cpputest for mbed-6
Embed:
(wiki syntax)
Show/hide line numbers
TestPlugin.cpp
00001 /* 00002 * Copyright (c) 2007, Michael Feathers, James Grenning and Bas Vodde 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions are met: 00007 * * Redistributions of source code must retain the above copyright 00008 * notice, this list of conditions and the following disclaimer. 00009 * * Redistributions in binary form must reproduce the above copyright 00010 * notice, this list of conditions and the following disclaimer in the 00011 * documentation and/or other materials provided with the distribution. 00012 * * Neither the name of the <organization> nor the 00013 * names of its contributors may be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE EARLIER MENTIONED AUTHORS ``AS IS'' AND ANY 00017 * EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00019 * DISCLAIMED. IN NO EVENT SHALL <copyright holder> BE LIABLE FOR ANY 00020 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00021 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00022 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00023 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00024 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00025 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00026 */ 00027 00028 #include "CppUTest/TestHarness.h" 00029 #include "CppUTest/TestPlugin.h" 00030 00031 TestPlugin::TestPlugin(const SimpleString& name) : 00032 next_(NullTestPlugin::instance()), name_(name), enabled_(true) 00033 { 00034 } 00035 00036 TestPlugin::TestPlugin(TestPlugin* next) : 00037 next_(next), name_("null"), enabled_(true) 00038 { 00039 } 00040 00041 TestPlugin::~TestPlugin() 00042 { 00043 } 00044 00045 TestPlugin* TestPlugin::addPlugin(TestPlugin* plugin) 00046 { 00047 next_ = plugin; 00048 return this; 00049 } 00050 00051 void TestPlugin::runAllPreTestAction(UtestShell& test, TestResult& result) 00052 { 00053 if (enabled_) preTestAction(test, result); 00054 next_->runAllPreTestAction(test, result); 00055 } 00056 00057 void TestPlugin::runAllPostTestAction(UtestShell& test, TestResult& result) 00058 { 00059 next_ ->runAllPostTestAction(test, result); 00060 if (enabled_) postTestAction(test, result); 00061 } 00062 00063 bool TestPlugin::parseAllArguments(int ac, char** av, int index) 00064 { 00065 return parseAllArguments(ac, const_cast<const char**> (av), index); 00066 } 00067 00068 bool TestPlugin::parseAllArguments(int ac, const char** av, int index) 00069 { 00070 if (parseArguments(ac, av, index)) return true; 00071 if (next_) return next_->parseAllArguments(ac, av, index); 00072 return false; 00073 } 00074 00075 const SimpleString& TestPlugin::getName() 00076 { 00077 return name_; 00078 } 00079 00080 TestPlugin* TestPlugin::getPluginByName(const SimpleString& name) 00081 { 00082 if (name == name_) return this; 00083 if (next_) return next_->getPluginByName(name); 00084 return (next_); 00085 } 00086 00087 TestPlugin* TestPlugin::getNext() 00088 { 00089 return next_; 00090 } 00091 TestPlugin* TestPlugin::removePluginByName(const SimpleString& name) 00092 { 00093 TestPlugin* removed = 0; 00094 if (next_ && next_->getName() == name) { 00095 removed = next_; 00096 next_ = next_->next_; 00097 } 00098 return removed; 00099 } 00100 00101 void TestPlugin::disable() 00102 { 00103 enabled_ = false; 00104 } 00105 00106 void TestPlugin::enable() 00107 { 00108 enabled_ = true; 00109 } 00110 00111 bool TestPlugin::isEnabled() 00112 { 00113 return enabled_; 00114 } 00115 00116 struct cpputest_pair 00117 { 00118 void **orig; 00119 void *orig_value; 00120 }; 00121 00122 //////// SetPlugin 00123 00124 static int pointerTableIndex; 00125 static cpputest_pair setlist[SetPointerPlugin::MAX_SET]; 00126 00127 SetPointerPlugin::SetPointerPlugin(const SimpleString& name) : 00128 TestPlugin(name) 00129 { 00130 pointerTableIndex = 0; 00131 } 00132 00133 SetPointerPlugin::~SetPointerPlugin() 00134 { 00135 } 00136 00137 void CppUTestStore(void**function) 00138 { 00139 if (pointerTableIndex >= SetPointerPlugin::MAX_SET) { 00140 FAIL("Maximum number of function pointers installed!"); 00141 } 00142 setlist[pointerTableIndex].orig_value = *function; 00143 setlist[pointerTableIndex].orig = function; 00144 pointerTableIndex++; 00145 } 00146 00147 void SetPointerPlugin::postTestAction(UtestShell& /*test*/, TestResult& /*result*/) 00148 { 00149 for (int i = pointerTableIndex - 1; i >= 0; i--) 00150 *((void**) setlist[i].orig) = setlist[i].orig_value; 00151 pointerTableIndex = 0; 00152 } 00153 00154 //////// NullPlugin 00155 00156 NullTestPlugin::NullTestPlugin() : 00157 TestPlugin(0) 00158 { 00159 } 00160 00161 NullTestPlugin* NullTestPlugin::instance() 00162 { 00163 static NullTestPlugin _instance; 00164 return &_instance; 00165 } 00166 00167 void NullTestPlugin::runAllPreTestAction(UtestShell&, TestResult&) 00168 { 00169 } 00170 00171 void NullTestPlugin::runAllPostTestAction(UtestShell&, TestResult&) 00172 { 00173 }
Generated on Fri Jul 15 2022 01:46:33 by
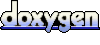