
Example host software for the Maxim Integrated MAX5715 12-bit 4-channel voltage-output DAC. Hosted on the MAX32625MBED.
Dependencies: MAX5715
Hello_MAX5715.cpp
00001 // /******************************************************************************* 00002 // * Copyright (C) 2019 Maxim Integrated Products, Inc., All Rights Reserved. 00003 // * 00004 // * Permission is hereby granted, free of charge, to any person obtaining a 00005 // * copy of this software and associated documentation files (the "Software"), 00006 // * to deal in the Software without restriction, including without limitation 00007 // * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 // * and/or sell copies of the Software, and to permit persons to whom the 00009 // * Software is furnished to do so, subject to the following conditions: 00010 // * 00011 // * The above copyright notice and this permission notice shall be included 00012 // * in all copies or substantial portions of the Software. 00013 // * 00014 // * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 // * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 // * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 // * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 // * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 // * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 // * OTHER DEALINGS IN THE SOFTWARE. 00021 // * 00022 // * Except as contained in this notice, the name of Maxim Integrated 00023 // * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 // * Products, Inc. Branding Policy. 00025 // * 00026 // * The mere transfer of this software does not imply any licenses 00027 // * of trade secrets, proprietary technology, copyrights, patents, 00028 // * trademarks, maskwork rights, or any other form of intellectual 00029 // * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 // * ownership rights. 00031 // ******************************************************************************* 00032 // */ 00033 // example code includes 00034 // standard include for target platform -- Platform_Include_Boilerplate 00035 #include "mbed.h" 00036 // Platforms: 00037 // - MAX32625MBED 00038 // - supports mbed-os-5.11, requires USBDevice library 00039 // - add https://developer.mbed.org/teams/MaximIntegrated/code/USBDevice/ 00040 // - remove max32630fthr library (if present) 00041 // - remove MAX32620FTHR library (if present) 00042 // - MAX32600MBED 00043 // - remove max32630fthr library (if present) 00044 // - remove MAX32620FTHR library (if present) 00045 // - Windows 10 note: Don't connect HDK until you are ready to load new firmware into the board. 00046 // - NUCLEO_F446RE 00047 // - remove USBDevice library 00048 // - remove max32630fthr library (if present) 00049 // - remove MAX32620FTHR library (if present) 00050 // - NUCLEO_F401RE 00051 // - remove USBDevice library 00052 // - remove max32630fthr library (if present) 00053 // - remove MAX32620FTHR library (if present) 00054 // - MAX32630FTHR 00055 // - #include "max32630fthr.h" 00056 // - add http://os.mbed.org/teams/MaximIntegrated/code/max32630fthr/ 00057 // - remove MAX32620FTHR library (if present) 00058 // - MAX32620FTHR 00059 // - #include "MAX32620FTHR.h" 00060 // - remove max32630fthr library (if present) 00061 // - add https://os.mbed.com/teams/MaximIntegrated/code/MAX32620FTHR/ 00062 // - not tested yet 00063 // - MAX32625PICO 00064 // - remove max32630fthr library (if present) 00065 // - remove MAX32620FTHR library (if present) 00066 // - not tested yet 00067 // 00068 // end Platform_Include_Boilerplate 00069 #include "MAX5715.h" 00070 00071 // example code board support 00072 //MAX32630FTHR pegasus(MAX32630FTHR::VIO_3V3); 00073 //DigitalOut rLED(LED1); 00074 //DigitalOut gLED(LED2); 00075 //DigitalOut bLED(LED3); 00076 // 00077 // Arduino "shield" connector port definitions (MAX32625MBED shown) 00078 #if defined(TARGET_MAX32625MBED) 00079 #define A0 AIN_0 00080 #define A1 AIN_1 00081 #define A2 AIN_2 00082 #define A3 AIN_3 00083 #define D0 P0_0 00084 #define D1 P0_1 00085 #define D2 P0_2 00086 #define D3 P0_3 00087 #define D4 P0_4 00088 #define D5 P0_5 00089 #define D6 P0_6 00090 #define D7 P0_7 00091 #define D8 P1_4 00092 #define D9 P1_5 00093 #define D10 P1_3 00094 #define D11 P1_1 00095 #define D12 P1_2 00096 #define D13 P1_0 00097 #endif 00098 00099 // example code declare SPI interface 00100 #if defined(TARGET_MAX32625MBED) 00101 SPI spi(SPI1_MOSI, SPI1_MISO, SPI1_SCK); // mosi, miso, sclk spi1 TARGET_MAX32625MBED: P1_1 P1_2 P1_0 Arduino 10-pin header D11 D12 D13 00102 DigitalOut spi_cs(SPI1_SS); // TARGET_MAX32625MBED: P1_3 Arduino 10-pin header D10 00103 #elif defined(TARGET_MAX32600MBED) 00104 SPI spi(SPI2_MOSI, SPI2_MISO, SPI2_SCK); // mosi, miso, sclk spi1 TARGET_MAX32600MBED: Arduino 10-pin header D11 D12 D13 00105 DigitalOut spi_cs(SPI2_SS); // Generic: Arduino 10-pin header D10 00106 #else 00107 SPI spi(D11, D12, D13); // mosi, miso, sclk spi1 TARGET_MAX32600MBED: Arduino 10-pin header D11 D12 D13 00108 DigitalOut spi_cs(D10); // Generic: Arduino 10-pin header D10 00109 #endif 00110 00111 // example code declare GPIO interface pins 00112 DigitalOut LDACb_pin(D9); // Digital Trigger Input to MAX5715 device 00113 DigitalOut CLRb_pin(D8); // Digital Trigger Input to MAX5715 device 00114 // AnalogOut REF_pin(Px_x_PortName_To_Be_Determined); // Reference Input to MAX5715 device 00115 // AnalogIn OUTA_pin(A0); // Analog Output from MAX5715 device 00116 // AnalogIn OUTB_pin(A1); // Analog Output from MAX5715 device 00117 // AnalogIn OUTC_pin(A2); // Analog Output from MAX5715 device 00118 // AnalogIn OUTD_pin(A3); // Analog Output from MAX5715 device 00119 // DigitalIn RDYb_pin(Px_x_PortName_To_Be_Determined); // Digital MAX5715 Output from MAX5715 device 00120 // example code declare device instance 00121 MAX5715 g_MAX5715_device(spi, spi_cs, LDACb_pin, CLRb_pin, MAX5715::MAX5715_IC); 00122 00123 // example code main function 00124 int main() 00125 { 00126 g_MAX5715_device.Init(); 00127 00128 while (1) 00129 { 00130 g_MAX5715_device.REF(MAX5715::REF_AlwaysOn_2V500); 00131 00132 uint16_t ch; 00133 uint16_t code; 00134 // 00135 ch = 0; 00136 code = 0x0ccc; // 80.0% of full scale REF(2.50V) = 2.00V 00137 g_MAX5715_device.CODEnLOADn(ch, code); 00138 // 00139 ch = 1; 00140 code = 0x07ff; // 50.0% of full scale REF(2.50V) = 1.25V 00141 g_MAX5715_device.CODEnLOADn(ch, code); 00142 // 00143 ch = 2; 00144 code = 0x0666; // 40.0% of full scale REF(2.50V) = 1.00V 00145 g_MAX5715_device.CODEnLOADn(ch, code); 00146 // 00147 ch = 3; 00148 code = 0x0fff; // 100.0% of full scale REF(2.50V) = 2.50V 00149 g_MAX5715_device.CODEnLOADn(ch, code); 00150 00151 // wait(3.0); 00152 } 00153 }
Generated on Thu Jul 14 2022 04:45:39 by
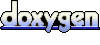