
mDot source code for the "sensor" mDot in this demo: https://twitter.com/MultiTechSys/status/621708791009124352
Dependencies: PinDetect libmDot mbed-rtos mbed
main.cpp
00001 // --------------------------------------------- 00002 // MultiTech Thermostat & Fan Demo (Sensor unit) 00003 // 00004 // This program runs on a MultiTech mDot with: 00005 // - 1 MultiTech UDK 00006 // - 1 Arduino base shield 00007 // - 2 Grove COM08211P push buttons 00008 // - 1 Grove SEN23292P v1.2 temperature sensor 00009 // --------------------------------------------- 00010 00011 #include "mbed.h" 00012 #include "mDot.h" 00013 #include "PinDetect.h" 00014 00015 mDot* dot; 00016 Ticker ledTick; 00017 Ticker temperatureMonitorTick; 00018 Ticker periodicSendTick; 00019 00020 PinDetect up_button(PB_1); //A0 on Arduino base shield 00021 PinDetect down_button(PB_0); //A1 on Arduino base shield 00022 AnalogIn temperatureSensor(PC_1); //A2 on Arduino base shield 00023 DigitalOut transmitLED(LED1); 00024 DigitalOut buttonPressLED(PA_1); //D6 on Arduino base shield 00025 00026 // Configuration variables 00027 static std::string config_network_name = "your_name_here"; 00028 static std::string config_network_pass = "your_key_here"; 00029 static uint8_t config_frequency_sub_band = 1; 00030 00031 //Global Variables 00032 static uint16_t setPoint = 21; //21 C is standard room temp 00033 static volatile bool timeToReadTemperature = true; 00034 static volatile bool dataChanged = true; 00035 static volatile bool thermostatActivated = false; 00036 static uint16_t sentSetPoint; 00037 00038 //Function prototypes 00039 void ledTock(); 00040 void periodicSendTock(); 00041 void temperatureMonitorTock(); 00042 void up_button_callback(); 00043 void down_button_callback(); 00044 void printError(mDot* dot, int32_t returnCode); 00045 void printVersion(); 00046 bool setFrequencySubBand(uint8_t subBand); 00047 bool setNetworkName(const std::string name); 00048 bool setNetworkPassphrase(const std::string passphrase); 00049 bool setPower(uint8_t power); 00050 bool setAck(uint8_t retries); 00051 bool joinNetwork(); 00052 bool send(const std::string text); 00053 00054 00055 int main() 00056 { 00057 //Start LED startup sequence 00058 ledTick.attach(&ledTock, 0.1); 00059 00060 printf("\r\n\r\n"); 00061 printf("=====================================\r\n"); 00062 printf("MTS Thermostat/Fan Demo (Sensor unit)\r\n"); 00063 printf("=====================================\r\n"); 00064 printVersion(); 00065 00066 // get the mDot handle 00067 dot = mDot::getInstance(); 00068 00069 // reset to default config so we know what state we're in 00070 dot->resetNetworkSession(); 00071 dot->resetConfig(); 00072 00073 // set up the mDot with our network information 00074 setNetworkName(config_network_name); 00075 setNetworkPassphrase(config_network_pass); 00076 setFrequencySubBand(config_frequency_sub_band); 00077 setPower(7); // Reduce latency for 868 units 00078 setAck(0); // Disable ack for less latency 00079 00080 while (!joinNetwork()) { wait(2); dot->resetNetworkSession(); } 00081 00082 // Stop LED startup sequence & configure them for operation 00083 ledTick.detach(); 00084 transmitLED = 1; 00085 buttonPressLED = 1; 00086 00087 // Configure timers 00088 periodicSendTick.attach(&periodicSendTock, 5*60); 00089 temperatureMonitorTick.attach(&temperatureMonitorTock, 0.15); 00090 00091 // Setup Interrupt callback function 00092 up_button.attach_deasserted(&up_button_callback); 00093 down_button.attach_deasserted(&down_button_callback); 00094 00095 // Start sampling buttons using interrupts 00096 up_button.setSampleFrequency(); 00097 down_button.setSampleFrequency(); 00098 00099 uint16_t temperature = 0; 00100 while (1) { 00101 if (timeToReadTemperature) { 00102 uint16_t B = 4250; //B value of the thermistor 00103 uint16_t analogReading; 00104 float resistance; 00105 00106 analogReading = temperatureSensor.read_u16(); 00107 00108 // get the resistance of the sensor 00109 resistance=(65534-analogReading)*10000/analogReading; 00110 // convert to temperature via datasheet 00111 temperature=1/(log(resistance/10000)/B+1/298.15)-273.15; 00112 //printf("read: %d, res: %d, temp: %d,set: %d\r\n", analogReading, (uint16_t)resistance, temperature, setPoint); 00113 00114 if (!thermostatActivated && temperature > setPoint) { 00115 thermostatActivated = true; 00116 dataChanged = true; 00117 } 00118 else if (thermostatActivated && temperature < setPoint) { 00119 thermostatActivated = false; 00120 dataChanged = true; 00121 } 00122 00123 timeToReadTemperature = false; 00124 } 00125 00126 if (dataChanged) { 00127 char latestData[100]; 00128 transmitLED = 1; 00129 sentSetPoint = setPoint; 00130 sprintf(latestData, "temp: %d,set: %d", temperature, sentSetPoint); 00131 printf("%s\r\n", latestData); 00132 00133 if (send(latestData)) { 00134 dataChanged = false; 00135 } 00136 transmitLED = 0; 00137 } 00138 } 00139 } 00140 00141 00142 void ledTock() { 00143 transmitLED = !transmitLED; 00144 buttonPressLED = !buttonPressLED; 00145 } 00146 00147 void periodicSendTock() { 00148 dataChanged = true; 00149 } 00150 00151 void temperatureMonitorTock() { 00152 timeToReadTemperature = true; 00153 00154 if (sentSetPoint != setPoint) { 00155 dataChanged = true; 00156 } 00157 } 00158 00159 void up_button_callback() { 00160 if (setPoint < 51) { 00161 setPoint += 1; 00162 buttonPressLED = !buttonPressLED; 00163 dataChanged = true; 00164 } 00165 } 00166 00167 void down_button_callback() { 00168 if (setPoint > 0) { 00169 setPoint -= 1; 00170 buttonPressLED = !buttonPressLED; 00171 dataChanged = true; 00172 } 00173 } 00174 00175 00176 void printVersion() 00177 { 00178 printf("%s\r\n\r\n", dot->getId().c_str()); 00179 } 00180 00181 bool setFrequencySubBand(uint8_t subBand) 00182 { 00183 int32_t returnCode; 00184 printf("Setting frequency sub band to '%d'...\r\n", subBand); 00185 if ((returnCode = dot->setFrequencySubBand(subBand)) != mDot::MDOT_OK) { 00186 printError(dot, returnCode); 00187 return false; 00188 } 00189 return true; 00190 } 00191 00192 bool setNetworkName(const std::string name) 00193 { 00194 int32_t returnCode; 00195 printf("Setting network name to '%s'...\r\n", name.c_str()); 00196 if ((returnCode = dot->setNetworkName(name)) != mDot::MDOT_OK) 00197 { 00198 printError(dot, returnCode); 00199 return false; 00200 } 00201 return true; 00202 } 00203 00204 bool setNetworkPassphrase(const std::string passphrase) 00205 { 00206 int32_t returnCode; 00207 printf("Setting passphrase to '%s'...\r\n", passphrase.c_str()); 00208 if ((returnCode = dot->setNetworkPassphrase(passphrase)) != mDot::MDOT_OK) 00209 { 00210 printError(dot, returnCode); 00211 return false; 00212 } 00213 return true; 00214 } 00215 00216 bool setPower(uint8_t power) 00217 { 00218 int32_t returnCode; 00219 printf("Setting tx power to '%d'...\r\n", power); 00220 if ((returnCode = dot->setTxPower(power)) != mDot::MDOT_OK) { 00221 printError(dot, returnCode); 00222 return false; 00223 } 00224 return true; 00225 } 00226 00227 00228 bool joinNetwork() 00229 { 00230 int32_t returnCode; 00231 printf("\r\nJoining network...\r\n"); 00232 if ((returnCode = dot->joinNetworkOnce()) != mDot::MDOT_OK) { 00233 printError(dot, returnCode); 00234 return false; 00235 } 00236 printf("Network Joined!\r\n"); 00237 return true; 00238 } 00239 00240 bool setAck(uint8_t retries) 00241 { 00242 int32_t returnCode; 00243 printf("Setting ack to '%d'...\r\n", retries); 00244 if ((returnCode = dot->setAck(retries)) != mDot::MDOT_OK) 00245 { 00246 printError(dot, returnCode); 00247 return false; 00248 } 00249 return true; 00250 } 00251 00252 bool send(const std::string text) 00253 { 00254 int32_t returnCode; 00255 uint32_t timeTillSend = dot->getNextTxMs(); 00256 if (timeTillSend != 0) { 00257 printf("waiting %lu ms to send\r\n", timeTillSend); 00258 return false; 00259 } 00260 00261 printf("Sending data... "); 00262 std::vector<uint8_t> data(text.begin(), text.end()); 00263 if ((returnCode = dot->send(data, 1)) != mDot::MDOT_OK) 00264 { 00265 printError(dot, returnCode); 00266 return false; 00267 } 00268 printf("Data sent!\r\n"); 00269 return true; 00270 } 00271 00272 void printError(mDot* dot, int32_t returnCode) 00273 { 00274 std::string error = mDot::getReturnCodeString(returnCode) + " - " + dot->getLastError(); 00275 printf("%s\r\n", error.c_str()); 00276 } 00277
Generated on Thu Jul 14 2022 05:20:13 by
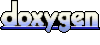