GPS NEMA String parser library. Only supports SkyTraq Venus chip at this time.
Dependents: MTDOT-EVB-LinkCheck-AL MTDOT-BOX-EVB-Factory-Firmware-LIB-108 TelitSensorToCloud mDot_sensor_to_cloud ... more
GPSPARSER.h
00001 /** 00002 * @file GpsParser.h 00003 * @brief NEMA String to Packet Parser - NEMA strings to compact packet data 00004 * @author Tim Barr 00005 * @version 1.0 00006 * @see http://www.kh-gps.de/nmea.faq 00007 * @see http://www.catb.org/gpsd/NMEA.html 00008 * @see http://www.skytraq.com.tw/products/Venus638LPx_PB_v3.pdf 00009 * 00010 * Copyright (c) 2015 00011 * 00012 * Licensed under the Apache License, Version 2.0 (the "License"); 00013 * you may not use this file except in compliance with the License. 00014 * You may obtain a copy of the License at 00015 * 00016 * http://www.apache.org/licenses/LICENSE-2.0 00017 * 00018 * Unless required by applicable law or agreed to in writing, software 00019 * distributed under the License is distributed on an "AS IS" BASIS, 00020 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00021 * See the License for the specific language governing permissions and 00022 * limitations under the License. 00023 * 00024 */ 00025 00026 #ifndef GPSPARSER_H 00027 #define GPSPARSER_H 00028 00029 #include "mbed.h" 00030 #include "rtos.h" 00031 #include "MTSSerial.h" 00032 #include "NCP5623B.h" 00033 #include <string> 00034 #include <vector> 00035 #include <ctime> 00036 #define START_THREAD 1 00037 00038 using namespace mts; 00039 00040 class GPSPARSER 00041 { 00042 public: 00043 00044 /** 00045 * @typedef struct latitude 00046 * @brief latitude degrees, minutes, seconds structure 00047 * range of degrees : 0 to 90 signed (negative degrees is South) 00048 * range of minutes : 0 to 60 unsigned 00049 * Range of seconds : 0-9999 unsigned or 1/10000 of a minute. 00050 * Multiply by 6 and divide or modulus by 1000 to get seconds and fractional seconds 00051 */ 00052 typedef struct{int8_t degrees; uint8_t minutes; uint16_t seconds;} latitude; 00053 00054 /** 00055 * @typedef struct longitude 00056 * @brief longitude degrees, minutes, seconds structure negative degrees is West 00057 * range of degrees : 0 to 180 signed (negative degrees is West) 00058 * range of minutes : 0 to 60 unsigned 00059 * Range of seconds : 0-9999 unsigned or 1/10000 of a minute. 00060 * Multiply by 6 and divide or modulus by 1000 to get seconds and fractional seconds 00061 */ 00062 typedef struct{int16_t degrees; uint8_t minutes; uint16_t seconds;} longitude; 00063 00064 /** 00065 * @typdef satellite_prn 00066 * @brief satellite prn list, up to 12 valid 00067 */ 00068 typedef uint8_t satellite_prn[12]; 00069 00070 /** Create the GPSPARSER object 00071 * @param uart - an MTSSerial object 00072 * @param led - an NCP5623B led controller object 00073 */ 00074 GPSPARSER(MTSSerial *uart, NCP5623B* led = NULL); 00075 00076 /** destroy the GPSPARSER object 00077 */ 00078 ~GPSPARSER(void); 00079 00080 /** GPS device detected 00081 * @return boolean whether GPS device detected 00082 */ 00083 bool gpsDetected(void); 00084 00085 /** get longitude structure 00086 * @return last valid NEMA string longitude data 00087 */ 00088 longitude getLongitude(void); 00089 00090 /** get latitude structure 00091 * @return last valid NEMA string latitude data 00092 */ 00093 latitude getLatitude(void); 00094 00095 /** get timestamp structure 00096 * @return last valid NEMA string time and date data 00097 * uses tm struc from ctime standard library 00098 */ 00099 struct tm getTimestamp(void); 00100 00101 /** get GPS Lock status 00102 * @return boolean of last valid NEMA string lock status data 00103 */ 00104 bool getLockStatus(void); 00105 00106 /** get GPS Fix status 00107 * @return last valid NEMA Fix status data 00108 * 1 = no fix 00109 * 2 = 2D fix - latitude, longitude, time only valid 00110 * 3 = 3D fix - all data valid 00111 */ 00112 uint8_t getFixStatus(void); 00113 00114 /** get GPS Fix qualty 00115 * @return last valid NEMA string Fix Quality data 00116 * 0 = invalid 00117 * 1 = GPS fix (SPS) 00118 * 2 = DGPS fix 00119 * 3 = PPS fix 00120 * 4 = Real Time Kinematic 00121 * 5 = Float RTK 00122 * 6 = estimated (dead reckoning) (2.3 feature) 00123 * 7 = Manual input mode 00124 * 8 = Simulation mode 00125 */ 00126 uint8_t getFixQuality(void); 00127 00128 /** get number of visible satellites 00129 * @return last valid NEMA string number of satellites visible 00130 */ 00131 uint8_t getNumSatellites(void); 00132 00133 /** get calculated altitude 00134 * @return last valid NEMA string altitude data in meters 00135 */ 00136 int16_t getAltitude(void); 00137 00138 private: 00139 00140 std::string _nema_buff; 00141 bool _gps_detected; 00142 latitude _gps_latitude; 00143 longitude _gps_longitude; 00144 struct tm _timestamp; 00145 bool _gps_status; 00146 uint8_t _fix_status; 00147 uint8_t _fix_quality; 00148 uint8_t _num_satellites; 00149 satellite_prn _satellite_prn; 00150 int16_t _msl_altitude; 00151 00152 MTSSerial *_gps_uart; 00153 Thread _getSentenceThread; 00154 NCP5623B* _led; 00155 int8_t _led_state; 00156 int8_t _led_state_out; 00157 Ticker _tick; 00158 bool _tick_running; 00159 Mutex _mutex; 00160 00161 /** Start sentence parser thread 00162 * 00163 */ 00164 static void startSentenceThread (void const *p); 00165 00166 /** Read NEMA sentences from specified serial port and call specific sentence parser 00167 */ 00168 void readNemaSentence (void); 00169 00170 /** Parse GGA NEMA sentence 00171 * @return status of parser attempt 00172 */ 00173 uint8_t parseGGA(char *nema_buf); 00174 00175 /** Parse GSA NEMA sentence 00176 * @return status of parser attempt 00177 */ 00178 uint8_t parseGSA(char *nema_buf); 00179 00180 /** Parse GSV NEMA sentence 00181 * @return status of parser attempt 00182 */ 00183 uint8_t parseGSV(char *nema_buf); 00184 00185 /** Parse RMC NEMA sentence 00186 * @return status of parser attempt 00187 */ 00188 uint8_t parseRMC(char *nema_buf); 00189 00190 /** Parse VTG NEMA sentence 00191 * @return status of parser attempt 00192 */ 00193 uint8_t parseVTG(char *nema_buf); 00194 00195 /** Parse GLL NEMA sentence 00196 * @return status of parser attempt 00197 */ 00198 uint8_t parseGLL(char *nema_buff); 00199 00200 /** Parse ZDA NEMA sentence 00201 * @return status of parser attempt 00202 */ 00203 uint8_t parseZDA(char *nema_buff); 00204 00205 void blinker(); 00206 00207 }; 00208 #endif
Generated on Thu Jul 14 2022 20:56:11 by
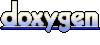