cpputest for mbed-6
Embed:
(wiki syntax)
Show/hide line numbers
TestRegistry.cpp
00001 /* 00002 * Copyright (c) 2007, Michael Feathers, James Grenning and Bas Vodde 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions are met: 00007 * * Redistributions of source code must retain the above copyright 00008 * notice, this list of conditions and the following disclaimer. 00009 * * Redistributions in binary form must reproduce the above copyright 00010 * notice, this list of conditions and the following disclaimer in the 00011 * documentation and/or other materials provided with the distribution. 00012 * * Neither the name of the <organization> nor the 00013 * names of its contributors may be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE EARLIER MENTIONED AUTHORS ``AS IS'' AND ANY 00017 * EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00019 * DISCLAIMED. IN NO EVENT SHALL <copyright holder> BE LIABLE FOR ANY 00020 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00021 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00022 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00023 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00024 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00025 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00026 */ 00027 00028 #include "CppUTest/TestHarness.h" 00029 #include "CppUTest/TestRegistry.h" 00030 00031 TestRegistry::TestRegistry() : 00032 tests_(&NullTestShell::instance()), firstPlugin_(NullTestPlugin::instance()), runInSeperateProcess_(false) 00033 { 00034 } 00035 00036 TestRegistry::~TestRegistry() 00037 { 00038 } 00039 00040 void TestRegistry::addTest(UtestShell *test) 00041 { 00042 tests_ = test->addTest(tests_); 00043 } 00044 00045 void TestRegistry::runAllTests(TestResult& result) 00046 { 00047 bool groupStart = true; 00048 00049 result.testsStarted(); 00050 for (UtestShell *test = tests_; !test->isNull(); test = test->getNext()) { 00051 if (runInSeperateProcess_) test->setRunInSeperateProcess(); 00052 00053 if (groupStart) { 00054 result.currentGroupStarted(test); 00055 groupStart = false; 00056 } 00057 00058 result.setProgressIndicator(test->getProgressIndicator()); 00059 result.countTest(); 00060 if (testShouldRun(test, result)) { 00061 result.currentTestStarted(test); 00062 test->runOneTest(firstPlugin_, result); 00063 result.currentTestEnded(test); 00064 } 00065 00066 if (endOfGroup(test)) { 00067 groupStart = true; 00068 result.currentGroupEnded(test); 00069 } 00070 } 00071 result.testsEnded(); 00072 } 00073 00074 bool TestRegistry::endOfGroup(UtestShell* test) 00075 { 00076 return (test->isNull() || test->getGroup() != test->getNext()->getGroup()); 00077 } 00078 00079 int TestRegistry::countTests() 00080 { 00081 return tests_->countTests(); 00082 } 00083 00084 TestRegistry* TestRegistry::currentRegistry_ = 0; 00085 00086 TestRegistry* TestRegistry::getCurrentRegistry() 00087 { 00088 static TestRegistry registry; 00089 return (currentRegistry_ == 0) ? ®istry : currentRegistry_; 00090 } 00091 00092 void TestRegistry::setCurrentRegistry(TestRegistry* registry) 00093 { 00094 currentRegistry_ = registry; 00095 } 00096 00097 void TestRegistry::unDoLastAddTest() 00098 { 00099 tests_ = tests_->getNext(); 00100 00101 } 00102 00103 void TestRegistry::nameFilter(const TestFilter& f) 00104 { 00105 nameFilter_ = f; 00106 } 00107 00108 void TestRegistry::groupFilter(const TestFilter& f) 00109 { 00110 groupFilter_ = f; 00111 } 00112 00113 TestFilter TestRegistry::getGroupFilter() 00114 { 00115 return groupFilter_; 00116 } 00117 00118 TestFilter TestRegistry::getNameFilter() 00119 { 00120 return nameFilter_; 00121 } 00122 00123 void TestRegistry::setRunTestsInSeperateProcess() 00124 { 00125 runInSeperateProcess_ = true; 00126 } 00127 00128 00129 bool TestRegistry::testShouldRun(UtestShell* test, TestResult& result) 00130 { 00131 if (test->shouldRun(groupFilter_, nameFilter_)) return true; 00132 else { 00133 result.countFilteredOut(); 00134 return false; 00135 } 00136 } 00137 00138 void TestRegistry::resetPlugins() 00139 { 00140 firstPlugin_ = NullTestPlugin::instance(); 00141 } 00142 00143 void TestRegistry::installPlugin(TestPlugin* plugin) 00144 { 00145 firstPlugin_ = plugin->addPlugin(firstPlugin_); 00146 } 00147 00148 TestPlugin* TestRegistry::getFirstPlugin() 00149 { 00150 return firstPlugin_; 00151 } 00152 00153 TestPlugin* TestRegistry::getPluginByName(const SimpleString& name) 00154 { 00155 return firstPlugin_->getPluginByName(name); 00156 } 00157 00158 void TestRegistry::removePluginByName(const SimpleString& name) 00159 { 00160 if (firstPlugin_->removePluginByName(name) == firstPlugin_) firstPlugin_ = firstPlugin_->getNext(); 00161 if (firstPlugin_->getName() == name) firstPlugin_ = firstPlugin_->getNext(); 00162 firstPlugin_->removePluginByName(name); 00163 } 00164 00165 int TestRegistry::countPlugins() 00166 { 00167 int count = 0; 00168 for (TestPlugin* plugin = firstPlugin_; plugin != NullTestPlugin::instance(); plugin = plugin->getNext()) 00169 count++; 00170 return count; 00171 } 00172 00173 00174 UtestShell* TestRegistry::getFirstTest() 00175 { 00176 return tests_; 00177 } 00178 00179 UtestShell* TestRegistry::getLastTest() 00180 { 00181 UtestShell* current = tests_; 00182 while (!current->getNext()->isNull()) 00183 current = current->getNext(); 00184 return current; 00185 } 00186 00187 UtestShell* TestRegistry::getTestWithNext(UtestShell* test) 00188 { 00189 UtestShell* current = tests_; 00190 while (!current->getNext()->isNull() && current->getNext() != test) 00191 current = current->getNext(); 00192 return current; 00193 } 00194 00195 UtestShell* TestRegistry::findTestWithName(const SimpleString& name) 00196 { 00197 UtestShell* current = tests_; 00198 while (!current->isNull()) { 00199 if (current->getName() == name) 00200 return current; 00201 current = current->getNext(); 00202 } 00203 return NULL; 00204 } 00205 00206 UtestShell* TestRegistry::findTestWithGroup(const SimpleString& group) 00207 { 00208 UtestShell* current = tests_; 00209 while (!current->isNull()) { 00210 if (current->getGroup() == group) 00211 return current; 00212 current = current->getNext(); 00213 } 00214 return NULL; 00215 } 00216
Generated on Fri Jul 15 2022 01:46:33 by
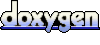