MAX77658 Ultra-Low Power PMIC Mbed Driver
Embed:
(wiki syntax)
Show/hide line numbers
MAX77658.h
00001 /******************************************************************************* 00002 * Copyright(C) Analog Devices Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files(the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Analog Devices Inc. 00023 * shall not be used except as stated in the Analog Devices Inc. 00024 * Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Analog Devices Inc.retains all ownership rights. 00030 ******************************************************************************* 00031 */ 00032 00033 #ifndef _MAX77658_H_ 00034 #define _MAX77658_H_ 00035 00036 #include "mbed.h" 00037 #include "MAX77658_regs.h" 00038 00039 #define MAX77658_NO_ERROR 0 00040 #define MAX77658_VALUE_NULL -1 00041 #define MAX77658_WRITE_DATA_FAILED -2 00042 #define MAX77658_READ_DATA_FAILED -3 00043 #define MAX77658_INVALID_DATA -4 00044 00045 #define MAX77658_I2C_ADDRESS_PMIC_0 0x80 00046 #define MAX77658_I2C_ADDRESS_PMIC_1 0x90 00047 #define MAX77658_I2C_ADDRESS_FG 0x6C 00048 00049 /** 00050 * @brief MAX77658 Ultra-Low Power PMIC Featuring Single-Inductor, 3-Output Buck-Boost, 2-LDOs, 00051 * Power-Path Charger for Small Li+, Fuel Gauge M5. 00052 * 00053 * @details The MAX77658 provides highly-integrated battery charging and power supply solutions 00054 * for low-power applications where size and efficiency are critical. 00055 * 00056 * @code 00057 * @endcode 00058 */ 00059 00060 class MAX77658 00061 { 00062 private: 00063 I2C *i2c_handler; 00064 InterruptIn *irq_pin; // interrupt pin 00065 00066 /** 00067 * @brief Register Addresses 00068 * @details Enumerated MAX77658 register addresses 00069 */ 00070 typedef enum { 00071 /*Global*/ 00072 INT_GLBL0 = 0x00, // Interrupt Status 0 00073 INT_GLBL1 = 0x04, // Interrupt Status 1 00074 ERCFLAG = 0x05, // Flags 00075 STAT_GLBL = 0x06, // Global Status 00076 INTM_GLBL1 = 0x08, // Interrupt Mask 1 00077 INTM_GLBL0 = 0x09, // Interrupt Mask 0 00078 CNFG_GLBL = 0x10, // Configuration Global 00079 CNFG_GPIO0 = 0x11, // GPIO0 Configuration 00080 CNFG_GPIO1 = 0x12, // GPIO1 Configuration 00081 CNFG_GPIO2 = 0x13, // GPIO2 Configuration 00082 CID = 0x14, // Chip Identification Code 00083 CNFG_WDT = 0x17, // Configuration WatchDog Timer 00084 /*Charger*/ 00085 INT_CHG = 0x01, // Charger Interrupt Status 00086 STAT_CHG_A = 0x02, // Charger Status A 00087 STAT_CHG_B = 0x03, // Charger Status B 00088 INT_M_CHG = 0x07, // Charger Interrupt Mask 00089 CNFG_CHG_A = 0x20, // Charger Configuration A 00090 CNFG_CHG_B = 0x21, // Charger Configuration B 00091 CNFG_CHG_C = 0x22, // Charger Configuration C 00092 CNFG_CHG_D = 0x23, // Charger Configuration D 00093 CNFG_CHG_E = 0x24, // Charger Configuration E 00094 CNFG_CHG_F = 0x25, // Charger Configuration F 00095 CNFG_CHG_G = 0x26, // Charger Configuration G 00096 CNFG_CHG_H = 0x27, // Charger Configuration H 00097 CNFG_CHG_I = 0x28, // Charger Configuration I 00098 /*SBB*/ 00099 CNFG_SBB_TOP = 0x38, // SIMO Buck-Boost Configuration 00100 CNFG_SBB0_A = 0x39, // SIMO Buck-Boost 0 Configuration A 00101 CNFG_SBB0_B = 0x3A, // SIMO Buck-Boost 0 Configuration B 00102 CNFG_SBB1_A = 0x3B, // SIMO Buck-Boost 1 Configuration A 00103 CNFG_SBB1_B = 0x3C, // SIMO Buck-Boost 1 Configuration B 00104 CNFG_SBB2_A = 0x3D, // SIMO Buck-Boost 2 Configuration A 00105 CNFG_SBB2_B = 0x3E, // SIMO Buck-Boost 2 Configuration B 00106 CNFG_DVS_SBB0_A = 0x3F, // SIMO Buck-Boost 0 DVS Configuration A 00107 /*LDO*/ 00108 CNFG_LDO0_A = 0x48, // LDO0 Output Voltage 00109 CNFG_LDO0_B = 0x49, // LDO0 Output Voltage Configuration 00110 CNFG_LDO1_A = 0x4A, // LDO1 Output Voltage 00111 CNFG_LDO1_B = 0x4B, // LDO2 Output Voltage Configuration 00112 /*FuelGauge*/ 00113 Status = 0x00, // Interrupt status register for the FG block 00114 VAlrtTh = 0x01, // Voltage AIrt 00115 TAlrtTh = 0x02, // Temperature AIrt 00116 SAlrtTh = 0x03, // Soc AIrt 00117 FullSocThr = 0x13, // Full Soc Thr 00118 DesignCap = 0x18, // Design Cap 00119 Config = 0x1D, // Configuration 00120 IChgTerm = 0x1E, // IChg Term 00121 DevName = 0x21, // Dev Name 00122 FilterCfg = 0x29, // Filter Configuration 00123 IAvgEmpty = 0x36, // IAvgEmpty 00124 VEmpty = 0x3A, // VEmpty 00125 Config2 = 0xBB, // Configuration 2 00126 Temp = 0x08, // Temp 00127 Vcell = 0x09, // Vcell 00128 Current = 0x0A, // Current 00129 AvgCurrent = 0x0B, // AvgCurrent 00130 AvgTA = 0x16, // AvgTA 00131 AvgVCell = 0x19, // AvgVCell 00132 MaxMinTemp = 0x1A, // MaxMinTemp 00133 MaxMinVolt = 0x1B, // MaxMinVolt 00134 MaxMinCurr = 0x1C, // MaxMinCurr 00135 AIN0 = 0x27, // AIN0 00136 Timer = 0x3E, // Timer 00137 ShdnTimer = 0x3F, // ShdnTimer 00138 TimerH = 0xBE, // TimerH 00139 RepCap = 0x05, // RepCap 00140 RepSOC = 0x06, // RepSOC 00141 AvSOC = 0x0E, // AvSOC 00142 FullCapRep = 0x10, // FullCapRep 00143 TTE = 0x11, // TTE 00144 RCell = 0x14, // RCell 00145 Cycles = 0x17, // Cycles 00146 AvCap = 0x1F, // AvCap 00147 TTF = 0x20 // TTF 00148 } reg_t; 00149 00150 void interrupt_handler(); 00151 00152 void (MAX77658::*funcptr)(void); 00153 00154 void post_interrupt_work(); 00155 00156 Thread *post_intr_work_thread; 00157 00158 struct handler { 00159 void (*func)(void *); 00160 void *cb; 00161 }; 00162 00163 handler *interrupt_handler_list; 00164 00165 public: 00166 /** 00167 * @brief MAX77658 constructor. 00168 */ 00169 MAX77658(I2C *i2c, PinName IRQPin = NC); 00170 00171 /** 00172 * @brief MAX77658 destructor. 00173 */ 00174 ~MAX77658(); 00175 00176 /** 00177 * @brief Function pointer type to interrupt handler function 00178 */ 00179 typedef void (*interrupt_handler_function)(void *); 00180 00181 /** 00182 * @brief Read from a register. 00183 * 00184 * @param[in] reg Address of a register to be written. 00185 * @param[out] value Pointer to save result value. 00186 * 00187 * @returns 0 on success, negative error code on failure. 00188 */ 00189 int read_register(uint8_t reg, uint8_t *value); 00190 00191 /** 00192 * @brief Write to a register. 00193 * 00194 * @param[in] reg Address of a register to be written. 00195 * @param[out] value Pointer of value to be written to register. 00196 * 00197 * @returns 0 on success, negative error code on failure. 00198 */ 00199 int write_register(uint8_t reg, const uint8_t *value); 00200 00201 /** 00202 * @brief Read from a fuel-gauge register. 00203 * 00204 * @param[in] reg Address of a fuel-gauge register to be written. 00205 * @param[out] value Pointer to save result value. 00206 * 00207 * @returns 0 on success, negative error code on failure. 00208 */ 00209 int read_fg_register(uint8_t reg, uint8_t *value); 00210 00211 /** 00212 * @brief Write to a fuel-gauge register. 00213 * 00214 * @param[in] reg Address of a fuel-gauge register to be written. 00215 * @param[out] value Pointer of value to be written to fuel-gauge register. 00216 * 00217 * @returns 0 on success, negative error code on failure. 00218 */ 00219 int write_fg_register(uint8_t reg, const uint8_t *value); 00220 00221 /** 00222 * @brief Register Configuration. 00223 * All Interrupt Flags combined from INT_GLBL0, INT_GLBL1 and INT_CHG. 00224 * 00225 * @details 00226 * - Register : INT_GLBL0 (0x00), INT_GLBL1(0x04) and INT_CHG (0x01) 00227 * - Bit Fields : 00228 * - Default : 0x0 00229 * - Description : Enumerated interrupts. 00230 */ 00231 typedef enum { 00232 INT_GLBL0_GPI0_F, 00233 INT_GLBL0_GPI0_R, 00234 INT_GLBL0_NEN_F, 00235 INT_GLBL0_NEN_R, 00236 INT_GLBL0_TJAL1_R, 00237 INT_GLBL0_TJAL2_R, 00238 INT_GLBL0_DOD1_R, 00239 INT_GLBL0_DOD0_R, 00240 INT_GLBL1_GPI1_F, 00241 INT_GLBL1_GPI1_R, 00242 INT_GLBL1_SBB0_F, 00243 INT_GLBL1_SBB1_F, 00244 INT_GLBL1_SBB2_F, 00245 INT_GLBL1_LDO0_F, 00246 INT_GLBL1_LDO1_F, 00247 INT_GLBL1_RSVD, 00248 INT_CHG_THM_I, 00249 INT_CHG_CHG_I, 00250 INT_CHG_CHGIN_I, 00251 INT_CHG_TJ_REG_I, 00252 INT_CHG_CHGIN_CTRL_I, 00253 INT_CHG_SYS_CTRL_I, 00254 INT_CHG_SYS_CNFG_I, 00255 INT_CHG_RSVD, 00256 INT_CHG_END 00257 } reg_bit_int_glbl_t; 00258 00259 /** 00260 * @brief Register Configuration 00261 * 00262 * @details 00263 * - Register : ERCFLAG (0x05) 00264 * - Bit Fields : [7:0] 00265 * - Default : 0x0 00266 * - Description : Event Recorder Flags. 00267 */ 00268 typedef enum { 00269 ERCFLAG_TOVLD, 00270 ERCFLAG_SYSOVLO, 00271 ERCFLAG_SYSUVLO, 00272 ERCFLAG_MRST_F, 00273 ERCFLAG_SFT_OFF_F, 00274 ERCFLAG_SFT_CRST_F, 00275 ERCFLAG_WDT_OFF, 00276 ERCFLAG_WDT_RST 00277 }reg_bit_ercflag_t; 00278 00279 /** 00280 * @brief Get bit field of ERCFLAG (0x05) register. 00281 * 00282 * @param[in] bit_field ERCFLAG register bit field to be written. 00283 * @param[out] flag Pointer to save result of ercglag bit states. 00284 * For individual bit 00285 * 0x0: ERCFLAG has not occurred, 00286 * 0x1: ERCFLAG has occurred. 00287 * 00288 * @return 0 on success, error code on failure. 00289 */ 00290 int get_ercflag(reg_bit_ercflag_t bit_field, uint8_t *flag); 00291 00292 /** 00293 * @brief Register Configuration 00294 * 00295 * @details 00296 * - Register : STAT_GLBL (0x06) 00297 * - Bit Fields : [7:0] 00298 * - Default : 0x0 00299 * - Description : Event Recorder Flags. 00300 */ 00301 typedef enum { 00302 STAT_GLBL_STAT_IRQ, 00303 STAT_GLBL_STAT_EN, 00304 STAT_GLBL_TJAL1_S, 00305 STAT_GLBL_TJAL2_S, 00306 STAT_GLBL_DOD1_S, 00307 STAT_GLBL_DOD0_S, 00308 STAT_GLBL_BOK, 00309 STAT_GLBL_DIDM 00310 }reg_bit_stat_glbl_t; 00311 00312 /** 00313 * @brief Get bit field of STAT_GLBL (0x06) register. 00314 * 00315 * @param[in] bit_field STAT_GLBL register bit field to be written. 00316 * @param[out] status Pointer to save result of Status Global bit state. 00317 * 00318 * @return 0 on success, error code on failure. 00319 */ 00320 int get_stat_glbl(reg_bit_stat_glbl_t bit_field, uint8_t *status); 00321 00322 /** 00323 * @brief Register Configuration 00324 * 00325 * @details 00326 * - Register : INT_M_CHG (0x07), INTM_GLBL0 (0x08) and INTM_GLBL1 (0x09) 00327 * - Bit Fields : [7:0] 00328 * - Default : 0x0 00329 * - Description : All interrupt mask bits. 00330 */ 00331 typedef enum { 00332 INT_M_CHG_THM_M, 00333 INT_M_CHG_CHG_M, 00334 INT_M_CHG_CHGIN_M, 00335 INT_M_CHG_TJ_REG_M, 00336 INT_M_CHG_CHGIN_CTRL_M, 00337 INT_M_CHG_SYS_CTRL_M, 00338 INT_M_CHG_SYS_CNFG_M, 00339 INT_M_CHG_DIS_AICL, 00340 INTM_GLBL0_GPI0_FM, 00341 INTM_GLBL0_GPI0_RM, 00342 INTM_GLBL0_nEN_FM, 00343 INTM_GLBL0_nEN_RM, 00344 INTM_GLBL0_TJAL1_RM, 00345 INTM_GLBL0_TJAL2_RM, 00346 INTM_GLBL0_DOD1_RM, 00347 INTM_GLBL0_DOD0_RM, 00348 INTM_GLBL1_GPI1_FM, 00349 INTM_GLBL1_GPI1_RM, 00350 INTM_GLBL1_SBB0_FM, 00351 INTM_GLBL1_SBB1_FM, 00352 INTM_GLBL1_SBB2_FM, 00353 INTM_GLBL1_LDO0_M, 00354 INTM_GLBL1_LDO1_M, 00355 INTM_GLBL1_RSVD, 00356 INTM_NUM_OF_BIT 00357 }reg_bit_int_mask_t; 00358 00359 /** 00360 * @brief Set bit field of INT_M_CHG (0x07), INTM_GLBL0 (0x08) or INTM_GLBL1 (0x09) register. 00361 * 00362 * @param[in] bit_field Register bit field to be set. 00363 * @param[out] maskBit 0x0: Interrupt is unmasked, 00364 * 0x1: Interrupt is masked. 00365 * 00366 * @return 0 on success, error code on failure. 00367 */ 00368 int set_interrupt_mask(reg_bit_int_mask_t bit_field, uint8_t maskBit); 00369 00370 /** 00371 * @brief Get bit field of INT_M_CHG (0x07), INTM_GLBL0 (0x08) or INTM_GLBL1 (0x09) register. 00372 * 00373 * @param[in] bit_field Register bit field to be written. 00374 * @param[out] maskBit 0x0: Interrupt is unmasked, 00375 * 0x1: Interrupt is masked. 00376 * 00377 * @return 0 on success, error code on failure. 00378 */ 00379 int get_interrupt_mask(reg_bit_int_mask_t bit_field, uint8_t *maskBit); 00380 00381 /** 00382 * @brief Register Configuration 00383 * 00384 * @details 00385 * - Register : CNFG_GLBL (0x10) 00386 * - Bit Fields : [7:0] 00387 * - Default : 0x0 00388 * - Description : Event Recorder Flags. 00389 */ 00390 typedef enum { 00391 CNFG_GLBL_SFT_CTRL, 00392 CNFG_GLBL_DBEN_nEN, 00393 CNFG_GLBL_nEN_MODE, 00394 CNFG_GLBL_SBIA_LPM, 00395 CNFG_GLBL_T_MRST, 00396 CNFG_GLBL_PU_DIS 00397 }reg_bit_cnfg_glbl_t; 00398 00399 /** 00400 * @brief Set CNFG_GLBL (0x10) register. 00401 * 00402 * @param[in] bit_field Register bit field to be written. 00403 * @param[in] config Register bit field to be written. 00404 * 00405 * @return 0 on success, error code on failure. 00406 */ 00407 int set_cnfg_glbl(reg_bit_cnfg_glbl_t bit_field, uint8_t config); 00408 00409 /** 00410 * @brief Get CNFG_GLBL (0x10) register. 00411 * 00412 * @param[in] bit_field Register bit field to be written. 00413 * @param[out] config Pointer of value to be read. 00414 * 00415 * @return 0 on success, error code on failure. 00416 */ 00417 int get_cnfg_glbl(reg_bit_cnfg_glbl_t bit_field, uint8_t *config); 00418 00419 /** 00420 * @brief Register Configuration 00421 * 00422 * @details 00423 * - Register : CNFG_GPIO0 (0x11), CNFG_GPIO1 (0x12) or CNFG_GPIO2 (0x13) 00424 * - Bit Fields : [7:0] 00425 * - Default : 0x0 00426 * - Description : Event Recorder Flags. 00427 */ 00428 typedef enum { 00429 CNFG_GPIO_DIR, 00430 CNFG_GPIO_DI, 00431 CNFG_GPIO_DRV, 00432 CNFG_GPIO_DO, 00433 CNFG_GPIO_DBEN_GPI, 00434 CNFG_GPIO_ALT_GPIO, 00435 CNFG_GPIO_RSVD 00436 }reg_bit_cnfg_gpio_t; 00437 00438 /** 00439 * @brief Set either CNFG_GPIO0 (0x11), CNFG_GPIO1 (0x12) or CNFG_GPIO2 (0x13). 00440 * 00441 * @param[in] bit_field Register bit field to be written. 00442 * @param[in] channel Channel number: 0, 1 or 2 00443 * @param[in] config Register bit field to be written. 00444 * 00445 * @return 0 on success, error code on failure. 00446 */ 00447 int set_cnfg_gpio(reg_bit_cnfg_gpio_t bit_field, uint8_t channel, uint8_t config); 00448 00449 /** 00450 * @brief Get either CNFG_GPIO0 (0x11), CNFG_GPIO1 (0x12) or CNFG_GPIO2 (0x13). 00451 * 00452 * @param[in] bit_field Register bit field to be written. 00453 * @param[in] channel Channel number: 0, 1 or 2 00454 * @param[out] config Pointer of value to be read. 00455 * 00456 * @return 0 on success, error code on failure. 00457 */ 00458 int get_cnfg_gpio(reg_bit_cnfg_gpio_t bit_field, uint8_t channel, uint8_t *config); 00459 00460 /** 00461 * @brief Get bit field of CID (0x14) register. 00462 * 00463 * @return CID on success, error code on failure. 00464 */ 00465 int get_cid(void); 00466 00467 /** 00468 * @brief Register Configuration 00469 * 00470 * @details 00471 * - Register : CNFG_WDT (0x17) 00472 * - Bit Fields : [7:0] 00473 * - Default : 0x0 00474 * - Description : Watchdog Timer Configuration. 00475 */ 00476 typedef enum { 00477 CNFG_WDT_WDT_LOCK, 00478 CNFG_WDT_WDT_EN, 00479 CNFG_WDT_WDT_CLR, 00480 CNFG_WDT_WDT_MODE, 00481 CNFG_WDT_WDT_PER, 00482 CNFG_WDT_RSVD 00483 }reg_bit_cnfg_wdt_t; 00484 00485 /** 00486 * @brief Set CNFG_WDT (0x17) register. 00487 * 00488 * @param[in] bit_field Register bit field to be written. 00489 * @param[in] config Field value to be written. 00490 * 00491 * @return 0 on success, error code on failure. 00492 */ 00493 int set_cnfg_wdt(reg_bit_cnfg_wdt_t bit_field, uint8_t config); 00494 00495 /** 00496 * @brief Get CNFG_WDT (0x17) register. 00497 * 00498 * @param[in] bit_field Register bit field to be written. 00499 * @param[out] config Pointer of value to be read. 00500 * 00501 * @return 0 on success, error code on failure. 00502 */ 00503 int get_cnfg_wdt(reg_bit_cnfg_wdt_t bit_field, uint8_t *config); 00504 00505 /** 00506 * @brief Register Configuration 00507 * 00508 * @details 00509 * - Register : STAT_CHG_A (0x02) 00510 * - Bit Fields : [7:0] 00511 * - Default : 0x0 00512 * - Description : Watchdog Timer Configuration. 00513 */ 00514 typedef enum { 00515 STAT_CHG_A_THM_DTLS, 00516 STAT_CHG_A_TJ_REG_STAT, 00517 STAT_CHG_A_VSYS_MIN_STAT, 00518 STAT_CHG_A_ICHGIN_LIM_STAT, 00519 STAT_CHG_A_VCHGIN_MIN_STAT, 00520 STAT_CHG_A_RSVD 00521 }reg_bit_stat_chg_a_t; 00522 00523 /** 00524 * @brief Get STAT_CHG_A (0x02) register. 00525 * 00526 * @param[in] bit_field Register bit field to be written. 00527 * @param[out] status Pointer of value to be read. 00528 * For individual bit, 00529 * 0x0 = It is not engaged, 00530 * 0x1 = It is engaged. 00531 * 00532 * @return 0 on success, error code on failure. 00533 */ 00534 int get_stat_chg_a(reg_bit_stat_chg_a_t bit_field, uint8_t *status); 00535 00536 /** 00537 * @brief Register Configuration 00538 * 00539 * @details 00540 * - Register : STAT_CHG_A (0x02) 00541 * - Bit Fields : [2:0] 00542 * - Default : 0x0 00543 * - Description : Battery Temperature Details. 00544 */ 00545 typedef enum { 00546 THM_DTLS_THERMISTOR_DISABLED, 00547 THM_DTLS_BATTERY_COLD, 00548 THM_DTLS_BATTERY_COOL, 00549 THM_DTLS_BATTERY_WARM, 00550 THM_DTLS_BATTERY_HOT, 00551 THM_DTLS_BATTERY_NORMAL, 00552 THM_DTLS_RESERVED_0x06, 00553 THM_DTLS_RESERVED_0x07 00554 }decode_thm_dtls_t; 00555 00556 /** 00557 * @brief Get Battery Temperature Details. 00558 * Valid only when CHGIN_DTLS[1:0] = 0b11. 00559 * 00560 * @param[out] thm_dtls Battery temperature details field to be read. 00561 * 00562 * @return 0 on success, error code on failure. 00563 */ 00564 int get_thm_dtls(decode_thm_dtls_t *thm_dtls); 00565 00566 /** 00567 * @brief Register Configuration 00568 * 00569 * @details 00570 * - Register : STAT_CHG_B (0x03) 00571 * - Bit Fields : [7:0] 00572 * - Default : 0x0 00573 * - Description : Watchdog Timer Configuration. 00574 */ 00575 typedef enum { 00576 STAT_CHG_B_TIME_SUS, 00577 STAT_CHG_B_CHG, 00578 STAT_CHG_B_CHGIN_DTLS, 00579 STAT_CHG_B_CHG_DTLS 00580 }reg_bit_stat_chg_b_t; 00581 00582 /** 00583 * @brief Get STAT_CHG_B (0x03) register. 00584 * 00585 * @param[in] bit_field Register bit field to be written. 00586 * @param[out] status Pointer of value to be read. 00587 * 00588 * @return 0 on success, error code on failure. 00589 */ 00590 int get_stat_chg_b(reg_bit_stat_chg_b_t bit_field, uint8_t *status); 00591 00592 /** 00593 * @brief Register Configuration 00594 * 00595 * @details 00596 * - Register : STAT_CHG_B (0x03) 00597 * - Bit Fields : [7:4] 00598 * - Default : 0x0 00599 * - Description : Charger Details. 00600 */ 00601 typedef enum { 00602 CHG_DTLS_OFF, 00603 CHG_DTLS_PREQUALIFICATION_MODE, 00604 CHG_DTLS_FAST_CHARGE_CC, 00605 CHG_DTLS_JEITA_FAST_CHARGE_CC, 00606 CHG_DTLS_FAST_CHARGE_CV, 00607 CHG_DTLS_JEITA_FAST_CHARGE_CV, 00608 CHG_DTLS_TOP_OFF_MODE, 00609 CHG_DTLS_JEITA_MODIFIED_TOP_OFF_MODE, 00610 CHG_DTLS_DONE, 00611 CHG_DTLS_JEITA_MODIFIED_DONE, 00612 CHG_DTLS_PREQUALIFICATION_TIMER_FAULT, 00613 CHG_DTLS_FAST_CHARGE_TIMER_FAULT, 00614 CHG_DTLS_BATTERY_TEMPERATURE_FAULT, 00615 CHG_DTLS_RESERVED_0x0D, 00616 CHG_DTLS_RESERVED_0x0E, 00617 CHG_DTLS_RESERVED_0x0F 00618 }decode_chg_dtls_t; 00619 00620 /** 00621 * @brief Get Charger Details. 00622 * 00623 * @param[out] chg_dtls Charger details field to be read. 00624 * 00625 * @return 0 on success, error code on failure. 00626 */ 00627 int get_chg_dtls(decode_chg_dtls_t *chg_dtls); 00628 00629 /** 00630 * @brief Set the VHOT JEITA Temperature Threshold. 00631 * Bit 7:6 of CNFG_CHG_A (0x20) register. 00632 * 00633 * @param[in] tempDegC Register bit field to be read. 00634 * 45ºC, 50ºC, 55ºC or 60ºC. 00635 * 00636 * @return 0 on success, error code on failure. 00637 */ 00638 int set_thm_hot(int tempDegC); 00639 00640 /** 00641 * @brief Get the VHOT JEITA Temperature Threshold. 00642 * Bit 7:6 of CNFG_CHG_A (0x20) register. 00643 * 00644 * @param[out] tempDegC Pointer of value to be read. 00645 * 45ºC, 50ºC, 55ºC or 60ºC. 00646 * 00647 * @return 0 on success, error code on failure. 00648 */ 00649 int get_thm_hot(int *tempDegC); 00650 00651 /** 00652 * @brief Set the VWARM JEITA Temperature Threshold. 00653 * Bit 5:4 of CNFG_CHG_A (0x20) register. 00654 * 00655 * @param[in] tempDegC Register bit field to be read. 00656 * 35ºC, 40ºC, 45ºC or 50ºC. 00657 * 00658 * @return 0 on success, error code on failure. 00659 */ 00660 int set_thm_warm(int tempDegC); 00661 00662 /** 00663 * @brief Get the VWARM JEITA Temperature Threshold. 00664 * Bit 5:4 of CNFG_CHG_A (0x20) register. 00665 * 00666 * @param[out] tempDegC Pointer of value to be read. 00667 * 35ºC, 40ºC, 45ºC or 50ºC. 00668 * 00669 * @return 0 on success, error code on failure. 00670 */ 00671 int get_thm_warm(int *tempDegC); 00672 00673 /** 00674 * @brief Set the VCOOL JEITA Temperature Threshold. 00675 * Bit 3:2 of CNFG_CHG_A (0x20) register. 00676 * 00677 * @param[in] tempDegC Register bit field to be read. 00678 * 0ºC, 5ºC, 10ºC or 15ºC. 00679 * 00680 * @return 0 on success, error code on failure. 00681 */ 00682 int set_thm_cool(int tempDegC); 00683 00684 /** 00685 * @brief Get the VCOOL JEITA Temperature Threshold. 00686 * Bit 3:2 of CNFG_CHG_A (0x20) register. 00687 * 00688 * @param[out] tempDegC Pointer of value to be read. 00689 * 0ºC, 5ºC, 10ºC or 15ºC. 00690 * 00691 * @return 0 on success, error code on failure. 00692 */ 00693 int get_thm_cool(int *tempDegC); 00694 00695 /** 00696 * @brief Set the VCOLD JEITA Temperature Threshold. 00697 * Bit 1:0 of CNFG_CHG_A (0x20) register. 00698 * 00699 * @param[in] tempDegC Register bit field to be read. 00700 * -10ºC, -5ºC, 0ºC or 5ºC. 00701 * 00702 * @return 0 on success, error code on failure. 00703 */ 00704 int set_thm_cold(int tempDegC); 00705 00706 /** 00707 * @brief Get the VCOLD JEITA Temperature Threshold. 00708 * Bit 1:0 of CNFG_CHG_A (0x20) register. 00709 * 00710 * @param[out] tempDegC Pointer of value to be read. 00711 * -10ºC, -5ºC, 0ºC or 5ºC. 00712 * 00713 * @return 0 on success, error code on failure. 00714 */ 00715 int get_thm_cold(int *tempDegC); 00716 00717 /** 00718 * @brief Register Configuration 00719 * 00720 * @details 00721 * - Register : CNFG_CHG_B (0x21) 00722 * - Bit Fields : [7:0] 00723 * - Default : 0x0 00724 * - Description : Watchdog Timer Configuration. 00725 */ 00726 typedef enum { 00727 CNFG_CHG_B_CHG_EN, 00728 CNFG_CHG_B_I_PQ, 00729 CNFG_CHG_B_ICHGIN_LIM, 00730 CNFG_CHG_B_VCHGIN_MIN 00731 }reg_bit_cnfg_chg_b_t; 00732 00733 /** 00734 * @brief Set CNFG_CHG_B (0x21) register. 00735 * 00736 * @param[in] bit_field Register bit field to be written. 00737 * @param[in] config Register bit field to be written. 00738 * 00739 * @return 0 on success, error code on failure. 00740 */ 00741 int set_cnfg_chg_b(reg_bit_cnfg_chg_b_t bit_field, uint8_t config); 00742 00743 /** 00744 * @brief Get CNFG_CHG_B (0x21) register. 00745 * 00746 * @param[in] bit_field Register bit field to be written. 00747 * @param[out] config Pointer of value to be read. 00748 * 00749 * @return 0 on success, error code on failure. 00750 */ 00751 int get_cnfg_chg_b(reg_bit_cnfg_chg_b_t bit_field, uint8_t *config); 00752 00753 /** 00754 * @brief Set Minimum CHGIN Regulation Voltage. 00755 * Bit 7:5 of CNFG_CHG_B (0x21) register. 00756 * 00757 * @param[in] voltV 00758 * 4.0V, 4.1V, 4.2V, 4.3V, 00759 * 4.4V, 4.5V, 4.6V, 4.7V. 00760 * 00761 * @return 0 on success, error code on failure. 00762 */ 00763 int set_vchgin_min(float voltV); 00764 00765 /** 00766 * @brief Get Minimum CHGIN Regulation Voltage. 00767 * Bit 7:5 of CNFG_CHG_B (0x21) register. 00768 * 00769 * @param[in] voltV Pointer of value to be read. 00770 * 4.0V, 4.1V, 4.2V, 4.3V, 00771 * 4.4V, 4.5V, 4.6V, 4.7V. 00772 * 00773 * @return 0 on success, error code on failure. 00774 */ 00775 int get_vchgin_min(float *voltV); 00776 00777 /** 00778 * @brief Set CHGIN Input Current Limit. 00779 * Bit 4:2 of CNFG_CHG_B (0x21) register. 00780 * 00781 * @param[in] currentmA 00782 * 95mA, 190mA, 285mA, 380mA, 475mA. 00783 * 00784 * @return 0 on success, error code on failure. 00785 */ 00786 int set_ichgin_lim(int currentmA); 00787 00788 /** 00789 * @brief Get CHGIN Input Current Limit. 00790 * Bit 4:2 of CNFG_CHG_B (0x21) register. 00791 * 00792 * @param[out] currentmA Pointer of value to be read. 00793 * 95mA, 190mA, 285mA, 380mA, 475mA. 00794 * 00795 * @return 0 on success, error code on failure. 00796 */ 00797 int get_ichgin_lim(int *currentmA); 00798 00799 /** 00800 * @brief Set Battery Prequalification Voltage Threshold (VPQ). 00801 * Bit 7:5 of CNFG_CHG_C (0x22) register. 00802 * 00803 * @param[in] voltV 00804 * 2.3V, 2.4V, 2.5V, 2.6V, 00805 * 2.7V, 2.8V, 2.9V, 3.0V. 00806 * 00807 * @return 0 on success, error code on failure. 00808 */ 00809 int set_chg_pq(float voltV); 00810 00811 /** 00812 * @brief Get Battery Prequalification Voltage Threshold (VPQ). 00813 * Bit 7:5 of CNFG_CHG_C (0x22) register. 00814 * 00815 * @param[out] voltV Pointer of value to be read. 00816 * 2.3V, 2.4V, 2.5V, 2.6V, 00817 * 2.7V, 2.8V, 2.9V, 3.0V. 00818 * 00819 * @return 0 on success, error code on failure. 00820 */ 00821 int get_chg_pq(float *voltV); 00822 00823 /** 00824 * @brief Set Charger Termination Current (ITERM). 00825 * I_TERM[1:0] sets the charger termination current 00826 * as a percentage of the fast charge current IFAST-CHG. 00827 * Bit 4:3 of CNFG_CHG_C (0x22) register. 00828 * 00829 * @param[in] percent 00830 * 5%, 7.5%, 10%, 15%. 00831 * 00832 * @return 0 on success, error code on failure. 00833 */ 00834 int set_i_term(float percent); 00835 00836 /** 00837 * @brief Get Charger Termination Current (ITERM). 00838 * I_TERM[1:0] sets the charger termination current 00839 * as a percentage of the fast charge current IFAST-CHG. 00840 * Bit 4:3 of CNFG_CHG_C (0x22) register. 00841 * 00842 * @param[out] percent Pointer of value to be read. 00843 * 5%, 7.5%, 10%, 15%. 00844 * 00845 * @return 0 on success, error code on failure. 00846 */ 00847 int get_i_term(float *percent); 00848 00849 /** 00850 * @brief Set Top-off Timer Value. 00851 * Bit 2:0 of CNFG_CHG_C (0x22) register. 00852 * 00853 * @param[in] minute 00854 * 0 minutes, 5 minutes, 10 minutes 00855 * 15 minutes, 20 minutes, 25 minutes, 00856 * 30 minutes, 35 minutes. 00857 * 00858 * @return 0 on success, error code on failure. 00859 */ 00860 int set_t_topoff(uint8_t minute); 00861 00862 /** 00863 * @brief Get Top-off Timer Value. 00864 * Bit 2:0 of CNFG_CHG_C (0x22) register. 00865 * 00866 * @param[out] minute Pointer of value to be read. 00867 0 minutes, 5 minutes, 10 minutes 00868 * 15 minutes, 20 minutes, 25 minutes, 00869 * 30 minutes, 35 minutes. 00870 * 00871 * @return 0 on success, error code on failure. 00872 */ 00873 int get_t_topoff(uint8_t *minute); 00874 00875 /** 00876 * @brief Set the Die Junction Temperature Regulation Point, TJ-REG. 00877 * Bit 7:5 of CNFG_CHG_D (0x23) register. 00878 * 00879 * @param[in] tempDegC 60ºC, 70ºC, 80ºC, 00880 * 90ºC, 100ºC. 00881 * 00882 * @return 0 on success, error code on failure. 00883 */ 00884 int set_tj_reg(uint8_t tempDegC); 00885 00886 /** 00887 * @brief Get the Die Junction Temperature Regulation Point, TJ-REG. 00888 * Bit 7:5 of CNFG_CHG_D (0x23) register. 00889 * 00890 * @param[out] tempDegC Pointer of value to be read. 00891 * 60ºC, 70ºC, 80ºC, 90ºC, 100ºC. 00892 * 00893 * @return 0 on success, error code on failure. 00894 */ 00895 int get_tj_reg(uint8_t *tempDegC); 00896 00897 /** 00898 * @brief Set System Voltage Regulation (VSYS-REG). 00899 * Bit 4:0 of CNFG_CHG_D (0x23) register. 00900 * 00901 * @param[in] voltV 3.300V, 3.350V, 3.400V, ... 00902 * 4.750V, 4.800V. 00903 * 00904 * @return 0 on success, error code on failure. 00905 */ 00906 int set_vsys_reg(float voltV); 00907 00908 /** 00909 * @brief Get System Voltage Regulation (VSYS-REG). 00910 * Bit 4:0 of CNFG_CHG_D (0x23) register. 00911 * 00912 * @param[out] voltV Pointer of value to be read. 00913 * 3.300V, 3.350V, 3.400V, ... 00914 * 4.750V, 4.800V. 00915 * 00916 * @return 0 on success, error code on failure. 00917 */ 00918 int get_vsys_reg(float *voltV); 00919 00920 /** 00921 * @brief Set the Fast-Charge Constant Current Value, IFAST-CHG. 00922 * Bit 7:2 of CNFG_CHG_E (0x24) register. 00923 * 00924 * @param[in] currentmA 7.5mA, 15.0mA, 22.5mA, ... 00925 * 292.5mA, 300.0mA. 00926 * 00927 * @return 0 on success, error code on failure. 00928 */ 00929 int set_chg_cc(float currentmA); 00930 00931 /** 00932 * @brief Get the Fast-Charge Constant Current Value, IFAST-CHG. 00933 * Bit 7:2 of CNFG_CHG_E (0x24) register. 00934 * 00935 * @param[out] currentmA Pointer of value to be read. 00936 * 7.5mA, 15.0mA, 22.5mA, ... 00937 * 292.5mA, 300.0mA. 00938 * 00939 * @return 0 on success, error code on failure. 00940 */ 00941 int get_chg_cc(float *currentmA); 00942 00943 /** 00944 * @brief Register Configuration 00945 * 00946 * @details 00947 * - Register : CNFG_CHG_E (0x24) 00948 * - Bit Fields : [1:0] 00949 * - Default : 0x0 00950 * - Description : Fast-charge Safety timer, tFC. 00951 */ 00952 typedef enum { 00953 T_FAST_CHG_TIMER_DISABLED, 00954 T_FAST_CHG_HOUR_3H, 00955 T_FAST_CHG_HOUR_5H, 00956 T_FAST_CHG_HOUR_7H 00957 }decode_t_fast_chg_t; 00958 00959 /** 00960 * @brief Set the Fast-charge Safety timer, tFC. 00961 * Bit 1:0 of CNFG_CHG_E (0x24) register. 00962 * 00963 * @param[in] t_fast_chg Fast-charge safety timer field to be written. 00964 * 00965 * @return 0 on success, error code on failure. 00966 */ 00967 int set_t_fast_chg(decode_t_fast_chg_t t_fast_chg); 00968 00969 /** 00970 * @brief Get the Fast-charge Safety timer, tFC. 00971 * Bit 1:0 of CNFG_CHG_E (0x24) register. 00972 * 00973 * @param[out] t_fast_chg Fast-charge safety timer field to be read. 00974 * 00975 * @return 0 on success, error code on failure. 00976 */ 00977 int get_t_fast_chg(decode_t_fast_chg_t *t_fast_chg); 00978 00979 /** 00980 * @brief Set IFAST-CHG-JEITA 00981 * when the battery is either cool or warm as defined by the 00982 * VCOOL and VWARM temperature thresholds. 00983 * Bit 7:2 of CNFG_CHG_F (0x25) register. 00984 * 00985 * @param[in] currentmA 7.5mA, 15.0mA, 22.5mA, ... 00986 * 292.5mA, 300.0mA. 00987 * 00988 * @return 0 on success, error code on failure. 00989 */ 00990 int set_chg_cc_jeita(float currentmA); 00991 00992 /** 00993 * @brief Get IFAST-CHG-JEITA 00994 * when the battery is either cool or warm as defined by the 00995 * VCOOL and VWARM temperature thresholds. 00996 * Bit 7:2 of CNFG_CHG_F (0x25) register. 00997 * 00998 * @param[out] currentmA Pointer of value to be read. 00999 * 7.5mA, 15.0mA, 22.5mA, ... 01000 * 292.5mA, 300.0mA. 01001 * 01002 * @return 0 on success, error code on failure. 01003 */ 01004 int get_chg_cc_jeita(float *currentmA); 01005 01006 /** 01007 * @brief Register Configuration 01008 * 01009 * @details 01010 * - Register : CNFG_CHG_G (0x26) 01011 * - Bit Fields : [7:0] 01012 * - Default : 0x0 01013 * - Description : Watchdog Timer Configuration. 01014 */ 01015 typedef enum { 01016 CNFG_CHG_G_FUS_M, 01017 CNFG_CHG_G_USBS, 01018 CNFG_CHG_G_CHG_CV 01019 }reg_bit_cnfg_chg_g_t; 01020 01021 /** 01022 * @brief Set CNFG_CHG_G (0x26) register. 01023 * 01024 * @param[in] bit_field Register bit field to be written. 01025 * @param[in] config Register bit field to be written. 01026 * 01027 * @return 0 on success, error code on failure. 01028 */ 01029 int set_cnfg_chg_g(reg_bit_cnfg_chg_g_t bit_field, uint8_t config); 01030 01031 /** 01032 * @brief Get CNFG_CHG_G (0x26) register. 01033 * 01034 * @param[in] bit_field Register bit field to be written. 01035 * @param[out] config Pointer of value to be read. 01036 * 01037 * @return 0 on success, error code on failure. 01038 */ 01039 int get_cnfg_chg_g(reg_bit_cnfg_chg_g_t bit_field, uint8_t *config); 01040 01041 /** 01042 * @brief Set Fast-Charge Battery Regulation Voltage, VFAST-CHG. 01043 * Bit 7:2 of CNFG_CHG_G (0x26) register. 01044 * 01045 * @param[in] voltV 3.600V, 3.625V, 3.650V, ... 01046 * 4.575V, 4.600V. 01047 * 01048 * @return 0 on success, error code on failure. 01049 */ 01050 int set_chg_cv(float voltV); 01051 01052 /** 01053 * @brief Get Fast-Charge Battery Regulation Voltage, VFAST-CHG. 01054 * Bit 7:2 of CNFG_CHG_G (0x26) register. 01055 * 01056 * @param[out] voltV Pointer of value to be read. 01057 * 3.600V, 3.625V, 3.650V, ... 01058 * 4.575V, 4.600V. 01059 * 01060 * @return 0 on success, error code on failure. 01061 */ 01062 int get_chg_cv(float *voltV); 01063 01064 /** 01065 * @brief Register Configuration 01066 * 01067 * @details 01068 * - Register : CNFG_CHG_H (0x27) 01069 * - Bit Fields : [7:0] 01070 * - Default : 0x0 01071 * - Description : Watchdog Timer Configuration. 01072 */ 01073 typedef enum { 01074 CNFG_CHG_H_CHR_TH_DIS, 01075 CNFG_CHG_H_SYS_BAT_PRT, 01076 CNFG_CHG_H_CHG_CV_JEITA 01077 }reg_bit_cnfg_chg_h_t; 01078 01079 /** 01080 * @brief Set CNFG_CHG_H (0x27) register. 01081 * 01082 * @param[in] bit_field Register bit field to be written. 01083 * @param[in] config Register bit field to be written. 01084 * 01085 * @return 0 on success, error code on failure. 01086 */ 01087 int set_cnfg_chg_h(reg_bit_cnfg_chg_h_t bit_field, uint8_t config); 01088 01089 /** 01090 * @brief Get CNFG_CHG_H (0x27) register. 01091 * 01092 * @param[in] bit_field Register bit field to be written. 01093 * @param[out] config Pointer of value to be read. 01094 * 01095 * @return 0 on success, error code on failure. 01096 */ 01097 int get_cnfg_chg_h(reg_bit_cnfg_chg_h_t bit_field, uint8_t *config); 01098 01099 /** 01100 * @brief Set the modified VFAST-CHG-JEITA for when the battery is either 01101 * cool or warm as defined by the VCOOL and VWARM temperature thresholds. 01102 * Bit 7:2 of CNFG_CHG_H (0x27) register. 01103 * 01104 * @param[in] voltV Pointer of value to be read. 01105 * 3.600V, 3.625V, 3.650V, ... 01106 * 4.575V, 4.600V. 01107 * 01108 * @return 0 on success, error code on failure. 01109 */ 01110 int set_chg_cv_jeita(float voltV); 01111 01112 /** 01113 * @brief Get the modified VFAST-CHG-JEITA for when the battery is either 01114 * cool or warm as defined by the VCOOL and VWARM temperature thresholds. 01115 * Bit 7:2 of CNFG_CHG_H (0x27) register. 01116 * 01117 * @param[out] voltV Pointer of value to be read. 01118 * 3.600V, 3.625V, 3.650V, ... 01119 * 4.575V, 4.600V. 01120 * 01121 * @return 0 on success, error code on failure. 01122 */ 01123 int get_chg_cv_jeita(float *voltV); 01124 01125 /** 01126 * @brief Set the Battery Discharge Current Full-Scale Current Value. 01127 * Bit 7:4 of CNFG_CHG_I (0x28) register. 01128 * 01129 * @param[in] currentmA 8.2mA, 40.5mA, 72.3mA, 103.4mA, 01130 * 134.1mA, 164.1mA, 193.7mA, 222.7mA, 01131 * 251.2mA, 279.3mA, 300.0mA 01132 * 01133 * @return 0 on success, error code on failure. 01134 */ 01135 int set_imon_dischg_scale(float currentmA); 01136 01137 /** 01138 * @brief Get the Battery Discharge Current Full-Scale Current Value. 01139 * Bit 7:4 of CNFG_CHG_I (0x28) register. 01140 * 01141 * @param[out] currentmA Pointer of value to be read. 01142 * 8.2mA, 40.5mA, 72.3mA, 103.4mA, 01143 * 134.1mA, 164.1mA, 193.7mA, 222.7mA, 01144 * 251.2mA, 279.3mA, 300.0mA 01145 * 01146 * @return 0 on success, error code on failure. 01147 */ 01148 int get_imon_dischg_scale(float *currentmA); 01149 01150 /** 01151 * @brief Register Configuration 01152 * 01153 * @details 01154 * - Register : CNFG_CHG_I (0x28) 01155 * - Bit Fields : [3:0] 01156 * - Default : 0x0 01157 * - Description : Analog channel to connect to AMUX. 01158 */ 01159 typedef enum { 01160 MUX_SEL_MULTIPLEXER_DISABLED, 01161 MUX_SEL_CHGIN_VOLTAGE_MONITOR, 01162 MUX_SEL_CHGIN_CURRENT_MONITOR, 01163 MUX_SEL_BATTERY_VOLTAGE_MONITOR, 01164 MUX_SEL_BATTERY_CHARGE_CURRENT_MONITOR, 01165 MUX_SEL_BATTERY_DISCHARGE_CURRENT_MONITOR_NORMAL, 01166 MUX_SEL_BATTERY_DISCHARGE_CURRENT_MONITOR_NULL, 01167 MUX_SEL_RESERVED_0x07, 01168 MUX_SEL_RESERVED_0x08, 01169 MUX_SEL_AGND_VOLTAGE_MONITOR, 01170 MUX_SEL_SYS_VOLTAGE_MONITOR, 01171 MUX_SEL_SYS_VOLTAGE_MONITOR_0x0B, 01172 MUX_SEL_SYS_VOLTAGE_MONITOR_0x0C, 01173 MUX_SEL_SYS_VOLTAGE_MONITOR_0x0D, 01174 MUX_SEL_SYS_VOLTAGE_MONITOR_0x0E, 01175 MUX_SEL_SYS_VOLTAGE_MONITOR_0x0F 01176 }decode_mux_sel_t; 01177 01178 /** 01179 * @brief Set the analog channel to connect to AMUX. 01180 * 01181 * @param[in] selection AMUX value field to be written. 01182 * 01183 * @return 0 on success, error code on failure. 01184 */ 01185 int set_mux_sel(decode_mux_sel_t selection); 01186 01187 /** 01188 * @brief Get the analog channel to connect to AMUX. 01189 * 01190 * @param[out] selection AMUX value field to be read. 01191 * 01192 * @return 0 on success, error code on failure. 01193 */ 01194 int get_mux_sel(decode_mux_sel_t *selection); 01195 01196 /*SBB*/ 01197 01198 /** 01199 * @brief Register Configuration 01200 * 01201 * @details 01202 * - Register : CNFG_SBB_TOP (0x38) 01203 * - Bit Fields : [7:0] 01204 * - Default : 0x0 01205 * - Description : Watchdog Timer Configuration. 01206 */ 01207 typedef enum { 01208 CNFG_SBB_TOP_DRV_SBB, 01209 CNFG_SBB_TOP_DIS_LPM 01210 }reg_bit_cnfg_sbb_top_t; 01211 01212 /** 01213 * @brief Set CNFG_SBB_TOP (0x38) register. 01214 * 01215 * @param[in] bit_field Register bit field to be written. 01216 * @param[in] config Configuration value to be written. 01217 * 01218 * @return 0 on success, error code on failure. 01219 */ 01220 int set_cnfg_sbb_top(reg_bit_cnfg_sbb_top_t bit_field, uint8_t config); 01221 01222 /** 01223 * @brief Get CNFG_SBB_TOP (0x38) register. 01224 * 01225 * @param[in] bit_field Register bit field to be written. 01226 * @param[out] config Configuration value to be read. 01227 * 01228 * @return 0 on success, error code on failure. 01229 */ 01230 int get_cnfg_sbb_top(reg_bit_cnfg_sbb_top_t bit_field, uint8_t *config); 01231 01232 /** 01233 * @brief Set SIMO Buck-Boost Channel x Target Output Voltage. 01234 * CNFG_SBB0_A (0x39), CNFG_SBB1_A (0x3B) and CNFG_SBB2_A (0x3D) 01235 * 01236 * @param[in] channel Channel number: 0, 1 or 2. 01237 * @param[in] voltV SIMO buck-boost channel x target output voltage field to be written. 01238 * SBBx = 500mV + 25mV x TV_SBBx[7:0] 01239 * 0.500V, 0.525V, 0.550V, 0.575V, 0.600V, 0.625V, 01240 * 0.650V, 0.675V, 0.700V, ... 01241 * 5.425V, 5.450V, 5.475V, 5.500V. 01242 * 01243 * @return 0 on success, error code on failure. 01244 */ 01245 int set_tv_sbb_a(uint8_t channel, float voltV); 01246 01247 /** 01248 * @brief Get SIMO Buck-Boost Channel x Target Output Voltage. 01249 * CNFG_SBB0_A (0x39), CNFG_SBB1_A (0x3B) and CNFG_SBB2_A (0x3D) 01250 * 01251 * @param[in] channel Channel number: 0, 1 or 2. 01252 * @param[out] voltV SIMO buck-boost channel x target output voltage field to be read. 01253 * SBBx = 500mV + 25mV x TV_SBBx[7:0] 01254 * 0.500V, 0.525V, 0.550V, 0.575V, 0.600V, 0.625V, 01255 * 0.650V, 0.675V, 0.700V, ... 01256 * 5.425V, 5.450V, 5.475V, 5.500V. 01257 * 01258 * @return 0 on success, error code on failure. 01259 */ 01260 int get_tv_sbb_a(uint8_t channel, float *voltV); 01261 01262 /** 01263 * @brief Register Configuration 01264 * 01265 * @details 01266 * - Register : CNFG_SBB0_B (0x3A), CNFG_SBB1_B (0x3C) and CNFG_SBB2_B (0x3E) 01267 * - Bit Fields : [7:6] 01268 * - Default : 0x0 01269 * - Description : Operation mode of SBB0, 1 or 2. 01270 */ 01271 typedef enum { 01272 OP_MODE_AUTOMATIC, 01273 OP_MODE_BUCK_MODE, 01274 OP_MODE_BOOST_MODE, 01275 OP_MODE_BUCK_BOOST_MODE 01276 }decode_op_mode_t; 01277 01278 /** 01279 * @brief Set Operation mode of SBBx. 01280 * 01281 * @param[in] channel Channel number: 0, 1 or 2. 01282 * @param[in] mode Operation mode of SBBx bit to be written. 01283 * 01284 * @return 0 on success, error code on failure. 01285 */ 01286 int set_op_mode(uint8_t channel, decode_op_mode_t mode); 01287 01288 /** 01289 * @brief Get Operation mode of SBBx. 01290 * 01291 * @param[in] channel Channel number: 0, 1 or 2. 01292 * @param[out] mode Operation mode of SBBx bit to be read. 01293 * 01294 * @return 0 on success, error code on failure. 01295 */ 01296 int get_op_mode(uint8_t channel, decode_op_mode_t *mode); 01297 01298 /** 01299 * @brief Register Configuration 01300 * 01301 * @details 01302 * - Register : CNFG_SBB0_B (0x3A), CNFG_SBB1_B (0x3C) and CNFG_SBB2_B (0x3E) 01303 * - Bit Fields : [5:4] 01304 * - Default : 0x0 01305 * - Description : SIMO Buck-Boost Channel 0, 1 or 2 Peak Current Limit. 01306 */ 01307 typedef enum { 01308 IP_SBB_AMP_1_000A, 01309 IP_SBB_AMP_0_750A, 01310 IP_SBB_AMP_0_500A, 01311 IP_SBB_AMP_0_333A 01312 }decode_ip_sbb_t; 01313 01314 /** 01315 * @brief Set SIMO Buck-Boost Channel x Peak Current Limit. 01316 * 01317 * @param[in] channel Channel number: 0, 1 or 2. 01318 * @param[in] ip_sbb SIMO buck-boost channel 2 peak current limit field to be written. 01319 * 01320 * @return 0 on success, error code on failure. 01321 */ 01322 int set_ip_sbb(uint8_t channel, decode_ip_sbb_t ip_sbb); 01323 01324 /** 01325 * @brief Get SIMO Buck-Boost Channel x Peak Current Limit. 01326 * 01327 * @param[in] channel Channel number: 0, 1 or 2. 01328 * @param[out] ip_sbb SIMO buck-boost channel 2 peak current limit field to be read. 01329 * 01330 * @return 0 on success, error code on failure. 01331 */ 01332 int get_ip_sbb(uint8_t channel, decode_ip_sbb_t *ip_sbb); 01333 01334 /** 01335 * @brief Register Configuration 01336 * 01337 * @details 01338 * - Register : CNFG_SBB0_B (0x3A), CNFG_SBB1_B (0x3C) and CNFG_SBB2_B (0x3E) 01339 * - Bit Fields : [3] 01340 * - Default : 0x0 01341 * - Description : SIMO Buck-Boost Channel 0, 1 or 2 Active-Discharge Enable. 01342 */ 01343 typedef enum { 01344 ADE_SBB_DISABLED, 01345 ADE_SBB_ENABLED 01346 }decode_ade_sbb_t; 01347 01348 /** 01349 * @brief Set SIMO Buck-Boost Channel x Active-Discharge Enable. 01350 * 01351 * @param[in] channel Channel number: 0, 1 or 2. 01352 * @param[in] ade_sbb SIMO buck-boost channel 2 active-discharge enable bit to be written. 01353 * 01354 * @return 0 on success, error code on failure. 01355 */ 01356 int set_ade_sbb(uint8_t channel, decode_ade_sbb_t ade_sbb); 01357 01358 /** 01359 * @brief Get SIMO Buck-Boost Channel x Active-Discharge Enable. 01360 * 01361 * @param[in] channel Channel number: 0, 1 or 2. 01362 * @param[out] ade_sbb SIMO buck-boost channel 2 active-discharge enable bit to be read. 01363 * 01364 * @return 0 on success, error code on failure. 01365 */ 01366 int get_ade_sbb(uint8_t channel, decode_ade_sbb_t *ade_sbb); 01367 01368 /** 01369 * @brief Register Configuration 01370 * 01371 * @details 01372 * - Register : CNFG_SBB0_B (0x3A), CNFG_SBB1_B (0x3C) and CNFG_SBB2_B (0x3E) 01373 * - Bit Fields : [2:0] 01374 * - Default : 0x0 01375 * - Description : Enable Control for SIMO Buck-Boost Channel 0, 1 or 2. 01376 */ 01377 typedef enum { 01378 EN_SBB_FPS_SLOT_0, 01379 EN_SBB_FPS_SLOT_1, 01380 EN_SBB_FPS_SLOT_2, 01381 EN_SBB_FPS_SLOT_3, 01382 EN_SBB_OFF, 01383 EN_SBB_SAME_AS_0X04, 01384 EN_SBB_ON, 01385 EN_SBB_SAME_AS_0X06 01386 }decode_en_sbb_t; 01387 01388 /** 01389 * @brief Set Enable Control for SIMO Buck-Boost Channel x. 01390 * 01391 * @param[in] channel Channel number: 0, 1 or 2. 01392 * @param[in] en_sbb Enable control for SIMO buck-boost channel x field to be written. 01393 * 01394 * @return 0 on success, error code on failure. 01395 */ 01396 int set_en_sbb(uint8_t channel, decode_en_sbb_t en_sbb); 01397 01398 /** 01399 * @brief Get Enable Control for SIMO Buck-Boost Channel x. 01400 * 01401 * @param[in] channel Channel number: 0, 1 or 2. 01402 * @param[out] en_sbb Enable control for SIMO buck-boost channel x field to be read. 01403 * 01404 * @return 0 on success, error code on failure. 01405 */ 01406 int get_en_sbb(uint8_t channel, decode_en_sbb_t *en_sbb); 01407 01408 /** 01409 * @brief Set SIMO Buck-Boost Channel 0 Target Output Voltage. 01410 * Bit 7:0 of CNFG_DVS_SBB0_A (0x3F). 01411 * 01412 * @param[in] voltV SIMO buck-boost channel 0 target output voltage field to be written. 01413 * SBBx = 500mV + 25mV x TV_SBBx[7:0] 01414 * 0.500V, 0.525V, 0.550V, 0.575V, 0.600V, 0.625V, 01415 * 0.650V, 0.675V, 0.700V, ... 01416 * 5.425V, 5.450V, 5.475V, 5.500V. 01417 * 01418 * @return 0 on success, error code on failure. 01419 */ 01420 int set_tv_sbb_dvs(float voltV); 01421 01422 /** 01423 * @brief Get SIMO Buck-Boost Channel 0 Target Output Voltage. 01424 * Bit 7:0 of CNFG_DVS_SBB0_A (0x3F). 01425 * 01426 * @param[out] voltV SIMO buck-boost channel 0 target output voltage field to be read. 01427 * SBBx = 500mV + 25mV x TV_SBBx[7:0] 01428 * 0.500V, 0.525V, 0.550V, 0.575V, 0.600V, 0.625V, 01429 * 0.650V, 0.675V, 0.700V, ... 01430 * 5.425V, 5.450V, 5.475V, 5.500V. 01431 * 01432 * @return 0 on success, error code on failure. 01433 */ 01434 int get_tv_sbb_dvs(float *voltV); 01435 01436 /*LDO*/ 01437 01438 /** 01439 * @brief Set LDO Output Channel x Target Output Voltage. Bit 6:0. 01440 * CNFG_LDO0_A (0x48) and CNFG_LDO1_A (0x4A) 01441 * 01442 * @param[in] channel Channel number: 0 or 1. 01443 * @param[in] voltV LDO Output Channel x target output voltage field to be read. 01444 * LDOx = 500mV + 25mV x TV_LDOx[6:0] 01445 * 0.500V, 0.525V, 0.550V, 0.575V, 0.600V, 0.625V, 01446 * 0.650V, 0.675V, 0.700V, ... 01447 * 3.650, 3.675. 01448 * 01449 * When TV_LDO[7] = 0, TV_LDO[6:0] sets the 01450 * LDO's output voltage range from 0.5V to 3.675V. 01451 * When TV_LDO[7] = 1, TV_LDO[6:0] sets the 01452 * LDO's output voltage from 1.825V to 5V. 01453 * 01454 * @return 0 on success, error code on failure. 01455 */ 01456 int set_tv_ldo_volt_a(uint8_t channel, float voltV); 01457 01458 /** 01459 * @brief Get LDO Output Channel x Target Output Voltage. Bit 6:0. 01460 * CNFG_LDO0_A (0x48) and CNFG_LDO1_A (0x4A) 01461 * 01462 * @param[in] channel Channel number: 0 or 1. 01463 * @param[out] voltV LDO Output Channel x target output voltage field to be read. 01464 * LDOx = 500mV + 25mV x TV_LDOx[6:0] 01465 * 0.500V, 0.525V, 0.550V, 0.575V, 0.600V, 0.625V, 01466 * 0.650V, 0.675V, 0.700V, ... 01467 * 3.650, 3.675. 01468 * 01469 * When TV_LDO[7] = 0, TV_LDO[6:0] sets the 01470 * LDO's output voltage range from 0.5V to 3.675V. 01471 * When TV_LDO[7] = 1, TV_LDO[6:0] sets the 01472 * LDO's output voltage from 1.825V to 5V. 01473 * 01474 * @return 0 on success, error code on failure. 01475 */ 01476 int get_tv_ldo_volt_a(uint8_t channel, float *voltV); 01477 01478 /** 01479 * @brief Register Configuration 01480 * 01481 * @details 01482 * - Register : CNFG_LDO0_A (0x48) and CNFG_LDO1_A (0x4A) 01483 * - Bit Fields : [7] 01484 * - Default : 0x0 01485 * - Description : SIMO Buck-Boost Channel 0, 1 or 2 Peak Current Limit. 01486 */ 01487 typedef enum { 01488 TV_LDO0_A_NO_OFFSET, 01489 TV_LDO0_A_NO_1_325V 01490 }decode_tv_ldo_offset_a_t; 01491 01492 /** 01493 * @brief Set LDO Output Channel x Target Output Voltage. Bit 7. 01494 * CNFG_LDO0_A (0x48) and CNFG_LDO1_A (0x4A) 01495 * 01496 * @param[in] channel Channel number: 0 or 1. 01497 * @param[in] offset LDO Output Channel x target output voltage offset field to be read. 01498 * 0b0 = No Offset 01499 * 0b1 = 1.325V Offset 01500 * 01501 * 01502 * @return 0 on success, error code on failure. 01503 */ 01504 int set_tv_ldo_offset_a(uint8_t channel, decode_tv_ldo_offset_a_t offset); 01505 01506 /** 01507 * @brief Get LDO Output Channel x Target Output Voltage. Bit 7. 01508 * CNFG_LDO0_A (0x48) and CNFG_LDO1_A (0x4A) 01509 * 01510 * @param[in] channel Channel number: 0 or 1. 01511 * @param[out] offset LDO Output Channel x target output voltage offset field to be read. 01512 * 0b0 = No Offset 01513 * 0b1 = 1.325V Offset 01514 * 01515 * @return 0 on success, error code on failure. 01516 */ 01517 int get_tv_ldo_offset_a(uint8_t channel, decode_tv_ldo_offset_a_t *offset); 01518 01519 /** 01520 * @brief Register Configuration 01521 * 01522 * @details 01523 * - Register : CNFG_LDO0_B (0x49) and CNFG_LDO1_B (0x4B) 01524 * - Bit Fields : [2:0] 01525 * - Default : 0x0 01526 * - Description : Enable Control for LDO 0 or 1. 01527 */ 01528 typedef enum { 01529 EN_LDO_FPS_SLOT_0, 01530 EN_LDO_FPS_SLOT_1, 01531 EN_LDO_FPS_SLOT_2, 01532 EN_LDO_FPS_SLOT_3, 01533 EN_LDO_OFF, 01534 EN_LDO_SAME_AS_0X04, 01535 EN_LDO_ON, 01536 EN_LDO_SAME_AS_0X06 01537 }decode_en_ldo_t; 01538 01539 /** 01540 * @brief Set Enable Control for LDO Channel x. 01541 * 01542 * @param[in] channel Channel number: 0, 1 or 2. 01543 * @param[in] en_ldo Enable control for LDO channel x field to be written. 01544 * 01545 * @return 0 on success, error code on failure. 01546 */ 01547 int set_en_ldo(uint8_t channel, decode_en_ldo_t en_ldo); 01548 01549 /** 01550 * @brief Get Enable Control for LDO Channel x. 01551 * 01552 * @param[in] channel Channel number: 0, 1 or 2. 01553 * @param[out] en_ldo Enable control for LDO channel x field to be read. 01554 * 01555 * @return 0 on success, error code on failure. 01556 */ 01557 int get_en_ldo(uint8_t channel, decode_en_ldo_t *en_ldo); 01558 01559 /** 01560 * @brief Register Configuration 01561 * 01562 * @details 01563 * - Register : CNFG_LDO0_B (0x49) and CNFG_LDO1_B (0x4B) 01564 * - Bit Fields : [3] 01565 * - Default : 0x0 01566 * - Description : LDO Channel 0 or 1 Active-Discharge Enable. 01567 */ 01568 typedef enum { 01569 ADE_LDO_DISABLED, 01570 ADE_LDO_ENABLED 01571 }decode_ade_ldo_t; 01572 01573 /** 01574 * @brief Set LDO Channel x Active-Discharge Enable. 01575 * 01576 * @param[in] channel Channel number: 0 or 1. 01577 * @param[in] ade_ldo LDO channel x active-discharge enable bit to be written. 01578 * 01579 * @return 0 on success, error code on failure. 01580 */ 01581 int set_ade_ldo(uint8_t channel, decode_ade_ldo_t ade_ldo); 01582 01583 /** 01584 * @brief Get LDO Channel x Active-Discharge Enable. 01585 * 01586 * @param[in] channel Channel number: 0 or 1. 01587 * @param[out] ade_ldo LDO channel x active-discharge enable bit to be read. 01588 * 01589 * @return 0 on success, error code on failure. 01590 */ 01591 int get_ade_ldo(uint8_t channel, decode_ade_ldo_t *ade_ldo); 01592 01593 /** 01594 * @brief Register Configuration 01595 * 01596 * @details 01597 * - Register : CNFG_LDO0_B (0x49) and CNFG_LDO1_B (0x4B) 01598 * - Bit Fields : [4] 01599 * - Default : 0x0 01600 * - Description : Operation mode of LDO 0 or 1. 01601 */ 01602 typedef enum { 01603 LDO_MD_LDO_MODE, 01604 LDO_MD_LSW_MODE 01605 }decode_ldo_md_t; 01606 01607 /** 01608 * @brief Set Operation mode of LDOx. 01609 * 01610 * @param[in] channel Channel number: 0 or 1. 01611 * @param[in] mode Operation mode of LDOx bit to be written. 01612 * 01613 * @return 0 on success, error code on failure. 01614 */ 01615 int set_ldo_md(uint8_t channel, decode_ldo_md_t mode); 01616 01617 /** 01618 * @brief Get Operation mode of LDOx. 01619 * 01620 * @param[in] channel Channel number: 0 or 1. 01621 * @param[out] mode Operation mode of LDOx bit to be read. 01622 * 01623 * @return 0 on success, error code on failure. 01624 */ 01625 int get_ldo_md(uint8_t channel, decode_ldo_md_t *mode); 01626 01627 /*FuelGauge*/ 01628 /*Status and Configuration Registers*/ 01629 01630 /** 01631 * @brief Register Configuration 01632 * 01633 * @details 01634 * - Register : Fuel-gauge Status (0x00) 01635 * - Bit Fields : [15:0] 01636 * - Default : 0x0000 01637 * - Description : All Status register bit fields. 01638 */ 01639 typedef enum { 01640 Status_Imn, 01641 Status_POR, 01642 Status_SPR_2, 01643 Status_Bst, 01644 Status_Isysmx, 01645 Status_SPR_5, 01646 Status_ThmHot, 01647 Status_dSOCi, 01648 Status_Vmn, 01649 Status_Tmn, 01650 Status_Smn, 01651 Status_Bi, 01652 Status_Vmx, 01653 Status_Tmx, 01654 Status_Smx, 01655 Status_Br 01656 }reg_bit_status_t; 01657 01658 /** 01659 * @brief Set bit field of Fuel-gauge Status[15:0] (0x00) register. 01660 * 01661 * @param[in] bit_field Status bit field to be written to register. 01662 * @param[in] status to be written to register. 01663 * 01664 * @return 0 on success, error code on failure. 01665 */ 01666 int set_fg_status(reg_bit_status_t bit_field, uint8_t status); 01667 01668 /** 01669 * @brief Get bit field of Fuel-gauge Status[15:0] (0x00) register. 01670 * 01671 * @param[in] bit_field Status bit field to be written to register. 01672 * @param[out] status Pointer to save result value. 01673 * 01674 * @return 0 on success, error code on failure. 01675 */ 01676 int get_fg_status(reg_bit_status_t bit_field, uint8_t *status); 01677 01678 /** 01679 * @brief Register Configuration 01680 * 01681 * @details 01682 * - Register : Fuel-gauge VAlrtTh (0x01) 01683 * - Bit Fields : [15:0] 01684 * - Default : 0xFF00 (Disabled) 01685 * - Description : The VAlrtTh register sets upper and lower limits that generate an alert if exceeded by the VCell register value. 01686 */ 01687 typedef enum { 01688 VAlrtTh_MinVoltageAlrt, 01689 VAlrtTh_MaxVoltageAlrt 01690 }reg_bit_valrt_th_t; 01691 01692 /** 01693 * @brief Set bit field of fuel-gauge VAlrtTh (0x01) register. 01694 * 01695 * @param[in] bit_field VAlrtTh register bit field to be written. 01696 * @param[in] voltV to be written to register. 01697 * Interrupt threshold limits are selectable with 20mV resolution 01698 * over the full operating range of the VCell register. 01699 * 01700 * @return 0 on success, error code on failure. 01701 */ 01702 int set_fg_valrt_th(reg_bit_valrt_th_t bit_field, float voltV); 01703 01704 /** 01705 * @brief Get bit field of fuel-gauge VAlrtTh (0x01) register. 01706 * 01707 * @param[in] bit_field VAlrtTh register bit field to be written. 01708 * @param[out] voltV Pointer to save result value. 01709 * Interrupt threshold limits are selectable with 20mV resolution 01710 * over the full operating range of the VCell register. 01711 * 01712 * @return 0 on success, error code on failure. 01713 */ 01714 int get_fg_valrt_th(reg_bit_valrt_th_t bit_field, float *voltV); 01715 01716 /** 01717 * @brief Register Configuration 01718 * 01719 * @details 01720 * - Register : Fuel-gauge TAlrtTh (0x02) 01721 * - Bit Fields : [15:0] 01722 * - Default : 0x7F80 (Disabled) 01723 * - Description : All TAlrtTh register bit fields. 01724 */ 01725 typedef enum { 01726 TAlrtTh_MinTempAlrt, 01727 TAlrtTh_MaxTempAlrt 01728 }reg_bit_talrt_th_t; 01729 01730 /** 01731 * @brief Set bit field of fuel-gauge TAlrtTh (0x02) register. 01732 * 01733 * @param[in] bit_field TAlrtTh register bit field to be written. 01734 * @param[in] tempDegC to be written to register. 01735 * Interrupt threshold limits are stored in two’s-complement format 01736 * with 1°C resolution over the full operating range of the Temp register. 01737 * 01738 * @return 0 on success, error code on failure. 01739 */ 01740 int set_fg_talrt_th(reg_bit_talrt_th_t bit_field, int tempDegC); 01741 01742 /** 01743 * @brief Get bit field of fuel-gauge TAlrtTh (0x02) register. 01744 * 01745 * @param[in] bit_field TAlrtTh register bit field to be written. 01746 * @param[out] tempDegC Pointer to save result value. 01747 * Interrupt threshold limits are stored in two’s-complement format 01748 * with 1°C resolution over the full operating range of the Temp register. 01749 * 01750 * @return 0 on success, error code on failure. 01751 */ 01752 int get_fg_talrt_th(reg_bit_talrt_th_t bit_field, int *tempDegC); 01753 01754 /** 01755 * @brief Register Configuration 01756 * 01757 * @details 01758 * - Register : Fuel-gauge SAlrtTh (0x03) 01759 * - Bit Fields : [15:0] 01760 * - Default : 0xFF00 01761 * - Description : The SAlrtTh register sets upper and lower limits that generate an alert if exceeded by RepSOC. 01762 */ 01763 typedef enum { 01764 SAlrtTh_MinSocAlrt, 01765 SAlrtTh_MaxSocAlrt 01766 }reg_bit_salrt_th_t; 01767 01768 /** 01769 * @brief Set bit field of fuel-gauge SAlrtTh (0x03) register. 01770 * 01771 * @param[in] bit_field SAlrtTh register bit field to be written. 01772 * @param[in] soc to be written to register. 01773 * Interrupt threshold limits are configurable with 1% resolution 01774 * over the full operating range of the RepSOC register. 01775 * 01776 * @return 0 on success, error code on failure. 01777 */ 01778 int set_fg_salrt_th(reg_bit_salrt_th_t bit_field, uint8_t soc); 01779 01780 /** 01781 * @brief Get bit field of fuel-gauge SAlrtTh (0x03) register. 01782 * 01783 * @param[in] bit_field SAlrtTh register bit field to be written. 01784 * @param[out] soc Pointer to save result value. 01785 * Interrupt threshold limits are configurable with 1% resolution 01786 * over the full operating range of the RepSOC register. 01787 * 01788 * @return 0 on success, error code on failure. 01789 */ 01790 int get_fg_salrt_th(reg_bit_salrt_th_t bit_field, uint8_t *soc); 01791 01792 /** 01793 * @brief Set bit field of fuel-gauge FullSocThr (0x13) register. 01794 * 01795 * @param[in] soc_thr to be written to register. 01796 * FullSOCThr comes from OTP if the OTP register is enabled. 01797 * Otherwise it POR’s to a default of 95%. 01798 * LSB unit is 1/256%. 01799 * 01800 * @return 0 on success, error code on failure. 01801 */ 01802 int set_fg_full_soc_thr(float soc_thr); 01803 01804 /** 01805 * @brief Get bit field of fuel-gauge FullSocThr (0x13) register. 01806 * 01807 * @param[out] soc_thr Pointer to save result value. 01808 * FullSOCThr comes from OTP if the OTP register is enabled. 01809 * Otherwise it POR’s to a default of 95%. 01810 * LSB unit is 1/256%. 01811 * 01812 * @return 0 on success, error code on failure. 01813 */ 01814 int get_fg_full_soc_thr(float *soc_thr); 01815 01816 /** 01817 * @brief Set bit field of fuel-gauge DesignCap (0x18) register. 01818 * 01819 * @param[in] capacitymAh to be written to register. 01820 * LSB unit is 0.1mAh. Min is 0.0mAh and Max is 6553.5mAh. 01821 * 01822 * @return 0 on success, error code on failure. 01823 */ 01824 int set_fg_design_cap(float capacitymAh); 01825 01826 /** 01827 * @brief Get bit field of fuel-gauge DesignCap (0x18) register. 01828 * 01829 * @param[out] capacitymAh Pointer to save result value. 01830 * LSB unit is 0.1mAh. Min is 0.0mAh and Max is 6553.5mAh. 01831 * 01832 * @return 0 on success, error code on failure. 01833 */ 01834 int get_fg_design_cap(float *capacitymAh); 01835 01836 /** 01837 * @brief Register Configuration 01838 * 01839 * @details 01840 * - Register : Fuel-gauge Config (0x1D) 01841 * - Bit Fields : [15:0] 01842 * - Default : 0x0000 01843 * - Description : The Config registers hold all shutdown enable, alert enable, 01844 * and temperature enable control bits. 01845 */ 01846 typedef enum { 01847 Config_Ber, 01848 Config_Bei, 01849 Config_Aen, 01850 Config_FTHRM, 01851 Config_ETHRM, 01852 Config_SPR_5, 01853 Config_I2CSH, 01854 Config_SHDN, 01855 Config_Tex, 01856 Config_Ten, 01857 Config_AINSH, 01858 Config_SPR_11, 01859 Config_Vs, 01860 Config_Ts, 01861 Config_Ss, 01862 Config_SPR_15 01863 }reg_bit_config_t; 01864 01865 /** 01866 * @brief Set bit field of fuel-gauge Config (0x1D) register. 01867 * 01868 * @param[in] bit_field Config bit field to be written to register. 01869 * @param[in] config to be written to register. 01870 * 01871 * @return 0 on success, error code on failure. 01872 */ 01873 int set_fg_config(reg_bit_config_t bit_field, uint8_t config); 01874 01875 /** 01876 * @brief Get bit field of fuel-gauge Config (0x1D) register. 01877 * 01878 * @param[in] bit_field Config bit field to be written to register. 01879 * @param[out] config Pointer to save result value. 01880 * 01881 * @return 0 on success, error code on failure. 01882 */ 01883 int get_fg_config(reg_bit_config_t bit_field, uint8_t *config); 01884 01885 /** 01886 * @brief Set bit field of fuel-gauge IChgTerm (0x1E) register. 01887 * 01888 * @param[in] currentA to be written to register. 01889 * The current register has a LSB value of 156.25μA, 01890 * a register scale range of ± 5.12 A. 01891 * 01892 * @return 0 on success, error code on failure. 01893 */ 01894 int set_fg_ichg_term(float currentA); 01895 01896 /** 01897 * @brief Get bit field of fuel-gauge IChgTerm (0x1E) register. 01898 * 01899 * @param[out] currentA Pointer to save result value. 01900 * The current register has a LSB value of 156.25μA, 01901 * a register scale range of ± 5.12 A. 01902 * 01903 * @return 0 on success, error code on failure. 01904 */ 01905 int get_fg_ichg_term(float *currentA); 01906 01907 /** 01908 * @brief Get bit field of fuel-gauge DevName (0x21) register. 01909 * 01910 * @param[out] value Pointer to save result value. 01911 * 01912 * @return 0 on success, error code on failure. 01913 */ 01914 int get_fg_dev_name(uint16_t *value); 01915 01916 /** 01917 * @brief Set the filtering for empty learning for both the I_Avgempty and QRTable registers. 01918 * Bit 15:14 of FilterCfg (0x29) register. 01919 * 01920 * @param[in] nempty Iavg_empty is learned with(NEMPTY+3) right shifts. QRTable is learned with 01921 * (NEMPTY + sizeof(Iavgempty) – sizeof (AvgCurrent)) right shifts. 01922 * @return 0 on success, error code on failure. 01923 */ 01924 int set_fg_nempty(uint8_t nempty); 01925 01926 /** 01927 * @brief Get the filtering for empty learning for both the I_Avgempty and QRTable registers. 01928 * Bit 15:14 of FilterCfg (0x29) register. 01929 * 01930 * @param[out] nempty Iavg_empty is learned with(NEMPTY+3) right shifts. QRTable is learned with 01931 * (NEMPTY + sizeof(Iavgempty) – sizeof (AvgCurrent)) right shifts. 01932 * 01933 * @return 0 on success, error code on failure. 01934 */ 01935 int get_fg_nempty(uint8_t *nempty); 01936 01937 /** 01938 * @brief Set the time constant for the mixing algorithm. 01939 * Bit 10:7 of FilterCfg (0x29) register. 01940 * 01941 * @param[in] second The equation setting the period is: 01942 * Mixing Period = 175.8ms × 2^(5+NMIX) to be written. 01943 * 01944 * @return 0 on success, error code on failure. 01945 */ 01946 int set_fg_nmix(float second); 01947 01948 /** 01949 * @brief Get the time constant for the mixing algorithm. 01950 * Bit 10:7 of FilterCfg (0x29) register. 01951 * 01952 * @param[out] second The equation setting the period is: 01953 * Mixing Period = 175.8ms × 2^(5+NMIX) to be read. 01954 * 01955 * @return 0 on success, error code on failure. 01956 */ 01957 int get_fg_nmix(float *second); 01958 01959 /** 01960 * @brief Set the time constant for the AverageVCELL register. 01961 * Bit 6:4 of FilterCfg (0x29) register. 01962 * 01963 * @param[in] second The equation setting the period is: 01964 * AverageVCELL time constant = 175.8ms × 2^(6+NAVGVCELL) to be written. 01965 * 01966 * @return 0 on success, error code on failure. 01967 */ 01968 int set_fg_navgcell(float second); 01969 01970 /** 01971 * @brief Get the time constant for the AverageVCELL register. 01972 * Bit 6:4 of FilterCfg (0x29) register. 01973 * 01974 * @param[out] second The equation setting the period is: 01975 * AverageVCELL time constant = 175.8ms × 2^(6+NAVGVCELL) to be read. 01976 * 01977 * @return 0 on success, error code on failure. 01978 */ 01979 int get_fg_navgcell(float *second); 01980 01981 /** 01982 * @brief Set the time constant for the AverageCurrent register. 01983 * Bit 3:0 of FilterCfg (0x29) register. 01984 * 01985 * @param[in] second The equation setting the period is: 01986 * AverageCurrent time constant = 175.8ms × 2^(2+NCURR) to be written. 01987 * 01988 * @return 0 on success, error code on failure. 01989 */ 01990 int set_fg_ncurr(float second); 01991 01992 /** 01993 * @brief Get the time constant for the AverageCurrent register. 01994 * Bit 3:0 of FilterCfg (0x29) register. 01995 * 01996 * @param[out] second The equation setting the period is: 01997 * AverageCurrent time constant = 175.8ms × 2^(2+NCURR) to be read. 01998 * 01999 * @return 0 on success, error code on failure. 02000 */ 02001 int get_fg_ncurr(float *second); 02002 02003 /** 02004 * @brief Set bit field of fuel-gauge IAvgEmpty (0x36) register. 02005 * 02006 * @param[in] currentA to be written to register. 02007 * The current register has a LSB value of 156.25μA, 02008 * a register scale range of ± 5.12 A. 02009 * 02010 * @return 0 on success, error code on failure. 02011 */ 02012 int set_fg_iavg_empty(float currentA); 02013 02014 /** 02015 * @brief Get bit field of fuel-gauge IAvgEmpty (0x36) register. 02016 * 02017 * @param[out] currentA Pointer to save result value. 02018 * The current register has a LSB value of 156.25μA, 02019 * a register scale range of ± 5.12 A. 02020 * 02021 * @return 0 on success, error code on failure. 02022 */ 02023 int get_fg_iavg_empty(float *currentA); 02024 02025 /** 02026 * @brief Set the voltage level for detecting empty. 02027 * Bit 15:7 of VEmpty(0x3A) register. 02028 * 02029 * @param[in] voltV Empty voltage to be written. 02030 * A 10mV resolution gives a 0 to 5.11V range. 02031 * 02032 * @return 0 on success, error code on failure. 02033 */ 02034 int set_fg_v_empty(float voltV); 02035 02036 /** 02037 * @brief Get the voltage level for detecting empty. 02038 * Bit 15:7 of VEmpty(0x3A) register. 02039 * 02040 * @param[out] voltV Empty voltage to be read. 02041 * A 10mV resolution gives a 0 to 5.11V range. 02042 * 02043 * @return 0 on success, error code on failure. 02044 */ 02045 int get_fg_v_empty(float *voltV); 02046 02047 /** 02048 * @brief Set the voltage level for clearing empty detection. 02049 * Bit 6:0 of VEmpty(0x3A) register. 02050 * 02051 * @param[in] voltV Recovery voltage to be written. 02052 * A 40mV resolution gives a 0 to 5.08V range. 02053 * 02054 * @return 0 on success, error code on failure. 02055 */ 02056 int set_fg_v_recover(float voltV); 02057 02058 /** 02059 * @brief Get the voltage level for clearing empty detection. 02060 * Bit 6:0 of VEmpty(0x3A) register. 02061 * 02062 * @param[out] voltV Recovery voltage to be read. 02063 * A 40mV resolution gives a 0 to 5.08V range. 02064 * 02065 * @return 0 on success, error code on failure. 02066 */ 02067 int get_fg_v_recover(float *voltV); 02068 02069 /** 02070 * @brief Register Configuration 02071 * 02072 * @details 02073 * - Register : Fuel-gauge Config2 (0xBB) 02074 * - Bit Fields : [15:0] 02075 * - Default : 0x0000 02076 * - Description : The Config registers hold all shutdown enable, alert enable, 02077 * and temperature enable control bits. 02078 */ 02079 typedef enum { 02080 Config2_ISysNCurr, 02081 Config2_OCVQen, 02082 Config2_LdMdl, 02083 Config2_TAlrtEn, 02084 Config2_dSOCen, 02085 Config2_ThmHotAlrtEn, 02086 Config2_ThmHotEn, 02087 Config2_FCThmHot, 02088 Config2_SPR 02089 }reg_bit_config2_t; 02090 02091 /** 02092 * @brief Set Config2 (0xBB) register holding all shutdown enable, alert enable, 02093 * and temperature enable control bits. 02094 * 02095 * @param[in] bit_field Config bit field to be written to register. 02096 * @param[in] config2 value to be written. 02097 * 02098 * @return 0 on success, error code on failure. 02099 */ 02100 int set_fg_config2(reg_bit_config2_t bit_field, uint8_t config2); 02101 02102 /** 02103 * @brief Get Config2 (0xBB) register holding all shutdown enable, alert enable, 02104 * and temperature enable control bits. 02105 * 02106 * @param[in] bit_field Config bit field to be written to register. 02107 * @param[out] config2 value to be read. 02108 * 02109 * @return 0 on success, error code on failure. 02110 */ 02111 int get_fg_config2(reg_bit_config2_t bit_field, uint8_t *config2); 02112 02113 /** 02114 * @brief Set the time constant for the AvgISys register. 02115 * Bit 3:0 of Config2(0xBB) register. 02116 * 02117 * @param[in] second Time constant to be written. 02118 * The default POR value of 0100b gives a time constant of 5.625. 02119 * The equation setting the period is: AvgISys time constant = 45s x 2^(ISysNCurr-7). 02120 * 02121 * @return 0 on success, error code on failure. 02122 */ 02123 int set_fg_isys_ncurr(float second); 02124 02125 /** 02126 * @brief Get the time constant for the AvgISys register. 02127 * Bit 3:0 of Config2(0xBB) register. 02128 * 02129 * @param[out] second Time constant to be read. 02130 * The default POR value of 0100b gives a time constant of 5.625. 02131 * The equation setting the period is: AvgISys time constant = 45s x 2^(ISysNCurr-7). 02132 * 02133 * @return 0 on success, error code on failure. 02134 */ 02135 int get_fg_isys_ncurr(float *second); 02136 02137 /*Measurement Registers*/ 02138 02139 /** 02140 * @brief Set bit field of fuel-gauge Temp (0x08) register. 02141 * 02142 * @param[in] tempDegC to be written to register. 02143 * The LSB is 0.0039˚C. 02144 * 02145 * @return 0 on success, error code on failure. 02146 */ 02147 int set_fg_temp(float tempDegC); 02148 02149 /** 02150 * @brief Get bit field of fuel-gauge Temp (0x08) register. 02151 * 02152 * @param[out] tempDegC Pointer to save result value. 02153 * The LSB is 0.0039˚C. 02154 * 02155 * @return 0 on success, error code on failure. 02156 */ 02157 int get_fg_temp(float *tempDegC); 02158 02159 /** 02160 * @brief Set bit field of fuel-gauge Vcell (0x09) register. 02161 * VCell reports the voltage measured between BATT and GND. 02162 * 02163 * @param[in] voltV to be written to register. 02164 * VCell register reports the 2.5X the voltage measured at the CELLX pin. 02165 * 02166 * @return 0 on success, error code on failure. 02167 */ 02168 int set_fg_vcell(float voltV); 02169 02170 /** 02171 * @brief Get bit field of fuel-gauge Vcell (0x09) register. 02172 * VCell reports the voltage measured between BATT and GND. 02173 * 02174 * @param[out] voltV Pointer to save result value. 02175 * VCell register reports the 2.5X the voltage measured at the CELLX pin. 02176 * 02177 * @return 0 on success, error code on failure. 02178 */ 02179 int get_fg_vcell(float *voltV); 02180 02181 /** 02182 * @brief Set bit field of fuel-gauge Current (0x0A) register. 02183 * 02184 * @param[in] currentA to be written to register. 02185 * The current register has a LSB value of 31.25uA, a register scale of 1.024A 02186 * 02187 * @return 0 on success, error code on failure. 02188 */ 02189 int set_fg_current(float currentA); 02190 02191 /** 02192 * @brief Get bit field of fuel-gauge Current (0x0A) register. 02193 * 02194 * @param[out] currentA Pointer to save result value. 02195 * The current register has a LSB value of 31.25uA, a register scale of 1.024A. 02196 * 02197 * @return 0 on success, error code on failure. 02198 */ 02199 int get_fg_current(float *currentA); 02200 02201 /** 02202 * @brief Set bit field of fuel-gauge AvgCurrent (0x0B) register. 02203 * 02204 * @param[in] currentA to be written to register. 02205 * The current register has a LSB value of 31.25uA, a register scale of 1.024A 02206 * 02207 * @return 0 on success, error code on failure. 02208 */ 02209 int set_fg_avg_current(float currentA); 02210 02211 /** 02212 * @brief Get bit field of fuel-gauge AvgCurrent (0x0B) register. 02213 * 02214 * @param[out] currentA Pointer to save result value. 02215 * The current register has a LSB value of 31.25uA, a register scale of 1.024A 02216 * 02217 * @return 0 on success, error code on failure. 02218 */ 02219 int get_fg_avg_current(float *currentA); 02220 02221 /** 02222 * @brief Set bit field of fuel-gauge AvgTA (0x16) register. 02223 * 02224 * @param[in] tempDegC to be written to register. 02225 * LSB is 1/256°C. Min value is -128.0°C and Max value is 127.996°C. 02226 * 02227 * @return 0 on success, error code on failure. 02228 */ 02229 int set_fg_avgta(float tempDegC); 02230 02231 /** 02232 * @brief Get bit field of fuel-gauge AvgTA (0x16) register. 02233 * 02234 * @param[out] tempDegC Pointer to save result value. 02235 * LSB is 1/256°C. Min value is -128.0°C and Max value is 127.996°C. 02236 * 02237 * @return 0 on success, error code on failure. 02238 */ 02239 int get_fg_avgta(float *tempDegC); 02240 02241 /** 02242 * @brief Set bit field of fuel-gauge AvgVCell (0x19) register. 02243 * 02244 * @param[in] voltV to be written to register. 02245 * LSB is 1.25mV/16. Min value is 0.0V and Max value is 5.11992V. 02246 * 02247 * @return 0 on success, error code on failure. 02248 */ 02249 int set_fg_avgvcell(float voltV); 02250 02251 /** 02252 * @brief Get bit field of fuel-gauge AvgVCell (0x19) register. 02253 * 02254 * @param[out] voltV Pointer to save result value. 02255 * LSB is 1.25mV/16. Min value is 0.0V and Max value is 5.11992V. 02256 * 02257 * @return 0 on success, error code on failure. 02258 */ 02259 int get_fg_avgvcell(float *voltV); 02260 02261 /** 02262 * @brief Register Configuration 02263 * 02264 * @details 02265 * - Register : Fuel-gauge MaxMinTemp (0x1A) 02266 * - Bit Fields : [15:0] 02267 * - Default : 0x7F80 02268 * - Description : All MaxMinTemp register bit fields. 02269 */ 02270 typedef enum { 02271 MaxMinTemp_MinTemperature, 02272 MaxMinTemp_MaxTemperature 02273 }reg_bit_max_min_temp_t; 02274 02275 /** 02276 * @brief Set bit field of fuel-gauge MaxMinTemp (0x1A) register. 02277 * 02278 * @param[in] bit_field to be written to register. 02279 * @param[in] tempDegC to be written to register. 02280 * The maximum and minimum temperatures are each stored as two’s complement 02281 * 8-bit values with 1°C resolution. 02282 * 02283 * @return 0 on success, error code on failure. 02284 */ 02285 int set_fg_max_min_temp(reg_bit_max_min_temp_t bit_field, int tempDegC); 02286 02287 /** 02288 * @brief Get bit field of fuel-gauge MaxMinTemp (0x1A) register. 02289 * 02290 * @param[in] bit_field to be written to register. 02291 * @param[out] tempDegC Pointer to save result value. 02292 * The maximum and minimum temperatures are each stored as two’s complement 02293 * 8-bit values with 1°C resolution. 02294 * 02295 * @return 0 on success, error code on failure. 02296 */ 02297 int get_fg_max_min_temp(reg_bit_max_min_temp_t bit_field, int *tempDegC); 02298 02299 /** 02300 * @brief Register Configuration 02301 * 02302 * @details 02303 * - Register : Fuel-gauge MaxMinVolt (0x1B) 02304 * - Bit Fields : [15:0] 02305 * - Default : 0x00FF 02306 * - Description : All MaxMinVolt register bit fields. 02307 */ 02308 typedef enum { 02309 MaxMinVolt_MinVoltage, 02310 MaxMinVolt_MaxVoltage 02311 }reg_bit_max_min_volt_t; 02312 02313 /** 02314 * @brief Set bit field of fuel-gauge MaxMinVolt (0x1B) register. 02315 * 02316 * @param[in] bit_field MaxMinVolt register bit field to be written. 02317 * @param[in] voltV to be written to register. 02318 * The maximum and minimum voltages are each stored as 8-bit 02319 * values with a 20mV resolution. 02320 * 02321 * @return 0 on success, error code on failure. 02322 */ 02323 int set_fg_max_min_volt(reg_bit_max_min_volt_t bit_field, float voltV); 02324 02325 /** 02326 * @brief Get bit field of fuel-gauge MaxMinVolt (0x1B) register. 02327 * 02328 * @param[in] bit_field MaxMinVolt register bit field to be written. 02329 * @param[out] voltV Pointer to save result value. 02330 * The maximum and minimum voltages are each stored as 8-bit 02331 * values with a 20mV resolution. 02332 * 02333 * @return 0 on success, error code on failure. 02334 */ 02335 int get_fg_max_min_volt(reg_bit_max_min_volt_t bit_field, float *voltV); 02336 02337 /** 02338 * @brief Register Configuration 02339 * 02340 * @details 02341 * - Register : Fuel-gauge MaxMinCurr (0x1C) 02342 * - Bit Fields : [15:0] 02343 * - Default : 0x807F 02344 * - Description : All MaxMinCurr register bit fields. 02345 */ 02346 typedef enum { 02347 MaxMinCurr_MaxDisCurrent, 02348 MaxMinCurr_MaxChargeCurrent 02349 }reg_bit_max_min_curr_t; 02350 02351 /** 02352 * @brief Set bit field of fuel-gauge MaxMinCurr (0x1C) register. 02353 * 02354 * @param[in] bit_field MaxMinCurr register bit field to be written. 02355 * @param[in] currentA to be written to register. 02356 * The maximum and minimum currents are each stored 02357 * as two’s complement 8-bit values with 8mA resolution. 02358 * 02359 * @return 0 on success, error code on failure. 02360 */ 02361 int set_fg_max_min_curr(reg_bit_max_min_curr_t bit_field, float currentA); 02362 02363 /** 02364 * @brief Get bit field of fuel-gauge MaxMinCurr (0x1C) register. 02365 * 02366 * @param[in] bit_field MaxMinCurr register bit field to be written. 02367 * @param[out] currentA Pointer to save result value. 02368 * The maximum and minimum currents are each stored 02369 * as two’s complement 8-bit values with 8mA resolution. 02370 * 02371 * @return 0 on success, error code on failure. 02372 */ 02373 int get_fg_max_min_curr(reg_bit_max_min_curr_t bit_field, float *currentA); 02374 02375 /** 02376 * @brief Set bit field of fuel-gauge AIN0 (0x27) register. 02377 * 02378 * @param[in] percent to be written to register. 02379 * AIN register with an LSB of 0.0122%. The value from 0% to 100%. 02380 * 02381 * @return 0 on success, error code on failure. 02382 */ 02383 int set_fg_ain0(float percent); 02384 02385 /** 02386 * @brief Get bit field of fuel-gauge AIN0 (0x27) register. 02387 * 02388 * @param[out] percent Pointer to save result value. 02389 * AIN register with an LSB of 0.0122%. The value from 0% to 100%. 02390 * 02391 * @return 0 on success, error code on failure. 02392 */ 02393 int get_fg_ain0(float *percent); 02394 02395 /** 02396 * @brief Set bit field of fuel-gauge Timer (0x3E) register. 02397 * 02398 * @param[in] second to be written to register. 02399 * The Timer register LSB is 175.8ms, giving a full-scale range of 0 to 3.2 hours. 02400 * 02401 * @return 0 on success, error code on failure. 02402 */ 02403 int set_fg_timer(float second); 02404 02405 /** 02406 * @brief Get bit field of fuel-gauge Timer (0x3E) register. 02407 * 02408 * @param[out] second Pointer to save result value. 02409 * The Timer register LSB is 175.8ms, giving a full-scale range of 0 to 3.2 hours. 02410 * 02411 * @return 0 on success, error code on failure. 02412 */ 02413 int get_fg_timer(float *second); 02414 02415 /** 02416 * @brief Set bit field of fuel-gauge ShdnTimer (0x3F) register. 02417 * Shutdown Counter. Bit 12:0. 02418 * 02419 * @param[in] second to be written to register. 02420 * The counter LSB is 1.4s. 02421 * 02422 * @return 0 on success, error code on failure. 02423 */ 02424 int set_fg_shdnctr(float second); 02425 02426 /** 02427 * @brief Get bit field of fuel-gauge ShdnTimer (0x3F) register. 02428 * Shutdown Counter. Bit 12:0. 02429 * 02430 * @param[out] second Pointer to save result value. 02431 * The counter LSB is 1.4s. 02432 * 02433 * @return 0 on success, error code on failure. 02434 */ 02435 int get_fg_shdnctr(float *second); 02436 02437 /** 02438 * @brief Set bit field of fuel-gauge ShdnTimer (0x3F) register. 02439 * Shutdown timeout period from a minimum of 45s to a maximum of 1.6h. Bit 15:13. 02440 * 02441 * @param[in] second to be written to register. 02442 * Shutdown Timeout Period = 175.8ms × 2^(8+THR). 02443 * 02444 * @return 0 on success, error code on failure. 02445 */ 02446 int set_fg_shdn_thr(float second); 02447 02448 /** 02449 * @brief Get bit field of fuel-gauge ShdnTimer (0x3F) register. 02450 * Shutdown timeout period from a minimum of 45s to a maximum of 1.6h. Bit 15:13. 02451 * 02452 * @param[out] second Pointer to save result value. 02453 * Shutdown Timeout Period = 175.8ms × 2^(8+THR). 02454 * 02455 * @return 0 on success, error code on failure. 02456 */ 02457 int get_fg_shdn_thr(float *second); 02458 02459 /** 02460 * @brief Set bit field of fuel-gauge TimerH (0xBE) register. 02461 * 02462 * @param[in] hour to be written to register. 02463 * A 3.2-hour LSB gives a full-scale range for the register of up to 23.94 years. 02464 * 02465 * @return 0 on success, error code on failure. 02466 */ 02467 int set_fg_timerh(float hour); 02468 02469 /** 02470 * @brief Get bit field of fuel-gauge TimerH (0xBE) register. 02471 * 02472 * @param[out] hour Pointer to save result value. 02473 * A 3.2-hour LSB gives a full-scale range for the register of up to 23.94 years. 02474 * 02475 * @return 0 on success, error code on failure. 02476 */ 02477 int get_fg_timerh(float *hour); 02478 02479 /*ModelGauge m5 Output Registers*/ 02480 02481 /** 02482 * @brief Set bit field of fuel-gauge RepCap (0x05) register. 02483 * RepCap is the reported remaining capacity in mAh. 02484 * 02485 * @param[in] repCapmAh to be written to register. 02486 * LSB is 0.1mAh. Min value is 0.0mAh and Max value is 6553.5mAh. 02487 * 02488 * @return 0 on success, error code on failure. 02489 */ 02490 int set_fg_rep_cap(float repCapmAh); 02491 02492 /** 02493 * @brief Get bit field of fuel-gauge RepCap (0x05) register. 02494 * RepCap is the reported remaining capacity in mAh. 02495 * 02496 * @param[out] repCapmAh Pointer to save result value. 02497 * LSB is 0.1mAh. Min value is 0.0mAh and Max value is 6553.5mAh. 02498 * 02499 * @return 0 on success, error code on failure. 02500 */ 02501 int get_fg_rep_cap(float *repCapmAh); 02502 02503 /** 02504 * @brief Set bit field of fuel-gauge RepSOC (0x06) register. 02505 * 02506 * @param[in] percent to be written to register. 02507 * LSB is 1/256%. Min value is 0.0% and Max value is 255.9961%. 02508 * 02509 * @return 0 on success, error code on failure. 02510 */ 02511 int set_fg_rep_soc(float percent); 02512 02513 /** 02514 * @brief Get bit field of fuel-gauge RepSOC (0x06) register. 02515 * 02516 * @param[out] percent Pointer to save result value. 02517 * LSB is 1/256%. Min value is 0.0% and Max value is 255.9961%. 02518 * 02519 * @return 0 on success, error code on failure. 02520 */ 02521 int get_fg_rep_soc(float *percent); 02522 02523 /** 02524 * @brief Set bit field of fuel-gauge AvSOC (0x0E) register. 02525 * 02526 * @param[in] percent to be written to register. 02527 * LSB is 1/256%. Min value is 0.0% and Max value is 255.9961%. 02528 * 02529 * @return 0 on success, error code on failure. 02530 */ 02531 int set_fg_av_soc(float percent); 02532 02533 /** 02534 * @brief Get bit field of fuel-gauge AvSOC (0x0E) register. 02535 * 02536 * @param[out] percent Pointer to save result value. 02537 * LSB is 1/256%. Min value is 0.0% and Max value is 255.9961%. 02538 * 02539 * @return 0 on success, error code on failure. 02540 */ 02541 int get_fg_av_soc(float *percent); 02542 02543 /** 02544 * @brief Set bit field of fuel-gauge FullCapRep (0x10) register. 02545 * 02546 * @param[in] repCapmAh to be written to register. 02547 * LSB is 0.1mAh. Min value is 0.0mAh and Max value is 6553.5mAh. 02548 * 02549 * @return 0 on success, error code on failure. 02550 */ 02551 int set_fg_full_cap_reg(float repCapmAh); 02552 02553 /** 02554 * @brief Get bit field of fuel-gauge FullCapRep (0x10) register. 02555 * 02556 * @param[out] repCapmAh Pointer to save result value. 02557 * LSB is 0.1mAh. Min value is 0.0mAh and Max value is 6553.5mAh. 02558 * 02559 * @return 0 on success, error code on failure. 02560 */ 02561 int get_fg_full_cap_reg(float *repCapmAh); 02562 02563 /** 02564 * @brief Set bit field of fuel-gauge TTE (0x11) register. 02565 * 02566 * @param[in] minute to be written to register. 02567 * LSB is 5.625s. Min value is 0.0s and Max value is 102.3984h. 02568 * 02569 * @return 0 on success, error code on failure. 02570 */ 02571 int set_fg_tte(float minute); 02572 02573 /** 02574 * @brief Get bit field of fuel-gauge TTE (0x11) register. 02575 * 02576 * @param[out] minute Pointer to save result value. 02577 * LSB is 5.625s. Min value is 0.0s and Max value is 102.3984h. 02578 * 02579 * @return 0 on success, error code on failure. 02580 */ 02581 int get_fg_tte(float *minute); 02582 02583 /** 02584 * @brief Set bit field of fuel-gauge RCell (0x14) register. 02585 * 02586 * @param[in] resOhm to be written to register. 02587 * LSB is 1/4096Ohm. Min value is 0.0Ohm and Max value is 15.99976Ohm. 02588 * 02589 * @return 0 on success, error code on failure. 02590 */ 02591 int set_fg_rcell(float resOhm); 02592 02593 /** 02594 * @brief Get bit field of fuel-gauge RCell (0x14) register. 02595 * 02596 * @param[out] resOhm Pointer to save result value. 02597 * LSB is 1/4096Ohm. Min value is 0.0Ohm and Max value is 15.99976Ohm. 02598 * 02599 * @return 0 on success, error code on failure. 02600 */ 02601 int get_fg_rcell(float *resOhm); 02602 02603 /** 02604 * @brief Set bit field of fuel-gauge Cycles (0x17) register. 02605 * 02606 * @param[in] percent to be written to register. 02607 * The LSB indicates 1% of a battery cycle (1% charge + 1% discharge). 02608 * 02609 * @return 0 on success, error code on failure. 02610 */ 02611 int set_fg_cycles(uint16_t percent); 02612 02613 /** 02614 * @brief Get bit field of fuel-gauge Cycles (0x17) register. 02615 * 02616 * @param[out] percent Pointer to save result value. 02617 * The LSB indicates 1% of a battery cycle (1% charge + 1% discharge). 02618 * 02619 * @return 0 on success, error code on failure. 02620 */ 02621 int get_fg_cycles(uint16_t *percent); 02622 02623 /** 02624 * @brief Set bit field of fuel-gauge AvCap (0x1F) register. 02625 * 02626 * @param[in] avCapmAh to be written to register. 02627 * LSB is 0.1mAh. Min value is 0.0mAh and Max value is 6553.5mAh. 02628 * 02629 * @return 0 on success, error code on failure. 02630 */ 02631 int set_fg_av_cap(float avCapmAh); 02632 02633 /** 02634 * @brief Get bit field of fuel-gauge AvCap (0x1F) register. 02635 * 02636 * @param[out] avCapmAh Pointer to save result value. 02637 * LSB is 0.1mAh. Min value is 0.0mAh and Max value is 6553.5mAh. 02638 * 02639 * @return 0 on success, error code on failure. 02640 */ 02641 int get_fg_av_cap(float *avCapmAh); 02642 02643 /** 02644 * @brief Set bit field of fuel-gauge TTF (0x20) register. 02645 * 02646 * @param[in] second to be written to register. 02647 * LSB is 5.625s. Min value is 0.0s and Max value is 102.3984h. 02648 * 02649 * @return 0 on success, error code on failure. 02650 */ 02651 int set_fg_ttf(float second); 02652 02653 /** 02654 * @brief Get bit field of fuel-gauge TTF (0x20) register. 02655 * 02656 * @param[out] second Pointer to save result value. 02657 * LSB is 5.625s. Min value is 0.0s and Max value is 102.3984h. 02658 * 02659 * @return 0 on success, error code on failure. 02660 */ 02661 int get_fg_ttf(float *second); 02662 02663 /** 02664 * @brief Disable all interrupts 02665 * 02666 * @return 0 on success, error code on failure 02667 */ 02668 int irq_disable_all(); 02669 02670 /** 02671 * @brief Set Interrupt Handler for a Specific Interrupt ID. 02672 * 02673 * @param[in] id reg_bit_reg_bit_int_glbl_t id, one of INTR_ID_*. 02674 * @param[in] func Interrupt handler function. 02675 * @param[in] cb Interrupt handler data. 02676 */ 02677 void set_interrupt_handler(reg_bit_int_glbl_t id, interrupt_handler_function func, void *cb); 02678 }; 02679 #endif
Generated on Fri Aug 26 2022 12:03:39 by
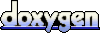