /** 00989 * Set preserve session to save network session info through reset or power down in AUTO_OTA mode 00990 * Defaults to off 00991 * @param enable 00992 * @return MDOT_OK if success 00993 */ 00994 uint32_t setPreserveSession(bool enable); 00995 00996 /** 00997 * Get data pending 00998 * only valid after sending data to the gateway 00999 * @returns true if server has available packet(s) 01000 */ 01001 bool getDataPending(); 01002 01003 /** 01004 * Get ack requested 01005 * only valid after sending data to the gateway 01006 * @returns true if server has requested ack 01007 */ 01008 bool getAckRequested(); 01009 01010 /** 01011 * Get is transmitting indicator 01012 * @returns true if currently transmitting 01013 */ 01014 bool getIsTransmitting(); 01015 01016 /** 01017 * Get is idle indicator 01018 * @returns true if not currently transmitting, waiting or receiving 01019 */ 01020 bool getIsIdle(); 01021 01022 /** 01023 * Set TX data rate 01024 * data rates affect maximum payload size 01025 * @param dr DR0-DR7 for Europe, DR0-DR4 for United States 01026 * @returns MDOT_OK if success 01027 */ 01028 int32_t setTxDataRate(const uint8_t& dr); 01029 01030 /** 01031 * Get TX data rate 01032 * @returns current TX data rate (DR0-DR15) 01033 */ 01034 uint8_t getTxDataRate(); 01035 01036 /** 01037 * Get a random value from the radio based on RSSI 01038 * @returns randome value 01039 */ 01040 uint32_t getRadioRandom(); 01041 01042 /** 01043 * Get data rate spreading factor and bandwidth 01044 * EU868 Datarates 01045 * --------------- 01046 * DR0 - SF12BW125 01047 * DR1 - SF11BW125 01048 * DR2 - SF10BW125 01049 * DR3 - SF9BW125 01050 * DR4 - SF8BW125 01051 * DR5 - SF7BW125 01052 * DR6 - SF7BW250 01053 * DR7 - FSK 01054 * 01055 * US915 Datarates 01056 * --------------- 01057 * DR0 - SF10BW125 01058 * DR1 - SF9BW125 01059 * DR2 - SF8BW125 01060 * DR3 - SF7BW125 01061 * DR4 - SF8BW500 01062 * 01063 * AU915 Datarates 01064 * --------------- 01065 * DR0 - SF10BW125 01066 * DR1 - SF9BW125 01067 * DR2 - SF8BW125 01068 * DR3 - SF7BW125 01069 * DR4 - SF8BW500 01070 * 01071 * @returns spreading factor and bandwidth 01072 */ 01073 std::string getDataRateDetails(uint8_t rate); 01074 MBED_DEPRECATED("Will be removed in 3.3.0") 01075 std::string getDateRateDetails(uint8_t rate); 01076 01077 01078 /** 01079 * Set TX power output of radio before antenna gain, default: 14 dBm 01080 * actual output power may be limited by local regulations for the chosen frequency 01081 * power affects maximum range 01082 * @param power 2 dBm - 20 dBm 01083 * @returns MDOT_OK if success 01084 */ 01085 int32_t setTxPower(const uint32_t& power); 01086 01087 /** 01088 * Get TX power 01089 * @returns TX power (2 dBm - 20 dBm) 01090 */ 01091 uint32_t getTxPower(); 01092 01093 /** 01094 * Get configured gain of installed antenna, default: +3 dBi 01095 * @returns gain of antenna in dBi 01096 */ 01097 int8_t getAntennaGain(); 01098 01099 /** 01100 * Set configured gain of installed antenna, default: +3 dBi 01101 * @param gain -127 dBi - 128 dBi 01102 * @returns MDOT_OK if success 01103 */ 01104 int32_t setAntennaGain(int8_t gain); 01105 01106 /** 01107 * Enable/disable TX waiting for rx windows 01108 * when enabled, send calls will block until a packet is received or RX timeout 01109 * @param enable set to true if expecting responses to transmitted packets 01110 * @returns MDOT_OK if success 01111 */ 01112 int32_t setTxWait(const bool& enable); 01113 01114 /** 01115 * Get TX wait 01116 * @returns true if TX wait is enabled 01117 */ 01118 bool getTxWait(); 01119 01120 /** 01121 * Cancel pending rx windows 01122 */ 01123 void cancelRxWindow(); 01124 01125 /** 01126 * Get time on air 01127 * @returns the amount of time (in ms) it would take to send bytes bytes based on current configuration 01128 */ 01129 uint32_t getTimeOnAir(uint8_t bytes); 01130 01131 /** 01132 * Get min frequency 01133 * @returns minimum frequency based on current channel plan 01134 */ 01135 uint32_t getMinFrequency(); 01136 01137 /** 01138 * Get max frequency 01139 * @returns maximum frequency based on current channel plan 01140 */ 01141 uint32_t getMaxFrequency(); 01142 01143 /** 01144 * Get min datarate 01145 * @returns minimum datarate based on current channel plan 01146 */ 01147 uint8_t getMinDatarate(); 01148 01149 /** 01150 * Get max datarate 01151 * @returns maximum datarate based on current channel plan 01152 */ 01153 uint8_t getMaxDatarate(); 01154 01155 /** 01156 * Get min datarate offset 01157 * @returns minimum datarate offset based on current channel plan 01158 */ 01159 uint8_t getMinDatarateOffset(); 01160 01161 /** 01162 * Get max datarate offset 01163 * @returns maximum datarate based on current channel plan 01164 */ 01165 uint8_t getMaxDatarateOffset(); 01166 01167 /** 01168 * Get min datarate 01169 * @returns minimum datarate based on current channel plan 01170 */ 01171 uint8_t getMinRx2Datarate(); 01172 01173 /** 01174 * Get max rx2 datarate 01175 * @returns maximum rx2 datarate based on current channel plan 01176 */ 01177 uint8_t getMaxRx2Datarate(); 01178 01179 /** 01180 * Get max tx power 01181 * @returns maximum tx power based on current channel plan 01182 */ 01183 uint8_t getMaxTxPower(); 01184 01185 /** 01186 * Get min tx power 01187 * @returns minimum tx power based on current channel plan 01188 */ 01189 uint8_t getMinTxPower(); 01190 01191 /** 01192 * Set ping slot periodicity 01193 * Specify the the number of ping slots in a given beacon interval 01194 * Note: Must switch back to class A for the change to take effect 01195 * @param exp - number_of_pings = 2^(7 - exp) where 0 <= exp <= 7 01196 * @returns MDOT_OK if success 01197 */ 01198 uint32_t setPingPeriodicity(uint8_t exp); 01199 01200 /** 01201 * Get ping slot periodicity 01202 * @returns exp = 7 - log2(number_of_pings) 01203 */ 01204 uint8_t getPingPeriodicity(); 01205 01206 /** 01207 * 01208 * get/set adaptive data rate 01209 * configure data rates and power levels based on signal to noise of packets received at gateway 01210 * true == adaptive data rate is on 01211 * set function returns MDOT_OK if success 01212 */ 01213 int32_t setAdr(const bool& on); 01214 bool getAdr(); 01215 01216 /** 01217 * Set the ADR ACK Limit 01218 * @param limit - ADR ACK limit 01219 */ 01220 void setAdrAckLimit(uint16_t limit); 01221 01222 /** 01223 * Get the ADR ACK Limit 01224 * @returns ADR ACK limit 01225 */ 01226 uint16_t getAdrAckLimit(); 01227 01228 /** 01229 * Set the ADR ACK Delay 01230 * @param delay - ADR ACK delay 01231 */ 01232 void setAdrAckDelay(uint16_t delay); 01233 01234 /** 01235 * Get the ADR ACK Delay 01236 * @returns ADR ACK delay 01237 */ 01238 uint16_t getAdrAckDelay(); 01239 01240 /** 01241 * Enable/disable CRC checking of packets 01242 * CRC checking must be enabled for use with Conduit gateway and MTAC_LORA card 01243 * @param on set to true to enable CRC checking 01244 * @returns MDOT_OK if success 01245 */ 01246 int32_t setCrc(const bool& on); 01247 01248 /** 01249 * Get CRC checking 01250 * @returns true if CRC checking is enabled 01251 */ 01252 bool getCrc(); 01253 01254 /** 01255 * Set ack 01256 * @param retries 0 to disable acks, otherwise 1 - 8 01257 * @returns MDOT_OK if success 01258 */ 01259 int32_t setAck(const uint8_t& retries); 01260 01261 /** 01262 * Get ack 01263 * @returns 0 if acks are disabled, otherwise retries (1 - 8) 01264 */ 01265 uint8_t getAck(); 01266 01267 /** 01268 * Set number of packet repeats for unconfirmed frames 01269 * @param repeat 0 or 1 for no repeats, otherwise 2-15 01270 * @returns MDOT_OK if success 01271 */ 01272 int32_t setRepeat(const uint8_t& repeat); 01273 01274 /** 01275 * Get number of packet repeats for unconfirmed frames 01276 * @returns 0 or 1 if no repeats, otherwise 2-15 01277 */ 01278 uint8_t getRepeat(); 01279 01280 /** 01281 * Send data to the gateway 01282 * validates data size (based on spreading factor) 01283 * @param data a vector of up to 242 bytes (may be less based on spreading factor) 01284 * @returns MDOT_OK if packet was sent successfully (ACKs disabled), or if an ACK was received (ACKs enabled) 01285 */ 01286 int32_t send(const std::vector<uint8_t>& data, const bool& blocking = true, const bool& highBw = false); 01287 01288 /** 01289 * Inject mac command 01290 * @param data a vector containing mac commands 01291 * @returns MDOT_OK 01292 */ 01293 int32_t injectMacCommand(const std::vector<uint8_t>& data); 01294 01295 /** 01296 * Clear MAC command buffer to be sent in next uplink 01297 * @returns MDOT_OK 01298 */ 01299 int32_t clearMacCommands(); 01300 01301 /** 01302 * Get MAC command buffer to be sent in next uplink 01303 * @returns command bytes 01304 */ 01305 std::vector<uint8_t> getMacCommands(); 01306 01307 /** 01308 * Fetch data received from the gateway 01309 * this function only checks to see if a packet has been received - it does not open a receive window 01310 * send() must be called before recv() 01311 * @param data a vector to put the received data into 01312 * @returns MDOT_OK if packet was successfully received 01313 */ 01314 int32_t recv(std::vector<uint8_t>& data); 01315 01316 /** 01317 * Ping 01318 * status will be MDOT_OK if ping succeeded 01319 * @returns ping_response struct containing status, RSSI, and SNR 01320 */ 01321 ping_response ping(); 01322 01323 /** 01324 * Get return code string 01325 * @returns string containing a description of the given error code 01326 */ 01327 static std::string getReturnCodeString(const int32_t& code); 01328 01329 /** 01330 * Get last error 01331 * @returns string explaining the last error that occured 01332 */ 01333 std::string getLastError(); 01334 01335 /** 01336 * Go to sleep 01337 * @param interval the number of seconds to sleep before waking up if wakeup_mode == RTC_ALARM or RTC_ALARM_OR_INTERRUPT, else ignored 01338 * @param wakeup_mode RTC_ALARM, INTERRUPT, RTC_ALARM_OR_INTERRUPT 01339 * if RTC_ALARM the real time clock is configured to wake the device up after the specified interval 01340 * if INTERRUPT the device will wake up on the rising edge of the interrupt pin 01341 * if RTC_ALARM_OR_INTERRUPT the device will wake on the first event to occur 01342 * @param deepsleep if true go into deep sleep mode (lowest power, all memory and registers are lost, peripherals turned off) 01343 * else go into sleep mode (low power, memory and registers are maintained, peripherals stay on) 01344 * 01345 * For the MDOT 01346 * in sleep mode, the device can be woken up on an XBEE_DI (2-8) pin or by the RTC alarm 01347 * in deepsleep mode, the device can only be woken up using the WKUP pin (PA0, XBEE_DIO7) or by the RTC alarm 01348 * For the XDOT 01349 * in sleep mode, the device can be woken up on GPIO (0-3), UART1_RX, WAKE or by the RTC alarm 01350 * in deepsleep mode, the device can only be woken up using the WKUP pin (PA0, WAKE) or by the RTC alarm 01351 * @returns MDOT_OK on success 01352 */ 01353 int32_t sleep(const uint32_t& interval, const uint8_t& wakeup_mode = RTC_ALARM, const bool& deepsleep = true); 01354 01355 /** 01356 * Set wake pin 01357 * @param pin the pin to use to wake the device from sleep mode 01358 * For MDOT, XBEE_DI (2-8) 01359 * For XDOT, GPIO (0-3), UART1_RX, or WAKE 01360 */ 01361 void setWakePin(const PinName& pin); 01362 01363 /** 01364 * Get wake pin 01365 * @returns the pin to use to wake the device from sleep mode 01366 * For MDOT, XBEE_DI (2-8) 01367 * For XDOT, GPIO (0-3), UART1_RX, or WAKE 01368 */ 01369 PinName getWakePin(); 01370 01371 /** 01372 * Write data in a user backup register 01373 * @param register one of UBR0 through UBR9 for MDOT, one of UBR0 through UBR21 for XDOT 01374 * @param data user data to back up 01375 * @returns true if success 01376 */ 01377 bool writeUserBackupRegister(uint32_t reg, uint32_t data); 01378 01379 /** 01380 * Read data in a user backup register 01381 * @param register one of UBR0 through UBR9 for MDOT, one of UBR0 through UBR21 for XDOT 01382 * @param data gets set to content of register 01383 * @returns true if success 01384 */ 01385 bool readUserBackupRegister(uint32_t reg, uint32_t& data); 01386 01387 /** 01388 * Set LBT time in us 01389 * @param ms time in us 01390 * @returns true if success 01391 */ 01392 bool setLbtTimeUs(uint16_t us); 01393 01394 /** 01395 * Get LBT time in us 01396 * @returns LBT time in us 01397 */ 01398 uint16_t getLbtTimeUs(); 01399 01400 /** 01401 * Set LBT threshold in dBm 01402 * @param rssi threshold in dBm 01403 * @returns true if success 01404 */ 01405 bool setLbtThreshold(int8_t rssi); 01406 01407 /** 01408 * Get LBT threshold in dBm 01409 * @returns LBT threshold in dBm 01410 */ 01411 int8_t getLbtThreshold(); 01412 01413 /** 01414 * Get Radio Frequency Offset 01415 * Used for fine calibration of radio frequencies 01416 * @returns frequency offset in MHz 01417 */ 01418 int32_t getFrequencyOffset(); 01419 /** 01420 * Get Radio Frequency Offset 01421 * Used for fine calibration of radio frequencies 01422 * @param offset frequency offset in MHz 01423 */ 01424 void setFrequencyOffset(int32_t offset); 01425 01426 01427 #if defined(TARGET_MTS_MDOT_F411RE) 01428 /////////////////////////////////////////////////////////////////// 01429 // Filesystem (Non Volatile Memory) Operation Functions for mDot // 01430 /////////////////////////////////////////////////////////////////// 01431 01432 // Save user file data to flash 01433 // file - name of file max 30 chars 01434 // data - data of file 01435 // size - size of file 01436 // returns true if successful 01437 bool saveUserFile(const char* file, void* data, uint32_t size); 01438 01439 // Append user file data to flash 01440 // file - name of file max 30 chars 01441 // data - data of file 01442 // size - size of file 01443 // returns true if successful 01444 bool appendUserFile(const char* file, void* data, uint32_t size); 01445 01446 // Read user file data from flash 01447 // file - name of file max 30 chars 01448 // data - data of file 01449 // size - size of file 01450 // returns true if successful 01451 bool readUserFile(const char* file, void* data, uint32_t size); 01452 01453 // Move a user file in flash 01454 // file - name of file 01455 // new_name - new name of file 01456 // returns true if successful 01457 bool moveUserFile(const char* file, const char* new_name); 01458 01459 // Delete user file data from flash 01460 // file - name of file max 30 chars 01461 // returns true if successful 01462 bool deleteUserFile(const char* file); 01463 01464 // Open user file in flash, max of 4 files open concurrently 01465 // file - name of file max 30 chars 01466 // mode - combination of FM_APPEND | FM_TRUNC | FM_CREAT | 01467 // FM_RDONLY | FM_WRONLY | FM_RDWR | FM_DIRECT 01468 // returns - mdot_file struct, fd field will be a negative value if file could not be opened 01469 mDot::mdot_file openUserFile(const char* file, int mode); 01470 01471 // Seek an open file 01472 // file - mdot file struct 01473 // offset - offset in bytes 01474 // whence - where offset is based SEEK_SET, SEEK_CUR, SEEK_END 01475 // returns true if successful 01476 bool seekUserFile(mDot::mdot_file& file, size_t offset, int whence); 01477 01478 // Read bytes from open file 01479 // file - mdot file struct 01480 // data - mem location to store data 01481 // length - number of bytes to read 01482 // returns - number of bytes read, negative if error 01483 int readUserFile(mDot::mdot_file& file, void* data, size_t length); 01484 01485 // Write bytes to open file 01486 // file - mdot file struct 01487 // data - data to write 01488 // length - number of bytes to write 01489 // returns - number of bytes written, negative if error 01490 int writeUserFile(mDot::mdot_file& file, void* data, size_t length); 01491 01492 // Close open file 01493 // file - mdot file struct 01494 // returns true if successful 01495 bool closeUserFile(mDot::mdot_file& file); 01496 01497 // List user files stored in flash 01498 std::vector<mDot::mdot_file> listUserFiles(); 01499 01500 // Move file into the firmware upgrade path to be flashed on next boot 01501 // file - name of file 01502 // returns true if successful 01503 bool moveUserFileToFirmwareUpgrade(const char* file); 01504 #else 01505 /////////////////////////////////////////////////////////////// 01506 // EEPROM (Non Volatile Memory) Operation Functions for xDot // 01507 /////////////////////////////////////////////////////////////// 01508 01509 // Write to EEPROM 01510 // addr - address to write to (0 - 0x17FF) 01511 // data - data to write 01512 // size - size of data 01513 // returns true if successful 01514 bool nvmWrite(uint16_t addr, void* data, uint16_t size); 01515 01516 // Read from EEPROM 01517 // addr - address to read from (0 - 0x17FF) 01518 // data - buffer for data 01519 // size - size of buffer 01520 // returns true if successful 01521 bool nvmRead(uint16_t addr, void* data, uint16_t size); 01522 #endif /* TARGET_MTS_MDOT_F411RE */ 01523 01524 // get current statistics 01525 // Join Attempts, Join Fails, Up Packets, Down Packets, Missed Acks 01526 mdot_stats getStats(); 01527 01528 // reset statistics 01529 // Join Attempts, Join Fails, Up Packets, Down Packets, Missed Acks 01530 void resetStats(); 01531 01532 // Convert pin number 2-8 to pin name DIO2-DI8 01533 static PinName pinNum2Name(uint8_t num); 01534 01535 // Convert pin name DIO2-DI8 to pin number 2-8 01536 static uint8_t pinName2Num(PinName name); 01537 01538 // Convert pin name DIO2-DI8 to string 01539 static std::string pinName2Str(PinName name); 01540 01541 uint64_t crc64(uint64_t crc, const unsigned char *s, uint64_t l); 01542 01543 /************************************************************************* 01544 * The following functions are only used by the AT command application and 01545 * should not be used by standard applications consuming the mDot library 01546 ************************************************************************/ 01547 01548 // set/get configured baud rate for command port 01549 // only for use in conjunction with AT interface 01550 // set function returns MDOT_OK if success 01551 int32_t setBaud(const uint32_t& baud); 01552 uint32_t getBaud(); 01553 01554 // set/get baud rate for debug port 01555 // set function returns MDOT_OK if success 01556 int32_t setDebugBaud(const uint32_t& baud); 01557 uint32_t getDebugBaud(); 01558 01559 // set/get command terminal echo 01560 // set function returns MDOT_OK if success 01561 int32_t setEcho(const bool& on); 01562 bool getEcho(); 01563 01564 // set/get command terminal verbose mode 01565 // set function returns MDOT_OK if success 01566 int32_t setVerbose(const bool& on); 01567 bool getVerbose(); 01568 01569 // set/get startup mode 01570 // COMMAND_MODE (default), starts up ready to accept AT commands 01571 // SERIAL_MODE, read serial data and send it as LoRa packets 01572 // set function returns MDOT_OK if success 01573 int32_t setStartUpMode(const uint8_t& mode); 01574 uint8_t getStartUpMode(); 01575 01576 int32_t setRxDataRate(const uint8_t& dr); 01577 uint8_t getRxDataRate(); 01578 01579 // get/set TX/RX frequency 01580 // if set to 0, device will hop frequencies 01581 // set function returns MDOT_OK if success 01582 int32_t setTxFrequency(const uint32_t& freq); 01583 uint32_t getTxFrequency(); 01584 int32_t setRxFrequency(const uint32_t& freq); 01585 uint32_t getRxFrequency(); 01586 01587 // get/set RX output mode 01588 // valid options are HEXADECIMAL, BINARY, and EXTENDED 01589 // set function returns MDOT_OK if success 01590 int32_t setRxOutput(const uint8_t& mode); 01591 uint8_t getRxOutput(); 01592 01593 // get/set serial wake interval 01594 // valid values are 2 s - INT_MAX (2147483647) s 01595 // set function returns MDOT_OK if success 01596 int32_t setWakeInterval(const uint32_t& interval); 01597 uint32_t getWakeInterval(); 01598 01599 // get/set serial wake delay 01600 // valid values are 2 ms - INT_MAX (2147483647) ms 01601 // set function returns MDOT_OK if success 01602 int32_t setWakeDelay(const uint32_t& delay); 01603 uint32_t getWakeDelay(); 01604 01605 // get/set serial receive timeout 01606 // valid values are 0 ms - 65000 ms 01607 // set function returns MDOT_OK if success 01608 int32_t setWakeTimeout(const uint16_t& timeout); 01609 uint16_t getWakeTimeout(); 01610 01611 // get/set serial wake mode 01612 // valid values are INTERRUPT or RTC_ALARM 01613 // set function returns MDOT_OK if success 01614 int32_t setWakeMode(const uint8_t& delay); 01615 uint8_t getWakeMode(); 01616 01617 // get/set serial flow control enabled 01618 // set function returns MDOT_OK if success 01619 int32_t setFlowControl(const bool& on); 01620 bool getFlowControl(); 01621 01622 // get/set serial clear on error 01623 // if enabled the data read from the serial port will be discarded if it cannot be sent or if the send fails 01624 // set function returns MDOT_OK if success 01625 int32_t setSerialClearOnError(const bool& on); 01626 bool getSerialClearOnError(); 01627 01628 // MTS_RADIO_DEBUG_COMMANDS 01629 01630 /** 01631 * Disable Duty cycle 01632 * enables or disables the duty cycle limitations 01633 * **** ONLY TO BE USED FOR TESTINGS PURPOSES **** 01634 * **** ALL DEPLOYABLE CODE MUST ADHERE TO LOCAL REGULATIONS **** 01635 * **** THIS SETTING WILL NOT BE SAVED TO CONFIGURATION ***** 01636 * @param val true to disable duty-cycle (default:false) 01637 */ 01638 int32_t setDisableDutyCycle(bool val); 01639 01640 /** 01641 * Disable Duty cycle 01642 * **** ONLY TO BE USED FOR TESTINGS PURPOSES **** 01643 * **** ALL DEPLOYABLE CODE MUST ADHERE TO LOCAL REGULATIONS **** 01644 * **** THIS SETTING WILL NOT BE SAVED TO CONFIGURATION ***** 01645 * @return true if duty-cycle is disabled (default:false) 01646 */ 01647 uint8_t getDisableDutyCycle(); 01648 01649 /** 01650 * LBT RSSI 01651 * @return the current RSSI on the configured frequency (SetTxFrequency) using configured LBT Time 01652 */ 01653 int16_t lbtRssi(); 01654 01655 void openRxWindow(uint32_t timeout, uint8_t bandwidth = 0); 01656 void closeRxWindow(); 01657 void sendContinuous(bool enable=true, uint32_t timeout=0, uint32_t frequency=0, int8_t txpower=-1); 01658 int32_t setDeviceId(const std::vector<uint8_t>& id); 01659 int32_t setProtectedAppEUI(const std::vector<uint8_t>& appEUI); 01660 int32_t setProtectedAppKey(const std::vector<uint8_t>& appKey); 01661 int32_t setDefaultFrequencyBand(const uint8_t& band); 01662 bool saveProtectedConfig(); 01663 // resets the radio/mac/link 01664 void resetRadio(); 01665 int32_t setRadioMode(const uint8_t& mode); 01666 std::map<uint8_t, uint8_t> dumpRegisters(); 01667 void eraseFlash(); 01668 01669 void setWakeupCallback(void (*function)(void)); 01670 01671 template<typename T> 01672 void setWakeupCallback(T *object, void (T::*member)(void)) { 01673 _wakeup_callback.attach(object, member); 01674 } 01675 01676 lora::ChannelPlan* getChannelPlan(void); 01677 01678 uint32_t setRx2DataRate(uint8_t dr); 01679 uint8_t getRx2DataRate(); 01680 01681 void mcGroupKeys(uint8_t *mcKeyEncrypt, uint32_t addr, uint8_t groupId, uint32_t frame_count); 01682 private: 01683 void sleep_ms(uint32_t interval, 01684 uint8_t wakeup_mode = RTC_ALARM, 01685 bool deepsleep = true); 01686 01687 mdot_stats _stats; 01688 01689 FunctionPointer _wakeup_callback; 01690 01691 bool _standbyFlag; 01692 bool _testMode; 01693 uint8_t _savedPort; 01694 void handleTestModePacket(); 01695 lora::ChannelPlan* _plan; 01696 }; 01697 01698 #endif
Generated on Tue Jul 12 2022 18:19:47 by
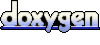