cpputest for mbed-6
Embed:
(wiki syntax)
Show/hide line numbers
TestHarness_c.h
00001 /* 00002 * Copyright (c) 2007, Michael Feathers, James Grenning and Bas Vodde 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions are met: 00007 * * Redistributions of source code must retain the above copyright 00008 * notice, this list of conditions and the following disclaimer. 00009 * * Redistributions in binary form must reproduce the above copyright 00010 * notice, this list of conditions and the following disclaimer in the 00011 * documentation and/or other materials provided with the distribution. 00012 * * Neither the name of the <organization> nor the 00013 * names of its contributors may be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE EARLIER MENTIONED AUTHORS ``AS IS'' AND ANY 00017 * EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00019 * DISCLAIMED. IN NO EVENT SHALL <copyright holder> BE LIABLE FOR ANY 00020 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00021 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00022 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00023 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00024 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00025 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00026 */ 00027 00028 /****************************************************************************** 00029 * 00030 * Provides an interface for when working with pure C 00031 * 00032 *******************************************************************************/ 00033 00034 #ifndef D_TestHarness_c_h 00035 #define D_TestHarness_c_h 00036 00037 #include "CppUTestConfig.h" 00038 00039 #define CHECK_EQUAL_C_INT(expected,actual) \ 00040 CHECK_EQUAL_C_INT_LOCATION(expected,actual,__FILE__,__LINE__) 00041 00042 #define CHECK_EQUAL_C_REAL(expected,actual,threshold) \ 00043 CHECK_EQUAL_C_REAL_LOCATION(expected,actual,threshold,__FILE__,__LINE__) 00044 00045 #define CHECK_EQUAL_C_CHAR(expected,actual) \ 00046 CHECK_EQUAL_C_CHAR_LOCATION(expected,actual,__FILE__,__LINE__) 00047 00048 #define CHECK_EQUAL_C_STRING(expected,actual) \ 00049 CHECK_EQUAL_C_STRING_LOCATION(expected,actual,__FILE__,__LINE__) 00050 00051 #define FAIL_TEXT_C(text) \ 00052 FAIL_TEXT_C_LOCATION(text,__FILE__,__LINE__) 00053 00054 #define FAIL_C() \ 00055 FAIL_C_LOCATION(__FILE__,__LINE__) 00056 00057 #define CHECK_C(condition) \ 00058 CHECK_C_LOCATION(condition, #condition, __FILE__,__LINE__) 00059 00060 00061 /****************************************************************************** 00062 * 00063 * TEST macros for in C. 00064 * 00065 *******************************************************************************/ 00066 00067 /* For use in C file */ 00068 #define TEST_GROUP_C_SETUP(group_name) \ 00069 extern void group_##group_name##_setup_wrapper_c(void); \ 00070 void group_##group_name##_setup_wrapper_c() 00071 00072 #define TEST_GROUP_C_TEARDOWN(group_name) \ 00073 extern void group_##group_name##_teardown_wrapper_c(void); \ 00074 void group_##group_name##_teardown_wrapper_c() 00075 00076 #define TEST_C(group_name, test_name) \ 00077 extern void test_##group_name##_##test_name##_wrapper_c(void);\ 00078 void test_##group_name##_##test_name##_wrapper_c() 00079 00080 00081 /* For use in C++ file */ 00082 00083 #define TEST_GROUP_C(group_name) \ 00084 extern "C" { \ 00085 extern void group_##group_name##_setup_wrapper_c(void); \ 00086 extern void group_##group_name##_teardown_wrapper_c(void); \ 00087 } \ 00088 TEST_GROUP(group_name) 00089 00090 #define TEST_GROUP_C_SETUP_WRAPPER(group_name) \ 00091 void setup() { \ 00092 group_##group_name##_setup_wrapper_c(); \ 00093 } 00094 00095 #define TEST_GROUP_C_TEARDOWN_WRAPPER(group_name) \ 00096 void teardown() { \ 00097 group_##group_name##_teardown_wrapper_c(); \ 00098 } 00099 00100 #define TEST_GROUP_C_WRAPPER(group_name, test_name) \ 00101 extern "C" { \ 00102 extern void test_##group_name##_##test_name##_wrapper_c(); \ 00103 } \ 00104 TEST(group_name, test_name) { \ 00105 test_##group_name##_##test_name##_wrapper_c(); \ 00106 } 00107 00108 #ifdef __cplusplus 00109 extern "C" 00110 { 00111 #endif 00112 00113 00114 /* CHECKS that can be used from C code */ 00115 extern void CHECK_EQUAL_C_INT_LOCATION(int expected, int actual, 00116 const char* fileName, int lineNumber); 00117 extern void CHECK_EQUAL_C_REAL_LOCATION(double expected, double actual, 00118 double threshold, const char* fileName, int lineNumber); 00119 extern void CHECK_EQUAL_C_CHAR_LOCATION(char expected, char actual, 00120 const char* fileName, int lineNumber); 00121 extern void CHECK_EQUAL_C_STRING_LOCATION(const char* expected, 00122 const char* actual, const char* fileName, int lineNumber); 00123 extern void FAIL_TEXT_C_LOCATION(const char* text, const char* fileName, 00124 int lineNumber); 00125 extern void FAIL_C_LOCATION(const char* fileName, int lineNumber); 00126 extern void CHECK_C_LOCATION(int condition, const char* conditionString, 00127 const char* fileName, int lineNumber); 00128 00129 extern void* cpputest_malloc(size_t size); 00130 extern void* cpputest_calloc(size_t num, size_t size); 00131 extern void* cpputest_realloc(void* ptr, size_t size); 00132 extern void cpputest_free(void* buffer); 00133 00134 extern void* cpputest_malloc_location(size_t size, const char* file, int line); 00135 extern void* cpputest_calloc_location(size_t num, size_t size, 00136 const char* file, int line); 00137 extern void* cpputest_realloc_location(void* memory, size_t size, 00138 const char* file, int line); 00139 extern void cpputest_free_location(void* buffer, const char* file, int line); 00140 00141 void cpputest_malloc_set_out_of_memory(void); 00142 void cpputest_malloc_set_not_out_of_memory(void); 00143 void cpputest_malloc_set_out_of_memory_countdown(int); 00144 void cpputest_malloc_count_reset(void); 00145 int cpputest_malloc_get_count(void); 00146 00147 #ifdef __cplusplus 00148 } 00149 #endif 00150 00151 00152 /* 00153 * Small additional macro for unused arguments. This is common when stubbing, but in C you cannot remove the 00154 * name of the parameter (as in C++). 00155 */ 00156 00157 #ifndef PUNUSED 00158 #if defined(__GNUC__) 00159 # define PUNUSED(x) PUNUSED_ ##x __attribute__((unused)) 00160 #else 00161 # define PUNUSED(x) x 00162 #endif 00163 #endif 00164 00165 #endif
Generated on Fri Jul 15 2022 01:46:33 by
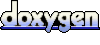