initial
Embed:
(wiki syntax)
Show/hide line numbers
BMG160.cpp
00001 /* Copyright (c) 2016 MtM Technology Corporation, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 #include "BMG160.h" 00019 00020 /******************************* 00021 * Public methods 00022 *******************************/ 00023 BMG160::BMG160(PinName i2c_sda, PinName i2c_scl, PinName interrupt1_pinname, PinName interrupt2_pinname,uint8_t range, uint8_t bandwith) 00024 : i2c_(i2c_sda, i2c_scl), 00025 interrupt1_pinname_(interrupt1_pinname), 00026 interrupt1_(interrupt1_pinname), 00027 interrupt2_pinname_(interrupt2_pinname), 00028 interrupt2_(interrupt2_pinname), 00029 range_(range), bandwith_(bandwith) { 00030 00031 /* Basic */ 00032 RegWrite(0x10, bandwith_); // bandwidth 00033 RegWrite(0x0F, range_); // range 00034 RegWrite(0x1A, 0x20); // slow_offset_unfilt = 1 00035 RegWrite(0x31, 0x07); // SOC_REG slow_offset_en_x/y/z = 1 00036 00037 } 00038 00039 void BMG160::ReadXYZ(int16_t *xyz) { 00040 char val[6]; 00041 00042 /* Read raw data */ 00043 RegRead(0x02, val, sizeof(val)); 00044 xyz[0] = ((int16_t)val[1] << 8) | (val[0] & 0xC0); 00045 xyz[1] = ((int16_t)val[3] << 8) | (val[2] & 0xC0); 00046 xyz[2] = ((int16_t)val[5] << 8) | (val[4] & 0xC0); 00047 00048 /* Align right */ 00049 xyz[0] >>= 6; 00050 xyz[1] >>= 6; 00051 xyz[2] >>= 6; 00052 } 00053 /******************************* 00054 * Private methods 00055 *******************************/ 00056 void BMG160::RegWrite(char reg, char val) { 00057 char data[2]; 00058 data[0] = reg; 00059 data[1] = val; 00060 i2c_.write(BMG160_SLAVE_ADDR, data, 2, 0); 00061 } 00062 00063 void BMG160::RegRead(char reg, char *val, int len) { 00064 i2c_.write(BMG160_SLAVE_ADDR, ®, 1, 0); 00065 i2c_.read (BMG160_SLAVE_ADDR, val, len); 00066 }
Generated on Thu Jul 14 2022 10:56:10 by
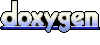