
// Simple button/swipe library for FRDM-KL25Z // AI Williams -- The Touch of a Button // Dr.Dobb's Journal // http://www.drdobbs.com/embedded-systems/the-touch-of-a-button/240169431
Dependencies: mbed tsi_sensor
Fork of 04_tsi_buttons by
main.cpp
00001 #include "mbed.h" 00002 #include "tsi_sensor.h" 00003 00004 // Simple button/swipe library for FRDM-KL25Z 00005 // AI Williams -- The Touch of a Button 00006 // Dr.Dobb's Journal 00007 // http://www.drdobbs.com/embedded-systems/the-touch-of-a-button/240169431 00008 00009 /* This defines will be replaced by PinNames soon */ 00010 #if defined (TARGET_KL25Z) || defined (TARGET_KL46Z) 00011 #define ELEC0 9 00012 #define ELEC1 10 00013 #elif defined (TARGET_KL05Z) 00014 #define ELEC0 9 00015 #define ELEC1 8 00016 #else 00017 #error TARGET NOT DEFINED 00018 #endif 00019 00020 // Define the "zones" considered to be buttons 00021 // 4 is about the limit and 3 might be even better 00022 // You could also equal space them automatically pretty easily 00023 // (e.g., specify 25% and fill in BTNMAX programmatically 00024 float BTNMAX[]= {0.25, 0.50, 0.75, 1.00 }; 00025 #define SWIPETIME .350 // seconds to wait before sensing a button push or swipe 00026 #define SWIPE_R 256 // virtual button code for right swipe 00027 #define SWIPE_L 512 // virtual button code for left swipe 00028 00029 Serial pc(USBTX, USBRX); // tx, rx for debugging 00030 TSIAnalogSlider tsi(ELEC0, ELEC1, 40); // The Analog slider 00031 Timeout scan; 00032 00033 00034 int candidate=-1; // possible button push (-1 is none) 00035 volatile int realbtn=-1; // actual button push (-1 is none) 00036 volatile int hold=0; // waiting for button release when 1 00037 00038 // internal function to get raw button state 00039 int getrawbtn() 00040 { 00041 float v=tsi.readPercentage(); // read slider 00042 if (v==0.0) return -1; // no button at all 00043 for (int i=0;i<sizeof(BTNMAX)/sizeof(BTNMAX[0]);i++) // classify by zone 00044 if (v<BTNMAX[i]) return i; 00045 return -1; // what? 00046 } 00047 00048 // This is called by the timeout to 00049 // either see there is no swipe 00050 // see that there is a swipe 00051 // or see that there is a button release 00052 void checkbtn(void) 00053 { 00054 int newbtn=getrawbtn(); 00055 if (hold!=0 && newbtn==-1) // wait for key release 00056 { 00057 hold=0; // released so 00058 return; // don't reschedule me 00059 } 00060 // reschedule us for next swipetime 00061 scan.attach(checkbtn,SWIPETIME); 00062 if (hold) return; // still waiting for release 00063 hold=1; // we will be waiting for release from now on 00064 if (newbtn==-1||newbtn==candidate) // if no touch or button is the same, the candidate is the button 00065 { 00066 realbtn=candidate; 00067 return; 00068 } 00069 // Otherwise we are swiping either left or right 00070 if (candidate<newbtn) realbtn=SWIPE_L; else realbtn=SWIPE_R; 00071 return; 00072 } 00073 00074 // This is the main API 00075 // Call it to get a button code (0-3 or 256 or 512) 00076 // You can block or not (default is to block) 00077 int getbtn(bool kwait=true) 00078 { 00079 while (hold) // if holding, either wait or return 00080 { 00081 if (!kwait) return -1; 00082 } 00083 realbtn=-1; // mark that we don't know 00084 do 00085 { 00086 candidate=getrawbtn(); // get a candidate (or return if not waiting) 00087 } while (candidate==-1 || !kwait); 00088 if (candidate==-1) return -1; 00089 scan.attach(checkbtn,SWIPETIME); // schedule the checkbtn routine 00090 while (realbtn==-1); // wait for realbtn to get set 00091 return realbtn; // return it 00092 } 00093 00094 // Simple test program 00095 int main(void) { 00096 00097 while (true) { 00098 pc.printf("Button %d\r\n",getbtn()); 00099 } 00100 }
Generated on Wed Jul 20 2022 10:36:45 by
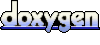