
52 51 with same code
Dependencies: BMP280 MtSense01 SHT2X
Fork of MtConnect04S_MtSense01 by
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2014 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include <events/mbed_events.h> 00018 #include <mbed.h> 00019 #include "ble/BLE.h" 00020 #include "ble/Gap.h" 00021 #include "ble/services/EnvironmentalService.h" 00022 #include "MtSense01.h" 00023 00024 /* UART printf */ 00025 #ifdef NRF52 00026 Serial pc(p20, p24); 00027 #else 00028 Serial pc(p5, p4); 00029 #endif 00030 00031 I2C i2c(p3, p2); 00032 DigitalOut led1(p16, 1); 00033 MtSense01 mtsense1(i2c, pc); 00034 00035 const static char DEVICE_NAME[] = "Mt5MtSense01"; 00036 static const uint16_t uuid16_list[] = {0x1822, 0x181A}; 00037 00038 static EnvironmentalService *environmentalServicePtr; 00039 00040 static EventQueue eventQueue(/* event count */ 16 * EVENTS_EVENT_SIZE); 00041 00042 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params) 00043 { 00044 BLE::Instance().gap().startAdvertising(); 00045 } 00046 00047 void initMtSense01() { 00048 mtsense1.Initial(); 00049 } 00050 00051 void readMtSense01() { 00052 int pressPa; 00053 float tempC, humidity; 00054 00055 mtsense1.readData(&tempC, &pressPa, &humidity); 00056 BLE &ble = BLE::Instance(); 00057 if (ble.gap().getState().connected) { 00058 environmentalServicePtr->updateHumidity(humidity); 00059 environmentalServicePtr->updatePressure(pressPa); 00060 environmentalServicePtr->updateTemperature(tempC); 00061 } 00062 00063 // pc.printf("tempC: %8f, pressPa: %8d, humidity: %8f \r\n", tempC, pressPa, humidity); 00064 00065 } 00066 00067 void blinkCallback(void) 00068 { 00069 led1 = !led1; /* Do blinky on LED1 while we're waiting for BLE events */ 00070 00071 BLE &ble = BLE::Instance(); 00072 } 00073 00074 /** 00075 * This function is called when the ble initialization process has failled 00076 */ 00077 void onBleInitError(BLE &ble, ble_error_t error) 00078 { 00079 /* Initialization error handling should go here */ 00080 } 00081 00082 /** 00083 * Callback triggered when the ble initialization process has finished 00084 */ 00085 void bleInitComplete(BLE::InitializationCompleteCallbackContext *params) 00086 { 00087 BLE& ble = params->ble; 00088 ble_error_t error = params->error; 00089 00090 if (error != BLE_ERROR_NONE) { 00091 /* In case of error, forward the error handling to onBleInitError */ 00092 onBleInitError(ble, error); 00093 return; 00094 } 00095 00096 /* Ensure that it is the default instance of BLE */ 00097 if(ble.getInstanceID() != BLE::DEFAULT_INSTANCE) { 00098 return; 00099 } 00100 00101 ble.gap().onDisconnection(disconnectionCallback); 00102 00103 /* Setup primary service */ 00104 environmentalServicePtr = new EnvironmentalService(ble); 00105 00106 /* Setup advertising */ 00107 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); 00108 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, (uint8_t *) uuid16_list, sizeof(uuid16_list)); 00109 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *) DEVICE_NAME, sizeof(DEVICE_NAME)); 00110 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00111 ble.gap().setAdvertisingInterval(100); /* 100ms */ 00112 ble.gap().startAdvertising(); 00113 } 00114 00115 void scheduleBleEventsProcessing(BLE::OnEventsToProcessCallbackContext* context) { 00116 BLE &ble = BLE::Instance(); 00117 eventQueue.call(Callback<void()>(&ble, &BLE::processEvents)); 00118 } 00119 00120 int main() 00121 { 00122 pc.set_flow_control(SerialBase::Disabled); 00123 pc.baud(115200); 00124 pc.printf("\r\n"); 00125 pc.printf("Welcome MTM Node !\r\n"); 00126 00127 00128 eventQueue.call_every(500, blinkCallback); 00129 00130 initMtSense01(); 00131 eventQueue.call_every(1000, readMtSense01); // 1000 ms repeat 00132 00133 BLE &ble = BLE::Instance(); 00134 ble.onEventsToProcess(scheduleBleEventsProcessing); 00135 ble.init(bleInitComplete); 00136 00137 eventQueue.dispatch_forever(); 00138 00139 return 0; 00140 }
Generated on Fri Jul 22 2022 05:30:37 by
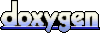