cpputest for mbed-6
Embed:
(wiki syntax)
Show/hide line numbers
TestFilter.cpp
00001 /* 00002 * Copyright (c) 2007, Michael Feathers, James Grenning and Bas Vodde 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions are met: 00007 * * Redistributions of source code must retain the above copyright 00008 * notice, this list of conditions and the following disclaimer. 00009 * * Redistributions in binary form must reproduce the above copyright 00010 * notice, this list of conditions and the following disclaimer in the 00011 * documentation and/or other materials provided with the distribution. 00012 * * Neither the name of the <organization> nor the 00013 * names of its contributors may be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE EARLIER MENTIONED AUTHORS ``AS IS'' AND ANY 00017 * EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00019 * DISCLAIMED. IN NO EVENT SHALL <copyright holder> BE LIABLE FOR ANY 00020 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00021 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00022 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00023 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00024 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00025 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00026 */ 00027 00028 #include "CppUTest/CppUTestConfig.h" 00029 #include "CppUTest/TestFilter.h" 00030 00031 TestFilter::TestFilter() : strictMatching_(false) 00032 { 00033 } 00034 00035 TestFilter::TestFilter(const SimpleString& filter) : strictMatching_(false) 00036 { 00037 filter_ = filter; 00038 } 00039 00040 TestFilter::TestFilter(const char* filter) : strictMatching_(false) 00041 { 00042 filter_ = filter; 00043 } 00044 00045 void TestFilter::strictMatching() 00046 { 00047 strictMatching_ = true; 00048 } 00049 00050 bool TestFilter::match(const SimpleString& name) const 00051 { 00052 if (strictMatching_) 00053 return name == filter_; 00054 return name.contains(filter_); 00055 } 00056 00057 bool TestFilter::operator==(const TestFilter& filter) const 00058 { 00059 return (filter_ == filter.filter_ && strictMatching_ == filter.strictMatching_); 00060 } 00061 00062 bool TestFilter::operator!=(const TestFilter& filter) const 00063 { 00064 return !(filter == *this); 00065 } 00066 00067 SimpleString TestFilter::asString() const 00068 { 00069 SimpleString textFilter = StringFromFormat("TestFilter: \"%s\"", filter_.asCharString()); 00070 if (strictMatching_) 00071 textFilter += " with strict matching"; 00072 return textFilter; 00073 } 00074 00075 SimpleString StringFrom(const TestFilter& filter) 00076 { 00077 return filter.asString(); 00078 } 00079
Generated on Fri Jul 15 2022 01:46:33 by
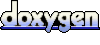