The MAX77734 is a tiny PMIC for applications where size and simplicity are critical. The IC integrates a linear-mode Li+ battery charger, low-dropout linear regulator (LDO), analog multiplexer, and dual-channel current sink driver. Datasheet: https://datasheets.maximintegrated.com/en/ds/MAX77734.pdf Applications ● Hearables: Headsets, Headphones, Earbuds ● Fitness Bands and other Bluetooth Wearables ● Action Cameras, Wearable/Body Cameras ● Low-Power Internet of Things (IoT) Gadgets
max77734.h
00001 /******************************************************************************* 00002 * Copyright (C) 2018 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #ifndef _MAX77734_H_ 00035 #define _MAX77734_H_ 00036 00037 #include "mbed.h" 00038 00039 class MAX77734 00040 { 00041 // https://datasheets.maximintegrated.com/en/ds/MAX77734.pdf 00042 public: 00043 00044 /** 00045 * @brief Register Addresses 00046 * @details Enumerated MAX77734 register addresses 00047 */ 00048 typedef enum { 00049 REG_INT_GLBL = 0x00, 00050 REG_INT_CHG, 00051 REG_STAT_CHG_A, 00052 REG_STAT_CHG_B, 00053 REG_ERCFLAG, 00054 REG_STAT_GLBL, 00055 REG_INTM_GLBL, 00056 REG_INTM_CHG, 00057 REG_CNFG_GLBL, 00058 REG_CID, 00059 REG_CNFG_WDT, 00060 00061 REG_CNFG_CHG_A = 0x20, 00062 REG_CNFG_CHG_B, 00063 REG_CNFG_CHG_C, 00064 REG_CNFG_CHG_D, 00065 REG_CNFG_CHG_E, 00066 REG_CNFG_CHG_F, 00067 REG_CNFG_CHG_G, 00068 REG_CNFG_CHG_H, 00069 REG_CNFG_CHG_I, 00070 00071 REG_CNFG_LDO_A = 0x30, 00072 REG_CNFG_LDO_B, 00073 00074 REG_CNFG_SNK1_A = 0x40, 00075 REG_CNFG_SNK1_B, 00076 REG_CNFG_SNK2_A, 00077 REG_CNFG_SNK2_B, 00078 REG_CNFG_SNK_TOP, 00079 } REG_TYPE; 00080 00081 typedef enum { 00082 I_TERM_5_PERCENT = 0x00, 00083 I_TERM_7P5_PERCENT, 00084 I_TERM_10_PERCENT, 00085 I_TERM_15_PERCENT, 00086 } I_TERM_TYPE; 00087 00088 typedef enum { 00089 VAL_DISABLE = 0, 00090 VAL_ENABLE, 00091 VAL_ENABLE_OPTION1, //OPTION 1: emable when GHGIN is valid 00092 } ENABLE_TYPE; 00093 00094 typedef enum { 00095 LDO_LOW_PWR_MODE = 0x00, 00096 LDO_NORMAL_PWR_MODE, 00097 LDO_PIN_ACT_HIGH, 00098 LDO_PIN_ACT_LOW, 00099 } POWER_MODE_TYPE; 00100 00101 /** 00102 * MAX77734 constructor. 00103 * 00104 * @param sda mbed pin to use for SDA line of I2C interface. 00105 * @param scl mbed pin to use for SCL line of I2C interface. 00106 */ 00107 MAX77734(PinName sda, PinName scl); 00108 00109 /** 00110 * MAX77734 constructor. 00111 * 00112 * @param i2c I2C object to use. 00113 */ 00114 MAX77734(I2C *i2c); 00115 00116 /** 00117 * MAX77734 destructor. 00118 */ 00119 ~MAX77734(); 00120 00121 /** 00122 * @brief Initialize MAX77734 00123 */ 00124 int32_t init(); 00125 00126 /** 00127 * @brief I2C Write 00128 * @details Writes data to MAX77734 register 00129 * 00130 * @param reg_addr Register to write 00131 * @param reg_data Data to write 00132 * @returns 0 if no errors, -1 if error. 00133 */ 00134 int32_t i2c_write_byte(MAX77734::REG_TYPE reg_addr, char reg_data); 00135 int32_t i2c_write_bytes(MAX77734::REG_TYPE startReg, 00136 MAX77734::REG_TYPE stopReg, 00137 const uint8_t *data); 00138 /** 00139 * @brief I2C Read 00140 * @details Reads data from MAX77734 register 00141 * 00142 * @param reg_addr Register to read 00143 * @returns data if no errors, -1 if error. 00144 */ 00145 int32_t i2c_read_byte(MAX77734::REG_TYPE reg_addr); 00146 int32_t i2c_read_bytes(MAX77734::REG_TYPE startReg, 00147 MAX77734::REG_TYPE stopReg, 00148 uint8_t *data); 00149 00150 int32_t i2c_update_byte(MAX77734::REG_TYPE reg_addr, 00151 char set_data, char mask); 00152 00153 00154 /** 00155 * @brief read_register 00156 * @details Reads from the chip. 00157 * 00158 * @param MAX77734 Register Type. 00159 * @returns register value. 00160 */ 00161 int32_t read_register(MAX77734::REG_TYPE reg_addr); 00162 00163 /** 00164 * @brief interrupt_isr 00165 * @details interrupt service routine. 00166 * 00167 * @param none. 00168 * @returns return interrupt status register values. 00169 */ 00170 int32_t interrupt_isr(); 00171 00172 /** 00173 * @brief fset_i_fastchg_uA 00174 * @details set fast charging current. 00175 * 00176 * @param current in uA. 00177 * @returns -1 for error. 00178 */ 00179 int32_t set_i_fastchg_uA(int32_t current); 00180 00181 /** 00182 * @brief set_time_fastchg_hour. 00183 * @details update REG_CNFG_CHG_E register. 00184 * 00185 * @param time in hours. 00186 * @returns -1 for error. 00187 */ 00188 int32_t set_time_fastchg_hour(int32_t hour); 00189 00190 /** 00191 * @brief set_v_fastchg_mA. 00192 * @details update REG_CNFG_CHG_G register. 00193 * 00194 * @param voltage in mV. 00195 * @returns -1 for error. 00196 */ 00197 int32_t set_v_fastchg_mA(int32_t voltage); 00198 00199 /** 00200 * @brief set_i_term_percent. 00201 * @details update REG_CNFG_CHG_C register.. 00202 * 00203 * @param termination percent. 00204 * @returns -1 for error. 00205 */ 00206 int32_t set_i_term_percent(MAX77734::I_TERM_TYPE percent); 00207 00208 /** 00209 * @brief set_time_topoff_min. 00210 * @details update REG_CNFG_CHG_C register.. 00211 * 00212 * @param top off time in minute. 00213 * @returns -1 for error. 00214 */ 00215 int32_t set_time_topoff_min(int32_t minute); 00216 00217 /** 00218 * @brief set_charger_enable. 00219 * @details update REG_CNFG_CHG_B register. 00220 * 00221 * @param . 00222 * @returns -1 for error. 00223 */ 00224 int32_t set_charger_enable(MAX77734::ENABLE_TYPE control); 00225 00226 /** 00227 * @brief set_ldo_enable. 00228 * @details update REG_CNFG_LDO_B register. 00229 * 00230 * @param enable/disable. 00231 * @returns -1 for error. 00232 */ 00233 int32_t set_ldo_enable(MAX77734::ENABLE_TYPE control); 00234 00235 /** 00236 * @brief set_ldo_pwr_mode. 00237 * @details update REG_CNFG_LDO_B register. 00238 * 00239 * @param power mode. 00240 * @returns -1 for error. 00241 */ 00242 int32_t set_ldo_pwr_mode(MAX77734::POWER_MODE_TYPE mode); 00243 00244 /** 00245 * @brief set_ldo_voltage_mV. 00246 * @details update REG_CNFG_LDO_A register. 00247 * 00248 * @param voltage in mV. 00249 * @returns -1 for error. 00250 */ 00251 int32_t set_ldo_voltage_mV(int32_t voltage); 00252 00253 00254 00255 private: 00256 00257 I2C *i2c_; 00258 bool i2c_owner; 00259 00260 }; 00261 00262 #endif /* _MAX77734_H_ */
Generated on Fri Jul 15 2022 08:43:39 by
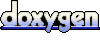