
Example programs for MultiTech Dot devices demonstrating how to use the Dot devices and the Dot libraries for LoRa communication.
Dependencies: ISL29011
Dependents: Dot-Examples-delujoc
peer_to_peer_example.cpp
00001 #include "dot_util.h" 00002 #include "RadioEvent.h" 00003 00004 #if ACTIVE_EXAMPLE == PEER_TO_PEER_EXAMPLE 00005 00006 ///////////////////////////////////////////////////////////////////////////// 00007 // -------------------- DOT LIBRARY REQUIRED ------------------------------// 00008 // * Because these example programs can be used for both mDot and xDot // 00009 // devices, the LoRa stack is not included. The libmDot library should // 00010 // be imported if building for mDot devices. The libxDot library // 00011 // should be imported if building for xDot devices. // 00012 // * https://developer.mbed.org/teams/MultiTech/code/libmDot-dev-mbed5/ // 00013 // * https://developer.mbed.org/teams/MultiTech/code/libmDot-mbed5/ // 00014 // * https://developer.mbed.org/teams/MultiTech/code/libxDot-dev-mbed5/ // 00015 // * https://developer.mbed.org/teams/MultiTech/code/libxDot-mbed5/ // 00016 ///////////////////////////////////////////////////////////////////////////// 00017 00018 ///////////////////////////////////////////////////////////// 00019 // * these options must match between the two devices in // 00020 // order for communication to be successful 00021 ///////////////////////////////////////////////////////////// 00022 static uint8_t network_address[] = { 0x01, 0x02, 0x03, 0x04 }; 00023 static uint8_t network_session_key[] = { 0x01, 0x02, 0x03, 0x04, 0x01, 0x02, 0x03, 0x04, 0x01, 0x02, 0x03, 0x04, 0x01, 0x02, 0x03, 0x04 }; 00024 static uint8_t data_session_key[] = { 0x01, 0x02, 0x03, 0x04, 0x01, 0x02, 0x03, 0x04, 0x01, 0x02, 0x03, 0x04, 0x01, 0x02, 0x03, 0x04 }; 00025 00026 mDot* dot = NULL; 00027 lora::ChannelPlan* plan = NULL; 00028 00029 mbed::UnbufferedSerial pc(USBTX, USBRX); 00030 00031 #if defined(TARGET_XDOT_L151CC) 00032 I2C i2c(I2C_SDA, I2C_SCL); 00033 ISL29011 lux(i2c); 00034 #else 00035 AnalogIn lux(XBEE_AD0); 00036 #endif 00037 00038 int main() { 00039 // Custom event handler for automatically displaying RX data 00040 RadioEvent events; 00041 uint32_t tx_frequency; 00042 uint8_t tx_datarate; 00043 uint8_t tx_power; 00044 uint8_t frequency_band; 00045 00046 pc.baud(115200); 00047 00048 #if defined(TARGET_XDOT_L151CC) 00049 i2c.frequency(400000); 00050 #endif 00051 00052 mts::MTSLog::setLogLevel(mts::MTSLog::TRACE_LEVEL); 00053 00054 // Create channel plan 00055 plan = create_channel_plan(); 00056 assert(plan); 00057 00058 dot = mDot::getInstance(plan); 00059 assert(dot); 00060 00061 logInfo("mbed-os library version: %d.%d.%d", MBED_MAJOR_VERSION, MBED_MINOR_VERSION, MBED_PATCH_VERSION); 00062 00063 // start from a well-known state 00064 logInfo("defaulting Dot configuration"); 00065 dot->resetConfig(); 00066 00067 // make sure library logging is turned on 00068 dot->setLogLevel(mts::MTSLog::INFO_LEVEL); 00069 00070 // attach the custom events handler 00071 dot->setEvents(&events); 00072 00073 // update configuration if necessary 00074 if (dot->getJoinMode() != mDot::PEER_TO_PEER) { 00075 logInfo("changing network join mode to PEER_TO_PEER"); 00076 if (dot->setJoinMode(mDot::PEER_TO_PEER) != mDot::MDOT_OK) { 00077 logError("failed to set network join mode to PEER_TO_PEER"); 00078 } 00079 } 00080 frequency_band = dot->getFrequencyBand(); 00081 switch (frequency_band) { 00082 case lora::ChannelPlan::EU868_OLD: 00083 case lora::ChannelPlan::EU868: 00084 // 250kHz channels achieve higher throughput 00085 // DR_6 : SF7 @ 250kHz 00086 // DR_0 - DR_5 (125kHz channels) available but much slower 00087 tx_frequency = 869850000; 00088 tx_datarate = lora::DR_6; 00089 // the 869850000 frequency is 100% duty cycle if the total power is under 7 dBm - tx power 4 + antenna gain 3 = 7 00090 tx_power = 4; 00091 break; 00092 00093 case lora::ChannelPlan::US915_OLD: 00094 case lora::ChannelPlan::US915: 00095 case lora::ChannelPlan::AU915_OLD: 00096 case lora::ChannelPlan::AU915: 00097 // 500kHz channels achieve highest throughput 00098 // DR_8 : SF12 @ 500kHz 00099 // DR_9 : SF11 @ 500kHz 00100 // DR_10 : SF10 @ 500kHz 00101 // DR_11 : SF9 @ 500kHz 00102 // DR_12 : SF8 @ 500kHz 00103 // DR_13 : SF7 @ 500kHz 00104 // DR_0 - DR_3 (125kHz channels) available but much slower 00105 tx_frequency = 915500000; 00106 tx_datarate = lora::DR_13; 00107 // 915 bands have no duty cycle restrictions, set tx power to max 00108 tx_power = 20; 00109 break; 00110 00111 case lora::ChannelPlan::AS923: 00112 case lora::ChannelPlan::AS923_JAPAN: 00113 // 250kHz channels achieve higher throughput 00114 // DR_6 : SF7 @ 250kHz 00115 // DR_0 - DR_5 (125kHz channels) available but much slower 00116 tx_frequency = 924800000; 00117 tx_datarate = lora::DR_6; 00118 tx_power = 16; 00119 break; 00120 00121 case lora::ChannelPlan::KR920: 00122 // DR_5 : SF7 @ 125kHz 00123 tx_frequency = 922700000; 00124 tx_datarate = lora::DR_5; 00125 tx_power = 14; 00126 break; 00127 00128 default: 00129 while (true) { 00130 logFatal("no known channel plan in use - extra configuration is needed!"); 00131 ThisThread::sleep_for(5s); 00132 } 00133 break; 00134 } 00135 // in PEER_TO_PEER mode there is no join request/response transaction 00136 // as long as both Dots are configured correctly, they should be able to communicate 00137 update_peer_to_peer_config(network_address, network_session_key, data_session_key, tx_frequency, tx_datarate, tx_power); 00138 00139 // save changes to configuration 00140 logInfo("saving configuration"); 00141 if (!dot->saveConfig()) { 00142 logError("failed to save configuration"); 00143 } 00144 00145 // display configuration 00146 display_config(); 00147 00148 while (true) { 00149 uint16_t light; 00150 std::vector<uint8_t> tx_data; 00151 00152 // join network if not joined 00153 if (!dot->getNetworkJoinStatus()) { 00154 join_network(); 00155 } 00156 00157 #if defined(TARGET_XDOT_L151CC) 00158 // configure the ISL29011 sensor on the xDot-DK for continuous ambient light sampling, 16 bit conversion, and maximum range 00159 lux.setMode(ISL29011::ALS_CONT); 00160 lux.setResolution(ISL29011::ADC_16BIT); 00161 lux.setRange(ISL29011::RNG_64000); 00162 00163 // get the latest light sample and send it to the gateway 00164 light = lux.getData(); 00165 tx_data.push_back((light >> 8) & 0xFF); 00166 tx_data.push_back(light & 0xFF); 00167 logInfo("light: %lu [0x%04X]", light, light); 00168 send_data(tx_data); 00169 00170 // put the LSL29011 ambient light sensor into a low power state 00171 lux.setMode(ISL29011::PWR_DOWN); 00172 #else 00173 // get some dummy data and send it to the gateway 00174 light = lux.read_u16(); 00175 tx_data.push_back((light >> 8) & 0xFF); 00176 tx_data.push_back(light & 0xFF); 00177 logInfo("light: %lu [0x%04X]", light, light); 00178 send_data(tx_data); 00179 #endif 00180 00181 // the Dot can't sleep in PEER_TO_PEER mode 00182 // it must be waiting for data from the other Dot 00183 // send data every 5 seconds 00184 logInfo("waiting for 5s"); 00185 ThisThread::sleep_for(5s); 00186 } 00187 00188 return 0; 00189 } 00190 00191 #endif
Generated on Tue Jul 12 2022 13:03:22 by
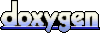