
Maxim Integrated MAX5719 20-bit, 0.05nV-sec DAC Test program running on MAX32625MBED. Control through USB Serial commands using a terminal emulator such as teraterm or putty.
Dependencies: MaximTinyTester CmdLine MAX5719 USBDevice
Test_Menu_MAX5719.cpp
00001 // /******************************************************************************* 00002 // * Copyright (C) 2021 Maxim Integrated Products, Inc., All Rights Reserved. 00003 // * 00004 // * Permission is hereby granted, free of charge, to any person obtaining a 00005 // * copy of this software and associated documentation files (the "Software"), 00006 // * to deal in the Software without restriction, including without limitation 00007 // * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 // * and/or sell copies of the Software, and to permit persons to whom the 00009 // * Software is furnished to do so, subject to the following conditions: 00010 // * 00011 // * The above copyright notice and this permission notice shall be included 00012 // * in all copies or substantial portions of the Software. 00013 // * 00014 // * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 // * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 // * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 // * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 // * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 // * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 // * OTHER DEALINGS IN THE SOFTWARE. 00021 // * 00022 // * Except as contained in this notice, the name of Maxim Integrated 00023 // * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 // * Products, Inc. Branding Policy. 00025 // * 00026 // * The mere transfer of this software does not imply any licenses 00027 // * of trade secrets, proprietary technology, copyrights, patents, 00028 // * trademarks, maskwork rights, or any other form of intellectual 00029 // * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 // * ownership rights. 00031 // ******************************************************************************* 00032 // */ 00033 // example code includes 00034 // standard include for target platform -- Platform_Include_Boilerplate 00035 #include "mbed.h" 00036 // Platforms: 00037 // - MAX32625MBED 00038 // - supports mbed-os-5.11, requires USBDevice library 00039 // - add https://developer.mbed.org/teams/MaximIntegrated/code/USBDevice/ 00040 // - remove max32630fthr library (if present) 00041 // - remove MAX32620FTHR library (if present) 00042 // - MAX32600MBED 00043 // - Please note the last supported version is Mbed OS 6.3. 00044 // - remove max32630fthr library (if present) 00045 // - remove MAX32620FTHR library (if present) 00046 // - Windows 10 note: Don't connect HDK until you are ready to load new firmware into the board. 00047 // - NUCLEO_F446RE 00048 // - remove USBDevice library 00049 // - remove max32630fthr library (if present) 00050 // - remove MAX32620FTHR library (if present) 00051 // - NUCLEO_F401RE 00052 // - remove USBDevice library 00053 // - remove max32630fthr library (if present) 00054 // - remove MAX32620FTHR library (if present) 00055 // - MAX32630FTHR 00056 // - #include "max32630fthr.h" 00057 // - add http://developer.mbed.org/teams/MaximIntegrated/code/max32630fthr/ 00058 // - remove MAX32620FTHR library (if present) 00059 // - MAX32620FTHR 00060 // - #include "MAX32620FTHR.h" 00061 // - remove max32630fthr library (if present) 00062 // - add https://os.mbed.com/teams/MaximIntegrated/code/MAX32620FTHR/ 00063 // - not tested yet 00064 // - MAX32625PICO 00065 // - #include "max32625pico.h" 00066 // - add https://os.mbed.com/users/switches/code/max32625pico/ 00067 // - remove max32630fthr library (if present) 00068 // - remove MAX32620FTHR library (if present) 00069 // - not tested yet 00070 // - see https://os.mbed.com/users/switches/code/max32625pico/ 00071 // - see https://os.mbed.com/users/switches/code/PICO_board_demo/ 00072 // - see https://os.mbed.com/users/switches/code/PICO_USB_I2C_SPI/ 00073 // - see https://os.mbed.com/users/switches/code/SerialInterface/ 00074 // - Note: To load the MAX32625PICO firmware, hold the button while 00075 // connecting the USB cable, then copy firmware bin file 00076 // to the MAINTENANCE drive. 00077 // - see https://os.mbed.com/platforms/MAX32625PICO/ 00078 // - see https://os.mbed.com/teams/MaximIntegrated/wiki/MAX32625PICO-Firmware-Updates 00079 // 00080 // end Platform_Include_Boilerplate 00081 #include "MAX5719.h" 00082 #include "CmdLine.h" 00083 #include "MaximTinyTester.h" 00084 00085 #include "MAX5719.h" 00086 extern MAX5719 g_MAX5719_device; // defined in main.cpp 00087 00088 00089 00090 void MAX5719_menu_help(CmdLine & cmdLine) 00091 { 00092 cmdLine.serial().printf("\r\n ! -- Init"); 00093 cmdLine.serial().printf("\r\n A code=?|code=1.234V -- CODE"); 00094 cmdLine.serial().printf("\r\n B -- LOAD"); 00095 cmdLine.serial().printf("\r\n C code=?|code=1.234V -- CODE_LOAD"); 00096 // 00097 cmdLine.serial().printf("\r\n @ -- print MAX5719 configuration"); 00098 00099 // 00100 // case 'G'..'Z','g'..'z' are reserved for GPIO commands 00101 // case 'A'..'F','a'..'f' may be available if not claimed by bitstream commands 00102 cmdLine.serial().printf("\r\n L -- LDACb output LH high LL low"); // TODO: ExternFunctionGPIOPinCommand testMenuGPIOItemsDict 00103 00104 // 00105 } 00106 00107 bool MAX5719_menu_onEOLcommandParser(CmdLine & cmdLine) 00108 { 00109 00110 00111 // parse argument int32_t DACCode 00112 int32_t DACCode = g_MAX5719_device.DACCode; // default to global property value 00113 if (cmdLine.parse_int32_dec("DACCode", DACCode)) 00114 { 00115 g_MAX5719_device.DACCode = DACCode; // update global property value 00116 } 00117 // "code" is an alias for argument "DACCode"; support DACCodeOfVoltage 00118 { // enclose temporary voltageV 00119 double voltageV; // support DACCodeOfVoltage 00120 switch (cmdLine.parse_double_or_int32("code", voltageV, DACCode)) 00121 { 00122 case 2: // 2: parsed as double 00123 // parse_double_or_uint32 returns 1: parsed as integer; 2: parsed as double 00124 DACCode = g_MAX5719_device.DACCodeOfVoltage((double)voltageV); 00125 g_MAX5719_device.DACCode = DACCode; // update global property value 00126 cmdLine.serial().printf("\r\nDACCodeOfVoltage(%1.6fV)=0x%6.6X\r\n", voltageV, g_MAX5719_device.DACCode); 00127 break; 00128 case 1: // 1: parsed as integer 00129 g_MAX5719_device.DACCode = DACCode; // update global property value 00130 break; 00131 } 00132 } // enclose temporary voltageV 00133 00134 // parse argument double VRef 00135 double VRef = g_MAX5719_device.VRef; // default to global property value 00136 if (cmdLine.parse_double("VRef", VRef)) 00137 { 00138 g_MAX5719_device.VRef = VRef; // update global property value 00139 } 00140 00141 switch (cmdLine[0]) 00142 { 00143 case '@': 00144 { 00145 cmdLine.serial().printf("VRef = "); 00146 cmdLine.serial().printf("%1.6f\r\n", g_MAX5719_device.VRef); 00147 cmdLine.serial().printf("DACCode = "); 00148 cmdLine.serial().printf("%ld = 0x%8.8lx\r\n", g_MAX5719_device.DACCode, g_MAX5719_device.DACCode); 00149 return true; // command handled by MAX5719 00150 break; 00151 } 00152 // case 'G'..'Z','g'..'z' are reserved for GPIO commands 00153 // case 'A'..'F','a'..'f' may be available if not claimed by bitstream commands 00154 case 'L': 00155 { 00156 switch (cmdLine[1]) 00157 { 00158 case 'H': 00159 { 00160 g_MAX5719_device.LDACboutputValue(1); 00161 break; 00162 } 00163 case 'L': 00164 { 00165 g_MAX5719_device.LDACboutputValue(0); 00166 break; 00167 } 00168 } 00169 return true; // command handled by MAX5719 00170 break; 00171 } 00172 // case '0'..'9','A'..'F','a'..'f' letters are reserved for bitstream commands 00173 case '!': 00174 { 00175 // test menu command '!' handler: 00176 // helpString='! -- Init' 00177 // CMD_='None' 00178 // CommandName='Init' 00179 // CommandParamIn='void' 00180 // CommandReturnType='void' 00181 // @Pre='' 00182 // @Param[in]='' 00183 // @Param[out]='' 00184 // @Post='' 00185 // displayPost='' 00186 // @Return='' 00187 // @Test='@test group CODE_LOAD // Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default)' 00188 // @Test='@test group CODE_LOAD tinyTester.print("VRef = 4.096 MAX5719 20-bit LSB = 0.000004V = 3.90625uV")' 00189 // @Test='@test group CODE_LOAD tinyTester.print("Wire MAX5719 OUT to platform AIN0 for analog loopback tests...")' 00190 // @Test='@test group CODE_LOAD VRef = 4.096' 00191 // @Test='@test group CODE_LOAD tinyTester.blink_time_msec = 75 // default 75 resume hardware self test' 00192 // @Test='@test group CODE_LOAD tinyTester.settle_time_msec = 500' 00193 // @Test='@test Init()' 00194 // @Test='@test VRef expect 4.096 // Nominal Full-Scale Voltage Reference' 00195 // @Test='@test group CODE_LOAD tinyTester.err_threshold = 0.050' 00196 // @Test='@test group CODE_LOAD tinyTester.print("0x000000 = 0.000V")' 00197 // @Test='@test group CODE_LOAD CODE_LOAD(0x000000) // 0.000V' 00198 // @Test='@test group CODE_LOAD tinyTester.Wait_Output_Settling()' 00199 // @Test='@test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(0.000000)' 00200 // @Test='/*mbed:@test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 */' 00201 // @Test='@test group CODE_LOAD tinyTester.print("0x01f400 = 0.500V")' 00202 // @Test='@test group CODE_LOAD CODE_LOAD(0x01f400) // 0.500V' 00203 // @Test='@test group CODE_LOAD tinyTester.Wait_Output_Settling()' 00204 // @Test='@test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(0.500000)' 00205 // @Test='/*mbed:@test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 */' 00206 // @Test='@test group CODE_LOAD tinyTester.print("0x03e800 = 1.000V")' 00207 // @Test='@test group CODE_LOAD CODE_LOAD(0x03e800) // 1.000V' 00208 // @Test='@test group CODE_LOAD tinyTester.Wait_Output_Settling()' 00209 // @Test='@test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(1.000000)' 00210 // @Test='/*mbed:@test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 */' 00211 // @Test='@test group CODE_LOAD tinyTester.err_threshold = /*Arduino:0.050*/ /*mbed:0.075*/ /*eabi:0.050*/' 00212 // @Test='@test group CODE_LOAD tinyTester.print("0x05dc00 = 1.500V")' 00213 // @Test='@test group CODE_LOAD CODE_LOAD(0x05dc00) // 1.500V' 00214 // @Test='@test group CODE_LOAD tinyTester.Wait_Output_Settling()' 00215 // @Test='@test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(1.500000)' 00216 // @Test='/*mbed:@test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 */' 00217 // @Test='@test group CODE_LOAD tinyTester.err_threshold = /*Arduino:0.050*/ /*mbed:0.100*/ /*eabi:0.050*/' 00218 // @Test='@test group CODE_LOAD tinyTester.print("0x07d000 = 2.000V")' 00219 // @Test='@test group CODE_LOAD CODE_LOAD(0x07d000) // 2.000V' 00220 // @Test='@test group CODE_LOAD tinyTester.Wait_Output_Settling()' 00221 // @Test='@test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(2.000000)' 00222 // @Test='/*mbed:@test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 */' 00223 // @Test='@test group CODE_LOAD tinyTester.err_threshold = /*Arduino:0.050*/ /*mbed:0.150*/ /*eabi:0.050*/' 00224 // @Test='@test group CODE_LOAD tinyTester.print("0x09c400 = 2.500V")' 00225 // @Test='@test group CODE_LOAD CODE_LOAD(0x09c400) // 2.500V' 00226 // @Test='@test group CODE_LOAD tinyTester.Wait_Output_Settling()' 00227 // @Test='@test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(2.500000)' 00228 // @Test='/*mbed:@test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 */' 00229 // @Test='@test group CODE_LOAD tinyTester.err_threshold = /*Arduino:0.200*/ /*mbed:0.200*/ /*eabi:0.050*/' 00230 // @Test='@test group CODE_LOAD tinyTester.print("0x0bb800 = 3.000V")' 00231 // @Test='@test group CODE_LOAD CODE_LOAD(0x0bb800) // 3.000V' 00232 // @Test='@test group CODE_LOAD tinyTester.Wait_Output_Settling()' 00233 // @Test='@test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(3.000000)' 00234 // @Test='/*mbed:@test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 */' 00235 // @Test='@test group CODE_LOAD tinyTester.err_threshold = /*Arduino:0.250*/ /*mbed:0.250*/ /*eabi:0.050*/' 00236 // @Test='@test group CODE_LOAD tinyTester.print("0x0dac00 = 3.500V")' 00237 // @Test='@test group CODE_LOAD CODE_LOAD(0x0dac00) // 3.500V' 00238 // @Test='@test group CODE_LOAD tinyTester.Wait_Output_Settling()' 00239 // @Test='@test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(3.500000)' 00240 // @Test='/*mbed:@test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 */' 00241 // @Test='@test group CODE_LOAD tinyTester.err_threshold = /*Arduino:0.500*/ /*mbed:0.500*/ /*eabi:0.050*/' 00242 // @Test='@test group CODE_LOAD tinyTester.print("0x0fa000 = 4.000V")' 00243 // @Test='@test group CODE_LOAD CODE_LOAD(0x0fa000) // 4.000V' 00244 // @Test='@test group CODE_LOAD tinyTester.Wait_Output_Settling()' 00245 // @Test='@test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(4.000000)' 00246 // @Test='/*mbed:@test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 */' 00247 // @Test='@test group CODE_LOAD tinyTester.err_threshold = /*Arduino:0.750*/ /*mbed:0.750*/ /*eabi:0.050*/' 00248 // @Test='@test group CODE_LOAD tinyTester.print("0x0fffff = 4.095V")' 00249 // @Test='@test group CODE_LOAD CODE_LOAD(0x0fffff) // 4.095V' 00250 // @Test='@test group CODE_LOAD tinyTester.Wait_Output_Settling()' 00251 // @Test='@test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(4.095000)' 00252 // @Test='/*mbed:@test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 */' 00253 // @Test='@test group CODE_LOAD tinyTester.err_threshold = /*Arduino:0.200*/ /*mbed:0.200*/ /*eabi:0.050*/' 00254 // @Test='@test group CODE_LOAD tinyTester.print("0x080000 // 2.048V")' 00255 // @Test='@test group CODE_LOAD CODE_LOAD(0x080000) // 2.048V' 00256 // @Test='@test group CODE_LOAD tinyTester.Wait_Output_Settling()' 00257 // @Test='@test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(2.048000)' 00258 // @Test='/*mbed:@test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 */' 00259 // @Test='@test group CODE_LOAD_2V5 // Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) (no run on button)' 00260 // @Test='@test group CODE_LOAD_2V5 tinyTester.err_threshold = /*Arduino:0.150*/ /*mbed:0.150*/ /*eabi:0.050*/' 00261 // @Test='@test group CODE_LOAD_2V5 tinyTester.print("0x09c400 = 2.500V")' 00262 // @Test='@test group CODE_LOAD_2V5 CODE_LOAD(0x09c400) // 2.500V' 00263 // @Test='@test group CODE_LOAD_2V5 tinyTester.Wait_Output_Settling()' 00264 // @Test='@test group CODE_LOAD_2V5 tinyTester.AnalogIn0_Read_Expect_voltageV(2.500000)' 00265 // @Test='/*mbed:@test group CODE_LOAD_2V5 tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 */' 00266 // @Test='@test group CODE_LOAD_3V0 // Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) (no run on button)' 00267 // @Test='@test group CODE_LOAD_3V0 tinyTester.err_threshold = /*Arduino:0.200*/ /*mbed:0.200*/ /*eabi:0.050*/' 00268 // @Test='@test group CODE_LOAD_3V0 tinyTester.print("0x0bb800 = 3.000V")' 00269 // @Test='@test group CODE_LOAD_3V0 CODE_LOAD(0x0bb800) // 3.000V' 00270 // @Test='@test group CODE_LOAD_3V0 tinyTester.Wait_Output_Settling()' 00271 // @Test='@test group CODE_LOAD_3V0 tinyTester.AnalogIn0_Read_Expect_voltageV(3.000000)' 00272 // @Test='/*mbed:@test group CODE_LOAD_3V0 tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 */' 00273 // @Test='@test group CODE_LOAD_4V1 // Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) (no run on button)' 00274 // @Test='@test group CODE_LOAD_4V1 tinyTester.err_threshold = /*Arduino:0.750*/ /*mbed:0.750*/ /*eabi:0.050*/' 00275 // @Test='@test group CODE_LOAD_4V1 tinyTester.print("0x0fffff = 4.095V")' 00276 // @Test='@test group CODE_LOAD_4V1 CODE_LOAD(0x0fffff) // 4.095V' 00277 // @Test='@test group CODE_LOAD_4V1 tinyTester.Wait_Output_Settling()' 00278 // @Test='@test group CODE_LOAD_4V1 tinyTester.AnalogIn0_Read_Expect_voltageV(4.095000)' 00279 // @Test='/*mbed:@test group CODE_LOAD_4V1 tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 */' 00280 cmdLine.serial().printf("Init"); 00281 // call function Init 00282 g_MAX5719_device.Init(); 00283 return true; // command handled by MAX5719 00284 } // end case '!' 00285 break; 00286 case 'A': 00287 { 00288 // test menu command 'A' handler: 00289 // helpString='A code=?|code=1.234V -- CODE' 00290 // CMD_='None' 00291 // CommandName='CODE' 00292 // CommandParamIn='uint32_t dacCodeLsbs' 00293 // CommandReturnType='uint8_t' 00294 // @Pre='' 00295 // @Param[in]='' 00296 // @Param[out]='' 00297 // @Post='' 00298 // displayPost='' 00299 // @Return='@return 1 on success; 0 on failure' 00300 // parse argument list 00301 // parse argument uint32_t dacCodeLsbs 00302 uint32_t dacCodeLsbs = 0; // --- g_MAX5719_device.__WARNING_no_match_for_argname_dacCodeLsbs_in_MAX5719_device_t__; // default to global property value 00303 if (cmdLine.parse_uint32_dec("dacCodeLsbs", dacCodeLsbs)) 00304 { 00305 // g_MAX5719_device.__WARNING_no_match_for_argname_dacCodeLsbs_in_MAX5719_device_t__ = dacCodeLsbs; // update global property value 00306 } 00307 // "code" is an alias for argument "dacCodeLsbs"; support DACCodeOfVoltage 00308 { // enclose temporary voltageV 00309 double voltageV; // support DACCodeOfVoltage 00310 switch (cmdLine.parse_double_or_uint32("code", voltageV, dacCodeLsbs)) 00311 { 00312 case 2: // 2: parsed as double 00313 // parse_double_or_uint32 returns 1: parsed as integer; 2: parsed as double 00314 dacCodeLsbs = g_MAX5719_device.DACCodeOfVoltage((double)voltageV); 00315 // g_MAX5719_device.__WARNING_no_match_for_argname_dacCodeLsbs_in_MAX5719_device_t__ = dacCodeLsbs; // update global property value 00316 cmdLine.serial().printf("\r\nDACCodeOfVoltage(%1.6fV)=0x%6.6X\r\n", voltageV, dacCodeLsbs); 00317 break; 00318 case 1: // 1: parsed as integer 00319 // g_MAX5719_device.__WARNING_no_match_for_argname_dacCodeLsbs_in_MAX5719_device_t__ = dacCodeLsbs; // update global property value 00320 break; 00321 } 00322 } // enclose temporary voltageV 00323 // print arguments 00324 cmdLine.serial().printf("CODE"); 00325 cmdLine.serial().printf(" dacCodeLsbs=%ld", dacCodeLsbs); 00326 cmdLine.serial().printf("\r\n"); 00327 // call function CODE(dacCodeLsbs) 00328 uint8_t result = g_MAX5719_device.CODE(dacCodeLsbs); 00329 cmdLine.serial().printf(" =%d\r\n", result); 00330 return true; // command handled by MAX5719 00331 } // end case 'A' 00332 break; 00333 case 'B': 00334 { 00335 // test menu command 'B' handler: 00336 // helpString='B -- LOAD' 00337 // CMD_='None' 00338 // CommandName='LOAD' 00339 // CommandParamIn='void' 00340 // CommandReturnType='uint8_t' 00341 // @Pre='' 00342 // @Param[in]='' 00343 // @Param[out]='' 00344 // @Post='' 00345 // displayPost='' 00346 // @Return='@return 1 on success; 0 on failure' 00347 cmdLine.serial().printf("LOAD"); 00348 // call function LOAD 00349 uint8_t result = g_MAX5719_device.LOAD(); 00350 cmdLine.serial().printf(" =%d\r\n", result); 00351 return true; // command handled by MAX5719 00352 } // end case 'B' 00353 break; 00354 case 'C': 00355 { 00356 // test menu command 'C' handler: 00357 // helpString='C code=?|code=1.234V -- CODE_LOAD' 00358 // CMD_='None' 00359 // CommandName='CODE_LOAD' 00360 // CommandParamIn='uint32_t dacCodeLsbs' 00361 // CommandReturnType='uint8_t' 00362 // @Pre='' 00363 // @Param[in]='' 00364 // @Param[out]='' 00365 // @Post='' 00366 // displayPost='' 00367 // @Return='@return 1 on success; 0 on failure' 00368 // parse argument list 00369 // parse argument uint32_t dacCodeLsbs 00370 uint32_t dacCodeLsbs = 0; // --- g_MAX5719_device.__WARNING_no_match_for_argname_dacCodeLsbs_in_MAX5719_device_t__; // default to global property value 00371 if (cmdLine.parse_uint32_dec("dacCodeLsbs", dacCodeLsbs)) 00372 { 00373 // g_MAX5719_device.__WARNING_no_match_for_argname_dacCodeLsbs_in_MAX5719_device_t__ = dacCodeLsbs; // update global property value 00374 } 00375 // "code" is an alias for argument "dacCodeLsbs"; support DACCodeOfVoltage 00376 { // enclose temporary voltageV 00377 double voltageV; // support DACCodeOfVoltage 00378 switch (cmdLine.parse_double_or_uint32("code", voltageV, dacCodeLsbs)) 00379 { 00380 case 2: // 2: parsed as double 00381 // parse_double_or_uint32 returns 1: parsed as integer; 2: parsed as double 00382 dacCodeLsbs = g_MAX5719_device.DACCodeOfVoltage((double)voltageV); 00383 // g_MAX5719_device.__WARNING_no_match_for_argname_dacCodeLsbs_in_MAX5719_device_t__ = dacCodeLsbs; // update global property value 00384 cmdLine.serial().printf("\r\nDACCodeOfVoltage(%1.6fV)=0x%6.6X\r\n", voltageV, dacCodeLsbs); 00385 break; 00386 case 1: // 1: parsed as integer 00387 // g_MAX5719_device.__WARNING_no_match_for_argname_dacCodeLsbs_in_MAX5719_device_t__ = dacCodeLsbs; // update global property value 00388 break; 00389 } 00390 } // enclose temporary voltageV 00391 // print arguments 00392 cmdLine.serial().printf("CODE_LOAD"); 00393 cmdLine.serial().printf(" dacCodeLsbs=%ld", dacCodeLsbs); 00394 cmdLine.serial().printf("\r\n"); 00395 // call function CODE_LOAD(dacCodeLsbs) 00396 uint8_t result = g_MAX5719_device.CODE_LOAD(dacCodeLsbs); 00397 cmdLine.serial().printf(" =%d\r\n", result); 00398 return true; // command handled by MAX5719 00399 } // end case 'C' 00400 break; 00401 } // end switch (cmdLine[0]) 00402 return false; // command not handled by MAX5719 00403 } // end bool MAX5719_menu_onEOLcommandParser(CmdLine & cmdLine) 00404
Generated on Tue Jul 12 2022 16:06:18 by
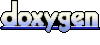