
RTOS timer usage
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /** 12_rtos_timer 00002 * LED blinking by four RTOS timer - a Handbook demo 00003 * Link: https://developer.mbed.org/users/mbed_official/code/rtos_timer/docs/9dd7d49be2c3/main_8cpp_source.html 00004 * 00005 * Hardware requirements: 00006 * - FRDM-KL25Z board 00007 * - 4 LEDs (with current limiting resistors) connected to the Arduino compatible D4,D5,D6,D7 pins 00008 */ 00009 00010 00011 #include "mbed.h" 00012 #include "rtos.h" 00013 00014 DigitalOut LEDs[4] = {DigitalOut(D4), DigitalOut(D5), DigitalOut(D6), DigitalOut(D7)}; 00015 00016 void blink(void const *n) { 00017 LEDs[(int)n] = !LEDs[(int)n]; 00018 } 00019 00020 int main(void) { 00021 RtosTimer led_1_timer(blink, osTimerPeriodic, (void *)0); 00022 RtosTimer led_2_timer(blink, osTimerPeriodic, (void *)1); 00023 RtosTimer led_3_timer(blink, osTimerPeriodic, (void *)2); 00024 RtosTimer led_4_timer(blink, osTimerPeriodic, (void *)3); 00025 00026 led_1_timer.start(2000); 00027 led_2_timer.start(1000); 00028 led_3_timer.start(500); 00029 led_4_timer.start(250); 00030 00031 Thread::wait(osWaitForever); 00032 }
Generated on Thu Jul 14 2022 19:55:52 by
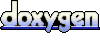