
Maxim Integrated MAX5719 20-bit, 0.05nV-sec DAC Test program running on MAX32625MBED. Control through USB Serial commands using a terminal emulator such as teraterm or putty.
Dependencies: MaximTinyTester CmdLine MAX5719 USBDevice
Test_Main_MAX5719.cpp
00001 // /******************************************************************************* 00002 // * Copyright (C) 2021 Maxim Integrated Products, Inc., All Rights Reserved. 00003 // * 00004 // * Permission is hereby granted, free of charge, to any person obtaining a 00005 // * copy of this software and associated documentation files (the "Software"), 00006 // * to deal in the Software without restriction, including without limitation 00007 // * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 // * and/or sell copies of the Software, and to permit persons to whom the 00009 // * Software is furnished to do so, subject to the following conditions: 00010 // * 00011 // * The above copyright notice and this permission notice shall be included 00012 // * in all copies or substantial portions of the Software. 00013 // * 00014 // * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 // * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 // * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 // * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 // * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 // * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 // * OTHER DEALINGS IN THE SOFTWARE. 00021 // * 00022 // * Except as contained in this notice, the name of Maxim Integrated 00023 // * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 // * Products, Inc. Branding Policy. 00025 // * 00026 // * The mere transfer of this software does not imply any licenses 00027 // * of trade secrets, proprietary technology, copyrights, patents, 00028 // * trademarks, maskwork rights, or any other form of intellectual 00029 // * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 // * ownership rights. 00031 // ******************************************************************************* 00032 // */ 00033 // example code includes 00034 // standard include for target platform -- Platform_Include_Boilerplate 00035 #include "mbed.h" 00036 // Platforms: 00037 // - MAX32625MBED 00038 // - supports mbed-os-5.11, requires USBDevice library 00039 // - add https://developer.mbed.org/teams/MaximIntegrated/code/USBDevice/ 00040 // - remove max32630fthr library (if present) 00041 // - remove MAX32620FTHR library (if present) 00042 // - MAX32600MBED 00043 // - Please note the last supported version is Mbed OS 6.3. 00044 // - remove max32630fthr library (if present) 00045 // - remove MAX32620FTHR library (if present) 00046 // - Windows 10 note: Don't connect HDK until you are ready to load new firmware into the board. 00047 // - NUCLEO_F446RE 00048 // - remove USBDevice library 00049 // - remove max32630fthr library (if present) 00050 // - remove MAX32620FTHR library (if present) 00051 // - NUCLEO_F401RE 00052 // - remove USBDevice library 00053 // - remove max32630fthr library (if present) 00054 // - remove MAX32620FTHR library (if present) 00055 // - MAX32630FTHR 00056 // - #include "max32630fthr.h" 00057 // - add http://developer.mbed.org/teams/MaximIntegrated/code/max32630fthr/ 00058 // - remove MAX32620FTHR library (if present) 00059 // - MAX32620FTHR 00060 // - #include "MAX32620FTHR.h" 00061 // - remove max32630fthr library (if present) 00062 // - add https://os.mbed.com/teams/MaximIntegrated/code/MAX32620FTHR/ 00063 // - not tested yet 00064 // - MAX32625PICO 00065 // - #include "max32625pico.h" 00066 // - add https://os.mbed.com/users/switches/code/max32625pico/ 00067 // - remove max32630fthr library (if present) 00068 // - remove MAX32620FTHR library (if present) 00069 // - not tested yet 00070 // - see https://os.mbed.com/users/switches/code/max32625pico/ 00071 // - see https://os.mbed.com/users/switches/code/PICO_board_demo/ 00072 // - see https://os.mbed.com/users/switches/code/PICO_USB_I2C_SPI/ 00073 // - see https://os.mbed.com/users/switches/code/SerialInterface/ 00074 // - Note: To load the MAX32625PICO firmware, hold the button while 00075 // connecting the USB cable, then copy firmware bin file 00076 // to the MAINTENANCE drive. 00077 // - see https://os.mbed.com/platforms/MAX32625PICO/ 00078 // - see https://os.mbed.com/teams/MaximIntegrated/wiki/MAX32625PICO-Firmware-Updates 00079 // 00080 // end Platform_Include_Boilerplate 00081 #include "MAX5719.h" 00082 #include "CmdLine.h" 00083 #include "MaximTinyTester.h" 00084 00085 // optional: serial port 00086 // note: some platforms such as Nucleo-F446RE do not support the USBSerial library. 00087 // In those cases, remove the USBDevice lib from the project and rebuild. 00088 #if defined(TARGET_MAX32625MBED) 00089 #include "USBSerial.h" 00090 USBSerial serial; // virtual serial port over USB (DEV connector) 00091 #elif defined(TARGET_MAX32625PICO) 00092 #include "USBSerial.h" 00093 USBSerial serial; // virtual serial port over USB (DEV connector) 00094 #elif defined(TARGET_MAX32600MBED) 00095 #include "USBSerial.h" 00096 USBSerial serial; // virtual serial port over USB (DEV connector) 00097 #elif defined(TARGET_MAX32630MBED) 00098 #include "USBSerial.h" 00099 USBSerial serial; // virtual serial port over USB (DEV connector) 00100 #else 00101 //#include "USBSerial.h" 00102 Serial serial(USBTX, USBRX); // tx, rx 00103 #endif 00104 00105 void on_immediate_0x21(); // Unicode (U+0021) ! EXCLAMATION MARK 00106 void on_immediate_0x7b(); // Unicode (U+007B) { LEFT CURLY BRACKET 00107 void on_immediate_0x7d(); // Unicode (U+007D) } RIGHT CURLY BRACKET 00108 00109 #include "CmdLine.h" 00110 00111 # if HAS_DAPLINK_SERIAL 00112 CmdLine cmdLine_DAPLINKserial(DAPLINKserial, "DAPLINK"); 00113 # endif // HAS_DAPLINK_SERIAL 00114 CmdLine cmdLine_serial(serial, "serial"); 00115 00116 00117 //-------------------------------------------------- 00118 00119 00120 #if defined(TARGET) 00121 // TARGET_NAME macros from targets/TARGET_Maxim/TARGET_MAX32625/device/mxc_device.h 00122 // Create a string definition for the TARGET 00123 #define STRING_ARG(arg) #arg 00124 #define STRING_NAME(name) STRING_ARG(name) 00125 #define TARGET_NAME STRING_NAME(TARGET) 00126 #elif defined(TARGET_MAX32600) 00127 #define TARGET_NAME "MAX32600" 00128 #elif defined(TARGET_LPC1768) 00129 #define TARGET_NAME "LPC1768" 00130 #elif defined(TARGET_NUCLEO_F446RE) 00131 #define TARGET_NAME "NUCLEO_F446RE" 00132 #elif defined(TARGET_NUCLEO_F401RE) 00133 #define TARGET_NAME "NUCLEO_F401RE" 00134 #else 00135 #error TARGET NOT DEFINED 00136 #endif 00137 #if defined(TARGET_MAX32630) 00138 //-------------------------------------------------- 00139 // TARGET=MAX32630FTHR ARM Cortex-M4F 96MHz 2048kB Flash 512kB SRAM 00140 // +-------------[microUSB]-------------+ 00141 // | J1 MAX32630FTHR J2 | 00142 // ______ | [ ] RST GND [ ] | 00143 // ______ | [ ] 3V3 BAT+[ ] | 00144 // ______ | [ ] 1V8 reset SW1 | 00145 // ______ | [ ] GND J4 J3 | 00146 // analogIn0/4 | [a] AIN_0 1.2Vfs (bat) SYS [ ] | switched BAT+ 00147 // analogIn1/5 | [a] AIN_1 1.2Vfs PWR [ ] | external pwr btn 00148 // analogIn2 | [a] AIN_2 1.2Vfs +5V VBUS [ ] | USB +5V power 00149 // analogIn3 | [a] AIN_3 1.2Vfs 1-WIRE P4_0 [d] | D0 dig9 00150 // (I2C2.SDA) | [d] P5_7 SDA2 SRN P5_6 [d] | D1 dig8 00151 // (I2C2.SCL) | [d] P6_0 SCL2 SDIO3 P5_5 [d] | D2 dig7 00152 // D13/SCLK | [s] P5_0 SCLK SDIO2 P5_4 [d] | D3 dig6 00153 // D11/MOSI | [s] P5_1 MOSI SSEL P5_3 [d] | D4 dig5 00154 // D12/MISO | [s] P5_2 MISO RTS P3_3 [d] | D5 dig4 00155 // D10/CS | [s] P3_0 RX CTS P3_2 [d] | D6 dig3 00156 // D9 dig0 | [d] P3_1 TX SCL P3_5 [d] | D7 dig2 00157 // ______ | [ ] GND SDA P3_4 [d] | D8 dig1 00158 // | | 00159 // | XIP Flash MAX14690N | 00160 // | XIP_SCLK P1_0 SDA2 P5_7 | 00161 // | XIP_MOSI P1_1 SCL2 P6_0 | 00162 // | XIP_MISO P1_2 PMIC_INIT P3_7 | 00163 // | XIP_SSEL P1_3 MPC P2_7 | 00164 // | XIP_DIO2 P1_4 MON AIN_0 | 00165 // | XIP_DIO3 P1_5 | 00166 // | | 00167 // | PAN1326B MicroSD LED | 00168 // | BT_RX P0_0 SD_SCLK P0_4 r P2_4 | 00169 // | BT_TX P0_1 SD_MOSI P0_5 g P2_5 | 00170 // | BT_CTS P0_2 SD_MISO P0_6 b P2_6 | 00171 // | BT_RTS P0_3 SD_SSEL P0_7 | 00172 // | BT_RST P1_6 DETECT P2_2 | 00173 // | BT_CLK P1_7 SW2 P2_3 | 00174 // +------------------------------------+ 00175 // MAX32630FTHR board has MAX14690 PMIC on I2C bus (P5_7 SDA, P6_0 SCL) at slave address 0101_000r 0x50 (or 0x28 for 7 MSbit address). 00176 // MAX32630FTHR board has BMI160 accelerometer on I2C bus (P5_7 SDA, P6_0 SCL) at slave address 1101_000r 0xD0 (or 0x68 for 7 MSbit address). 00177 // AIN_0 = AIN0 pin fullscale is 1.2V 00178 // AIN_1 = AIN1 pin fullscale is 1.2V 00179 // AIN_2 = AIN2 pin fullscale is 1.2V 00180 // AIN_3 = AIN3 pin fullscale is 1.2V 00181 // AIN_4 = AIN0 / 5.0 fullscale is 6.0V 00182 // AIN_5 = AIN1 / 5.0 fullscale is 6.0V 00183 // AIN_6 = VDDB / 4.0 fullscale is 4.8V 00184 // AIN_7 = VDD18 fullscale is 1.2V 00185 // AIN_8 = VDD12 fullscale is 1.2V 00186 // AIN_9 = VRTC / 2.0 fullscale is 2.4V 00187 // AIN_10 = x undefined? 00188 // AIN_11 = VDDIO / 4.0 fullscale is 4.8V 00189 // AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 00190 // 00191 #include "max32630fthr.h" 00192 MAX32630FTHR pegasus(MAX32630FTHR::VIO_3V3); 00193 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 1 00194 // MAX32630FTHR board supports only internal VREF = 1.200V at bypass capacitor C15 00195 const float ADC_FULL_SCALE_VOLTAGE = 1.200; 00196 // Arduino connector 00197 #ifndef A0 00198 #define A0 AIN_0 00199 #endif 00200 #ifndef A1 00201 #define A1 AIN_1 00202 #endif 00203 #ifndef A2 00204 #define A2 AIN_2 00205 #endif 00206 #ifndef A3 00207 #define A3 AIN_3 00208 #endif 00209 #ifndef D0 00210 #define D0 P4_0 00211 #endif 00212 #ifndef D1 00213 #define D1 P5_6 00214 #endif 00215 #ifndef D2 00216 #define D2 P5_5 00217 #endif 00218 #ifndef D3 00219 #define D3 P5_4 00220 #endif 00221 #ifndef D4 00222 #define D4 P5_3 00223 #endif 00224 #ifndef D5 00225 #define D5 P3_3 00226 #endif 00227 #ifndef D6 00228 #define D6 P3_2 00229 #endif 00230 #ifndef D7 00231 #define D7 P3_5 00232 #endif 00233 #ifndef D8 00234 #define D8 P3_4 00235 #endif 00236 #ifndef D9 00237 #define D9 P3_1 00238 #endif 00239 #ifndef D10 00240 #define D10 P3_0 00241 #endif 00242 #ifndef D11 00243 #define D11 P5_1 00244 #endif 00245 #ifndef D12 00246 #define D12 P5_2 00247 #endif 00248 #ifndef D13 00249 #define D13 P5_0 00250 #endif 00251 //-------------------------------------------------- 00252 #elif defined(TARGET_MAX32625MBED) 00253 //-------------------------------------------------- 00254 // TARGET=MAX32625MBED ARM Cortex-M4F 96MHz 512kB Flash 160kB SRAM 00255 // +-------------------------------------+ 00256 // | MAX32625MBED Arduino UNO header | 00257 // | | 00258 // | A5/SCL[ ] | P1_7 dig15 00259 // | A4/SDA[ ] | P1_6 dig14 00260 // | AREF=N/C[ ] | 00261 // | GND[ ] | 00262 // | [ ]N/C SCK/13[ ] | P1_0 dig13 00263 // | [ ]IOREF=3V3 MISO/12[ ] | P1_2 dig12 00264 // | [ ]RST MOSI/11[ ]~| P1_1 dig11 00265 // | [ ]3V3 CS/10[ ]~| P1_3 dig10 00266 // | [ ]5V0 9[ ]~| P1_5 dig9 00267 // | [ ]GND 8[ ] | P1_4 dig8 00268 // | [ ]GND | 00269 // | [ ]Vin 7[ ] | P0_7 dig7 00270 // | 6[ ]~| P0_6 dig6 00271 // AIN_0 | [ ]A0 5[ ]~| P0_5 dig5 00272 // AIN_1 | [ ]A1 4[ ] | P0_4 dig4 00273 // AIN_2 | [ ]A2 INT1/3[ ]~| P0_3 dig3 00274 // AIN_3 | [ ]A3 INT0/2[ ] | P0_2 dig2 00275 // dig16 P3_4 | [ ]A4/SDA RST SCK MISO TX>1[ ] | P0_1 dig1 00276 // dig17 P3_5 | [ ]A5/SCL [ ] [ ] [ ] RX<0[ ] | P0_0 dig0 00277 // | [ ] [ ] [ ] | 00278 // | UNO_R3 GND MOSI 5V ____________/ 00279 // \_______________________/ 00280 // 00281 // +------------------------+ 00282 // | | 00283 // | MicroSD LED | 00284 // | SD_SCLK P2_4 r P3_0 | 00285 // | SD_MOSI P2_5 g P3_1 | 00286 // | SD_MISO P2_6 b P3_2 | 00287 // | SD_SSEL P2_7 y P3_3 | 00288 // | | 00289 // | DAPLINK BUTTONS | 00290 // | TX P2_1 SW3 P2_3 | 00291 // | RX P2_0 SW2 P2_2 | 00292 // +------------------------+ 00293 // 00294 // AIN_0 = AIN0 pin fullscale is 1.2V 00295 // AIN_1 = AIN1 pin fullscale is 1.2V 00296 // AIN_2 = AIN2 pin fullscale is 1.2V 00297 // AIN_3 = AIN3 pin fullscale is 1.2V 00298 // AIN_4 = AIN0 / 5.0 fullscale is 6.0V 00299 // AIN_5 = AIN1 / 5.0 fullscale is 6.0V 00300 // AIN_6 = VDDB / 4.0 fullscale is 4.8V 00301 // AIN_7 = VDD18 fullscale is 1.2V 00302 // AIN_8 = VDD12 fullscale is 1.2V 00303 // AIN_9 = VRTC / 2.0 fullscale is 2.4V 00304 // AIN_10 = x undefined? 00305 // AIN_11 = VDDIO / 4.0 fullscale is 4.8V 00306 // AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 00307 // 00308 //#include "max32625mbed.h" // ? 00309 //MAX32625MBED mbed(MAX32625MBED::VIO_3V3); // ? 00310 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 1 00311 // MAX32630FTHR board supports only internal VREF = 1.200V at bypass capacitor C15 00312 const float ADC_FULL_SCALE_VOLTAGE = 1.200; // TODO: ADC_FULL_SCALE_VOLTAGE Pico? 00313 // Arduino connector 00314 #ifndef A0 00315 #define A0 AIN_0 00316 #endif 00317 #ifndef A1 00318 #define A1 AIN_1 00319 #endif 00320 #ifndef A2 00321 #define A2 AIN_2 00322 #endif 00323 #ifndef A3 00324 #define A3 AIN_3 00325 #endif 00326 #ifndef D0 00327 #define D0 P0_0 00328 #endif 00329 #ifndef D1 00330 #define D1 P0_1 00331 #endif 00332 #ifndef D2 00333 #define D2 P0_2 00334 #endif 00335 #ifndef D3 00336 #define D3 P0_3 00337 #endif 00338 #ifndef D4 00339 #define D4 P0_4 00340 #endif 00341 #ifndef D5 00342 #define D5 P0_5 00343 #endif 00344 #ifndef D6 00345 #define D6 P0_6 00346 #endif 00347 #ifndef D7 00348 #define D7 P0_7 00349 #endif 00350 #ifndef D8 00351 #define D8 P1_4 00352 #endif 00353 #ifndef D9 00354 #define D9 P1_5 00355 #endif 00356 #ifndef D10 00357 #define D10 P1_3 00358 #endif 00359 #ifndef D11 00360 #define D11 P1_1 00361 #endif 00362 #ifndef D12 00363 #define D12 P1_2 00364 #endif 00365 #ifndef D13 00366 #define D13 P1_0 00367 #endif 00368 //-------------------------------------------------- 00369 #elif defined(TARGET_MAX32600) 00370 // target MAX32600 00371 // 00372 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 0 00373 const float ADC_FULL_SCALE_VOLTAGE = 1.500; 00374 // 00375 //-------------------------------------------------- 00376 #elif defined(TARGET_MAX32620FTHR) 00377 #warning "TARGET_MAX32620FTHR not previously tested; need to define pins..." 00378 #include "MAX32620FTHR.h" 00379 // Initialize I/O voltages on MAX32620FTHR board 00380 MAX32620FTHR fthr(MAX32620FTHR::VIO_3V3); 00381 //#define USE_LEDS 0 ? 00382 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 1 00383 #warning "TARGET_MAX32620FTHR not previously tested; need to verify ADC_FULL_SCALE_VOLTAGE..." 00384 const float ADC_FULL_SCALE_VOLTAGE = 1.200; 00385 // 00386 //-------------------------------------------------- 00387 #elif defined(TARGET_MAX32625PICO) 00388 #warning "TARGET_MAX32625PICO not previously tested; need to define pins..." 00389 #include "max32625pico.h" 00390 // configure MAX32625PICO VDDIOH mode, and I/O voltages for DIP pins and SWD pins 00391 MAX32625PICO pico( 00392 // vddioh_mode_t iohMode 00393 //~ MAX32625PICO::IOH_OFF, // No connections to VDDIOH 00394 //~ MAX32625PICO::IOH_DIP_IN, // VDDIOH input from DIP pin 1 (AIN0) 00395 //~ MAX32625PICO::IOH_SWD_IN, // VDDIOH input from SWD pin 1 00396 MAX32625PICO::IOH_3V3, // VDDIOH = 3.3V from local supply 00397 //~ MAX32625PICO::IOH_DIP_OUT, // VDDIOH = 3.3V output to DIP pin 1 00398 //~ MAX32625PICO::IOH_SWD_OUT, // VDDIOH = 3.3V output to SWD pin 1 00399 // 00400 // vio_t dipVio = MAX32625PICO::VIO_1V8 or MAX32625PICO::VIO_IOH 00401 //~ MAX32625PICO::VIO_1V8, // 1.8V IO (local) 00402 MAX32625PICO::VIO_IOH, // Use VDDIOH (from DIP pin 1, or SWD pin1, or local 3.3V) 00403 // 00404 // vio_t swdVio 00405 //~ MAX32625PICO::VIO_1V8 // 1.8V IO (local) 00406 MAX32625PICO::VIO_IOH // Use VDDIOH (from DIP pin 1, or SWD pin1, or local 3.3V) 00407 ); 00408 //#define USE_LEDS 0 ? 00409 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 1 00410 #warning "TARGET_MAX32625PICO not previously tested; need to verify ADC_FULL_SCALE_VOLTAGE..." 00411 const float ADC_FULL_SCALE_VOLTAGE = 1.200; 00412 // 00413 //-------------------------------------------------- 00414 #elif defined(TARGET_NUCLEO_F446RE) || defined(TARGET_NUCLEO_F401RE) 00415 // TODO1: target NUCLEO_F446RE 00416 // 00417 // USER_BUTTON PC13 00418 // LED1 is shared with SPI_SCK on NUCLEO_F446RE PA_5, so don't use LED1. 00419 #define USE_LEDS 0 00420 // SPI spi(SPI_MOSI, SPI_MISO, SPI_SCK); 00421 // Serial serial(SERIAL_TX, SERIAL_RX); 00422 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 0 00423 const float ADC_FULL_SCALE_VOLTAGE = 3.300; // TODO: ADC_FULL_SCALE_VOLTAGE Pico? 00424 // 00425 //-------------------------------------------------- 00426 #elif defined(TARGET_LPC1768) 00427 //-------------------------------------------------- 00428 // TARGET=LPC1768 ARM Cortex-M3 100 MHz 512kB flash 64kB SRAM 00429 // +-------------[microUSB]-------------+ 00430 // ______ | [ ] GND +3.3V VOUT [ ] | ______ 00431 // ______ | [ ] 4.5V<VIN<9.0V +5.0V VU [ ] | ______ 00432 // ______ | [ ] VB USB.IF- [ ] | ______ 00433 // ______ | [ ] nR USB.IF+ [ ] | ______ 00434 // digitalInOut0 | [ ] p5 MOSI ETHERNET.RD- [ ] | ______ 00435 // digitalInOut1 | [ ] p6 MISO ETHERNET.RD+ [ ] | ______ 00436 // digitalInOut2 | [ ] p7 SCLK ETHERNET.TD- [ ] | ______ 00437 // digitalInOut3 | [ ] p8 ETHERNET.TD+ [ ] | ______ 00438 // digitalInOut4 | [ ] p9 TX SDA USB.D- [ ] | ______ 00439 // digitalInOut5 | [ ] p10 RX SCL USB.D+ [ ] | ______ 00440 // digitalInOut6 | [ ] p11 MOSI CAN-RD p30 [ ] | digitalInOut13 00441 // digitalInOut7 | [ ] p12 MISO CAN-TD p29 [ ] | digitalInOut12 00442 // digitalInOut8 | [ ] p13 TX SCLK SDA TX p28 [ ] | digitalInOut11 00443 // digitalInOut9 | [ ] p14 RX SCL RX p27 [ ] | digitalInOut10 00444 // analogIn0 | [ ] p15 AIN0 3.3Vfs PWM1 p26 [ ] | pwmDriver1 00445 // analogIn1 | [ ] p16 AIN1 3.3Vfs PWM2 p25 [ ] | pwmDriver2 00446 // analogIn2 | [ ] p17 AIN2 3.3Vfs PWM3 p24 [ ] | pwmDriver3 00447 // analogIn3 | [ ] p18 AIN3 AOUT PWM4 p23 [ ] | pwmDriver4 00448 // analogIn4 | [ ] p19 AIN4 3.3Vfs PWM5 p22 [ ] | pwmDriver5 00449 // analogIn5 | [ ] p20 AIN5 3.3Vfs PWM6 p21 [ ] | pwmDriver6 00450 // +------------------------------------+ 00451 // AIN6 = P0.3 = TGT_SBL_RXD? 00452 // AIN7 = P0.2 = TGT_SBL_TXD? 00453 // 00454 //-------------------------------------------------- 00455 // LPC1768 board uses VREF = 3.300V +A3,3V thru L1 to bypass capacitor C14 00456 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 0 00457 const float ADC_FULL_SCALE_VOLTAGE = 3.300; 00458 #else // not defined(TARGET_LPC1768 etc.) 00459 //-------------------------------------------------- 00460 // unknown target 00461 //-------------------------------------------------- 00462 #endif // target definition 00463 00464 00465 //-------------------------------------------------- 00466 // Option to dedicate SPI port pins 00467 // 00468 // SPI2_MOSI = P5_1 00469 // SPI2_MISO = P5_2 00470 // SPI2_SCK = P5_0 00471 // On this board I'm using P3_0 as spi_cs 00472 // SPI2_SS = P5_3 00473 // SPI2_SDIO2 = P5_4 00474 // SPI2_SDIO3 = P5_5 00475 // SPI2_SRN = P5_6 00476 // 00477 #ifndef HAS_SPI 00478 #define HAS_SPI 1 00479 #endif 00480 #if HAS_SPI 00481 #define SPI_MODE0 0 00482 #define SPI_MODE1 1 00483 #define SPI_MODE2 2 00484 #define SPI_MODE3 3 00485 // 00486 #if defined(TARGET_MAX32630) 00487 // Before setting global variables g_SPI_SCLK_Hz and g_SPI_dataMode, 00488 // workaround for TARGET_MAX32630 SPI_MODE2 SPI_MODE3 problem (issue #30) 00489 #warning "MAX32630 SPI workaround..." 00490 // replace SPI_MODE2 (CPOL=1,CPHA=0) with SPI_MODE1 (CPOL=0,CPHA=1) Falling Edge stable 00491 // replace SPI_MODE3 (CPOL=1,CPHA=1) with SPI_MODE0 (CPOL=0,CPHA=0) Rising Edge stable 00492 # if ((SPI_dataMode) == (SPI_MODE2)) 00493 #warning "MAX32630 SPI_MODE2 workaround, changing SPI_dataMode to SPI_MODE1..." 00494 // SPI_dataMode SPI_MODE2 // CPOL=1,CPHA=0: Falling Edge stable; SCLK idle High 00495 # undef SPI_dataMode 00496 # define SPI_dataMode SPI_MODE1 // CPOL=0,CPHA=1: Falling Edge stable; SCLK idle Low 00497 # elif ((SPI_dataMode) == (SPI_MODE3)) 00498 #warning "MAX32630 SPI_MODE3 workaround, changing SPI_dataMode to SPI_MODE0..." 00499 // SPI_dataMode SPI_MODE3 // CPOL=1,CPHA=1: Rising Edge stable; SCLK idle High 00500 # undef SPI_dataMode 00501 # define SPI_dataMode SPI_MODE0 // CPOL=0,CPHA=0: Rising Edge stable; SCLK idle Low 00502 # endif // workaround for TARGET_MAX32630 SPI_MODE2 SPI_MODE3 problem 00503 // workaround for TARGET_MAX32630 SPI_MODE2 SPI_MODE3 problem (issue #30) 00504 // limit SPI SCLK speed to 6MHz or less 00505 # if ((SPI_SCLK_Hz) > (6000000)) 00506 #warning "MAX32630 SPI speed workaround, changing SPI_SCLK_Hz to 6000000 or 6MHz..." 00507 # undef SPI_SCLK_Hz 00508 # define SPI_SCLK_Hz 6000000 // 6MHz 00509 # endif 00510 #endif 00511 // 00512 uint32_t g_SPI_SCLK_Hz = 12000000; // platform limit 12MHz intSPI_SCLK_Platform_Max_MHz * 1000000 00513 // TODO1: validate g_SPI_SCLK_Hz against system clock frequency SystemCoreClock F_CPU 00514 #if defined(TARGET_NUCLEO_F446RE) || defined(TARGET_NUCLEO_F401RE) 00515 // Nucleo SPI frequency isn't working quite as expected... 00516 // Looks like STMF4 has an spi clock prescaler (2,4,8,16,32,64,128,256) 00517 // so 180MHz->[90.0, 45.0, 22.5, 11.25, 5.625, 2.8125, 1.40625, 0.703125] 00518 // %SC SCLK=1MHz sets spi frequency 703.125kHz 00519 // %SC SCLK=2MHz sets spi frequency 1.40625MHz 00520 // %SC SCLK=3MHz sets spi frequency 2.8125MHz 00521 // %SC SCLK=6MHz sets spi frequency 5.625MHz 00522 // %SC SCLK=12MHz sets spi frequency 11.25MHz 00523 // %SC SCLK=23MHz sets spi frequency 22.5MHz 00524 // %SC SCLK=45MHz sets spi frequency 45.0MHz 00525 // Don't know why I can't reach spi frequency 90.0MHz, but ok whatever. 00526 const uint32_t limit_min_SPI_SCLK_divisor = 2; 00527 const uint32_t limit_max_SPI_SCLK_divisor = 256; 00528 // not really a divisor, just a powers-of-two prescaler with no intermediate divisors. 00529 #else 00530 const uint32_t limit_min_SPI_SCLK_divisor = 2; 00531 const uint32_t limit_max_SPI_SCLK_divisor = 8191; 00532 #endif 00533 const uint32_t limit_max_SPI_SCLK_Hz = (SystemCoreClock / limit_min_SPI_SCLK_divisor); // F_CPU / 2; // 8MHz / 2 = 4MHz 00534 const uint32_t limit_min_SPI_SCLK_Hz = (SystemCoreClock / limit_max_SPI_SCLK_divisor); // F_CPU / 128; // 8MHz / 128 = 62.5kHz 00535 // 00536 uint8_t g_SPI_dataMode = SPI_MODE0; // TODO: missing definition SPI_dataMode; 00537 uint8_t g_SPI_cs_state = 1; 00538 // 00539 #endif 00540 00541 00542 // uncrustify-0.66.1 *INDENT-OFF* 00543 //-------------------------------------------------- 00544 // Declare the DigitalInOut GPIO pins 00545 // Optional digitalInOut support. If there is only one it should be digitalInOut1. 00546 // D) Digital High/Low/Input Pin 00547 #if defined(TARGET_MAX32630) 00548 // +-------------[microUSB]-------------+ 00549 // | J1 MAX32630FTHR J2 | 00550 // | [ ] RST GND [ ] | 00551 // | [ ] 3V3 BAT+[ ] | 00552 // | [ ] 1V8 reset SW1 | 00553 // | [ ] GND J4 J3 | 00554 // | [ ] AIN_0 1.2Vfs (bat) SYS [ ] | 00555 // | [ ] AIN_1 1.2Vfs PWR [ ] | 00556 // | [ ] AIN_2 1.2Vfs +5V VBUS [ ] | 00557 // | [ ] AIN_3 1.2Vfs 1-WIRE P4_0 [ ] | dig9 00558 // dig10 | [x] P5_7 SDA2 SRN P5_6 [ ] | dig8 00559 // dig11 | [x] P6_0 SCL2 SDIO3 P5_5 [ ] | dig7 00560 // dig12 | [x] P5_0 SCLK SDIO2 P5_4 [ ] | dig6 00561 // dig13 | [x] P5_1 MOSI SSEL P5_3 [x] | dig5 00562 // dig14 | [ ] P5_2 MISO RTS P3_3 [ ] | dig4 00563 // dig15 | [ ] P3_0 RX CTS P3_2 [ ] | dig3 00564 // dig0 | [ ] P3_1 TX SCL P3_5 [x] | dig2 00565 // | [ ] GND SDA P3_4 [x] | dig1 00566 // +------------------------------------+ 00567 #define HAS_digitalInOut0 1 // P3_1 TARGET_MAX32630 J1.15 00568 #define HAS_digitalInOut1 1 // P3_4 TARGET_MAX32630 J3.12 00569 #define HAS_digitalInOut2 1 // P3_5 TARGET_MAX32630 J3.11 00570 #define HAS_digitalInOut3 1 // P3_2 TARGET_MAX32630 J3.10 00571 #define HAS_digitalInOut4 1 // P3_3 TARGET_MAX32630 J3.9 00572 #define HAS_digitalInOut5 1 // P5_3 TARGET_MAX32630 J3.8 00573 #define HAS_digitalInOut6 1 // P5_4 TARGET_MAX32630 J3.7 00574 #define HAS_digitalInOut7 1 // P5_5 TARGET_MAX32630 J3.6 00575 #define HAS_digitalInOut8 1 // P5_6 TARGET_MAX32630 J3.5 00576 #define HAS_digitalInOut9 1 // P4_0 TARGET_MAX32630 J3.4 00577 #if HAS_I2C 00578 // avoid resource conflict between P5_7, P6_0 I2C and DigitalInOut 00579 #define HAS_digitalInOut10 0 // P5_7 TARGET_MAX32630 J1.9 00580 #define HAS_digitalInOut11 0 // P6_0 TARGET_MAX32630 J1.10 00581 #else // HAS_I2C 00582 #define HAS_digitalInOut10 1 // P5_7 TARGET_MAX32630 J1.9 00583 #define HAS_digitalInOut11 1 // P6_0 TARGET_MAX32630 J1.10 00584 #endif // HAS_I2C 00585 #if HAS_SPI 00586 // avoid resource conflict between P5_0, P5_1, P5_2 SPI and DigitalInOut 00587 #define HAS_digitalInOut12 0 // P5_0 TARGET_MAX32630 J1.11 00588 #define HAS_digitalInOut13 0 // P5_1 TARGET_MAX32630 J1.12 00589 #define HAS_digitalInOut14 0 // P5_2 TARGET_MAX32630 J1.13 00590 #define HAS_digitalInOut15 0 // P3_0 TARGET_MAX32630 J1.14 00591 #else // HAS_SPI 00592 #define HAS_digitalInOut12 1 // P5_0 TARGET_MAX32630 J1.11 00593 #define HAS_digitalInOut13 1 // P5_1 TARGET_MAX32630 J1.12 00594 #define HAS_digitalInOut14 1 // P5_2 TARGET_MAX32630 J1.13 00595 #define HAS_digitalInOut15 1 // P3_0 TARGET_MAX32630 J1.14 00596 #endif // HAS_SPI 00597 #if HAS_digitalInOut0 00598 DigitalInOut digitalInOut0(P3_1, PIN_INPUT, PullUp, 1); // P3_1 TARGET_MAX32630 J1.15 00599 #endif 00600 #if HAS_digitalInOut1 00601 DigitalInOut digitalInOut1(P3_4, PIN_INPUT, PullUp, 1); // P3_4 TARGET_MAX32630 J3.12 00602 #endif 00603 #if HAS_digitalInOut2 00604 DigitalInOut digitalInOut2(P3_5, PIN_INPUT, PullUp, 1); // P3_5 TARGET_MAX32630 J3.11 00605 #endif 00606 #if HAS_digitalInOut3 00607 DigitalInOut digitalInOut3(P3_2, PIN_INPUT, PullUp, 1); // P3_2 TARGET_MAX32630 J3.10 00608 #endif 00609 #if HAS_digitalInOut4 00610 DigitalInOut digitalInOut4(P3_3, PIN_INPUT, PullUp, 1); // P3_3 TARGET_MAX32630 J3.9 00611 #endif 00612 #if HAS_digitalInOut5 00613 DigitalInOut digitalInOut5(P5_3, PIN_INPUT, PullUp, 1); // P5_3 TARGET_MAX32630 J3.8 00614 #endif 00615 #if HAS_digitalInOut6 00616 DigitalInOut digitalInOut6(P5_4, PIN_INPUT, PullUp, 1); // P5_4 TARGET_MAX32630 J3.7 00617 #endif 00618 #if HAS_digitalInOut7 00619 DigitalInOut digitalInOut7(P5_5, PIN_INPUT, PullUp, 1); // P5_5 TARGET_MAX32630 J3.6 00620 #endif 00621 #if HAS_digitalInOut8 00622 DigitalInOut digitalInOut8(P5_6, PIN_INPUT, PullUp, 1); // P5_6 TARGET_MAX32630 J3.5 00623 #endif 00624 #if HAS_digitalInOut9 00625 DigitalInOut digitalInOut9(P4_0, PIN_INPUT, PullUp, 1); // P4_0 TARGET_MAX32630 J3.4 00626 #endif 00627 #if HAS_digitalInOut10 00628 DigitalInOut digitalInOut10(P5_7, PIN_INPUT, PullUp, 1); // P5_7 TARGET_MAX32630 J1.9 00629 #endif 00630 #if HAS_digitalInOut11 00631 DigitalInOut digitalInOut11(P6_0, PIN_INPUT, PullUp, 1); // P6_0 TARGET_MAX32630 J1.10 00632 #endif 00633 #if HAS_digitalInOut12 00634 DigitalInOut digitalInOut12(P5_0, PIN_INPUT, PullUp, 1); // P5_0 TARGET_MAX32630 J1.11 00635 #endif 00636 #if HAS_digitalInOut13 00637 DigitalInOut digitalInOut13(P5_1, PIN_INPUT, PullUp, 1); // P5_1 TARGET_MAX32630 J1.12 00638 #endif 00639 #if HAS_digitalInOut14 00640 DigitalInOut digitalInOut14(P5_2, PIN_INPUT, PullUp, 1); // P5_2 TARGET_MAX32630 J1.13 00641 #endif 00642 #if HAS_digitalInOut15 00643 DigitalInOut digitalInOut15(P3_0, PIN_INPUT, PullUp, 1); // P3_0 TARGET_MAX32630 J1.14 00644 #endif 00645 //-------------------------------------------------- 00646 #elif defined(TARGET_MAX32625MBED) 00647 // TARGET=MAX32625MBED ARM Cortex-M4F 96MHz 512kB Flash 160kB SRAM 00648 // +-------------------------------------+ 00649 // | MAX32625MBED Arduino UNO header | 00650 // | | 00651 // | A5/SCL[ ] | P1_7 dig15 00652 // | A4/SDA[ ] | P1_6 dig14 00653 // | AREF=N/C[ ] | 00654 // | GND[ ] | 00655 // | [ ]N/C SCK/13[ ] | P1_0 dig13 00656 // | [ ]IOREF=3V3 MISO/12[ ] | P1_2 dig12 00657 // | [ ]RST MOSI/11[ ]~| P1_1 dig11 00658 // | [ ]3V3 CS/10[ ]~| P1_3 dig10 00659 // | [ ]5V0 9[ ]~| P1_5 dig9 00660 // | [ ]GND 8[ ] | P1_4 dig8 00661 // | [ ]GND | 00662 // | [ ]Vin 7[ ] | P0_7 dig7 00663 // | 6[ ]~| P0_6 dig6 00664 // AIN_0 | [ ]A0 5[ ]~| P0_5 dig5 00665 // AIN_1 | [ ]A1 4[ ] | P0_4 dig4 00666 // AIN_2 | [ ]A2 INT1/3[ ]~| P0_3 dig3 00667 // AIN_3 | [ ]A3 INT0/2[ ] | P0_2 dig2 00668 // dig16 P3_4 | [ ]A4/SDA RST SCK MISO TX>1[ ] | P0_1 dig1 00669 // dig17 P3_5 | [ ]A5/SCL [ ] [ ] [ ] RX<0[ ] | P0_0 dig0 00670 // | [ ] [ ] [ ] | 00671 // | UNO_R3 GND MOSI 5V ____________/ 00672 // \_______________________/ 00673 // 00674 #define HAS_digitalInOut0 1 // P0_0 TARGET_MAX32625MBED D0 00675 #define HAS_digitalInOut1 1 // P0_1 TARGET_MAX32625MBED D1 00676 #if APPLICATION_MAX11131 00677 #define HAS_digitalInOut2 0 // P0_2 TARGET_MAX32625MBED D2 -- MAX11131 EOC DigitalIn 00678 #else 00679 #define HAS_digitalInOut2 1 // P0_2 TARGET_MAX32625MBED D2 00680 #endif 00681 #define HAS_digitalInOut3 1 // P0_3 TARGET_MAX32625MBED D3 00682 #define HAS_digitalInOut4 1 // P0_4 TARGET_MAX32625MBED D4 00683 #define HAS_digitalInOut5 1 // P0_5 TARGET_MAX32625MBED D5 00684 #define HAS_digitalInOut6 1 // P0_6 TARGET_MAX32625MBED D6 00685 #define HAS_digitalInOut7 1 // P0_7 TARGET_MAX32625MBED D7 00686 #define HAS_digitalInOut8 1 // P1_4 TARGET_MAX32625MBED D8 00687 #if APPLICATION_MAX11131 00688 #define HAS_digitalInOut9 0 // P1_5 TARGET_MAX32625MBED D9 -- MAX11131 CNVST DigitalOut 00689 #else 00690 #define HAS_digitalInOut9 1 // P1_5 TARGET_MAX32625MBED D9 00691 #endif 00692 #if HAS_SPI 00693 // avoid resource conflict between P5_0, P5_1, P5_2 SPI and DigitalInOut 00694 #define HAS_digitalInOut10 0 // P1_3 TARGET_MAX32635MBED CS/10 00695 #define HAS_digitalInOut11 0 // P1_1 TARGET_MAX32635MBED MOSI/11 00696 #define HAS_digitalInOut12 0 // P1_2 TARGET_MAX32635MBED MISO/12 00697 #define HAS_digitalInOut13 0 // P1_0 TARGET_MAX32635MBED SCK/13 00698 #else // HAS_SPI 00699 #define HAS_digitalInOut10 1 // P1_3 TARGET_MAX32635MBED CS/10 00700 #define HAS_digitalInOut11 1 // P1_1 TARGET_MAX32635MBED MOSI/11 00701 #define HAS_digitalInOut12 1 // P1_2 TARGET_MAX32635MBED MISO/12 00702 #define HAS_digitalInOut13 1 // P1_0 TARGET_MAX32635MBED SCK/13 00703 #endif // HAS_SPI 00704 #if HAS_I2C 00705 // avoid resource conflict between P5_7, P6_0 I2C and DigitalInOut 00706 #define HAS_digitalInOut14 0 // P1_6 TARGET_MAX32635MBED A4/SDA (10pin digital connector) 00707 #define HAS_digitalInOut15 0 // P1_7 TARGET_MAX32635MBED A5/SCL (10pin digital connector) 00708 #define HAS_digitalInOut16 0 // P3_4 TARGET_MAX32635MBED A4/SDA (6pin analog connector) 00709 #define HAS_digitalInOut17 0 // P3_5 TARGET_MAX32635MBED A5/SCL (6pin analog connector) 00710 #else // HAS_I2C 00711 #define HAS_digitalInOut14 1 // P1_6 TARGET_MAX32635MBED A4/SDA (10pin digital connector) 00712 #define HAS_digitalInOut15 1 // P1_7 TARGET_MAX32635MBED A5/SCL (10pin digital connector) 00713 #define HAS_digitalInOut16 1 // P3_4 TARGET_MAX32635MBED A4/SDA (6pin analog connector) 00714 #define HAS_digitalInOut17 1 // P3_5 TARGET_MAX32635MBED A5/SCL (6pin analog connector) 00715 #endif // HAS_I2C 00716 #if HAS_digitalInOut0 00717 DigitalInOut digitalInOut0(P0_0, PIN_INPUT, PullUp, 1); // P0_0 TARGET_MAX32625MBED D0 00718 #endif 00719 #if HAS_digitalInOut1 00720 DigitalInOut digitalInOut1(P0_1, PIN_INPUT, PullUp, 1); // P0_1 TARGET_MAX32625MBED D1 00721 #endif 00722 #if HAS_digitalInOut2 00723 DigitalInOut digitalInOut2(P0_2, PIN_INPUT, PullUp, 1); // P0_2 TARGET_MAX32625MBED D2 00724 #endif 00725 #if HAS_digitalInOut3 00726 DigitalInOut digitalInOut3(P0_3, PIN_INPUT, PullUp, 1); // P0_3 TARGET_MAX32625MBED D3 00727 #endif 00728 #if HAS_digitalInOut4 00729 DigitalInOut digitalInOut4(P0_4, PIN_INPUT, PullUp, 1); // P0_4 TARGET_MAX32625MBED D4 00730 #endif 00731 #if HAS_digitalInOut5 00732 DigitalInOut digitalInOut5(P0_5, PIN_INPUT, PullUp, 1); // P0_5 TARGET_MAX32625MBED D5 00733 #endif 00734 #if HAS_digitalInOut6 00735 DigitalInOut digitalInOut6(P0_6, PIN_INPUT, PullUp, 1); // P0_6 TARGET_MAX32625MBED D6 00736 #endif 00737 #if HAS_digitalInOut7 00738 DigitalInOut digitalInOut7(P0_7, PIN_INPUT, PullUp, 1); // P0_7 TARGET_MAX32625MBED D7 00739 #endif 00740 #if HAS_digitalInOut8 00741 DigitalInOut digitalInOut8(P1_4, PIN_INPUT, PullUp, 1); // P1_4 TARGET_MAX32625MBED D8 00742 #endif 00743 #if HAS_digitalInOut9 00744 DigitalInOut digitalInOut9(P1_5, PIN_INPUT, PullUp, 1); // P1_5 TARGET_MAX32625MBED D9 00745 #endif 00746 #if HAS_digitalInOut10 00747 DigitalInOut digitalInOut10(P1_3, PIN_INPUT, PullUp, 1); // P1_3 TARGET_MAX32635MBED CS/10 00748 #endif 00749 #if HAS_digitalInOut11 00750 DigitalInOut digitalInOut11(P1_1, PIN_INPUT, PullUp, 1); // P1_1 TARGET_MAX32635MBED MOSI/11 00751 #endif 00752 #if HAS_digitalInOut12 00753 DigitalInOut digitalInOut12(P1_2, PIN_INPUT, PullUp, 1); // P1_2 TARGET_MAX32635MBED MISO/12 00754 #endif 00755 #if HAS_digitalInOut13 00756 DigitalInOut digitalInOut13(P1_0, PIN_INPUT, PullUp, 1); // P1_0 TARGET_MAX32635MBED SCK/13 00757 #endif 00758 #if HAS_digitalInOut14 00759 // Ensure that the unused I2C pins do not interfere with analog inputs A4 and A5 00760 // DigitalInOut mode can be one of PullUp, PullDown, PullNone, OpenDrain 00761 DigitalInOut digitalInOut14(P1_6, PIN_INPUT, OpenDrain, 1); // P1_6 TARGET_MAX32635MBED A4/SDA (10pin digital connector) 00762 #endif 00763 #if HAS_digitalInOut15 00764 // Ensure that the unused I2C pins do not interfere with analog inputs A4 and A5 00765 DigitalInOut digitalInOut15(P1_7, PIN_INPUT, OpenDrain, 1); // P1_7 TARGET_MAX32635MBED A5/SCL (10pin digital connector) 00766 #endif 00767 #if HAS_digitalInOut16 00768 // Ensure that the unused I2C pins do not interfere with analog inputs A4 and A5 00769 // DigitalInOut mode can be one of PullUp, PullDown, PullNone, OpenDrain 00770 // PullUp-->3.4V, PullDown-->1.7V, PullNone-->3.5V, OpenDrain-->0.00V 00771 DigitalInOut digitalInOut16(P3_4, PIN_INPUT, OpenDrain, 0); // P3_4 TARGET_MAX32635MBED A4/SDA (6pin analog connector) 00772 #endif 00773 #if HAS_digitalInOut17 00774 // Ensure that the unused I2C pins do not interfere with analog inputs A4 and A5 00775 DigitalInOut digitalInOut17(P3_5, PIN_INPUT, OpenDrain, 0); // P3_5 TARGET_MAX32635MBED A5/SCL (6pin analog connector) 00776 #endif 00777 //-------------------------------------------------- 00778 #elif defined(TARGET_NUCLEO_F446RE) || defined(TARGET_NUCLEO_F401RE) 00779 #define HAS_digitalInOut0 0 00780 #define HAS_digitalInOut1 0 00781 #if APPLICATION_MAX11131 00782 // D2 -- MAX11131 EOC DigitalIn 00783 #define HAS_digitalInOut2 0 00784 #else 00785 #define HAS_digitalInOut2 1 00786 #endif 00787 #define HAS_digitalInOut3 1 00788 #define HAS_digitalInOut4 1 00789 #define HAS_digitalInOut5 1 00790 #define HAS_digitalInOut6 1 00791 #define HAS_digitalInOut7 1 00792 #if APPLICATION_MAX5715 00793 // D8 -- MAX5715 CLRb DigitalOut 00794 #define HAS_digitalInOut8 0 00795 #else 00796 #define HAS_digitalInOut8 1 00797 #endif 00798 #if APPLICATION_MAX5715 00799 // D9 -- MAX5715 LDACb DigitalOut 00800 #define HAS_digitalInOut9 0 00801 #elif APPLICATION_MAX11131 00802 // D9 -- MAX11131 CNVST DigitalOut 00803 #define HAS_digitalInOut9 0 00804 #else 00805 #define HAS_digitalInOut9 1 00806 #endif 00807 #if HAS_SPI 00808 // avoid resource conflict between P5_0, P5_1, P5_2 SPI and DigitalInOut 00809 // Arduino digital pin D10 SPI function is CS/10 00810 // Arduino digital pin D11 SPI function is MOSI/11 00811 // Arduino digital pin D12 SPI function is MISO/12 00812 // Arduino digital pin D13 SPI function is SCK/13 00813 #define HAS_digitalInOut10 0 00814 #define HAS_digitalInOut11 0 00815 #define HAS_digitalInOut12 0 00816 #define HAS_digitalInOut13 0 00817 #else // HAS_SPI 00818 #define HAS_digitalInOut10 1 00819 #define HAS_digitalInOut11 1 00820 #define HAS_digitalInOut12 1 00821 #define HAS_digitalInOut13 1 00822 #endif // HAS_SPI 00823 #if HAS_I2C 00824 // avoid resource conflict between P5_7, P6_0 I2C and DigitalInOut 00825 // Arduino digital pin D14 I2C function is A4/SDA (10pin digital connector) 00826 // Arduino digital pin D15 I2C function is A5/SCL (10pin digital connector) 00827 // Arduino digital pin D16 I2C function is A4/SDA (6pin analog connector) 00828 // Arduino digital pin D17 I2C function is A5/SCL (6pin analog connector) 00829 #define HAS_digitalInOut14 0 00830 #define HAS_digitalInOut15 0 00831 #define HAS_digitalInOut16 0 00832 #define HAS_digitalInOut17 0 00833 #else // HAS_I2C 00834 #define HAS_digitalInOut14 1 00835 #define HAS_digitalInOut15 1 00836 #define HAS_digitalInOut16 0 00837 #define HAS_digitalInOut17 0 00838 #endif // HAS_I2C 00839 #if HAS_digitalInOut0 00840 DigitalInOut digitalInOut0(D0, PIN_INPUT, PullUp, 1); 00841 #endif 00842 #if HAS_digitalInOut1 00843 DigitalInOut digitalInOut1(D1, PIN_INPUT, PullUp, 1); 00844 #endif 00845 #if HAS_digitalInOut2 00846 DigitalInOut digitalInOut2(D2, PIN_INPUT, PullUp, 1); 00847 #endif 00848 #if HAS_digitalInOut3 00849 DigitalInOut digitalInOut3(D3, PIN_INPUT, PullUp, 1); 00850 #endif 00851 #if HAS_digitalInOut4 00852 DigitalInOut digitalInOut4(D4, PIN_INPUT, PullUp, 1); 00853 #endif 00854 #if HAS_digitalInOut5 00855 DigitalInOut digitalInOut5(D5, PIN_INPUT, PullUp, 1); 00856 #endif 00857 #if HAS_digitalInOut6 00858 DigitalInOut digitalInOut6(D6, PIN_INPUT, PullUp, 1); 00859 #endif 00860 #if HAS_digitalInOut7 00861 DigitalInOut digitalInOut7(D7, PIN_INPUT, PullUp, 1); 00862 #endif 00863 #if HAS_digitalInOut8 00864 DigitalInOut digitalInOut8(D8, PIN_INPUT, PullUp, 1); 00865 #endif 00866 #if HAS_digitalInOut9 00867 DigitalInOut digitalInOut9(D9, PIN_INPUT, PullUp, 1); 00868 #endif 00869 #if HAS_digitalInOut10 00870 // Arduino digital pin D10 SPI function is CS/10 00871 DigitalInOut digitalInOut10(D10, PIN_INPUT, PullUp, 1); 00872 #endif 00873 #if HAS_digitalInOut11 00874 // Arduino digital pin D11 SPI function is MOSI/11 00875 DigitalInOut digitalInOut11(D11, PIN_INPUT, PullUp, 1); 00876 #endif 00877 #if HAS_digitalInOut12 00878 // Arduino digital pin D12 SPI function is MISO/12 00879 DigitalInOut digitalInOut12(D12, PIN_INPUT, PullUp, 1); 00880 #endif 00881 #if HAS_digitalInOut13 00882 // Arduino digital pin D13 SPI function is SCK/13 00883 DigitalInOut digitalInOut13(D13, PIN_INPUT, PullUp, 1); 00884 #endif 00885 #if HAS_digitalInOut14 00886 // Arduino digital pin D14 I2C function is A4/SDA (10pin digital connector) 00887 DigitalInOut digitalInOut14(D14, PIN_INPUT, PullUp, 1); 00888 #endif 00889 #if HAS_digitalInOut15 00890 // Arduino digital pin D15 I2C function is A5/SCL (10pin digital connector) 00891 DigitalInOut digitalInOut15(D15, PIN_INPUT, PullUp, 1); 00892 #endif 00893 #if HAS_digitalInOut16 00894 // Arduino digital pin D16 I2C function is A4/SDA (6pin analog connector) 00895 DigitalInOut digitalInOut16(D16, PIN_INPUT, PullUp, 1); 00896 #endif 00897 #if HAS_digitalInOut17 00898 // Arduino digital pin D17 I2C function is A5/SCL (6pin analog connector) 00899 DigitalInOut digitalInOut17(D17, PIN_INPUT, PullUp, 1); 00900 #endif 00901 //-------------------------------------------------- 00902 #elif defined(TARGET_LPC1768) 00903 #define HAS_digitalInOut0 1 00904 #define HAS_digitalInOut1 1 00905 #define HAS_digitalInOut2 1 00906 #define HAS_digitalInOut3 1 00907 #define HAS_digitalInOut4 1 00908 #define HAS_digitalInOut5 1 00909 #define HAS_digitalInOut6 1 00910 #define HAS_digitalInOut7 1 00911 #define HAS_digitalInOut8 1 00912 #define HAS_digitalInOut9 1 00913 // #define HAS_digitalInOut10 1 00914 // #define HAS_digitalInOut11 1 00915 // #define HAS_digitalInOut12 1 00916 // #define HAS_digitalInOut13 1 00917 // #define HAS_digitalInOut14 1 00918 // #define HAS_digitalInOut15 1 00919 #if HAS_digitalInOut0 00920 DigitalInOut digitalInOut0(p5, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.9/I2STX_SDA/MOSI1/MAT2.3 00921 #endif 00922 #if HAS_digitalInOut1 00923 DigitalInOut digitalInOut1(p6, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.8/I2STX_WS/MISO1/MAT2.2 00924 #endif 00925 #if HAS_digitalInOut2 00926 DigitalInOut digitalInOut2(p7, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.7/I2STX_CLK/SCK1/MAT2.1 00927 #endif 00928 #if HAS_digitalInOut3 00929 DigitalInOut digitalInOut3(p8, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.6/I2SRX_SDA/SSEL1/MAT2.0 00930 #endif 00931 #if HAS_digitalInOut4 00932 DigitalInOut digitalInOut4(p9, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.0/CAN_RX1/TXD3/SDA1 00933 #endif 00934 #if HAS_digitalInOut5 00935 DigitalInOut digitalInOut5(p10, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.1/CAN_TX1/RXD3/SCL1 00936 #endif 00937 #if HAS_digitalInOut6 00938 DigitalInOut digitalInOut6(p11, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.18/DCD1/MOSI0/MOSI1 00939 #endif 00940 #if HAS_digitalInOut7 00941 DigitalInOut digitalInOut7(p12, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.17/CTS1/MISO0/MISO 00942 #endif 00943 #if HAS_digitalInOut8 00944 DigitalInOut digitalInOut8(p13, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.15/TXD1/SCK0/SCK 00945 #endif 00946 #if HAS_digitalInOut9 00947 DigitalInOut digitalInOut9(p14, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.16/RXD1/SSEL0/SSEL 00948 #endif 00949 // 00950 // these pins support analog input analogIn0 .. analogIn5 00951 //DigitalInOut digitalInOut_(p15, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.23/AD0.0/I2SRX_CLK/CAP3.0 00952 //DigitalInOut digitalInOut_(p16, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.24/AD0.1/I2SRX_WS/CAP3.1 00953 //DigitalInOut digitalInOut_(p17, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.25/AD0.2/I2SRX_SDA/TXD3 00954 //DigitalInOut digitalInOut_(p18, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.26/AD0.3/AOUT/RXD3 00955 //DigitalInOut digitalInOut_(p19, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P1.30/VBUS/AD0.4 00956 //DigitalInOut digitalInOut_(p20, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P1.31/SCK1/AD0.5 00957 // 00958 // these pins support PWM pwmDriver1 .. pwmDriver6 00959 //DigitalInOut digitalInOut_(p21, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P2.5/PWM1.6/DTR1/TRACEDATA0 00960 //DigitalInOut digitalInOut_(p22, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P2.4/PWM1.5/DSR1/TRACEDATA1 00961 //DigitalInOut digitalInOut_(p23, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P2.3/PWM1.4/DCD1/TRACEDATA2 00962 //DigitalInOut digitalInOut_(p24, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P2.2/PWM1.3/CTS1/TRACEDATA3 00963 //DigitalInOut digitalInOut_(p25, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P2.1/PWM1.2/RXD1 00964 //DigitalInOut digitalInOut_(p26, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P2.0/PWM1.1/TXD1/TRACECLK 00965 // 00966 // these could be additional digitalInOut pins 00967 #if HAS_digitalInOut10 00968 DigitalInOut digitalInOut10(p27, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.11/RXD2/SCL2/MAT3.1 00969 #endif 00970 #if HAS_digitalInOut11 00971 DigitalInOut digitalInOut11(p28, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.10/TXD2/SDA2/MAT3.0 00972 #endif 00973 #if HAS_digitalInOut12 00974 DigitalInOut digitalInOut12(p29, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.5/I2SRX_WS/CAN_TX2/CAP2.1 00975 #endif 00976 #if HAS_digitalInOut13 00977 DigitalInOut digitalInOut13(p30, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.4/I2SRX_CLK/CAN_RX2/CAP2.0 00978 #endif 00979 #if HAS_digitalInOut14 00980 DigitalInOut digitalInOut14(___, PIN_INPUT, PullUp, 1); 00981 #endif 00982 #if HAS_digitalInOut15 00983 DigitalInOut digitalInOut15(___, PIN_INPUT, PullUp, 1); 00984 #endif 00985 #else 00986 // unknown target 00987 #endif 00988 // uncrustify-0.66.1 *INDENT-ON* 00989 #if HAS_digitalInOut0 || HAS_digitalInOut1 \ 00990 || HAS_digitalInOut2 || HAS_digitalInOut3 \ 00991 || HAS_digitalInOut4 || HAS_digitalInOut5 \ 00992 || HAS_digitalInOut6 || HAS_digitalInOut7 \ 00993 || HAS_digitalInOut8 || HAS_digitalInOut9 \ 00994 || HAS_digitalInOut10 || HAS_digitalInOut11 \ 00995 || HAS_digitalInOut12 || HAS_digitalInOut13 \ 00996 || HAS_digitalInOut14 || HAS_digitalInOut15 \ 00997 || HAS_digitalInOut16 || HAS_digitalInOut17 00998 #define HAS_digitalInOuts 1 00999 #else 01000 #warning "Note: There are no digitalInOut resources defined" 01001 #endif 01002 01003 // uncrustify-0.66.1 *INDENT-OFF* 01004 //-------------------------------------------------- 01005 // Declare the AnalogIn driver 01006 // Optional analogIn support. If there is only one it should be analogIn1. 01007 // A) analog input 01008 #if defined(TARGET_MAX32630) 01009 #define HAS_analogIn0 1 01010 #define HAS_analogIn1 1 01011 #define HAS_analogIn2 1 01012 #define HAS_analogIn3 1 01013 #define HAS_analogIn4 1 01014 #define HAS_analogIn5 1 01015 #define HAS_analogIn6 1 01016 #define HAS_analogIn7 1 01017 #define HAS_analogIn8 1 01018 #define HAS_analogIn9 1 01019 // #define HAS_analogIn10 0 01020 // #define HAS_analogIn11 0 01021 // #define HAS_analogIn12 0 01022 // #define HAS_analogIn13 0 01023 // #define HAS_analogIn14 0 01024 // #define HAS_analogIn15 0 01025 #if HAS_analogIn0 01026 AnalogIn analogIn0(AIN_0); // TARGET_MAX32630 J1.5 AIN_0 = AIN0 pin fullscale is 1.2V 01027 #endif 01028 #if HAS_analogIn1 01029 AnalogIn analogIn1(AIN_1); // TARGET_MAX32630 J1.6 AIN_1 = AIN1 pin fullscale is 1.2V 01030 #endif 01031 #if HAS_analogIn2 01032 AnalogIn analogIn2(AIN_2); // TARGET_MAX32630 J1.7 AIN_2 = AIN2 pin fullscale is 1.2V 01033 #endif 01034 #if HAS_analogIn3 01035 AnalogIn analogIn3(AIN_3); // TARGET_MAX32630 J1.8 AIN_3 = AIN3 pin fullscale is 1.2V 01036 #endif 01037 #if HAS_analogIn4 01038 AnalogIn analogIn4(AIN_4); // TARGET_MAX32630 J1.5 AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01039 #endif 01040 #if HAS_analogIn5 01041 AnalogIn analogIn5(AIN_5); // TARGET_MAX32630 J1.6 AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01042 #endif 01043 #if HAS_analogIn6 01044 AnalogIn analogIn6(AIN_6); // TARGET_MAX32630 AIN_6 = VDDB / 4.0 fullscale is 4.8V 01045 #endif 01046 #if HAS_analogIn7 01047 AnalogIn analogIn7(AIN_7); // TARGET_MAX32630 AIN_7 = VDD18 fullscale is 1.2V 01048 #endif 01049 #if HAS_analogIn8 01050 AnalogIn analogIn8(AIN_8); // TARGET_MAX32630 AIN_8 = VDD12 fullscale is 1.2V 01051 #endif 01052 #if HAS_analogIn9 01053 AnalogIn analogIn9(AIN_9); // TARGET_MAX32630 AIN_9 = VRTC / 2.0 fullscale is 2.4V 01054 #endif 01055 #if HAS_analogIn10 01056 AnalogIn analogIn10(____); // TARGET_MAX32630 AIN_10 = x undefined? 01057 #endif 01058 #if HAS_analogIn11 01059 AnalogIn analogIn11(____); // TARGET_MAX32630 AIN_11 = VDDIO / 4.0 fullscale is 4.8V 01060 #endif 01061 #if HAS_analogIn12 01062 AnalogIn analogIn12(____); // TARGET_MAX32630 AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 01063 #endif 01064 #if HAS_analogIn13 01065 AnalogIn analogIn13(____); 01066 #endif 01067 #if HAS_analogIn14 01068 AnalogIn analogIn14(____); 01069 #endif 01070 #if HAS_analogIn15 01071 AnalogIn analogIn15(____); 01072 #endif 01073 //-------------------------------------------------- 01074 #elif defined(TARGET_MAX32625MBED) 01075 #define HAS_analogIn0 1 01076 #define HAS_analogIn1 1 01077 #define HAS_analogIn2 1 01078 #define HAS_analogIn3 1 01079 #define HAS_analogIn4 1 01080 #define HAS_analogIn5 1 01081 #if HAS_analogIn0 01082 AnalogIn analogIn0(AIN_0); // TARGET_MAX32630 J1.5 AIN_0 = AIN0 pin fullscale is 1.2V 01083 #endif 01084 #if HAS_analogIn1 01085 AnalogIn analogIn1(AIN_1); // TARGET_MAX32630 J1.6 AIN_1 = AIN1 pin fullscale is 1.2V 01086 #endif 01087 #if HAS_analogIn2 01088 AnalogIn analogIn2(AIN_2); // TARGET_MAX32630 J1.7 AIN_2 = AIN2 pin fullscale is 1.2V 01089 #endif 01090 #if HAS_analogIn3 01091 AnalogIn analogIn3(AIN_3); // TARGET_MAX32630 J1.8 AIN_3 = AIN3 pin fullscale is 1.2V 01092 #endif 01093 #if HAS_analogIn4 01094 AnalogIn analogIn4(AIN_4); // TARGET_MAX32630 J1.5 AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01095 #endif 01096 #if HAS_analogIn5 01097 AnalogIn analogIn5(AIN_5); // TARGET_MAX32630 J1.6 AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01098 #endif 01099 //-------------------------------------------------- 01100 #elif defined(TARGET_MAX32620FTHR) 01101 #warning "TARGET_MAX32620FTHR not previously tested; need to verify analogIn0..." 01102 #define HAS_analogIn0 1 01103 #define HAS_analogIn1 1 01104 #define HAS_analogIn2 1 01105 #define HAS_analogIn3 1 01106 #define HAS_analogIn4 1 01107 #define HAS_analogIn5 1 01108 #define HAS_analogIn6 1 01109 #define HAS_analogIn7 1 01110 #define HAS_analogIn8 1 01111 #define HAS_analogIn9 1 01112 // #define HAS_analogIn10 0 01113 // #define HAS_analogIn11 0 01114 // #define HAS_analogIn12 0 01115 // #define HAS_analogIn13 0 01116 // #define HAS_analogIn14 0 01117 // #define HAS_analogIn15 0 01118 #if HAS_analogIn0 01119 AnalogIn analogIn0(AIN_0); // TARGET_MAX32620FTHR J1.5 AIN_0 = AIN0 pin fullscale is 1.2V 01120 #endif 01121 #if HAS_analogIn1 01122 AnalogIn analogIn1(AIN_1); // TARGET_MAX32620FTHR J1.6 AIN_1 = AIN1 pin fullscale is 1.2V 01123 #endif 01124 #if HAS_analogIn2 01125 AnalogIn analogIn2(AIN_2); // TARGET_MAX32620FTHR J1.7 AIN_2 = AIN2 pin fullscale is 1.2V 01126 #endif 01127 #if HAS_analogIn3 01128 AnalogIn analogIn3(AIN_3); // TARGET_MAX32620FTHR J1.8 AIN_3 = AIN3 pin fullscale is 1.2V 01129 #endif 01130 #if HAS_analogIn4 01131 AnalogIn analogIn4(AIN_4); // TARGET_MAX32620FTHR J1.5 AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01132 #endif 01133 #if HAS_analogIn5 01134 AnalogIn analogIn5(AIN_5); // TARGET_MAX32620FTHR J1.6 AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01135 #endif 01136 #if HAS_analogIn6 01137 AnalogIn analogIn6(AIN_6); // TARGET_MAX32620FTHR AIN_6 = VDDB / 4.0 fullscale is 4.8V 01138 #endif 01139 #if HAS_analogIn7 01140 AnalogIn analogIn7(AIN_7); // TARGET_MAX32620FTHR AIN_7 = VDD18 fullscale is 1.2V 01141 #endif 01142 #if HAS_analogIn8 01143 AnalogIn analogIn8(AIN_8); // TARGET_MAX32620FTHR AIN_8 = VDD12 fullscale is 1.2V 01144 #endif 01145 #if HAS_analogIn9 01146 AnalogIn analogIn9(AIN_9); // TARGET_MAX32620FTHR AIN_9 = VRTC / 2.0 fullscale is 2.4V 01147 #endif 01148 #if HAS_analogIn10 01149 AnalogIn analogIn10(____); // TARGET_MAX32620FTHR AIN_10 = x undefined? 01150 #endif 01151 #if HAS_analogIn11 01152 AnalogIn analogIn11(____); // TARGET_MAX32620FTHR AIN_11 = VDDIO / 4.0 fullscale is 4.8V 01153 #endif 01154 #if HAS_analogIn12 01155 AnalogIn analogIn12(____); // TARGET_MAX32620FTHR AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 01156 #endif 01157 #if HAS_analogIn13 01158 AnalogIn analogIn13(____); 01159 #endif 01160 #if HAS_analogIn14 01161 AnalogIn analogIn14(____); 01162 #endif 01163 #if HAS_analogIn15 01164 AnalogIn analogIn15(____); 01165 #endif 01166 //-------------------------------------------------- 01167 #elif defined(TARGET_MAX32625PICO) 01168 #warning "TARGET_MAX32625PICO not previously tested; need to verify analogIn0..." 01169 #define HAS_analogIn0 1 01170 #define HAS_analogIn1 1 01171 #define HAS_analogIn2 1 01172 #define HAS_analogIn3 1 01173 #define HAS_analogIn4 1 01174 #define HAS_analogIn5 1 01175 #if HAS_analogIn0 01176 AnalogIn analogIn0(AIN_0); // TARGET_MAX32630 J1.5 AIN_0 = AIN0 pin fullscale is 1.2V 01177 #endif 01178 #if HAS_analogIn1 01179 AnalogIn analogIn1(AIN_1); // TARGET_MAX32630 J1.6 AIN_1 = AIN1 pin fullscale is 1.2V 01180 #endif 01181 #if HAS_analogIn2 01182 AnalogIn analogIn2(AIN_2); // TARGET_MAX32630 J1.7 AIN_2 = AIN2 pin fullscale is 1.2V 01183 #endif 01184 #if HAS_analogIn3 01185 AnalogIn analogIn3(AIN_3); // TARGET_MAX32630 J1.8 AIN_3 = AIN3 pin fullscale is 1.2V 01186 #endif 01187 #if HAS_analogIn4 01188 AnalogIn analogIn4(AIN_4); // TARGET_MAX32630 J1.5 AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01189 #endif 01190 #if HAS_analogIn5 01191 AnalogIn analogIn5(AIN_5); // TARGET_MAX32630 J1.6 AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01192 #endif 01193 //-------------------------------------------------- 01194 #elif defined(TARGET_MAX32600) 01195 #define HAS_analogIn0 1 01196 #define HAS_analogIn1 1 01197 #define HAS_analogIn2 1 01198 #define HAS_analogIn3 1 01199 #define HAS_analogIn4 1 01200 #define HAS_analogIn5 1 01201 #if HAS_analogIn0 01202 AnalogIn analogIn0(A0); 01203 #endif 01204 #if HAS_analogIn1 01205 AnalogIn analogIn1(A1); 01206 #endif 01207 #if HAS_analogIn2 01208 AnalogIn analogIn2(A2); 01209 #endif 01210 #if HAS_analogIn3 01211 AnalogIn analogIn3(A3); 01212 #endif 01213 #if HAS_analogIn4 01214 AnalogIn analogIn4(A4); 01215 #endif 01216 #if HAS_analogIn5 01217 AnalogIn analogIn5(A5); 01218 #endif 01219 //-------------------------------------------------- 01220 #elif defined(TARGET_NUCLEO_F446RE) 01221 #define HAS_analogIn0 1 01222 #define HAS_analogIn1 1 01223 #define HAS_analogIn2 1 01224 #define HAS_analogIn3 1 01225 #define HAS_analogIn4 1 01226 #define HAS_analogIn5 1 01227 #if HAS_analogIn0 01228 AnalogIn analogIn0(A0); 01229 #endif 01230 #if HAS_analogIn1 01231 AnalogIn analogIn1(A1); 01232 #endif 01233 #if HAS_analogIn2 01234 AnalogIn analogIn2(A2); 01235 #endif 01236 #if HAS_analogIn3 01237 AnalogIn analogIn3(A3); 01238 #endif 01239 #if HAS_analogIn4 01240 AnalogIn analogIn4(A4); 01241 #endif 01242 #if HAS_analogIn5 01243 AnalogIn analogIn5(A5); 01244 #endif 01245 //-------------------------------------------------- 01246 #elif defined(TARGET_NUCLEO_F401RE) 01247 #define HAS_analogIn0 1 01248 #define HAS_analogIn1 1 01249 #define HAS_analogIn2 1 01250 #define HAS_analogIn3 1 01251 #define HAS_analogIn4 1 01252 #define HAS_analogIn5 1 01253 #if HAS_analogIn0 01254 AnalogIn analogIn0(A0); 01255 #endif 01256 #if HAS_analogIn1 01257 AnalogIn analogIn1(A1); 01258 #endif 01259 #if HAS_analogIn2 01260 AnalogIn analogIn2(A2); 01261 #endif 01262 #if HAS_analogIn3 01263 AnalogIn analogIn3(A3); 01264 #endif 01265 #if HAS_analogIn4 01266 AnalogIn analogIn4(A4); 01267 #endif 01268 #if HAS_analogIn5 01269 AnalogIn analogIn5(A5); 01270 #endif 01271 //-------------------------------------------------- 01272 // TODO1: TARGET=MAX32625MBED ARM Cortex-M4F 96MHz 512kB Flash 160kB SRAM 01273 #elif defined(TARGET_LPC1768) 01274 #define HAS_analogIn0 1 01275 #define HAS_analogIn1 1 01276 #define HAS_analogIn2 1 01277 #define HAS_analogIn3 1 01278 #define HAS_analogIn4 1 01279 #define HAS_analogIn5 1 01280 // #define HAS_analogIn6 1 01281 // #define HAS_analogIn7 1 01282 // #define HAS_analogIn8 1 01283 // #define HAS_analogIn9 1 01284 // #define HAS_analogIn10 1 01285 // #define HAS_analogIn11 1 01286 // #define HAS_analogIn12 1 01287 // #define HAS_analogIn13 1 01288 // #define HAS_analogIn14 1 01289 // #define HAS_analogIn15 1 01290 #if HAS_analogIn0 01291 AnalogIn analogIn0(p15); // TARGET_LPC1768 P0.23/AD0.0/I2SRX_CLK/CAP3.0 01292 #endif 01293 #if HAS_analogIn1 01294 AnalogIn analogIn1(p16); // TARGET_LPC1768 P0.24/AD0.1/I2SRX_WS/CAP3.1 01295 #endif 01296 #if HAS_analogIn2 01297 AnalogIn analogIn2(p17); // TARGET_LPC1768 P0.25/AD0.2/I2SRX_SDA/TXD3 01298 #endif 01299 #if HAS_analogIn3 01300 AnalogIn analogIn3(p18); // TARGET_LPC1768 P0.26/AD0.3/AOUT/RXD3 01301 #endif 01302 #if HAS_analogIn4 01303 AnalogIn analogIn4(p19); // TARGET_LPC1768 P1.30/VBUS/AD0.4 01304 #endif 01305 #if HAS_analogIn5 01306 AnalogIn analogIn5(p20); // TARGET_LPC1768 P1.31/SCK1/AD0.5 01307 #endif 01308 #if HAS_analogIn6 01309 AnalogIn analogIn6(____); 01310 #endif 01311 #if HAS_analogIn7 01312 AnalogIn analogIn7(____); 01313 #endif 01314 #if HAS_analogIn8 01315 AnalogIn analogIn8(____); 01316 #endif 01317 #if HAS_analogIn9 01318 AnalogIn analogIn9(____); 01319 #endif 01320 #if HAS_analogIn10 01321 AnalogIn analogIn10(____); 01322 #endif 01323 #if HAS_analogIn11 01324 AnalogIn analogIn11(____); 01325 #endif 01326 #if HAS_analogIn12 01327 AnalogIn analogIn12(____); 01328 #endif 01329 #if HAS_analogIn13 01330 AnalogIn analogIn13(____); 01331 #endif 01332 #if HAS_analogIn14 01333 AnalogIn analogIn14(____); 01334 #endif 01335 #if HAS_analogIn15 01336 AnalogIn analogIn15(____); 01337 #endif 01338 #else 01339 // unknown target 01340 #endif 01341 // uncrustify-0.66.1 *INDENT-ON* 01342 #if HAS_analogIn0 || HAS_analogIn1 \ 01343 || HAS_analogIn2 || HAS_analogIn3 \ 01344 || HAS_analogIn4 || HAS_analogIn5 \ 01345 || HAS_analogIn6 || HAS_analogIn7 \ 01346 || HAS_analogIn8 || HAS_analogIn9 \ 01347 || HAS_analogIn10 || HAS_analogIn11 \ 01348 || HAS_analogIn12 || HAS_analogIn13 \ 01349 || HAS_analogIn14 || HAS_analogIn15 01350 #define HAS_analogIns 1 01351 #else 01352 #warning "Note: There are no analogIn resources defined" 01353 #endif 01354 01355 // DigitalInOut pin resource: print the pin index names to serial 01356 #if HAS_digitalInOuts 01357 void list_digitalInOutPins(Stream& serialStream) 01358 { 01359 #if HAS_digitalInOut0 01360 serialStream.printf(" 0"); 01361 #endif 01362 #if HAS_digitalInOut1 01363 serialStream.printf(" 1"); 01364 #endif 01365 #if HAS_digitalInOut2 01366 serialStream.printf(" 2"); 01367 #endif 01368 #if HAS_digitalInOut3 01369 serialStream.printf(" 3"); 01370 #endif 01371 #if HAS_digitalInOut4 01372 serialStream.printf(" 4"); 01373 #endif 01374 #if HAS_digitalInOut5 01375 serialStream.printf(" 5"); 01376 #endif 01377 #if HAS_digitalInOut6 01378 serialStream.printf(" 6"); 01379 #endif 01380 #if HAS_digitalInOut7 01381 serialStream.printf(" 7"); 01382 #endif 01383 #if HAS_digitalInOut8 01384 serialStream.printf(" 8"); 01385 #endif 01386 #if HAS_digitalInOut9 01387 serialStream.printf(" 9"); 01388 #endif 01389 #if HAS_digitalInOut10 01390 serialStream.printf(" 10"); 01391 #endif 01392 #if HAS_digitalInOut11 01393 serialStream.printf(" 11"); 01394 #endif 01395 #if HAS_digitalInOut12 01396 serialStream.printf(" 12"); 01397 #endif 01398 #if HAS_digitalInOut13 01399 serialStream.printf(" 13"); 01400 #endif 01401 #if HAS_digitalInOut14 01402 serialStream.printf(" 14"); 01403 #endif 01404 #if HAS_digitalInOut15 01405 serialStream.printf(" 15"); 01406 #endif 01407 #if HAS_digitalInOut16 01408 serialStream.printf(" 16"); 01409 #endif 01410 #if HAS_digitalInOut17 01411 serialStream.printf(" 17"); 01412 #endif 01413 } 01414 #endif 01415 01416 01417 // DigitalInOut pin resource: search index 01418 #if HAS_digitalInOuts 01419 DigitalInOut& find_digitalInOutPin(int cPinIndex) 01420 { 01421 switch (cPinIndex) 01422 { 01423 default: // default to the first defined digitalInOut pin 01424 #if HAS_digitalInOut0 01425 case '0': case 0x00: return digitalInOut0; 01426 #endif 01427 #if HAS_digitalInOut1 01428 case '1': case 0x01: return digitalInOut1; 01429 #endif 01430 #if HAS_digitalInOut2 01431 case '2': case 0x02: return digitalInOut2; 01432 #endif 01433 #if HAS_digitalInOut3 01434 case '3': case 0x03: return digitalInOut3; 01435 #endif 01436 #if HAS_digitalInOut4 01437 case '4': case 0x04: return digitalInOut4; 01438 #endif 01439 #if HAS_digitalInOut5 01440 case '5': case 0x05: return digitalInOut5; 01441 #endif 01442 #if HAS_digitalInOut6 01443 case '6': case 0x06: return digitalInOut6; 01444 #endif 01445 #if HAS_digitalInOut7 01446 case '7': case 0x07: return digitalInOut7; 01447 #endif 01448 #if HAS_digitalInOut8 01449 case '8': case 0x08: return digitalInOut8; 01450 #endif 01451 #if HAS_digitalInOut9 01452 case '9': case 0x09: return digitalInOut9; 01453 #endif 01454 #if HAS_digitalInOut10 01455 case 'a': case 0x0a: return digitalInOut10; 01456 #endif 01457 #if HAS_digitalInOut11 01458 case 'b': case 0x0b: return digitalInOut11; 01459 #endif 01460 #if HAS_digitalInOut12 01461 case 'c': case 0x0c: return digitalInOut12; 01462 #endif 01463 #if HAS_digitalInOut13 01464 case 'd': case 0x0d: return digitalInOut13; 01465 #endif 01466 #if HAS_digitalInOut14 01467 case 'e': case 0x0e: return digitalInOut14; 01468 #endif 01469 #if HAS_digitalInOut15 01470 case 'f': case 0x0f: return digitalInOut15; 01471 #endif 01472 #if HAS_digitalInOut16 01473 case 'g': case 0x10: return digitalInOut16; 01474 #endif 01475 #if HAS_digitalInOut17 01476 case 'h': case 0x11: return digitalInOut17; 01477 #endif 01478 } 01479 } 01480 #endif 01481 01482 01483 // AnalogIn pin resource: search index 01484 #if HAS_analogIns 01485 AnalogIn& find_analogInPin(int cPinIndex) 01486 { 01487 switch (cPinIndex) 01488 { 01489 default: // default to the first defined analogIn pin 01490 #if HAS_analogIn0 01491 case '0': case 0x00: return analogIn0; 01492 #endif 01493 #if HAS_analogIn1 01494 case '1': case 0x01: return analogIn1; 01495 #endif 01496 #if HAS_analogIn2 01497 case '2': case 0x02: return analogIn2; 01498 #endif 01499 #if HAS_analogIn3 01500 case '3': case 0x03: return analogIn3; 01501 #endif 01502 #if HAS_analogIn4 01503 case '4': case 0x04: return analogIn4; 01504 #endif 01505 #if HAS_analogIn5 01506 case '5': case 0x05: return analogIn5; 01507 #endif 01508 #if HAS_analogIn6 01509 case '6': case 0x06: return analogIn6; 01510 #endif 01511 #if HAS_analogIn7 01512 case '7': case 0x07: return analogIn7; 01513 #endif 01514 #if HAS_analogIn8 01515 case '8': case 0x08: return analogIn8; 01516 #endif 01517 #if HAS_analogIn9 01518 case '9': case 0x09: return analogIn9; 01519 #endif 01520 #if HAS_analogIn10 01521 case 'a': case 0x0a: return analogIn10; 01522 #endif 01523 #if HAS_analogIn11 01524 case 'b': case 0x0b: return analogIn11; 01525 #endif 01526 #if HAS_analogIn12 01527 case 'c': case 0x0c: return analogIn12; 01528 #endif 01529 #if HAS_analogIn13 01530 case 'd': case 0x0d: return analogIn13; 01531 #endif 01532 #if HAS_analogIn14 01533 case 'e': case 0x0e: return analogIn14; 01534 #endif 01535 #if HAS_analogIn15 01536 case 'f': case 0x0f: return analogIn15; 01537 #endif 01538 } 01539 } 01540 #endif 01541 01542 #if HAS_analogIns 01543 const float analogInPin_fullScaleVoltage[] = { 01544 # if defined(TARGET_MAX32630) 01545 ADC_FULL_SCALE_VOLTAGE, // analogIn0 01546 ADC_FULL_SCALE_VOLTAGE, // analogIn1 01547 ADC_FULL_SCALE_VOLTAGE, // analogIn2 01548 ADC_FULL_SCALE_VOLTAGE, // analogIn3 01549 ADC_FULL_SCALE_VOLTAGE * 5.0f, // analogIn4 // AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01550 ADC_FULL_SCALE_VOLTAGE * 5.0f, // analogIn4 // AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01551 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn6 // AIN_6 = VDDB / 4.0 fullscale is 4.8V 01552 ADC_FULL_SCALE_VOLTAGE, // analogIn7 // AIN_7 = VDD18 fullscale is 1.2V 01553 ADC_FULL_SCALE_VOLTAGE, // analogIn8 // AIN_8 = VDD12 fullscale is 1.2V 01554 ADC_FULL_SCALE_VOLTAGE * 2.0f, // analogIn9 // AIN_9 = VRTC / 2.0 fullscale is 2.4V 01555 ADC_FULL_SCALE_VOLTAGE, // analogIn10 // AIN_10 = x undefined? 01556 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn11 // AIN_11 = VDDIO / 4.0 fullscale is 4.8V 01557 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn12 // AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 01558 ADC_FULL_SCALE_VOLTAGE, // analogIn13 01559 ADC_FULL_SCALE_VOLTAGE, // analogIn14 01560 ADC_FULL_SCALE_VOLTAGE // analogIn15 01561 # elif defined(TARGET_MAX32620FTHR) 01562 #warning "TARGET_MAX32620FTHR not previously tested; need to verify analogIn0..." 01563 ADC_FULL_SCALE_VOLTAGE, // analogIn0 01564 ADC_FULL_SCALE_VOLTAGE, // analogIn1 01565 ADC_FULL_SCALE_VOLTAGE, // analogIn2 01566 ADC_FULL_SCALE_VOLTAGE, // analogIn3 01567 ADC_FULL_SCALE_VOLTAGE * 5.0f, // analogIn4 // AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01568 ADC_FULL_SCALE_VOLTAGE * 5.0f, // analogIn4 // AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01569 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn6 // AIN_6 = VDDB / 4.0 fullscale is 4.8V 01570 ADC_FULL_SCALE_VOLTAGE, // analogIn7 // AIN_7 = VDD18 fullscale is 1.2V 01571 ADC_FULL_SCALE_VOLTAGE, // analogIn8 // AIN_8 = VDD12 fullscale is 1.2V 01572 ADC_FULL_SCALE_VOLTAGE * 2.0f, // analogIn9 // AIN_9 = VRTC / 2.0 fullscale is 2.4V 01573 ADC_FULL_SCALE_VOLTAGE, // analogIn10 // AIN_10 = x undefined? 01574 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn11 // AIN_11 = VDDIO / 4.0 fullscale is 4.8V 01575 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn12 // AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 01576 ADC_FULL_SCALE_VOLTAGE, // analogIn13 01577 ADC_FULL_SCALE_VOLTAGE, // analogIn14 01578 ADC_FULL_SCALE_VOLTAGE // analogIn15 01579 #elif defined(TARGET_MAX32625MBED) || defined(TARGET_MAX32625PICO) 01580 ADC_FULL_SCALE_VOLTAGE * 1.0f, // analogIn0 // fullscale is 1.2V 01581 ADC_FULL_SCALE_VOLTAGE * 1.0f, // analogIn1 // fullscale is 1.2V 01582 ADC_FULL_SCALE_VOLTAGE * 1.0f, // analogIn2 // fullscale is 1.2V 01583 ADC_FULL_SCALE_VOLTAGE * 1.0f, // analogIn3 // fullscale is 1.2V 01584 ADC_FULL_SCALE_VOLTAGE * 5.0f, // analogIn4 // AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01585 ADC_FULL_SCALE_VOLTAGE * 5.0f, // analogIn4 // AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01586 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn6 // AIN_6 = VDDB / 4.0 fullscale is 4.8V 01587 ADC_FULL_SCALE_VOLTAGE, // analogIn7 // AIN_7 = VDD18 fullscale is 1.2V 01588 ADC_FULL_SCALE_VOLTAGE, // analogIn8 // AIN_8 = VDD12 fullscale is 1.2V 01589 ADC_FULL_SCALE_VOLTAGE * 2.0f, // analogIn9 // AIN_9 = VRTC / 2.0 fullscale is 2.4V 01590 ADC_FULL_SCALE_VOLTAGE, // analogIn10 // AIN_10 = x undefined? 01591 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn11 // AIN_11 = VDDIO / 4.0 fullscale is 4.8V 01592 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn12 // AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 01593 ADC_FULL_SCALE_VOLTAGE, // analogIn13 01594 ADC_FULL_SCALE_VOLTAGE, // analogIn14 01595 ADC_FULL_SCALE_VOLTAGE // analogIn15 01596 #elif defined(TARGET_NUCLEO_F446RE) 01597 ADC_FULL_SCALE_VOLTAGE, // analogIn0 01598 ADC_FULL_SCALE_VOLTAGE, // analogIn1 01599 ADC_FULL_SCALE_VOLTAGE, // analogIn2 01600 ADC_FULL_SCALE_VOLTAGE, // analogIn3 01601 ADC_FULL_SCALE_VOLTAGE, // analogIn4 01602 ADC_FULL_SCALE_VOLTAGE, // analogIn5 01603 ADC_FULL_SCALE_VOLTAGE, // analogIn6 01604 ADC_FULL_SCALE_VOLTAGE, // analogIn7 01605 ADC_FULL_SCALE_VOLTAGE, // analogIn8 01606 ADC_FULL_SCALE_VOLTAGE, // analogIn9 01607 ADC_FULL_SCALE_VOLTAGE, // analogIn10 01608 ADC_FULL_SCALE_VOLTAGE, // analogIn11 01609 ADC_FULL_SCALE_VOLTAGE, // analogIn12 01610 ADC_FULL_SCALE_VOLTAGE, // analogIn13 01611 ADC_FULL_SCALE_VOLTAGE, // analogIn14 01612 ADC_FULL_SCALE_VOLTAGE // analogIn15 01613 #elif defined(TARGET_NUCLEO_F401RE) 01614 ADC_FULL_SCALE_VOLTAGE, // analogIn0 01615 ADC_FULL_SCALE_VOLTAGE, // analogIn1 01616 ADC_FULL_SCALE_VOLTAGE, // analogIn2 01617 ADC_FULL_SCALE_VOLTAGE, // analogIn3 01618 ADC_FULL_SCALE_VOLTAGE, // analogIn4 01619 ADC_FULL_SCALE_VOLTAGE, // analogIn5 01620 ADC_FULL_SCALE_VOLTAGE, // analogIn6 01621 ADC_FULL_SCALE_VOLTAGE, // analogIn7 01622 ADC_FULL_SCALE_VOLTAGE, // analogIn8 01623 ADC_FULL_SCALE_VOLTAGE, // analogIn9 01624 ADC_FULL_SCALE_VOLTAGE, // analogIn10 01625 ADC_FULL_SCALE_VOLTAGE, // analogIn11 01626 ADC_FULL_SCALE_VOLTAGE, // analogIn12 01627 ADC_FULL_SCALE_VOLTAGE, // analogIn13 01628 ADC_FULL_SCALE_VOLTAGE, // analogIn14 01629 ADC_FULL_SCALE_VOLTAGE // analogIn15 01630 //#elif defined(TARGET_LPC1768) 01631 #else 01632 // unknown target 01633 ADC_FULL_SCALE_VOLTAGE, // analogIn0 01634 ADC_FULL_SCALE_VOLTAGE, // analogIn1 01635 ADC_FULL_SCALE_VOLTAGE, // analogIn2 01636 ADC_FULL_SCALE_VOLTAGE, // analogIn3 01637 ADC_FULL_SCALE_VOLTAGE, // analogIn4 01638 ADC_FULL_SCALE_VOLTAGE, // analogIn5 01639 ADC_FULL_SCALE_VOLTAGE, // analogIn6 01640 ADC_FULL_SCALE_VOLTAGE, // analogIn7 01641 ADC_FULL_SCALE_VOLTAGE, // analogIn8 01642 ADC_FULL_SCALE_VOLTAGE, // analogIn9 01643 ADC_FULL_SCALE_VOLTAGE, // analogIn10 01644 ADC_FULL_SCALE_VOLTAGE, // analogIn11 01645 ADC_FULL_SCALE_VOLTAGE, // analogIn12 01646 ADC_FULL_SCALE_VOLTAGE, // analogIn13 01647 ADC_FULL_SCALE_VOLTAGE, // analogIn14 01648 ADC_FULL_SCALE_VOLTAGE // analogIn15 01649 # endif 01650 }; 01651 #endif 01652 01653 01654 01655 01656 //-------------------------------------------------- 01657 // Option to use LEDs to show status 01658 #ifndef USE_LEDS 01659 #define USE_LEDS 1 01660 #endif 01661 #if USE_LEDS 01662 #if defined(TARGET_MAX32630) 01663 # define LED_ON 0 01664 # define LED_OFF 1 01665 //-------------------------------------------------- 01666 #elif defined(TARGET_MAX32625MBED) 01667 # define LED_ON 0 01668 # define LED_OFF 1 01669 #elif defined(TARGET_MAX32625PICO) 01670 # define LED_ON 0 01671 # define LED_OFF 1 01672 //-------------------------------------------------- 01673 // TODO1: TARGET=MAX32625MBED ARM Cortex-M4F 96MHz 512kB Flash 160kB SRAM 01674 #elif defined(TARGET_LPC1768) 01675 # define LED_ON 1 01676 # define LED_OFF 0 01677 #else // not defined(TARGET_LPC1768 etc.) 01678 // USE_LEDS with some platform other than MAX32630, MAX32625MBED, LPC1768 01679 // bugfix for MAX32600MBED LED blink pattern: check if LED_ON/LED_OFF already defined 01680 # ifndef LED_ON 01681 # define LED_ON 0 01682 # endif 01683 # ifndef LED_OFF 01684 # define LED_OFF 1 01685 # endif 01686 //# define LED_ON 1 01687 //# define LED_OFF 0 01688 #endif // target definition 01689 DigitalOut led1(LED1, LED_OFF); // MAX32630FTHR: LED1 = LED_RED 01690 DigitalOut led2(LED2, LED_OFF); // MAX32630FTHR: LED2 = LED_GREEN 01691 DigitalOut led3(LED3, LED_OFF); // MAX32630FTHR: LED3 = LED_BLUE 01692 DigitalOut led4(LED4, LED_OFF); 01693 #else // USE_LEDS=0 01694 // issue #41 support Nucleo_F446RE 01695 // there are no LED indicators on the board, LED1 interferes with SPI; 01696 // but we still need placeholders led1 led2 led3 led4. 01697 // Declare DigitalOut led1 led2 led3 led4 targeting safe pins. 01698 // PinName NC means NOT_CONNECTED; DigitalOut::is_connected() returns false 01699 # define LED_ON 0 01700 # define LED_OFF 1 01701 DigitalOut led1(NC, LED_OFF); 01702 DigitalOut led2(NC, LED_OFF); 01703 DigitalOut led3(NC, LED_OFF); 01704 DigitalOut led4(NC, LED_OFF); 01705 #endif // USE_LEDS 01706 #define led1_RFailLED led1 01707 #define led2_GPassLED led2 01708 #define led3_BBusyLED led3 01709 01710 //-------------------------------------------------- 01711 01712 01713 // example code board support 01714 //MAX32630FTHR pegasus(MAX32630FTHR::VIO_3V3); 01715 //DigitalOut rLED(LED1); 01716 //DigitalOut gLED(LED2); 01717 //DigitalOut bLED(LED3); 01718 // 01719 // Arduino "shield" connector port definitions (MAX32625MBED shown) 01720 #if defined(TARGET_MAX32625MBED) 01721 #define A0 AIN_0 01722 #define A1 AIN_1 01723 #define A2 AIN_2 01724 #define A3 AIN_3 01725 #define D0 P0_0 01726 #define D1 P0_1 01727 #define D2 P0_2 01728 #define D3 P0_3 01729 #define D4 P0_4 01730 #define D5 P0_5 01731 #define D6 P0_6 01732 #define D7 P0_7 01733 #define D8 P1_4 01734 #define D9 P1_5 01735 #define D10 P1_3 01736 #define D11 P1_1 01737 #define D12 P1_2 01738 #define D13 P1_0 01739 #elif defined(TARGET_MAX32625PICO) 01740 #warning "TARGET_MAX32625PICO not previously tested; need to define pins..." 01741 #define A0 AIN_1 01742 #define A1 AIN_2 01743 // #define A2 AIN_3 01744 // #define A3 AIN_0 01745 #define D0 P0_0 01746 #define D1 P0_1 01747 #define D2 P0_2 01748 #define D3 P0_3 01749 #define D4 P1_7 01750 #define D5 P1_6 01751 #define D6 P4_4 01752 #define D7 P4_5 01753 #define D8 P4_6 01754 #define D9 P4_7 01755 #define D10 P0_7 01756 #define D11 P0_6 01757 #define D12 P0_5 01758 #define D13 P0_4 01759 #endif 01760 01761 // example code declare SPI interface (GPIO controlled CS) 01762 #if defined(TARGET_MAX32625MBED) 01763 SPI spi(SPI1_MOSI, SPI1_MISO, SPI1_SCK); // mosi, miso, sclk spi1 TARGET_MAX32625MBED: P1_1 P1_2 P1_0 Arduino 10-pin header D11 D12 D13 01764 DigitalOut spi_cs(SPI1_SS); // TARGET_MAX32625MBED: P1_3 Arduino 10-pin header D10 01765 #elif defined(TARGET_MAX32625PICO) 01766 #warning "TARGET_MAX32625PICO not previously tested; need to define pins..." 01767 SPI spi(SPI0_MOSI, SPI0_MISO, SPI0_SCK); // mosi, miso, sclk spi1 TARGET_MAX32625PICO: pin P0_5 P0_6 P0_4 01768 DigitalOut spi_cs(SPI0_SS); // TARGET_MAX32625PICO: pin P0_7 01769 #elif defined(TARGET_MAX32600MBED) 01770 SPI spi(SPI2_MOSI, SPI2_MISO, SPI2_SCK); // mosi, miso, sclk spi1 TARGET_MAX32600MBED: Arduino 10-pin header D11 D12 D13 01771 DigitalOut spi_cs(SPI2_SS); // Generic: Arduino 10-pin header D10 01772 #elif defined(TARGET_NUCLEO_F446RE) || defined(TARGET_NUCLEO_F401RE) 01773 // TODO1: avoid resource conflict between P5_0, P5_1, P5_2 SPI and DigitalInOut 01774 // void spi_init(spi_t *obj, PinName mosi, PinName miso, PinName sclk, PinName ssel) 01775 // 01776 // TODO1: NUCLEO_F446RE SPI not working; CS and MOSI data looks OK but no SCLK clock pulses. 01777 SPI spi(SPI_MOSI, SPI_MISO, SPI_SCK); // mosi, miso, sclk spi1 TARGET_NUCLEO_F446RE: Arduino 10-pin header D11 D12 D13 01778 DigitalOut spi_cs(SPI_CS); // TARGET_NUCLEO_F446RE: PB_6 Arduino 10-pin header D10 01779 // 01780 #else 01781 SPI spi(D11, D12, D13); // mosi, miso, sclk spi1 TARGET_MAX32600MBED: Arduino 10-pin header D11 D12 D13 01782 DigitalOut spi_cs(D10); // Generic: Arduino 10-pin header D10 01783 #endif 01784 01785 // example code declare GPIO interface pins 01786 // AnalogOut RFB_pin(Px_x_PortName_To_Be_Determined); // Analog Input to MAX5719 device 01787 // AnalogOut INV_pin(Px_x_PortName_To_Be_Determined); // Analog Input to MAX5719 device 01788 DigitalOut LDACb_pin(D9); // Digital Trigger Input to MAX5719 device 01789 // AnalogIn OUT_pin(A0); // Analog Output from MAX5719 device 01790 // example code declare device instance 01791 MAX5719 g_MAX5719_device(spi, spi_cs, LDACb_pin, MAX5719::MAX5719_IC); 01792 01793 01794 //---------------------------------------- 01795 // Global SPI options 01796 // 01797 01798 //-------------------------------------------------- 01799 // Optional Diagnostic function to print SPI transactions 01800 #ifndef MAX5719_ONSPIPRINT 01801 #define MAX5719_ONSPIPRINT 1 01802 #endif // MAX5719_ONSPIPRINT 01803 // Enable the onSPIprint diagnostic at startup (toggle with %SD menu item) 01804 #ifndef MAX5719_ONSPIPRINT_ENABLED 01805 #define MAX5719_ONSPIPRINT_ENABLED 1 01806 #endif // MAX5719_ONSPIPRINT_ENABLED 01807 01808 #define APPLICATION_ArduinoPinsMonitor 1 01809 01810 //-------------------------------------------------- 01811 // use BUTTON1 trigger some action 01812 #if defined(TARGET_MAX32630) 01813 #define HAS_BUTTON1_DEMO_INTERRUPT 1 01814 #define HAS_BUTTON2_DEMO 0 01815 #define HAS_BUTTON2_DEMO_INTERRUPT 0 01816 #elif defined(TARGET_MAX32625PICO) 01817 #warning "TARGET_MAX32625PICO not previously tested; need to define buttons..." 01818 #define HAS_BUTTON1_DEMO_INTERRUPT 1 01819 #define HAS_BUTTON2_DEMO 0 01820 #define HAS_BUTTON2_DEMO_INTERRUPT 0 01821 #elif defined(TARGET_MAX32625) 01822 #define HAS_BUTTON1_DEMO_INTERRUPT 1 01823 #define HAS_BUTTON2_DEMO_INTERRUPT 1 01824 #elif defined(TARGET_MAX32620FTHR) 01825 #warning "TARGET_MAX32620FTHR not previously tested; need to define buttons..." 01826 #define BUTTON1 SW1 01827 #define HAS_BUTTON1_DEMO_INTERRUPT 1 01828 #define HAS_BUTTON2_DEMO 0 01829 #define HAS_BUTTON2_DEMO_INTERRUPT 0 01830 #elif defined(TARGET_NUCLEO_F446RE) 01831 #define HAS_BUTTON1_DEMO_INTERRUPT 0 01832 #define HAS_BUTTON2_DEMO_INTERRUPT 0 01833 #elif defined(TARGET_NUCLEO_F401RE) 01834 #define HAS_BUTTON1_DEMO_INTERRUPT 0 01835 #define HAS_BUTTON2_DEMO_INTERRUPT 0 01836 #else 01837 #warning "target not previously tested; need to define buttons..." 01838 #endif 01839 // 01840 #ifndef HAS_BUTTON1_DEMO 01841 #define HAS_BUTTON1_DEMO 0 01842 #endif 01843 #ifndef HAS_BUTTON2_DEMO 01844 #define HAS_BUTTON2_DEMO 0 01845 #endif 01846 // 01847 // avoid runtime error on button1 press [mbed-os-5.11] 01848 // instead of using InterruptIn, use DigitalIn and poll in main while(1) 01849 #ifndef HAS_BUTTON1_DEMO_INTERRUPT_POLLING 01850 #define HAS_BUTTON1_DEMO_INTERRUPT_POLLING 1 01851 #endif 01852 // 01853 #ifndef HAS_BUTTON1_DEMO_INTERRUPT 01854 #define HAS_BUTTON1_DEMO_INTERRUPT 1 01855 #endif 01856 #ifndef HAS_BUTTON2_DEMO_INTERRUPT 01857 #define HAS_BUTTON2_DEMO_INTERRUPT 1 01858 #endif 01859 // 01860 #if HAS_BUTTON1_DEMO_INTERRUPT 01861 # if HAS_BUTTON1_DEMO_INTERRUPT_POLLING 01862 // avoid runtime error on button1 press [mbed-os-5.11] 01863 // instead of using InterruptIn, use DigitalIn and poll in main while(1) 01864 DigitalIn button1(BUTTON1); 01865 # else 01866 InterruptIn button1(BUTTON1); 01867 # endif 01868 #elif HAS_BUTTON1_DEMO 01869 DigitalIn button1(BUTTON1); 01870 #endif 01871 #if HAS_BUTTON2_DEMO_INTERRUPT 01872 # if HAS_BUTTON1_DEMO_INTERRUPT_POLLING 01873 // avoid runtime error on button1 press [mbed-os-5.11] 01874 // instead of using InterruptIn, use DigitalIn and poll in main while(1) 01875 DigitalIn button2(BUTTON2); 01876 # else 01877 InterruptIn button2(BUTTON2); 01878 # endif 01879 #elif HAS_BUTTON2_DEMO 01880 DigitalIn button2(BUTTON2); 01881 #endif 01882 01883 //-------------------------------------------------- 01884 // functions tested by SelfTest() 01885 extern void fn_MAX5719_Init(void); 01886 extern uint8_t fn_MAX5719_CODE_LOAD(uint32_t dacCodeLsbs); 01887 extern uint32_t fn_MAX5719_DACCodeOfVoltage(double voltageV); 01888 01889 //-------------------------------------------------- 01890 // SelfTestGroupEnable selects which of the included tests will run 01891 int SelfTestGroupEnable = 0 01892 // | 0x0001 // -- halt-on-first-failure configuration flag 01893 // | 0x0002 // -- repeat-until-failure configuration flag 01894 | 0x0004 // CODE_LOAD -- Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) 01895 | 0x0008 // CODE_LOAD_2V5 -- Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) (no run on button) 01896 | 0x0010 // CODE_LOAD_3V0 -- Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) (no run on button) 01897 | 0x0020 // CODE_LOAD_4V1 -- Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) (no run on button) 01898 | 0x0040 // DACCodeOfVoltage -- Verify function DACCodeOfVoltage (enabled by default) (no run on button) 01899 ; 01900 01901 //-------------------------------------------------- 01902 // optional self-test groups for self test function SelfTest() 01903 // enable by changing the #define value from 0 to 1 01904 01905 // SelfTest group CODE_LOAD description: 01906 // Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) 01907 // SelfTestGroupEnable bitmask 0x0004 01908 // self test command: 01909 // . run=0x0004 01910 #ifndef MAX5719_SELFTEST_CODE_LOAD 01911 #define MAX5719_SELFTEST_CODE_LOAD 1 01912 #endif 01913 01914 // SelfTest group CODE_LOAD_2V5 description: 01915 // Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) (no run on button) 01916 // SelfTestGroupEnable bitmask 0x0008 01917 // self test command: 01918 // . run=0x0008 01919 #ifndef MAX5719_SELFTEST_CODE_LOAD_2V5 01920 #define MAX5719_SELFTEST_CODE_LOAD_2V5 1 01921 #endif 01922 01923 // SelfTest group CODE_LOAD_3V0 description: 01924 // Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) (no run on button) 01925 // SelfTestGroupEnable bitmask 0x0010 01926 // self test command: 01927 // . run=0x0010 01928 #ifndef MAX5719_SELFTEST_CODE_LOAD_3V0 01929 #define MAX5719_SELFTEST_CODE_LOAD_3V0 1 01930 #endif 01931 01932 // SelfTest group CODE_LOAD_4V1 description: 01933 // Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) (no run on button) 01934 // SelfTestGroupEnable bitmask 0x0020 01935 // self test command: 01936 // . run=0x0020 01937 #ifndef MAX5719_SELFTEST_CODE_LOAD_4V1 01938 #define MAX5719_SELFTEST_CODE_LOAD_4V1 1 01939 #endif 01940 01941 // SelfTest group DACCodeOfVoltage description: 01942 // Verify function DACCodeOfVoltage (enabled by default) (no run on button) 01943 // SelfTestGroupEnable bitmask 0x0040 01944 // self test command: 01945 // . run=0x0040 01946 #ifndef MAX5719_SELFTEST_DACCodeOfVoltage 01947 #define MAX5719_SELFTEST_DACCodeOfVoltage 1 01948 #endif 01949 01950 //-------------------------------------------------- 01951 // When user presses button BUTTON1, perform self test 01952 #if HAS_BUTTON1_DEMO_INTERRUPT 01953 void onButton1FallingEdge(void) 01954 { 01955 void SelfTest(CmdLine & cmdLine); 01956 // SelfTestGroupEnable selects which of the included tests will run 01957 SelfTestGroupEnable = 0 01958 // | 0x0001 // -- halt-on-first-failure configuration flag 01959 // | 0x0002 // -- repeat-until-failure configuration flag 01960 | 0x0004 // CODE_LOAD -- Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) 01961 // | 0x0008 // CODE_LOAD_2V5 -- Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) (no run on button) 01962 // | 0x0010 // CODE_LOAD_3V0 -- Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) (no run on button) 01963 // | 0x0020 // CODE_LOAD_4V1 -- Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) (no run on button) 01964 // | 0x0040 // DACCodeOfVoltage -- Verify function DACCodeOfVoltage (enabled by default) (no run on button) 01965 ; 01966 SelfTest(cmdLine_serial); 01967 } 01968 #endif // HAS_BUTTON1_DEMO_INTERRUPT 01969 01970 //-------------------------------------------------- 01971 // When user presses button BUTTON2, perform demo configuration 01972 #if HAS_BUTTON2_DEMO_INTERRUPT 01973 void onButton2FallingEdge(void) 01974 { 01975 // TBD demo configuration 01976 // When user presses button BUTTON2, perform self test until failure 01977 void SelfTest(CmdLine & cmdLine); 01978 // SelfTestGroupEnable selects which of the included tests will run 01979 SelfTestGroupEnable = 0 01980 | 0x0001 // -- halt-on-first-failure configuration flag 01981 | 0x0002 // -- repeat-until-failure configuration flag 01982 | 0x0004 // CODE_LOAD -- Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) 01983 // | 0x0008 // CODE_LOAD_2V5 -- Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) (no run on button) 01984 // | 0x0010 // CODE_LOAD_3V0 -- Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) (no run on button) 01985 // | 0x0020 // CODE_LOAD_4V1 -- Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) (no run on button) 01986 // | 0x0040 // DACCodeOfVoltage -- Verify function DACCodeOfVoltage (enabled by default) (no run on button) 01987 ; 01988 SelfTest(cmdLine_serial); 01989 } 01990 #endif // HAS_BUTTON2_DEMO_INTERRUPT 01991 01992 //-------------------------------------------------- 01993 void SelfTest(CmdLine & cmdLine) 01994 { 01995 //-------------------------------------------------- 01996 #if analogIn4_IS_HIGH_RANGE_OF_analogIn0 01997 // Platform board uses AIN4,AIN5,.. as high range of AIN0,AIN1,.. 01998 MaximTinyTester tinyTester(cmdLine, analogIn4, analogIn5, analogIn2, analogIn3, analogIn0, analogIn4, led1_RFailLED, led2_GPassLED, led3_BBusyLED); 01999 tinyTester.analogInPin_fullScaleVoltage[0] = analogInPin_fullScaleVoltage[4]; // board support 02000 tinyTester.analogInPin_fullScaleVoltage[1] = analogInPin_fullScaleVoltage[5]; // board support 02001 tinyTester.analogInPin_fullScaleVoltage[2] = analogInPin_fullScaleVoltage[2]; // board support 02002 tinyTester.analogInPin_fullScaleVoltage[3] = analogInPin_fullScaleVoltage[3]; // board support 02003 tinyTester.analogInPin_fullScaleVoltage[4] = analogInPin_fullScaleVoltage[0]; // board support 02004 tinyTester.analogInPin_fullScaleVoltage[5] = analogInPin_fullScaleVoltage[1]; // board support 02005 // low range channels AIN0, AIN1, AIN2, AIN3 02006 #else // analogIn4_IS_HIGH_RANGE_OF_analogIn0 02007 // Platform board uses simple analog inputs 02008 MaximTinyTester tinyTester(cmdLine, analogIn0, analogIn1, analogIn2, analogIn3, analogIn4, analogIn5, led1_RFailLED, led2_GPassLED, led3_BBusyLED); 02009 tinyTester.analogInPin_fullScaleVoltage[0] = analogInPin_fullScaleVoltage[0]; // board support 02010 tinyTester.analogInPin_fullScaleVoltage[1] = analogInPin_fullScaleVoltage[1]; // board support 02011 tinyTester.analogInPin_fullScaleVoltage[2] = analogInPin_fullScaleVoltage[2]; // board support 02012 tinyTester.analogInPin_fullScaleVoltage[3] = analogInPin_fullScaleVoltage[3]; // board support 02013 tinyTester.analogInPin_fullScaleVoltage[4] = analogInPin_fullScaleVoltage[4]; // board support 02014 tinyTester.analogInPin_fullScaleVoltage[5] = analogInPin_fullScaleVoltage[5]; // board support 02015 #endif 02016 tinyTester.clear(); 02017 02018 // repeat-until-failure logic 02019 repeatUntilFailure: 02020 cmdLine.serial().printf("\r\n. run=0x%4.4x", SelfTestGroupEnable); 02021 if ((SelfTestGroupEnable & 0x0003) == 0x0000) { cmdLine.serial().printf("\r\n. runall=0x%4.4x", SelfTestGroupEnable &~ 0x0003); } 02022 if ((SelfTestGroupEnable & 0x0003) == 0x0001) { cmdLine.serial().printf("\r\n. runfail=0x%4.4x", SelfTestGroupEnable &~ 0x0003); } 02023 if ((SelfTestGroupEnable & 0x0003) == 0x0002) { cmdLine.serial().printf("\r\n. loopall=0x%4.4x", SelfTestGroupEnable &~ 0x0003); } 02024 if ((SelfTestGroupEnable & 0x0003) == 0x0003) { cmdLine.serial().printf("\r\n. loopfail=0x%4.4x", SelfTestGroupEnable &~ 0x0003); } 02025 cmdLine.serial().printf("\r\n 0x0004 %s CODE_LOAD", (SelfTestGroupEnable & 0x0004) ? "run " : " skip"); 02026 cmdLine.serial().printf("\r\n 0x0008 %s CODE_LOAD_2V5", (SelfTestGroupEnable & 0x0008) ? "run " : " skip"); 02027 cmdLine.serial().printf("\r\n 0x0010 %s CODE_LOAD_3V0", (SelfTestGroupEnable & 0x0010) ? "run " : " skip"); 02028 cmdLine.serial().printf("\r\n 0x0020 %s CODE_LOAD_4V1", (SelfTestGroupEnable & 0x0020) ? "run " : " skip"); 02029 cmdLine.serial().printf("\r\n 0x0040 %s DACCodeOfVoltage", (SelfTestGroupEnable & 0x0040) ? "run " : " skip"); 02030 // Report number of pass and number of fail test results 02031 tinyTester.Report_Summary(); 02032 // 02033 // @test group CODE_LOAD // Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) 02034 // @test group CODE_LOAD tinyTester.print("VRef = 4.096 MAX5719 20-bit LSB = 0.000004V = 3.90625uV") 02035 // docTest_item['actionType'] = 'print-string' 02036 // docTest_item['group-id-value'] = 'CODE_LOAD' 02037 // docTest_item['action'] = 'tinyTester.print("VRef = 4.096 MAX5719 20-bit LSB = 0.000004V = 3.90625uV")' 02038 // docTest_item['arglist'] = 'VRef = 4.096 MAX5719 20-bit LSB = 0.000004V = 3.90625uV' 02039 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02040 if (SelfTestGroupEnable & 0x0004) { 02041 // print-string 02042 // tinyTesterFuncName = "tinyTester.print" 02043 // tinyTesterPrintStringLiteral = "VRef = 4.096 MAX5719 20-bit LSB = 0.000004V = 3.90625uV" 02044 tinyTester.print("VRef = 4.096 MAX5719 20-bit LSB = 0.000004V = 3.90625uV"); 02045 // halt-on-first-failure logic 02046 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02047 } // if (SelfTestGroupEnable & 0x0004) 02048 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02049 02050 // @test group CODE_LOAD tinyTester.print("Wire MAX5719 OUT to platform AIN0 for analog loopback tests...") 02051 // docTest_item['actionType'] = 'print-string' 02052 // docTest_item['group-id-value'] = 'CODE_LOAD' 02053 // docTest_item['action'] = 'tinyTester.print("Wire MAX5719 OUT to platform AIN0 for analog loopback tests...")' 02054 // docTest_item['arglist'] = 'Wire MAX5719 OUT to platform AIN0 for analog loopback tests...' 02055 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02056 if (SelfTestGroupEnable & 0x0004) { 02057 // print-string 02058 // tinyTesterFuncName = "tinyTester.print" 02059 // tinyTesterPrintStringLiteral = "Wire MAX5719 OUT to platform AIN0 for analog loopback tests..." 02060 tinyTester.print("Wire MAX5719 OUT to platform AIN0 for analog loopback tests..."); 02061 // halt-on-first-failure logic 02062 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02063 } // if (SelfTestGroupEnable & 0x0004) 02064 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02065 02066 // @test group CODE_LOAD VRef = 4.096 02067 // docTest_item['actionType'] = 'assign-propname-value' 02068 // docTest_item['group-id-value'] = 'CODE_LOAD' 02069 // docTest_item['action'] = 'VRef = 4.096' 02070 // docTest_item['propName'] = 'VRef' 02071 // docTest_item['propValue'] = '4.096' 02072 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02073 if (SelfTestGroupEnable & 0x0004) { 02074 // assign-propname-value 02075 // tinyTesterPropName = "VRef" 02076 // tinyTesterPropValue = "4.096" 02077 g_MAX5719_device.VRef = 4.096; 02078 // halt-on-first-failure logic 02079 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02080 } // if (SelfTestGroupEnable & 0x0004) 02081 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02082 02083 // @test group CODE_LOAD tinyTester.blink_time_msec = 75 // default 75 resume hardware self test 02084 // docTest_item['actionType'] = 'assign-propname-value' 02085 // docTest_item['group-id-value'] = 'CODE_LOAD' 02086 // docTest_item['action'] = 'tinyTester.blink_time_msec = 75' 02087 // docTest_item['remarks'] = 'default 75 resume hardware self test' 02088 // docTest_item['propName'] = 'tinyTester.blink_time_msec' 02089 // docTest_item['propValue'] = '75' 02090 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None default 75 resume hardware self test 02091 if (SelfTestGroupEnable & 0x0004) { 02092 // assign-propname-value 02093 // tinyTesterPropName = "tinyTester.blink_time_msec" 02094 // tinyTesterPropValue = "75" 02095 tinyTester.blink_time_msec = 75; 02096 // halt-on-first-failure logic 02097 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02098 } // if (SelfTestGroupEnable & 0x0004) 02099 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02100 02101 // @test group CODE_LOAD tinyTester.settle_time_msec = 500 02102 // docTest_item['actionType'] = 'assign-propname-value' 02103 // docTest_item['group-id-value'] = 'CODE_LOAD' 02104 // docTest_item['action'] = 'tinyTester.settle_time_msec = 500' 02105 // docTest_item['propName'] = 'tinyTester.settle_time_msec' 02106 // docTest_item['propValue'] = '500' 02107 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02108 if (SelfTestGroupEnable & 0x0004) { 02109 // assign-propname-value 02110 // tinyTesterPropName = "tinyTester.settle_time_msec" 02111 // tinyTesterPropValue = "500" 02112 tinyTester.settle_time_msec = 500; 02113 // halt-on-first-failure logic 02114 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02115 } // if (SelfTestGroupEnable & 0x0004) 02116 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02117 02118 // @test Init() 02119 // docTest_item['actionType'] = 'call-function' 02120 // docTest_item['action'] = 'Init()' 02121 // docTest_item['funcName'] = 'Init' 02122 // call-function 02123 // selfTestFunctionClosures['Init']['returnType'] = 'void' 02124 // ASSERT_EQ(g_MAX5719_device.Init(()), (void)None); // 02125 // tinyTester.FunctionCall_Expect("MAX5719.Init", fn_MAX5719_Init, /* empty docTest_argList */ /* empty expect: */ (void)None); // 02126 g_MAX5719_device.Init(); // 02127 02128 // @test VRef expect 4.096 // Nominal Full-Scale Voltage Reference 02129 // docTest_item['actionType'] = 'test-propname-expect-value' 02130 // docTest_item['action'] = 'VRef expect 4.096' 02131 // docTest_item['remarks'] = 'Nominal Full-Scale Voltage Reference' 02132 // docTest_item['expect-value'] = '4.096' 02133 // docTest_item['propName'] = 'VRef' 02134 // test-propname-expect-value 02135 tinyTester.Expect("MAX5719.VRef", g_MAX5719_device.VRef, /* expect: */ 4.096); // Nominal Full-Scale Voltage Reference 02136 02137 // @test group CODE_LOAD tinyTester.err_threshold = 0.050 02138 // docTest_item['actionType'] = 'assign-propname-value' 02139 // docTest_item['group-id-value'] = 'CODE_LOAD' 02140 // docTest_item['action'] = 'tinyTester.err_threshold = 0.050' 02141 // docTest_item['propName'] = 'tinyTester.err_threshold' 02142 // docTest_item['propValue'] = '0.050' 02143 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02144 if (SelfTestGroupEnable & 0x0004) { 02145 // assign-propname-value 02146 // tinyTesterPropName = "tinyTester.err_threshold" 02147 // tinyTesterPropValue = "0.050" 02148 tinyTester.err_threshold = 0.050; 02149 // halt-on-first-failure logic 02150 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02151 } // if (SelfTestGroupEnable & 0x0004) 02152 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02153 02154 // @test group CODE_LOAD tinyTester.print("0x000000 = 0.000V") 02155 // docTest_item['actionType'] = 'print-string' 02156 // docTest_item['group-id-value'] = 'CODE_LOAD' 02157 // docTest_item['action'] = 'tinyTester.print("0x000000 = 0.000V")' 02158 // docTest_item['arglist'] = '0x000000 = 0.000V' 02159 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02160 if (SelfTestGroupEnable & 0x0004) { 02161 // print-string 02162 // tinyTesterFuncName = "tinyTester.print" 02163 // tinyTesterPrintStringLiteral = "0x000000 = 0.000V" 02164 tinyTester.print("0x000000 = 0.000V"); 02165 // halt-on-first-failure logic 02166 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02167 } // if (SelfTestGroupEnable & 0x0004) 02168 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02169 02170 // @test group CODE_LOAD CODE_LOAD(0x000000) // 0.000V 02171 // docTest_item['actionType'] = 'call-function' 02172 // docTest_item['group-id-value'] = 'CODE_LOAD' 02173 // docTest_item['action'] = 'CODE_LOAD(0x000000)' 02174 // docTest_item['remarks'] = '0.000V' 02175 // docTest_item['funcName'] = 'CODE_LOAD' 02176 // docTest_item['arglist'] = '0x000000' 02177 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD CODE_LOAD 0.000V 02178 if (SelfTestGroupEnable & 0x0004) { 02179 // call-function 02180 // selfTestFunctionClosures['CODE_LOAD']['returnType'] = 'uint8_t' 02181 // ASSERT_EQ(g_MAX5719_device.CODE_LOAD((uint32_t)0x000000), (uint8_t)None); // 0.000V 02182 // tinyTester.FunctionCall_Expect("MAX5719.CODE_LOAD", fn_MAX5719_CODE_LOAD, (uint32_t)0x000000, /* empty expect: */ (uint8_t)None); // 0.000V 02183 g_MAX5719_device.CODE_LOAD((uint32_t)0x000000); // 0.000V 02184 // halt-on-first-failure logic 02185 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02186 } // if (SelfTestGroupEnable & 0x0004) 02187 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02188 02189 // @test group CODE_LOAD tinyTester.Wait_Output_Settling() 02190 // docTest_item['actionType'] = 'call-tinytester-function' 02191 // docTest_item['group-id-value'] = 'CODE_LOAD' 02192 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 02193 // docTest_item['propName'] = 'Wait_Output_Settling' 02194 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02195 if (SelfTestGroupEnable & 0x0004) { 02196 // call-tinytester-function 02197 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 02198 // docTest_argList = "" 02199 tinyTester.Wait_Output_Settling(); // 02200 // halt-on-first-failure logic 02201 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02202 } // if (SelfTestGroupEnable & 0x0004) 02203 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02204 02205 // @test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(0.000000) 02206 // docTest_item['actionType'] = 'call-tinytester-function' 02207 // docTest_item['group-id-value'] = 'CODE_LOAD' 02208 // docTest_item['action'] = 'tinyTester.AnalogIn0_Read_Expect_voltageV(0.000000)' 02209 // docTest_item['arglist'] = '0.000000' 02210 // docTest_item['propName'] = 'AnalogIn0_Read_Expect_voltageV' 02211 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02212 if (SelfTestGroupEnable & 0x0004) { 02213 // call-tinytester-function 02214 // tinyTesterFuncName = "tinyTester.AnalogIn0_Read_Expect_voltageV" 02215 // docTest_argList = "0.000000" 02216 tinyTester.AnalogIn0_Read_Expect_voltageV(0.000000); // 02217 // halt-on-first-failure logic 02218 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02219 } // if (SelfTestGroupEnable & 0x0004) 02220 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02221 02222 // @test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 02223 // docTest_item['actionType'] = 'call-tinytester-function' 02224 // docTest_item['group-id-value'] = 'CODE_LOAD' 02225 // docTest_item['action'] = 'tinyTester.AnalogIn1_Read_Report_voltageV()' 02226 // docTest_item['propName'] = 'AnalogIn1_Read_Report_voltageV' 02227 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02228 if (SelfTestGroupEnable & 0x0004) { 02229 // call-tinytester-function 02230 // tinyTesterFuncName = "tinyTester.AnalogIn1_Read_Report_voltageV" 02231 // docTest_argList = "" 02232 tinyTester.AnalogIn1_Read_Report_voltageV(); // 02233 // halt-on-first-failure logic 02234 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02235 } // if (SelfTestGroupEnable & 0x0004) 02236 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02237 02238 // @test group CODE_LOAD tinyTester.print("0x01f400 = 0.500V") 02239 // docTest_item['actionType'] = 'print-string' 02240 // docTest_item['group-id-value'] = 'CODE_LOAD' 02241 // docTest_item['action'] = 'tinyTester.print("0x01f400 = 0.500V")' 02242 // docTest_item['arglist'] = '0x01f400 = 0.500V' 02243 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02244 if (SelfTestGroupEnable & 0x0004) { 02245 // print-string 02246 // tinyTesterFuncName = "tinyTester.print" 02247 // tinyTesterPrintStringLiteral = "0x01f400 = 0.500V" 02248 tinyTester.print("0x01f400 = 0.500V"); 02249 // halt-on-first-failure logic 02250 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02251 } // if (SelfTestGroupEnable & 0x0004) 02252 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02253 02254 // @test group CODE_LOAD CODE_LOAD(0x01f400) // 0.500V 02255 // docTest_item['actionType'] = 'call-function' 02256 // docTest_item['group-id-value'] = 'CODE_LOAD' 02257 // docTest_item['action'] = 'CODE_LOAD(0x01f400)' 02258 // docTest_item['remarks'] = '0.500V' 02259 // docTest_item['funcName'] = 'CODE_LOAD' 02260 // docTest_item['arglist'] = '0x01f400' 02261 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD CODE_LOAD 0.500V 02262 if (SelfTestGroupEnable & 0x0004) { 02263 // call-function 02264 // selfTestFunctionClosures['CODE_LOAD']['returnType'] = 'uint8_t' 02265 // ASSERT_EQ(g_MAX5719_device.CODE_LOAD((uint32_t)0x01f400), (uint8_t)None); // 0.500V 02266 // tinyTester.FunctionCall_Expect("MAX5719.CODE_LOAD", fn_MAX5719_CODE_LOAD, (uint32_t)0x01f400, /* empty expect: */ (uint8_t)None); // 0.500V 02267 g_MAX5719_device.CODE_LOAD((uint32_t)0x01f400); // 0.500V 02268 // halt-on-first-failure logic 02269 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02270 } // if (SelfTestGroupEnable & 0x0004) 02271 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02272 02273 // @test group CODE_LOAD tinyTester.Wait_Output_Settling() 02274 // docTest_item['actionType'] = 'call-tinytester-function' 02275 // docTest_item['group-id-value'] = 'CODE_LOAD' 02276 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 02277 // docTest_item['propName'] = 'Wait_Output_Settling' 02278 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02279 if (SelfTestGroupEnable & 0x0004) { 02280 // call-tinytester-function 02281 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 02282 // docTest_argList = "" 02283 tinyTester.Wait_Output_Settling(); // 02284 // halt-on-first-failure logic 02285 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02286 } // if (SelfTestGroupEnable & 0x0004) 02287 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02288 02289 // @test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(0.500000) 02290 // docTest_item['actionType'] = 'call-tinytester-function' 02291 // docTest_item['group-id-value'] = 'CODE_LOAD' 02292 // docTest_item['action'] = 'tinyTester.AnalogIn0_Read_Expect_voltageV(0.500000)' 02293 // docTest_item['arglist'] = '0.500000' 02294 // docTest_item['propName'] = 'AnalogIn0_Read_Expect_voltageV' 02295 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02296 if (SelfTestGroupEnable & 0x0004) { 02297 // call-tinytester-function 02298 // tinyTesterFuncName = "tinyTester.AnalogIn0_Read_Expect_voltageV" 02299 // docTest_argList = "0.500000" 02300 tinyTester.AnalogIn0_Read_Expect_voltageV(0.500000); // 02301 // halt-on-first-failure logic 02302 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02303 } // if (SelfTestGroupEnable & 0x0004) 02304 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02305 02306 // @test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 02307 // docTest_item['actionType'] = 'call-tinytester-function' 02308 // docTest_item['group-id-value'] = 'CODE_LOAD' 02309 // docTest_item['action'] = 'tinyTester.AnalogIn1_Read_Report_voltageV()' 02310 // docTest_item['propName'] = 'AnalogIn1_Read_Report_voltageV' 02311 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02312 if (SelfTestGroupEnable & 0x0004) { 02313 // call-tinytester-function 02314 // tinyTesterFuncName = "tinyTester.AnalogIn1_Read_Report_voltageV" 02315 // docTest_argList = "" 02316 tinyTester.AnalogIn1_Read_Report_voltageV(); // 02317 // halt-on-first-failure logic 02318 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02319 } // if (SelfTestGroupEnable & 0x0004) 02320 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02321 02322 // @test group CODE_LOAD tinyTester.print("0x03e800 = 1.000V") 02323 // docTest_item['actionType'] = 'print-string' 02324 // docTest_item['group-id-value'] = 'CODE_LOAD' 02325 // docTest_item['action'] = 'tinyTester.print("0x03e800 = 1.000V")' 02326 // docTest_item['arglist'] = '0x03e800 = 1.000V' 02327 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02328 if (SelfTestGroupEnable & 0x0004) { 02329 // print-string 02330 // tinyTesterFuncName = "tinyTester.print" 02331 // tinyTesterPrintStringLiteral = "0x03e800 = 1.000V" 02332 tinyTester.print("0x03e800 = 1.000V"); 02333 // halt-on-first-failure logic 02334 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02335 } // if (SelfTestGroupEnable & 0x0004) 02336 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02337 02338 // @test group CODE_LOAD CODE_LOAD(0x03e800) // 1.000V 02339 // docTest_item['actionType'] = 'call-function' 02340 // docTest_item['group-id-value'] = 'CODE_LOAD' 02341 // docTest_item['action'] = 'CODE_LOAD(0x03e800)' 02342 // docTest_item['remarks'] = '1.000V' 02343 // docTest_item['funcName'] = 'CODE_LOAD' 02344 // docTest_item['arglist'] = '0x03e800' 02345 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD CODE_LOAD 1.000V 02346 if (SelfTestGroupEnable & 0x0004) { 02347 // call-function 02348 // selfTestFunctionClosures['CODE_LOAD']['returnType'] = 'uint8_t' 02349 // ASSERT_EQ(g_MAX5719_device.CODE_LOAD((uint32_t)0x03e800), (uint8_t)None); // 1.000V 02350 // tinyTester.FunctionCall_Expect("MAX5719.CODE_LOAD", fn_MAX5719_CODE_LOAD, (uint32_t)0x03e800, /* empty expect: */ (uint8_t)None); // 1.000V 02351 g_MAX5719_device.CODE_LOAD((uint32_t)0x03e800); // 1.000V 02352 // halt-on-first-failure logic 02353 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02354 } // if (SelfTestGroupEnable & 0x0004) 02355 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02356 02357 // @test group CODE_LOAD tinyTester.Wait_Output_Settling() 02358 // docTest_item['actionType'] = 'call-tinytester-function' 02359 // docTest_item['group-id-value'] = 'CODE_LOAD' 02360 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 02361 // docTest_item['propName'] = 'Wait_Output_Settling' 02362 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02363 if (SelfTestGroupEnable & 0x0004) { 02364 // call-tinytester-function 02365 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 02366 // docTest_argList = "" 02367 tinyTester.Wait_Output_Settling(); // 02368 // halt-on-first-failure logic 02369 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02370 } // if (SelfTestGroupEnable & 0x0004) 02371 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02372 02373 // @test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(1.000000) 02374 // docTest_item['actionType'] = 'call-tinytester-function' 02375 // docTest_item['group-id-value'] = 'CODE_LOAD' 02376 // docTest_item['action'] = 'tinyTester.AnalogIn0_Read_Expect_voltageV(1.000000)' 02377 // docTest_item['arglist'] = '1.000000' 02378 // docTest_item['propName'] = 'AnalogIn0_Read_Expect_voltageV' 02379 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02380 if (SelfTestGroupEnable & 0x0004) { 02381 // call-tinytester-function 02382 // tinyTesterFuncName = "tinyTester.AnalogIn0_Read_Expect_voltageV" 02383 // docTest_argList = "1.000000" 02384 tinyTester.AnalogIn0_Read_Expect_voltageV(1.000000); // 02385 // halt-on-first-failure logic 02386 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02387 } // if (SelfTestGroupEnable & 0x0004) 02388 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02389 02390 // @test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 02391 // docTest_item['actionType'] = 'call-tinytester-function' 02392 // docTest_item['group-id-value'] = 'CODE_LOAD' 02393 // docTest_item['action'] = 'tinyTester.AnalogIn1_Read_Report_voltageV()' 02394 // docTest_item['propName'] = 'AnalogIn1_Read_Report_voltageV' 02395 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02396 if (SelfTestGroupEnable & 0x0004) { 02397 // call-tinytester-function 02398 // tinyTesterFuncName = "tinyTester.AnalogIn1_Read_Report_voltageV" 02399 // docTest_argList = "" 02400 tinyTester.AnalogIn1_Read_Report_voltageV(); // 02401 // halt-on-first-failure logic 02402 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02403 } // if (SelfTestGroupEnable & 0x0004) 02404 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02405 02406 // @test group CODE_LOAD tinyTester.err_threshold = 0.075 02407 // docTest_item['actionType'] = 'assign-propname-value' 02408 // docTest_item['group-id-value'] = 'CODE_LOAD' 02409 // docTest_item['action'] = 'tinyTester.err_threshold = 0.075' 02410 // docTest_item['propName'] = 'tinyTester.err_threshold' 02411 // docTest_item['propValue'] = '0.075' 02412 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02413 if (SelfTestGroupEnable & 0x0004) { 02414 // assign-propname-value 02415 // tinyTesterPropName = "tinyTester.err_threshold" 02416 // tinyTesterPropValue = "0.075" 02417 tinyTester.err_threshold = 0.075; 02418 // halt-on-first-failure logic 02419 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02420 } // if (SelfTestGroupEnable & 0x0004) 02421 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02422 02423 // @test group CODE_LOAD tinyTester.print("0x05dc00 = 1.500V") 02424 // docTest_item['actionType'] = 'print-string' 02425 // docTest_item['group-id-value'] = 'CODE_LOAD' 02426 // docTest_item['action'] = 'tinyTester.print("0x05dc00 = 1.500V")' 02427 // docTest_item['arglist'] = '0x05dc00 = 1.500V' 02428 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02429 if (SelfTestGroupEnable & 0x0004) { 02430 // print-string 02431 // tinyTesterFuncName = "tinyTester.print" 02432 // tinyTesterPrintStringLiteral = "0x05dc00 = 1.500V" 02433 tinyTester.print("0x05dc00 = 1.500V"); 02434 // halt-on-first-failure logic 02435 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02436 } // if (SelfTestGroupEnable & 0x0004) 02437 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02438 02439 // @test group CODE_LOAD CODE_LOAD(0x05dc00) // 1.500V 02440 // docTest_item['actionType'] = 'call-function' 02441 // docTest_item['group-id-value'] = 'CODE_LOAD' 02442 // docTest_item['action'] = 'CODE_LOAD(0x05dc00)' 02443 // docTest_item['remarks'] = '1.500V' 02444 // docTest_item['funcName'] = 'CODE_LOAD' 02445 // docTest_item['arglist'] = '0x05dc00' 02446 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD CODE_LOAD 1.500V 02447 if (SelfTestGroupEnable & 0x0004) { 02448 // call-function 02449 // selfTestFunctionClosures['CODE_LOAD']['returnType'] = 'uint8_t' 02450 // ASSERT_EQ(g_MAX5719_device.CODE_LOAD((uint32_t)0x05dc00), (uint8_t)None); // 1.500V 02451 // tinyTester.FunctionCall_Expect("MAX5719.CODE_LOAD", fn_MAX5719_CODE_LOAD, (uint32_t)0x05dc00, /* empty expect: */ (uint8_t)None); // 1.500V 02452 g_MAX5719_device.CODE_LOAD((uint32_t)0x05dc00); // 1.500V 02453 // halt-on-first-failure logic 02454 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02455 } // if (SelfTestGroupEnable & 0x0004) 02456 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02457 02458 // @test group CODE_LOAD tinyTester.Wait_Output_Settling() 02459 // docTest_item['actionType'] = 'call-tinytester-function' 02460 // docTest_item['group-id-value'] = 'CODE_LOAD' 02461 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 02462 // docTest_item['propName'] = 'Wait_Output_Settling' 02463 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02464 if (SelfTestGroupEnable & 0x0004) { 02465 // call-tinytester-function 02466 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 02467 // docTest_argList = "" 02468 tinyTester.Wait_Output_Settling(); // 02469 // halt-on-first-failure logic 02470 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02471 } // if (SelfTestGroupEnable & 0x0004) 02472 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02473 02474 // @test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(1.500000) 02475 // docTest_item['actionType'] = 'call-tinytester-function' 02476 // docTest_item['group-id-value'] = 'CODE_LOAD' 02477 // docTest_item['action'] = 'tinyTester.AnalogIn0_Read_Expect_voltageV(1.500000)' 02478 // docTest_item['arglist'] = '1.500000' 02479 // docTest_item['propName'] = 'AnalogIn0_Read_Expect_voltageV' 02480 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02481 if (SelfTestGroupEnable & 0x0004) { 02482 // call-tinytester-function 02483 // tinyTesterFuncName = "tinyTester.AnalogIn0_Read_Expect_voltageV" 02484 // docTest_argList = "1.500000" 02485 tinyTester.AnalogIn0_Read_Expect_voltageV(1.500000); // 02486 // halt-on-first-failure logic 02487 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02488 } // if (SelfTestGroupEnable & 0x0004) 02489 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02490 02491 // @test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 02492 // docTest_item['actionType'] = 'call-tinytester-function' 02493 // docTest_item['group-id-value'] = 'CODE_LOAD' 02494 // docTest_item['action'] = 'tinyTester.AnalogIn1_Read_Report_voltageV()' 02495 // docTest_item['propName'] = 'AnalogIn1_Read_Report_voltageV' 02496 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02497 if (SelfTestGroupEnable & 0x0004) { 02498 // call-tinytester-function 02499 // tinyTesterFuncName = "tinyTester.AnalogIn1_Read_Report_voltageV" 02500 // docTest_argList = "" 02501 tinyTester.AnalogIn1_Read_Report_voltageV(); // 02502 // halt-on-first-failure logic 02503 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02504 } // if (SelfTestGroupEnable & 0x0004) 02505 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02506 02507 // @test group CODE_LOAD tinyTester.err_threshold = 0.100 02508 // docTest_item['actionType'] = 'assign-propname-value' 02509 // docTest_item['group-id-value'] = 'CODE_LOAD' 02510 // docTest_item['action'] = 'tinyTester.err_threshold = 0.100' 02511 // docTest_item['propName'] = 'tinyTester.err_threshold' 02512 // docTest_item['propValue'] = '0.100' 02513 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02514 if (SelfTestGroupEnable & 0x0004) { 02515 // assign-propname-value 02516 // tinyTesterPropName = "tinyTester.err_threshold" 02517 // tinyTesterPropValue = "0.100" 02518 tinyTester.err_threshold = 0.100; 02519 // halt-on-first-failure logic 02520 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02521 } // if (SelfTestGroupEnable & 0x0004) 02522 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02523 02524 // @test group CODE_LOAD tinyTester.print("0x07d000 = 2.000V") 02525 // docTest_item['actionType'] = 'print-string' 02526 // docTest_item['group-id-value'] = 'CODE_LOAD' 02527 // docTest_item['action'] = 'tinyTester.print("0x07d000 = 2.000V")' 02528 // docTest_item['arglist'] = '0x07d000 = 2.000V' 02529 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02530 if (SelfTestGroupEnable & 0x0004) { 02531 // print-string 02532 // tinyTesterFuncName = "tinyTester.print" 02533 // tinyTesterPrintStringLiteral = "0x07d000 = 2.000V" 02534 tinyTester.print("0x07d000 = 2.000V"); 02535 // halt-on-first-failure logic 02536 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02537 } // if (SelfTestGroupEnable & 0x0004) 02538 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02539 02540 // @test group CODE_LOAD CODE_LOAD(0x07d000) // 2.000V 02541 // docTest_item['actionType'] = 'call-function' 02542 // docTest_item['group-id-value'] = 'CODE_LOAD' 02543 // docTest_item['action'] = 'CODE_LOAD(0x07d000)' 02544 // docTest_item['remarks'] = '2.000V' 02545 // docTest_item['funcName'] = 'CODE_LOAD' 02546 // docTest_item['arglist'] = '0x07d000' 02547 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD CODE_LOAD 2.000V 02548 if (SelfTestGroupEnable & 0x0004) { 02549 // call-function 02550 // selfTestFunctionClosures['CODE_LOAD']['returnType'] = 'uint8_t' 02551 // ASSERT_EQ(g_MAX5719_device.CODE_LOAD((uint32_t)0x07d000), (uint8_t)None); // 2.000V 02552 // tinyTester.FunctionCall_Expect("MAX5719.CODE_LOAD", fn_MAX5719_CODE_LOAD, (uint32_t)0x07d000, /* empty expect: */ (uint8_t)None); // 2.000V 02553 g_MAX5719_device.CODE_LOAD((uint32_t)0x07d000); // 2.000V 02554 // halt-on-first-failure logic 02555 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02556 } // if (SelfTestGroupEnable & 0x0004) 02557 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02558 02559 // @test group CODE_LOAD tinyTester.Wait_Output_Settling() 02560 // docTest_item['actionType'] = 'call-tinytester-function' 02561 // docTest_item['group-id-value'] = 'CODE_LOAD' 02562 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 02563 // docTest_item['propName'] = 'Wait_Output_Settling' 02564 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02565 if (SelfTestGroupEnable & 0x0004) { 02566 // call-tinytester-function 02567 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 02568 // docTest_argList = "" 02569 tinyTester.Wait_Output_Settling(); // 02570 // halt-on-first-failure logic 02571 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02572 } // if (SelfTestGroupEnable & 0x0004) 02573 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02574 02575 // @test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(2.000000) 02576 // docTest_item['actionType'] = 'call-tinytester-function' 02577 // docTest_item['group-id-value'] = 'CODE_LOAD' 02578 // docTest_item['action'] = 'tinyTester.AnalogIn0_Read_Expect_voltageV(2.000000)' 02579 // docTest_item['arglist'] = '2.000000' 02580 // docTest_item['propName'] = 'AnalogIn0_Read_Expect_voltageV' 02581 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02582 if (SelfTestGroupEnable & 0x0004) { 02583 // call-tinytester-function 02584 // tinyTesterFuncName = "tinyTester.AnalogIn0_Read_Expect_voltageV" 02585 // docTest_argList = "2.000000" 02586 tinyTester.AnalogIn0_Read_Expect_voltageV(2.000000); // 02587 // halt-on-first-failure logic 02588 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02589 } // if (SelfTestGroupEnable & 0x0004) 02590 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02591 02592 // @test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 02593 // docTest_item['actionType'] = 'call-tinytester-function' 02594 // docTest_item['group-id-value'] = 'CODE_LOAD' 02595 // docTest_item['action'] = 'tinyTester.AnalogIn1_Read_Report_voltageV()' 02596 // docTest_item['propName'] = 'AnalogIn1_Read_Report_voltageV' 02597 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02598 if (SelfTestGroupEnable & 0x0004) { 02599 // call-tinytester-function 02600 // tinyTesterFuncName = "tinyTester.AnalogIn1_Read_Report_voltageV" 02601 // docTest_argList = "" 02602 tinyTester.AnalogIn1_Read_Report_voltageV(); // 02603 // halt-on-first-failure logic 02604 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02605 } // if (SelfTestGroupEnable & 0x0004) 02606 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02607 02608 // @test group CODE_LOAD tinyTester.err_threshold = 0.150 02609 // docTest_item['actionType'] = 'assign-propname-value' 02610 // docTest_item['group-id-value'] = 'CODE_LOAD' 02611 // docTest_item['action'] = 'tinyTester.err_threshold = 0.150' 02612 // docTest_item['propName'] = 'tinyTester.err_threshold' 02613 // docTest_item['propValue'] = '0.150' 02614 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02615 if (SelfTestGroupEnable & 0x0004) { 02616 // assign-propname-value 02617 // tinyTesterPropName = "tinyTester.err_threshold" 02618 // tinyTesterPropValue = "0.150" 02619 tinyTester.err_threshold = 0.150; 02620 // halt-on-first-failure logic 02621 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02622 } // if (SelfTestGroupEnable & 0x0004) 02623 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02624 02625 // @test group CODE_LOAD tinyTester.print("0x09c400 = 2.500V") 02626 // docTest_item['actionType'] = 'print-string' 02627 // docTest_item['group-id-value'] = 'CODE_LOAD' 02628 // docTest_item['action'] = 'tinyTester.print("0x09c400 = 2.500V")' 02629 // docTest_item['arglist'] = '0x09c400 = 2.500V' 02630 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02631 if (SelfTestGroupEnable & 0x0004) { 02632 // print-string 02633 // tinyTesterFuncName = "tinyTester.print" 02634 // tinyTesterPrintStringLiteral = "0x09c400 = 2.500V" 02635 tinyTester.print("0x09c400 = 2.500V"); 02636 // halt-on-first-failure logic 02637 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02638 } // if (SelfTestGroupEnable & 0x0004) 02639 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02640 02641 // @test group CODE_LOAD CODE_LOAD(0x09c400) // 2.500V 02642 // docTest_item['actionType'] = 'call-function' 02643 // docTest_item['group-id-value'] = 'CODE_LOAD' 02644 // docTest_item['action'] = 'CODE_LOAD(0x09c400)' 02645 // docTest_item['remarks'] = '2.500V' 02646 // docTest_item['funcName'] = 'CODE_LOAD' 02647 // docTest_item['arglist'] = '0x09c400' 02648 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD CODE_LOAD 2.500V 02649 if (SelfTestGroupEnable & 0x0004) { 02650 // call-function 02651 // selfTestFunctionClosures['CODE_LOAD']['returnType'] = 'uint8_t' 02652 // ASSERT_EQ(g_MAX5719_device.CODE_LOAD((uint32_t)0x09c400), (uint8_t)None); // 2.500V 02653 // tinyTester.FunctionCall_Expect("MAX5719.CODE_LOAD", fn_MAX5719_CODE_LOAD, (uint32_t)0x09c400, /* empty expect: */ (uint8_t)None); // 2.500V 02654 g_MAX5719_device.CODE_LOAD((uint32_t)0x09c400); // 2.500V 02655 // halt-on-first-failure logic 02656 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02657 } // if (SelfTestGroupEnable & 0x0004) 02658 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02659 02660 // @test group CODE_LOAD tinyTester.Wait_Output_Settling() 02661 // docTest_item['actionType'] = 'call-tinytester-function' 02662 // docTest_item['group-id-value'] = 'CODE_LOAD' 02663 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 02664 // docTest_item['propName'] = 'Wait_Output_Settling' 02665 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02666 if (SelfTestGroupEnable & 0x0004) { 02667 // call-tinytester-function 02668 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 02669 // docTest_argList = "" 02670 tinyTester.Wait_Output_Settling(); // 02671 // halt-on-first-failure logic 02672 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02673 } // if (SelfTestGroupEnable & 0x0004) 02674 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02675 02676 // @test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(2.500000) 02677 // docTest_item['actionType'] = 'call-tinytester-function' 02678 // docTest_item['group-id-value'] = 'CODE_LOAD' 02679 // docTest_item['action'] = 'tinyTester.AnalogIn0_Read_Expect_voltageV(2.500000)' 02680 // docTest_item['arglist'] = '2.500000' 02681 // docTest_item['propName'] = 'AnalogIn0_Read_Expect_voltageV' 02682 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02683 if (SelfTestGroupEnable & 0x0004) { 02684 // call-tinytester-function 02685 // tinyTesterFuncName = "tinyTester.AnalogIn0_Read_Expect_voltageV" 02686 // docTest_argList = "2.500000" 02687 tinyTester.AnalogIn0_Read_Expect_voltageV(2.500000); // 02688 // halt-on-first-failure logic 02689 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02690 } // if (SelfTestGroupEnable & 0x0004) 02691 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02692 02693 // @test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 02694 // docTest_item['actionType'] = 'call-tinytester-function' 02695 // docTest_item['group-id-value'] = 'CODE_LOAD' 02696 // docTest_item['action'] = 'tinyTester.AnalogIn1_Read_Report_voltageV()' 02697 // docTest_item['propName'] = 'AnalogIn1_Read_Report_voltageV' 02698 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02699 if (SelfTestGroupEnable & 0x0004) { 02700 // call-tinytester-function 02701 // tinyTesterFuncName = "tinyTester.AnalogIn1_Read_Report_voltageV" 02702 // docTest_argList = "" 02703 tinyTester.AnalogIn1_Read_Report_voltageV(); // 02704 // halt-on-first-failure logic 02705 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02706 } // if (SelfTestGroupEnable & 0x0004) 02707 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02708 02709 // @test group CODE_LOAD tinyTester.err_threshold = 0.200 02710 // docTest_item['actionType'] = 'assign-propname-value' 02711 // docTest_item['group-id-value'] = 'CODE_LOAD' 02712 // docTest_item['action'] = 'tinyTester.err_threshold = 0.200' 02713 // docTest_item['propName'] = 'tinyTester.err_threshold' 02714 // docTest_item['propValue'] = '0.200' 02715 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02716 if (SelfTestGroupEnable & 0x0004) { 02717 // assign-propname-value 02718 // tinyTesterPropName = "tinyTester.err_threshold" 02719 // tinyTesterPropValue = "0.200" 02720 tinyTester.err_threshold = 0.200; 02721 // halt-on-first-failure logic 02722 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02723 } // if (SelfTestGroupEnable & 0x0004) 02724 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02725 02726 // @test group CODE_LOAD tinyTester.print("0x0bb800 = 3.000V") 02727 // docTest_item['actionType'] = 'print-string' 02728 // docTest_item['group-id-value'] = 'CODE_LOAD' 02729 // docTest_item['action'] = 'tinyTester.print("0x0bb800 = 3.000V")' 02730 // docTest_item['arglist'] = '0x0bb800 = 3.000V' 02731 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02732 if (SelfTestGroupEnable & 0x0004) { 02733 // print-string 02734 // tinyTesterFuncName = "tinyTester.print" 02735 // tinyTesterPrintStringLiteral = "0x0bb800 = 3.000V" 02736 tinyTester.print("0x0bb800 = 3.000V"); 02737 // halt-on-first-failure logic 02738 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02739 } // if (SelfTestGroupEnable & 0x0004) 02740 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02741 02742 // @test group CODE_LOAD CODE_LOAD(0x0bb800) // 3.000V 02743 // docTest_item['actionType'] = 'call-function' 02744 // docTest_item['group-id-value'] = 'CODE_LOAD' 02745 // docTest_item['action'] = 'CODE_LOAD(0x0bb800)' 02746 // docTest_item['remarks'] = '3.000V' 02747 // docTest_item['funcName'] = 'CODE_LOAD' 02748 // docTest_item['arglist'] = '0x0bb800' 02749 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD CODE_LOAD 3.000V 02750 if (SelfTestGroupEnable & 0x0004) { 02751 // call-function 02752 // selfTestFunctionClosures['CODE_LOAD']['returnType'] = 'uint8_t' 02753 // ASSERT_EQ(g_MAX5719_device.CODE_LOAD((uint32_t)0x0bb800), (uint8_t)None); // 3.000V 02754 // tinyTester.FunctionCall_Expect("MAX5719.CODE_LOAD", fn_MAX5719_CODE_LOAD, (uint32_t)0x0bb800, /* empty expect: */ (uint8_t)None); // 3.000V 02755 g_MAX5719_device.CODE_LOAD((uint32_t)0x0bb800); // 3.000V 02756 // halt-on-first-failure logic 02757 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02758 } // if (SelfTestGroupEnable & 0x0004) 02759 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02760 02761 // @test group CODE_LOAD tinyTester.Wait_Output_Settling() 02762 // docTest_item['actionType'] = 'call-tinytester-function' 02763 // docTest_item['group-id-value'] = 'CODE_LOAD' 02764 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 02765 // docTest_item['propName'] = 'Wait_Output_Settling' 02766 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02767 if (SelfTestGroupEnable & 0x0004) { 02768 // call-tinytester-function 02769 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 02770 // docTest_argList = "" 02771 tinyTester.Wait_Output_Settling(); // 02772 // halt-on-first-failure logic 02773 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02774 } // if (SelfTestGroupEnable & 0x0004) 02775 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02776 02777 // @test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(3.000000) 02778 // docTest_item['actionType'] = 'call-tinytester-function' 02779 // docTest_item['group-id-value'] = 'CODE_LOAD' 02780 // docTest_item['action'] = 'tinyTester.AnalogIn0_Read_Expect_voltageV(3.000000)' 02781 // docTest_item['arglist'] = '3.000000' 02782 // docTest_item['propName'] = 'AnalogIn0_Read_Expect_voltageV' 02783 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02784 if (SelfTestGroupEnable & 0x0004) { 02785 // call-tinytester-function 02786 // tinyTesterFuncName = "tinyTester.AnalogIn0_Read_Expect_voltageV" 02787 // docTest_argList = "3.000000" 02788 tinyTester.AnalogIn0_Read_Expect_voltageV(3.000000); // 02789 // halt-on-first-failure logic 02790 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02791 } // if (SelfTestGroupEnable & 0x0004) 02792 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02793 02794 // @test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 02795 // docTest_item['actionType'] = 'call-tinytester-function' 02796 // docTest_item['group-id-value'] = 'CODE_LOAD' 02797 // docTest_item['action'] = 'tinyTester.AnalogIn1_Read_Report_voltageV()' 02798 // docTest_item['propName'] = 'AnalogIn1_Read_Report_voltageV' 02799 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02800 if (SelfTestGroupEnable & 0x0004) { 02801 // call-tinytester-function 02802 // tinyTesterFuncName = "tinyTester.AnalogIn1_Read_Report_voltageV" 02803 // docTest_argList = "" 02804 tinyTester.AnalogIn1_Read_Report_voltageV(); // 02805 // halt-on-first-failure logic 02806 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02807 } // if (SelfTestGroupEnable & 0x0004) 02808 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02809 02810 // @test group CODE_LOAD tinyTester.err_threshold = 0.250 02811 // docTest_item['actionType'] = 'assign-propname-value' 02812 // docTest_item['group-id-value'] = 'CODE_LOAD' 02813 // docTest_item['action'] = 'tinyTester.err_threshold = 0.250' 02814 // docTest_item['propName'] = 'tinyTester.err_threshold' 02815 // docTest_item['propValue'] = '0.250' 02816 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02817 if (SelfTestGroupEnable & 0x0004) { 02818 // assign-propname-value 02819 // tinyTesterPropName = "tinyTester.err_threshold" 02820 // tinyTesterPropValue = "0.250" 02821 tinyTester.err_threshold = 0.250; 02822 // halt-on-first-failure logic 02823 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02824 } // if (SelfTestGroupEnable & 0x0004) 02825 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02826 02827 // @test group CODE_LOAD tinyTester.print("0x0dac00 = 3.500V") 02828 // docTest_item['actionType'] = 'print-string' 02829 // docTest_item['group-id-value'] = 'CODE_LOAD' 02830 // docTest_item['action'] = 'tinyTester.print("0x0dac00 = 3.500V")' 02831 // docTest_item['arglist'] = '0x0dac00 = 3.500V' 02832 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02833 if (SelfTestGroupEnable & 0x0004) { 02834 // print-string 02835 // tinyTesterFuncName = "tinyTester.print" 02836 // tinyTesterPrintStringLiteral = "0x0dac00 = 3.500V" 02837 tinyTester.print("0x0dac00 = 3.500V"); 02838 // halt-on-first-failure logic 02839 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02840 } // if (SelfTestGroupEnable & 0x0004) 02841 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02842 02843 // @test group CODE_LOAD CODE_LOAD(0x0dac00) // 3.500V 02844 // docTest_item['actionType'] = 'call-function' 02845 // docTest_item['group-id-value'] = 'CODE_LOAD' 02846 // docTest_item['action'] = 'CODE_LOAD(0x0dac00)' 02847 // docTest_item['remarks'] = '3.500V' 02848 // docTest_item['funcName'] = 'CODE_LOAD' 02849 // docTest_item['arglist'] = '0x0dac00' 02850 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD CODE_LOAD 3.500V 02851 if (SelfTestGroupEnable & 0x0004) { 02852 // call-function 02853 // selfTestFunctionClosures['CODE_LOAD']['returnType'] = 'uint8_t' 02854 // ASSERT_EQ(g_MAX5719_device.CODE_LOAD((uint32_t)0x0dac00), (uint8_t)None); // 3.500V 02855 // tinyTester.FunctionCall_Expect("MAX5719.CODE_LOAD", fn_MAX5719_CODE_LOAD, (uint32_t)0x0dac00, /* empty expect: */ (uint8_t)None); // 3.500V 02856 g_MAX5719_device.CODE_LOAD((uint32_t)0x0dac00); // 3.500V 02857 // halt-on-first-failure logic 02858 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02859 } // if (SelfTestGroupEnable & 0x0004) 02860 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02861 02862 // @test group CODE_LOAD tinyTester.Wait_Output_Settling() 02863 // docTest_item['actionType'] = 'call-tinytester-function' 02864 // docTest_item['group-id-value'] = 'CODE_LOAD' 02865 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 02866 // docTest_item['propName'] = 'Wait_Output_Settling' 02867 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02868 if (SelfTestGroupEnable & 0x0004) { 02869 // call-tinytester-function 02870 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 02871 // docTest_argList = "" 02872 tinyTester.Wait_Output_Settling(); // 02873 // halt-on-first-failure logic 02874 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02875 } // if (SelfTestGroupEnable & 0x0004) 02876 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02877 02878 // @test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(3.500000) 02879 // docTest_item['actionType'] = 'call-tinytester-function' 02880 // docTest_item['group-id-value'] = 'CODE_LOAD' 02881 // docTest_item['action'] = 'tinyTester.AnalogIn0_Read_Expect_voltageV(3.500000)' 02882 // docTest_item['arglist'] = '3.500000' 02883 // docTest_item['propName'] = 'AnalogIn0_Read_Expect_voltageV' 02884 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02885 if (SelfTestGroupEnable & 0x0004) { 02886 // call-tinytester-function 02887 // tinyTesterFuncName = "tinyTester.AnalogIn0_Read_Expect_voltageV" 02888 // docTest_argList = "3.500000" 02889 tinyTester.AnalogIn0_Read_Expect_voltageV(3.500000); // 02890 // halt-on-first-failure logic 02891 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02892 } // if (SelfTestGroupEnable & 0x0004) 02893 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02894 02895 // @test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 02896 // docTest_item['actionType'] = 'call-tinytester-function' 02897 // docTest_item['group-id-value'] = 'CODE_LOAD' 02898 // docTest_item['action'] = 'tinyTester.AnalogIn1_Read_Report_voltageV()' 02899 // docTest_item['propName'] = 'AnalogIn1_Read_Report_voltageV' 02900 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02901 if (SelfTestGroupEnable & 0x0004) { 02902 // call-tinytester-function 02903 // tinyTesterFuncName = "tinyTester.AnalogIn1_Read_Report_voltageV" 02904 // docTest_argList = "" 02905 tinyTester.AnalogIn1_Read_Report_voltageV(); // 02906 // halt-on-first-failure logic 02907 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02908 } // if (SelfTestGroupEnable & 0x0004) 02909 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02910 02911 // @test group CODE_LOAD tinyTester.err_threshold = 0.500 02912 // docTest_item['actionType'] = 'assign-propname-value' 02913 // docTest_item['group-id-value'] = 'CODE_LOAD' 02914 // docTest_item['action'] = 'tinyTester.err_threshold = 0.500' 02915 // docTest_item['propName'] = 'tinyTester.err_threshold' 02916 // docTest_item['propValue'] = '0.500' 02917 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02918 if (SelfTestGroupEnable & 0x0004) { 02919 // assign-propname-value 02920 // tinyTesterPropName = "tinyTester.err_threshold" 02921 // tinyTesterPropValue = "0.500" 02922 tinyTester.err_threshold = 0.500; 02923 // halt-on-first-failure logic 02924 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02925 } // if (SelfTestGroupEnable & 0x0004) 02926 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02927 02928 // @test group CODE_LOAD tinyTester.print("0x0fa000 = 4.000V") 02929 // docTest_item['actionType'] = 'print-string' 02930 // docTest_item['group-id-value'] = 'CODE_LOAD' 02931 // docTest_item['action'] = 'tinyTester.print("0x0fa000 = 4.000V")' 02932 // docTest_item['arglist'] = '0x0fa000 = 4.000V' 02933 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02934 if (SelfTestGroupEnable & 0x0004) { 02935 // print-string 02936 // tinyTesterFuncName = "tinyTester.print" 02937 // tinyTesterPrintStringLiteral = "0x0fa000 = 4.000V" 02938 tinyTester.print("0x0fa000 = 4.000V"); 02939 // halt-on-first-failure logic 02940 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02941 } // if (SelfTestGroupEnable & 0x0004) 02942 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02943 02944 // @test group CODE_LOAD CODE_LOAD(0x0fa000) // 4.000V 02945 // docTest_item['actionType'] = 'call-function' 02946 // docTest_item['group-id-value'] = 'CODE_LOAD' 02947 // docTest_item['action'] = 'CODE_LOAD(0x0fa000)' 02948 // docTest_item['remarks'] = '4.000V' 02949 // docTest_item['funcName'] = 'CODE_LOAD' 02950 // docTest_item['arglist'] = '0x0fa000' 02951 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD CODE_LOAD 4.000V 02952 if (SelfTestGroupEnable & 0x0004) { 02953 // call-function 02954 // selfTestFunctionClosures['CODE_LOAD']['returnType'] = 'uint8_t' 02955 // ASSERT_EQ(g_MAX5719_device.CODE_LOAD((uint32_t)0x0fa000), (uint8_t)None); // 4.000V 02956 // tinyTester.FunctionCall_Expect("MAX5719.CODE_LOAD", fn_MAX5719_CODE_LOAD, (uint32_t)0x0fa000, /* empty expect: */ (uint8_t)None); // 4.000V 02957 g_MAX5719_device.CODE_LOAD((uint32_t)0x0fa000); // 4.000V 02958 // halt-on-first-failure logic 02959 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02960 } // if (SelfTestGroupEnable & 0x0004) 02961 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02962 02963 // @test group CODE_LOAD tinyTester.Wait_Output_Settling() 02964 // docTest_item['actionType'] = 'call-tinytester-function' 02965 // docTest_item['group-id-value'] = 'CODE_LOAD' 02966 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 02967 // docTest_item['propName'] = 'Wait_Output_Settling' 02968 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02969 if (SelfTestGroupEnable & 0x0004) { 02970 // call-tinytester-function 02971 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 02972 // docTest_argList = "" 02973 tinyTester.Wait_Output_Settling(); // 02974 // halt-on-first-failure logic 02975 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02976 } // if (SelfTestGroupEnable & 0x0004) 02977 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02978 02979 // @test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(4.000000) 02980 // docTest_item['actionType'] = 'call-tinytester-function' 02981 // docTest_item['group-id-value'] = 'CODE_LOAD' 02982 // docTest_item['action'] = 'tinyTester.AnalogIn0_Read_Expect_voltageV(4.000000)' 02983 // docTest_item['arglist'] = '4.000000' 02984 // docTest_item['propName'] = 'AnalogIn0_Read_Expect_voltageV' 02985 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 02986 if (SelfTestGroupEnable & 0x0004) { 02987 // call-tinytester-function 02988 // tinyTesterFuncName = "tinyTester.AnalogIn0_Read_Expect_voltageV" 02989 // docTest_argList = "4.000000" 02990 tinyTester.AnalogIn0_Read_Expect_voltageV(4.000000); // 02991 // halt-on-first-failure logic 02992 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 02993 } // if (SelfTestGroupEnable & 0x0004) 02994 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 02995 02996 // @test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 02997 // docTest_item['actionType'] = 'call-tinytester-function' 02998 // docTest_item['group-id-value'] = 'CODE_LOAD' 02999 // docTest_item['action'] = 'tinyTester.AnalogIn1_Read_Report_voltageV()' 03000 // docTest_item['propName'] = 'AnalogIn1_Read_Report_voltageV' 03001 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 03002 if (SelfTestGroupEnable & 0x0004) { 03003 // call-tinytester-function 03004 // tinyTesterFuncName = "tinyTester.AnalogIn1_Read_Report_voltageV" 03005 // docTest_argList = "" 03006 tinyTester.AnalogIn1_Read_Report_voltageV(); // 03007 // halt-on-first-failure logic 03008 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03009 } // if (SelfTestGroupEnable & 0x0004) 03010 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 03011 03012 // @test group CODE_LOAD tinyTester.err_threshold = 0.750 03013 // docTest_item['actionType'] = 'assign-propname-value' 03014 // docTest_item['group-id-value'] = 'CODE_LOAD' 03015 // docTest_item['action'] = 'tinyTester.err_threshold = 0.750' 03016 // docTest_item['propName'] = 'tinyTester.err_threshold' 03017 // docTest_item['propValue'] = '0.750' 03018 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 03019 if (SelfTestGroupEnable & 0x0004) { 03020 // assign-propname-value 03021 // tinyTesterPropName = "tinyTester.err_threshold" 03022 // tinyTesterPropValue = "0.750" 03023 tinyTester.err_threshold = 0.750; 03024 // halt-on-first-failure logic 03025 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03026 } // if (SelfTestGroupEnable & 0x0004) 03027 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 03028 03029 // @test group CODE_LOAD tinyTester.print("0x0fffff = 4.095V") 03030 // docTest_item['actionType'] = 'print-string' 03031 // docTest_item['group-id-value'] = 'CODE_LOAD' 03032 // docTest_item['action'] = 'tinyTester.print("0x0fffff = 4.095V")' 03033 // docTest_item['arglist'] = '0x0fffff = 4.095V' 03034 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 03035 if (SelfTestGroupEnable & 0x0004) { 03036 // print-string 03037 // tinyTesterFuncName = "tinyTester.print" 03038 // tinyTesterPrintStringLiteral = "0x0fffff = 4.095V" 03039 tinyTester.print("0x0fffff = 4.095V"); 03040 // halt-on-first-failure logic 03041 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03042 } // if (SelfTestGroupEnable & 0x0004) 03043 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 03044 03045 // @test group CODE_LOAD CODE_LOAD(0x0fffff) // 4.095V 03046 // docTest_item['actionType'] = 'call-function' 03047 // docTest_item['group-id-value'] = 'CODE_LOAD' 03048 // docTest_item['action'] = 'CODE_LOAD(0x0fffff)' 03049 // docTest_item['remarks'] = '4.095V' 03050 // docTest_item['funcName'] = 'CODE_LOAD' 03051 // docTest_item['arglist'] = '0x0fffff' 03052 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD CODE_LOAD 4.095V 03053 if (SelfTestGroupEnable & 0x0004) { 03054 // call-function 03055 // selfTestFunctionClosures['CODE_LOAD']['returnType'] = 'uint8_t' 03056 // ASSERT_EQ(g_MAX5719_device.CODE_LOAD((uint32_t)0x0fffff), (uint8_t)None); // 4.095V 03057 // tinyTester.FunctionCall_Expect("MAX5719.CODE_LOAD", fn_MAX5719_CODE_LOAD, (uint32_t)0x0fffff, /* empty expect: */ (uint8_t)None); // 4.095V 03058 g_MAX5719_device.CODE_LOAD((uint32_t)0x0fffff); // 4.095V 03059 // halt-on-first-failure logic 03060 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03061 } // if (SelfTestGroupEnable & 0x0004) 03062 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 03063 03064 // @test group CODE_LOAD tinyTester.Wait_Output_Settling() 03065 // docTest_item['actionType'] = 'call-tinytester-function' 03066 // docTest_item['group-id-value'] = 'CODE_LOAD' 03067 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 03068 // docTest_item['propName'] = 'Wait_Output_Settling' 03069 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 03070 if (SelfTestGroupEnable & 0x0004) { 03071 // call-tinytester-function 03072 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 03073 // docTest_argList = "" 03074 tinyTester.Wait_Output_Settling(); // 03075 // halt-on-first-failure logic 03076 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03077 } // if (SelfTestGroupEnable & 0x0004) 03078 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 03079 03080 // @test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(4.095000) 03081 // docTest_item['actionType'] = 'call-tinytester-function' 03082 // docTest_item['group-id-value'] = 'CODE_LOAD' 03083 // docTest_item['action'] = 'tinyTester.AnalogIn0_Read_Expect_voltageV(4.095000)' 03084 // docTest_item['arglist'] = '4.095000' 03085 // docTest_item['propName'] = 'AnalogIn0_Read_Expect_voltageV' 03086 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 03087 if (SelfTestGroupEnable & 0x0004) { 03088 // call-tinytester-function 03089 // tinyTesterFuncName = "tinyTester.AnalogIn0_Read_Expect_voltageV" 03090 // docTest_argList = "4.095000" 03091 tinyTester.AnalogIn0_Read_Expect_voltageV(4.095000); // 03092 // halt-on-first-failure logic 03093 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03094 } // if (SelfTestGroupEnable & 0x0004) 03095 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 03096 03097 // @test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 03098 // docTest_item['actionType'] = 'call-tinytester-function' 03099 // docTest_item['group-id-value'] = 'CODE_LOAD' 03100 // docTest_item['action'] = 'tinyTester.AnalogIn1_Read_Report_voltageV()' 03101 // docTest_item['propName'] = 'AnalogIn1_Read_Report_voltageV' 03102 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 03103 if (SelfTestGroupEnable & 0x0004) { 03104 // call-tinytester-function 03105 // tinyTesterFuncName = "tinyTester.AnalogIn1_Read_Report_voltageV" 03106 // docTest_argList = "" 03107 tinyTester.AnalogIn1_Read_Report_voltageV(); // 03108 // halt-on-first-failure logic 03109 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03110 } // if (SelfTestGroupEnable & 0x0004) 03111 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 03112 03113 // @test group CODE_LOAD tinyTester.err_threshold = 0.200 03114 // docTest_item['actionType'] = 'assign-propname-value' 03115 // docTest_item['group-id-value'] = 'CODE_LOAD' 03116 // docTest_item['action'] = 'tinyTester.err_threshold = 0.200' 03117 // docTest_item['propName'] = 'tinyTester.err_threshold' 03118 // docTest_item['propValue'] = '0.200' 03119 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 03120 if (SelfTestGroupEnable & 0x0004) { 03121 // assign-propname-value 03122 // tinyTesterPropName = "tinyTester.err_threshold" 03123 // tinyTesterPropValue = "0.200" 03124 tinyTester.err_threshold = 0.200; 03125 // halt-on-first-failure logic 03126 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03127 } // if (SelfTestGroupEnable & 0x0004) 03128 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 03129 03130 // @test group CODE_LOAD tinyTester.print("0x080000 // 2.048V") 03131 // @test group CODE_LOAD CODE_LOAD(0x080000) // 2.048V 03132 // docTest_item['actionType'] = 'call-function' 03133 // docTest_item['group-id-value'] = 'CODE_LOAD' 03134 // docTest_item['action'] = 'CODE_LOAD(0x080000)' 03135 // docTest_item['remarks'] = '2.048V' 03136 // docTest_item['funcName'] = 'CODE_LOAD' 03137 // docTest_item['arglist'] = '0x080000' 03138 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD CODE_LOAD 2.048V 03139 if (SelfTestGroupEnable & 0x0004) { 03140 // call-function 03141 // selfTestFunctionClosures['CODE_LOAD']['returnType'] = 'uint8_t' 03142 // ASSERT_EQ(g_MAX5719_device.CODE_LOAD((uint32_t)0x080000), (uint8_t)None); // 2.048V 03143 // tinyTester.FunctionCall_Expect("MAX5719.CODE_LOAD", fn_MAX5719_CODE_LOAD, (uint32_t)0x080000, /* empty expect: */ (uint8_t)None); // 2.048V 03144 g_MAX5719_device.CODE_LOAD((uint32_t)0x080000); // 2.048V 03145 // halt-on-first-failure logic 03146 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03147 } // if (SelfTestGroupEnable & 0x0004) 03148 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 03149 03150 // @test group CODE_LOAD tinyTester.Wait_Output_Settling() 03151 // docTest_item['actionType'] = 'call-tinytester-function' 03152 // docTest_item['group-id-value'] = 'CODE_LOAD' 03153 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 03154 // docTest_item['propName'] = 'Wait_Output_Settling' 03155 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 03156 if (SelfTestGroupEnable & 0x0004) { 03157 // call-tinytester-function 03158 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 03159 // docTest_argList = "" 03160 tinyTester.Wait_Output_Settling(); // 03161 // halt-on-first-failure logic 03162 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03163 } // if (SelfTestGroupEnable & 0x0004) 03164 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 03165 03166 // @test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(2.048000) 03167 // docTest_item['actionType'] = 'call-tinytester-function' 03168 // docTest_item['group-id-value'] = 'CODE_LOAD' 03169 // docTest_item['action'] = 'tinyTester.AnalogIn0_Read_Expect_voltageV(2.048000)' 03170 // docTest_item['arglist'] = '2.048000' 03171 // docTest_item['propName'] = 'AnalogIn0_Read_Expect_voltageV' 03172 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 03173 if (SelfTestGroupEnable & 0x0004) { 03174 // call-tinytester-function 03175 // tinyTesterFuncName = "tinyTester.AnalogIn0_Read_Expect_voltageV" 03176 // docTest_argList = "2.048000" 03177 tinyTester.AnalogIn0_Read_Expect_voltageV(2.048000); // 03178 // halt-on-first-failure logic 03179 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03180 } // if (SelfTestGroupEnable & 0x0004) 03181 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 03182 03183 // @test group CODE_LOAD tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 03184 // docTest_item['actionType'] = 'call-tinytester-function' 03185 // docTest_item['group-id-value'] = 'CODE_LOAD' 03186 // docTest_item['action'] = 'tinyTester.AnalogIn1_Read_Report_voltageV()' 03187 // docTest_item['propName'] = 'AnalogIn1_Read_Report_voltageV' 03188 #if MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD None 03189 if (SelfTestGroupEnable & 0x0004) { 03190 // call-tinytester-function 03191 // tinyTesterFuncName = "tinyTester.AnalogIn1_Read_Report_voltageV" 03192 // docTest_argList = "" 03193 tinyTester.AnalogIn1_Read_Report_voltageV(); // 03194 // halt-on-first-failure logic 03195 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03196 } // if (SelfTestGroupEnable & 0x0004) 03197 #endif // MAX5719_SELFTEST_CODE_LOAD // group CODE_LOAD 03198 03199 // @test group CODE_LOAD_2V5 // Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) (no run on button) 03200 // @test group CODE_LOAD_2V5 tinyTester.err_threshold = 0.150 03201 // docTest_item['actionType'] = 'assign-propname-value' 03202 // docTest_item['group-id-value'] = 'CODE_LOAD_2V5' 03203 // docTest_item['action'] = 'tinyTester.err_threshold = 0.150' 03204 // docTest_item['propName'] = 'tinyTester.err_threshold' 03205 // docTest_item['propValue'] = '0.150' 03206 #if MAX5719_SELFTEST_CODE_LOAD_2V5 // group CODE_LOAD_2V5 None 03207 if (SelfTestGroupEnable & 0x0008) { 03208 // assign-propname-value 03209 // tinyTesterPropName = "tinyTester.err_threshold" 03210 // tinyTesterPropValue = "0.150" 03211 tinyTester.err_threshold = 0.150; 03212 // halt-on-first-failure logic 03213 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03214 } // if (SelfTestGroupEnable & 0x0008) 03215 #endif // MAX5719_SELFTEST_CODE_LOAD_2V5 // group CODE_LOAD_2V5 03216 03217 // @test group CODE_LOAD_2V5 tinyTester.print("0x09c400 = 2.500V") 03218 // docTest_item['actionType'] = 'print-string' 03219 // docTest_item['group-id-value'] = 'CODE_LOAD_2V5' 03220 // docTest_item['action'] = 'tinyTester.print("0x09c400 = 2.500V")' 03221 // docTest_item['arglist'] = '0x09c400 = 2.500V' 03222 #if MAX5719_SELFTEST_CODE_LOAD_2V5 // group CODE_LOAD_2V5 None 03223 if (SelfTestGroupEnable & 0x0008) { 03224 // print-string 03225 // tinyTesterFuncName = "tinyTester.print" 03226 // tinyTesterPrintStringLiteral = "0x09c400 = 2.500V" 03227 tinyTester.print("0x09c400 = 2.500V"); 03228 // halt-on-first-failure logic 03229 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03230 } // if (SelfTestGroupEnable & 0x0008) 03231 #endif // MAX5719_SELFTEST_CODE_LOAD_2V5 // group CODE_LOAD_2V5 03232 03233 // @test group CODE_LOAD_2V5 CODE_LOAD(0x09c400) // 2.500V 03234 // docTest_item['actionType'] = 'call-function' 03235 // docTest_item['group-id-value'] = 'CODE_LOAD_2V5' 03236 // docTest_item['action'] = 'CODE_LOAD(0x09c400)' 03237 // docTest_item['remarks'] = '2.500V' 03238 // docTest_item['funcName'] = 'CODE_LOAD' 03239 // docTest_item['arglist'] = '0x09c400' 03240 #if MAX5719_SELFTEST_CODE_LOAD_2V5 // group CODE_LOAD_2V5 CODE_LOAD 2.500V 03241 if (SelfTestGroupEnable & 0x0008) { 03242 // call-function 03243 // selfTestFunctionClosures['CODE_LOAD']['returnType'] = 'uint8_t' 03244 // ASSERT_EQ(g_MAX5719_device.CODE_LOAD((uint32_t)0x09c400), (uint8_t)None); // 2.500V 03245 // tinyTester.FunctionCall_Expect("MAX5719.CODE_LOAD", fn_MAX5719_CODE_LOAD, (uint32_t)0x09c400, /* empty expect: */ (uint8_t)None); // 2.500V 03246 g_MAX5719_device.CODE_LOAD((uint32_t)0x09c400); // 2.500V 03247 // halt-on-first-failure logic 03248 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03249 } // if (SelfTestGroupEnable & 0x0008) 03250 #endif // MAX5719_SELFTEST_CODE_LOAD_2V5 // group CODE_LOAD_2V5 03251 03252 // @test group CODE_LOAD_2V5 tinyTester.Wait_Output_Settling() 03253 // docTest_item['actionType'] = 'call-tinytester-function' 03254 // docTest_item['group-id-value'] = 'CODE_LOAD_2V5' 03255 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 03256 // docTest_item['propName'] = 'Wait_Output_Settling' 03257 #if MAX5719_SELFTEST_CODE_LOAD_2V5 // group CODE_LOAD_2V5 None 03258 if (SelfTestGroupEnable & 0x0008) { 03259 // call-tinytester-function 03260 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 03261 // docTest_argList = "" 03262 tinyTester.Wait_Output_Settling(); // 03263 // halt-on-first-failure logic 03264 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03265 } // if (SelfTestGroupEnable & 0x0008) 03266 #endif // MAX5719_SELFTEST_CODE_LOAD_2V5 // group CODE_LOAD_2V5 03267 03268 // @test group CODE_LOAD_2V5 tinyTester.AnalogIn0_Read_Expect_voltageV(2.500000) 03269 // docTest_item['actionType'] = 'call-tinytester-function' 03270 // docTest_item['group-id-value'] = 'CODE_LOAD_2V5' 03271 // docTest_item['action'] = 'tinyTester.AnalogIn0_Read_Expect_voltageV(2.500000)' 03272 // docTest_item['arglist'] = '2.500000' 03273 // docTest_item['propName'] = 'AnalogIn0_Read_Expect_voltageV' 03274 #if MAX5719_SELFTEST_CODE_LOAD_2V5 // group CODE_LOAD_2V5 None 03275 if (SelfTestGroupEnable & 0x0008) { 03276 // call-tinytester-function 03277 // tinyTesterFuncName = "tinyTester.AnalogIn0_Read_Expect_voltageV" 03278 // docTest_argList = "2.500000" 03279 tinyTester.AnalogIn0_Read_Expect_voltageV(2.500000); // 03280 // halt-on-first-failure logic 03281 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03282 } // if (SelfTestGroupEnable & 0x0008) 03283 #endif // MAX5719_SELFTEST_CODE_LOAD_2V5 // group CODE_LOAD_2V5 03284 03285 // @test group CODE_LOAD_2V5 tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 03286 // docTest_item['actionType'] = 'call-tinytester-function' 03287 // docTest_item['group-id-value'] = 'CODE_LOAD_2V5' 03288 // docTest_item['action'] = 'tinyTester.AnalogIn1_Read_Report_voltageV()' 03289 // docTest_item['propName'] = 'AnalogIn1_Read_Report_voltageV' 03290 #if MAX5719_SELFTEST_CODE_LOAD_2V5 // group CODE_LOAD_2V5 None 03291 if (SelfTestGroupEnable & 0x0008) { 03292 // call-tinytester-function 03293 // tinyTesterFuncName = "tinyTester.AnalogIn1_Read_Report_voltageV" 03294 // docTest_argList = "" 03295 tinyTester.AnalogIn1_Read_Report_voltageV(); // 03296 // halt-on-first-failure logic 03297 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03298 } // if (SelfTestGroupEnable & 0x0008) 03299 #endif // MAX5719_SELFTEST_CODE_LOAD_2V5 // group CODE_LOAD_2V5 03300 03301 // @test group CODE_LOAD_3V0 // Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) (no run on button) 03302 // @test group CODE_LOAD_3V0 tinyTester.err_threshold = 0.200 03303 // docTest_item['actionType'] = 'assign-propname-value' 03304 // docTest_item['group-id-value'] = 'CODE_LOAD_3V0' 03305 // docTest_item['action'] = 'tinyTester.err_threshold = 0.200' 03306 // docTest_item['propName'] = 'tinyTester.err_threshold' 03307 // docTest_item['propValue'] = '0.200' 03308 #if MAX5719_SELFTEST_CODE_LOAD_3V0 // group CODE_LOAD_3V0 None 03309 if (SelfTestGroupEnable & 0x0010) { 03310 // assign-propname-value 03311 // tinyTesterPropName = "tinyTester.err_threshold" 03312 // tinyTesterPropValue = "0.200" 03313 tinyTester.err_threshold = 0.200; 03314 // halt-on-first-failure logic 03315 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03316 } // if (SelfTestGroupEnable & 0x0010) 03317 #endif // MAX5719_SELFTEST_CODE_LOAD_3V0 // group CODE_LOAD_3V0 03318 03319 // @test group CODE_LOAD_3V0 tinyTester.print("0x0bb800 = 3.000V") 03320 // docTest_item['actionType'] = 'print-string' 03321 // docTest_item['group-id-value'] = 'CODE_LOAD_3V0' 03322 // docTest_item['action'] = 'tinyTester.print("0x0bb800 = 3.000V")' 03323 // docTest_item['arglist'] = '0x0bb800 = 3.000V' 03324 #if MAX5719_SELFTEST_CODE_LOAD_3V0 // group CODE_LOAD_3V0 None 03325 if (SelfTestGroupEnable & 0x0010) { 03326 // print-string 03327 // tinyTesterFuncName = "tinyTester.print" 03328 // tinyTesterPrintStringLiteral = "0x0bb800 = 3.000V" 03329 tinyTester.print("0x0bb800 = 3.000V"); 03330 // halt-on-first-failure logic 03331 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03332 } // if (SelfTestGroupEnable & 0x0010) 03333 #endif // MAX5719_SELFTEST_CODE_LOAD_3V0 // group CODE_LOAD_3V0 03334 03335 // @test group CODE_LOAD_3V0 CODE_LOAD(0x0bb800) // 3.000V 03336 // docTest_item['actionType'] = 'call-function' 03337 // docTest_item['group-id-value'] = 'CODE_LOAD_3V0' 03338 // docTest_item['action'] = 'CODE_LOAD(0x0bb800)' 03339 // docTest_item['remarks'] = '3.000V' 03340 // docTest_item['funcName'] = 'CODE_LOAD' 03341 // docTest_item['arglist'] = '0x0bb800' 03342 #if MAX5719_SELFTEST_CODE_LOAD_3V0 // group CODE_LOAD_3V0 CODE_LOAD 3.000V 03343 if (SelfTestGroupEnable & 0x0010) { 03344 // call-function 03345 // selfTestFunctionClosures['CODE_LOAD']['returnType'] = 'uint8_t' 03346 // ASSERT_EQ(g_MAX5719_device.CODE_LOAD((uint32_t)0x0bb800), (uint8_t)None); // 3.000V 03347 // tinyTester.FunctionCall_Expect("MAX5719.CODE_LOAD", fn_MAX5719_CODE_LOAD, (uint32_t)0x0bb800, /* empty expect: */ (uint8_t)None); // 3.000V 03348 g_MAX5719_device.CODE_LOAD((uint32_t)0x0bb800); // 3.000V 03349 // halt-on-first-failure logic 03350 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03351 } // if (SelfTestGroupEnable & 0x0010) 03352 #endif // MAX5719_SELFTEST_CODE_LOAD_3V0 // group CODE_LOAD_3V0 03353 03354 // @test group CODE_LOAD_3V0 tinyTester.Wait_Output_Settling() 03355 // docTest_item['actionType'] = 'call-tinytester-function' 03356 // docTest_item['group-id-value'] = 'CODE_LOAD_3V0' 03357 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 03358 // docTest_item['propName'] = 'Wait_Output_Settling' 03359 #if MAX5719_SELFTEST_CODE_LOAD_3V0 // group CODE_LOAD_3V0 None 03360 if (SelfTestGroupEnable & 0x0010) { 03361 // call-tinytester-function 03362 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 03363 // docTest_argList = "" 03364 tinyTester.Wait_Output_Settling(); // 03365 // halt-on-first-failure logic 03366 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03367 } // if (SelfTestGroupEnable & 0x0010) 03368 #endif // MAX5719_SELFTEST_CODE_LOAD_3V0 // group CODE_LOAD_3V0 03369 03370 // @test group CODE_LOAD_3V0 tinyTester.AnalogIn0_Read_Expect_voltageV(3.000000) 03371 // docTest_item['actionType'] = 'call-tinytester-function' 03372 // docTest_item['group-id-value'] = 'CODE_LOAD_3V0' 03373 // docTest_item['action'] = 'tinyTester.AnalogIn0_Read_Expect_voltageV(3.000000)' 03374 // docTest_item['arglist'] = '3.000000' 03375 // docTest_item['propName'] = 'AnalogIn0_Read_Expect_voltageV' 03376 #if MAX5719_SELFTEST_CODE_LOAD_3V0 // group CODE_LOAD_3V0 None 03377 if (SelfTestGroupEnable & 0x0010) { 03378 // call-tinytester-function 03379 // tinyTesterFuncName = "tinyTester.AnalogIn0_Read_Expect_voltageV" 03380 // docTest_argList = "3.000000" 03381 tinyTester.AnalogIn0_Read_Expect_voltageV(3.000000); // 03382 // halt-on-first-failure logic 03383 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03384 } // if (SelfTestGroupEnable & 0x0010) 03385 #endif // MAX5719_SELFTEST_CODE_LOAD_3V0 // group CODE_LOAD_3V0 03386 03387 // @test group CODE_LOAD_3V0 tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 03388 // docTest_item['actionType'] = 'call-tinytester-function' 03389 // docTest_item['group-id-value'] = 'CODE_LOAD_3V0' 03390 // docTest_item['action'] = 'tinyTester.AnalogIn1_Read_Report_voltageV()' 03391 // docTest_item['propName'] = 'AnalogIn1_Read_Report_voltageV' 03392 #if MAX5719_SELFTEST_CODE_LOAD_3V0 // group CODE_LOAD_3V0 None 03393 if (SelfTestGroupEnable & 0x0010) { 03394 // call-tinytester-function 03395 // tinyTesterFuncName = "tinyTester.AnalogIn1_Read_Report_voltageV" 03396 // docTest_argList = "" 03397 tinyTester.AnalogIn1_Read_Report_voltageV(); // 03398 // halt-on-first-failure logic 03399 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03400 } // if (SelfTestGroupEnable & 0x0010) 03401 #endif // MAX5719_SELFTEST_CODE_LOAD_3V0 // group CODE_LOAD_3V0 03402 03403 // @test group CODE_LOAD_4V1 // Verify function CODE_LOAD vs platform AIN0 analog input (enabled by default) (no run on button) 03404 // @test group CODE_LOAD_4V1 tinyTester.err_threshold = 0.750 03405 // docTest_item['actionType'] = 'assign-propname-value' 03406 // docTest_item['group-id-value'] = 'CODE_LOAD_4V1' 03407 // docTest_item['action'] = 'tinyTester.err_threshold = 0.750' 03408 // docTest_item['propName'] = 'tinyTester.err_threshold' 03409 // docTest_item['propValue'] = '0.750' 03410 #if MAX5719_SELFTEST_CODE_LOAD_4V1 // group CODE_LOAD_4V1 None 03411 if (SelfTestGroupEnable & 0x0020) { 03412 // assign-propname-value 03413 // tinyTesterPropName = "tinyTester.err_threshold" 03414 // tinyTesterPropValue = "0.750" 03415 tinyTester.err_threshold = 0.750; 03416 // halt-on-first-failure logic 03417 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03418 } // if (SelfTestGroupEnable & 0x0020) 03419 #endif // MAX5719_SELFTEST_CODE_LOAD_4V1 // group CODE_LOAD_4V1 03420 03421 // @test group CODE_LOAD_4V1 tinyTester.print("0x0fffff = 4.095V") 03422 // docTest_item['actionType'] = 'print-string' 03423 // docTest_item['group-id-value'] = 'CODE_LOAD_4V1' 03424 // docTest_item['action'] = 'tinyTester.print("0x0fffff = 4.095V")' 03425 // docTest_item['arglist'] = '0x0fffff = 4.095V' 03426 #if MAX5719_SELFTEST_CODE_LOAD_4V1 // group CODE_LOAD_4V1 None 03427 if (SelfTestGroupEnable & 0x0020) { 03428 // print-string 03429 // tinyTesterFuncName = "tinyTester.print" 03430 // tinyTesterPrintStringLiteral = "0x0fffff = 4.095V" 03431 tinyTester.print("0x0fffff = 4.095V"); 03432 // halt-on-first-failure logic 03433 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03434 } // if (SelfTestGroupEnable & 0x0020) 03435 #endif // MAX5719_SELFTEST_CODE_LOAD_4V1 // group CODE_LOAD_4V1 03436 03437 // @test group CODE_LOAD_4V1 CODE_LOAD(0x0fffff) // 4.095V 03438 // docTest_item['actionType'] = 'call-function' 03439 // docTest_item['group-id-value'] = 'CODE_LOAD_4V1' 03440 // docTest_item['action'] = 'CODE_LOAD(0x0fffff)' 03441 // docTest_item['remarks'] = '4.095V' 03442 // docTest_item['funcName'] = 'CODE_LOAD' 03443 // docTest_item['arglist'] = '0x0fffff' 03444 #if MAX5719_SELFTEST_CODE_LOAD_4V1 // group CODE_LOAD_4V1 CODE_LOAD 4.095V 03445 if (SelfTestGroupEnable & 0x0020) { 03446 // call-function 03447 // selfTestFunctionClosures['CODE_LOAD']['returnType'] = 'uint8_t' 03448 // ASSERT_EQ(g_MAX5719_device.CODE_LOAD((uint32_t)0x0fffff), (uint8_t)None); // 4.095V 03449 // tinyTester.FunctionCall_Expect("MAX5719.CODE_LOAD", fn_MAX5719_CODE_LOAD, (uint32_t)0x0fffff, /* empty expect: */ (uint8_t)None); // 4.095V 03450 g_MAX5719_device.CODE_LOAD((uint32_t)0x0fffff); // 4.095V 03451 // halt-on-first-failure logic 03452 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03453 } // if (SelfTestGroupEnable & 0x0020) 03454 #endif // MAX5719_SELFTEST_CODE_LOAD_4V1 // group CODE_LOAD_4V1 03455 03456 // @test group CODE_LOAD_4V1 tinyTester.Wait_Output_Settling() 03457 // docTest_item['actionType'] = 'call-tinytester-function' 03458 // docTest_item['group-id-value'] = 'CODE_LOAD_4V1' 03459 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 03460 // docTest_item['propName'] = 'Wait_Output_Settling' 03461 #if MAX5719_SELFTEST_CODE_LOAD_4V1 // group CODE_LOAD_4V1 None 03462 if (SelfTestGroupEnable & 0x0020) { 03463 // call-tinytester-function 03464 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 03465 // docTest_argList = "" 03466 tinyTester.Wait_Output_Settling(); // 03467 // halt-on-first-failure logic 03468 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03469 } // if (SelfTestGroupEnable & 0x0020) 03470 #endif // MAX5719_SELFTEST_CODE_LOAD_4V1 // group CODE_LOAD_4V1 03471 03472 // @test group CODE_LOAD_4V1 tinyTester.AnalogIn0_Read_Expect_voltageV(4.095000) 03473 // docTest_item['actionType'] = 'call-tinytester-function' 03474 // docTest_item['group-id-value'] = 'CODE_LOAD_4V1' 03475 // docTest_item['action'] = 'tinyTester.AnalogIn0_Read_Expect_voltageV(4.095000)' 03476 // docTest_item['arglist'] = '4.095000' 03477 // docTest_item['propName'] = 'AnalogIn0_Read_Expect_voltageV' 03478 #if MAX5719_SELFTEST_CODE_LOAD_4V1 // group CODE_LOAD_4V1 None 03479 if (SelfTestGroupEnable & 0x0020) { 03480 // call-tinytester-function 03481 // tinyTesterFuncName = "tinyTester.AnalogIn0_Read_Expect_voltageV" 03482 // docTest_argList = "4.095000" 03483 tinyTester.AnalogIn0_Read_Expect_voltageV(4.095000); // 03484 // halt-on-first-failure logic 03485 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03486 } // if (SelfTestGroupEnable & 0x0020) 03487 #endif // MAX5719_SELFTEST_CODE_LOAD_4V1 // group CODE_LOAD_4V1 03488 03489 // @test group CODE_LOAD_4V1 tinyTester.AnalogIn1_Read_Report_voltageV(); // remove unwanted loading on AIN0 03490 // docTest_item['actionType'] = 'call-tinytester-function' 03491 // docTest_item['group-id-value'] = 'CODE_LOAD_4V1' 03492 // docTest_item['action'] = 'tinyTester.AnalogIn1_Read_Report_voltageV()' 03493 // docTest_item['propName'] = 'AnalogIn1_Read_Report_voltageV' 03494 #if MAX5719_SELFTEST_CODE_LOAD_4V1 // group CODE_LOAD_4V1 None 03495 if (SelfTestGroupEnable & 0x0020) { 03496 // call-tinytester-function 03497 // tinyTesterFuncName = "tinyTester.AnalogIn1_Read_Report_voltageV" 03498 // docTest_argList = "" 03499 tinyTester.AnalogIn1_Read_Report_voltageV(); // 03500 // halt-on-first-failure logic 03501 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03502 } // if (SelfTestGroupEnable & 0x0020) 03503 #endif // MAX5719_SELFTEST_CODE_LOAD_4V1 // group CODE_LOAD_4V1 03504 03505 // @test group DACCodeOfVoltage // Verify function DACCodeOfVoltage (enabled by default) (no run on button) 03506 // @test group DACCodeOfVoltage tinyTester.blink_time_msec = 20 // quickly speed through the software verification 03507 // docTest_item['actionType'] = 'assign-propname-value' 03508 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03509 // docTest_item['action'] = 'tinyTester.blink_time_msec = 20' 03510 // docTest_item['remarks'] = 'quickly speed through the software verification' 03511 // docTest_item['propName'] = 'tinyTester.blink_time_msec' 03512 // docTest_item['propValue'] = '20' 03513 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage None quickly speed through the software verification 03514 if (SelfTestGroupEnable & 0x0040) { 03515 // assign-propname-value 03516 // tinyTesterPropName = "tinyTester.blink_time_msec" 03517 // tinyTesterPropValue = "20" 03518 tinyTester.blink_time_msec = 20; 03519 // halt-on-first-failure logic 03520 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03521 } // if (SelfTestGroupEnable & 0x0040) 03522 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03523 03524 // @test group DACCodeOfVoltage tinyTester.print("VRef = 4.096 MAX5719 20-bit LSB = 0.000004V = 3.90625uV") 03525 // docTest_item['actionType'] = 'print-string' 03526 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03527 // docTest_item['action'] = 'tinyTester.print("VRef = 4.096 MAX5719 20-bit LSB = 0.000004V = 3.90625uV")' 03528 // docTest_item['arglist'] = 'VRef = 4.096 MAX5719 20-bit LSB = 0.000004V = 3.90625uV' 03529 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage None 03530 if (SelfTestGroupEnable & 0x0040) { 03531 // print-string 03532 // tinyTesterFuncName = "tinyTester.print" 03533 // tinyTesterPrintStringLiteral = "VRef = 4.096 MAX5719 20-bit LSB = 0.000004V = 3.90625uV" 03534 tinyTester.print("VRef = 4.096 MAX5719 20-bit LSB = 0.000004V = 3.90625uV"); 03535 // halt-on-first-failure logic 03536 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03537 } // if (SelfTestGroupEnable & 0x0040) 03538 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03539 03540 // @test group DACCodeOfVoltage VRef = 4.096 03541 // docTest_item['actionType'] = 'assign-propname-value' 03542 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03543 // docTest_item['action'] = 'VRef = 4.096' 03544 // docTest_item['propName'] = 'VRef' 03545 // docTest_item['propValue'] = '4.096' 03546 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage None 03547 if (SelfTestGroupEnable & 0x0040) { 03548 // assign-propname-value 03549 // tinyTesterPropName = "VRef" 03550 // tinyTesterPropValue = "4.096" 03551 g_MAX5719_device.VRef = 4.096; 03552 // halt-on-first-failure logic 03553 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03554 } // if (SelfTestGroupEnable & 0x0040) 03555 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03556 03557 // @test group DACCodeOfVoltage tinyTester.print("test_voltage_sweep V = 0.000000V to 4.096000V precision 0.100000V step 0.500000V") 03558 // docTest_item['actionType'] = 'print-string' 03559 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03560 // docTest_item['action'] = 'tinyTester.print("test_voltage_sweep V = 0.000000V to 4.096000V precision 0.100000V step 0.500000V")' 03561 // docTest_item['arglist'] = 'test_voltage_sweep V = 0.000000V to 4.096000V precision 0.100000V step 0.500000V' 03562 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage None 03563 if (SelfTestGroupEnable & 0x0040) { 03564 // print-string 03565 // tinyTesterFuncName = "tinyTester.print" 03566 // tinyTesterPrintStringLiteral = "test_voltage_sweep V = 0.000000V to 4.096000V precision 0.100000V step 0.500000V" 03567 tinyTester.print("test_voltage_sweep V = 0.000000V to 4.096000V precision 0.100000V step 0.500000V"); 03568 // halt-on-first-failure logic 03569 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03570 } // if (SelfTestGroupEnable & 0x0040) 03571 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03572 03573 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.000000) expect 0x000000 // 0.000V 03574 // docTest_item['actionType'] = 'call-function' 03575 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03576 // docTest_item['action'] = 'DACCodeOfVoltage(0.000000) expect 0x000000' 03577 // docTest_item['remarks'] = '0.000V' 03578 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03579 // docTest_item['arglist'] = '0.000000' 03580 // docTest_item['expect-value'] = '0x000000' 03581 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.000V 03582 if (SelfTestGroupEnable & 0x0040) { 03583 // call-function 03584 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03585 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.000000), (uint32_t)0x000000); // 0.000V 03586 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.000000, /* expect: */ (uint32_t)0x000000); // 0.000V 03587 // halt-on-first-failure logic 03588 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03589 } // if (SelfTestGroupEnable & 0x0040) 03590 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03591 03592 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.500000) expect 0x01f400 // 0.500V 03593 // docTest_item['actionType'] = 'call-function' 03594 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03595 // docTest_item['action'] = 'DACCodeOfVoltage(0.500000) expect 0x01f400' 03596 // docTest_item['remarks'] = '0.500V' 03597 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03598 // docTest_item['arglist'] = '0.500000' 03599 // docTest_item['expect-value'] = '0x01f400' 03600 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.500V 03601 if (SelfTestGroupEnable & 0x0040) { 03602 // call-function 03603 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03604 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.500000), (uint32_t)0x01f400); // 0.500V 03605 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.500000, /* expect: */ (uint32_t)0x01f400); // 0.500V 03606 // halt-on-first-failure logic 03607 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03608 } // if (SelfTestGroupEnable & 0x0040) 03609 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03610 03611 // @test group DACCodeOfVoltage DACCodeOfVoltage(1.000000) expect 0x03e800 // 1.000V 03612 // docTest_item['actionType'] = 'call-function' 03613 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03614 // docTest_item['action'] = 'DACCodeOfVoltage(1.000000) expect 0x03e800' 03615 // docTest_item['remarks'] = '1.000V' 03616 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03617 // docTest_item['arglist'] = '1.000000' 03618 // docTest_item['expect-value'] = '0x03e800' 03619 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 1.000V 03620 if (SelfTestGroupEnable & 0x0040) { 03621 // call-function 03622 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03623 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)1.000000), (uint32_t)0x03e800); // 1.000V 03624 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)1.000000, /* expect: */ (uint32_t)0x03e800); // 1.000V 03625 // halt-on-first-failure logic 03626 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03627 } // if (SelfTestGroupEnable & 0x0040) 03628 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03629 03630 // @test group DACCodeOfVoltage DACCodeOfVoltage(1.500000) expect 0x05dc00 // 1.500V 03631 // docTest_item['actionType'] = 'call-function' 03632 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03633 // docTest_item['action'] = 'DACCodeOfVoltage(1.500000) expect 0x05dc00' 03634 // docTest_item['remarks'] = '1.500V' 03635 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03636 // docTest_item['arglist'] = '1.500000' 03637 // docTest_item['expect-value'] = '0x05dc00' 03638 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 1.500V 03639 if (SelfTestGroupEnable & 0x0040) { 03640 // call-function 03641 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03642 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)1.500000), (uint32_t)0x05dc00); // 1.500V 03643 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)1.500000, /* expect: */ (uint32_t)0x05dc00); // 1.500V 03644 // halt-on-first-failure logic 03645 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03646 } // if (SelfTestGroupEnable & 0x0040) 03647 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03648 03649 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.000000) expect 0x07d000 // 2.000V 03650 // docTest_item['actionType'] = 'call-function' 03651 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03652 // docTest_item['action'] = 'DACCodeOfVoltage(2.000000) expect 0x07d000' 03653 // docTest_item['remarks'] = '2.000V' 03654 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03655 // docTest_item['arglist'] = '2.000000' 03656 // docTest_item['expect-value'] = '0x07d000' 03657 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.000V 03658 if (SelfTestGroupEnable & 0x0040) { 03659 // call-function 03660 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03661 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.000000), (uint32_t)0x07d000); // 2.000V 03662 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.000000, /* expect: */ (uint32_t)0x07d000); // 2.000V 03663 // halt-on-first-failure logic 03664 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03665 } // if (SelfTestGroupEnable & 0x0040) 03666 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03667 03668 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.500000) expect 0x09c400 // 2.500V 03669 // docTest_item['actionType'] = 'call-function' 03670 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03671 // docTest_item['action'] = 'DACCodeOfVoltage(2.500000) expect 0x09c400' 03672 // docTest_item['remarks'] = '2.500V' 03673 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03674 // docTest_item['arglist'] = '2.500000' 03675 // docTest_item['expect-value'] = '0x09c400' 03676 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.500V 03677 if (SelfTestGroupEnable & 0x0040) { 03678 // call-function 03679 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03680 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.500000), (uint32_t)0x09c400); // 2.500V 03681 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.500000, /* expect: */ (uint32_t)0x09c400); // 2.500V 03682 // halt-on-first-failure logic 03683 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03684 } // if (SelfTestGroupEnable & 0x0040) 03685 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03686 03687 // @test group DACCodeOfVoltage DACCodeOfVoltage(3.000000) expect 0x0bb800 // 3.000V 03688 // docTest_item['actionType'] = 'call-function' 03689 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03690 // docTest_item['action'] = 'DACCodeOfVoltage(3.000000) expect 0x0bb800' 03691 // docTest_item['remarks'] = '3.000V' 03692 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03693 // docTest_item['arglist'] = '3.000000' 03694 // docTest_item['expect-value'] = '0x0bb800' 03695 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 3.000V 03696 if (SelfTestGroupEnable & 0x0040) { 03697 // call-function 03698 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03699 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)3.000000), (uint32_t)0x0bb800); // 3.000V 03700 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)3.000000, /* expect: */ (uint32_t)0x0bb800); // 3.000V 03701 // halt-on-first-failure logic 03702 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03703 } // if (SelfTestGroupEnable & 0x0040) 03704 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03705 03706 // @test group DACCodeOfVoltage DACCodeOfVoltage(3.500000) expect 0x0dac00 // 3.500V 03707 // docTest_item['actionType'] = 'call-function' 03708 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03709 // docTest_item['action'] = 'DACCodeOfVoltage(3.500000) expect 0x0dac00' 03710 // docTest_item['remarks'] = '3.500V' 03711 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03712 // docTest_item['arglist'] = '3.500000' 03713 // docTest_item['expect-value'] = '0x0dac00' 03714 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 3.500V 03715 if (SelfTestGroupEnable & 0x0040) { 03716 // call-function 03717 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03718 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)3.500000), (uint32_t)0x0dac00); // 3.500V 03719 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)3.500000, /* expect: */ (uint32_t)0x0dac00); // 3.500V 03720 // halt-on-first-failure logic 03721 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03722 } // if (SelfTestGroupEnable & 0x0040) 03723 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03724 03725 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.000000) expect 0x0fa000 // 4.000V 03726 // docTest_item['actionType'] = 'call-function' 03727 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03728 // docTest_item['action'] = 'DACCodeOfVoltage(4.000000) expect 0x0fa000' 03729 // docTest_item['remarks'] = '4.000V' 03730 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03731 // docTest_item['arglist'] = '4.000000' 03732 // docTest_item['expect-value'] = '0x0fa000' 03733 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.000V 03734 if (SelfTestGroupEnable & 0x0040) { 03735 // call-function 03736 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03737 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.000000), (uint32_t)0x0fa000); // 4.000V 03738 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.000000, /* expect: */ (uint32_t)0x0fa000); // 4.000V 03739 // halt-on-first-failure logic 03740 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03741 } // if (SelfTestGroupEnable & 0x0040) 03742 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03743 03744 // @test group DACCodeOfVoltage tinyTester.print("test_voltage_sweep V = -0.010000V to 0.100000V precision 0.010000V step 0.010000V") 03745 // docTest_item['actionType'] = 'print-string' 03746 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03747 // docTest_item['action'] = 'tinyTester.print("test_voltage_sweep V = -0.010000V to 0.100000V precision 0.010000V step 0.010000V")' 03748 // docTest_item['arglist'] = 'test_voltage_sweep V = -0.010000V to 0.100000V precision 0.010000V step 0.010000V' 03749 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage None 03750 if (SelfTestGroupEnable & 0x0040) { 03751 // print-string 03752 // tinyTesterFuncName = "tinyTester.print" 03753 // tinyTesterPrintStringLiteral = "test_voltage_sweep V = -0.010000V to 0.100000V precision 0.010000V step 0.010000V" 03754 tinyTester.print("test_voltage_sweep V = -0.010000V to 0.100000V precision 0.010000V step 0.010000V"); 03755 // halt-on-first-failure logic 03756 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03757 } // if (SelfTestGroupEnable & 0x0040) 03758 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03759 03760 // @test group DACCodeOfVoltage DACCodeOfVoltage(-0.010000) expect 0x000000 // -0.010V 03761 // docTest_item['actionType'] = 'call-function' 03762 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03763 // docTest_item['action'] = 'DACCodeOfVoltage(-0.010000) expect 0x000000' 03764 // docTest_item['remarks'] = '-0.010V' 03765 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03766 // docTest_item['arglist'] = '-0.010000' 03767 // docTest_item['expect-value'] = '0x000000' 03768 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage -0.010V 03769 if (SelfTestGroupEnable & 0x0040) { 03770 // call-function 03771 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03772 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)-0.010000), (uint32_t)0x000000); // -0.010V 03773 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)-0.010000, /* expect: */ (uint32_t)0x000000); // -0.010V 03774 // halt-on-first-failure logic 03775 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03776 } // if (SelfTestGroupEnable & 0x0040) 03777 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03778 03779 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.000000) expect 0x000000 // 0.000V 03780 // docTest_item['actionType'] = 'call-function' 03781 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03782 // docTest_item['action'] = 'DACCodeOfVoltage(0.000000) expect 0x000000' 03783 // docTest_item['remarks'] = '0.000V' 03784 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03785 // docTest_item['arglist'] = '0.000000' 03786 // docTest_item['expect-value'] = '0x000000' 03787 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.000V 03788 if (SelfTestGroupEnable & 0x0040) { 03789 // call-function 03790 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03791 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.000000), (uint32_t)0x000000); // 0.000V 03792 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.000000, /* expect: */ (uint32_t)0x000000); // 0.000V 03793 // halt-on-first-failure logic 03794 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03795 } // if (SelfTestGroupEnable & 0x0040) 03796 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03797 03798 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.010000) expect 0x000a00 // 0.010V 03799 // docTest_item['actionType'] = 'call-function' 03800 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03801 // docTest_item['action'] = 'DACCodeOfVoltage(0.010000) expect 0x000a00' 03802 // docTest_item['remarks'] = '0.010V' 03803 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03804 // docTest_item['arglist'] = '0.010000' 03805 // docTest_item['expect-value'] = '0x000a00' 03806 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.010V 03807 if (SelfTestGroupEnable & 0x0040) { 03808 // call-function 03809 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03810 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.010000), (uint32_t)0x000a00); // 0.010V 03811 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.010000, /* expect: */ (uint32_t)0x000a00); // 0.010V 03812 // halt-on-first-failure logic 03813 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03814 } // if (SelfTestGroupEnable & 0x0040) 03815 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03816 03817 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.020000) expect 0x001400 // 0.020V 03818 // docTest_item['actionType'] = 'call-function' 03819 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03820 // docTest_item['action'] = 'DACCodeOfVoltage(0.020000) expect 0x001400' 03821 // docTest_item['remarks'] = '0.020V' 03822 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03823 // docTest_item['arglist'] = '0.020000' 03824 // docTest_item['expect-value'] = '0x001400' 03825 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.020V 03826 if (SelfTestGroupEnable & 0x0040) { 03827 // call-function 03828 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03829 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.020000), (uint32_t)0x001400); // 0.020V 03830 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.020000, /* expect: */ (uint32_t)0x001400); // 0.020V 03831 // halt-on-first-failure logic 03832 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03833 } // if (SelfTestGroupEnable & 0x0040) 03834 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03835 03836 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.030000) expect 0x001e00 // 0.030V 03837 // docTest_item['actionType'] = 'call-function' 03838 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03839 // docTest_item['action'] = 'DACCodeOfVoltage(0.030000) expect 0x001e00' 03840 // docTest_item['remarks'] = '0.030V' 03841 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03842 // docTest_item['arglist'] = '0.030000' 03843 // docTest_item['expect-value'] = '0x001e00' 03844 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.030V 03845 if (SelfTestGroupEnable & 0x0040) { 03846 // call-function 03847 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03848 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.030000), (uint32_t)0x001e00); // 0.030V 03849 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.030000, /* expect: */ (uint32_t)0x001e00); // 0.030V 03850 // halt-on-first-failure logic 03851 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03852 } // if (SelfTestGroupEnable & 0x0040) 03853 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03854 03855 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.040000) expect 0x002800 // 0.040V 03856 // docTest_item['actionType'] = 'call-function' 03857 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03858 // docTest_item['action'] = 'DACCodeOfVoltage(0.040000) expect 0x002800' 03859 // docTest_item['remarks'] = '0.040V' 03860 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03861 // docTest_item['arglist'] = '0.040000' 03862 // docTest_item['expect-value'] = '0x002800' 03863 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.040V 03864 if (SelfTestGroupEnable & 0x0040) { 03865 // call-function 03866 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03867 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.040000), (uint32_t)0x002800); // 0.040V 03868 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.040000, /* expect: */ (uint32_t)0x002800); // 0.040V 03869 // halt-on-first-failure logic 03870 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03871 } // if (SelfTestGroupEnable & 0x0040) 03872 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03873 03874 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.050000) expect 0x003200 // 0.050V 03875 // docTest_item['actionType'] = 'call-function' 03876 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03877 // docTest_item['action'] = 'DACCodeOfVoltage(0.050000) expect 0x003200' 03878 // docTest_item['remarks'] = '0.050V' 03879 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03880 // docTest_item['arglist'] = '0.050000' 03881 // docTest_item['expect-value'] = '0x003200' 03882 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.050V 03883 if (SelfTestGroupEnable & 0x0040) { 03884 // call-function 03885 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03886 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.050000), (uint32_t)0x003200); // 0.050V 03887 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.050000, /* expect: */ (uint32_t)0x003200); // 0.050V 03888 // halt-on-first-failure logic 03889 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03890 } // if (SelfTestGroupEnable & 0x0040) 03891 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03892 03893 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.060000) expect 0x003c00 // 0.060V 03894 // docTest_item['actionType'] = 'call-function' 03895 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03896 // docTest_item['action'] = 'DACCodeOfVoltage(0.060000) expect 0x003c00' 03897 // docTest_item['remarks'] = '0.060V' 03898 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03899 // docTest_item['arglist'] = '0.060000' 03900 // docTest_item['expect-value'] = '0x003c00' 03901 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.060V 03902 if (SelfTestGroupEnable & 0x0040) { 03903 // call-function 03904 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03905 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.060000), (uint32_t)0x003c00); // 0.060V 03906 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.060000, /* expect: */ (uint32_t)0x003c00); // 0.060V 03907 // halt-on-first-failure logic 03908 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03909 } // if (SelfTestGroupEnable & 0x0040) 03910 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03911 03912 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.070000) expect 0x004600 // 0.070V 03913 // docTest_item['actionType'] = 'call-function' 03914 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03915 // docTest_item['action'] = 'DACCodeOfVoltage(0.070000) expect 0x004600' 03916 // docTest_item['remarks'] = '0.070V' 03917 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03918 // docTest_item['arglist'] = '0.070000' 03919 // docTest_item['expect-value'] = '0x004600' 03920 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.070V 03921 if (SelfTestGroupEnable & 0x0040) { 03922 // call-function 03923 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03924 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.070000), (uint32_t)0x004600); // 0.070V 03925 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.070000, /* expect: */ (uint32_t)0x004600); // 0.070V 03926 // halt-on-first-failure logic 03927 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03928 } // if (SelfTestGroupEnable & 0x0040) 03929 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03930 03931 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.080000) expect 0x005000 // 0.080V 03932 // docTest_item['actionType'] = 'call-function' 03933 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03934 // docTest_item['action'] = 'DACCodeOfVoltage(0.080000) expect 0x005000' 03935 // docTest_item['remarks'] = '0.080V' 03936 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03937 // docTest_item['arglist'] = '0.080000' 03938 // docTest_item['expect-value'] = '0x005000' 03939 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.080V 03940 if (SelfTestGroupEnable & 0x0040) { 03941 // call-function 03942 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03943 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.080000), (uint32_t)0x005000); // 0.080V 03944 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.080000, /* expect: */ (uint32_t)0x005000); // 0.080V 03945 // halt-on-first-failure logic 03946 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03947 } // if (SelfTestGroupEnable & 0x0040) 03948 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03949 03950 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.090000) expect 0x005a00 // 0.090V 03951 // docTest_item['actionType'] = 'call-function' 03952 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03953 // docTest_item['action'] = 'DACCodeOfVoltage(0.090000) expect 0x005a00' 03954 // docTest_item['remarks'] = '0.090V' 03955 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03956 // docTest_item['arglist'] = '0.090000' 03957 // docTest_item['expect-value'] = '0x005a00' 03958 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.090V 03959 if (SelfTestGroupEnable & 0x0040) { 03960 // call-function 03961 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03962 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.090000), (uint32_t)0x005a00); // 0.090V 03963 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.090000, /* expect: */ (uint32_t)0x005a00); // 0.090V 03964 // halt-on-first-failure logic 03965 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03966 } // if (SelfTestGroupEnable & 0x0040) 03967 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03968 03969 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.100000) expect 0x006400 // 0.100V 03970 // docTest_item['actionType'] = 'call-function' 03971 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03972 // docTest_item['action'] = 'DACCodeOfVoltage(0.100000) expect 0x006400' 03973 // docTest_item['remarks'] = '0.100V' 03974 // docTest_item['funcName'] = 'DACCodeOfVoltage' 03975 // docTest_item['arglist'] = '0.100000' 03976 // docTest_item['expect-value'] = '0x006400' 03977 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.100V 03978 if (SelfTestGroupEnable & 0x0040) { 03979 // call-function 03980 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 03981 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.100000), (uint32_t)0x006400); // 0.100V 03982 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.100000, /* expect: */ (uint32_t)0x006400); // 0.100V 03983 // halt-on-first-failure logic 03984 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 03985 } // if (SelfTestGroupEnable & 0x0040) 03986 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 03987 03988 // @test group DACCodeOfVoltage tinyTester.print("test_voltage_sweep V = 2.047900V to 2.048100V precision 0.000010V step 0.000010V") 03989 // docTest_item['actionType'] = 'print-string' 03990 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 03991 // docTest_item['action'] = 'tinyTester.print("test_voltage_sweep V = 2.047900V to 2.048100V precision 0.000010V step 0.000010V")' 03992 // docTest_item['arglist'] = 'test_voltage_sweep V = 2.047900V to 2.048100V precision 0.000010V step 0.000010V' 03993 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage None 03994 if (SelfTestGroupEnable & 0x0040) { 03995 // print-string 03996 // tinyTesterFuncName = "tinyTester.print" 03997 // tinyTesterPrintStringLiteral = "test_voltage_sweep V = 2.047900V to 2.048100V precision 0.000010V step 0.000010V" 03998 tinyTester.print("test_voltage_sweep V = 2.047900V to 2.048100V precision 0.000010V step 0.000010V"); 03999 // halt-on-first-failure logic 04000 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04001 } // if (SelfTestGroupEnable & 0x0040) 04002 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04003 04004 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.047890) expect 0x07ffe4 // 2.048V 04005 // docTest_item['actionType'] = 'call-function' 04006 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04007 // docTest_item['action'] = 'DACCodeOfVoltage(2.047890) expect 0x07ffe4' 04008 // docTest_item['remarks'] = '2.048V' 04009 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04010 // docTest_item['arglist'] = '2.047890' 04011 // docTest_item['expect-value'] = '0x07ffe4' 04012 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04013 if (SelfTestGroupEnable & 0x0040) { 04014 // call-function 04015 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04016 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.047890), (uint32_t)0x07ffe4); // 2.048V 04017 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.047890, /* expect: */ (uint32_t)0x07ffe4); // 2.048V 04018 // halt-on-first-failure logic 04019 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04020 } // if (SelfTestGroupEnable & 0x0040) 04021 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04022 04023 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.047900) expect 0x07ffe6 // 2.048V 04024 // docTest_item['actionType'] = 'call-function' 04025 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04026 // docTest_item['action'] = 'DACCodeOfVoltage(2.047900) expect 0x07ffe6' 04027 // docTest_item['remarks'] = '2.048V' 04028 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04029 // docTest_item['arglist'] = '2.047900' 04030 // docTest_item['expect-value'] = '0x07ffe6' 04031 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04032 if (SelfTestGroupEnable & 0x0040) { 04033 // call-function 04034 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04035 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.047900), (uint32_t)0x07ffe6); // 2.048V 04036 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.047900, /* expect: */ (uint32_t)0x07ffe6); // 2.048V 04037 // halt-on-first-failure logic 04038 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04039 } // if (SelfTestGroupEnable & 0x0040) 04040 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04041 04042 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.047910) expect 0x07ffe9 // 2.048V 04043 // docTest_item['actionType'] = 'call-function' 04044 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04045 // docTest_item['action'] = 'DACCodeOfVoltage(2.047910) expect 0x07ffe9' 04046 // docTest_item['remarks'] = '2.048V' 04047 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04048 // docTest_item['arglist'] = '2.047910' 04049 // docTest_item['expect-value'] = '0x07ffe9' 04050 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04051 if (SelfTestGroupEnable & 0x0040) { 04052 // call-function 04053 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04054 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.047910), (uint32_t)0x07ffe9); // 2.048V 04055 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.047910, /* expect: */ (uint32_t)0x07ffe9); // 2.048V 04056 // halt-on-first-failure logic 04057 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04058 } // if (SelfTestGroupEnable & 0x0040) 04059 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04060 04061 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.047920) expect 0x07ffec // 2.048V 04062 // docTest_item['actionType'] = 'call-function' 04063 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04064 // docTest_item['action'] = 'DACCodeOfVoltage(2.047920) expect 0x07ffec' 04065 // docTest_item['remarks'] = '2.048V' 04066 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04067 // docTest_item['arglist'] = '2.047920' 04068 // docTest_item['expect-value'] = '0x07ffec' 04069 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04070 if (SelfTestGroupEnable & 0x0040) { 04071 // call-function 04072 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04073 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.047920), (uint32_t)0x07ffec); // 2.048V 04074 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.047920, /* expect: */ (uint32_t)0x07ffec); // 2.048V 04075 // halt-on-first-failure logic 04076 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04077 } // if (SelfTestGroupEnable & 0x0040) 04078 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04079 04080 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.047930) expect 0x07ffee // 2.048V 04081 // docTest_item['actionType'] = 'call-function' 04082 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04083 // docTest_item['action'] = 'DACCodeOfVoltage(2.047930) expect 0x07ffee' 04084 // docTest_item['remarks'] = '2.048V' 04085 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04086 // docTest_item['arglist'] = '2.047930' 04087 // docTest_item['expect-value'] = '0x07ffee' 04088 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04089 if (SelfTestGroupEnable & 0x0040) { 04090 // call-function 04091 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04092 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.047930), (uint32_t)0x07ffee); // 2.048V 04093 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.047930, /* expect: */ (uint32_t)0x07ffee); // 2.048V 04094 // halt-on-first-failure logic 04095 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04096 } // if (SelfTestGroupEnable & 0x0040) 04097 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04098 04099 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.047940) expect 0x07fff1 // 2.048V 04100 // docTest_item['actionType'] = 'call-function' 04101 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04102 // docTest_item['action'] = 'DACCodeOfVoltage(2.047940) expect 0x07fff1' 04103 // docTest_item['remarks'] = '2.048V' 04104 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04105 // docTest_item['arglist'] = '2.047940' 04106 // docTest_item['expect-value'] = '0x07fff1' 04107 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04108 if (SelfTestGroupEnable & 0x0040) { 04109 // call-function 04110 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04111 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.047940), (uint32_t)0x07fff1); // 2.048V 04112 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.047940, /* expect: */ (uint32_t)0x07fff1); // 2.048V 04113 // halt-on-first-failure logic 04114 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04115 } // if (SelfTestGroupEnable & 0x0040) 04116 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04117 04118 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.047950) expect 0x07fff3 // 2.048V 04119 // docTest_item['actionType'] = 'call-function' 04120 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04121 // docTest_item['action'] = 'DACCodeOfVoltage(2.047950) expect 0x07fff3' 04122 // docTest_item['remarks'] = '2.048V' 04123 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04124 // docTest_item['arglist'] = '2.047950' 04125 // docTest_item['expect-value'] = '0x07fff3' 04126 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04127 if (SelfTestGroupEnable & 0x0040) { 04128 // call-function 04129 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04130 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.047950), (uint32_t)0x07fff3); // 2.048V 04131 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.047950, /* expect: */ (uint32_t)0x07fff3); // 2.048V 04132 // halt-on-first-failure logic 04133 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04134 } // if (SelfTestGroupEnable & 0x0040) 04135 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04136 04137 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.047960) expect 0x07fff6 // 2.048V 04138 // docTest_item['actionType'] = 'call-function' 04139 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04140 // docTest_item['action'] = 'DACCodeOfVoltage(2.047960) expect 0x07fff6' 04141 // docTest_item['remarks'] = '2.048V' 04142 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04143 // docTest_item['arglist'] = '2.047960' 04144 // docTest_item['expect-value'] = '0x07fff6' 04145 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04146 if (SelfTestGroupEnable & 0x0040) { 04147 // call-function 04148 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04149 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.047960), (uint32_t)0x07fff6); // 2.048V 04150 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.047960, /* expect: */ (uint32_t)0x07fff6); // 2.048V 04151 // halt-on-first-failure logic 04152 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04153 } // if (SelfTestGroupEnable & 0x0040) 04154 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04155 04156 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.047970) expect 0x07fff8 // 2.048V 04157 // docTest_item['actionType'] = 'call-function' 04158 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04159 // docTest_item['action'] = 'DACCodeOfVoltage(2.047970) expect 0x07fff8' 04160 // docTest_item['remarks'] = '2.048V' 04161 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04162 // docTest_item['arglist'] = '2.047970' 04163 // docTest_item['expect-value'] = '0x07fff8' 04164 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04165 if (SelfTestGroupEnable & 0x0040) { 04166 // call-function 04167 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04168 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.047970), (uint32_t)0x07fff8); // 2.048V 04169 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.047970, /* expect: */ (uint32_t)0x07fff8); // 2.048V 04170 // halt-on-first-failure logic 04171 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04172 } // if (SelfTestGroupEnable & 0x0040) 04173 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04174 04175 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.047980) expect 0x07fffb // 2.048V 04176 // docTest_item['actionType'] = 'call-function' 04177 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04178 // docTest_item['action'] = 'DACCodeOfVoltage(2.047980) expect 0x07fffb' 04179 // docTest_item['remarks'] = '2.048V' 04180 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04181 // docTest_item['arglist'] = '2.047980' 04182 // docTest_item['expect-value'] = '0x07fffb' 04183 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04184 if (SelfTestGroupEnable & 0x0040) { 04185 // call-function 04186 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04187 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.047980), (uint32_t)0x07fffb); // 2.048V 04188 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.047980, /* expect: */ (uint32_t)0x07fffb); // 2.048V 04189 // halt-on-first-failure logic 04190 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04191 } // if (SelfTestGroupEnable & 0x0040) 04192 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04193 04194 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.047990) expect 0x07fffd // 2.048V 04195 // docTest_item['actionType'] = 'call-function' 04196 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04197 // docTest_item['action'] = 'DACCodeOfVoltage(2.047990) expect 0x07fffd' 04198 // docTest_item['remarks'] = '2.048V' 04199 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04200 // docTest_item['arglist'] = '2.047990' 04201 // docTest_item['expect-value'] = '0x07fffd' 04202 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04203 if (SelfTestGroupEnable & 0x0040) { 04204 // call-function 04205 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04206 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.047990), (uint32_t)0x07fffd); // 2.048V 04207 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.047990, /* expect: */ (uint32_t)0x07fffd); // 2.048V 04208 // halt-on-first-failure logic 04209 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04210 } // if (SelfTestGroupEnable & 0x0040) 04211 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04212 04213 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.048000) expect 0x080000 // 2.048V 04214 // docTest_item['actionType'] = 'call-function' 04215 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04216 // docTest_item['action'] = 'DACCodeOfVoltage(2.048000) expect 0x080000' 04217 // docTest_item['remarks'] = '2.048V' 04218 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04219 // docTest_item['arglist'] = '2.048000' 04220 // docTest_item['expect-value'] = '0x080000' 04221 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04222 if (SelfTestGroupEnable & 0x0040) { 04223 // call-function 04224 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04225 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.048000), (uint32_t)0x080000); // 2.048V 04226 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.048000, /* expect: */ (uint32_t)0x080000); // 2.048V 04227 // halt-on-first-failure logic 04228 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04229 } // if (SelfTestGroupEnable & 0x0040) 04230 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04231 04232 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.048010) expect 0x080003 // 2.048V 04233 // docTest_item['actionType'] = 'call-function' 04234 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04235 // docTest_item['action'] = 'DACCodeOfVoltage(2.048010) expect 0x080003' 04236 // docTest_item['remarks'] = '2.048V' 04237 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04238 // docTest_item['arglist'] = '2.048010' 04239 // docTest_item['expect-value'] = '0x080003' 04240 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04241 if (SelfTestGroupEnable & 0x0040) { 04242 // call-function 04243 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04244 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.048010), (uint32_t)0x080003); // 2.048V 04245 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.048010, /* expect: */ (uint32_t)0x080003); // 2.048V 04246 // halt-on-first-failure logic 04247 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04248 } // if (SelfTestGroupEnable & 0x0040) 04249 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04250 04251 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.048020) expect 0x080005 // 2.048V 04252 // docTest_item['actionType'] = 'call-function' 04253 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04254 // docTest_item['action'] = 'DACCodeOfVoltage(2.048020) expect 0x080005' 04255 // docTest_item['remarks'] = '2.048V' 04256 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04257 // docTest_item['arglist'] = '2.048020' 04258 // docTest_item['expect-value'] = '0x080005' 04259 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04260 if (SelfTestGroupEnable & 0x0040) { 04261 // call-function 04262 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04263 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.048020), (uint32_t)0x080005); // 2.048V 04264 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.048020, /* expect: */ (uint32_t)0x080005); // 2.048V 04265 // halt-on-first-failure logic 04266 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04267 } // if (SelfTestGroupEnable & 0x0040) 04268 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04269 04270 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.048030) expect 0x080008 // 2.048V 04271 // docTest_item['actionType'] = 'call-function' 04272 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04273 // docTest_item['action'] = 'DACCodeOfVoltage(2.048030) expect 0x080008' 04274 // docTest_item['remarks'] = '2.048V' 04275 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04276 // docTest_item['arglist'] = '2.048030' 04277 // docTest_item['expect-value'] = '0x080008' 04278 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04279 if (SelfTestGroupEnable & 0x0040) { 04280 // call-function 04281 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04282 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.048030), (uint32_t)0x080008); // 2.048V 04283 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.048030, /* expect: */ (uint32_t)0x080008); // 2.048V 04284 // halt-on-first-failure logic 04285 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04286 } // if (SelfTestGroupEnable & 0x0040) 04287 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04288 04289 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.048040) expect 0x08000a // 2.048V 04290 // docTest_item['actionType'] = 'call-function' 04291 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04292 // docTest_item['action'] = 'DACCodeOfVoltage(2.048040) expect 0x08000a' 04293 // docTest_item['remarks'] = '2.048V' 04294 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04295 // docTest_item['arglist'] = '2.048040' 04296 // docTest_item['expect-value'] = '0x08000a' 04297 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04298 if (SelfTestGroupEnable & 0x0040) { 04299 // call-function 04300 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04301 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.048040), (uint32_t)0x08000a); // 2.048V 04302 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.048040, /* expect: */ (uint32_t)0x08000a); // 2.048V 04303 // halt-on-first-failure logic 04304 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04305 } // if (SelfTestGroupEnable & 0x0040) 04306 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04307 04308 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.048050) expect 0x08000d // 2.048V 04309 // docTest_item['actionType'] = 'call-function' 04310 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04311 // docTest_item['action'] = 'DACCodeOfVoltage(2.048050) expect 0x08000d' 04312 // docTest_item['remarks'] = '2.048V' 04313 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04314 // docTest_item['arglist'] = '2.048050' 04315 // docTest_item['expect-value'] = '0x08000d' 04316 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04317 if (SelfTestGroupEnable & 0x0040) { 04318 // call-function 04319 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04320 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.048050), (uint32_t)0x08000d); // 2.048V 04321 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.048050, /* expect: */ (uint32_t)0x08000d); // 2.048V 04322 // halt-on-first-failure logic 04323 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04324 } // if (SelfTestGroupEnable & 0x0040) 04325 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04326 04327 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.048060) expect 0x08000f // 2.048V 04328 // docTest_item['actionType'] = 'call-function' 04329 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04330 // docTest_item['action'] = 'DACCodeOfVoltage(2.048060) expect 0x08000f' 04331 // docTest_item['remarks'] = '2.048V' 04332 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04333 // docTest_item['arglist'] = '2.048060' 04334 // docTest_item['expect-value'] = '0x08000f' 04335 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04336 if (SelfTestGroupEnable & 0x0040) { 04337 // call-function 04338 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04339 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.048060), (uint32_t)0x08000f); // 2.048V 04340 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.048060, /* expect: */ (uint32_t)0x08000f); // 2.048V 04341 // halt-on-first-failure logic 04342 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04343 } // if (SelfTestGroupEnable & 0x0040) 04344 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04345 04346 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.048070) expect 0x080012 // 2.048V 04347 // docTest_item['actionType'] = 'call-function' 04348 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04349 // docTest_item['action'] = 'DACCodeOfVoltage(2.048070) expect 0x080012' 04350 // docTest_item['remarks'] = '2.048V' 04351 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04352 // docTest_item['arglist'] = '2.048070' 04353 // docTest_item['expect-value'] = '0x080012' 04354 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04355 if (SelfTestGroupEnable & 0x0040) { 04356 // call-function 04357 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04358 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.048070), (uint32_t)0x080012); // 2.048V 04359 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.048070, /* expect: */ (uint32_t)0x080012); // 2.048V 04360 // halt-on-first-failure logic 04361 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04362 } // if (SelfTestGroupEnable & 0x0040) 04363 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04364 04365 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.048080) expect 0x080014 // 2.048V 04366 // docTest_item['actionType'] = 'call-function' 04367 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04368 // docTest_item['action'] = 'DACCodeOfVoltage(2.048080) expect 0x080014' 04369 // docTest_item['remarks'] = '2.048V' 04370 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04371 // docTest_item['arglist'] = '2.048080' 04372 // docTest_item['expect-value'] = '0x080014' 04373 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04374 if (SelfTestGroupEnable & 0x0040) { 04375 // call-function 04376 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04377 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.048080), (uint32_t)0x080014); // 2.048V 04378 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.048080, /* expect: */ (uint32_t)0x080014); // 2.048V 04379 // halt-on-first-failure logic 04380 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04381 } // if (SelfTestGroupEnable & 0x0040) 04382 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04383 04384 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.048090) expect 0x080017 // 2.048V 04385 // docTest_item['actionType'] = 'call-function' 04386 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04387 // docTest_item['action'] = 'DACCodeOfVoltage(2.048090) expect 0x080017' 04388 // docTest_item['remarks'] = '2.048V' 04389 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04390 // docTest_item['arglist'] = '2.048090' 04391 // docTest_item['expect-value'] = '0x080017' 04392 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04393 if (SelfTestGroupEnable & 0x0040) { 04394 // call-function 04395 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04396 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.048090), (uint32_t)0x080017); // 2.048V 04397 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.048090, /* expect: */ (uint32_t)0x080017); // 2.048V 04398 // halt-on-first-failure logic 04399 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04400 } // if (SelfTestGroupEnable & 0x0040) 04401 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04402 04403 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.048100) expect 0x08001a // 2.048V 04404 // docTest_item['actionType'] = 'call-function' 04405 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04406 // docTest_item['action'] = 'DACCodeOfVoltage(2.048100) expect 0x08001a' 04407 // docTest_item['remarks'] = '2.048V' 04408 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04409 // docTest_item['arglist'] = '2.048100' 04410 // docTest_item['expect-value'] = '0x08001a' 04411 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V 04412 if (SelfTestGroupEnable & 0x0040) { 04413 // call-function 04414 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04415 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.048100), (uint32_t)0x08001a); // 2.048V 04416 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.048100, /* expect: */ (uint32_t)0x08001a); // 2.048V 04417 // halt-on-first-failure logic 04418 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04419 } // if (SelfTestGroupEnable & 0x0040) 04420 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04421 04422 // @test group DACCodeOfVoltage tinyTester.print("test_voltage_sweep V = 3.996000V to 4.106000V precision 0.010000V step 0.010000V") 04423 // docTest_item['actionType'] = 'print-string' 04424 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04425 // docTest_item['action'] = 'tinyTester.print("test_voltage_sweep V = 3.996000V to 4.106000V precision 0.010000V step 0.010000V")' 04426 // docTest_item['arglist'] = 'test_voltage_sweep V = 3.996000V to 4.106000V precision 0.010000V step 0.010000V' 04427 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage None 04428 if (SelfTestGroupEnable & 0x0040) { 04429 // print-string 04430 // tinyTesterFuncName = "tinyTester.print" 04431 // tinyTesterPrintStringLiteral = "test_voltage_sweep V = 3.996000V to 4.106000V precision 0.010000V step 0.010000V" 04432 tinyTester.print("test_voltage_sweep V = 3.996000V to 4.106000V precision 0.010000V step 0.010000V"); 04433 // halt-on-first-failure logic 04434 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04435 } // if (SelfTestGroupEnable & 0x0040) 04436 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04437 04438 // @test group DACCodeOfVoltage DACCodeOfVoltage(3.990000) expect 0x0f9600 // 3.990V 04439 // docTest_item['actionType'] = 'call-function' 04440 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04441 // docTest_item['action'] = 'DACCodeOfVoltage(3.990000) expect 0x0f9600' 04442 // docTest_item['remarks'] = '3.990V' 04443 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04444 // docTest_item['arglist'] = '3.990000' 04445 // docTest_item['expect-value'] = '0x0f9600' 04446 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 3.990V 04447 if (SelfTestGroupEnable & 0x0040) { 04448 // call-function 04449 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04450 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)3.990000), (uint32_t)0x0f9600); // 3.990V 04451 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)3.990000, /* expect: */ (uint32_t)0x0f9600); // 3.990V 04452 // halt-on-first-failure logic 04453 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04454 } // if (SelfTestGroupEnable & 0x0040) 04455 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04456 04457 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.000000) expect 0x0fa000 // 4.000V 04458 // docTest_item['actionType'] = 'call-function' 04459 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04460 // docTest_item['action'] = 'DACCodeOfVoltage(4.000000) expect 0x0fa000' 04461 // docTest_item['remarks'] = '4.000V' 04462 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04463 // docTest_item['arglist'] = '4.000000' 04464 // docTest_item['expect-value'] = '0x0fa000' 04465 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.000V 04466 if (SelfTestGroupEnable & 0x0040) { 04467 // call-function 04468 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04469 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.000000), (uint32_t)0x0fa000); // 4.000V 04470 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.000000, /* expect: */ (uint32_t)0x0fa000); // 4.000V 04471 // halt-on-first-failure logic 04472 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04473 } // if (SelfTestGroupEnable & 0x0040) 04474 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04475 04476 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.010000) expect 0x0faa00 // 4.010V 04477 // docTest_item['actionType'] = 'call-function' 04478 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04479 // docTest_item['action'] = 'DACCodeOfVoltage(4.010000) expect 0x0faa00' 04480 // docTest_item['remarks'] = '4.010V' 04481 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04482 // docTest_item['arglist'] = '4.010000' 04483 // docTest_item['expect-value'] = '0x0faa00' 04484 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.010V 04485 if (SelfTestGroupEnable & 0x0040) { 04486 // call-function 04487 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04488 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.010000), (uint32_t)0x0faa00); // 4.010V 04489 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.010000, /* expect: */ (uint32_t)0x0faa00); // 4.010V 04490 // halt-on-first-failure logic 04491 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04492 } // if (SelfTestGroupEnable & 0x0040) 04493 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04494 04495 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.020000) expect 0x0fb400 // 4.020V 04496 // docTest_item['actionType'] = 'call-function' 04497 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04498 // docTest_item['action'] = 'DACCodeOfVoltage(4.020000) expect 0x0fb400' 04499 // docTest_item['remarks'] = '4.020V' 04500 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04501 // docTest_item['arglist'] = '4.020000' 04502 // docTest_item['expect-value'] = '0x0fb400' 04503 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.020V 04504 if (SelfTestGroupEnable & 0x0040) { 04505 // call-function 04506 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04507 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.020000), (uint32_t)0x0fb400); // 4.020V 04508 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.020000, /* expect: */ (uint32_t)0x0fb400); // 4.020V 04509 // halt-on-first-failure logic 04510 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04511 } // if (SelfTestGroupEnable & 0x0040) 04512 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04513 04514 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.030000) expect 0x0fbe00 // 4.030V 04515 // docTest_item['actionType'] = 'call-function' 04516 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04517 // docTest_item['action'] = 'DACCodeOfVoltage(4.030000) expect 0x0fbe00' 04518 // docTest_item['remarks'] = '4.030V' 04519 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04520 // docTest_item['arglist'] = '4.030000' 04521 // docTest_item['expect-value'] = '0x0fbe00' 04522 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.030V 04523 if (SelfTestGroupEnable & 0x0040) { 04524 // call-function 04525 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04526 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.030000), (uint32_t)0x0fbe00); // 4.030V 04527 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.030000, /* expect: */ (uint32_t)0x0fbe00); // 4.030V 04528 // halt-on-first-failure logic 04529 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04530 } // if (SelfTestGroupEnable & 0x0040) 04531 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04532 04533 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.040000) expect 0x0fc800 // 4.040V 04534 // docTest_item['actionType'] = 'call-function' 04535 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04536 // docTest_item['action'] = 'DACCodeOfVoltage(4.040000) expect 0x0fc800' 04537 // docTest_item['remarks'] = '4.040V' 04538 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04539 // docTest_item['arglist'] = '4.040000' 04540 // docTest_item['expect-value'] = '0x0fc800' 04541 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.040V 04542 if (SelfTestGroupEnable & 0x0040) { 04543 // call-function 04544 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04545 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.040000), (uint32_t)0x0fc800); // 4.040V 04546 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.040000, /* expect: */ (uint32_t)0x0fc800); // 4.040V 04547 // halt-on-first-failure logic 04548 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04549 } // if (SelfTestGroupEnable & 0x0040) 04550 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04551 04552 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.050000) expect 0x0fd200 // 4.050V 04553 // docTest_item['actionType'] = 'call-function' 04554 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04555 // docTest_item['action'] = 'DACCodeOfVoltage(4.050000) expect 0x0fd200' 04556 // docTest_item['remarks'] = '4.050V' 04557 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04558 // docTest_item['arglist'] = '4.050000' 04559 // docTest_item['expect-value'] = '0x0fd200' 04560 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.050V 04561 if (SelfTestGroupEnable & 0x0040) { 04562 // call-function 04563 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04564 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.050000), (uint32_t)0x0fd200); // 4.050V 04565 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.050000, /* expect: */ (uint32_t)0x0fd200); // 4.050V 04566 // halt-on-first-failure logic 04567 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04568 } // if (SelfTestGroupEnable & 0x0040) 04569 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04570 04571 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.060000) expect 0x0fdc00 // 4.060V 04572 // docTest_item['actionType'] = 'call-function' 04573 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04574 // docTest_item['action'] = 'DACCodeOfVoltage(4.060000) expect 0x0fdc00' 04575 // docTest_item['remarks'] = '4.060V' 04576 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04577 // docTest_item['arglist'] = '4.060000' 04578 // docTest_item['expect-value'] = '0x0fdc00' 04579 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.060V 04580 if (SelfTestGroupEnable & 0x0040) { 04581 // call-function 04582 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04583 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.060000), (uint32_t)0x0fdc00); // 4.060V 04584 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.060000, /* expect: */ (uint32_t)0x0fdc00); // 4.060V 04585 // halt-on-first-failure logic 04586 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04587 } // if (SelfTestGroupEnable & 0x0040) 04588 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04589 04590 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.070000) expect 0x0fe600 // 4.070V 04591 // docTest_item['actionType'] = 'call-function' 04592 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04593 // docTest_item['action'] = 'DACCodeOfVoltage(4.070000) expect 0x0fe600' 04594 // docTest_item['remarks'] = '4.070V' 04595 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04596 // docTest_item['arglist'] = '4.070000' 04597 // docTest_item['expect-value'] = '0x0fe600' 04598 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.070V 04599 if (SelfTestGroupEnable & 0x0040) { 04600 // call-function 04601 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04602 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.070000), (uint32_t)0x0fe600); // 4.070V 04603 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.070000, /* expect: */ (uint32_t)0x0fe600); // 4.070V 04604 // halt-on-first-failure logic 04605 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04606 } // if (SelfTestGroupEnable & 0x0040) 04607 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04608 04609 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.080000) expect 0x0ff000 // 4.080V 04610 // docTest_item['actionType'] = 'call-function' 04611 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04612 // docTest_item['action'] = 'DACCodeOfVoltage(4.080000) expect 0x0ff000' 04613 // docTest_item['remarks'] = '4.080V' 04614 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04615 // docTest_item['arglist'] = '4.080000' 04616 // docTest_item['expect-value'] = '0x0ff000' 04617 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.080V 04618 if (SelfTestGroupEnable & 0x0040) { 04619 // call-function 04620 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04621 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.080000), (uint32_t)0x0ff000); // 4.080V 04622 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.080000, /* expect: */ (uint32_t)0x0ff000); // 4.080V 04623 // halt-on-first-failure logic 04624 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04625 } // if (SelfTestGroupEnable & 0x0040) 04626 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04627 04628 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.090000) expect 0x0ffa00 // 4.090V 04629 // docTest_item['actionType'] = 'call-function' 04630 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04631 // docTest_item['action'] = 'DACCodeOfVoltage(4.090000) expect 0x0ffa00' 04632 // docTest_item['remarks'] = '4.090V' 04633 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04634 // docTest_item['arglist'] = '4.090000' 04635 // docTest_item['expect-value'] = '0x0ffa00' 04636 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.090V 04637 if (SelfTestGroupEnable & 0x0040) { 04638 // call-function 04639 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04640 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.090000), (uint32_t)0x0ffa00); // 4.090V 04641 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.090000, /* expect: */ (uint32_t)0x0ffa00); // 4.090V 04642 // halt-on-first-failure logic 04643 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04644 } // if (SelfTestGroupEnable & 0x0040) 04645 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04646 04647 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.100000) expect 0x0fffff // 4.100V 04648 // docTest_item['actionType'] = 'call-function' 04649 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04650 // docTest_item['action'] = 'DACCodeOfVoltage(4.100000) expect 0x0fffff' 04651 // docTest_item['remarks'] = '4.100V' 04652 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04653 // docTest_item['arglist'] = '4.100000' 04654 // docTest_item['expect-value'] = '0x0fffff' 04655 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.100V 04656 if (SelfTestGroupEnable & 0x0040) { 04657 // call-function 04658 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04659 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.100000), (uint32_t)0x0fffff); // 4.100V 04660 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.100000, /* expect: */ (uint32_t)0x0fffff); // 4.100V 04661 // halt-on-first-failure logic 04662 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04663 } // if (SelfTestGroupEnable & 0x0040) 04664 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04665 04666 // @test group DACCodeOfVoltage tinyTester.print("test_lsb_sweep V = 4.096000V LSBradius = 3LSB") 04667 // docTest_item['actionType'] = 'print-string' 04668 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04669 // docTest_item['action'] = 'tinyTester.print("test_lsb_sweep V = 4.096000V LSBradius = 3LSB")' 04670 // docTest_item['arglist'] = 'test_lsb_sweep V = 4.096000V LSBradius = 3LSB' 04671 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage None 04672 if (SelfTestGroupEnable & 0x0040) { 04673 // print-string 04674 // tinyTesterFuncName = "tinyTester.print" 04675 // tinyTesterPrintStringLiteral = "test_lsb_sweep V = 4.096000V LSBradius = 3LSB" 04676 tinyTester.print("test_lsb_sweep V = 4.096000V LSBradius = 3LSB"); 04677 // halt-on-first-failure logic 04678 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04679 } // if (SelfTestGroupEnable & 0x0040) 04680 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04681 04682 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.095988) expect 0x0ffffd // 4.096V + -3.0LSB 04683 // docTest_item['actionType'] = 'call-function' 04684 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04685 // docTest_item['action'] = 'DACCodeOfVoltage(4.095988) expect 0x0ffffd' 04686 // docTest_item['remarks'] = '4.096V + -3.0LSB' 04687 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04688 // docTest_item['arglist'] = '4.095988' 04689 // docTest_item['expect-value'] = '0x0ffffd' 04690 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.096V + -3.0LSB 04691 if (SelfTestGroupEnable & 0x0040) { 04692 // call-function 04693 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04694 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.095988), (uint32_t)0x0ffffd); // 4.096V + -3.0LSB 04695 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.095988, /* expect: */ (uint32_t)0x0ffffd); // 4.096V + -3.0LSB 04696 // halt-on-first-failure logic 04697 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04698 } // if (SelfTestGroupEnable & 0x0040) 04699 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04700 04701 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.095990) expect 0x0ffffd // 4.096V + -2.5LSB 0x0ffffd not 0x0ffffe 04702 // docTest_item['actionType'] = 'call-function' 04703 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04704 // docTest_item['action'] = 'DACCodeOfVoltage(4.095990) expect 0x0ffffd' 04705 // docTest_item['remarks'] = '4.096V + -2.5LSB 0x0ffffd not 0x0ffffe' 04706 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04707 // docTest_item['arglist'] = '4.095990' 04708 // docTest_item['expect-value'] = '0x0ffffd' 04709 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.096V + -2.5LSB 0x0ffffd not 0x0ffffe 04710 if (SelfTestGroupEnable & 0x0040) { 04711 // call-function 04712 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04713 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.095990), (uint32_t)0x0ffffd); // 4.096V + -2.5LSB 0x0ffffd not 0x0ffffe 04714 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.095990, /* expect: */ (uint32_t)0x0ffffd); // 4.096V + -2.5LSB 0x0ffffd not 0x0ffffe 04715 // halt-on-first-failure logic 04716 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04717 } // if (SelfTestGroupEnable & 0x0040) 04718 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04719 04720 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.095992) expect 0x0ffffe // 4.096V + -2.0LSB 04721 // docTest_item['actionType'] = 'call-function' 04722 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04723 // docTest_item['action'] = 'DACCodeOfVoltage(4.095992) expect 0x0ffffe' 04724 // docTest_item['remarks'] = '4.096V + -2.0LSB' 04725 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04726 // docTest_item['arglist'] = '4.095992' 04727 // docTest_item['expect-value'] = '0x0ffffe' 04728 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.096V + -2.0LSB 04729 if (SelfTestGroupEnable & 0x0040) { 04730 // call-function 04731 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04732 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.095992), (uint32_t)0x0ffffe); // 4.096V + -2.0LSB 04733 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.095992, /* expect: */ (uint32_t)0x0ffffe); // 4.096V + -2.0LSB 04734 // halt-on-first-failure logic 04735 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04736 } // if (SelfTestGroupEnable & 0x0040) 04737 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04738 04739 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.095994) expect 0x0ffffe // 4.096V + -1.5LSB 04740 // docTest_item['actionType'] = 'call-function' 04741 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04742 // docTest_item['action'] = 'DACCodeOfVoltage(4.095994) expect 0x0ffffe' 04743 // docTest_item['remarks'] = '4.096V + -1.5LSB' 04744 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04745 // docTest_item['arglist'] = '4.095994' 04746 // docTest_item['expect-value'] = '0x0ffffe' 04747 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.096V + -1.5LSB 04748 if (SelfTestGroupEnable & 0x0040) { 04749 // call-function 04750 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04751 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.095994), (uint32_t)0x0ffffe); // 4.096V + -1.5LSB 04752 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.095994, /* expect: */ (uint32_t)0x0ffffe); // 4.096V + -1.5LSB 04753 // halt-on-first-failure logic 04754 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04755 } // if (SelfTestGroupEnable & 0x0040) 04756 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04757 04758 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.095996) expect 0x0fffff // 4.096V + -1.0LSB 04759 // docTest_item['actionType'] = 'call-function' 04760 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04761 // docTest_item['action'] = 'DACCodeOfVoltage(4.095996) expect 0x0fffff' 04762 // docTest_item['remarks'] = '4.096V + -1.0LSB' 04763 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04764 // docTest_item['arglist'] = '4.095996' 04765 // docTest_item['expect-value'] = '0x0fffff' 04766 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.096V + -1.0LSB 04767 if (SelfTestGroupEnable & 0x0040) { 04768 // call-function 04769 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04770 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.095996), (uint32_t)0x0fffff); // 4.096V + -1.0LSB 04771 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.095996, /* expect: */ (uint32_t)0x0fffff); // 4.096V + -1.0LSB 04772 // halt-on-first-failure logic 04773 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04774 } // if (SelfTestGroupEnable & 0x0040) 04775 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04776 04777 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.095998) expect 0x0fffff // 4.096V + -0.5LSB 04778 // docTest_item['actionType'] = 'call-function' 04779 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04780 // docTest_item['action'] = 'DACCodeOfVoltage(4.095998) expect 0x0fffff' 04781 // docTest_item['remarks'] = '4.096V + -0.5LSB' 04782 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04783 // docTest_item['arglist'] = '4.095998' 04784 // docTest_item['expect-value'] = '0x0fffff' 04785 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.096V + -0.5LSB 04786 if (SelfTestGroupEnable & 0x0040) { 04787 // call-function 04788 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04789 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.095998), (uint32_t)0x0fffff); // 4.096V + -0.5LSB 04790 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.095998, /* expect: */ (uint32_t)0x0fffff); // 4.096V + -0.5LSB 04791 // halt-on-first-failure logic 04792 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04793 } // if (SelfTestGroupEnable & 0x0040) 04794 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04795 04796 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.096000) expect 0x0fffff // 4.096V + 0.0LSB 04797 // docTest_item['actionType'] = 'call-function' 04798 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04799 // docTest_item['action'] = 'DACCodeOfVoltage(4.096000) expect 0x0fffff' 04800 // docTest_item['remarks'] = '4.096V + 0.0LSB' 04801 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04802 // docTest_item['arglist'] = '4.096000' 04803 // docTest_item['expect-value'] = '0x0fffff' 04804 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.096V + 0.0LSB 04805 if (SelfTestGroupEnable & 0x0040) { 04806 // call-function 04807 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04808 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.096000), (uint32_t)0x0fffff); // 4.096V + 0.0LSB 04809 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.096000, /* expect: */ (uint32_t)0x0fffff); // 4.096V + 0.0LSB 04810 // halt-on-first-failure logic 04811 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04812 } // if (SelfTestGroupEnable & 0x0040) 04813 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04814 04815 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.096002) expect 0x0fffff // 4.096V + 0.5LSB 04816 // docTest_item['actionType'] = 'call-function' 04817 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04818 // docTest_item['action'] = 'DACCodeOfVoltage(4.096002) expect 0x0fffff' 04819 // docTest_item['remarks'] = '4.096V + 0.5LSB' 04820 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04821 // docTest_item['arglist'] = '4.096002' 04822 // docTest_item['expect-value'] = '0x0fffff' 04823 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.096V + 0.5LSB 04824 if (SelfTestGroupEnable & 0x0040) { 04825 // call-function 04826 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04827 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.096002), (uint32_t)0x0fffff); // 4.096V + 0.5LSB 04828 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.096002, /* expect: */ (uint32_t)0x0fffff); // 4.096V + 0.5LSB 04829 // halt-on-first-failure logic 04830 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04831 } // if (SelfTestGroupEnable & 0x0040) 04832 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04833 04834 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.096004) expect 0x0fffff // 4.096V + 1.0LSB 04835 // docTest_item['actionType'] = 'call-function' 04836 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04837 // docTest_item['action'] = 'DACCodeOfVoltage(4.096004) expect 0x0fffff' 04838 // docTest_item['remarks'] = '4.096V + 1.0LSB' 04839 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04840 // docTest_item['arglist'] = '4.096004' 04841 // docTest_item['expect-value'] = '0x0fffff' 04842 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.096V + 1.0LSB 04843 if (SelfTestGroupEnable & 0x0040) { 04844 // call-function 04845 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04846 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.096004), (uint32_t)0x0fffff); // 4.096V + 1.0LSB 04847 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.096004, /* expect: */ (uint32_t)0x0fffff); // 4.096V + 1.0LSB 04848 // halt-on-first-failure logic 04849 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04850 } // if (SelfTestGroupEnable & 0x0040) 04851 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04852 04853 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.096006) expect 0x0fffff // 4.096V + 1.5LSB 04854 // docTest_item['actionType'] = 'call-function' 04855 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04856 // docTest_item['action'] = 'DACCodeOfVoltage(4.096006) expect 0x0fffff' 04857 // docTest_item['remarks'] = '4.096V + 1.5LSB' 04858 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04859 // docTest_item['arglist'] = '4.096006' 04860 // docTest_item['expect-value'] = '0x0fffff' 04861 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.096V + 1.5LSB 04862 if (SelfTestGroupEnable & 0x0040) { 04863 // call-function 04864 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04865 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.096006), (uint32_t)0x0fffff); // 4.096V + 1.5LSB 04866 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.096006, /* expect: */ (uint32_t)0x0fffff); // 4.096V + 1.5LSB 04867 // halt-on-first-failure logic 04868 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04869 } // if (SelfTestGroupEnable & 0x0040) 04870 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04871 04872 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.096008) expect 0x0fffff // 4.096V + 2.0LSB 04873 // docTest_item['actionType'] = 'call-function' 04874 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04875 // docTest_item['action'] = 'DACCodeOfVoltage(4.096008) expect 0x0fffff' 04876 // docTest_item['remarks'] = '4.096V + 2.0LSB' 04877 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04878 // docTest_item['arglist'] = '4.096008' 04879 // docTest_item['expect-value'] = '0x0fffff' 04880 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.096V + 2.0LSB 04881 if (SelfTestGroupEnable & 0x0040) { 04882 // call-function 04883 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04884 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.096008), (uint32_t)0x0fffff); // 4.096V + 2.0LSB 04885 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.096008, /* expect: */ (uint32_t)0x0fffff); // 4.096V + 2.0LSB 04886 // halt-on-first-failure logic 04887 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04888 } // if (SelfTestGroupEnable & 0x0040) 04889 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04890 04891 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.096010) expect 0x0fffff // 4.096V + 2.5LSB 04892 // docTest_item['actionType'] = 'call-function' 04893 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04894 // docTest_item['action'] = 'DACCodeOfVoltage(4.096010) expect 0x0fffff' 04895 // docTest_item['remarks'] = '4.096V + 2.5LSB' 04896 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04897 // docTest_item['arglist'] = '4.096010' 04898 // docTest_item['expect-value'] = '0x0fffff' 04899 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.096V + 2.5LSB 04900 if (SelfTestGroupEnable & 0x0040) { 04901 // call-function 04902 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04903 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.096010), (uint32_t)0x0fffff); // 4.096V + 2.5LSB 04904 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.096010, /* expect: */ (uint32_t)0x0fffff); // 4.096V + 2.5LSB 04905 // halt-on-first-failure logic 04906 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04907 } // if (SelfTestGroupEnable & 0x0040) 04908 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04909 04910 // @test group DACCodeOfVoltage DACCodeOfVoltage(4.096012) expect 0x0fffff // 4.096V + 3.0LSB 04911 // docTest_item['actionType'] = 'call-function' 04912 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04913 // docTest_item['action'] = 'DACCodeOfVoltage(4.096012) expect 0x0fffff' 04914 // docTest_item['remarks'] = '4.096V + 3.0LSB' 04915 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04916 // docTest_item['arglist'] = '4.096012' 04917 // docTest_item['expect-value'] = '0x0fffff' 04918 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 4.096V + 3.0LSB 04919 if (SelfTestGroupEnable & 0x0040) { 04920 // call-function 04921 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04922 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)4.096012), (uint32_t)0x0fffff); // 4.096V + 3.0LSB 04923 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)4.096012, /* expect: */ (uint32_t)0x0fffff); // 4.096V + 3.0LSB 04924 // halt-on-first-failure logic 04925 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04926 } // if (SelfTestGroupEnable & 0x0040) 04927 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04928 04929 // @test group DACCodeOfVoltage tinyTester.print("test_lsb_sweep V = 3.072000V LSBradius = 3LSB") 04930 // docTest_item['actionType'] = 'print-string' 04931 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04932 // docTest_item['action'] = 'tinyTester.print("test_lsb_sweep V = 3.072000V LSBradius = 3LSB")' 04933 // docTest_item['arglist'] = 'test_lsb_sweep V = 3.072000V LSBradius = 3LSB' 04934 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage None 04935 if (SelfTestGroupEnable & 0x0040) { 04936 // print-string 04937 // tinyTesterFuncName = "tinyTester.print" 04938 // tinyTesterPrintStringLiteral = "test_lsb_sweep V = 3.072000V LSBradius = 3LSB" 04939 tinyTester.print("test_lsb_sweep V = 3.072000V LSBradius = 3LSB"); 04940 // halt-on-first-failure logic 04941 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04942 } // if (SelfTestGroupEnable & 0x0040) 04943 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04944 04945 // @test group DACCodeOfVoltage DACCodeOfVoltage(3.071988) expect 0x0bfffd // 3.072V + -3.0LSB 04946 // docTest_item['actionType'] = 'call-function' 04947 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04948 // docTest_item['action'] = 'DACCodeOfVoltage(3.071988) expect 0x0bfffd' 04949 // docTest_item['remarks'] = '3.072V + -3.0LSB' 04950 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04951 // docTest_item['arglist'] = '3.071988' 04952 // docTest_item['expect-value'] = '0x0bfffd' 04953 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 3.072V + -3.0LSB 04954 if (SelfTestGroupEnable & 0x0040) { 04955 // call-function 04956 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04957 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)3.071988), (uint32_t)0x0bfffd); // 3.072V + -3.0LSB 04958 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)3.071988, /* expect: */ (uint32_t)0x0bfffd); // 3.072V + -3.0LSB 04959 // halt-on-first-failure logic 04960 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04961 } // if (SelfTestGroupEnable & 0x0040) 04962 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04963 04964 // @test group DACCodeOfVoltage DACCodeOfVoltage(3.071990) expect 0x0bfffd // 3.072V + -2.5LSB 0x0bfffd not 0x0bfffe 04965 // docTest_item['actionType'] = 'call-function' 04966 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04967 // docTest_item['action'] = 'DACCodeOfVoltage(3.071990) expect 0x0bfffd' 04968 // docTest_item['remarks'] = '3.072V + -2.5LSB 0x0bfffd not 0x0bfffe' 04969 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04970 // docTest_item['arglist'] = '3.071990' 04971 // docTest_item['expect-value'] = '0x0bfffd' 04972 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 3.072V + -2.5LSB 0x0bfffd not 0x0bfffe 04973 if (SelfTestGroupEnable & 0x0040) { 04974 // call-function 04975 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04976 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)3.071990), (uint32_t)0x0bfffd); // 3.072V + -2.5LSB 0x0bfffd not 0x0bfffe 04977 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)3.071990, /* expect: */ (uint32_t)0x0bfffd); // 3.072V + -2.5LSB 0x0bfffd not 0x0bfffe 04978 // halt-on-first-failure logic 04979 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04980 } // if (SelfTestGroupEnable & 0x0040) 04981 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 04982 04983 // @test group DACCodeOfVoltage DACCodeOfVoltage(3.071992) expect 0x0bfffe // 3.072V + -2.0LSB 04984 // docTest_item['actionType'] = 'call-function' 04985 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 04986 // docTest_item['action'] = 'DACCodeOfVoltage(3.071992) expect 0x0bfffe' 04987 // docTest_item['remarks'] = '3.072V + -2.0LSB' 04988 // docTest_item['funcName'] = 'DACCodeOfVoltage' 04989 // docTest_item['arglist'] = '3.071992' 04990 // docTest_item['expect-value'] = '0x0bfffe' 04991 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 3.072V + -2.0LSB 04992 if (SelfTestGroupEnable & 0x0040) { 04993 // call-function 04994 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 04995 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)3.071992), (uint32_t)0x0bfffe); // 3.072V + -2.0LSB 04996 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)3.071992, /* expect: */ (uint32_t)0x0bfffe); // 3.072V + -2.0LSB 04997 // halt-on-first-failure logic 04998 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 04999 } // if (SelfTestGroupEnable & 0x0040) 05000 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05001 05002 // @test group DACCodeOfVoltage DACCodeOfVoltage(3.071994) expect 0x0bfffe // 3.072V + -1.5LSB 0x0bfffe not 0x0bffff 05003 // docTest_item['actionType'] = 'call-function' 05004 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05005 // docTest_item['action'] = 'DACCodeOfVoltage(3.071994) expect 0x0bfffe' 05006 // docTest_item['remarks'] = '3.072V + -1.5LSB 0x0bfffe not 0x0bffff' 05007 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05008 // docTest_item['arglist'] = '3.071994' 05009 // docTest_item['expect-value'] = '0x0bfffe' 05010 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 3.072V + -1.5LSB 0x0bfffe not 0x0bffff 05011 if (SelfTestGroupEnable & 0x0040) { 05012 // call-function 05013 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05014 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)3.071994), (uint32_t)0x0bfffe); // 3.072V + -1.5LSB 0x0bfffe not 0x0bffff 05015 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)3.071994, /* expect: */ (uint32_t)0x0bfffe); // 3.072V + -1.5LSB 0x0bfffe not 0x0bffff 05016 // halt-on-first-failure logic 05017 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05018 } // if (SelfTestGroupEnable & 0x0040) 05019 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05020 05021 // @test group DACCodeOfVoltage DACCodeOfVoltage(3.071996) expect 0x0bffff // 3.072V + -1.0LSB 05022 // docTest_item['actionType'] = 'call-function' 05023 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05024 // docTest_item['action'] = 'DACCodeOfVoltage(3.071996) expect 0x0bffff' 05025 // docTest_item['remarks'] = '3.072V + -1.0LSB' 05026 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05027 // docTest_item['arglist'] = '3.071996' 05028 // docTest_item['expect-value'] = '0x0bffff' 05029 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 3.072V + -1.0LSB 05030 if (SelfTestGroupEnable & 0x0040) { 05031 // call-function 05032 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05033 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)3.071996), (uint32_t)0x0bffff); // 3.072V + -1.0LSB 05034 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)3.071996, /* expect: */ (uint32_t)0x0bffff); // 3.072V + -1.0LSB 05035 // halt-on-first-failure logic 05036 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05037 } // if (SelfTestGroupEnable & 0x0040) 05038 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05039 05040 // @test group DACCodeOfVoltage DACCodeOfVoltage(3.071998) expect 0x0bffff // 3.072V + -0.5LSB 0x0bffff not 0x0c0000 05041 // docTest_item['actionType'] = 'call-function' 05042 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05043 // docTest_item['action'] = 'DACCodeOfVoltage(3.071998) expect 0x0bffff' 05044 // docTest_item['remarks'] = '3.072V + -0.5LSB 0x0bffff not 0x0c0000' 05045 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05046 // docTest_item['arglist'] = '3.071998' 05047 // docTest_item['expect-value'] = '0x0bffff' 05048 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 3.072V + -0.5LSB 0x0bffff not 0x0c0000 05049 if (SelfTestGroupEnable & 0x0040) { 05050 // call-function 05051 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05052 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)3.071998), (uint32_t)0x0bffff); // 3.072V + -0.5LSB 0x0bffff not 0x0c0000 05053 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)3.071998, /* expect: */ (uint32_t)0x0bffff); // 3.072V + -0.5LSB 0x0bffff not 0x0c0000 05054 // halt-on-first-failure logic 05055 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05056 } // if (SelfTestGroupEnable & 0x0040) 05057 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05058 05059 // @test group DACCodeOfVoltage DACCodeOfVoltage(3.072000) expect 0x0c0000 // 3.072V + 0.0LSB 05060 // docTest_item['actionType'] = 'call-function' 05061 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05062 // docTest_item['action'] = 'DACCodeOfVoltage(3.072000) expect 0x0c0000' 05063 // docTest_item['remarks'] = '3.072V + 0.0LSB' 05064 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05065 // docTest_item['arglist'] = '3.072000' 05066 // docTest_item['expect-value'] = '0x0c0000' 05067 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 3.072V + 0.0LSB 05068 if (SelfTestGroupEnable & 0x0040) { 05069 // call-function 05070 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05071 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)3.072000), (uint32_t)0x0c0000); // 3.072V + 0.0LSB 05072 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)3.072000, /* expect: */ (uint32_t)0x0c0000); // 3.072V + 0.0LSB 05073 // halt-on-first-failure logic 05074 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05075 } // if (SelfTestGroupEnable & 0x0040) 05076 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05077 05078 // @test group DACCodeOfVoltage DACCodeOfVoltage(3.072002) expect 0x0c0001 // 3.072V + 0.5LSB 05079 // docTest_item['actionType'] = 'call-function' 05080 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05081 // docTest_item['action'] = 'DACCodeOfVoltage(3.072002) expect 0x0c0001' 05082 // docTest_item['remarks'] = '3.072V + 0.5LSB' 05083 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05084 // docTest_item['arglist'] = '3.072002' 05085 // docTest_item['expect-value'] = '0x0c0001' 05086 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 3.072V + 0.5LSB 05087 if (SelfTestGroupEnable & 0x0040) { 05088 // call-function 05089 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05090 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)3.072002), (uint32_t)0x0c0001); // 3.072V + 0.5LSB 05091 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)3.072002, /* expect: */ (uint32_t)0x0c0001); // 3.072V + 0.5LSB 05092 // halt-on-first-failure logic 05093 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05094 } // if (SelfTestGroupEnable & 0x0040) 05095 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05096 05097 // @test group DACCodeOfVoltage DACCodeOfVoltage(3.072004) expect 0x0c0001 // 3.072V + 1.0LSB 05098 // docTest_item['actionType'] = 'call-function' 05099 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05100 // docTest_item['action'] = 'DACCodeOfVoltage(3.072004) expect 0x0c0001' 05101 // docTest_item['remarks'] = '3.072V + 1.0LSB' 05102 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05103 // docTest_item['arglist'] = '3.072004' 05104 // docTest_item['expect-value'] = '0x0c0001' 05105 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 3.072V + 1.0LSB 05106 if (SelfTestGroupEnable & 0x0040) { 05107 // call-function 05108 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05109 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)3.072004), (uint32_t)0x0c0001); // 3.072V + 1.0LSB 05110 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)3.072004, /* expect: */ (uint32_t)0x0c0001); // 3.072V + 1.0LSB 05111 // halt-on-first-failure logic 05112 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05113 } // if (SelfTestGroupEnable & 0x0040) 05114 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05115 05116 // @test group DACCodeOfVoltage DACCodeOfVoltage(3.072006) expect 0x0c0002 // 3.072V + 1.5LSB 05117 // docTest_item['actionType'] = 'call-function' 05118 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05119 // docTest_item['action'] = 'DACCodeOfVoltage(3.072006) expect 0x0c0002' 05120 // docTest_item['remarks'] = '3.072V + 1.5LSB' 05121 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05122 // docTest_item['arglist'] = '3.072006' 05123 // docTest_item['expect-value'] = '0x0c0002' 05124 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 3.072V + 1.5LSB 05125 if (SelfTestGroupEnable & 0x0040) { 05126 // call-function 05127 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05128 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)3.072006), (uint32_t)0x0c0002); // 3.072V + 1.5LSB 05129 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)3.072006, /* expect: */ (uint32_t)0x0c0002); // 3.072V + 1.5LSB 05130 // halt-on-first-failure logic 05131 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05132 } // if (SelfTestGroupEnable & 0x0040) 05133 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05134 05135 // @test group DACCodeOfVoltage DACCodeOfVoltage(3.072008) expect 0x0c0002 // 3.072V + 2.0LSB 05136 // docTest_item['actionType'] = 'call-function' 05137 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05138 // docTest_item['action'] = 'DACCodeOfVoltage(3.072008) expect 0x0c0002' 05139 // docTest_item['remarks'] = '3.072V + 2.0LSB' 05140 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05141 // docTest_item['arglist'] = '3.072008' 05142 // docTest_item['expect-value'] = '0x0c0002' 05143 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 3.072V + 2.0LSB 05144 if (SelfTestGroupEnable & 0x0040) { 05145 // call-function 05146 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05147 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)3.072008), (uint32_t)0x0c0002); // 3.072V + 2.0LSB 05148 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)3.072008, /* expect: */ (uint32_t)0x0c0002); // 3.072V + 2.0LSB 05149 // halt-on-first-failure logic 05150 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05151 } // if (SelfTestGroupEnable & 0x0040) 05152 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05153 05154 // @test group DACCodeOfVoltage DACCodeOfVoltage(3.072010) expect 0x0c0003 // 3.072V + 2.5LSB 05155 // docTest_item['actionType'] = 'call-function' 05156 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05157 // docTest_item['action'] = 'DACCodeOfVoltage(3.072010) expect 0x0c0003' 05158 // docTest_item['remarks'] = '3.072V + 2.5LSB' 05159 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05160 // docTest_item['arglist'] = '3.072010' 05161 // docTest_item['expect-value'] = '0x0c0003' 05162 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 3.072V + 2.5LSB 05163 if (SelfTestGroupEnable & 0x0040) { 05164 // call-function 05165 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05166 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)3.072010), (uint32_t)0x0c0003); // 3.072V + 2.5LSB 05167 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)3.072010, /* expect: */ (uint32_t)0x0c0003); // 3.072V + 2.5LSB 05168 // halt-on-first-failure logic 05169 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05170 } // if (SelfTestGroupEnable & 0x0040) 05171 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05172 05173 // @test group DACCodeOfVoltage DACCodeOfVoltage(3.072012) expect 0x0c0003 // 3.072V + 3.0LSB 05174 // docTest_item['actionType'] = 'call-function' 05175 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05176 // docTest_item['action'] = 'DACCodeOfVoltage(3.072012) expect 0x0c0003' 05177 // docTest_item['remarks'] = '3.072V + 3.0LSB' 05178 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05179 // docTest_item['arglist'] = '3.072012' 05180 // docTest_item['expect-value'] = '0x0c0003' 05181 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 3.072V + 3.0LSB 05182 if (SelfTestGroupEnable & 0x0040) { 05183 // call-function 05184 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05185 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)3.072012), (uint32_t)0x0c0003); // 3.072V + 3.0LSB 05186 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)3.072012, /* expect: */ (uint32_t)0x0c0003); // 3.072V + 3.0LSB 05187 // halt-on-first-failure logic 05188 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05189 } // if (SelfTestGroupEnable & 0x0040) 05190 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05191 05192 // @test group DACCodeOfVoltage tinyTester.print("test_lsb_sweep V = 2.048000V LSBradius = 3LSB") 05193 // docTest_item['actionType'] = 'print-string' 05194 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05195 // docTest_item['action'] = 'tinyTester.print("test_lsb_sweep V = 2.048000V LSBradius = 3LSB")' 05196 // docTest_item['arglist'] = 'test_lsb_sweep V = 2.048000V LSBradius = 3LSB' 05197 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage None 05198 if (SelfTestGroupEnable & 0x0040) { 05199 // print-string 05200 // tinyTesterFuncName = "tinyTester.print" 05201 // tinyTesterPrintStringLiteral = "test_lsb_sweep V = 2.048000V LSBradius = 3LSB" 05202 tinyTester.print("test_lsb_sweep V = 2.048000V LSBradius = 3LSB"); 05203 // halt-on-first-failure logic 05204 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05205 } // if (SelfTestGroupEnable & 0x0040) 05206 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05207 05208 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.047988) expect 0x07fffd // 2.048V + -3.0LSB 05209 // docTest_item['actionType'] = 'call-function' 05210 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05211 // docTest_item['action'] = 'DACCodeOfVoltage(2.047988) expect 0x07fffd' 05212 // docTest_item['remarks'] = '2.048V + -3.0LSB' 05213 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05214 // docTest_item['arglist'] = '2.047988' 05215 // docTest_item['expect-value'] = '0x07fffd' 05216 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V + -3.0LSB 05217 if (SelfTestGroupEnable & 0x0040) { 05218 // call-function 05219 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05220 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.047988), (uint32_t)0x07fffd); // 2.048V + -3.0LSB 05221 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.047988, /* expect: */ (uint32_t)0x07fffd); // 2.048V + -3.0LSB 05222 // halt-on-first-failure logic 05223 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05224 } // if (SelfTestGroupEnable & 0x0040) 05225 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05226 05227 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.047990) expect 0x07fffd // 2.048V + -2.5LSB 05228 // docTest_item['actionType'] = 'call-function' 05229 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05230 // docTest_item['action'] = 'DACCodeOfVoltage(2.047990) expect 0x07fffd' 05231 // docTest_item['remarks'] = '2.048V + -2.5LSB' 05232 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05233 // docTest_item['arglist'] = '2.047990' 05234 // docTest_item['expect-value'] = '0x07fffd' 05235 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V + -2.5LSB 05236 if (SelfTestGroupEnable & 0x0040) { 05237 // call-function 05238 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05239 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.047990), (uint32_t)0x07fffd); // 2.048V + -2.5LSB 05240 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.047990, /* expect: */ (uint32_t)0x07fffd); // 2.048V + -2.5LSB 05241 // halt-on-first-failure logic 05242 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05243 } // if (SelfTestGroupEnable & 0x0040) 05244 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05245 05246 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.047992) expect 0x07fffe // 2.048V + -2.0LSB 05247 // docTest_item['actionType'] = 'call-function' 05248 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05249 // docTest_item['action'] = 'DACCodeOfVoltage(2.047992) expect 0x07fffe' 05250 // docTest_item['remarks'] = '2.048V + -2.0LSB' 05251 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05252 // docTest_item['arglist'] = '2.047992' 05253 // docTest_item['expect-value'] = '0x07fffe' 05254 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V + -2.0LSB 05255 if (SelfTestGroupEnable & 0x0040) { 05256 // call-function 05257 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05258 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.047992), (uint32_t)0x07fffe); // 2.048V + -2.0LSB 05259 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.047992, /* expect: */ (uint32_t)0x07fffe); // 2.048V + -2.0LSB 05260 // halt-on-first-failure logic 05261 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05262 } // if (SelfTestGroupEnable & 0x0040) 05263 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05264 05265 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.047994) expect 0x07fffe // 2.048V + -1.5LSB 0x07fffe not 0x07ffff 05266 // docTest_item['actionType'] = 'call-function' 05267 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05268 // docTest_item['action'] = 'DACCodeOfVoltage(2.047994) expect 0x07fffe' 05269 // docTest_item['remarks'] = '2.048V + -1.5LSB 0x07fffe not 0x07ffff' 05270 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05271 // docTest_item['arglist'] = '2.047994' 05272 // docTest_item['expect-value'] = '0x07fffe' 05273 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V + -1.5LSB 0x07fffe not 0x07ffff 05274 if (SelfTestGroupEnable & 0x0040) { 05275 // call-function 05276 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05277 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.047994), (uint32_t)0x07fffe); // 2.048V + -1.5LSB 0x07fffe not 0x07ffff 05278 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.047994, /* expect: */ (uint32_t)0x07fffe); // 2.048V + -1.5LSB 0x07fffe not 0x07ffff 05279 // halt-on-first-failure logic 05280 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05281 } // if (SelfTestGroupEnable & 0x0040) 05282 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05283 05284 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.047996) expect 0x07ffff // 2.048V + -1.0LSB 05285 // docTest_item['actionType'] = 'call-function' 05286 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05287 // docTest_item['action'] = 'DACCodeOfVoltage(2.047996) expect 0x07ffff' 05288 // docTest_item['remarks'] = '2.048V + -1.0LSB' 05289 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05290 // docTest_item['arglist'] = '2.047996' 05291 // docTest_item['expect-value'] = '0x07ffff' 05292 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V + -1.0LSB 05293 if (SelfTestGroupEnable & 0x0040) { 05294 // call-function 05295 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05296 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.047996), (uint32_t)0x07ffff); // 2.048V + -1.0LSB 05297 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.047996, /* expect: */ (uint32_t)0x07ffff); // 2.048V + -1.0LSB 05298 // halt-on-first-failure logic 05299 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05300 } // if (SelfTestGroupEnable & 0x0040) 05301 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05302 05303 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.047998) expect 0x07ffff // 2.048V + -0.5LSB 0x07ffff not 0x080000 05304 // docTest_item['actionType'] = 'call-function' 05305 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05306 // docTest_item['action'] = 'DACCodeOfVoltage(2.047998) expect 0x07ffff' 05307 // docTest_item['remarks'] = '2.048V + -0.5LSB 0x07ffff not 0x080000' 05308 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05309 // docTest_item['arglist'] = '2.047998' 05310 // docTest_item['expect-value'] = '0x07ffff' 05311 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V + -0.5LSB 0x07ffff not 0x080000 05312 if (SelfTestGroupEnable & 0x0040) { 05313 // call-function 05314 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05315 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.047998), (uint32_t)0x07ffff); // 2.048V + -0.5LSB 0x07ffff not 0x080000 05316 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.047998, /* expect: */ (uint32_t)0x07ffff); // 2.048V + -0.5LSB 0x07ffff not 0x080000 05317 // halt-on-first-failure logic 05318 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05319 } // if (SelfTestGroupEnable & 0x0040) 05320 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05321 05322 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.048000) expect 0x080000 // 2.048V + 0.0LSB 05323 // docTest_item['actionType'] = 'call-function' 05324 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05325 // docTest_item['action'] = 'DACCodeOfVoltage(2.048000) expect 0x080000' 05326 // docTest_item['remarks'] = '2.048V + 0.0LSB' 05327 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05328 // docTest_item['arglist'] = '2.048000' 05329 // docTest_item['expect-value'] = '0x080000' 05330 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V + 0.0LSB 05331 if (SelfTestGroupEnable & 0x0040) { 05332 // call-function 05333 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05334 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.048000), (uint32_t)0x080000); // 2.048V + 0.0LSB 05335 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.048000, /* expect: */ (uint32_t)0x080000); // 2.048V + 0.0LSB 05336 // halt-on-first-failure logic 05337 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05338 } // if (SelfTestGroupEnable & 0x0040) 05339 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05340 05341 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.048002) expect 0x080001 // 2.048V + 0.5LSB 05342 // docTest_item['actionType'] = 'call-function' 05343 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05344 // docTest_item['action'] = 'DACCodeOfVoltage(2.048002) expect 0x080001' 05345 // docTest_item['remarks'] = '2.048V + 0.5LSB' 05346 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05347 // docTest_item['arglist'] = '2.048002' 05348 // docTest_item['expect-value'] = '0x080001' 05349 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V + 0.5LSB 05350 if (SelfTestGroupEnable & 0x0040) { 05351 // call-function 05352 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05353 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.048002), (uint32_t)0x080001); // 2.048V + 0.5LSB 05354 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.048002, /* expect: */ (uint32_t)0x080001); // 2.048V + 0.5LSB 05355 // halt-on-first-failure logic 05356 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05357 } // if (SelfTestGroupEnable & 0x0040) 05358 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05359 05360 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.048004) expect 0x080001 // 2.048V + 1.0LSB 05361 // docTest_item['actionType'] = 'call-function' 05362 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05363 // docTest_item['action'] = 'DACCodeOfVoltage(2.048004) expect 0x080001' 05364 // docTest_item['remarks'] = '2.048V + 1.0LSB' 05365 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05366 // docTest_item['arglist'] = '2.048004' 05367 // docTest_item['expect-value'] = '0x080001' 05368 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V + 1.0LSB 05369 if (SelfTestGroupEnable & 0x0040) { 05370 // call-function 05371 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05372 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.048004), (uint32_t)0x080001); // 2.048V + 1.0LSB 05373 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.048004, /* expect: */ (uint32_t)0x080001); // 2.048V + 1.0LSB 05374 // halt-on-first-failure logic 05375 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05376 } // if (SelfTestGroupEnable & 0x0040) 05377 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05378 05379 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.048006) expect 0x080002 // 2.048V + 1.5LSB 05380 // docTest_item['actionType'] = 'call-function' 05381 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05382 // docTest_item['action'] = 'DACCodeOfVoltage(2.048006) expect 0x080002' 05383 // docTest_item['remarks'] = '2.048V + 1.5LSB' 05384 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05385 // docTest_item['arglist'] = '2.048006' 05386 // docTest_item['expect-value'] = '0x080002' 05387 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V + 1.5LSB 05388 if (SelfTestGroupEnable & 0x0040) { 05389 // call-function 05390 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05391 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.048006), (uint32_t)0x080002); // 2.048V + 1.5LSB 05392 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.048006, /* expect: */ (uint32_t)0x080002); // 2.048V + 1.5LSB 05393 // halt-on-first-failure logic 05394 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05395 } // if (SelfTestGroupEnable & 0x0040) 05396 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05397 05398 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.048008) expect 0x080002 // 2.048V + 2.0LSB 05399 // docTest_item['actionType'] = 'call-function' 05400 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05401 // docTest_item['action'] = 'DACCodeOfVoltage(2.048008) expect 0x080002' 05402 // docTest_item['remarks'] = '2.048V + 2.0LSB' 05403 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05404 // docTest_item['arglist'] = '2.048008' 05405 // docTest_item['expect-value'] = '0x080002' 05406 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V + 2.0LSB 05407 if (SelfTestGroupEnable & 0x0040) { 05408 // call-function 05409 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05410 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.048008), (uint32_t)0x080002); // 2.048V + 2.0LSB 05411 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.048008, /* expect: */ (uint32_t)0x080002); // 2.048V + 2.0LSB 05412 // halt-on-first-failure logic 05413 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05414 } // if (SelfTestGroupEnable & 0x0040) 05415 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05416 05417 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.048010) expect 0x080003 // 2.048V + 2.5LSB 05418 // docTest_item['actionType'] = 'call-function' 05419 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05420 // docTest_item['action'] = 'DACCodeOfVoltage(2.048010) expect 0x080003' 05421 // docTest_item['remarks'] = '2.048V + 2.5LSB' 05422 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05423 // docTest_item['arglist'] = '2.048010' 05424 // docTest_item['expect-value'] = '0x080003' 05425 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V + 2.5LSB 05426 if (SelfTestGroupEnable & 0x0040) { 05427 // call-function 05428 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05429 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.048010), (uint32_t)0x080003); // 2.048V + 2.5LSB 05430 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.048010, /* expect: */ (uint32_t)0x080003); // 2.048V + 2.5LSB 05431 // halt-on-first-failure logic 05432 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05433 } // if (SelfTestGroupEnable & 0x0040) 05434 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05435 05436 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.048012) expect 0x080003 // 2.048V + 3.0LSB 05437 // docTest_item['actionType'] = 'call-function' 05438 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05439 // docTest_item['action'] = 'DACCodeOfVoltage(2.048012) expect 0x080003' 05440 // docTest_item['remarks'] = '2.048V + 3.0LSB' 05441 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05442 // docTest_item['arglist'] = '2.048012' 05443 // docTest_item['expect-value'] = '0x080003' 05444 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 2.048V + 3.0LSB 05445 if (SelfTestGroupEnable & 0x0040) { 05446 // call-function 05447 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05448 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)2.048012), (uint32_t)0x080003); // 2.048V + 3.0LSB 05449 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)2.048012, /* expect: */ (uint32_t)0x080003); // 2.048V + 3.0LSB 05450 // halt-on-first-failure logic 05451 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05452 } // if (SelfTestGroupEnable & 0x0040) 05453 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05454 05455 // @test group DACCodeOfVoltage tinyTester.print("test_lsb_sweep V = 1.024000V LSBradius = 3LSB") 05456 // docTest_item['actionType'] = 'print-string' 05457 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05458 // docTest_item['action'] = 'tinyTester.print("test_lsb_sweep V = 1.024000V LSBradius = 3LSB")' 05459 // docTest_item['arglist'] = 'test_lsb_sweep V = 1.024000V LSBradius = 3LSB' 05460 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage None 05461 if (SelfTestGroupEnable & 0x0040) { 05462 // print-string 05463 // tinyTesterFuncName = "tinyTester.print" 05464 // tinyTesterPrintStringLiteral = "test_lsb_sweep V = 1.024000V LSBradius = 3LSB" 05465 tinyTester.print("test_lsb_sweep V = 1.024000V LSBradius = 3LSB"); 05466 // halt-on-first-failure logic 05467 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05468 } // if (SelfTestGroupEnable & 0x0040) 05469 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05470 05471 // @test group DACCodeOfVoltage DACCodeOfVoltage(1.023988) expect 0x03fffd // 1.024V + -3.0LSB 05472 // docTest_item['actionType'] = 'call-function' 05473 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05474 // docTest_item['action'] = 'DACCodeOfVoltage(1.023988) expect 0x03fffd' 05475 // docTest_item['remarks'] = '1.024V + -3.0LSB' 05476 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05477 // docTest_item['arglist'] = '1.023988' 05478 // docTest_item['expect-value'] = '0x03fffd' 05479 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 1.024V + -3.0LSB 05480 if (SelfTestGroupEnable & 0x0040) { 05481 // call-function 05482 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05483 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)1.023988), (uint32_t)0x03fffd); // 1.024V + -3.0LSB 05484 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)1.023988, /* expect: */ (uint32_t)0x03fffd); // 1.024V + -3.0LSB 05485 // halt-on-first-failure logic 05486 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05487 } // if (SelfTestGroupEnable & 0x0040) 05488 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05489 05490 // @test group DACCodeOfVoltage DACCodeOfVoltage(1.023990) expect 0x03fffd // 1.024V + -2.5LSB 0x03fffd not 0x03fffe 05491 // docTest_item['actionType'] = 'call-function' 05492 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05493 // docTest_item['action'] = 'DACCodeOfVoltage(1.023990) expect 0x03fffd' 05494 // docTest_item['remarks'] = '1.024V + -2.5LSB 0x03fffd not 0x03fffe' 05495 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05496 // docTest_item['arglist'] = '1.023990' 05497 // docTest_item['expect-value'] = '0x03fffd' 05498 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 1.024V + -2.5LSB 0x03fffd not 0x03fffe 05499 if (SelfTestGroupEnable & 0x0040) { 05500 // call-function 05501 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05502 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)1.023990), (uint32_t)0x03fffd); // 1.024V + -2.5LSB 0x03fffd not 0x03fffe 05503 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)1.023990, /* expect: */ (uint32_t)0x03fffd); // 1.024V + -2.5LSB 0x03fffd not 0x03fffe 05504 // halt-on-first-failure logic 05505 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05506 } // if (SelfTestGroupEnable & 0x0040) 05507 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05508 05509 // @test group DACCodeOfVoltage DACCodeOfVoltage(1.023992) expect 0x03fffe // 1.024V + -2.0LSB 05510 // docTest_item['actionType'] = 'call-function' 05511 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05512 // docTest_item['action'] = 'DACCodeOfVoltage(1.023992) expect 0x03fffe' 05513 // docTest_item['remarks'] = '1.024V + -2.0LSB' 05514 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05515 // docTest_item['arglist'] = '1.023992' 05516 // docTest_item['expect-value'] = '0x03fffe' 05517 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 1.024V + -2.0LSB 05518 if (SelfTestGroupEnable & 0x0040) { 05519 // call-function 05520 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05521 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)1.023992), (uint32_t)0x03fffe); // 1.024V + -2.0LSB 05522 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)1.023992, /* expect: */ (uint32_t)0x03fffe); // 1.024V + -2.0LSB 05523 // halt-on-first-failure logic 05524 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05525 } // if (SelfTestGroupEnable & 0x0040) 05526 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05527 05528 // @test group DACCodeOfVoltage DACCodeOfVoltage(1.023994) expect 0x03fffe // 1.024V + -1.5LSB 05529 // docTest_item['actionType'] = 'call-function' 05530 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05531 // docTest_item['action'] = 'DACCodeOfVoltage(1.023994) expect 0x03fffe' 05532 // docTest_item['remarks'] = '1.024V + -1.5LSB' 05533 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05534 // docTest_item['arglist'] = '1.023994' 05535 // docTest_item['expect-value'] = '0x03fffe' 05536 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 1.024V + -1.5LSB 05537 if (SelfTestGroupEnable & 0x0040) { 05538 // call-function 05539 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05540 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)1.023994), (uint32_t)0x03fffe); // 1.024V + -1.5LSB 05541 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)1.023994, /* expect: */ (uint32_t)0x03fffe); // 1.024V + -1.5LSB 05542 // halt-on-first-failure logic 05543 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05544 } // if (SelfTestGroupEnable & 0x0040) 05545 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05546 05547 // @test group DACCodeOfVoltage DACCodeOfVoltage(1.023996) expect 0x03ffff // 1.024V + -1.0LSB 05548 // docTest_item['actionType'] = 'call-function' 05549 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05550 // docTest_item['action'] = 'DACCodeOfVoltage(1.023996) expect 0x03ffff' 05551 // docTest_item['remarks'] = '1.024V + -1.0LSB' 05552 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05553 // docTest_item['arglist'] = '1.023996' 05554 // docTest_item['expect-value'] = '0x03ffff' 05555 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 1.024V + -1.0LSB 05556 if (SelfTestGroupEnable & 0x0040) { 05557 // call-function 05558 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05559 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)1.023996), (uint32_t)0x03ffff); // 1.024V + -1.0LSB 05560 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)1.023996, /* expect: */ (uint32_t)0x03ffff); // 1.024V + -1.0LSB 05561 // halt-on-first-failure logic 05562 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05563 } // if (SelfTestGroupEnable & 0x0040) 05564 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05565 05566 // @test group DACCodeOfVoltage DACCodeOfVoltage(1.023998) expect 0x03ffff // 1.024V + -0.5LSB 0x03ffff not 0x040000 05567 // docTest_item['actionType'] = 'call-function' 05568 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05569 // docTest_item['action'] = 'DACCodeOfVoltage(1.023998) expect 0x03ffff' 05570 // docTest_item['remarks'] = '1.024V + -0.5LSB 0x03ffff not 0x040000' 05571 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05572 // docTest_item['arglist'] = '1.023998' 05573 // docTest_item['expect-value'] = '0x03ffff' 05574 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 1.024V + -0.5LSB 0x03ffff not 0x040000 05575 if (SelfTestGroupEnable & 0x0040) { 05576 // call-function 05577 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05578 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)1.023998), (uint32_t)0x03ffff); // 1.024V + -0.5LSB 0x03ffff not 0x040000 05579 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)1.023998, /* expect: */ (uint32_t)0x03ffff); // 1.024V + -0.5LSB 0x03ffff not 0x040000 05580 // halt-on-first-failure logic 05581 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05582 } // if (SelfTestGroupEnable & 0x0040) 05583 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05584 05585 // @test group DACCodeOfVoltage DACCodeOfVoltage(1.024000) expect 0x040000 // 1.024V + 0.0LSB 05586 // docTest_item['actionType'] = 'call-function' 05587 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05588 // docTest_item['action'] = 'DACCodeOfVoltage(1.024000) expect 0x040000' 05589 // docTest_item['remarks'] = '1.024V + 0.0LSB' 05590 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05591 // docTest_item['arglist'] = '1.024000' 05592 // docTest_item['expect-value'] = '0x040000' 05593 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 1.024V + 0.0LSB 05594 if (SelfTestGroupEnable & 0x0040) { 05595 // call-function 05596 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05597 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)1.024000), (uint32_t)0x040000); // 1.024V + 0.0LSB 05598 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)1.024000, /* expect: */ (uint32_t)0x040000); // 1.024V + 0.0LSB 05599 // halt-on-first-failure logic 05600 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05601 } // if (SelfTestGroupEnable & 0x0040) 05602 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05603 05604 // @test group DACCodeOfVoltage DACCodeOfVoltage(1.024002) expect 0x040001 // 1.024V + 0.5LSB 05605 // docTest_item['actionType'] = 'call-function' 05606 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05607 // docTest_item['action'] = 'DACCodeOfVoltage(1.024002) expect 0x040001' 05608 // docTest_item['remarks'] = '1.024V + 0.5LSB' 05609 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05610 // docTest_item['arglist'] = '1.024002' 05611 // docTest_item['expect-value'] = '0x040001' 05612 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 1.024V + 0.5LSB 05613 if (SelfTestGroupEnable & 0x0040) { 05614 // call-function 05615 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05616 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)1.024002), (uint32_t)0x040001); // 1.024V + 0.5LSB 05617 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)1.024002, /* expect: */ (uint32_t)0x040001); // 1.024V + 0.5LSB 05618 // halt-on-first-failure logic 05619 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05620 } // if (SelfTestGroupEnable & 0x0040) 05621 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05622 05623 // @test group DACCodeOfVoltage DACCodeOfVoltage(1.024004) expect 0x040001 // 1.024V + 1.0LSB 05624 // docTest_item['actionType'] = 'call-function' 05625 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05626 // docTest_item['action'] = 'DACCodeOfVoltage(1.024004) expect 0x040001' 05627 // docTest_item['remarks'] = '1.024V + 1.0LSB' 05628 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05629 // docTest_item['arglist'] = '1.024004' 05630 // docTest_item['expect-value'] = '0x040001' 05631 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 1.024V + 1.0LSB 05632 if (SelfTestGroupEnable & 0x0040) { 05633 // call-function 05634 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05635 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)1.024004), (uint32_t)0x040001); // 1.024V + 1.0LSB 05636 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)1.024004, /* expect: */ (uint32_t)0x040001); // 1.024V + 1.0LSB 05637 // halt-on-first-failure logic 05638 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05639 } // if (SelfTestGroupEnable & 0x0040) 05640 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05641 05642 // @test group DACCodeOfVoltage DACCodeOfVoltage(1.024006) expect 0x040002 // 1.024V + 1.5LSB 05643 // docTest_item['actionType'] = 'call-function' 05644 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05645 // docTest_item['action'] = 'DACCodeOfVoltage(1.024006) expect 0x040002' 05646 // docTest_item['remarks'] = '1.024V + 1.5LSB' 05647 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05648 // docTest_item['arglist'] = '1.024006' 05649 // docTest_item['expect-value'] = '0x040002' 05650 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 1.024V + 1.5LSB 05651 if (SelfTestGroupEnable & 0x0040) { 05652 // call-function 05653 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05654 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)1.024006), (uint32_t)0x040002); // 1.024V + 1.5LSB 05655 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)1.024006, /* expect: */ (uint32_t)0x040002); // 1.024V + 1.5LSB 05656 // halt-on-first-failure logic 05657 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05658 } // if (SelfTestGroupEnable & 0x0040) 05659 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05660 05661 // @test group DACCodeOfVoltage DACCodeOfVoltage(1.024008) expect 0x040002 // 1.024V + 2.0LSB 05662 // docTest_item['actionType'] = 'call-function' 05663 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05664 // docTest_item['action'] = 'DACCodeOfVoltage(1.024008) expect 0x040002' 05665 // docTest_item['remarks'] = '1.024V + 2.0LSB' 05666 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05667 // docTest_item['arglist'] = '1.024008' 05668 // docTest_item['expect-value'] = '0x040002' 05669 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 1.024V + 2.0LSB 05670 if (SelfTestGroupEnable & 0x0040) { 05671 // call-function 05672 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05673 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)1.024008), (uint32_t)0x040002); // 1.024V + 2.0LSB 05674 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)1.024008, /* expect: */ (uint32_t)0x040002); // 1.024V + 2.0LSB 05675 // halt-on-first-failure logic 05676 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05677 } // if (SelfTestGroupEnable & 0x0040) 05678 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05679 05680 // @test group DACCodeOfVoltage DACCodeOfVoltage(1.024010) expect 0x040003 // 1.024V + 2.5LSB 05681 // docTest_item['actionType'] = 'call-function' 05682 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05683 // docTest_item['action'] = 'DACCodeOfVoltage(1.024010) expect 0x040003' 05684 // docTest_item['remarks'] = '1.024V + 2.5LSB' 05685 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05686 // docTest_item['arglist'] = '1.024010' 05687 // docTest_item['expect-value'] = '0x040003' 05688 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 1.024V + 2.5LSB 05689 if (SelfTestGroupEnable & 0x0040) { 05690 // call-function 05691 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05692 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)1.024010), (uint32_t)0x040003); // 1.024V + 2.5LSB 05693 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)1.024010, /* expect: */ (uint32_t)0x040003); // 1.024V + 2.5LSB 05694 // halt-on-first-failure logic 05695 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05696 } // if (SelfTestGroupEnable & 0x0040) 05697 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05698 05699 // @test group DACCodeOfVoltage DACCodeOfVoltage(1.024012) expect 0x040003 // 1.024V + 3.0LSB 05700 // docTest_item['actionType'] = 'call-function' 05701 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05702 // docTest_item['action'] = 'DACCodeOfVoltage(1.024012) expect 0x040003' 05703 // docTest_item['remarks'] = '1.024V + 3.0LSB' 05704 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05705 // docTest_item['arglist'] = '1.024012' 05706 // docTest_item['expect-value'] = '0x040003' 05707 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 1.024V + 3.0LSB 05708 if (SelfTestGroupEnable & 0x0040) { 05709 // call-function 05710 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05711 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)1.024012), (uint32_t)0x040003); // 1.024V + 3.0LSB 05712 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)1.024012, /* expect: */ (uint32_t)0x040003); // 1.024V + 3.0LSB 05713 // halt-on-first-failure logic 05714 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05715 } // if (SelfTestGroupEnable & 0x0040) 05716 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05717 05718 // @test group DACCodeOfVoltage tinyTester.print("test_lsb_sweep V = 0.000000V LSBradius = 3LSB") 05719 // docTest_item['actionType'] = 'print-string' 05720 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05721 // docTest_item['action'] = 'tinyTester.print("test_lsb_sweep V = 0.000000V LSBradius = 3LSB")' 05722 // docTest_item['arglist'] = 'test_lsb_sweep V = 0.000000V LSBradius = 3LSB' 05723 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage None 05724 if (SelfTestGroupEnable & 0x0040) { 05725 // print-string 05726 // tinyTesterFuncName = "tinyTester.print" 05727 // tinyTesterPrintStringLiteral = "test_lsb_sweep V = 0.000000V LSBradius = 3LSB" 05728 tinyTester.print("test_lsb_sweep V = 0.000000V LSBradius = 3LSB"); 05729 // halt-on-first-failure logic 05730 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05731 } // if (SelfTestGroupEnable & 0x0040) 05732 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05733 05734 // @test group DACCodeOfVoltage DACCodeOfVoltage(-0.000012) expect 0x000000 // 0.000V + -3.0LSB 05735 // docTest_item['actionType'] = 'call-function' 05736 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05737 // docTest_item['action'] = 'DACCodeOfVoltage(-0.000012) expect 0x000000' 05738 // docTest_item['remarks'] = '0.000V + -3.0LSB' 05739 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05740 // docTest_item['arglist'] = '-0.000012' 05741 // docTest_item['expect-value'] = '0x000000' 05742 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.000V + -3.0LSB 05743 if (SelfTestGroupEnable & 0x0040) { 05744 // call-function 05745 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05746 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)-0.000012), (uint32_t)0x000000); // 0.000V + -3.0LSB 05747 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)-0.000012, /* expect: */ (uint32_t)0x000000); // 0.000V + -3.0LSB 05748 // halt-on-first-failure logic 05749 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05750 } // if (SelfTestGroupEnable & 0x0040) 05751 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05752 05753 // @test group DACCodeOfVoltage DACCodeOfVoltage(-0.000010) expect 0x000000 // 0.000V + -2.5LSB 05754 // docTest_item['actionType'] = 'call-function' 05755 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05756 // docTest_item['action'] = 'DACCodeOfVoltage(-0.000010) expect 0x000000' 05757 // docTest_item['remarks'] = '0.000V + -2.5LSB' 05758 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05759 // docTest_item['arglist'] = '-0.000010' 05760 // docTest_item['expect-value'] = '0x000000' 05761 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.000V + -2.5LSB 05762 if (SelfTestGroupEnable & 0x0040) { 05763 // call-function 05764 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05765 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)-0.000010), (uint32_t)0x000000); // 0.000V + -2.5LSB 05766 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)-0.000010, /* expect: */ (uint32_t)0x000000); // 0.000V + -2.5LSB 05767 // halt-on-first-failure logic 05768 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05769 } // if (SelfTestGroupEnable & 0x0040) 05770 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05771 05772 // @test group DACCodeOfVoltage DACCodeOfVoltage(-0.000008) expect 0x000000 // 0.000V + -2.0LSB 05773 // docTest_item['actionType'] = 'call-function' 05774 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05775 // docTest_item['action'] = 'DACCodeOfVoltage(-0.000008) expect 0x000000' 05776 // docTest_item['remarks'] = '0.000V + -2.0LSB' 05777 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05778 // docTest_item['arglist'] = '-0.000008' 05779 // docTest_item['expect-value'] = '0x000000' 05780 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.000V + -2.0LSB 05781 if (SelfTestGroupEnable & 0x0040) { 05782 // call-function 05783 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05784 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)-0.000008), (uint32_t)0x000000); // 0.000V + -2.0LSB 05785 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)-0.000008, /* expect: */ (uint32_t)0x000000); // 0.000V + -2.0LSB 05786 // halt-on-first-failure logic 05787 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05788 } // if (SelfTestGroupEnable & 0x0040) 05789 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05790 05791 // @test group DACCodeOfVoltage DACCodeOfVoltage(-0.000006) expect 0x000000 // 0.000V + -1.5LSB 05792 // docTest_item['actionType'] = 'call-function' 05793 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05794 // docTest_item['action'] = 'DACCodeOfVoltage(-0.000006) expect 0x000000' 05795 // docTest_item['remarks'] = '0.000V + -1.5LSB' 05796 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05797 // docTest_item['arglist'] = '-0.000006' 05798 // docTest_item['expect-value'] = '0x000000' 05799 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.000V + -1.5LSB 05800 if (SelfTestGroupEnable & 0x0040) { 05801 // call-function 05802 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05803 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)-0.000006), (uint32_t)0x000000); // 0.000V + -1.5LSB 05804 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)-0.000006, /* expect: */ (uint32_t)0x000000); // 0.000V + -1.5LSB 05805 // halt-on-first-failure logic 05806 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05807 } // if (SelfTestGroupEnable & 0x0040) 05808 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05809 05810 // @test group DACCodeOfVoltage DACCodeOfVoltage(-0.000004) expect 0x000000 // 0.000V + -1.0LSB 05811 // docTest_item['actionType'] = 'call-function' 05812 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05813 // docTest_item['action'] = 'DACCodeOfVoltage(-0.000004) expect 0x000000' 05814 // docTest_item['remarks'] = '0.000V + -1.0LSB' 05815 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05816 // docTest_item['arglist'] = '-0.000004' 05817 // docTest_item['expect-value'] = '0x000000' 05818 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.000V + -1.0LSB 05819 if (SelfTestGroupEnable & 0x0040) { 05820 // call-function 05821 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05822 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)-0.000004), (uint32_t)0x000000); // 0.000V + -1.0LSB 05823 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)-0.000004, /* expect: */ (uint32_t)0x000000); // 0.000V + -1.0LSB 05824 // halt-on-first-failure logic 05825 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05826 } // if (SelfTestGroupEnable & 0x0040) 05827 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05828 05829 // @test group DACCodeOfVoltage DACCodeOfVoltage(-0.000002) expect 0x000000 // 0.000V + -0.5LSB 05830 // docTest_item['actionType'] = 'call-function' 05831 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05832 // docTest_item['action'] = 'DACCodeOfVoltage(-0.000002) expect 0x000000' 05833 // docTest_item['remarks'] = '0.000V + -0.5LSB' 05834 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05835 // docTest_item['arglist'] = '-0.000002' 05836 // docTest_item['expect-value'] = '0x000000' 05837 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.000V + -0.5LSB 05838 if (SelfTestGroupEnable & 0x0040) { 05839 // call-function 05840 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05841 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)-0.000002), (uint32_t)0x000000); // 0.000V + -0.5LSB 05842 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)-0.000002, /* expect: */ (uint32_t)0x000000); // 0.000V + -0.5LSB 05843 // halt-on-first-failure logic 05844 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05845 } // if (SelfTestGroupEnable & 0x0040) 05846 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05847 05848 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.000000) expect 0x000000 // 0.000V + 0.0LSB 05849 // docTest_item['actionType'] = 'call-function' 05850 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05851 // docTest_item['action'] = 'DACCodeOfVoltage(0.000000) expect 0x000000' 05852 // docTest_item['remarks'] = '0.000V + 0.0LSB' 05853 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05854 // docTest_item['arglist'] = '0.000000' 05855 // docTest_item['expect-value'] = '0x000000' 05856 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.000V + 0.0LSB 05857 if (SelfTestGroupEnable & 0x0040) { 05858 // call-function 05859 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05860 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.000000), (uint32_t)0x000000); // 0.000V + 0.0LSB 05861 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.000000, /* expect: */ (uint32_t)0x000000); // 0.000V + 0.0LSB 05862 // halt-on-first-failure logic 05863 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05864 } // if (SelfTestGroupEnable & 0x0040) 05865 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05866 05867 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.000002) expect 0x000001 // 0.000V + 0.5LSB 05868 // docTest_item['actionType'] = 'call-function' 05869 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05870 // docTest_item['action'] = 'DACCodeOfVoltage(0.000002) expect 0x000001' 05871 // docTest_item['remarks'] = '0.000V + 0.5LSB' 05872 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05873 // docTest_item['arglist'] = '0.000002' 05874 // docTest_item['expect-value'] = '0x000001' 05875 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.000V + 0.5LSB 05876 if (SelfTestGroupEnable & 0x0040) { 05877 // call-function 05878 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05879 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.000002), (uint32_t)0x000001); // 0.000V + 0.5LSB 05880 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.000002, /* expect: */ (uint32_t)0x000001); // 0.000V + 0.5LSB 05881 // halt-on-first-failure logic 05882 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05883 } // if (SelfTestGroupEnable & 0x0040) 05884 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05885 05886 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.000004) expect 0x000001 // 0.000V + 1.0LSB 05887 // docTest_item['actionType'] = 'call-function' 05888 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05889 // docTest_item['action'] = 'DACCodeOfVoltage(0.000004) expect 0x000001' 05890 // docTest_item['remarks'] = '0.000V + 1.0LSB' 05891 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05892 // docTest_item['arglist'] = '0.000004' 05893 // docTest_item['expect-value'] = '0x000001' 05894 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.000V + 1.0LSB 05895 if (SelfTestGroupEnable & 0x0040) { 05896 // call-function 05897 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05898 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.000004), (uint32_t)0x000001); // 0.000V + 1.0LSB 05899 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.000004, /* expect: */ (uint32_t)0x000001); // 0.000V + 1.0LSB 05900 // halt-on-first-failure logic 05901 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05902 } // if (SelfTestGroupEnable & 0x0040) 05903 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05904 05905 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.000006) expect 0x000002 // 0.000V + 1.5LSB 05906 // docTest_item['actionType'] = 'call-function' 05907 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05908 // docTest_item['action'] = 'DACCodeOfVoltage(0.000006) expect 0x000002' 05909 // docTest_item['remarks'] = '0.000V + 1.5LSB' 05910 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05911 // docTest_item['arglist'] = '0.000006' 05912 // docTest_item['expect-value'] = '0x000002' 05913 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.000V + 1.5LSB 05914 if (SelfTestGroupEnable & 0x0040) { 05915 // call-function 05916 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05917 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.000006), (uint32_t)0x000002); // 0.000V + 1.5LSB 05918 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.000006, /* expect: */ (uint32_t)0x000002); // 0.000V + 1.5LSB 05919 // halt-on-first-failure logic 05920 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05921 } // if (SelfTestGroupEnable & 0x0040) 05922 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05923 05924 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.000008) expect 0x000002 // 0.000V + 2.0LSB 05925 // docTest_item['actionType'] = 'call-function' 05926 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05927 // docTest_item['action'] = 'DACCodeOfVoltage(0.000008) expect 0x000002' 05928 // docTest_item['remarks'] = '0.000V + 2.0LSB' 05929 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05930 // docTest_item['arglist'] = '0.000008' 05931 // docTest_item['expect-value'] = '0x000002' 05932 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.000V + 2.0LSB 05933 if (SelfTestGroupEnable & 0x0040) { 05934 // call-function 05935 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05936 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.000008), (uint32_t)0x000002); // 0.000V + 2.0LSB 05937 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.000008, /* expect: */ (uint32_t)0x000002); // 0.000V + 2.0LSB 05938 // halt-on-first-failure logic 05939 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05940 } // if (SelfTestGroupEnable & 0x0040) 05941 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05942 05943 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.000010) expect 0x000003 // 0.000V + 2.5LSB 05944 // docTest_item['actionType'] = 'call-function' 05945 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05946 // docTest_item['action'] = 'DACCodeOfVoltage(0.000010) expect 0x000003' 05947 // docTest_item['remarks'] = '0.000V + 2.5LSB' 05948 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05949 // docTest_item['arglist'] = '0.000010' 05950 // docTest_item['expect-value'] = '0x000003' 05951 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.000V + 2.5LSB 05952 if (SelfTestGroupEnable & 0x0040) { 05953 // call-function 05954 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05955 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.000010), (uint32_t)0x000003); // 0.000V + 2.5LSB 05956 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.000010, /* expect: */ (uint32_t)0x000003); // 0.000V + 2.5LSB 05957 // halt-on-first-failure logic 05958 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05959 } // if (SelfTestGroupEnable & 0x0040) 05960 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05961 05962 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.000012) expect 0x000003 // 0.000V + 3.0LSB 05963 // docTest_item['actionType'] = 'call-function' 05964 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05965 // docTest_item['action'] = 'DACCodeOfVoltage(0.000012) expect 0x000003' 05966 // docTest_item['remarks'] = '0.000V + 3.0LSB' 05967 // docTest_item['funcName'] = 'DACCodeOfVoltage' 05968 // docTest_item['arglist'] = '0.000012' 05969 // docTest_item['expect-value'] = '0x000003' 05970 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 0.000V + 3.0LSB 05971 if (SelfTestGroupEnable & 0x0040) { 05972 // call-function 05973 // selfTestFunctionClosures['DACCodeOfVoltage']['returnType'] = 'uint32_t' 05974 // ASSERT_EQ(g_MAX5719_device.DACCodeOfVoltage((double)0.000012), (uint32_t)0x000003); // 0.000V + 3.0LSB 05975 tinyTester.FunctionCall_lu_f_Expect("MAX5719.DACCodeOfVoltage", fn_MAX5719_DACCodeOfVoltage, (double)0.000012, /* expect: */ (uint32_t)0x000003); // 0.000V + 3.0LSB 05976 // halt-on-first-failure logic 05977 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05978 } // if (SelfTestGroupEnable & 0x0040) 05979 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05980 05981 // @test group DACCodeOfVoltage tinyTester.blink_time_msec = 75 // default 75 resume hardware self test 05982 // docTest_item['actionType'] = 'assign-propname-value' 05983 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 05984 // docTest_item['action'] = 'tinyTester.blink_time_msec = 75' 05985 // docTest_item['remarks'] = 'default 75 resume hardware self test' 05986 // docTest_item['propName'] = 'tinyTester.blink_time_msec' 05987 // docTest_item['propValue'] = '75' 05988 #if MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage None default 75 resume hardware self test 05989 if (SelfTestGroupEnable & 0x0040) { 05990 // assign-propname-value 05991 // tinyTesterPropName = "tinyTester.blink_time_msec" 05992 // tinyTesterPropValue = "75" 05993 tinyTester.blink_time_msec = 75; 05994 // halt-on-first-failure logic 05995 if ((SelfTestGroupEnable & 0x0001) && (tinyTester.nFail != 0)) { goto exitTesting; } 05996 } // if (SelfTestGroupEnable & 0x0040) 05997 #endif // MAX5719_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 05998 05999 // 06000 #if INJECT_SELFTEST_FAIL 06001 // Test of the pass/fail report mechanism 06002 tinyTester.FAIL(); 06003 cmdLine.serial().print(F("injecting one false failure for test reporting")); 06004 #endif 06005 // 06006 // repeat-until-failure logic 06007 // if (cmdLine.serial().readable()) { goto exitTesting; } 06008 if (serial.readable()) { goto exitTesting; } 06009 if ((SelfTestGroupEnable & 0x0002) && (tinyTester.nFail == 0)) { goto repeatUntilFailure; } 06010 // 06011 // halt-on-first-failure logic 06012 exitTesting: 06013 // 06014 // Report number of pass and number of fail test results 06015 tinyTester.Report_Summary(); 06016 } 06017 06018 //-------------------------------------------------- 06019 // selfTestFunctionClosures[functionName]['functionName'] = 'Init' 06020 // selfTestFunctionClosures[functionName]['argListDeclaration'] = 'void' 06021 // selfTestFunctionClosures[functionName]['returnType'] = 'void' 06022 // selfTestFunctionClosures[functionName]['argNames'] = '' 06023 // CommandParamIn_declaration = 'void' 06024 // argNames_recast_implementation = '' 06025 //-------------------------------------------------- 06026 // selftest: define function under test 06027 // void MAX5719::Init(void) 06028 void fn_MAX5719_Init(void) 06029 { 06030 return g_MAX5719_device.Init(); 06031 } 06032 06033 //-------------------------------------------------- 06034 // selfTestFunctionClosures[functionName]['functionName'] = 'CODE_LOAD' 06035 // selfTestFunctionClosures[functionName]['argListDeclaration'] = 'uint32_t dacCodeLsbs' 06036 // selfTestFunctionClosures[functionName]['returnType'] = 'uint8_t' 06037 // selfTestFunctionClosures[functionName]['argNames'] = 'dacCodeLsbs' 06038 // CommandParamIn_declaration = 'uint32_t dacCodeLsbs' 06039 // argNames_recast_implementation = '(uint32_t)dacCodeLsbs' 06040 //-------------------------------------------------- 06041 // selftest: define function under test 06042 // uint8_t MAX5719::CODE_LOAD(uint32_t dacCodeLsbs) 06043 uint8_t fn_MAX5719_CODE_LOAD(uint32_t dacCodeLsbs) 06044 { 06045 return g_MAX5719_device.CODE_LOAD((uint32_t)dacCodeLsbs); 06046 } 06047 06048 //-------------------------------------------------- 06049 // selfTestFunctionClosures[functionName]['functionName'] = 'DACCodeOfVoltage' 06050 // selfTestFunctionClosures[functionName]['argListDeclaration'] = 'double voltageV' 06051 // selfTestFunctionClosures[functionName]['returnType'] = 'uint32_t' 06052 // selfTestFunctionClosures[functionName]['argNames'] = 'voltageV' 06053 // CommandParamIn_declaration = 'double voltageV' 06054 // argNames_recast_implementation = '(double)voltageV' 06055 //-------------------------------------------------- 06056 // selftest: define function under test 06057 // uint32_t MAX5719::DACCodeOfVoltage(double voltageV) 06058 uint32_t fn_MAX5719_DACCodeOfVoltage(double voltageV) 06059 { 06060 return g_MAX5719_device.DACCodeOfVoltage((double)voltageV); 06061 } 06062 06063 06064 //-------------------------------------------------- 06065 inline void print_command_prompt() 06066 { 06067 cmdLine_serial.serial().printf("\r\n> "); 06068 06069 } 06070 06071 06072 //-------------------------------------------------- 06073 void pinsMonitor_submenu_onEOLcommandParser(CmdLine& cmdLine) 06074 { 06075 // % diagnostic commands submenu 06076 // %Hpin -- digital output high 06077 // %Lpin -- digital output low 06078 // %?pin -- digital input 06079 // %A %Apin -- analog input 06080 // %Ppin df=xx -- pwm output 06081 // %Wpin -- measure high pulsewidth input in usec 06082 // %wpin -- measure low pulsewidth input in usec 06083 // %I... -- I2C diagnostics 06084 // %IP -- I2C probe 06085 // %IC scl=100khz ADDR=? -- I2C configure 06086 // %IW byte byte ... byte RD=? ADDR=0x -- write 06087 // %IR ADDR=? RD=? -- read 06088 // %I^ cmd=? -- i2c_smbus_read_word_data 06089 // %S... -- SPI diagnostics 06090 // %SC sclk=1Mhz -- SPI configure 06091 // %SW -- write (write and read) 06092 // %SR -- read (alias for %SW because SPI always write and read) 06093 // A-Z,a-z,0-9 reserved for application use 06094 // 06095 char strPinIndex[3]; 06096 strPinIndex[0] = cmdLine[2]; 06097 strPinIndex[1] = cmdLine[3]; 06098 strPinIndex[2] = '\0'; 06099 int pinIndex = strtoul(strPinIndex, NULL, 10); // strtol(str, NULL, 10): get decimal value 06100 //cmdLine.serial().printf(" pinIndex=%d ", pinIndex); 06101 // 06102 // get next character 06103 switch (cmdLine[1]) 06104 { 06105 #if HAS_digitalInOuts 06106 case 'H': case 'h': 06107 { 06108 // %Hpin -- digital output high 06109 #if ARDUINO_STYLE 06110 pinMode(pinIndex, OUTPUT); // digital pins 0, 1, 2, .. 13, analog input pins A0, A1, .. A5 06111 digitalWrite(pinIndex, HIGH); // digital pins 0, 1, 2, .. 13, analog input pins A0, A1, .. A5 06112 #else 06113 DigitalInOut& digitalInOutPin = find_digitalInOutPin(pinIndex); 06114 digitalInOutPin.output(); 06115 digitalInOutPin.write(1); 06116 #endif 06117 cmdLine.serial().printf(" digitalInOutPin %d Output High ", pinIndex); 06118 } 06119 break; 06120 case 'L': case 'l': 06121 { 06122 // %Lpin -- digital output low 06123 #if ARDUINO_STYLE 06124 pinMode(pinIndex, OUTPUT); // digital pins 0, 1, 2, .. 13, analog input pins A0, A1, .. A5 06125 digitalWrite(pinIndex, LOW); // digital pins 0, 1, 2, .. 13, analog input pins A0, A1, .. A5 06126 #else 06127 DigitalInOut& digitalInOutPin = find_digitalInOutPin(pinIndex); 06128 digitalInOutPin.output(); 06129 digitalInOutPin.write(0); 06130 #endif 06131 cmdLine.serial().printf(" digitalInOutPin %d Output Low ", pinIndex); 06132 } 06133 break; 06134 case '?': 06135 { 06136 // %?pin -- digital input 06137 #if ARDUINO_STYLE 06138 pinMode(pinIndex, INPUT); // digital pins 0, 1, 2, .. 13, analog input pins A0, A1, .. A5 06139 #else 06140 DigitalInOut& digitalInOutPin = find_digitalInOutPin(pinIndex); 06141 digitalInOutPin.input(); 06142 #endif 06143 serial.printf(" digitalInOutPin %d Input ", pinIndex); 06144 #if ARDUINO_STYLE 06145 int value = digitalRead(pinIndex); 06146 #else 06147 int value = digitalInOutPin.read(); 06148 #endif 06149 cmdLine.serial().printf("%d ", value); 06150 } 06151 break; 06152 #endif 06153 // 06154 #if HAS_analogIns 06155 case 'A': case 'a': 06156 { 06157 // %A %Apin -- analog input 06158 #if analogIn4_IS_HIGH_RANGE_OF_analogIn0 06159 // Platform board uses AIN4,AIN5,.. as high range of AIN0,AIN1,.. 06160 for (int pinIndex = 0; pinIndex < 2; pinIndex++) 06161 { 06162 int cPinIndex = '0' + pinIndex; 06163 AnalogIn& analogInPin = find_analogInPin(cPinIndex); 06164 float adc_full_scale_voltage = analogInPin_fullScaleVoltage[pinIndex]; 06165 float normValue_0_1 = analogInPin.read(); 06166 // 06167 int pinIndexH = pinIndex + 4; 06168 int cPinIndexH = '0' + pinIndexH; 06169 AnalogIn& analogInPinH = find_analogInPin(cPinIndexH); 06170 float adc_full_scale_voltageH = analogInPin_fullScaleVoltage[pinIndexH]; 06171 float normValueH_0_1 = analogInPinH.read(); 06172 // 06173 cmdLine.serial().printf("AIN%c = %7.3f%% = %1.3fV AIN%c = %7.3f%% = %1.3fV \r\n", 06174 cPinIndex, 06175 normValue_0_1 * 100.0, 06176 normValue_0_1 * adc_full_scale_voltage, 06177 cPinIndexH, 06178 normValueH_0_1 * 100.0, 06179 normValueH_0_1 * adc_full_scale_voltageH 06180 ); 06181 } 06182 for (int pinIndex = 2; pinIndex < 4; pinIndex++) 06183 { 06184 int cPinIndex = '0' + pinIndex; 06185 AnalogIn& analogInPin = find_analogInPin(cPinIndex); 06186 float adc_full_scale_voltage = analogInPin_fullScaleVoltage[pinIndex]; 06187 float normValue_0_1 = analogInPin.read(); 06188 // 06189 cmdLine.serial().printf("AIN%c = %7.3f%% = %1.3fV\r\n", 06190 cPinIndex, 06191 normValue_0_1 * 100.0, 06192 normValue_0_1 * adc_full_scale_voltage 06193 ); 06194 } 06195 #else // analogIn4_IS_HIGH_RANGE_OF_analogIn0 06196 // Platform board uses simple analog inputs 06197 // assume standard Arduino analog inputs A0-A5 06198 for (int pinIndex = 0; pinIndex < 6; pinIndex++) 06199 { 06200 int cPinIndex = '0' + pinIndex; 06201 AnalogIn& analogInPin = find_analogInPin(cPinIndex); 06202 float adc_full_scale_voltage = analogInPin_fullScaleVoltage[pinIndex]; 06203 float normValue_0_1 = analogInPin.read(); 06204 // 06205 cmdLine.serial().printf("AIN%c = %7.3f%% = %1.3fV\r\n", 06206 cPinIndex, 06207 normValue_0_1 * 100.0, 06208 normValue_0_1 * adc_full_scale_voltage 06209 ); 06210 } 06211 #endif // analogIn4_IS_HIGH_RANGE_OF_analogIn0 06212 } 06213 break; 06214 #endif 06215 // 06216 #if HAS_SPI2_MAX541 06217 case 'D': case 'd': 06218 { 06219 // %D -- DAC output MAX541 (SPI2) -- need cmdLine.parse_float(voltageV) 06220 // MAX541 max541(spi2_max541, spi2_max541_cs); 06221 float voltageV = max541.Get_Voltage(); 06222 // if (cmdLine[2] == '+') { 06223 // // %D+ 06224 // voltageV = voltageV * 1.25f; 06225 // if (voltageV >= max541.VRef) voltageV = max541.VRef; 06226 // SelfTest_MAX541_Voltage(cmdLine, max541, voltageV); 06227 // } 06228 // else if (cmdLine[2] == '-') { 06229 // // %D- 06230 // voltageV = voltageV * 0.75f; 06231 // if (voltageV < 0.1f) voltageV = 0.1f; 06232 // SelfTest_MAX541_Voltage(cmdLine, max541, voltageV); 06233 // } 06234 if (cmdLine.parse_float("V", voltageV)) 06235 { 06236 // %D V=1.234 -- set voltage 06237 max541.Set_Voltage(voltageV); 06238 } 06239 else if (cmdLine.parse_float("TEST", voltageV)) 06240 { 06241 // %D TEST=1.234 -- set voltage and compare with AIN0 06242 SelfTest_MAX541_Voltage(cmdLine, max541, voltageV); 06243 } 06244 else if (cmdLine.parse_float("CAL", voltageV)) 06245 { 06246 // %D CAL=1.234 -- calibrate VRef and compare with AIN0 06247 06248 max541.Set_Code(0x8000); // we don't know the fullscale voltage yet, so set code to midscale 06249 double max541_midscale_V = analogInPin_fullScaleVoltage[4] * analogIn4.read(); // TARGET_MAX32630 J1.5 AIN_4 = AIN0 / 5.0 fullscale is 6.0V 06250 const int average_count = 100; 06251 const double average_K = 0.25; 06252 for (int count = 0; count < average_count; count++) { 06253 double measurement_V = analogInPin_fullScaleVoltage[4] * analogIn4.read(); // TARGET_MAX32630 J1.5 AIN_4 = AIN0 / 5.0 fullscale is 6.0V 06254 max541_midscale_V = ((1 - average_K) * max541_midscale_V) + (average_K * measurement_V); 06255 } 06256 max541.VRef = 2.0 * max541_midscale_V; 06257 cmdLine.serial().printf( 06258 "\r\n MAX541 midscale = %1.3fV, so fullscale = %1.3fV", 06259 max541_midscale_V, max541.VRef); 06260 // Detect whether MAX541 is really connected to MAX32625MBED.AIN0/AIN4 06261 voltageV = 1.0f; 06262 SelfTest_MAX541_Voltage(cmdLine, max541, voltageV); 06263 } 06264 else { 06265 // %D -- print MAX541 DAC status 06266 cmdLine.serial().printf("MAX541 code=0x%4.4x = %1.3fV VRef=%1.3fV\r\n", 06267 max541.Get_Code(), max541.Get_Voltage(), max541.VRef); 06268 } 06269 } 06270 break; 06271 #endif 06272 06273 // 06274 #if HAS_I2C // SUPPORT_I2C 06275 case 'I': case 'i': 06276 // %I... -- I2C diagnostics 06277 // %IP -- I2C probe 06278 // %IC scl=100khz ADDR=? -- I2C configure 06279 // %IW byte byte ... byte RD=? ADDR=0x -- write 06280 // %IR ADDR=? RD=? -- read 06281 // %I^ cmd=? -- i2c_smbus_read_word_data 06282 // get next character 06283 // TODO: parse cmdLine arg (ADDR=\d+)? --> g_I2C_deviceAddress7 06284 cmdLine.parse_byte_hex("ADDR", g_I2C_deviceAddress7); 06285 // TODO: parse cmdLine arg (RD=\d)? --> g_I2C_read_count 06286 g_I2C_read_count = 0; // read count must be reset every command 06287 cmdLine.parse_byte_dec("RD", g_I2C_read_count); 06288 // TODO: parse cmdLine arg (CMD=\d)? --> g_I2C_command_regAddress 06289 cmdLine.parse_byte_hex("CMD", g_I2C_command_regAddress); 06290 switch (cmdLine[2]) 06291 { 06292 case 'P': case 'p': 06293 { 06294 // %IP -- I2C probe 06295 HuntAttachedI2CDevices(cmdLine, 0x03, 0x77); 06296 } 06297 break; 06298 case 'C': case 'c': 06299 { 06300 bool isUpdatedI2CConfig = false; 06301 // %IC scl=100khz ADDR=? -- I2C configure 06302 // parse cmdLine arg (SCL=\d+(kHZ|MHZ)?)? --> g_I2C_SCL_Hz 06303 if (cmdLine.parse_frequency_Hz("SCL", g_I2C_SCL_Hz)) 06304 { 06305 isUpdatedI2CConfig = true; 06306 // TODO1: validate g_I2C_SCL_Hz against system clock frequency F_CPU 06307 if (g_I2C_SCL_Hz > limit_max_I2C_SCL_Hz) 06308 { 06309 g_I2C_SCL_Hz = limit_max_I2C_SCL_Hz; 06310 } 06311 if (g_I2C_SCL_Hz < limit_min_I2C_SCL_Hz) 06312 { 06313 g_I2C_SCL_Hz = limit_min_I2C_SCL_Hz; 06314 } 06315 } 06316 if (isUpdatedI2CConfig) 06317 { 06318 // declare in narrower scope: MAX32625MBED I2C i2cMaster(...) 06319 I2C i2cMaster(I2C0_SDA, I2C0_SCL); // sda scl TARGET_MAX32635MBED: P1_6, P1_7 Arduino 10-pin header 06320 i2cMaster.frequency(g_I2C_SCL_Hz); 06321 i2cMaster.start(); 06322 i2cMaster.stop(); 06323 i2cMaster.frequency(g_I2C_SCL_Hz); 06324 cmdLine.serial().printf( 06325 "\r\n %%IC ADDR=0x%2.2x=(0x%2.2x>>1) SCL=%d=%1.3fkHz -- I2C config", 06326 g_I2C_deviceAddress7, (g_I2C_deviceAddress7 << 1), g_I2C_SCL_Hz, 06327 (g_I2C_SCL_Hz / 1000.)); 06328 i2cMaster.start(); 06329 i2cMaster.stop(); 06330 } 06331 } 06332 break; 06333 case 'W': case 'w': 06334 { 06335 // declare in narrower scope: MAX32625MBED I2C i2cMaster(...) 06336 I2C i2cMaster(I2C0_SDA, I2C0_SCL); // sda scl TARGET_MAX32635MBED: P1_6, P1_7 Arduino 10-pin header 06337 i2cMaster.frequency(g_I2C_SCL_Hz); 06338 // %IW byte byte ... byte RD=? ADDR=0x -- write 06339 // parse cmdLine byte list --> int byteCount; int mosiData[MAX_SPI_BYTE_COUNT]; 06340 #define MAX_I2C_BYTE_COUNT 32 06341 size_t byteCount = byteCount; 06342 static char mosiData[MAX_I2C_BYTE_COUNT]; 06343 static char misoData[MAX_I2C_BYTE_COUNT]; 06344 if (cmdLine.parse_byteCount_byteList_hex(byteCount, mosiData, 06345 MAX_I2C_BYTE_COUNT)) 06346 { 06347 // hex dump mosiData[0..byteCount-1] 06348 cmdLine.serial().printf( 06349 "\r\nADDR=0x%2.2x=(0x%2.2x>>1) byteCount:%d RD=%d\r\nI2C MOSI->", 06350 g_I2C_deviceAddress7, 06351 (g_I2C_deviceAddress7 << 1), byteCount, g_I2C_read_count); 06352 for (unsigned int byteIndex = 0; byteIndex < byteCount; byteIndex++) 06353 { 06354 cmdLine.serial().printf(" 0x%2.2X", mosiData[byteIndex]); 06355 } 06356 // 06357 // TODO: i2c transfer 06358 //const int addr7bit = 0x48; // 7 bit I2C address 06359 //const int addr8bit = 0x48 << 1; // 8bit I2C address, 0x90 06360 // /* int */ i2cMaster.read (int addr8bit, char *data, int length, bool repeated=false) // Read from an I2C slave. 06361 // /* int */ i2cMaster.read (int ack) // Read a single byte from the I2C bus. 06362 // /* int */ i2cMaster.write (int addr8bit, const char *data, int length, bool repeated=false) // Write to an I2C slave. 06363 // /* int */ i2cMaster.write (int data) // Write single byte out on the I2C bus. 06364 // /* void */ i2cMaster.start (void) // Creates a start condition on the I2C bus. 06365 // /* void */ i2cMaster.stop (void) // Creates a stop condition on the I2C bus. 06366 // /* int */ i2cMaster.transfer (int addr8bit, const char *tx_buffer, int tx_length, char *rx_buffer, int rx_length, const event_callback_t &callback, int event=I2C_EVENT_TRANSFER_COMPLETE, bool repeated=false) // Start nonblocking I2C transfer. More... 06367 // /* void */ i2cMaster.abort_transfer () // Abort the ongoing I2C transfer. More... 06368 const int addr8bit = g_I2C_deviceAddress7 << 1; // 8bit I2C address, 0x90 06369 unsigned int misoLength = 0; 06370 bool repeated = (g_I2C_read_count > 0); 06371 // 06372 int writeStatus = i2cMaster.write (addr8bit, mosiData, byteCount, repeated); 06373 switch (writeStatus) 06374 { 06375 case 0: cmdLine.serial().printf(" ack "); break; 06376 case 1: cmdLine.serial().printf(" nack "); break; 06377 default: cmdLine.serial().printf(" {writeStatus 0x%2.2X} ", 06378 writeStatus); 06379 } 06380 if (repeated) 06381 { 06382 int readStatus = 06383 i2cMaster.read (addr8bit, misoData, g_I2C_read_count, false); 06384 switch (readStatus) 06385 { 06386 case 1: cmdLine.serial().printf(" nack "); break; 06387 case 0: cmdLine.serial().printf(" ack "); break; 06388 default: cmdLine.serial().printf(" {readStatus 0x%2.2X} ", 06389 readStatus); 06390 } 06391 } 06392 // 06393 if (misoLength > 0) 06394 { 06395 // hex dump misoData[0..byteCount-1] 06396 cmdLine.serial().printf(" MISO<-"); 06397 for (unsigned int byteIndex = 0; byteIndex < g_I2C_read_count; 06398 byteIndex++) 06399 { 06400 cmdLine.serial().printf(" 0x%2.2X", misoData[byteIndex]); 06401 } 06402 } 06403 cmdLine.serial().printf(" "); 06404 } 06405 } 06406 break; 06407 case 'R': case 'r': 06408 { 06409 // declare in narrower scope: MAX32625MBED I2C i2cMaster(...) 06410 I2C i2cMaster(I2C0_SDA, I2C0_SCL); // sda scl TARGET_MAX32635MBED: P1_6, P1_7 Arduino 10-pin header 06411 i2cMaster.frequency(g_I2C_SCL_Hz); 06412 // %IR ADDR=? RD=? -- read 06413 // TODO: i2c transfer 06414 //const int addr7bit = 0x48; // 7 bit I2C address 06415 //const int addr8bit = 0x48 << 1; // 8bit I2C address, 0x90 06416 // /* int */ i2cMaster.read (int addr8bit, char *data, int length, bool repeated=false) // Read from an I2C slave. 06417 // /* int */ i2cMaster.read (int ack) // Read a single byte from the I2C bus. 06418 // /* int */ i2cMaster.write (int addr8bit, const char *data, int length, bool repeated=false) // Write to an I2C slave. 06419 // /* int */ i2cMaster.write (int data) // Write single byte out on the I2C bus. 06420 // /* void */ i2cMaster.start (void) // Creates a start condition on the I2C bus. 06421 // /* void */ i2cMaster.stop (void) // Creates a stop condition on the I2C bus. 06422 // /* int */ i2cMaster.transfer (int addr8bit, const char *tx_buffer, int tx_length, char *rx_buffer, int rx_length, const event_callback_t &callback, int event=I2C_EVENT_TRANSFER_COMPLETE, bool repeated=false) // Start nonblocking I2C transfer. More... 06423 // /* void */ i2cMaster.abort_transfer () // Abort the ongoing I2C transfer. More... 06424 } 06425 break; 06426 case '^': 06427 { 06428 // declare in narrower scope: MAX32625MBED I2C i2cMaster(...) 06429 I2C i2cMaster(I2C0_SDA, I2C0_SCL); // sda scl TARGET_MAX32635MBED: P1_6, P1_7 Arduino 10-pin header 06430 i2cMaster.frequency(g_I2C_SCL_Hz); 06431 // %I^ cmd=? -- i2c_smbus_read_word_data 06432 // TODO: i2c transfer 06433 //const int addr7bit = 0x48; // 7 bit I2C address 06434 //const int addr8bit = 0x48 << 1; // 8bit I2C address, 0x90 06435 // /* int */ i2cMaster.read (int addr8bit, char *data, int length, bool repeated=false) // Read from an I2C slave. 06436 // /* int */ i2cMaster.read (int ack) // Read a single byte from the I2C bus. 06437 // /* int */ i2cMaster.write (int addr8bit, const char *data, int length, bool repeated=false) // Write to an I2C slave. 06438 // /* int */ i2cMaster.write (int data) // Write single byte out on the I2C bus. 06439 // /* void */ i2cMaster.start (void) // Creates a start condition on the I2C bus. 06440 // /* void */ i2cMaster.stop (void) // Creates a stop condition on the I2C bus. 06441 // /* int */ i2cMaster.transfer (int addr8bit, const char *tx_buffer, int tx_length, char *rx_buffer, int rx_length, const event_callback_t &callback, int event=I2C_EVENT_TRANSFER_COMPLETE, bool repeated=false) // Start nonblocking I2C transfer. More... 06442 // /* void */ i2cMaster.abort_transfer () // Abort the ongoing I2C transfer. More... 06443 } 06444 break; 06445 } // switch(cmdLine[2]) 06446 break; 06447 #endif 06448 // 06449 #if HAS_SPI // SUPPORT_SPI 06450 case 'S': case 's': 06451 { 06452 // %S... -- SPI diagnostics 06453 // %SC sclk=1Mhz -- SPI configure 06454 // %SW -- write (write and read) 06455 // %SR -- read (alias for %SW because SPI always write and read) 06456 // 06457 // Process arguments SCLK=\d+(kHZ|MHZ) CPOL=\d CPHA=\d 06458 bool isUpdatedSPIConfig = false; 06459 // parse cmdLine arg (CPOL=\d)? --> g_SPI_dataMode | SPI_MODE2 06460 // parse cmdLine arg (CPHA=\d)? --> g_SPI_dataMode | SPI_MODE1 06461 if (cmdLine.parse_flag("CPOL", g_SPI_dataMode, SPI_MODE2)) 06462 { 06463 isUpdatedSPIConfig = true; 06464 } 06465 if (cmdLine.parse_flag("CPHA", g_SPI_dataMode, SPI_MODE1)) 06466 { 06467 isUpdatedSPIConfig = true; 06468 } 06469 if (cmdLine.parse_flag("CS", g_SPI_cs_state, 1)) 06470 { 06471 isUpdatedSPIConfig = true; 06472 } 06473 // parse cmdLine arg (SCLK=\d+(kHZ|MHZ)?)? --> g_SPI_SCLK_Hz 06474 if (cmdLine.parse_frequency_Hz("SCLK", g_SPI_SCLK_Hz)) 06475 { 06476 isUpdatedSPIConfig = true; 06477 // TODO1: validate g_SPI_SCLK_Hz against system clock frequency F_CPU 06478 if (g_SPI_SCLK_Hz > limit_max_SPI_SCLK_Hz) 06479 { 06480 g_SPI_SCLK_Hz = limit_max_SPI_SCLK_Hz; 06481 } 06482 if (g_SPI_SCLK_Hz < limit_min_SPI_SCLK_Hz) 06483 { 06484 g_SPI_SCLK_Hz = limit_min_SPI_SCLK_Hz; 06485 } 06486 } 06487 // Update SPI configuration 06488 if (isUpdatedSPIConfig) 06489 { 06490 // %SC sclk=1Mhz -- SPI configure 06491 spi_cs = g_SPI_cs_state; 06492 spi.format(8,g_SPI_dataMode); // int bits_must_be_8, int mode=0_3 CPOL=0,CPHA=0 06493 #if APPLICATION_MAX5715 06494 g_MAX5715_device.spi_frequency(g_SPI_SCLK_Hz); 06495 #elif APPLICATION_MAX11131 06496 g_MAX11131_device.spi_frequency(g_SPI_SCLK_Hz); 06497 #elif APPLICATION_MAX5171 06498 g_MAX5171_device.spi_frequency(g_SPI_SCLK_Hz); 06499 #elif APPLICATION_MAX11410 06500 g_MAX11410_device.spi_frequency(g_SPI_SCLK_Hz); 06501 #elif APPLICATION_MAX12345 06502 g_MAX12345_device.spi_frequency(g_SPI_SCLK_Hz); 06503 #else 06504 spi.frequency(g_SPI_SCLK_Hz); // int SCLK_Hz=1000000 = 1MHz (initial default) 06505 #endif 06506 // 06507 double ideal_divisor = ((double)SystemCoreClock) / g_SPI_SCLK_Hz; 06508 int actual_divisor = (int)(ideal_divisor + 0.0); // frequency divisor truncate 06509 double actual_SCLK_Hz = SystemCoreClock / actual_divisor; 06510 // 06511 // fixed: mbed-os-5.11: [Warning] format '%d' expects argument of type 'int', but argument 6 has type 'uint32_t {aka long unsigned int}' [-Wformat=] 06512 cmdLine.serial().printf( 06513 "\r\n %%SC CPOL=%d CPHA=%d CS=%d SCLK=%ld=%1.3fMHz (%1.1fMHz/%1.2f = actual %1.3fMHz) -- SPI config", 06514 ((g_SPI_dataMode & SPI_MODE2) ? 1 : 0), 06515 ((g_SPI_dataMode & SPI_MODE1) ? 1 : 0), 06516 g_SPI_cs_state, 06517 g_SPI_SCLK_Hz, 06518 (g_SPI_SCLK_Hz / 1000000.), 06519 ((double)(SystemCoreClock / 1000000.)), 06520 ideal_divisor, 06521 (actual_SCLK_Hz / 1000000.) 06522 ); 06523 } 06524 // get next character 06525 switch (cmdLine[2]) 06526 { 06527 case 'C': case 's': 06528 // %SC sclk=1Mhz -- SPI configure 06529 break; 06530 case 'D': case 'd': 06531 // %SD -- SPI diagnostic messages enable 06532 if (g_MAX5719_device.onSPIprint) { 06533 g_MAX5719_device.onSPIprint = NULL; 06534 // no g_MAX5719_device.loop_limit property; device_has_property(Device, 'loop_limit') != None is false 06535 } 06536 else { 06537 void onSPIprint_handler(size_t byteCount, uint8_t mosiData[], uint8_t misoData[]); 06538 g_MAX5719_device.onSPIprint = onSPIprint_handler; 06539 // no g_MAX5719_device.loop_limit property; device_has_property(Device, 'loop_limit') is false 06540 } 06541 break; 06542 case 'W': case 'R': case 'w': case 'r': 06543 { 06544 // %SW -- write (write and read) 06545 // %SR -- read (alias for %SW because SPI always write and read) 06546 // parse cmdLine byte list --> int byteCount; int mosiData[MAX_SPI_BYTE_COUNT]; 06547 #define MAX_SPI_BYTE_COUNT 32 06548 size_t byteCount = byteCount; 06549 static char mosiData[MAX_SPI_BYTE_COUNT]; 06550 static char misoData[MAX_SPI_BYTE_COUNT]; 06551 if (cmdLine.parse_byteCount_byteList_hex(byteCount, mosiData, 06552 MAX_SPI_BYTE_COUNT)) 06553 { 06554 // hex dump mosiData[0..byteCount-1] 06555 cmdLine.serial().printf("\r\nSPI"); 06556 if (byteCount > 7) { 06557 cmdLine.serial().printf(" byteCount:%d", byteCount); 06558 } 06559 cmdLine.serial().printf(" MOSI->"); 06560 for (unsigned int byteIndex = 0; byteIndex < byteCount; byteIndex++) 06561 { 06562 cmdLine.serial().printf(" 0x%2.2X", mosiData[byteIndex]); 06563 } 06564 spi_cs = 0; 06565 unsigned int numBytesTransferred = 06566 spi.write(mosiData, byteCount, misoData, byteCount); 06567 spi_cs = 1; 06568 // hex dump misoData[0..byteCount-1] 06569 cmdLine.serial().printf(" MISO<-"); 06570 for (unsigned int byteIndex = 0; byteIndex < numBytesTransferred; 06571 byteIndex++) 06572 { 06573 cmdLine.serial().printf(" 0x%2.2X", misoData[byteIndex]); 06574 } 06575 cmdLine.serial().printf(" "); 06576 } 06577 } 06578 break; 06579 } // switch(cmdLine[2]) 06580 } // case 'S': // %S... -- SPI diagnostics 06581 break; 06582 #endif 06583 // 06584 // A-Z,a-z,0-9 reserved for application use 06585 } // switch(cmdLine[1]) 06586 } // end void pinsMonitor_submenu_onEOLcommandParser(CmdLine & cmdLine) 06587 06588 06589 //-------------------------------------------------- 06590 void main_menu_status(CmdLine & cmdLine) 06591 { 06592 cmdLine.serial().printf("\r\nMain menu"); 06593 06594 cmdLine.serial().printf(" MAX5719 20-bit 0.05nV-sec DAC"); 06595 06596 //cmdLine.serial().print(" %s", TARGET_NAME); 06597 if (cmdLine.nameStr()) 06598 { 06599 cmdLine.serial().printf(" [%s]", cmdLine.nameStr()); 06600 06601 } 06602 cmdLine.serial().printf("\r\n ? -- help"); 06603 06604 } 06605 06606 06607 //-------------------------------------------------- 06608 void main_menu_help(CmdLine & cmdLine) 06609 { 06610 // ? -- help 06611 //~ cmdLine.serial().print(F("\r\nMenu:")); 06612 cmdLine.serial().printf("\r\n # -- lines beginning with # are comments"); 06613 06614 cmdLine.serial().printf("\r\n . runall=? runfail=? loopall=? loopfail=? -- SelfTest"); 06615 06616 //cmdLine.serial().print(F("\r\n ! -- Initial Configuration")); 06617 // 06618 // % standardize diagnostic commands 06619 // %Hpin -- digital output high 06620 // %Lpin -- digital output low 06621 // %?pin -- digital input 06622 // %A %Apin -- analog input 06623 // %Ppin df=xx -- pwm output 06624 // %Wpin -- measure high pulsewidth input in usec 06625 // %wpin -- measure low pulsewidth input in usec 06626 // %I... -- I2C diagnostics 06627 // %IP -- I2C probe 06628 // %IC scl=100khz ADDR=? -- I2C configure 06629 // %IW ADDR=? cmd=? data,data,data -- write 06630 // %IR ADDR=? RD=? -- read 06631 // %I^ cmd=? -- i2c_smbus_read_word_data 06632 // %S... -- SPI diagnostics 06633 // %SC sclk=1Mhz -- SPI configure 06634 // %SW -- write (write and read) 06635 // %SR -- read (alias for %SW because SPI always write and read) 06636 // A-Z,a-z,0-9 reserved for application use 06637 // 06638 #if HAS_digitalInOuts 06639 // %Hpin -- digital output high 06640 // %Lpin -- digital output low 06641 // %?pin -- digital input 06642 cmdLine.serial().printf("\r\n %%Hn {pin:"); 06643 list_digitalInOutPins(cmdLine.serial()); 06644 cmdLine.serial().printf("} -- High Output"); 06645 cmdLine.serial().printf("\r\n %%Ln {pin:"); 06646 list_digitalInOutPins(cmdLine.serial()); 06647 cmdLine.serial().printf("} -- Low Output"); 06648 cmdLine.serial().printf("\r\n %%?n {pin:"); 06649 list_digitalInOutPins(cmdLine.serial()); 06650 cmdLine.serial().printf("} -- Input"); 06651 #endif 06652 06653 #if HAS_analogIns 06654 // Menu A) analogRead A0..7 06655 // %A %Apin -- analog input 06656 // analogRead(pinIndex) // analog input pins A0, A1, A2, A3, A4, A5; float voltage = analogRead(A0) * (5.0 / 1023.0) 06657 cmdLine.serial().printf("\r\n %%A -- analogRead"); 06658 #endif 06659 06660 #if HAS_SPI2_MAX541 06661 // TODO1: MAX541 max541(spi2_max541, spi2_max541_cs); 06662 cmdLine.serial().printf("\r\n %%D -- DAC output MAX541 (SPI2)"); 06663 #endif 06664 06665 #if HAS_I2C // SUPPORT_I2C 06666 // TODO: support I2C HAS_I2C // SUPPORT_I2C 06667 // VERIFY: I2C utility commands SUPPORT_I2C 06668 // VERIFY: report g_I2C_SCL_Hz = (F_CPU / ((TWBR * 2) + 16)) from last Wire_Sr.setClock(I2C_SCL_Hz); 06669 // %I... -- I2C diagnostics 06670 // %IP -- I2C probe 06671 // %IC scl=100khz ADDR=? -- I2C configure 06672 // %IW byte byte ... byte RD=? ADDR=0x -- write 06673 // %IR ADDR=? RD=? -- read 06674 // %I^ cmd=? -- i2c_smbus_read_word_data 06675 //g_I2C_SCL_Hz = (F_CPU / ((TWBR * 2) + 16)); // 'F_CPU' 'TWBR' not declared in this scope 06676 cmdLine.serial().printf("\r\n %%IC ADDR=0x%2.2x=(0x%2.2x>>1) SCL=%d=%1.3fkHz -- I2C config", 06677 g_I2C_deviceAddress7, (g_I2C_deviceAddress7 << 1), g_I2C_SCL_Hz, 06678 (g_I2C_SCL_Hz / 1000.)); 06679 cmdLine.serial().printf("\r\n %%IW byte byte ... byte RD=? ADDR=0x%2.2x -- I2C write/read", 06680 g_I2C_deviceAddress7); 06681 // 06682 #if SUPPORT_I2C 06683 // Menu ^ cmd=?) i2c_smbus_read_word_data 06684 cmdLine.serial().printf("\r\n %%I^ cmd=? -- i2c_smbus_read_word_data"); 06685 // test low-level I2C i2c_smbus_read_word_data 06686 #endif // SUPPORT_I2C 06687 //cmdLine.serial().printf(" H) Hunt for attached I2C devices"); 06688 cmdLine.serial().printf("\r\n %%IP -- I2C Probe for attached devices"); 06689 // cmdLine.serial().printf(" s) search i2c address"); 06690 #endif // SUPPORT_I2C 06691 06692 #if HAS_SPI // SUPPORT_SPI 06693 // TODO: support SPI HAS_SPI // SUPPORT_SPI 06694 // SPI test command S (mosiData)+ 06695 // %S... -- SPI diagnostics 06696 // %SC sclk=1Mhz -- SPI configure 06697 // %SW -- write (write and read) 06698 // %SR -- read (alias for %SW because SPI always write and read) 06699 // spi.format(8,0); // int bits_must_be_8, int mode=0_3 CPOL=0,CPHA=0 rising edge (initial default) 06700 // spi.format(8,1); // int bits_must_be_8, int mode=0_3 CPOL=0,CPHA=1 falling edge (initial default) 06701 // spi.format(8,2); // int bits_must_be_8, int mode=0_3 CPOL=1,CPHA=0 falling edge (initial default) 06702 // spi.format(8,3); // int bits_must_be_8, int mode=0_3 CPOL=1,CPHA=1 rising edge (initial default) 06703 // spi.frequency(1000000); // int SCLK_Hz=1000000 = 1MHz (initial default) 06704 // mode | POL PHA 06705 // -----+-------- 06706 // 0 | 0 0 06707 // 1 | 0 1 06708 // 2 | 1 0 06709 // 3 | 1 1 06710 //cmdLine.serial().printf(" S) SPI mosi,mosi,...mosi hex bytes SCLK=1000000 CPOL=0 CPHA=0"); 06711 // fixed: mbed-os-5.11: [Warning] format '%d' expects argument of type 'int', but argument 3 has type 'uint32_t {aka long unsigned int}' [-Wformat=] 06712 cmdLine.serial().printf("\r\n %%SC SCLK=%ld=%1.3fMHz CPOL=%d CPHA=%d -- SPI config", 06713 g_SPI_SCLK_Hz, (g_SPI_SCLK_Hz / 1000000.), 06714 ((g_SPI_dataMode & SPI_MODE2) ? 1 : 0), 06715 ((g_SPI_dataMode & SPI_MODE1) ? 1 : 0)); 06716 cmdLine.serial().printf("\r\n %%SD -- SPI diagnostic messages "); 06717 if (g_MAX5719_device.onSPIprint) { 06718 cmdLine.serial().printf("hide"); 06719 } 06720 else { 06721 cmdLine.serial().printf("show"); 06722 } 06723 cmdLine.serial().printf("\r\n %%SW mosi,mosi,...mosi -- SPI write hex bytes"); 06724 // VERIFY: parse new SPI settings parse_strCommandArgs() SCLK=1000000 CPOL=0 CPHA=0 06725 #endif // SUPPORT_SPI 06726 // 06727 // Application-specific commands (help text) here 06728 // 06729 #if APPLICATION_ArduinoPinsMonitor 06730 cmdLine.serial().printf("\r\n A-Z,a-z,0-9 -- reserved for application use"); // ArduinoPinsMonitor 06731 #endif // APPLICATION_ArduinoPinsMonitor 06732 // 06733 06734 extern void MAX5719_menu_help(CmdLine & cmdLine); // defined in Test_Menu_MAX5719.cpp\n 06735 MAX5719_menu_help(cmdLine); 06736 } 06737 06738 06739 06740 //-------------------------------------------------- 06741 // main menu command-line parser 06742 // invoked by CmdLine::append(char ch) or CmdLine::idleAppendIfReadable() 06743 void main_menu_onEOLcommandParser(CmdLine & cmdLine) 06744 { 06745 // DIAGNOSTIC: print line buffer 06746 //~ cmdLine.serial().printf("\r\nmain_menu_onEOLcommandParser: ~%s~\r\n", cmdLine.str()); 06747 // 06748 switch (cmdLine[0]) 06749 { 06750 case '?': 06751 main_menu_status(cmdLine); 06752 main_menu_help(cmdLine); 06753 // print command prompt 06754 //cmdLine.serial().printf("\r\n>"); 06755 break; 06756 case '\r': case '\n': // ignore blank line 06757 case '\0': // ignore empty line 06758 case '#': // ignore comment line 06759 // # -- lines beginning with # are comments 06760 main_menu_status(cmdLine); 06761 //~ main_menu_help(cmdLine); 06762 // print command prompt 06763 //cmdLine.serial().printf("\r\n>"); 06764 break; 06765 #if ECHO_EOF_ON_EOL 06766 case '\x04': // Unicode (U+0004) EOT END OF TRANSMISSION = CTRL+D as EOF end of file 06767 cmdLine.serial().printf("\x04"); // immediately echo EOF for test scripting 06768 diagnostic_led_EOF(); 06769 break; 06770 case '\x1a': // Unicode (U+001A) SUB SUBSTITUTE = CTRL+Z as EOF end of file 06771 cmdLine.serial().printf("\x1a"); // immediately echo EOF for test scripting 06772 diagnostic_led_EOF(); 06773 break; 06774 #endif 06775 #if APPLICATION_ArduinoPinsMonitor 06776 case '.': 06777 { 06778 // . -- SelfTest 06779 cmdLine.serial().printf("SelfTest()"); 06780 // parse "run=0x0004" -- run selected tests 06781 if (cmdLine.parse_int_dec("run", SelfTestGroupEnable)) 06782 { 06783 } 06784 // parse "runall=0x0004" -- run selected tests, continue even if tests fail 06785 if (cmdLine.parse_int_dec("runall", SelfTestGroupEnable)) 06786 { 06787 SelfTestGroupEnable &=~ 2; // xxxxxx0x: no repeat-until-failure 06788 SelfTestGroupEnable &=~ 1; // xxxxxxx0: no halt-on-first-failure 06789 } 06790 // parse "runfail=0x0004" -- run selected tests, halt on first failure 06791 if (cmdLine.parse_int_dec("runfail", SelfTestGroupEnable)) 06792 { 06793 SelfTestGroupEnable &=~ 2; // xxxxxx0x: no repeat-until-failure 06794 SelfTestGroupEnable |= 1; // xxxxxxx1: halt-on-first-failure 06795 } 06796 // parse "loopall=0x0004" -- run selected tests, repeat until keypress 06797 if (cmdLine.parse_int_dec("loopall", SelfTestGroupEnable)) 06798 { 06799 SelfTestGroupEnable |= 2; // xxxxxx1x: repeat-until-failure 06800 SelfTestGroupEnable &=~ 1; // xxxxxx10 06801 } 06802 // parse "loopfail=0x0004" -- run selected tests, repeat until first failure 06803 if (cmdLine.parse_int_dec("loopfail", SelfTestGroupEnable)) 06804 { 06805 SelfTestGroupEnable |= 2; // xxxxxx1x: repeat-until-failure 06806 SelfTestGroupEnable |= 1; // xxxxxxx1: halt-on-first-failure 06807 } 06808 SelfTest(cmdLine); 06809 } 06810 break; 06811 case '%': 06812 { 06813 pinsMonitor_submenu_onEOLcommandParser(cmdLine); 06814 } 06815 break; // case '%' 06816 #endif // APPLICATION_ArduinoPinsMonitor 06817 // 06818 // Application-specific commands here 06819 // alphanumeric command codes A-Z,a-z,0-9 reserved for application use 06820 // 06821 #if APPLICATION_ArduinoPinsMonitor 06822 #endif // APPLICATION_ArduinoPinsMonitor 06823 06824 // 06825 // add new commands here 06826 // 06827 default: 06828 extern bool MAX5719_menu_onEOLcommandParser(CmdLine & cmdLine); // defined in Test_Menu_MAX5719.cpp 06829 if (!MAX5719_menu_onEOLcommandParser(cmdLine)) 06830 { // not_handled_by_device_submenu 06831 cmdLine.serial().printf("\r\n unknown command 0x%2.2x \"%s\"\r\n", cmdLine.str()[0], cmdLine.str()); 06832 06833 # if HAS_DAPLINK_SERIAL 06834 cmdLine_DAPLINKserial.serial().printf("\r\n unknown command 0x%2.2x \"%s\"\r\n", cmdLine.str()[0], cmdLine.str()); 06835 06836 # endif // HAS_DAPLINK_SERIAL 06837 } 06838 } // switch (cmdLine[0]) 06839 // 06840 // print command prompt 06841 cmdLine.serial().printf("\r\nMAX5719 > "); 06842 06843 } // end void main_menu_onEOLcommandParser(CmdLine & cmdLine) 06844 06845 //-------------------------------------------------- 06846 #if MAX5719_ONSPIPRINT 06847 // Optional Diagnostic function to print SPI transactions 06848 void onSPIprint_handler(size_t byteCount, uint8_t mosiData[], uint8_t misoData[]) 06849 { 06850 cmdLine_serial.serial().printf("\r\n SPI MOSI->"); 06851 for (uint8_t index = 0; index < byteCount; index++) { 06852 cmdLine_serial.serial().printf(" 0x%2.2X", mosiData[index]); 06853 } 06854 cmdLine_serial.serial().printf(" MISO<-"); 06855 for (uint8_t index = 0; index < byteCount; index++) { 06856 cmdLine_serial.serial().printf(" 0x%2.2X", misoData[index]); 06857 } 06858 cmdLine_serial.serial().printf(" "); 06859 } 06860 #endif // MAX5719_ONSPIPRINT 06861 06862 //-------------------------------------------------- 06863 void InitializeConfiguration() 06864 { 06865 // CODE GENERATOR: example code: member function Init 06866 # if HAS_DAPLINK_SERIAL 06867 cmdLine_DAPLINKserial.serial().printf("\r\nMAX5719_Init()"); 06868 06869 # endif 06870 cmdLine_serial.serial().printf("\r\nMAX5719_Init()"); 06871 06872 g_MAX5719_device.Init(); // defined in #include MAX5719.h 06873 # if MAX5719_ONSPIPRINT 06874 // Optional Diagnostic function to print SPI transactions 06875 # if MAX5719_ONSPIPRINT_ENABLED 06876 g_MAX5719_device.onSPIprint = onSPIprint_handler; 06877 # else 06878 g_MAX5719_device.onSPIprint = NULL; 06879 # endif 06880 # endif 06881 } // end of void InitializeConfiguration() 06882 06883 //-------------------------------------------------- 06884 // diagnostic rbg led GREEN 06885 void diagnostic_led_EOF() 06886 { 06887 #if USE_LEDS 06888 led1 = LED_ON; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led RED+GREEN=YELLOW 06889 // TODO1: mbed-os-5.11: [Warning] 'static osStatus rtos::Thread::wait(uint32_t)' is deprecated: Static methods only affecting current thread cause confusion. Replaced by ThisThread::sleep_for. [since mbed-os-5.10] [-Wdeprecated-declarations] 06890 ThisThread::sleep_for(250); // [since mbed-os-5.10] vs Thread::wait(250); 06891 led1 = LED_OFF; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led GREEN 06892 ThisThread::sleep_for(250); // [since mbed-os-5.10] vs Thread::wait(250); 06893 led1 = LED_ON; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led RED+GREEN=YELLOW 06894 ThisThread::sleep_for(250); // [since mbed-os-5.10] vs Thread::wait(250); 06895 led1 = LED_OFF; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led GREEN 06896 ThisThread::sleep_for(250); // [since mbed-os-5.10] vs Thread::wait(250); 06897 #endif // USE_LEDS 06898 } 06899 06900 //-------------------------------------------------- 06901 // Support commands that get handled immediately w/o waiting for EOL 06902 // handled as immediate command, do not append to buffer 06903 void on_immediate_0x21() // Unicode (U+0021) ! EXCLAMATION MARK 06904 { 06905 #if USE_LEDS 06906 led1 = LED_OFF; led2 = LED_OFF; led3 = LED_ON; // diagnostic rbg led BLUE 06907 #endif // USE_LEDS 06908 InitializeConfiguration(); 06909 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 06910 } 06911 06912 //-------------------------------------------------- 06913 // Support commands that get handled immediately w/o waiting for EOL 06914 // handled as immediate command, do not append to buffer 06915 void on_immediate_0x7b() // Unicode (U+007B) { LEFT CURLY BRACKET 06916 { 06917 #if HAS_BUTTON2_DEMO_INTERRUPT 06918 onButton2FallingEdge(); 06919 #endif 06920 } 06921 06922 //-------------------------------------------------- 06923 // Support commands that get handled immediately w/o waiting for EOL 06924 // handled as immediate command, do not append to buffer 06925 void on_immediate_0x7d() // Unicode (U+007D) } RIGHT CURLY BRACKET 06926 { 06927 #if HAS_BUTTON1_DEMO_INTERRUPT 06928 onButton1FallingEdge(); 06929 #endif 06930 } 06931 06932 //---------------------------------------- 06933 // example code main function 06934 int main() 06935 { 06936 // Configure serial ports 06937 cmdLine_serial.clear(); 06938 //~ cmdLine_serial.serial().printf("\r\n cmdLine_serial.serial().printf test\r\n"); 06939 cmdLine_serial.onEOLcommandParser = main_menu_onEOLcommandParser; 06940 cmdLine_serial.diagnostic_led_EOF = diagnostic_led_EOF; 06941 /// CmdLine::set_immediate_handler(char, functionPointer_void_void_on_immediate_0x21); 06942 cmdLine_serial.on_immediate_0x21 = on_immediate_0x21; 06943 cmdLine_serial.on_immediate_0x7b = on_immediate_0x7b; 06944 cmdLine_serial.on_immediate_0x7d = on_immediate_0x7d; 06945 # if HAS_DAPLINK_SERIAL 06946 cmdLine_DAPLINKserial.clear(); 06947 //~ cmdLine_DAPLINKserial.serial().printf("\r\n cmdLine_DAPLINKserial.serial().printf test\r\n"); 06948 cmdLine_DAPLINKserial.onEOLcommandParser = main_menu_onEOLcommandParser; 06949 /// @todo CmdLine::set_immediate_handler(char, functionPointer_void_void_on_immediate_0x21); 06950 cmdLine_DAPLINKserial.on_immediate_0x21 = on_immediate_0x21; 06951 cmdLine_DAPLINKserial.on_immediate_0x7b = on_immediate_0x7b; 06952 cmdLine_DAPLINKserial.on_immediate_0x7d = on_immediate_0x7d; 06953 # endif 06954 06955 06956 //print_banner(); 06957 06958 #if HAS_I2C 06959 // i2c init 06960 // declare in narrower scope: MAX32625MBED I2C i2cMaster(...) 06961 // i2cMaster.frequency(g_I2C_SCL_Hz); 06962 #else 06963 // Ensure that the unused I2C pins do not interfere with analog inputs A4 and A5 06964 #if HAS_digitalInOut14 06965 // DigitalInOut digitalInOut14(P1_6, PIN_INPUT, PullUp, 1); // P1_6 TARGET_MAX32635MBED A4/SDA (10pin digital connector) 06966 digitalInOut14.input(); 06967 #endif 06968 #if HAS_digitalInOut15 06969 // DigitalInOut digitalInOut15(P1_7, PIN_INPUT, PullUp, 1); // P1_7 TARGET_MAX32635MBED A5/SCL (10pin digital connector) 06970 digitalInOut15.input(); 06971 #endif 06972 #if HAS_digitalInOut16 06973 // DigitalInOut mode can be one of PullUp, PullDown, PullNone, OpenDrain 06974 // PullUp-->3.4V, PullDown-->1.7V, PullNone-->3.5V, OpenDrain-->0.00V 06975 //DigitalInOut digitalInOut16(P3_4, PIN_INPUT, OpenDrain, 0); // P3_4 TARGET_MAX32635MBED A4/SDA (6pin analog connector) 06976 digitalInOut16.input(); 06977 #endif 06978 #if HAS_digitalInOut17 06979 //DigitalInOut digitalInOut17(P3_5, PIN_INPUT, OpenDrain, 0); // P3_5 TARGET_MAX32635MBED A5/SCL (6pin analog connector) 06980 digitalInOut17.input(); 06981 #endif 06982 #endif // HAS_I2C 06983 06984 06985 #if USE_LEDS 06986 #if defined(TARGET_MAX32630) 06987 led1 = LED_ON; led2 = LED_OFF; led3 = LED_OFF; // diagnostic rbg led RED 06988 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 06989 led1 = LED_OFF; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led GREEN 06990 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 06991 led1 = LED_OFF; led2 = LED_OFF; led3 = LED_ON; // diagnostic rbg led BLUE 06992 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 06993 led1 = LED_ON; led2 = LED_ON; led3 = LED_ON; // diagnostic rbg led RED+GREEN+BLUE=WHITE 06994 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 06995 led1 = LED_OFF; led2 = LED_ON; led3 = LED_ON; // diagnostic rbg led GREEN+BLUE=CYAN 06996 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 06997 led1 = LED_ON; led2 = LED_OFF; led3 = LED_ON; // diagnostic rbg led RED+BLUE=MAGENTA 06998 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 06999 led1 = LED_ON; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led RED+GREEN=YELLOW 07000 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 07001 led1 = LED_OFF; led2 = LED_OFF; led3 = LED_OFF; // diagnostic rbg led BLACK 07002 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 07003 #elif defined(TARGET_MAX32625MBED) 07004 led1 = LED_ON; led2 = LED_OFF; led3 = LED_OFF; // diagnostic rbg led RED 07005 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 07006 led1 = LED_OFF; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led GREEN 07007 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 07008 led1 = LED_OFF; led2 = LED_OFF; led3 = LED_ON; // diagnostic rbg led BLUE 07009 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 07010 led1 = LED_ON; led2 = LED_ON; led3 = LED_ON; // diagnostic rbg led RED+GREEN+BLUE=WHITE 07011 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 07012 led1 = LED_OFF; led2 = LED_ON; led3 = LED_ON; // diagnostic rbg led GREEN+BLUE=CYAN 07013 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 07014 led1 = LED_ON; led2 = LED_OFF; led3 = LED_ON; // diagnostic rbg led RED+BLUE=MAGENTA 07015 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 07016 led1 = LED_ON; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led RED+GREEN=YELLOW 07017 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 07018 led1 = LED_OFF; led2 = LED_OFF; led3 = LED_OFF; // diagnostic rbg led BLACK 07019 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 07020 #else // not defined(TARGET_LPC1768 etc.) 07021 led1 = LED_ON; 07022 led2 = LED_OFF; 07023 led3 = LED_OFF; 07024 led4 = LED_OFF; 07025 ThisThread::sleep_for(75); // [since mbed-os-5.10] vs Thread::wait(75); 07026 //led1 = LED_ON; 07027 led2 = LED_ON; 07028 ThisThread::sleep_for(75); // [since mbed-os-5.10] vs Thread::wait(75); 07029 led1 = LED_OFF; 07030 //led2 = LED_ON; 07031 led3 = LED_ON; 07032 ThisThread::sleep_for(75); // [since mbed-os-5.10] vs Thread::wait(75); 07033 led2 = LED_OFF; 07034 //led3 = LED_ON; 07035 led4 = LED_ON; 07036 ThisThread::sleep_for(75); // [since mbed-os-5.10] vs Thread::wait(75); 07037 led3 = LED_OFF; 07038 led4 = LED_ON; 07039 // 07040 #endif // target definition 07041 #endif 07042 07043 // cmd_TE(); 07044 07045 // #if USE_LEDS 07046 // rgb_led.white(); // diagnostic rbg led RED+GREEN+BLUE=WHITE 07047 // #endif // USE_LEDS 07048 if (led1.is_connected()) { 07049 led1 = LED_ON; 07050 } 07051 if (led2.is_connected()) { 07052 led2 = LED_ON; 07053 } 07054 if (led3.is_connected()) { 07055 led3 = LED_ON; 07056 } 07057 07058 InitializeConfiguration(); 07059 // example code: serial port banner message 07060 #if defined(TARGET_MAX32625MBED) 07061 serial.printf("MAX32625MBED "); 07062 #elif defined(TARGET_MAX32625PICO) 07063 serial.printf("MAX32625PICO "); 07064 #elif defined(TARGET_MAX32600MBED) 07065 serial.printf("MAX32600MBED "); 07066 #elif defined(TARGET_NUCLEO_F446RE) 07067 serial.printf("NUCLEO_F446RE "); 07068 #endif 07069 serial.printf("MAX5719BOB\r\n"); 07070 07071 07072 while (1) { 07073 #if HAS_BUTTON1_DEMO_INTERRUPT_POLLING 07074 // avoid runtime error on button1 press [mbed-os-5.11] 07075 // instead of using InterruptIn, use DigitalIn and poll in main while(1) 07076 # if HAS_BUTTON1_DEMO_INTERRUPT 07077 static int button1_value_prev = 1; 07078 static int button1_value_now = 1; 07079 button1_value_prev = button1_value_now; 07080 button1_value_now = button1.read(); 07081 if ((button1_value_prev - button1_value_now) == 1) 07082 { 07083 // on button1 falling edge (button1 press) 07084 onButton1FallingEdge(); 07085 } 07086 # endif // HAS_BUTTON1_DEMO_INTERRUPT 07087 # if HAS_BUTTON2_DEMO_INTERRUPT 07088 static int button2_value_prev = 1; 07089 static int button2_value_now = 1; 07090 button2_value_prev = button2_value_now; 07091 button2_value_now = button2.read(); 07092 if ((button2_value_prev - button2_value_now) == 1) 07093 { 07094 // on button2 falling edge (button2 press) 07095 onButton2FallingEdge(); 07096 } 07097 # endif // HAS_BUTTON2_DEMO_INTERRUPT 07098 #endif 07099 # if HAS_DAPLINK_SERIAL 07100 if (DAPLINKserial.readable()) { 07101 cmdLine_DAPLINKserial.append(DAPLINKserial.getc()); 07102 } 07103 # endif // HAS_DAPLINK_SERIAL 07104 if (serial.readable()) { 07105 int c = serial.getc(); 07106 cmdLine_serial.append(c); 07107 #if IGNORE_AT_COMMANDS 07108 # if HAS_DAPLINK_SERIAL 07109 cmdLine_DAPLINKserial.serial().printf("%c", c); 07110 # endif // HAS_DAPLINK_SERIAL 07111 #endif // IGNORE_AT_COMMANDS 07112 // 07113 } 07114 } // while(1) 07115 }
Generated on Tue Jul 12 2022 16:06:18 by
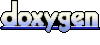