Utilities for BORCH accelerometer BMA250E
Dependents: MtConnect04S_Bike_Proximity Mt05_MtSense03
BMA250E.h
00001 /* Copyright (c) 2016 MtM Technology Corporation, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 #ifndef BMA250E_h 00019 #define BMA250E_h 00020 00021 #include "mbed.h" 00022 00023 #define BMA250E_SLAVE_ADDR 0x30 00024 00025 class BMA250E { 00026 public: 00027 BMA250E(PinName i2c_sda, PinName i2c_scl, PinName int1 = NC, PinName int2 = NC, uint8_t range = 0x03, uint8_t bandwith =0x0C); 00028 00029 void ReadXYZ(int16_t *xyz); 00030 00031 void NewData (void(*fptr)(void)); 00032 void AnyMotion (void(*fptr)(void)); 00033 void TapSening (void(*fptr)(void), bool double_tap); 00034 void OrientationRecognition (void(*fptr)(void)); 00035 void FlatDetection (void(*fptr)(void)); 00036 void LowHighGDetection (void(*fptr)(void), bool high_g); 00037 void ShakeDetection (void(*fptr)(void)); 00038 00039 void EnterStandbyMode(void); 00040 void LeaveStandbyMode(void); 00041 00042 private: 00043 I2C i2c_; 00044 PinName interrupt1_pinname_; 00045 InterruptIn interrupt1_; 00046 PinName interrupt2_pinname_; 00047 InterruptIn interrupt2_; 00048 uint8_t range_; 00049 uint8_t bandwith_; 00050 00051 void RegWrite(char reg, char val); 00052 void RegRead (char reg, char *val, int len); 00053 void RegReadModifyWrite(char reg, char clr_mask, char set_mask); 00054 }; 00055 00056 #endif
Generated on Sun Jul 17 2022 18:16:28 by
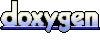