
Sense multiple keypresses from a 4x4 keypad
Dependencies: Hotboards_keypad mbed
Fork of Hotboards_MultiKey by
main.cpp
00001 /* @file MultiKey.cpp 00002 || @vesion 1.2 00003 || @modified by Istvan Cserny 00004 || @contact https://developer.mbed.org/users/icserny/ 00005 || @version 1.1 00006 || @modified by Diego (http://hotboards.org) 00007 || @version 1.0 00008 || @author Mark Stanley 00009 || @contact mstanley@technologist.com 00010 || 00011 || @description 00012 || | The latest version, 3.0, of the keypad library supports up to 10 00013 || | active keys all being pressed at the same time. This sketch is an 00014 || | example of how you can get multiple key presses from a keypad or 00015 || | keyboard. 00016 00017 */ 00018 #include "mbed.h" 00019 #include "Hotboards_keypad.h" 00020 #include <string> 00021 00022 using std::string; 00023 00024 // Defines the keys array with it's respective number of rows & cols, 00025 // and with the value of each key 00026 char keys[ 4 ][ 4 ] = 00027 { 00028 { '1' , '2' , '3' , 'A' }, 00029 { '4' , '5' , '6' , 'B' }, 00030 { '7' , '8' , '9' , 'C' }, 00031 { '*' , '0' , '#' , 'D' } 00032 }; 00033 00034 // Defines the pins connected to the rows 00035 DigitalInOut rowPins[ 4 ] = { PTB8 , PTB9 , PTB10 , PTB11 }; 00036 // Defines the pins connected to the cols 00037 DigitalInOut colPins[ 4 ] = { PTE2 , PTE3 , PTE4 , PTE5 }; 00038 00039 // Creates a new keyboard with the values entered before 00040 Keypad kpd( makeKeymap( keys ) , rowPins , colPins , 4 , 4 ); 00041 00042 // Configures the serial port 00043 Serial pc( USBTX , USBRX ); 00044 00045 int i; 00046 00047 int main() 00048 { 00049 string msg; 00050 while(1) 00051 { 00052 // Fills kpd.key[ ] array with up-to 10 active keys. 00053 // Returns true if there are ANY active keys. 00054 if( kpd.getKeys( ) ) 00055 { 00056 // Scan the whole key list. 00057 for( i = 0 ; i < LIST_MAX ; i++ ) 00058 { 00059 // Only find keys that have changed state. 00060 if( kpd.key[ i ].stateChanged ) 00061 { 00062 // Report active key state : IDLE, PRESSED, HOLD, or RELEASED 00063 switch( kpd.key[ i ].kstate ) 00064 { 00065 case PRESSED: 00066 msg = " PRESSED. "; 00067 break; 00068 case HOLD: 00069 msg = " HOLD. "; 00070 break; 00071 case RELEASED: 00072 msg = " RELEASED. "; 00073 break; 00074 case IDLE: 00075 msg = " IDLE. "; 00076 break; 00077 default: 00078 break; 00079 } 00080 // Print the current state of the key pressed 00081 pc.printf( "Key " ); 00082 pc.printf( "%c" , kpd.key[ i ].kchar ); 00083 pc.printf( "%s" , msg.c_str() ); 00084 pc.printf( "\n\r" ); 00085 } 00086 } 00087 } 00088 } 00089 }
Generated on Fri Jul 22 2022 12:20:45 by
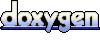