
Example program that reads in sensor data and sends it to the 2lemetry cloud.
Dependencies: FXLS8471Q MPL3115A2 MQTT mbed
Fork of 2lemetry_Sensor_Example by
main.cpp
00001 /* This is an example program which reads in the 3-axis acceleration, pressure, and temperature data from 00002 * a FRDM-FXS-MULTI sensor board. It then uses an MTSAS SocketModem Shield board to send the read data over 00003 * a cellular connection to the 2lemetry cloud using an MQTT client protocol. 00004 */ 00005 00006 #include "mbed.h" 00007 #include "mtsas.h" 00008 #include "PubSubClient.h" 00009 #include "FXLS8471Q.h" 00010 #include "MPL3115A2.h" 00011 00012 /* PLEASE READ THIS! 00013 * The following fields must be populated in order to properly send data to the "Default ThingFabric Project" in your 2lemetry account using the MQTT client 00014 * You must have a hacker (or higher) account at http://app.thingfabric.com 00015 * After you register and login, follow the steps below to set up your client 00016 * Click on "Default ThingFabric Project" 00017 * Set _2LEMETRY_DOMAIN to the string after "Project" at the top of the page 00018 * Click on "Credentials" 00019 * Click on "Default ThingFabric Credential" 00020 * Set _2LEMETRY_USER_ID to the value in the "Key (Username)" field 00021 * Set _2LEMETRY_TOKEN to the value in the "MD5 Secret" field 00022 * If you are running this code on multiple devices, you will want to make the _2LEMETRY_DEVICE_ID field unique for each device 00023 * Set the _APN field to the APN provided with your sim card 00024 * Build, flash, and run 00025 * This code sends a random integer value approximately every 30 seconds 00026 * Click on "Default ThingFabric Project" 00027 * Click on "Analytics" 00028 * You should be able to see your test data (page needs to be refreshed periodically, it doesn't automatically refresh) 00029 */ 00030 00031 char _2LEMETRY_USERID[] = ""; 00032 char _2LEMETRY_TOKEN[] = ""; 00033 char _2LEMETRY_DOMAIN[] = ""; 00034 char _2LEMETRY_STUFF[] = "mbed"; 00035 char _2LEMETRY_DEVICE_ID[] = "nucleo-0001"; 00036 00037 char _APN[] = ""; 00038 00039 char _host[] = "q.mq.tt"; 00040 int _port = 1883; 00041 00042 #define MPL3115A2_I2C_ADDRESS (0x60<<1) 00043 00044 #define DATA_INTERVAL 30 00045 00046 void callback(char* topic, char* payload, unsigned int len) { 00047 logInfo("topic: [%s]\r\npayload: [%s]", topic, payload); 00048 } 00049 00050 int main() { 00051 MTSLog::setLogLevel(MTSLog::TRACE_LEVEL); 00052 00053 /** STMicro Nucelo F401RE 00054 * The supported jumper configurations of the MTSAS do not line up with 00055 * the pin mapping of the Nucleo F401RE. Therefore, the MTSAS serial TX 00056 * pin (JP8 Pin 2) must be manually jumped to Serial1 RX (Shield pin D2) 00057 * and the MTSAS serial RX pin (JP9 Pin 2) pin must be manually jumped to 00058 * Serial1 TX (Shield pin D8). 00059 * Uncomment the following line to use the STMicro Nuceleo F401RE 00060 */ 00061 MTSSerialFlowControl io(D8, D2, D3, D6); 00062 00063 /** Freescale KL46Z 00064 * To configure the serial pins for the Freescale KL46Z board, use MTSAS jumper 00065 * configuration B. Uncomment the following line to use the Freescale KL46Z board 00066 */ 00067 //MTSSerialFlowControl io(D2, D9, D3, D6); 00068 00069 /** Freescale K64F 00070 * To configure the serial pins for the Freescale K64F board, use MTSAS jumper 00071 * configuration A. Uncomment the following line to use the Freescale K64F board 00072 */ 00073 //MTSSerialFlowControl io(D1, D0, D3, D6); 00074 00075 //Set baudrate between the board and the radio to 115200 00076 io.baud(115200); 00077 00078 Cellular* radio = CellularFactory::create(&io); 00079 if (! radio) { 00080 logFatal("failed to create Cellular object - exiting"); 00081 return 1; 00082 } 00083 00084 Transport::setTransport(radio); 00085 00086 while (radio->setApn(_APN) != MTS_SUCCESS) { 00087 logError("failed to set APN [%s]", _APN); 00088 wait(2); 00089 } 00090 00091 while (! radio->connect()) { 00092 logError("failed to bring up PPP link"); 00093 wait(2); 00094 } 00095 00096 PubSubClient mqtt(_host, _port, callback); 00097 00098 char topicStr[128]; 00099 char buf[128]; 00100 snprintf(topicStr, sizeof(topicStr), "%s/%s/%s", _2LEMETRY_DOMAIN, _2LEMETRY_STUFF, _2LEMETRY_DEVICE_ID); 00101 00102 FXLS8471Q acc(D11, D12, D13, D10); 00103 MPL3115A2 alt(D14, D15, MPL3115A2_I2C_ADDRESS, D4, D3); 00104 alt.Barometric_Mode(); 00105 00106 float acc_data[3]; 00107 00108 while (true) { 00109 if (! mqtt.connect(_2LEMETRY_DEVICE_ID, _2LEMETRY_USERID, _2LEMETRY_TOKEN)) { 00110 logError("failed to connect to 2lemetry server"); 00111 continue; 00112 } 00113 00114 acc.ReadXYZ(acc_data); 00115 00116 snprintf(buf, sizeof(buf), "{\"x\":%f,\"y\":%f,\"z\":%f,\"pressure\":%f,\"temperature\":%f}", acc_data[0],acc_data[1],acc_data[2], alt.getPressure(), alt.getTemperature()); 00117 logInfo("publishing: [%s]", buf); 00118 if (! mqtt.publish(topicStr, buf)) { 00119 logError("failed to publish: [%s]", buf); 00120 } 00121 wait(1); 00122 mqtt.loop(); 00123 mqtt.disconnect(); 00124 wait(DATA_INTERVAL); 00125 } 00126 00127 }
Generated on Mon Jul 25 2022 01:16:17 by
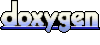