
Test program running on MAX32625MBED. Control through USB Serial commands using a terminal emulator such as teraterm or putty.
Dependencies: MaximTinyTester CmdLine MAX5171 USBDevice
Test_Menu_MAX5171.cpp
00001 // /******************************************************************************* 00002 // * Copyright (C) 2020 Maxim Integrated Products, Inc., All Rights Reserved. 00003 // * 00004 // * Permission is hereby granted, free of charge, to any person obtaining a 00005 // * copy of this software and associated documentation files (the "Software"), 00006 // * to deal in the Software without restriction, including without limitation 00007 // * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 // * and/or sell copies of the Software, and to permit persons to whom the 00009 // * Software is furnished to do so, subject to the following conditions: 00010 // * 00011 // * The above copyright notice and this permission notice shall be included 00012 // * in all copies or substantial portions of the Software. 00013 // * 00014 // * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 // * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 // * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 // * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 // * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 // * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 // * OTHER DEALINGS IN THE SOFTWARE. 00021 // * 00022 // * Except as contained in this notice, the name of Maxim Integrated 00023 // * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 // * Products, Inc. Branding Policy. 00025 // * 00026 // * The mere transfer of this software does not imply any licenses 00027 // * of trade secrets, proprietary technology, copyrights, patents, 00028 // * trademarks, maskwork rights, or any other form of intellectual 00029 // * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 // * ownership rights. 00031 // ******************************************************************************* 00032 // */ 00033 // example code includes 00034 // standard include for target platform -- Platform_Include_Boilerplate 00035 #include "mbed.h" 00036 // Platforms: 00037 // - MAX32625MBED 00038 // - supports mbed-os-5.11, requires USBDevice library 00039 // - add https://developer.mbed.org/teams/MaximIntegrated/code/USBDevice/ 00040 // - remove max32630fthr library (if present) 00041 // - remove MAX32620FTHR library (if present) 00042 // - MAX32600MBED 00043 // - remove max32630fthr library (if present) 00044 // - remove MAX32620FTHR library (if present) 00045 // - Windows 10 note: Don't connect HDK until you are ready to load new firmware into the board. 00046 // - NUCLEO_F446RE 00047 // - remove USBDevice library 00048 // - remove max32630fthr library (if present) 00049 // - remove MAX32620FTHR library (if present) 00050 // - NUCLEO_F401RE 00051 // - remove USBDevice library 00052 // - remove max32630fthr library (if present) 00053 // - remove MAX32620FTHR library (if present) 00054 // - MAX32630FTHR 00055 // - #include "max32630fthr.h" 00056 // - add http://os.mbed.org/teams/MaximIntegrated/code/max32630fthr/ 00057 // - remove MAX32620FTHR library (if present) 00058 // - MAX32620FTHR 00059 // - #include "MAX32620FTHR.h" 00060 // - remove max32630fthr library (if present) 00061 // - add https://os.mbed.com/teams/MaximIntegrated/code/MAX32620FTHR/ 00062 // - not tested yet 00063 // - MAX32625PICO 00064 // - remove max32630fthr library (if present) 00065 // - remove MAX32620FTHR library (if present) 00066 // - not tested yet 00067 // 00068 // end Platform_Include_Boilerplate 00069 #include "MAX5171.h" 00070 #include "CmdLine.h" 00071 #include "MaximTinyTester.h" 00072 00073 #include "MAX5171.h" 00074 extern MAX5171 g_MAX5171_device; // defined in main.cpp 00075 00076 00077 00078 void MAX5171_menu_help(CmdLine & cmdLine) 00079 { 00080 cmdLine.serial().printf("\r\n ! -- Init"); 00081 cmdLine.serial().printf("\r\n 0 code=? -- CODE"); 00082 cmdLine.serial().printf("\r\n 4 code=? -- CODE_LOAD"); 00083 cmdLine.serial().printf("\r\n 8 -- LOAD"); 00084 cmdLine.serial().printf("\r\n c -- NOP"); 00085 cmdLine.serial().printf("\r\n d -- SHUTDOWN"); 00086 cmdLine.serial().printf("\r\n e0 -- UPO_LOW"); 00087 cmdLine.serial().printf("\r\n e8 -- UPO_HIGH"); 00088 cmdLine.serial().printf("\r\n f0 -- MODE1_DOUT_SCLK_RISING_EDGE"); 00089 cmdLine.serial().printf("\r\n f8 -- MODE0_DOUT_SCLK_FALLING_EDGE"); 00090 // 00091 cmdLine.serial().printf("\r\n @ -- print MAX5171 configuration"); 00092 00093 // 00094 // case 'G'..'Z','g'..'z' are reserved for GPIO commands 00095 // case 'A'..'F','a'..'f' may be available if not claimed by bitstream commands 00096 00097 // 00098 } 00099 00100 bool MAX5171_menu_onEOLcommandParser(CmdLine & cmdLine) 00101 { 00102 00103 00104 // parse argument int16_t DACCode 00105 int16_t DACCode = g_MAX5171_device.DACCode; // default to global property value 00106 if (cmdLine.parse_int16_dec("DACCode", DACCode)) 00107 { 00108 g_MAX5171_device.DACCode = DACCode; // update global property value 00109 } 00110 // "code" is an alias for argument "DACCode" 00111 if (cmdLine.parse_int16_dec("code", DACCode)) 00112 { 00113 g_MAX5171_device.DACCode = DACCode; // update global property value 00114 } 00115 00116 // parse argument double VRef 00117 double VRef = g_MAX5171_device.VRef; // default to global property value 00118 if (cmdLine.parse_double("VRef", VRef)) 00119 { 00120 g_MAX5171_device.VRef = VRef; // update global property value 00121 } 00122 00123 // parse argument uint16_t dacCodeLsbs 00124 uint16_t dacCodeLsbs = g_MAX5171_device.DACCode; // default to global property value 00125 if (cmdLine.parse_uint16_dec("dacCodeLsbs", dacCodeLsbs)) 00126 { 00127 g_MAX5171_device.DACCode = dacCodeLsbs; // update global property value 00128 } 00129 // "code" is an alias for argument "dacCodeLsbs" 00130 if (cmdLine.parse_uint16_dec("code", dacCodeLsbs)) 00131 { 00132 g_MAX5171_device.DACCode = dacCodeLsbs; // update global property value 00133 } 00134 00135 switch (cmdLine[0]) 00136 { 00137 case '@': 00138 { 00139 cmdLine.serial().printf("VRef = "); 00140 cmdLine.serial().printf("%1.6f\r\n", g_MAX5171_device.VRef); 00141 cmdLine.serial().printf("DACCode = "); 00142 cmdLine.serial().printf("%d = 0x%4.4x\r\n", g_MAX5171_device.DACCode, g_MAX5171_device.DACCode); 00143 return true; // command handled by MAX5171 00144 break; 00145 } 00146 // case 'G'..'Z','g'..'z' are reserved for GPIO commands 00147 // case 'A'..'F','a'..'f' may be available if not claimed by bitstream commands 00148 // case '0'..'9','A'..'F','a'..'f' letters are reserved for bitstream commands 00149 case '!': 00150 { 00151 // test menu command '!' handler: 00152 // helpString='! -- Init' 00153 // CMD_='None' 00154 // CommandName='Init' 00155 // CommandParamIn='void' 00156 // CommandReturnType='void' 00157 // @Pre='' 00158 // @Param[in]='' 00159 // @Param[out]='' 00160 // @Post='' 00161 // displayPost='' 00162 // @Return='' 00163 // @Test='@test group DACCodeOfVoltage // Verify function DACCodeOfVoltage (enabled by default)' 00164 // @Test='@test group DACCodeOfVoltage tinyTester.blink_time_msec = 20 // quickly speed through the software verification' 00165 // @Test='@test group DACCodeOfVoltage tinyTester.print("VRef = 2.500 MAX5171 14-bit LSB = 0.00015V")' 00166 // @Test='@test group DACCodeOfVoltage VRef = 2.500' 00167 // @Test='@test group DACCodeOfVoltage tinyTester.err_threshold = 0.00015259720441921504 // 14-bit LSB (2.500/16383)' 00168 // @Test='@test group DACCodeOfVoltage DACCodeOfVoltage(2.499847412109375) expect 0x3FFF' 00169 // @Test='@test group DACCodeOfVoltage DACCodeOfVoltage(2.49969482421875) expect 0x3FFE' 00170 // @Test='@test group DACCodeOfVoltage DACCodeOfVoltage(2.499542236328125) expect 0x3FFD' 00171 // @Test='@test group DACCodeOfVoltage DACCodeOfVoltage(2.4993896484375) expect 0x3FFC' 00172 // @Test='@test group DACCodeOfVoltage DACCodeOfVoltage(1.250152587890625) expect 0x2001' 00173 // @Test='@test group DACCodeOfVoltage DACCodeOfVoltage(1.25) expect 0x2000' 00174 // @Test='@test group DACCodeOfVoltage DACCodeOfVoltage(1.249847412109375) expect 0x1FFF' 00175 // @Test='@test group DACCodeOfVoltage DACCodeOfVoltage(1.24969482421875) expect 0x1FFE' 00176 // @Test='@test group DACCodeOfVoltage DACCodeOfVoltage(0.000457763671875) expect 0x0003' 00177 // @Test='@test group DACCodeOfVoltage DACCodeOfVoltage(0.00030517578125) expect 0x0002' 00178 // @Test='@test group DACCodeOfVoltage DACCodeOfVoltage(0.000152587890625) expect 0x0001' 00179 // @Test='@test group DACCodeOfVoltage DACCodeOfVoltage(0.00000) expect 0x0000' 00180 // @Test='@test group DACCodeOfVoltage tinyTester.blink_time_msec = 75 // default 75 resume hardware self test' 00181 // @Test='@test group VoltageOfCode // Verify function VoltageOfCode (enabled by default)' 00182 // @Test='@test group VoltageOfCode tinyTester.blink_time_msec = 20 // quickly speed through the software verification' 00183 // @Test='@test group VoltageOfCode tinyTester.print("VRef = 2.500 MAX5171 14-bit LSB = 0.00015V")' 00184 // @Test='@test group VoltageOfCode VRef = 2.500' 00185 // @Test='@test group VoltageOfCode tinyTester.err_threshold = 0.00015259720441921504 // 14-bit LSB (2.500/16383)' 00186 // @Test='@test group VoltageOfCode VoltageOfCode(0x3FFF) expect 2.499847412109375' 00187 // @Test='@test group VoltageOfCode VoltageOfCode(0x3FFE) expect 2.49969482421875' 00188 // @Test='@test group VoltageOfCode VoltageOfCode(0x3FFD) expect 2.499542236328125' 00189 // @Test='@test group VoltageOfCode VoltageOfCode(0x3FFC) expect 2.4993896484375' 00190 // @Test='@test group VoltageOfCode VoltageOfCode(0x2001) expect 1.250152587890625' 00191 // @Test='@test group VoltageOfCode VoltageOfCode(0x2000) expect 1.25' 00192 // @Test='@test group VoltageOfCode VoltageOfCode(0x1FFF) expect 1.249847412109375' 00193 // @Test='@test group VoltageOfCode VoltageOfCode(0x1FFE) expect 1.24969482421875' 00194 // @Test='@test group VoltageOfCode VoltageOfCode(0x0003) expect 0.000457763671875' 00195 // @Test='@test group VoltageOfCode VoltageOfCode(0x0002) expect 0.00030517578125' 00196 // @Test='@test group VoltageOfCode VoltageOfCode(0x0001) expect 0.000152587890625' 00197 // @Test='@test group VoltageOfCode VoltageOfCode(0x0000) expect 0.00000' 00198 // @Test='@test group VoltageOfCode tinyTester.blink_time_msec = 75 // default 75 resume hardware self test' 00199 // @Test='@test group CODE_LOAD // Verify function CODE_LOAD (enabled by default)' 00200 // @Test='@test group CODE_LOAD tinyTester.blink_time_msec = 75 // default 75 resume hardware self test' 00201 // @Test='@test group CODE_LOAD tinyTester.settle_time_msec = 500' 00202 // @Test='@test Init()' 00203 // @Test='@test VRef expect 2.500 // Nominal Full-Scale Voltage Reference' 00204 // @Test='@test group CODE_LOAD tinyTester.err_threshold = 0.050' 00205 // @Test='@test group CODE_LOAD tinyTester.print("100.0% of full scale REF(2.50V) = 2.50V Jumper FB=1-2")' 00206 // @Test='@test group CODE_LOAD CODE_LOAD(0x3FFF) // 100.0% of full scale REF(2.50V) = 2.50V' 00207 // @Test='@test group CODE_LOAD tinyTester.Wait_Output_Settling()' 00208 // @Test='@test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(2.500)' 00209 // @Test='@test group CODE_LOAD tinyTester.print("0.0% of full scale REF(2.50V) = 0.000V")' 00210 // @Test='@test group CODE_LOAD CODE_LOAD(0x0000) // 0.0% of full scale REF(2.50V) = 0.000V' 00211 // @Test='@test group CODE_LOAD tinyTester.Wait_Output_Settling()' 00212 // @Test='@test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(0.0000)' 00213 // @Test='@test group CODE_LOAD tinyTester.print("50.0% of full scale REF(2.50V) = 1.25V")' 00214 // @Test='@test group CODE_LOAD CODE_LOAD(0x1FFF) // 50.0% of full scale REF(2.50V) = 1.25V' 00215 // @Test='@test group CODE_LOAD tinyTester.Wait_Output_Settling()' 00216 // @Test='@test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(1.2500)' 00217 // @Test='@test group UPO // Verify User Programmable Output functions UPO_HIGH and UPO_LOW (enabled by default)' 00218 // @Test='@test group UPO tinyTester.blink_time_msec = 75 // default 75 resume hardware self test' 00219 // @Test='@test group UPO tinyTester.settle_time_msec = 500 // default 250' 00220 // @Test='@test group UPO UPO_HIGH()' 00221 // @Test='@test group UPO tinyTester.Wait_Output_Settling()' 00222 // @Test='@test group CODE_LOAD tinyTester.DigitalIn_Read_Expect_WarnOnly(UPO_pin, "UPO", 1, "UPO_pin is high after MAX5171 UPO_HIGH command")' 00223 // @Test='@test group UPO UPO_LOW()' 00224 // @Test='@test group UPO tinyTester.Wait_Output_Settling()' 00225 // @Test='@test group CODE_LOAD tinyTester.DigitalIn_Read_Expect_WarnOnly(UPO_pin, "UPO", 0, "UPO_pin is low after MAX5171 UPO_LOW command")' 00226 // @Test='@test group UPO UPO_HIGH()' 00227 // @Test='@test group UPO tinyTester.Wait_Output_Settling()' 00228 // @Test='@test group CODE_LOAD tinyTester.DigitalIn_Read_Expect_WarnOnly(UPO_pin, "UPO", 1, "UPO_pin is high after MAX5171 UPO_HIGH command")' 00229 cmdLine.serial().printf("Init"); 00230 // call function Init 00231 g_MAX5171_device.Init(); 00232 return true; // command handled by MAX5171 00233 } // end case '!' 00234 break; 00235 case '0': 00236 { 00237 // test menu command '0' handler: 00238 // helpString='0 code=? -- CODE' 00239 // CMD_='CMD_00dd_dddd_dddd_dddd_CODE' 00240 // CommandName='CODE' 00241 // CommandParamIn='uint16_t dacCodeLsbs' 00242 // CommandReturnType='uint8_t' 00243 // @Pre='' 00244 // @Param[in]='' 00245 // @Param[out]='' 00246 // @Post='' 00247 // @Return='@return 1 on success; 0 on failure' 00248 // parse argument list 00249 // argument dacCodeLsbs alias code already parsed 00250 // print arguments 00251 cmdLine.serial().printf("CODE"); 00252 cmdLine.serial().printf(" dacCodeLsbs=%d", dacCodeLsbs); 00253 cmdLine.serial().printf("\r\n"); 00254 // call function CODE(dacCodeLsbs) 00255 uint8_t result = g_MAX5171_device.CODE(dacCodeLsbs); 00256 cmdLine.serial().printf(" =%d\r\n", result); 00257 return true; // command handled by MAX5171 00258 } // end case '0' 00259 break; 00260 case '4': 00261 { 00262 // test menu command '4' handler: 00263 // helpString='4 code=? -- CODE_LOAD' 00264 // CMD_='CMD_01dd_dddd_dddd_dddd_CODE_LOAD' 00265 // CommandName='CODE_LOAD' 00266 // CommandParamIn='uint16_t dacCodeLsbs' 00267 // CommandReturnType='uint8_t' 00268 // @Pre='' 00269 // @Param[in]='' 00270 // @Param[out]='' 00271 // @Post='' 00272 // @Return='@return 1 on success; 0 on failure' 00273 // parse argument list 00274 // argument dacCodeLsbs alias code already parsed 00275 // print arguments 00276 cmdLine.serial().printf("CODE_LOAD"); 00277 cmdLine.serial().printf(" dacCodeLsbs=%d", dacCodeLsbs); 00278 cmdLine.serial().printf("\r\n"); 00279 // call function CODE_LOAD(dacCodeLsbs) 00280 uint8_t result = g_MAX5171_device.CODE_LOAD(dacCodeLsbs); 00281 cmdLine.serial().printf(" =%d\r\n", result); 00282 return true; // command handled by MAX5171 00283 } // end case '4' 00284 break; 00285 case '8': 00286 { 00287 // test menu command '8' handler: 00288 // helpString='8 -- LOAD' 00289 // CMD_='CMD_10xx_xxxx_xxxx_xxxx_LOAD' 00290 // CommandName='LOAD' 00291 // CommandParamIn='void' 00292 // CommandReturnType='uint8_t' 00293 // @Pre='' 00294 // @Param[in]='' 00295 // @Param[out]='' 00296 // @Post='' 00297 // @Return='@return 1 on success; 0 on failure' 00298 cmdLine.serial().printf("LOAD"); 00299 // call function LOAD 00300 uint8_t result = g_MAX5171_device.LOAD(); 00301 cmdLine.serial().printf(" =%d\r\n", result); 00302 return true; // command handled by MAX5171 00303 } // end case '8' 00304 break; 00305 case 'c': 00306 { 00307 // test menu command 'c' handler: 00308 // helpString='c -- NOP' 00309 // CMD_='CMD_1100_xxxx_xxxx_xxxx_NOP' 00310 // CommandName='NOP' 00311 // CommandParamIn='void' 00312 // CommandReturnType='uint8_t' 00313 // @Pre='' 00314 // @Param[in]='' 00315 // @Param[out]='' 00316 // @Post='' 00317 // @Return='@return 1 on success; 0 on failure' 00318 cmdLine.serial().printf("NOP"); 00319 // call function NOP 00320 uint8_t result = g_MAX5171_device.NOP(); 00321 cmdLine.serial().printf(" =%d\r\n", result); 00322 return true; // command handled by MAX5171 00323 } // end case 'c' 00324 break; 00325 case 'd': 00326 { 00327 // test menu command 'd' handler: 00328 // helpString='d -- SHUTDOWN' 00329 // CMD_='CMD_1101_xxxx_xxxx_xxxx_SHUTDOWN' 00330 // CommandName='SHUTDOWN' 00331 // CommandParamIn='void' 00332 // CommandReturnType='uint8_t' 00333 // @Pre='' 00334 // @Param[in]='' 00335 // @Param[out]='' 00336 // @Post='' 00337 // @Return='@return 1 on success; 0 on failure' 00338 cmdLine.serial().printf("SHUTDOWN"); 00339 // call function SHUTDOWN 00340 uint8_t result = g_MAX5171_device.SHUTDOWN(); 00341 cmdLine.serial().printf(" =%d\r\n", result); 00342 return true; // command handled by MAX5171 00343 } // end case 'd' 00344 break; 00345 case 'e': // (multiple characters) (testMenuFirstCharHandler="e"): 00346 { 00347 switch (cmdLine[1]) 00348 { 00349 case '0': // (nested inside case 'e') 00350 { 00351 // test menu command 'e0' handler: 00352 // helpString='e0 -- UPO_LOW' 00353 // CMD_='CMD_1110_0xxx_xxxx_xxxx_UPO_LOW' 00354 // CommandName='UPO_LOW' 00355 // CommandParamIn='void' 00356 // CommandReturnType='uint8_t' 00357 // @Pre='' 00358 // @Param[in]='' 00359 // @Param[out]='' 00360 // @Post='' 00361 // @Return='@return 1 on success; 0 on failure' 00362 cmdLine.serial().printf("UPO_LOW"); 00363 // call function UPO_LOW 00364 uint8_t result = g_MAX5171_device.UPO_LOW(); 00365 cmdLine.serial().printf(" =%d\r\n", result); 00366 return true; // command handled by MAX5171 00367 } // end nested case 'e0' 00368 break; 00369 case '8': // (nested inside case 'e') 00370 { 00371 // test menu command 'e8' handler: 00372 // helpString='e8 -- UPO_HIGH' 00373 // CMD_='CMD_1110_1xxx_xxxx_xxxx_UPO_HIGH' 00374 // CommandName='UPO_HIGH' 00375 // CommandParamIn='void' 00376 // CommandReturnType='uint8_t' 00377 // @Pre='' 00378 // @Param[in]='' 00379 // @Param[out]='' 00380 // @Post='' 00381 // @Return='@return 1 on success; 0 on failure' 00382 cmdLine.serial().printf("UPO_HIGH"); 00383 // call function UPO_HIGH 00384 uint8_t result = g_MAX5171_device.UPO_HIGH(); 00385 cmdLine.serial().printf(" =%d\r\n", result); 00386 return true; // command handled by MAX5171 00387 } // end nested case 'e8' 00388 break; 00389 } // end nested switch (cmdLine[1]) inside case 'e' 00390 break; 00391 } // end case 'e' 00392 case 'f': // (multiple characters) (testMenuFirstCharHandler="f"): 00393 { 00394 switch (cmdLine[1]) 00395 { 00396 case '0': // (nested inside case 'f') 00397 { 00398 // test menu command 'f0' handler: 00399 // helpString='f0 -- MODE1_DOUT_SCLK_RISING_EDGE' 00400 // CMD_='CMD_1111_0xxx_xxxx_xxxx_MODE1_DOUT_SCLK_RISING_EDGE' 00401 // CommandName='MODE1_DOUT_SCLK_RISING_EDGE' 00402 // CommandParamIn='void' 00403 // CommandReturnType='uint8_t' 00404 // @Pre='' 00405 // @Param[in]='' 00406 // @Param[out]='' 00407 // @Post='' 00408 // @Return='@return 1 on success; 0 on failure' 00409 cmdLine.serial().printf("MODE1_DOUT_SCLK_RISING_EDGE"); 00410 // call function MODE1_DOUT_SCLK_RISING_EDGE 00411 uint8_t result = g_MAX5171_device.MODE1_DOUT_SCLK_RISING_EDGE(); 00412 cmdLine.serial().printf(" =%d\r\n", result); 00413 return true; // command handled by MAX5171 00414 } // end nested case 'f0' 00415 break; 00416 case '8': // (nested inside case 'f') 00417 { 00418 // test menu command 'f8' handler: 00419 // helpString='f8 -- MODE0_DOUT_SCLK_FALLING_EDGE' 00420 // CMD_='CMD_1111_1xxx_xxxx_xxxx_MODE0_DOUT_SCLK_FALLING_EDGE' 00421 // CommandName='MODE0_DOUT_SCLK_FALLING_EDGE' 00422 // CommandParamIn='void' 00423 // CommandReturnType='uint8_t' 00424 // @Pre='' 00425 // @Param[in]='' 00426 // @Param[out]='' 00427 // @Post='' 00428 // @Return='@return 1 on success; 0 on failure' 00429 cmdLine.serial().printf("MODE0_DOUT_SCLK_FALLING_EDGE"); 00430 // call function MODE0_DOUT_SCLK_FALLING_EDGE 00431 uint8_t result = g_MAX5171_device.MODE0_DOUT_SCLK_FALLING_EDGE(); 00432 cmdLine.serial().printf(" =%d\r\n", result); 00433 return true; // command handled by MAX5171 00434 } // end nested case 'f8' 00435 break; 00436 } // end nested switch (cmdLine[1]) inside case 'f' 00437 break; 00438 } // end case 'f' 00439 } // end switch (cmdLine[0]) 00440 return false; // command not handled by MAX5171 00441 } // end bool MAX5171_menu_onEOLcommandParser(CmdLine & cmdLine) 00442
Generated on Thu Jul 14 2022 04:42:56 by
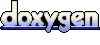