A library for setting up Secure Socket Layer (SSL) connections and verifying remote hosts using certificates. Contains only the source files for mbed platform implementation of the library.
Dependents: HTTPClient-SSL HTTPClient-SSL HTTPClient-SSL HTTPClient-SSL
blake2-int.h
00001 /* 00002 BLAKE2 reference source code package - reference C implementations 00003 00004 Written in 2012 by Samuel Neves <sneves@dei.uc.pt> 00005 00006 To the extent possible under law, the author(s) have dedicated all copyright 00007 and related and neighboring rights to this software to the public domain 00008 worldwide. This software is distributed without any warranty. 00009 00010 You should have received a copy of the CC0 Public Domain Dedication along with 00011 this software. If not, see <http://creativecommons.org/publicdomain/zero/1.0/>. 00012 */ 00013 /* blake2-int.h 00014 * 00015 * Copyright (C) 2006-2014 wolfSSL Inc. 00016 * 00017 * This file is part of CyaSSL. 00018 * 00019 * CyaSSL is free software; you can redistribute it and/or modify 00020 * it under the terms of the GNU General Public License as published by 00021 * the Free Software Foundation; either version 2 of the License, or 00022 * (at your option) any later version. 00023 * 00024 * CyaSSL is distributed in the hope that it will be useful, 00025 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00026 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00027 * GNU General Public License for more details. 00028 * 00029 * You should have received a copy of the GNU General Public License 00030 * along with this program; if not, write to the Free Software 00031 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA 00032 */ 00033 00034 00035 00036 #ifndef CTAOCRYPT_BLAKE2_INT_H 00037 #define CTAOCRYPT_BLAKE2_INT_H 00038 00039 #include <cyassl/ctaocrypt/types.h> 00040 00041 00042 #if defined(_MSC_VER) 00043 #define ALIGN(x) __declspec(align(x)) 00044 #elif defined(__GNUC__) 00045 #define ALIGN(x) __attribute__((aligned(x))) 00046 #else 00047 #define ALIGN(x) 00048 #endif 00049 00050 00051 #if defined(__cplusplus) 00052 extern "C" { 00053 #endif 00054 00055 enum blake2s_constant 00056 { 00057 BLAKE2S_BLOCKBYTES = 64, 00058 BLAKE2S_OUTBYTES = 32, 00059 BLAKE2S_KEYBYTES = 32, 00060 BLAKE2S_SALTBYTES = 8, 00061 BLAKE2S_PERSONALBYTES = 8 00062 }; 00063 00064 enum blake2b_constant 00065 { 00066 BLAKE2B_BLOCKBYTES = 128, 00067 BLAKE2B_OUTBYTES = 64, 00068 BLAKE2B_KEYBYTES = 64, 00069 BLAKE2B_SALTBYTES = 16, 00070 BLAKE2B_PERSONALBYTES = 16 00071 }; 00072 00073 #pragma pack(push, 1) 00074 typedef struct __blake2s_param 00075 { 00076 byte digest_length; /* 1 */ 00077 byte key_length; /* 2 */ 00078 byte fanout; /* 3 */ 00079 byte depth; /* 4 */ 00080 word32 leaf_length; /* 8 */ 00081 byte node_offset[6];/* 14 */ 00082 byte node_depth; /* 15 */ 00083 byte inner_length; /* 16 */ 00084 /* byte reserved[0]; */ 00085 byte salt[BLAKE2B_SALTBYTES]; /* 24 */ 00086 byte personal[BLAKE2S_PERSONALBYTES]; /* 32 */ 00087 } blake2s_param; 00088 00089 ALIGN( 64 ) typedef struct __blake2s_state 00090 { 00091 word32 h[8]; 00092 word32 t[2]; 00093 word32 f[2]; 00094 byte buf[2 * BLAKE2S_BLOCKBYTES]; 00095 word64 buflen; 00096 byte last_node; 00097 } blake2s_state ; 00098 00099 typedef struct __blake2b_param 00100 { 00101 byte digest_length; /* 1 */ 00102 byte key_length; /* 2 */ 00103 byte fanout; /* 3 */ 00104 byte depth; /* 4 */ 00105 word32 leaf_length; /* 8 */ 00106 word64 node_offset; /* 16 */ 00107 byte node_depth; /* 17 */ 00108 byte inner_length; /* 18 */ 00109 byte reserved[14]; /* 32 */ 00110 byte salt[BLAKE2B_SALTBYTES]; /* 48 */ 00111 byte personal[BLAKE2B_PERSONALBYTES]; /* 64 */ 00112 } blake2b_param; 00113 00114 ALIGN( 64 ) typedef struct __blake2b_state 00115 { 00116 word64 h[8]; 00117 word64 t[2]; 00118 word64 f[2]; 00119 byte buf[2 * BLAKE2B_BLOCKBYTES]; 00120 word64 buflen; 00121 byte last_node; 00122 } blake2b_state; 00123 00124 typedef struct __blake2sp_state 00125 { 00126 blake2s_state S[8][1]; 00127 blake2s_state R[1]; 00128 byte buf[8 * BLAKE2S_BLOCKBYTES]; 00129 word64 buflen; 00130 } blake2sp_state; 00131 00132 typedef struct __blake2bp_state 00133 { 00134 blake2b_state S[4][1]; 00135 blake2b_state R[1]; 00136 byte buf[4 * BLAKE2B_BLOCKBYTES]; 00137 word64 buflen; 00138 } blake2bp_state; 00139 #pragma pack(pop) 00140 00141 /* Streaming API */ 00142 int blake2s_init( blake2s_state *S, const byte outlen ); 00143 int blake2s_init_key( blake2s_state *S, const byte outlen, const void *key, const byte keylen ); 00144 int blake2s_init_param( blake2s_state *S, const blake2s_param *P ); 00145 int blake2s_update( blake2s_state *S, const byte *in, word64 inlen ); 00146 int blake2s_final( blake2s_state *S, byte *out, byte outlen ); 00147 00148 int blake2b_init( blake2b_state *S, const byte outlen ); 00149 int blake2b_init_key( blake2b_state *S, const byte outlen, const void *key, const byte keylen ); 00150 int blake2b_init_param( blake2b_state *S, const blake2b_param *P ); 00151 int blake2b_update( blake2b_state *S, const byte *in, word64 inlen ); 00152 int blake2b_final( blake2b_state *S, byte *out, byte outlen ); 00153 00154 int blake2sp_init( blake2sp_state *S, const byte outlen ); 00155 int blake2sp_init_key( blake2sp_state *S, const byte outlen, const void *key, const byte keylen ); 00156 int blake2sp_update( blake2sp_state *S, const byte *in, word64 inlen ); 00157 int blake2sp_final( blake2sp_state *S, byte *out, byte outlen ); 00158 00159 int blake2bp_init( blake2bp_state *S, const byte outlen ); 00160 int blake2bp_init_key( blake2bp_state *S, const byte outlen, const void *key, const byte keylen ); 00161 int blake2bp_update( blake2bp_state *S, const byte *in, word64 inlen ); 00162 int blake2bp_final( blake2bp_state *S, byte *out, byte outlen ); 00163 00164 /* Simple API */ 00165 int blake2s( byte *out, const void *in, const void *key, const byte outlen, const word64 inlen, byte keylen ); 00166 int blake2b( byte *out, const void *in, const void *key, const byte outlen, const word64 inlen, byte keylen ); 00167 00168 int blake2sp( byte *out, const void *in, const void *key, const byte outlen, const word64 inlen, byte keylen ); 00169 int blake2bp( byte *out, const void *in, const void *key, const byte outlen, const word64 inlen, byte keylen ); 00170 00171 static inline int blake2( byte *out, const void *in, const void *key, const byte outlen, const word64 inlen, byte keylen ) 00172 { 00173 return blake2b( out, in, key, outlen, inlen, keylen ); 00174 } 00175 00176 00177 00178 #if defined(__cplusplus) 00179 } 00180 #endif 00181 00182 #endif /* CTAOCRYPT_BLAKE2_INT_H */ 00183
Generated on Wed Jul 13 2022 02:33:55 by
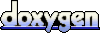