
Example programs for MultiTech Dot devices demonstrating how to use the Dot devices and the Dot libraries for LoRa communication.
Dependencies: ISL29011
Dependents: Dot-Examples-delujoc
fota_example.cpp
00001 #include "dot_util.h" 00002 #include "RadioEvent.h" 00003 00004 #if ACTIVE_EXAMPLE == FOTA_EXAMPLE 00005 00006 00007 #if defined(TARGET_XDOT_L151CC) 00008 //#include "SPIFBlockDevice.h" 00009 //#include "DataFlashBlockDevice.h" 00010 #endif 00011 00012 ///////////////////////////////////////////////////////////////////////////// 00013 // -------------------- DOT LIBRARY REQUIRED ------------------------------// 00014 // * Because these example programs can be used for both mDot and xDot // 00015 // devices, the LoRa stack is not included. The libmDot library should // 00016 // be imported if building for mDot devices. The libxDot library // 00017 // should be imported if building for xDot devices. // 00018 // * https://developer.mbed.org/teams/MultiTech/code/libmDot-dev-mbed5/ // 00019 // * https://developer.mbed.org/teams/MultiTech/code/libmDot-mbed5/ // 00020 // * https://developer.mbed.org/teams/MultiTech/code/libxDot-dev-mbed5/ // 00021 // * https://developer.mbed.org/teams/MultiTech/code/libxDot-mbed5/ // 00022 ///////////////////////////////////////////////////////////////////////////// 00023 00024 00025 //////////////////////////////////////////////////////////////////////////// 00026 // -------------------- DEFINITIONS REQUIRED ---------------------------- // 00027 // Add define for FOTA in mbed_app.json or on command line // 00028 // Command line // 00029 // mbed compile -t GCC_ARM -m MTS_MDOT_F411RE -DFOTA=1 // 00030 // mbed_app.json // 00031 // { // 00032 // "macros": [ // 00033 // "FOTA" // 00034 // ] // 00035 // } // 00036 // // 00037 //////////////////////////////////////////////////////////////////////////// 00038 00039 00040 //////////////////////////////////////////////////////////////////////////// 00041 // -------------------------- XDOT EXTERNAL STORAGE --------------------- // 00042 // An external storage device is required for FOTA on an XDot. The // 00043 // storage device must meet the following criteria: // 00044 // * Work with MBed OS DataFlashBlockDevice or SPIFBlockDevice classes // 00045 // * Maximum 4KB sector erase size // 00046 // * Maximum 512 byte page size // 00047 // * SPIF type components must support Serial Flash Discoverable // 00048 // Parameters (SFDP) // 00049 // // 00050 // Refer to mbed_app.json included in this project for configuration // 00051 // parameters requried for external storage. // 00052 // // 00053 // Modify code below to create a BlockDevice object. // 00054 //////////////////////////////////////////////////////////////////////////// 00055 00056 00057 ///////////////////////////////////////////////////////////// 00058 // * these options must match the settings on your gateway // 00059 // * edit their values to match your configuration // 00060 // * frequency sub band is only relevant for the 915 bands // 00061 // * either the network name and passphrase can be used or // 00062 // the network ID (8 bytes) and KEY (16 bytes) // 00063 ///////////////////////////////////////////////////////////// 00064 00065 static std::string network_name = "MultiTech"; 00066 static std::string network_passphrase = "MultiTech"; 00067 static uint8_t network_id[] = { 0x6C, 0x4E, 0xEF, 0x66, 0xF4, 0x79, 0x86, 0xA6 }; 00068 static uint8_t network_key[] = { 0x1F, 0x33, 0xA1, 0x70, 0xA5, 0xF1, 0xFD, 0xA0, 0xAB, 0x69, 0x7A, 0xAE, 0x2B, 0x95, 0x91, 0x6B }; 00069 static uint8_t frequency_sub_band = 0; 00070 static lora::NetworkType network_type = lora::PUBLIC_LORAWAN; 00071 static uint8_t join_delay = 5; 00072 static uint8_t ack = 1; 00073 static bool adr = true; 00074 00075 mDot* dot = NULL; 00076 lora::ChannelPlan* plan = NULL; 00077 00078 mbed::UnbufferedSerial pc(USBTX, USBRX); 00079 00080 #if defined(TARGET_XDOT_L151CC) 00081 I2C i2c(I2C_SDA, I2C_SCL); 00082 ISL29011 lux(i2c); 00083 #else 00084 AnalogIn lux(XBEE_AD0); 00085 #endif 00086 00087 int main() { 00088 // Custom event handler for automatically displaying RX data 00089 RadioEvent events; 00090 00091 pc.baud(115200); 00092 00093 #if defined(TARGET_XDOT_L151CC) 00094 i2c.frequency(400000); 00095 #endif 00096 00097 mts::MTSLog::setLogLevel(mts::MTSLog::TRACE_LEVEL); 00098 00099 // Create channel plan 00100 plan = create_channel_plan(); 00101 assert(plan); 00102 00103 #if defined(TARGET_XDOT_L151CC) 00104 00105 mbed::BlockDevice* ext_bd = NULL; 00106 00107 // XDot requires an external storage device for FOTA (see above). 00108 // If one is connected provide the block device object to the mDot instance. 00109 // 00110 // ** Uncomment the appropriate block device here and include statement above 00111 // 00112 //ext_bd = new SPIFBlockDevice(); 00113 //ext_bd = new DataFlashBlockDevice(); 00114 ext_bd->init(); 00115 dot = mDot::getInstance(plan, ext_bd); 00116 #else 00117 dot = mDot::getInstance(plan); 00118 #endif 00119 assert(dot); 00120 00121 logInfo("mbed-os library version: %d.%d.%d", MBED_MAJOR_VERSION, MBED_MINOR_VERSION, MBED_PATCH_VERSION); 00122 00123 // Initialize FOTA singleton 00124 Fota::getInstance(dot); 00125 00126 00127 // start from a well-known state 00128 logInfo("defaulting Dot configuration"); 00129 dot->resetConfig(); 00130 dot->resetNetworkSession(); 00131 00132 // make sure library logging is turned on 00133 dot->setLogLevel(mts::MTSLog::INFO_LEVEL); 00134 00135 // attach the custom events handler 00136 dot->setEvents(&events); 00137 00138 // update configuration if necessary 00139 if (dot->getJoinMode() != mDot::OTA) { 00140 logInfo("changing network join mode to OTA"); 00141 if (dot->setJoinMode(mDot::OTA) != mDot::MDOT_OK) { 00142 logError("failed to set network join mode to OTA"); 00143 } 00144 } 00145 // in OTA and AUTO_OTA join modes, the credentials can be passed to the library as a name and passphrase or an ID and KEY 00146 // only one method or the other should be used! 00147 // network ID = crc64(network name) 00148 // network KEY = cmac(network passphrase) 00149 update_ota_config_name_phrase(network_name, network_passphrase, frequency_sub_band, network_type, ack); 00150 //update_ota_config_id_key(network_id, network_key, frequency_sub_band, network_type, ack); 00151 00152 // configure the Dot for class C operation 00153 // the Dot must also be configured on the gateway for class C 00154 // use the lora-query application to do this on a Conduit: http://www.multitech.net/developer/software/lora/lora-network-server/ 00155 // to provision your Dot for class C operation with a 3rd party gateway, see the gateway or network provider documentation 00156 logInfo("changing network mode to class C"); 00157 if (dot->setClass("C") != mDot::MDOT_OK) { 00158 logError("failed to set network mode to class C"); 00159 } 00160 00161 // enable or disable Adaptive Data Rate 00162 dot->setAdr(adr); 00163 00164 // Configure the join delay 00165 dot->setJoinDelay(join_delay); 00166 00167 // save changes to configuration 00168 logInfo("saving configuration"); 00169 if (!dot->saveConfig()) { 00170 logError("failed to save configuration"); 00171 } 00172 00173 // display configuration 00174 display_config(); 00175 00176 while (true) { 00177 uint16_t light; 00178 std::vector<uint8_t> tx_data; 00179 00180 // join network if not joined 00181 if (!dot->getNetworkJoinStatus()) { 00182 join_network(); 00183 } 00184 00185 #if defined(TARGET_XDOT_L151CC) 00186 // configure the ISL29011 sensor on the xDot-DK for continuous ambient light sampling, 16 bit conversion, and maximum range 00187 lux.setMode(ISL29011::ALS_CONT); 00188 lux.setResolution(ISL29011::ADC_16BIT); 00189 lux.setRange(ISL29011::RNG_64000); 00190 00191 // get the latest light sample and send it to the gateway 00192 light = lux.getData(); 00193 tx_data.push_back((light >> 8) & 0xFF); 00194 tx_data.push_back(light & 0xFF); 00195 logInfo("light: %lu [0x%04X]", light, light); 00196 send_data(tx_data); 00197 00198 // put the LSL29011 ambient light sensor into a low power state 00199 lux.setMode(ISL29011::PWR_DOWN); 00200 #else 00201 // get some dummy data and send it to the gateway 00202 light = lux.read_u16(); 00203 tx_data.push_back((light >> 8) & 0xFF); 00204 tx_data.push_back(light & 0xFF); 00205 logInfo("light: %lu [0x%04X]", light, light); 00206 send_data(tx_data); 00207 #endif 00208 00209 // the Dot can't sleep in class C mode 00210 // it must be waiting for data from the gateway 00211 // send data every 30s 00212 if (Fota::getInstance()->timeToStart() != 0) { 00213 logInfo("waiting for 30s"); 00214 ThisThread::sleep_for(30s); 00215 } else { 00216 // Reduce uplinks during FOTA, dot cannot receive while transmitting 00217 // Too many lost packets will cause FOTA to fail 00218 logInfo("FOTA starting in %d seconds", Fota::getInstance()->timeToStart()); 00219 logInfo("waiting for 300s"); 00220 ThisThread::sleep_for(300s); 00221 } 00222 00223 } 00224 00225 return 0; 00226 } 00227 00228 #endif 00229
Generated on Tue Jul 12 2022 13:03:22 by
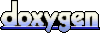