
Test program running on MAX32625MBED. Control through USB Serial commands using a terminal emulator such as teraterm or putty.
Dependencies: MaximTinyTester CmdLine MAX5171 USBDevice
Test_Main_MAX5171.cpp
00001 // /******************************************************************************* 00002 // * Copyright (C) 2020 Maxim Integrated Products, Inc., All Rights Reserved. 00003 // * 00004 // * Permission is hereby granted, free of charge, to any person obtaining a 00005 // * copy of this software and associated documentation files (the "Software"), 00006 // * to deal in the Software without restriction, including without limitation 00007 // * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 // * and/or sell copies of the Software, and to permit persons to whom the 00009 // * Software is furnished to do so, subject to the following conditions: 00010 // * 00011 // * The above copyright notice and this permission notice shall be included 00012 // * in all copies or substantial portions of the Software. 00013 // * 00014 // * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 // * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 // * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 // * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 // * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 // * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 // * OTHER DEALINGS IN THE SOFTWARE. 00021 // * 00022 // * Except as contained in this notice, the name of Maxim Integrated 00023 // * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 // * Products, Inc. Branding Policy. 00025 // * 00026 // * The mere transfer of this software does not imply any licenses 00027 // * of trade secrets, proprietary technology, copyrights, patents, 00028 // * trademarks, maskwork rights, or any other form of intellectual 00029 // * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 // * ownership rights. 00031 // ******************************************************************************* 00032 // */ 00033 // example code includes 00034 // standard include for target platform -- Platform_Include_Boilerplate 00035 #include "mbed.h" 00036 // Platforms: 00037 // - MAX32625MBED 00038 // - supports mbed-os-5.11, requires USBDevice library 00039 // - add https://developer.mbed.org/teams/MaximIntegrated/code/USBDevice/ 00040 // - remove max32630fthr library (if present) 00041 // - remove MAX32620FTHR library (if present) 00042 // - MAX32600MBED 00043 // - remove max32630fthr library (if present) 00044 // - remove MAX32620FTHR library (if present) 00045 // - Windows 10 note: Don't connect HDK until you are ready to load new firmware into the board. 00046 // - NUCLEO_F446RE 00047 // - remove USBDevice library 00048 // - remove max32630fthr library (if present) 00049 // - remove MAX32620FTHR library (if present) 00050 // - NUCLEO_F401RE 00051 // - remove USBDevice library 00052 // - remove max32630fthr library (if present) 00053 // - remove MAX32620FTHR library (if present) 00054 // - MAX32630FTHR 00055 // - #include "max32630fthr.h" 00056 // - add http://os.mbed.org/teams/MaximIntegrated/code/max32630fthr/ 00057 // - remove MAX32620FTHR library (if present) 00058 // - MAX32620FTHR 00059 // - #include "MAX32620FTHR.h" 00060 // - remove max32630fthr library (if present) 00061 // - add https://os.mbed.com/teams/MaximIntegrated/code/MAX32620FTHR/ 00062 // - not tested yet 00063 // - MAX32625PICO 00064 // - remove max32630fthr library (if present) 00065 // - remove MAX32620FTHR library (if present) 00066 // - not tested yet 00067 // 00068 // end Platform_Include_Boilerplate 00069 #include "MAX5171.h" 00070 #include "CmdLine.h" 00071 #include "MaximTinyTester.h" 00072 00073 // optional: serial port 00074 // note: some platforms such as Nucleo-F446RE do not support the USBSerial library. 00075 // In those cases, remove the USBDevice lib from the project and rebuild. 00076 #if defined(TARGET_MAX32625MBED) 00077 #include "USBSerial.h" 00078 USBSerial serial; // virtual serial port over USB (DEV connector) 00079 #elif defined(TARGET_MAX32600MBED) 00080 #include "USBSerial.h" 00081 USBSerial serial; // virtual serial port over USB (DEV connector) 00082 #elif defined(TARGET_MAX32630MBED) 00083 #include "USBSerial.h" 00084 USBSerial serial; // virtual serial port over USB (DEV connector) 00085 #else 00086 //#include "USBSerial.h" 00087 Serial serial(USBTX, USBRX); // tx, rx 00088 #endif 00089 00090 void on_immediate_0x21(); // Unicode (U+0021) ! EXCLAMATION MARK 00091 void on_immediate_0x7b(); // Unicode (U+007B) { LEFT CURLY BRACKET 00092 void on_immediate_0x7d(); // Unicode (U+007D) } RIGHT CURLY BRACKET 00093 00094 #include "CmdLine.h" 00095 00096 # if HAS_DAPLINK_SERIAL 00097 CmdLine cmdLine_DAPLINKserial(DAPLINKserial, "DAPLINK"); 00098 # endif // HAS_DAPLINK_SERIAL 00099 CmdLine cmdLine_serial(serial, "serial"); 00100 00101 00102 //-------------------------------------------------- 00103 00104 00105 #if defined(TARGET) 00106 // TARGET_NAME macros from targets/TARGET_Maxim/TARGET_MAX32625/device/mxc_device.h 00107 // Create a string definition for the TARGET 00108 #define STRING_ARG(arg) #arg 00109 #define STRING_NAME(name) STRING_ARG(name) 00110 #define TARGET_NAME STRING_NAME(TARGET) 00111 #elif defined(TARGET_MAX32600) 00112 #define TARGET_NAME "MAX32600" 00113 #elif defined(TARGET_LPC1768) 00114 #define TARGET_NAME "LPC1768" 00115 #elif defined(TARGET_NUCLEO_F446RE) 00116 #define TARGET_NAME "NUCLEO_F446RE" 00117 #elif defined(TARGET_NUCLEO_F401RE) 00118 #define TARGET_NAME "NUCLEO_F401RE" 00119 #else 00120 #error TARGET NOT DEFINED 00121 #endif 00122 #if defined(TARGET_MAX32630) 00123 //-------------------------------------------------- 00124 // TARGET=MAX32630FTHR ARM Cortex-M4F 96MHz 2048kB Flash 512kB SRAM 00125 // +-------------[microUSB]-------------+ 00126 // | J1 MAX32630FTHR J2 | 00127 // ______ | [ ] RST GND [ ] | 00128 // ______ | [ ] 3V3 BAT+[ ] | 00129 // ______ | [ ] 1V8 reset SW1 | 00130 // ______ | [ ] GND J4 J3 | 00131 // analogIn0/4 | [a] AIN_0 1.2Vfs (bat) SYS [ ] | switched BAT+ 00132 // analogIn1/5 | [a] AIN_1 1.2Vfs PWR [ ] | external pwr btn 00133 // analogIn2 | [a] AIN_2 1.2Vfs +5V VBUS [ ] | USB +5V power 00134 // analogIn3 | [a] AIN_3 1.2Vfs 1-WIRE P4_0 [d] | D0 dig9 00135 // (I2C2.SDA) | [d] P5_7 SDA2 SRN P5_6 [d] | D1 dig8 00136 // (I2C2.SCL) | [d] P6_0 SCL2 SDIO3 P5_5 [d] | D2 dig7 00137 // D13/SCLK | [s] P5_0 SCLK SDIO2 P5_4 [d] | D3 dig6 00138 // D11/MOSI | [s] P5_1 MOSI SSEL P5_3 [d] | D4 dig5 00139 // D12/MISO | [s] P5_2 MISO RTS P3_3 [d] | D5 dig4 00140 // D10/CS | [s] P3_0 RX CTS P3_2 [d] | D6 dig3 00141 // D9 dig0 | [d] P3_1 TX SCL P3_5 [d] | D7 dig2 00142 // ______ | [ ] GND SDA P3_4 [d] | D8 dig1 00143 // | | 00144 // | XIP Flash MAX14690N | 00145 // | XIP_SCLK P1_0 SDA2 P5_7 | 00146 // | XIP_MOSI P1_1 SCL2 P6_0 | 00147 // | XIP_MISO P1_2 PMIC_INIT P3_7 | 00148 // | XIP_SSEL P1_3 MPC P2_7 | 00149 // | XIP_DIO2 P1_4 MON AIN_0 | 00150 // | XIP_DIO3 P1_5 | 00151 // | | 00152 // | PAN1326B MicroSD LED | 00153 // | BT_RX P0_0 SD_SCLK P0_4 r P2_4 | 00154 // | BT_TX P0_1 SD_MOSI P0_5 g P2_5 | 00155 // | BT_CTS P0_2 SD_MISO P0_6 b P2_6 | 00156 // | BT_RTS P0_3 SD_SSEL P0_7 | 00157 // | BT_RST P1_6 DETECT P2_2 | 00158 // | BT_CLK P1_7 SW2 P2_3 | 00159 // +------------------------------------+ 00160 // MAX32630FTHR board has MAX14690 PMIC on I2C bus (P5_7 SDA, P6_0 SCL) at slave address 0101_000r 0x50 (or 0x28 for 7 MSbit address). 00161 // MAX32630FTHR board has BMI160 accelerometer on I2C bus (P5_7 SDA, P6_0 SCL) at slave address 1101_000r 0xD0 (or 0x68 for 7 MSbit address). 00162 // AIN_0 = AIN0 pin fullscale is 1.2V 00163 // AIN_1 = AIN1 pin fullscale is 1.2V 00164 // AIN_2 = AIN2 pin fullscale is 1.2V 00165 // AIN_3 = AIN3 pin fullscale is 1.2V 00166 // AIN_4 = AIN0 / 5.0 fullscale is 6.0V 00167 // AIN_5 = AIN1 / 5.0 fullscale is 6.0V 00168 // AIN_6 = VDDB / 4.0 fullscale is 4.8V 00169 // AIN_7 = VDD18 fullscale is 1.2V 00170 // AIN_8 = VDD12 fullscale is 1.2V 00171 // AIN_9 = VRTC / 2.0 fullscale is 2.4V 00172 // AIN_10 = x undefined? 00173 // AIN_11 = VDDIO / 4.0 fullscale is 4.8V 00174 // AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 00175 // 00176 #include "max32630fthr.h" 00177 MAX32630FTHR pegasus(MAX32630FTHR::VIO_3V3); 00178 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 1 00179 // MAX32630FTHR board supports only internal VREF = 1.200V at bypass capacitor C15 00180 const float ADC_FULL_SCALE_VOLTAGE = 1.200; 00181 // Arduino connector 00182 #ifndef A0 00183 #define A0 AIN_0 00184 #endif 00185 #ifndef A1 00186 #define A1 AIN_1 00187 #endif 00188 #ifndef A2 00189 #define A2 AIN_2 00190 #endif 00191 #ifndef A3 00192 #define A3 AIN_3 00193 #endif 00194 #ifndef D0 00195 #define D0 P4_0 00196 #endif 00197 #ifndef D1 00198 #define D1 P5_6 00199 #endif 00200 #ifndef D2 00201 #define D2 P5_5 00202 #endif 00203 #ifndef D3 00204 #define D3 P5_4 00205 #endif 00206 #ifndef D4 00207 #define D4 P5_3 00208 #endif 00209 #ifndef D5 00210 #define D5 P3_3 00211 #endif 00212 #ifndef D6 00213 #define D6 P3_2 00214 #endif 00215 #ifndef D7 00216 #define D7 P3_5 00217 #endif 00218 #ifndef D8 00219 #define D8 P3_4 00220 #endif 00221 #ifndef D9 00222 #define D9 P3_1 00223 #endif 00224 #ifndef D10 00225 #define D10 P3_0 00226 #endif 00227 #ifndef D11 00228 #define D11 P5_1 00229 #endif 00230 #ifndef D12 00231 #define D12 P5_2 00232 #endif 00233 #ifndef D13 00234 #define D13 P5_0 00235 #endif 00236 //-------------------------------------------------- 00237 #elif defined(TARGET_MAX32625MBED) 00238 //-------------------------------------------------- 00239 // TARGET=MAX32625MBED ARM Cortex-M4F 96MHz 512kB Flash 160kB SRAM 00240 // +-------------------------------------+ 00241 // | MAX32625MBED Arduino UNO header | 00242 // | | 00243 // | A5/SCL[ ] | P1_7 dig15 00244 // | A4/SDA[ ] | P1_6 dig14 00245 // | AREF=N/C[ ] | 00246 // | GND[ ] | 00247 // | [ ]N/C SCK/13[ ] | P1_0 dig13 00248 // | [ ]IOREF=3V3 MISO/12[ ] | P1_2 dig12 00249 // | [ ]RST MOSI/11[ ]~| P1_1 dig11 00250 // | [ ]3V3 CS/10[ ]~| P1_3 dig10 00251 // | [ ]5V0 9[ ]~| P1_5 dig9 00252 // | [ ]GND 8[ ] | P1_4 dig8 00253 // | [ ]GND | 00254 // | [ ]Vin 7[ ] | P0_7 dig7 00255 // | 6[ ]~| P0_6 dig6 00256 // AIN_0 | [ ]A0 5[ ]~| P0_5 dig5 00257 // AIN_1 | [ ]A1 4[ ] | P0_4 dig4 00258 // AIN_2 | [ ]A2 INT1/3[ ]~| P0_3 dig3 00259 // AIN_3 | [ ]A3 INT0/2[ ] | P0_2 dig2 00260 // dig16 P3_4 | [ ]A4/SDA RST SCK MISO TX>1[ ] | P0_1 dig1 00261 // dig17 P3_5 | [ ]A5/SCL [ ] [ ] [ ] RX<0[ ] | P0_0 dig0 00262 // | [ ] [ ] [ ] | 00263 // | UNO_R3 GND MOSI 5V ____________/ 00264 // \_______________________/ 00265 // 00266 // +------------------------+ 00267 // | | 00268 // | MicroSD LED | 00269 // | SD_SCLK P2_4 r P3_0 | 00270 // | SD_MOSI P2_5 g P3_1 | 00271 // | SD_MISO P2_6 b P3_2 | 00272 // | SD_SSEL P2_7 y P3_3 | 00273 // | | 00274 // | DAPLINK BUTTONS | 00275 // | TX P2_1 SW3 P2_3 | 00276 // | RX P2_0 SW2 P2_2 | 00277 // +------------------------+ 00278 // 00279 // AIN_0 = AIN0 pin fullscale is 1.2V 00280 // AIN_1 = AIN1 pin fullscale is 1.2V 00281 // AIN_2 = AIN2 pin fullscale is 1.2V 00282 // AIN_3 = AIN3 pin fullscale is 1.2V 00283 // AIN_4 = AIN0 / 5.0 fullscale is 6.0V 00284 // AIN_5 = AIN1 / 5.0 fullscale is 6.0V 00285 // AIN_6 = VDDB / 4.0 fullscale is 4.8V 00286 // AIN_7 = VDD18 fullscale is 1.2V 00287 // AIN_8 = VDD12 fullscale is 1.2V 00288 // AIN_9 = VRTC / 2.0 fullscale is 2.4V 00289 // AIN_10 = x undefined? 00290 // AIN_11 = VDDIO / 4.0 fullscale is 4.8V 00291 // AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 00292 // 00293 //#include "max32625mbed.h" // ? 00294 //MAX32625MBED mbed(MAX32625MBED::VIO_3V3); // ? 00295 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 1 00296 // MAX32630FTHR board supports only internal VREF = 1.200V at bypass capacitor C15 00297 const float ADC_FULL_SCALE_VOLTAGE = 1.200; // TODO: ADC_FULL_SCALE_VOLTAGE Pico? 00298 // Arduino connector 00299 #ifndef A0 00300 #define A0 AIN_0 00301 #endif 00302 #ifndef A1 00303 #define A1 AIN_1 00304 #endif 00305 #ifndef A2 00306 #define A2 AIN_2 00307 #endif 00308 #ifndef A3 00309 #define A3 AIN_3 00310 #endif 00311 #ifndef D0 00312 #define D0 P0_0 00313 #endif 00314 #ifndef D1 00315 #define D1 P0_1 00316 #endif 00317 #ifndef D2 00318 #define D2 P0_2 00319 #endif 00320 #ifndef D3 00321 #define D3 P0_3 00322 #endif 00323 #ifndef D4 00324 #define D4 P0_4 00325 #endif 00326 #ifndef D5 00327 #define D5 P0_5 00328 #endif 00329 #ifndef D6 00330 #define D6 P0_6 00331 #endif 00332 #ifndef D7 00333 #define D7 P0_7 00334 #endif 00335 #ifndef D8 00336 #define D8 P1_4 00337 #endif 00338 #ifndef D9 00339 #define D9 P1_5 00340 #endif 00341 #ifndef D10 00342 #define D10 P1_3 00343 #endif 00344 #ifndef D11 00345 #define D11 P1_1 00346 #endif 00347 #ifndef D12 00348 #define D12 P1_2 00349 #endif 00350 #ifndef D13 00351 #define D13 P1_0 00352 #endif 00353 //-------------------------------------------------- 00354 #elif defined(TARGET_MAX32600) 00355 // target MAX32600 00356 // 00357 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 0 00358 const float ADC_FULL_SCALE_VOLTAGE = 1.500; 00359 // 00360 //-------------------------------------------------- 00361 #elif defined(TARGET_MAX32620FTHR) 00362 #warning "TARGET_MAX32620FTHR not previously tested; need to define pins..." 00363 #include "MAX32620FTHR.h" 00364 // Initialize I/O voltages on MAX32620FTHR board 00365 MAX32620FTHR fthr(MAX32620FTHR::VIO_3V3); 00366 //#define USE_LEDS 0 ? 00367 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 1 00368 #warning "TARGET_MAX32620FTHR not previously tested; need to verify ADC_FULL_SCALE_VOLTAGE..." 00369 const float ADC_FULL_SCALE_VOLTAGE = 1.200; 00370 // 00371 //-------------------------------------------------- 00372 #elif defined(TARGET_MAX32625PICO) 00373 #warning "TARGET_MAX32625PICO not previously tested; need to define pins..." 00374 //#define USE_LEDS 0 ? 00375 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 1 00376 #warning "TARGET_MAX32625PICO not previously tested; need to verify ADC_FULL_SCALE_VOLTAGE..." 00377 const float ADC_FULL_SCALE_VOLTAGE = 1.200; 00378 // 00379 //-------------------------------------------------- 00380 #elif defined(TARGET_NUCLEO_F446RE) || defined(TARGET_NUCLEO_F401RE) 00381 // TODO1: target NUCLEO_F446RE 00382 // 00383 // USER_BUTTON PC13 00384 // LED1 is shared with SPI_SCK on NUCLEO_F446RE PA_5, so don't use LED1. 00385 #define USE_LEDS 0 00386 // SPI spi(SPI_MOSI, SPI_MISO, SPI_SCK); 00387 // Serial serial(SERIAL_TX, SERIAL_RX); 00388 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 0 00389 const float ADC_FULL_SCALE_VOLTAGE = 3.300; // TODO: ADC_FULL_SCALE_VOLTAGE Pico? 00390 // 00391 //-------------------------------------------------- 00392 #elif defined(TARGET_LPC1768) 00393 //-------------------------------------------------- 00394 // TARGET=LPC1768 ARM Cortex-M3 100 MHz 512kB flash 64kB SRAM 00395 // +-------------[microUSB]-------------+ 00396 // ______ | [ ] GND +3.3V VOUT [ ] | ______ 00397 // ______ | [ ] 4.5V<VIN<9.0V +5.0V VU [ ] | ______ 00398 // ______ | [ ] VB USB.IF- [ ] | ______ 00399 // ______ | [ ] nR USB.IF+ [ ] | ______ 00400 // digitalInOut0 | [ ] p5 MOSI ETHERNET.RD- [ ] | ______ 00401 // digitalInOut1 | [ ] p6 MISO ETHERNET.RD+ [ ] | ______ 00402 // digitalInOut2 | [ ] p7 SCLK ETHERNET.TD- [ ] | ______ 00403 // digitalInOut3 | [ ] p8 ETHERNET.TD+ [ ] | ______ 00404 // digitalInOut4 | [ ] p9 TX SDA USB.D- [ ] | ______ 00405 // digitalInOut5 | [ ] p10 RX SCL USB.D+ [ ] | ______ 00406 // digitalInOut6 | [ ] p11 MOSI CAN-RD p30 [ ] | digitalInOut13 00407 // digitalInOut7 | [ ] p12 MISO CAN-TD p29 [ ] | digitalInOut12 00408 // digitalInOut8 | [ ] p13 TX SCLK SDA TX p28 [ ] | digitalInOut11 00409 // digitalInOut9 | [ ] p14 RX SCL RX p27 [ ] | digitalInOut10 00410 // analogIn0 | [ ] p15 AIN0 3.3Vfs PWM1 p26 [ ] | pwmDriver1 00411 // analogIn1 | [ ] p16 AIN1 3.3Vfs PWM2 p25 [ ] | pwmDriver2 00412 // analogIn2 | [ ] p17 AIN2 3.3Vfs PWM3 p24 [ ] | pwmDriver3 00413 // analogIn3 | [ ] p18 AIN3 AOUT PWM4 p23 [ ] | pwmDriver4 00414 // analogIn4 | [ ] p19 AIN4 3.3Vfs PWM5 p22 [ ] | pwmDriver5 00415 // analogIn5 | [ ] p20 AIN5 3.3Vfs PWM6 p21 [ ] | pwmDriver6 00416 // +------------------------------------+ 00417 // AIN6 = P0.3 = TGT_SBL_RXD? 00418 // AIN7 = P0.2 = TGT_SBL_TXD? 00419 // 00420 //-------------------------------------------------- 00421 // LPC1768 board uses VREF = 3.300V +A3,3V thru L1 to bypass capacitor C14 00422 #define analogIn4_IS_HIGH_RANGE_OF_analogIn0 0 00423 const float ADC_FULL_SCALE_VOLTAGE = 3.300; 00424 #else // not defined(TARGET_LPC1768 etc.) 00425 //-------------------------------------------------- 00426 // unknown target 00427 //-------------------------------------------------- 00428 #endif // target definition 00429 00430 00431 //-------------------------------------------------- 00432 // Option to dedicate SPI port pins 00433 // 00434 // SPI2_MOSI = P5_1 00435 // SPI2_MISO = P5_2 00436 // SPI2_SCK = P5_0 00437 // On this board I'm using P3_0 as spi_cs 00438 // SPI2_SS = P5_3 00439 // SPI2_SDIO2 = P5_4 00440 // SPI2_SDIO3 = P5_5 00441 // SPI2_SRN = P5_6 00442 // 00443 #ifndef HAS_SPI 00444 #define HAS_SPI 1 00445 #endif 00446 #if HAS_SPI 00447 #define SPI_MODE0 0 00448 #define SPI_MODE1 1 00449 #define SPI_MODE2 2 00450 #define SPI_MODE3 3 00451 // 00452 #if defined(TARGET_MAX32630) 00453 // Before setting global variables g_SPI_SCLK_Hz and g_SPI_dataMode, 00454 // workaround for TARGET_MAX32630 SPI_MODE2 SPI_MODE3 problem (issue #30) 00455 #warning "MAX32630 SPI workaround..." 00456 // replace SPI_MODE2 (CPOL=1,CPHA=0) with SPI_MODE1 (CPOL=0,CPHA=1) Falling Edge stable 00457 // replace SPI_MODE3 (CPOL=1,CPHA=1) with SPI_MODE0 (CPOL=0,CPHA=0) Rising Edge stable 00458 # if ((SPI_dataMode) == (SPI_MODE2)) 00459 #warning "MAX32630 SPI_MODE2 workaround, changing SPI_dataMode to SPI_MODE1..." 00460 // SPI_dataMode SPI_MODE2 // CPOL=1,CPHA=0: Falling Edge stable; SCLK idle High 00461 # undef SPI_dataMode 00462 # define SPI_dataMode SPI_MODE1 // CPOL=0,CPHA=1: Falling Edge stable; SCLK idle Low 00463 # elif ((SPI_dataMode) == (SPI_MODE3)) 00464 #warning "MAX32630 SPI_MODE3 workaround, changing SPI_dataMode to SPI_MODE0..." 00465 // SPI_dataMode SPI_MODE3 // CPOL=1,CPHA=1: Rising Edge stable; SCLK idle High 00466 # undef SPI_dataMode 00467 # define SPI_dataMode SPI_MODE0 // CPOL=0,CPHA=0: Rising Edge stable; SCLK idle Low 00468 # endif // workaround for TARGET_MAX32630 SPI_MODE2 SPI_MODE3 problem 00469 // workaround for TARGET_MAX32630 SPI_MODE2 SPI_MODE3 problem (issue #30) 00470 // limit SPI SCLK speed to 6MHz or less 00471 # if ((SPI_SCLK_Hz) > (6000000)) 00472 #warning "MAX32630 SPI speed workaround, changing SPI_SCLK_Hz to 6000000 or 6MHz..." 00473 # undef SPI_SCLK_Hz 00474 # define SPI_SCLK_Hz 6000000 // 6MHz 00475 # endif 00476 #endif 00477 // 00478 uint32_t g_SPI_SCLK_Hz = 24000000; // platform limit 24MHz intSPI_SCLK_Platform_Max_MHz * 1000000 00479 // TODO1: validate g_SPI_SCLK_Hz against system clock frequency SystemCoreClock F_CPU 00480 #if defined(TARGET_NUCLEO_F446RE) || defined(TARGET_NUCLEO_F401RE) 00481 // Nucleo SPI frequency isn't working quite as expected... 00482 // Looks like STMF4 has an spi clock prescaler (2,4,8,16,32,64,128,256) 00483 // so 180MHz->[90.0, 45.0, 22.5, 11.25, 5.625, 2.8125, 1.40625, 0.703125] 00484 // %SC SCLK=1MHz sets spi frequency 703.125kHz 00485 // %SC SCLK=2MHz sets spi frequency 1.40625MHz 00486 // %SC SCLK=3MHz sets spi frequency 2.8125MHz 00487 // %SC SCLK=6MHz sets spi frequency 5.625MHz 00488 // %SC SCLK=12MHz sets spi frequency 11.25MHz 00489 // %SC SCLK=23MHz sets spi frequency 22.5MHz 00490 // %SC SCLK=45MHz sets spi frequency 45.0MHz 00491 // Don't know why I can't reach spi frequency 90.0MHz, but ok whatever. 00492 const uint32_t limit_min_SPI_SCLK_divisor = 2; 00493 const uint32_t limit_max_SPI_SCLK_divisor = 256; 00494 // not really a divisor, just a powers-of-two prescaler with no intermediate divisors. 00495 #else 00496 const uint32_t limit_min_SPI_SCLK_divisor = 2; 00497 const uint32_t limit_max_SPI_SCLK_divisor = 8191; 00498 #endif 00499 const uint32_t limit_max_SPI_SCLK_Hz = (SystemCoreClock / limit_min_SPI_SCLK_divisor); // F_CPU / 2; // 8MHz / 2 = 4MHz 00500 const uint32_t limit_min_SPI_SCLK_Hz = (SystemCoreClock / limit_max_SPI_SCLK_divisor); // F_CPU / 128; // 8MHz / 128 = 62.5kHz 00501 // 00502 uint8_t g_SPI_dataMode = SPI_MODE0; // TODO: missing definition SPI_dataMode; 00503 uint8_t g_SPI_cs_state = 1; 00504 // 00505 #endif 00506 00507 00508 // uncrustify-0.66.1 *INDENT-OFF* 00509 //-------------------------------------------------- 00510 // Declare the DigitalInOut GPIO pins 00511 // Optional digitalInOut support. If there is only one it should be digitalInOut1. 00512 // D) Digital High/Low/Input Pin 00513 #if defined(TARGET_MAX32630) 00514 // +-------------[microUSB]-------------+ 00515 // | J1 MAX32630FTHR J2 | 00516 // | [ ] RST GND [ ] | 00517 // | [ ] 3V3 BAT+[ ] | 00518 // | [ ] 1V8 reset SW1 | 00519 // | [ ] GND J4 J3 | 00520 // | [ ] AIN_0 1.2Vfs (bat) SYS [ ] | 00521 // | [ ] AIN_1 1.2Vfs PWR [ ] | 00522 // | [ ] AIN_2 1.2Vfs +5V VBUS [ ] | 00523 // | [ ] AIN_3 1.2Vfs 1-WIRE P4_0 [ ] | dig9 00524 // dig10 | [x] P5_7 SDA2 SRN P5_6 [ ] | dig8 00525 // dig11 | [x] P6_0 SCL2 SDIO3 P5_5 [ ] | dig7 00526 // dig12 | [x] P5_0 SCLK SDIO2 P5_4 [ ] | dig6 00527 // dig13 | [x] P5_1 MOSI SSEL P5_3 [x] | dig5 00528 // dig14 | [ ] P5_2 MISO RTS P3_3 [ ] | dig4 00529 // dig15 | [ ] P3_0 RX CTS P3_2 [ ] | dig3 00530 // dig0 | [ ] P3_1 TX SCL P3_5 [x] | dig2 00531 // | [ ] GND SDA P3_4 [x] | dig1 00532 // +------------------------------------+ 00533 #define HAS_digitalInOut0 1 // P3_1 TARGET_MAX32630 J1.15 00534 #define HAS_digitalInOut1 1 // P3_4 TARGET_MAX32630 J3.12 00535 #define HAS_digitalInOut2 1 // P3_5 TARGET_MAX32630 J3.11 00536 #define HAS_digitalInOut3 1 // P3_2 TARGET_MAX32630 J3.10 00537 #define HAS_digitalInOut4 1 // P3_3 TARGET_MAX32630 J3.9 00538 #define HAS_digitalInOut5 1 // P5_3 TARGET_MAX32630 J3.8 00539 #define HAS_digitalInOut6 1 // P5_4 TARGET_MAX32630 J3.7 00540 #define HAS_digitalInOut7 1 // P5_5 TARGET_MAX32630 J3.6 00541 #define HAS_digitalInOut8 1 // P5_6 TARGET_MAX32630 J3.5 00542 #define HAS_digitalInOut9 1 // P4_0 TARGET_MAX32630 J3.4 00543 #if HAS_I2C 00544 // avoid resource conflict between P5_7, P6_0 I2C and DigitalInOut 00545 #define HAS_digitalInOut10 0 // P5_7 TARGET_MAX32630 J1.9 00546 #define HAS_digitalInOut11 0 // P6_0 TARGET_MAX32630 J1.10 00547 #else // HAS_I2C 00548 #define HAS_digitalInOut10 1 // P5_7 TARGET_MAX32630 J1.9 00549 #define HAS_digitalInOut11 1 // P6_0 TARGET_MAX32630 J1.10 00550 #endif // HAS_I2C 00551 #if HAS_SPI 00552 // avoid resource conflict between P5_0, P5_1, P5_2 SPI and DigitalInOut 00553 #define HAS_digitalInOut12 0 // P5_0 TARGET_MAX32630 J1.11 00554 #define HAS_digitalInOut13 0 // P5_1 TARGET_MAX32630 J1.12 00555 #define HAS_digitalInOut14 0 // P5_2 TARGET_MAX32630 J1.13 00556 #define HAS_digitalInOut15 0 // P3_0 TARGET_MAX32630 J1.14 00557 #else // HAS_SPI 00558 #define HAS_digitalInOut12 1 // P5_0 TARGET_MAX32630 J1.11 00559 #define HAS_digitalInOut13 1 // P5_1 TARGET_MAX32630 J1.12 00560 #define HAS_digitalInOut14 1 // P5_2 TARGET_MAX32630 J1.13 00561 #define HAS_digitalInOut15 1 // P3_0 TARGET_MAX32630 J1.14 00562 #endif // HAS_SPI 00563 #if HAS_digitalInOut0 00564 DigitalInOut digitalInOut0(P3_1, PIN_INPUT, PullUp, 1); // P3_1 TARGET_MAX32630 J1.15 00565 #endif 00566 #if HAS_digitalInOut1 00567 DigitalInOut digitalInOut1(P3_4, PIN_INPUT, PullUp, 1); // P3_4 TARGET_MAX32630 J3.12 00568 #endif 00569 #if HAS_digitalInOut2 00570 DigitalInOut digitalInOut2(P3_5, PIN_INPUT, PullUp, 1); // P3_5 TARGET_MAX32630 J3.11 00571 #endif 00572 #if HAS_digitalInOut3 00573 DigitalInOut digitalInOut3(P3_2, PIN_INPUT, PullUp, 1); // P3_2 TARGET_MAX32630 J3.10 00574 #endif 00575 #if HAS_digitalInOut4 00576 DigitalInOut digitalInOut4(P3_3, PIN_INPUT, PullUp, 1); // P3_3 TARGET_MAX32630 J3.9 00577 #endif 00578 #if HAS_digitalInOut5 00579 DigitalInOut digitalInOut5(P5_3, PIN_INPUT, PullUp, 1); // P5_3 TARGET_MAX32630 J3.8 00580 #endif 00581 #if HAS_digitalInOut6 00582 DigitalInOut digitalInOut6(P5_4, PIN_INPUT, PullUp, 1); // P5_4 TARGET_MAX32630 J3.7 00583 #endif 00584 #if HAS_digitalInOut7 00585 DigitalInOut digitalInOut7(P5_5, PIN_INPUT, PullUp, 1); // P5_5 TARGET_MAX32630 J3.6 00586 #endif 00587 #if HAS_digitalInOut8 00588 DigitalInOut digitalInOut8(P5_6, PIN_INPUT, PullUp, 1); // P5_6 TARGET_MAX32630 J3.5 00589 #endif 00590 #if HAS_digitalInOut9 00591 DigitalInOut digitalInOut9(P4_0, PIN_INPUT, PullUp, 1); // P4_0 TARGET_MAX32630 J3.4 00592 #endif 00593 #if HAS_digitalInOut10 00594 DigitalInOut digitalInOut10(P5_7, PIN_INPUT, PullUp, 1); // P5_7 TARGET_MAX32630 J1.9 00595 #endif 00596 #if HAS_digitalInOut11 00597 DigitalInOut digitalInOut11(P6_0, PIN_INPUT, PullUp, 1); // P6_0 TARGET_MAX32630 J1.10 00598 #endif 00599 #if HAS_digitalInOut12 00600 DigitalInOut digitalInOut12(P5_0, PIN_INPUT, PullUp, 1); // P5_0 TARGET_MAX32630 J1.11 00601 #endif 00602 #if HAS_digitalInOut13 00603 DigitalInOut digitalInOut13(P5_1, PIN_INPUT, PullUp, 1); // P5_1 TARGET_MAX32630 J1.12 00604 #endif 00605 #if HAS_digitalInOut14 00606 DigitalInOut digitalInOut14(P5_2, PIN_INPUT, PullUp, 1); // P5_2 TARGET_MAX32630 J1.13 00607 #endif 00608 #if HAS_digitalInOut15 00609 DigitalInOut digitalInOut15(P3_0, PIN_INPUT, PullUp, 1); // P3_0 TARGET_MAX32630 J1.14 00610 #endif 00611 //-------------------------------------------------- 00612 #elif defined(TARGET_MAX32625MBED) 00613 // TARGET=MAX32625MBED ARM Cortex-M4F 96MHz 512kB Flash 160kB SRAM 00614 // +-------------------------------------+ 00615 // | MAX32625MBED Arduino UNO header | 00616 // | | 00617 // | A5/SCL[ ] | P1_7 dig15 00618 // | A4/SDA[ ] | P1_6 dig14 00619 // | AREF=N/C[ ] | 00620 // | GND[ ] | 00621 // | [ ]N/C SCK/13[ ] | P1_0 dig13 00622 // | [ ]IOREF=3V3 MISO/12[ ] | P1_2 dig12 00623 // | [ ]RST MOSI/11[ ]~| P1_1 dig11 00624 // | [ ]3V3 CS/10[ ]~| P1_3 dig10 00625 // | [ ]5V0 9[ ]~| P1_5 dig9 00626 // | [ ]GND 8[ ] | P1_4 dig8 00627 // | [ ]GND | 00628 // | [ ]Vin 7[ ] | P0_7 dig7 00629 // | 6[ ]~| P0_6 dig6 00630 // AIN_0 | [ ]A0 5[ ]~| P0_5 dig5 00631 // AIN_1 | [ ]A1 4[ ] | P0_4 dig4 00632 // AIN_2 | [ ]A2 INT1/3[ ]~| P0_3 dig3 00633 // AIN_3 | [ ]A3 INT0/2[ ] | P0_2 dig2 00634 // dig16 P3_4 | [ ]A4/SDA RST SCK MISO TX>1[ ] | P0_1 dig1 00635 // dig17 P3_5 | [ ]A5/SCL [ ] [ ] [ ] RX<0[ ] | P0_0 dig0 00636 // | [ ] [ ] [ ] | 00637 // | UNO_R3 GND MOSI 5V ____________/ 00638 // \_______________________/ 00639 // 00640 #define HAS_digitalInOut0 1 // P0_0 TARGET_MAX32625MBED D0 00641 #define HAS_digitalInOut1 1 // P0_1 TARGET_MAX32625MBED D1 00642 #if APPLICATION_MAX11131 00643 #define HAS_digitalInOut2 0 // P0_2 TARGET_MAX32625MBED D2 -- MAX11131 EOC DigitalIn 00644 #else 00645 #define HAS_digitalInOut2 1 // P0_2 TARGET_MAX32625MBED D2 00646 #endif 00647 #define HAS_digitalInOut3 1 // P0_3 TARGET_MAX32625MBED D3 00648 #define HAS_digitalInOut4 1 // P0_4 TARGET_MAX32625MBED D4 00649 #define HAS_digitalInOut5 1 // P0_5 TARGET_MAX32625MBED D5 00650 #define HAS_digitalInOut6 1 // P0_6 TARGET_MAX32625MBED D6 00651 #define HAS_digitalInOut7 1 // P0_7 TARGET_MAX32625MBED D7 00652 #define HAS_digitalInOut8 1 // P1_4 TARGET_MAX32625MBED D8 00653 #if APPLICATION_MAX11131 00654 #define HAS_digitalInOut9 0 // P1_5 TARGET_MAX32625MBED D9 -- MAX11131 CNVST DigitalOut 00655 #else 00656 #define HAS_digitalInOut9 1 // P1_5 TARGET_MAX32625MBED D9 00657 #endif 00658 #if HAS_SPI 00659 // avoid resource conflict between P5_0, P5_1, P5_2 SPI and DigitalInOut 00660 #define HAS_digitalInOut10 0 // P1_3 TARGET_MAX32635MBED CS/10 00661 #define HAS_digitalInOut11 0 // P1_1 TARGET_MAX32635MBED MOSI/11 00662 #define HAS_digitalInOut12 0 // P1_2 TARGET_MAX32635MBED MISO/12 00663 #define HAS_digitalInOut13 0 // P1_0 TARGET_MAX32635MBED SCK/13 00664 #else // HAS_SPI 00665 #define HAS_digitalInOut10 1 // P1_3 TARGET_MAX32635MBED CS/10 00666 #define HAS_digitalInOut11 1 // P1_1 TARGET_MAX32635MBED MOSI/11 00667 #define HAS_digitalInOut12 1 // P1_2 TARGET_MAX32635MBED MISO/12 00668 #define HAS_digitalInOut13 1 // P1_0 TARGET_MAX32635MBED SCK/13 00669 #endif // HAS_SPI 00670 #if HAS_I2C 00671 // avoid resource conflict between P5_7, P6_0 I2C and DigitalInOut 00672 #define HAS_digitalInOut14 0 // P1_6 TARGET_MAX32635MBED A4/SDA (10pin digital connector) 00673 #define HAS_digitalInOut15 0 // P1_7 TARGET_MAX32635MBED A5/SCL (10pin digital connector) 00674 #define HAS_digitalInOut16 0 // P3_4 TARGET_MAX32635MBED A4/SDA (6pin analog connector) 00675 #define HAS_digitalInOut17 0 // P3_5 TARGET_MAX32635MBED A5/SCL (6pin analog connector) 00676 #else // HAS_I2C 00677 #define HAS_digitalInOut14 1 // P1_6 TARGET_MAX32635MBED A4/SDA (10pin digital connector) 00678 #define HAS_digitalInOut15 1 // P1_7 TARGET_MAX32635MBED A5/SCL (10pin digital connector) 00679 #define HAS_digitalInOut16 1 // P3_4 TARGET_MAX32635MBED A4/SDA (6pin analog connector) 00680 #define HAS_digitalInOut17 1 // P3_5 TARGET_MAX32635MBED A5/SCL (6pin analog connector) 00681 #endif // HAS_I2C 00682 #if HAS_digitalInOut0 00683 DigitalInOut digitalInOut0(P0_0, PIN_INPUT, PullUp, 1); // P0_0 TARGET_MAX32625MBED D0 00684 #endif 00685 #if HAS_digitalInOut1 00686 DigitalInOut digitalInOut1(P0_1, PIN_INPUT, PullUp, 1); // P0_1 TARGET_MAX32625MBED D1 00687 #endif 00688 #if HAS_digitalInOut2 00689 DigitalInOut digitalInOut2(P0_2, PIN_INPUT, PullUp, 1); // P0_2 TARGET_MAX32625MBED D2 00690 #endif 00691 #if HAS_digitalInOut3 00692 DigitalInOut digitalInOut3(P0_3, PIN_INPUT, PullUp, 1); // P0_3 TARGET_MAX32625MBED D3 00693 #endif 00694 #if HAS_digitalInOut4 00695 DigitalInOut digitalInOut4(P0_4, PIN_INPUT, PullUp, 1); // P0_4 TARGET_MAX32625MBED D4 00696 #endif 00697 #if HAS_digitalInOut5 00698 DigitalInOut digitalInOut5(P0_5, PIN_INPUT, PullUp, 1); // P0_5 TARGET_MAX32625MBED D5 00699 #endif 00700 #if HAS_digitalInOut6 00701 DigitalInOut digitalInOut6(P0_6, PIN_INPUT, PullUp, 1); // P0_6 TARGET_MAX32625MBED D6 00702 #endif 00703 #if HAS_digitalInOut7 00704 DigitalInOut digitalInOut7(P0_7, PIN_INPUT, PullUp, 1); // P0_7 TARGET_MAX32625MBED D7 00705 #endif 00706 #if HAS_digitalInOut8 00707 DigitalInOut digitalInOut8(P1_4, PIN_INPUT, PullUp, 1); // P1_4 TARGET_MAX32625MBED D8 00708 #endif 00709 #if HAS_digitalInOut9 00710 DigitalInOut digitalInOut9(P1_5, PIN_INPUT, PullUp, 1); // P1_5 TARGET_MAX32625MBED D9 00711 #endif 00712 #if HAS_digitalInOut10 00713 DigitalInOut digitalInOut10(P1_3, PIN_INPUT, PullUp, 1); // P1_3 TARGET_MAX32635MBED CS/10 00714 #endif 00715 #if HAS_digitalInOut11 00716 DigitalInOut digitalInOut11(P1_1, PIN_INPUT, PullUp, 1); // P1_1 TARGET_MAX32635MBED MOSI/11 00717 #endif 00718 #if HAS_digitalInOut12 00719 DigitalInOut digitalInOut12(P1_2, PIN_INPUT, PullUp, 1); // P1_2 TARGET_MAX32635MBED MISO/12 00720 #endif 00721 #if HAS_digitalInOut13 00722 DigitalInOut digitalInOut13(P1_0, PIN_INPUT, PullUp, 1); // P1_0 TARGET_MAX32635MBED SCK/13 00723 #endif 00724 #if HAS_digitalInOut14 00725 // Ensure that the unused I2C pins do not interfere with analog inputs A4 and A5 00726 // DigitalInOut mode can be one of PullUp, PullDown, PullNone, OpenDrain 00727 DigitalInOut digitalInOut14(P1_6, PIN_INPUT, OpenDrain, 1); // P1_6 TARGET_MAX32635MBED A4/SDA (10pin digital connector) 00728 #endif 00729 #if HAS_digitalInOut15 00730 // Ensure that the unused I2C pins do not interfere with analog inputs A4 and A5 00731 DigitalInOut digitalInOut15(P1_7, PIN_INPUT, OpenDrain, 1); // P1_7 TARGET_MAX32635MBED A5/SCL (10pin digital connector) 00732 #endif 00733 #if HAS_digitalInOut16 00734 // Ensure that the unused I2C pins do not interfere with analog inputs A4 and A5 00735 // DigitalInOut mode can be one of PullUp, PullDown, PullNone, OpenDrain 00736 // PullUp-->3.4V, PullDown-->1.7V, PullNone-->3.5V, OpenDrain-->0.00V 00737 DigitalInOut digitalInOut16(P3_4, PIN_INPUT, OpenDrain, 0); // P3_4 TARGET_MAX32635MBED A4/SDA (6pin analog connector) 00738 #endif 00739 #if HAS_digitalInOut17 00740 // Ensure that the unused I2C pins do not interfere with analog inputs A4 and A5 00741 DigitalInOut digitalInOut17(P3_5, PIN_INPUT, OpenDrain, 0); // P3_5 TARGET_MAX32635MBED A5/SCL (6pin analog connector) 00742 #endif 00743 //-------------------------------------------------- 00744 #elif defined(TARGET_NUCLEO_F446RE) || defined(TARGET_NUCLEO_F401RE) 00745 #define HAS_digitalInOut0 0 00746 #define HAS_digitalInOut1 0 00747 #if APPLICATION_MAX11131 00748 // D2 -- MAX11131 EOC DigitalIn 00749 #define HAS_digitalInOut2 0 00750 #else 00751 #define HAS_digitalInOut2 1 00752 #endif 00753 #define HAS_digitalInOut3 1 00754 #define HAS_digitalInOut4 1 00755 #define HAS_digitalInOut5 1 00756 #define HAS_digitalInOut6 1 00757 #define HAS_digitalInOut7 1 00758 #if APPLICATION_MAX5715 00759 // D8 -- MAX5715 CLRb DigitalOut 00760 #define HAS_digitalInOut8 0 00761 #else 00762 #define HAS_digitalInOut8 1 00763 #endif 00764 #if APPLICATION_MAX5715 00765 // D9 -- MAX5715 LDACb DigitalOut 00766 #define HAS_digitalInOut9 0 00767 #elif APPLICATION_MAX11131 00768 // D9 -- MAX11131 CNVST DigitalOut 00769 #define HAS_digitalInOut9 0 00770 #else 00771 #define HAS_digitalInOut9 1 00772 #endif 00773 #if HAS_SPI 00774 // avoid resource conflict between P5_0, P5_1, P5_2 SPI and DigitalInOut 00775 // Arduino digital pin D10 SPI function is CS/10 00776 // Arduino digital pin D11 SPI function is MOSI/11 00777 // Arduino digital pin D12 SPI function is MISO/12 00778 // Arduino digital pin D13 SPI function is SCK/13 00779 #define HAS_digitalInOut10 0 00780 #define HAS_digitalInOut11 0 00781 #define HAS_digitalInOut12 0 00782 #define HAS_digitalInOut13 0 00783 #else // HAS_SPI 00784 #define HAS_digitalInOut10 1 00785 #define HAS_digitalInOut11 1 00786 #define HAS_digitalInOut12 1 00787 #define HAS_digitalInOut13 1 00788 #endif // HAS_SPI 00789 #if HAS_I2C 00790 // avoid resource conflict between P5_7, P6_0 I2C and DigitalInOut 00791 // Arduino digital pin D14 I2C function is A4/SDA (10pin digital connector) 00792 // Arduino digital pin D15 I2C function is A5/SCL (10pin digital connector) 00793 // Arduino digital pin D16 I2C function is A4/SDA (6pin analog connector) 00794 // Arduino digital pin D17 I2C function is A5/SCL (6pin analog connector) 00795 #define HAS_digitalInOut14 0 00796 #define HAS_digitalInOut15 0 00797 #define HAS_digitalInOut16 0 00798 #define HAS_digitalInOut17 0 00799 #else // HAS_I2C 00800 #define HAS_digitalInOut14 1 00801 #define HAS_digitalInOut15 1 00802 #define HAS_digitalInOut16 0 00803 #define HAS_digitalInOut17 0 00804 #endif // HAS_I2C 00805 #if HAS_digitalInOut0 00806 DigitalInOut digitalInOut0(D0, PIN_INPUT, PullUp, 1); 00807 #endif 00808 #if HAS_digitalInOut1 00809 DigitalInOut digitalInOut1(D1, PIN_INPUT, PullUp, 1); 00810 #endif 00811 #if HAS_digitalInOut2 00812 DigitalInOut digitalInOut2(D2, PIN_INPUT, PullUp, 1); 00813 #endif 00814 #if HAS_digitalInOut3 00815 DigitalInOut digitalInOut3(D3, PIN_INPUT, PullUp, 1); 00816 #endif 00817 #if HAS_digitalInOut4 00818 DigitalInOut digitalInOut4(D4, PIN_INPUT, PullUp, 1); 00819 #endif 00820 #if HAS_digitalInOut5 00821 DigitalInOut digitalInOut5(D5, PIN_INPUT, PullUp, 1); 00822 #endif 00823 #if HAS_digitalInOut6 00824 DigitalInOut digitalInOut6(D6, PIN_INPUT, PullUp, 1); 00825 #endif 00826 #if HAS_digitalInOut7 00827 DigitalInOut digitalInOut7(D7, PIN_INPUT, PullUp, 1); 00828 #endif 00829 #if HAS_digitalInOut8 00830 DigitalInOut digitalInOut8(D8, PIN_INPUT, PullUp, 1); 00831 #endif 00832 #if HAS_digitalInOut9 00833 DigitalInOut digitalInOut9(D9, PIN_INPUT, PullUp, 1); 00834 #endif 00835 #if HAS_digitalInOut10 00836 // Arduino digital pin D10 SPI function is CS/10 00837 DigitalInOut digitalInOut10(D10, PIN_INPUT, PullUp, 1); 00838 #endif 00839 #if HAS_digitalInOut11 00840 // Arduino digital pin D11 SPI function is MOSI/11 00841 DigitalInOut digitalInOut11(D11, PIN_INPUT, PullUp, 1); 00842 #endif 00843 #if HAS_digitalInOut12 00844 // Arduino digital pin D12 SPI function is MISO/12 00845 DigitalInOut digitalInOut12(D12, PIN_INPUT, PullUp, 1); 00846 #endif 00847 #if HAS_digitalInOut13 00848 // Arduino digital pin D13 SPI function is SCK/13 00849 DigitalInOut digitalInOut13(D13, PIN_INPUT, PullUp, 1); 00850 #endif 00851 #if HAS_digitalInOut14 00852 // Arduino digital pin D14 I2C function is A4/SDA (10pin digital connector) 00853 DigitalInOut digitalInOut14(D14, PIN_INPUT, PullUp, 1); 00854 #endif 00855 #if HAS_digitalInOut15 00856 // Arduino digital pin D15 I2C function is A5/SCL (10pin digital connector) 00857 DigitalInOut digitalInOut15(D15, PIN_INPUT, PullUp, 1); 00858 #endif 00859 #if HAS_digitalInOut16 00860 // Arduino digital pin D16 I2C function is A4/SDA (6pin analog connector) 00861 DigitalInOut digitalInOut16(D16, PIN_INPUT, PullUp, 1); 00862 #endif 00863 #if HAS_digitalInOut17 00864 // Arduino digital pin D17 I2C function is A5/SCL (6pin analog connector) 00865 DigitalInOut digitalInOut17(D17, PIN_INPUT, PullUp, 1); 00866 #endif 00867 //-------------------------------------------------- 00868 #elif defined(TARGET_LPC1768) 00869 #define HAS_digitalInOut0 1 00870 #define HAS_digitalInOut1 1 00871 #define HAS_digitalInOut2 1 00872 #define HAS_digitalInOut3 1 00873 #define HAS_digitalInOut4 1 00874 #define HAS_digitalInOut5 1 00875 #define HAS_digitalInOut6 1 00876 #define HAS_digitalInOut7 1 00877 #define HAS_digitalInOut8 1 00878 #define HAS_digitalInOut9 1 00879 // #define HAS_digitalInOut10 1 00880 // #define HAS_digitalInOut11 1 00881 // #define HAS_digitalInOut12 1 00882 // #define HAS_digitalInOut13 1 00883 // #define HAS_digitalInOut14 1 00884 // #define HAS_digitalInOut15 1 00885 #if HAS_digitalInOut0 00886 DigitalInOut digitalInOut0(p5, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.9/I2STX_SDA/MOSI1/MAT2.3 00887 #endif 00888 #if HAS_digitalInOut1 00889 DigitalInOut digitalInOut1(p6, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.8/I2STX_WS/MISO1/MAT2.2 00890 #endif 00891 #if HAS_digitalInOut2 00892 DigitalInOut digitalInOut2(p7, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.7/I2STX_CLK/SCK1/MAT2.1 00893 #endif 00894 #if HAS_digitalInOut3 00895 DigitalInOut digitalInOut3(p8, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.6/I2SRX_SDA/SSEL1/MAT2.0 00896 #endif 00897 #if HAS_digitalInOut4 00898 DigitalInOut digitalInOut4(p9, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.0/CAN_RX1/TXD3/SDA1 00899 #endif 00900 #if HAS_digitalInOut5 00901 DigitalInOut digitalInOut5(p10, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.1/CAN_TX1/RXD3/SCL1 00902 #endif 00903 #if HAS_digitalInOut6 00904 DigitalInOut digitalInOut6(p11, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.18/DCD1/MOSI0/MOSI1 00905 #endif 00906 #if HAS_digitalInOut7 00907 DigitalInOut digitalInOut7(p12, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.17/CTS1/MISO0/MISO 00908 #endif 00909 #if HAS_digitalInOut8 00910 DigitalInOut digitalInOut8(p13, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.15/TXD1/SCK0/SCK 00911 #endif 00912 #if HAS_digitalInOut9 00913 DigitalInOut digitalInOut9(p14, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.16/RXD1/SSEL0/SSEL 00914 #endif 00915 // 00916 // these pins support analog input analogIn0 .. analogIn5 00917 //DigitalInOut digitalInOut_(p15, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.23/AD0.0/I2SRX_CLK/CAP3.0 00918 //DigitalInOut digitalInOut_(p16, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.24/AD0.1/I2SRX_WS/CAP3.1 00919 //DigitalInOut digitalInOut_(p17, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.25/AD0.2/I2SRX_SDA/TXD3 00920 //DigitalInOut digitalInOut_(p18, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.26/AD0.3/AOUT/RXD3 00921 //DigitalInOut digitalInOut_(p19, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P1.30/VBUS/AD0.4 00922 //DigitalInOut digitalInOut_(p20, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P1.31/SCK1/AD0.5 00923 // 00924 // these pins support PWM pwmDriver1 .. pwmDriver6 00925 //DigitalInOut digitalInOut_(p21, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P2.5/PWM1.6/DTR1/TRACEDATA0 00926 //DigitalInOut digitalInOut_(p22, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P2.4/PWM1.5/DSR1/TRACEDATA1 00927 //DigitalInOut digitalInOut_(p23, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P2.3/PWM1.4/DCD1/TRACEDATA2 00928 //DigitalInOut digitalInOut_(p24, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P2.2/PWM1.3/CTS1/TRACEDATA3 00929 //DigitalInOut digitalInOut_(p25, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P2.1/PWM1.2/RXD1 00930 //DigitalInOut digitalInOut_(p26, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P2.0/PWM1.1/TXD1/TRACECLK 00931 // 00932 // these could be additional digitalInOut pins 00933 #if HAS_digitalInOut10 00934 DigitalInOut digitalInOut10(p27, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.11/RXD2/SCL2/MAT3.1 00935 #endif 00936 #if HAS_digitalInOut11 00937 DigitalInOut digitalInOut11(p28, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.10/TXD2/SDA2/MAT3.0 00938 #endif 00939 #if HAS_digitalInOut12 00940 DigitalInOut digitalInOut12(p29, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.5/I2SRX_WS/CAN_TX2/CAP2.1 00941 #endif 00942 #if HAS_digitalInOut13 00943 DigitalInOut digitalInOut13(p30, PIN_INPUT, PullUp, 1); // TARGET_LPC1768 P0.4/I2SRX_CLK/CAN_RX2/CAP2.0 00944 #endif 00945 #if HAS_digitalInOut14 00946 DigitalInOut digitalInOut14(___, PIN_INPUT, PullUp, 1); 00947 #endif 00948 #if HAS_digitalInOut15 00949 DigitalInOut digitalInOut15(___, PIN_INPUT, PullUp, 1); 00950 #endif 00951 #else 00952 // unknown target 00953 #endif 00954 // uncrustify-0.66.1 *INDENT-ON* 00955 #if HAS_digitalInOut0 || HAS_digitalInOut1 \ 00956 || HAS_digitalInOut2 || HAS_digitalInOut3 \ 00957 || HAS_digitalInOut4 || HAS_digitalInOut5 \ 00958 || HAS_digitalInOut6 || HAS_digitalInOut7 \ 00959 || HAS_digitalInOut8 || HAS_digitalInOut9 \ 00960 || HAS_digitalInOut10 || HAS_digitalInOut11 \ 00961 || HAS_digitalInOut12 || HAS_digitalInOut13 \ 00962 || HAS_digitalInOut14 || HAS_digitalInOut15 \ 00963 || HAS_digitalInOut16 || HAS_digitalInOut17 00964 #define HAS_digitalInOuts 1 00965 #else 00966 #warning "Note: There are no digitalInOut resources defined" 00967 #endif 00968 00969 // uncrustify-0.66.1 *INDENT-OFF* 00970 //-------------------------------------------------- 00971 // Declare the AnalogIn driver 00972 // Optional analogIn support. If there is only one it should be analogIn1. 00973 // A) analog input 00974 #if defined(TARGET_MAX32630) 00975 #define HAS_analogIn0 1 00976 #define HAS_analogIn1 1 00977 #define HAS_analogIn2 1 00978 #define HAS_analogIn3 1 00979 #define HAS_analogIn4 1 00980 #define HAS_analogIn5 1 00981 #define HAS_analogIn6 1 00982 #define HAS_analogIn7 1 00983 #define HAS_analogIn8 1 00984 #define HAS_analogIn9 1 00985 // #define HAS_analogIn10 0 00986 // #define HAS_analogIn11 0 00987 // #define HAS_analogIn12 0 00988 // #define HAS_analogIn13 0 00989 // #define HAS_analogIn14 0 00990 // #define HAS_analogIn15 0 00991 #if HAS_analogIn0 00992 AnalogIn analogIn0(AIN_0); // TARGET_MAX32630 J1.5 AIN_0 = AIN0 pin fullscale is 1.2V 00993 #endif 00994 #if HAS_analogIn1 00995 AnalogIn analogIn1(AIN_1); // TARGET_MAX32630 J1.6 AIN_1 = AIN1 pin fullscale is 1.2V 00996 #endif 00997 #if HAS_analogIn2 00998 AnalogIn analogIn2(AIN_2); // TARGET_MAX32630 J1.7 AIN_2 = AIN2 pin fullscale is 1.2V 00999 #endif 01000 #if HAS_analogIn3 01001 AnalogIn analogIn3(AIN_3); // TARGET_MAX32630 J1.8 AIN_3 = AIN3 pin fullscale is 1.2V 01002 #endif 01003 #if HAS_analogIn4 01004 AnalogIn analogIn4(AIN_4); // TARGET_MAX32630 J1.5 AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01005 #endif 01006 #if HAS_analogIn5 01007 AnalogIn analogIn5(AIN_5); // TARGET_MAX32630 J1.6 AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01008 #endif 01009 #if HAS_analogIn6 01010 AnalogIn analogIn6(AIN_6); // TARGET_MAX32630 AIN_6 = VDDB / 4.0 fullscale is 4.8V 01011 #endif 01012 #if HAS_analogIn7 01013 AnalogIn analogIn7(AIN_7); // TARGET_MAX32630 AIN_7 = VDD18 fullscale is 1.2V 01014 #endif 01015 #if HAS_analogIn8 01016 AnalogIn analogIn8(AIN_8); // TARGET_MAX32630 AIN_8 = VDD12 fullscale is 1.2V 01017 #endif 01018 #if HAS_analogIn9 01019 AnalogIn analogIn9(AIN_9); // TARGET_MAX32630 AIN_9 = VRTC / 2.0 fullscale is 2.4V 01020 #endif 01021 #if HAS_analogIn10 01022 AnalogIn analogIn10(____); // TARGET_MAX32630 AIN_10 = x undefined? 01023 #endif 01024 #if HAS_analogIn11 01025 AnalogIn analogIn11(____); // TARGET_MAX32630 AIN_11 = VDDIO / 4.0 fullscale is 4.8V 01026 #endif 01027 #if HAS_analogIn12 01028 AnalogIn analogIn12(____); // TARGET_MAX32630 AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 01029 #endif 01030 #if HAS_analogIn13 01031 AnalogIn analogIn13(____); 01032 #endif 01033 #if HAS_analogIn14 01034 AnalogIn analogIn14(____); 01035 #endif 01036 #if HAS_analogIn15 01037 AnalogIn analogIn15(____); 01038 #endif 01039 //-------------------------------------------------- 01040 #elif defined(TARGET_MAX32625MBED) 01041 #define HAS_analogIn0 1 01042 #define HAS_analogIn1 1 01043 #define HAS_analogIn2 1 01044 #define HAS_analogIn3 1 01045 #define HAS_analogIn4 1 01046 #define HAS_analogIn5 1 01047 #if HAS_analogIn0 01048 AnalogIn analogIn0(AIN_0); // TARGET_MAX32630 J1.5 AIN_0 = AIN0 pin fullscale is 1.2V 01049 #endif 01050 #if HAS_analogIn1 01051 AnalogIn analogIn1(AIN_1); // TARGET_MAX32630 J1.6 AIN_1 = AIN1 pin fullscale is 1.2V 01052 #endif 01053 #if HAS_analogIn2 01054 AnalogIn analogIn2(AIN_2); // TARGET_MAX32630 J1.7 AIN_2 = AIN2 pin fullscale is 1.2V 01055 #endif 01056 #if HAS_analogIn3 01057 AnalogIn analogIn3(AIN_3); // TARGET_MAX32630 J1.8 AIN_3 = AIN3 pin fullscale is 1.2V 01058 #endif 01059 #if HAS_analogIn4 01060 AnalogIn analogIn4(AIN_4); // TARGET_MAX32630 J1.5 AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01061 #endif 01062 #if HAS_analogIn5 01063 AnalogIn analogIn5(AIN_5); // TARGET_MAX32630 J1.6 AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01064 #endif 01065 //-------------------------------------------------- 01066 #elif defined(TARGET_MAX32620FTHR) 01067 #warning "TARGET_MAX32620FTHR not previously tested; need to verify analogIn0..." 01068 #define HAS_analogIn0 1 01069 #define HAS_analogIn1 1 01070 #define HAS_analogIn2 1 01071 #define HAS_analogIn3 1 01072 #define HAS_analogIn4 1 01073 #define HAS_analogIn5 1 01074 #define HAS_analogIn6 1 01075 #define HAS_analogIn7 1 01076 #define HAS_analogIn8 1 01077 #define HAS_analogIn9 1 01078 // #define HAS_analogIn10 0 01079 // #define HAS_analogIn11 0 01080 // #define HAS_analogIn12 0 01081 // #define HAS_analogIn13 0 01082 // #define HAS_analogIn14 0 01083 // #define HAS_analogIn15 0 01084 #if HAS_analogIn0 01085 AnalogIn analogIn0(AIN_0); // TARGET_MAX32620FTHR J1.5 AIN_0 = AIN0 pin fullscale is 1.2V 01086 #endif 01087 #if HAS_analogIn1 01088 AnalogIn analogIn1(AIN_1); // TARGET_MAX32620FTHR J1.6 AIN_1 = AIN1 pin fullscale is 1.2V 01089 #endif 01090 #if HAS_analogIn2 01091 AnalogIn analogIn2(AIN_2); // TARGET_MAX32620FTHR J1.7 AIN_2 = AIN2 pin fullscale is 1.2V 01092 #endif 01093 #if HAS_analogIn3 01094 AnalogIn analogIn3(AIN_3); // TARGET_MAX32620FTHR J1.8 AIN_3 = AIN3 pin fullscale is 1.2V 01095 #endif 01096 #if HAS_analogIn4 01097 AnalogIn analogIn4(AIN_4); // TARGET_MAX32620FTHR J1.5 AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01098 #endif 01099 #if HAS_analogIn5 01100 AnalogIn analogIn5(AIN_5); // TARGET_MAX32620FTHR J1.6 AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01101 #endif 01102 #if HAS_analogIn6 01103 AnalogIn analogIn6(AIN_6); // TARGET_MAX32620FTHR AIN_6 = VDDB / 4.0 fullscale is 4.8V 01104 #endif 01105 #if HAS_analogIn7 01106 AnalogIn analogIn7(AIN_7); // TARGET_MAX32620FTHR AIN_7 = VDD18 fullscale is 1.2V 01107 #endif 01108 #if HAS_analogIn8 01109 AnalogIn analogIn8(AIN_8); // TARGET_MAX32620FTHR AIN_8 = VDD12 fullscale is 1.2V 01110 #endif 01111 #if HAS_analogIn9 01112 AnalogIn analogIn9(AIN_9); // TARGET_MAX32620FTHR AIN_9 = VRTC / 2.0 fullscale is 2.4V 01113 #endif 01114 #if HAS_analogIn10 01115 AnalogIn analogIn10(____); // TARGET_MAX32620FTHR AIN_10 = x undefined? 01116 #endif 01117 #if HAS_analogIn11 01118 AnalogIn analogIn11(____); // TARGET_MAX32620FTHR AIN_11 = VDDIO / 4.0 fullscale is 4.8V 01119 #endif 01120 #if HAS_analogIn12 01121 AnalogIn analogIn12(____); // TARGET_MAX32620FTHR AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 01122 #endif 01123 #if HAS_analogIn13 01124 AnalogIn analogIn13(____); 01125 #endif 01126 #if HAS_analogIn14 01127 AnalogIn analogIn14(____); 01128 #endif 01129 #if HAS_analogIn15 01130 AnalogIn analogIn15(____); 01131 #endif 01132 //-------------------------------------------------- 01133 #elif defined(TARGET_MAX32625PICO) 01134 #warning "TARGET_MAX32625PICO not previously tested; need to verify analogIn0..." 01135 #define HAS_analogIn0 1 01136 #define HAS_analogIn1 1 01137 #define HAS_analogIn2 1 01138 #define HAS_analogIn3 1 01139 #define HAS_analogIn4 1 01140 #define HAS_analogIn5 1 01141 #if HAS_analogIn0 01142 AnalogIn analogIn0(AIN_0); // TARGET_MAX32630 J1.5 AIN_0 = AIN0 pin fullscale is 1.2V 01143 #endif 01144 #if HAS_analogIn1 01145 AnalogIn analogIn1(AIN_1); // TARGET_MAX32630 J1.6 AIN_1 = AIN1 pin fullscale is 1.2V 01146 #endif 01147 #if HAS_analogIn2 01148 AnalogIn analogIn2(AIN_2); // TARGET_MAX32630 J1.7 AIN_2 = AIN2 pin fullscale is 1.2V 01149 #endif 01150 #if HAS_analogIn3 01151 AnalogIn analogIn3(AIN_3); // TARGET_MAX32630 J1.8 AIN_3 = AIN3 pin fullscale is 1.2V 01152 #endif 01153 #if HAS_analogIn4 01154 AnalogIn analogIn4(AIN_4); // TARGET_MAX32630 J1.5 AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01155 #endif 01156 #if HAS_analogIn5 01157 AnalogIn analogIn5(AIN_5); // TARGET_MAX32630 J1.6 AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01158 #endif 01159 //-------------------------------------------------- 01160 #elif defined(TARGET_MAX32600) 01161 #define HAS_analogIn0 1 01162 #define HAS_analogIn1 1 01163 #define HAS_analogIn2 1 01164 #define HAS_analogIn3 1 01165 #define HAS_analogIn4 1 01166 #define HAS_analogIn5 1 01167 #if HAS_analogIn0 01168 AnalogIn analogIn0(A0); 01169 #endif 01170 #if HAS_analogIn1 01171 AnalogIn analogIn1(A1); 01172 #endif 01173 #if HAS_analogIn2 01174 AnalogIn analogIn2(A2); 01175 #endif 01176 #if HAS_analogIn3 01177 AnalogIn analogIn3(A3); 01178 #endif 01179 #if HAS_analogIn4 01180 AnalogIn analogIn4(A4); 01181 #endif 01182 #if HAS_analogIn5 01183 AnalogIn analogIn5(A5); 01184 #endif 01185 //-------------------------------------------------- 01186 #elif defined(TARGET_NUCLEO_F446RE) 01187 #define HAS_analogIn0 1 01188 #define HAS_analogIn1 1 01189 #define HAS_analogIn2 1 01190 #define HAS_analogIn3 1 01191 #define HAS_analogIn4 1 01192 #define HAS_analogIn5 1 01193 #if HAS_analogIn0 01194 AnalogIn analogIn0(A0); 01195 #endif 01196 #if HAS_analogIn1 01197 AnalogIn analogIn1(A1); 01198 #endif 01199 #if HAS_analogIn2 01200 AnalogIn analogIn2(A2); 01201 #endif 01202 #if HAS_analogIn3 01203 AnalogIn analogIn3(A3); 01204 #endif 01205 #if HAS_analogIn4 01206 AnalogIn analogIn4(A4); 01207 #endif 01208 #if HAS_analogIn5 01209 AnalogIn analogIn5(A5); 01210 #endif 01211 //-------------------------------------------------- 01212 #elif defined(TARGET_NUCLEO_F401RE) 01213 #define HAS_analogIn0 1 01214 #define HAS_analogIn1 1 01215 #define HAS_analogIn2 1 01216 #define HAS_analogIn3 1 01217 #define HAS_analogIn4 1 01218 #define HAS_analogIn5 1 01219 #if HAS_analogIn0 01220 AnalogIn analogIn0(A0); 01221 #endif 01222 #if HAS_analogIn1 01223 AnalogIn analogIn1(A1); 01224 #endif 01225 #if HAS_analogIn2 01226 AnalogIn analogIn2(A2); 01227 #endif 01228 #if HAS_analogIn3 01229 AnalogIn analogIn3(A3); 01230 #endif 01231 #if HAS_analogIn4 01232 AnalogIn analogIn4(A4); 01233 #endif 01234 #if HAS_analogIn5 01235 AnalogIn analogIn5(A5); 01236 #endif 01237 //-------------------------------------------------- 01238 // TODO1: TARGET=MAX32625MBED ARM Cortex-M4F 96MHz 512kB Flash 160kB SRAM 01239 #elif defined(TARGET_LPC1768) 01240 #define HAS_analogIn0 1 01241 #define HAS_analogIn1 1 01242 #define HAS_analogIn2 1 01243 #define HAS_analogIn3 1 01244 #define HAS_analogIn4 1 01245 #define HAS_analogIn5 1 01246 // #define HAS_analogIn6 1 01247 // #define HAS_analogIn7 1 01248 // #define HAS_analogIn8 1 01249 // #define HAS_analogIn9 1 01250 // #define HAS_analogIn10 1 01251 // #define HAS_analogIn11 1 01252 // #define HAS_analogIn12 1 01253 // #define HAS_analogIn13 1 01254 // #define HAS_analogIn14 1 01255 // #define HAS_analogIn15 1 01256 #if HAS_analogIn0 01257 AnalogIn analogIn0(p15); // TARGET_LPC1768 P0.23/AD0.0/I2SRX_CLK/CAP3.0 01258 #endif 01259 #if HAS_analogIn1 01260 AnalogIn analogIn1(p16); // TARGET_LPC1768 P0.24/AD0.1/I2SRX_WS/CAP3.1 01261 #endif 01262 #if HAS_analogIn2 01263 AnalogIn analogIn2(p17); // TARGET_LPC1768 P0.25/AD0.2/I2SRX_SDA/TXD3 01264 #endif 01265 #if HAS_analogIn3 01266 AnalogIn analogIn3(p18); // TARGET_LPC1768 P0.26/AD0.3/AOUT/RXD3 01267 #endif 01268 #if HAS_analogIn4 01269 AnalogIn analogIn4(p19); // TARGET_LPC1768 P1.30/VBUS/AD0.4 01270 #endif 01271 #if HAS_analogIn5 01272 AnalogIn analogIn5(p20); // TARGET_LPC1768 P1.31/SCK1/AD0.5 01273 #endif 01274 #if HAS_analogIn6 01275 AnalogIn analogIn6(____); 01276 #endif 01277 #if HAS_analogIn7 01278 AnalogIn analogIn7(____); 01279 #endif 01280 #if HAS_analogIn8 01281 AnalogIn analogIn8(____); 01282 #endif 01283 #if HAS_analogIn9 01284 AnalogIn analogIn9(____); 01285 #endif 01286 #if HAS_analogIn10 01287 AnalogIn analogIn10(____); 01288 #endif 01289 #if HAS_analogIn11 01290 AnalogIn analogIn11(____); 01291 #endif 01292 #if HAS_analogIn12 01293 AnalogIn analogIn12(____); 01294 #endif 01295 #if HAS_analogIn13 01296 AnalogIn analogIn13(____); 01297 #endif 01298 #if HAS_analogIn14 01299 AnalogIn analogIn14(____); 01300 #endif 01301 #if HAS_analogIn15 01302 AnalogIn analogIn15(____); 01303 #endif 01304 #else 01305 // unknown target 01306 #endif 01307 // uncrustify-0.66.1 *INDENT-ON* 01308 #if HAS_analogIn0 || HAS_analogIn1 \ 01309 || HAS_analogIn2 || HAS_analogIn3 \ 01310 || HAS_analogIn4 || HAS_analogIn5 \ 01311 || HAS_analogIn6 || HAS_analogIn7 \ 01312 || HAS_analogIn8 || HAS_analogIn9 \ 01313 || HAS_analogIn10 || HAS_analogIn11 \ 01314 || HAS_analogIn12 || HAS_analogIn13 \ 01315 || HAS_analogIn14 || HAS_analogIn15 01316 #define HAS_analogIns 1 01317 #else 01318 #warning "Note: There are no analogIn resources defined" 01319 #endif 01320 01321 // DigitalInOut pin resource: print the pin index names to serial 01322 #if HAS_digitalInOuts 01323 void list_digitalInOutPins(Stream& serialStream) 01324 { 01325 #if HAS_digitalInOut0 01326 serialStream.printf(" 0"); 01327 #endif 01328 #if HAS_digitalInOut1 01329 serialStream.printf(" 1"); 01330 #endif 01331 #if HAS_digitalInOut2 01332 serialStream.printf(" 2"); 01333 #endif 01334 #if HAS_digitalInOut3 01335 serialStream.printf(" 3"); 01336 #endif 01337 #if HAS_digitalInOut4 01338 serialStream.printf(" 4"); 01339 #endif 01340 #if HAS_digitalInOut5 01341 serialStream.printf(" 5"); 01342 #endif 01343 #if HAS_digitalInOut6 01344 serialStream.printf(" 6"); 01345 #endif 01346 #if HAS_digitalInOut7 01347 serialStream.printf(" 7"); 01348 #endif 01349 #if HAS_digitalInOut8 01350 serialStream.printf(" 8"); 01351 #endif 01352 #if HAS_digitalInOut9 01353 serialStream.printf(" 9"); 01354 #endif 01355 #if HAS_digitalInOut10 01356 serialStream.printf(" 10"); 01357 #endif 01358 #if HAS_digitalInOut11 01359 serialStream.printf(" 11"); 01360 #endif 01361 #if HAS_digitalInOut12 01362 serialStream.printf(" 12"); 01363 #endif 01364 #if HAS_digitalInOut13 01365 serialStream.printf(" 13"); 01366 #endif 01367 #if HAS_digitalInOut14 01368 serialStream.printf(" 14"); 01369 #endif 01370 #if HAS_digitalInOut15 01371 serialStream.printf(" 15"); 01372 #endif 01373 #if HAS_digitalInOut16 01374 serialStream.printf(" 16"); 01375 #endif 01376 #if HAS_digitalInOut17 01377 serialStream.printf(" 17"); 01378 #endif 01379 } 01380 #endif 01381 01382 01383 // DigitalInOut pin resource: search index 01384 #if HAS_digitalInOuts 01385 DigitalInOut& find_digitalInOutPin(int cPinIndex) 01386 { 01387 switch (cPinIndex) 01388 { 01389 default: // default to the first defined digitalInOut pin 01390 #if HAS_digitalInOut0 01391 case '0': case 0x00: return digitalInOut0; 01392 #endif 01393 #if HAS_digitalInOut1 01394 case '1': case 0x01: return digitalInOut1; 01395 #endif 01396 #if HAS_digitalInOut2 01397 case '2': case 0x02: return digitalInOut2; 01398 #endif 01399 #if HAS_digitalInOut3 01400 case '3': case 0x03: return digitalInOut3; 01401 #endif 01402 #if HAS_digitalInOut4 01403 case '4': case 0x04: return digitalInOut4; 01404 #endif 01405 #if HAS_digitalInOut5 01406 case '5': case 0x05: return digitalInOut5; 01407 #endif 01408 #if HAS_digitalInOut6 01409 case '6': case 0x06: return digitalInOut6; 01410 #endif 01411 #if HAS_digitalInOut7 01412 case '7': case 0x07: return digitalInOut7; 01413 #endif 01414 #if HAS_digitalInOut8 01415 case '8': case 0x08: return digitalInOut8; 01416 #endif 01417 #if HAS_digitalInOut9 01418 case '9': case 0x09: return digitalInOut9; 01419 #endif 01420 #if HAS_digitalInOut10 01421 case 'a': case 0x0a: return digitalInOut10; 01422 #endif 01423 #if HAS_digitalInOut11 01424 case 'b': case 0x0b: return digitalInOut11; 01425 #endif 01426 #if HAS_digitalInOut12 01427 case 'c': case 0x0c: return digitalInOut12; 01428 #endif 01429 #if HAS_digitalInOut13 01430 case 'd': case 0x0d: return digitalInOut13; 01431 #endif 01432 #if HAS_digitalInOut14 01433 case 'e': case 0x0e: return digitalInOut14; 01434 #endif 01435 #if HAS_digitalInOut15 01436 case 'f': case 0x0f: return digitalInOut15; 01437 #endif 01438 #if HAS_digitalInOut16 01439 case 'g': case 0x10: return digitalInOut16; 01440 #endif 01441 #if HAS_digitalInOut17 01442 case 'h': case 0x11: return digitalInOut17; 01443 #endif 01444 } 01445 } 01446 #endif 01447 01448 01449 // AnalogIn pin resource: search index 01450 #if HAS_analogIns 01451 AnalogIn& find_analogInPin(int cPinIndex) 01452 { 01453 switch (cPinIndex) 01454 { 01455 default: // default to the first defined analogIn pin 01456 #if HAS_analogIn0 01457 case '0': case 0x00: return analogIn0; 01458 #endif 01459 #if HAS_analogIn1 01460 case '1': case 0x01: return analogIn1; 01461 #endif 01462 #if HAS_analogIn2 01463 case '2': case 0x02: return analogIn2; 01464 #endif 01465 #if HAS_analogIn3 01466 case '3': case 0x03: return analogIn3; 01467 #endif 01468 #if HAS_analogIn4 01469 case '4': case 0x04: return analogIn4; 01470 #endif 01471 #if HAS_analogIn5 01472 case '5': case 0x05: return analogIn5; 01473 #endif 01474 #if HAS_analogIn6 01475 case '6': case 0x06: return analogIn6; 01476 #endif 01477 #if HAS_analogIn7 01478 case '7': case 0x07: return analogIn7; 01479 #endif 01480 #if HAS_analogIn8 01481 case '8': case 0x08: return analogIn8; 01482 #endif 01483 #if HAS_analogIn9 01484 case '9': case 0x09: return analogIn9; 01485 #endif 01486 #if HAS_analogIn10 01487 case 'a': case 0x0a: return analogIn10; 01488 #endif 01489 #if HAS_analogIn11 01490 case 'b': case 0x0b: return analogIn11; 01491 #endif 01492 #if HAS_analogIn12 01493 case 'c': case 0x0c: return analogIn12; 01494 #endif 01495 #if HAS_analogIn13 01496 case 'd': case 0x0d: return analogIn13; 01497 #endif 01498 #if HAS_analogIn14 01499 case 'e': case 0x0e: return analogIn14; 01500 #endif 01501 #if HAS_analogIn15 01502 case 'f': case 0x0f: return analogIn15; 01503 #endif 01504 } 01505 } 01506 #endif 01507 01508 #if HAS_analogIns 01509 const float analogInPin_fullScaleVoltage[] = { 01510 # if defined(TARGET_MAX32630) 01511 ADC_FULL_SCALE_VOLTAGE, // analogIn0 01512 ADC_FULL_SCALE_VOLTAGE, // analogIn1 01513 ADC_FULL_SCALE_VOLTAGE, // analogIn2 01514 ADC_FULL_SCALE_VOLTAGE, // analogIn3 01515 ADC_FULL_SCALE_VOLTAGE * 5.0f, // analogIn4 // AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01516 ADC_FULL_SCALE_VOLTAGE * 5.0f, // analogIn4 // AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01517 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn6 // AIN_6 = VDDB / 4.0 fullscale is 4.8V 01518 ADC_FULL_SCALE_VOLTAGE, // analogIn7 // AIN_7 = VDD18 fullscale is 1.2V 01519 ADC_FULL_SCALE_VOLTAGE, // analogIn8 // AIN_8 = VDD12 fullscale is 1.2V 01520 ADC_FULL_SCALE_VOLTAGE * 2.0f, // analogIn9 // AIN_9 = VRTC / 2.0 fullscale is 2.4V 01521 ADC_FULL_SCALE_VOLTAGE, // analogIn10 // AIN_10 = x undefined? 01522 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn11 // AIN_11 = VDDIO / 4.0 fullscale is 4.8V 01523 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn12 // AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 01524 ADC_FULL_SCALE_VOLTAGE, // analogIn13 01525 ADC_FULL_SCALE_VOLTAGE, // analogIn14 01526 ADC_FULL_SCALE_VOLTAGE // analogIn15 01527 # elif defined(TARGET_MAX32620FTHR) 01528 #warning "TARGET_MAX32620FTHR not previously tested; need to verify analogIn0..." 01529 ADC_FULL_SCALE_VOLTAGE, // analogIn0 01530 ADC_FULL_SCALE_VOLTAGE, // analogIn1 01531 ADC_FULL_SCALE_VOLTAGE, // analogIn2 01532 ADC_FULL_SCALE_VOLTAGE, // analogIn3 01533 ADC_FULL_SCALE_VOLTAGE * 5.0f, // analogIn4 // AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01534 ADC_FULL_SCALE_VOLTAGE * 5.0f, // analogIn4 // AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01535 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn6 // AIN_6 = VDDB / 4.0 fullscale is 4.8V 01536 ADC_FULL_SCALE_VOLTAGE, // analogIn7 // AIN_7 = VDD18 fullscale is 1.2V 01537 ADC_FULL_SCALE_VOLTAGE, // analogIn8 // AIN_8 = VDD12 fullscale is 1.2V 01538 ADC_FULL_SCALE_VOLTAGE * 2.0f, // analogIn9 // AIN_9 = VRTC / 2.0 fullscale is 2.4V 01539 ADC_FULL_SCALE_VOLTAGE, // analogIn10 // AIN_10 = x undefined? 01540 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn11 // AIN_11 = VDDIO / 4.0 fullscale is 4.8V 01541 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn12 // AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 01542 ADC_FULL_SCALE_VOLTAGE, // analogIn13 01543 ADC_FULL_SCALE_VOLTAGE, // analogIn14 01544 ADC_FULL_SCALE_VOLTAGE // analogIn15 01545 #elif defined(TARGET_MAX32625MBED) 01546 ADC_FULL_SCALE_VOLTAGE * 1.0f, // analogIn0 // fullscale is 1.2V 01547 ADC_FULL_SCALE_VOLTAGE * 1.0f, // analogIn1 // fullscale is 1.2V 01548 ADC_FULL_SCALE_VOLTAGE * 1.0f, // analogIn2 // fullscale is 1.2V 01549 ADC_FULL_SCALE_VOLTAGE * 1.0f, // analogIn3 // fullscale is 1.2V 01550 ADC_FULL_SCALE_VOLTAGE * 5.0f, // analogIn4 // AIN_4 = AIN0 / 5.0 fullscale is 6.0V 01551 ADC_FULL_SCALE_VOLTAGE * 5.0f, // analogIn4 // AIN_5 = AIN1 / 5.0 fullscale is 6.0V 01552 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn6 // AIN_6 = VDDB / 4.0 fullscale is 4.8V 01553 ADC_FULL_SCALE_VOLTAGE, // analogIn7 // AIN_7 = VDD18 fullscale is 1.2V 01554 ADC_FULL_SCALE_VOLTAGE, // analogIn8 // AIN_8 = VDD12 fullscale is 1.2V 01555 ADC_FULL_SCALE_VOLTAGE * 2.0f, // analogIn9 // AIN_9 = VRTC / 2.0 fullscale is 2.4V 01556 ADC_FULL_SCALE_VOLTAGE, // analogIn10 // AIN_10 = x undefined? 01557 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn11 // AIN_11 = VDDIO / 4.0 fullscale is 4.8V 01558 ADC_FULL_SCALE_VOLTAGE * 4.0f, // analogIn12 // AIN_12 = VDDIOH / 4.0 fullscale is 4.8V 01559 ADC_FULL_SCALE_VOLTAGE, // analogIn13 01560 ADC_FULL_SCALE_VOLTAGE, // analogIn14 01561 ADC_FULL_SCALE_VOLTAGE // analogIn15 01562 #elif defined(TARGET_NUCLEO_F446RE) 01563 ADC_FULL_SCALE_VOLTAGE, // analogIn0 01564 ADC_FULL_SCALE_VOLTAGE, // analogIn1 01565 ADC_FULL_SCALE_VOLTAGE, // analogIn2 01566 ADC_FULL_SCALE_VOLTAGE, // analogIn3 01567 ADC_FULL_SCALE_VOLTAGE, // analogIn4 01568 ADC_FULL_SCALE_VOLTAGE, // analogIn5 01569 ADC_FULL_SCALE_VOLTAGE, // analogIn6 01570 ADC_FULL_SCALE_VOLTAGE, // analogIn7 01571 ADC_FULL_SCALE_VOLTAGE, // analogIn8 01572 ADC_FULL_SCALE_VOLTAGE, // analogIn9 01573 ADC_FULL_SCALE_VOLTAGE, // analogIn10 01574 ADC_FULL_SCALE_VOLTAGE, // analogIn11 01575 ADC_FULL_SCALE_VOLTAGE, // analogIn12 01576 ADC_FULL_SCALE_VOLTAGE, // analogIn13 01577 ADC_FULL_SCALE_VOLTAGE, // analogIn14 01578 ADC_FULL_SCALE_VOLTAGE // analogIn15 01579 #elif defined(TARGET_NUCLEO_F401RE) 01580 ADC_FULL_SCALE_VOLTAGE, // analogIn0 01581 ADC_FULL_SCALE_VOLTAGE, // analogIn1 01582 ADC_FULL_SCALE_VOLTAGE, // analogIn2 01583 ADC_FULL_SCALE_VOLTAGE, // analogIn3 01584 ADC_FULL_SCALE_VOLTAGE, // analogIn4 01585 ADC_FULL_SCALE_VOLTAGE, // analogIn5 01586 ADC_FULL_SCALE_VOLTAGE, // analogIn6 01587 ADC_FULL_SCALE_VOLTAGE, // analogIn7 01588 ADC_FULL_SCALE_VOLTAGE, // analogIn8 01589 ADC_FULL_SCALE_VOLTAGE, // analogIn9 01590 ADC_FULL_SCALE_VOLTAGE, // analogIn10 01591 ADC_FULL_SCALE_VOLTAGE, // analogIn11 01592 ADC_FULL_SCALE_VOLTAGE, // analogIn12 01593 ADC_FULL_SCALE_VOLTAGE, // analogIn13 01594 ADC_FULL_SCALE_VOLTAGE, // analogIn14 01595 ADC_FULL_SCALE_VOLTAGE // analogIn15 01596 //#elif defined(TARGET_LPC1768) 01597 #else 01598 // unknown target 01599 ADC_FULL_SCALE_VOLTAGE, // analogIn0 01600 ADC_FULL_SCALE_VOLTAGE, // analogIn1 01601 ADC_FULL_SCALE_VOLTAGE, // analogIn2 01602 ADC_FULL_SCALE_VOLTAGE, // analogIn3 01603 ADC_FULL_SCALE_VOLTAGE, // analogIn4 01604 ADC_FULL_SCALE_VOLTAGE, // analogIn5 01605 ADC_FULL_SCALE_VOLTAGE, // analogIn6 01606 ADC_FULL_SCALE_VOLTAGE, // analogIn7 01607 ADC_FULL_SCALE_VOLTAGE, // analogIn8 01608 ADC_FULL_SCALE_VOLTAGE, // analogIn9 01609 ADC_FULL_SCALE_VOLTAGE, // analogIn10 01610 ADC_FULL_SCALE_VOLTAGE, // analogIn11 01611 ADC_FULL_SCALE_VOLTAGE, // analogIn12 01612 ADC_FULL_SCALE_VOLTAGE, // analogIn13 01613 ADC_FULL_SCALE_VOLTAGE, // analogIn14 01614 ADC_FULL_SCALE_VOLTAGE // analogIn15 01615 # endif 01616 }; 01617 #endif 01618 01619 01620 01621 01622 //-------------------------------------------------- 01623 // Option to use LEDs to show status 01624 #ifndef USE_LEDS 01625 #define USE_LEDS 1 01626 #endif 01627 #if USE_LEDS 01628 #if defined(TARGET_MAX32630) 01629 # define LED_ON 0 01630 # define LED_OFF 1 01631 //-------------------------------------------------- 01632 #elif defined(TARGET_MAX32625MBED) 01633 # define LED_ON 0 01634 # define LED_OFF 1 01635 //-------------------------------------------------- 01636 // TODO1: TARGET=MAX32625MBED ARM Cortex-M4F 96MHz 512kB Flash 160kB SRAM 01637 #elif defined(TARGET_LPC1768) 01638 # define LED_ON 1 01639 # define LED_OFF 0 01640 #else // not defined(TARGET_LPC1768 etc.) 01641 // USE_LEDS with some platform other than MAX32630, MAX32625MBED, LPC1768 01642 // bugfix for MAX32600MBED LED blink pattern: check if LED_ON/LED_OFF already defined 01643 # ifndef LED_ON 01644 # define LED_ON 0 01645 # endif 01646 # ifndef LED_OFF 01647 # define LED_OFF 1 01648 # endif 01649 //# define LED_ON 1 01650 //# define LED_OFF 0 01651 #endif // target definition 01652 DigitalOut led1(LED1, LED_OFF); // MAX32630FTHR: LED1 = LED_RED 01653 DigitalOut led2(LED2, LED_OFF); // MAX32630FTHR: LED2 = LED_GREEN 01654 DigitalOut led3(LED3, LED_OFF); // MAX32630FTHR: LED3 = LED_BLUE 01655 DigitalOut led4(LED4, LED_OFF); 01656 #else // USE_LEDS=0 01657 // issue #41 support Nucleo_F446RE 01658 // there are no LED indicators on the board, LED1 interferes with SPI; 01659 // but we still need placeholders led1 led2 led3 led4. 01660 // Declare DigitalOut led1 led2 led3 led4 targeting safe pins. 01661 // PinName NC means NOT_CONNECTED; DigitalOut::is_connected() returns false 01662 # define LED_ON 0 01663 # define LED_OFF 1 01664 DigitalOut led1(NC, LED_OFF); 01665 DigitalOut led2(NC, LED_OFF); 01666 DigitalOut led3(NC, LED_OFF); 01667 DigitalOut led4(NC, LED_OFF); 01668 #endif // USE_LEDS 01669 #define led1_RFailLED led1 01670 #define led2_GPassLED led2 01671 #define led3_BBusyLED led3 01672 01673 //-------------------------------------------------- 01674 01675 01676 // example code board support 01677 //MAX32630FTHR pegasus(MAX32630FTHR::VIO_3V3); 01678 //DigitalOut rLED(LED1); 01679 //DigitalOut gLED(LED2); 01680 //DigitalOut bLED(LED3); 01681 // 01682 // Arduino "shield" connector port definitions (MAX32625MBED shown) 01683 #if defined(TARGET_MAX32625MBED) 01684 #define A0 AIN_0 01685 #define A1 AIN_1 01686 #define A2 AIN_2 01687 #define A3 AIN_3 01688 #define D0 P0_0 01689 #define D1 P0_1 01690 #define D2 P0_2 01691 #define D3 P0_3 01692 #define D4 P0_4 01693 #define D5 P0_5 01694 #define D6 P0_6 01695 #define D7 P0_7 01696 #define D8 P1_4 01697 #define D9 P1_5 01698 #define D10 P1_3 01699 #define D11 P1_1 01700 #define D12 P1_2 01701 #define D13 P1_0 01702 #endif 01703 01704 // example code declare SPI interface (GPIO controlled CS) 01705 #if defined(TARGET_MAX32625MBED) 01706 SPI spi(SPI1_MOSI, SPI1_MISO, SPI1_SCK); // mosi, miso, sclk spi1 TARGET_MAX32625MBED: P1_1 P1_2 P1_0 Arduino 10-pin header D11 D12 D13 01707 DigitalOut spi_cs(SPI1_SS); // TARGET_MAX32625MBED: P1_3 Arduino 10-pin header D10 01708 #elif defined(TARGET_MAX32600MBED) 01709 SPI spi(SPI2_MOSI, SPI2_MISO, SPI2_SCK); // mosi, miso, sclk spi1 TARGET_MAX32600MBED: Arduino 10-pin header D11 D12 D13 01710 DigitalOut spi_cs(SPI2_SS); // Generic: Arduino 10-pin header D10 01711 #elif defined(TARGET_NUCLEO_F446RE) || defined(TARGET_NUCLEO_F401RE) 01712 // TODO1: avoid resource conflict between P5_0, P5_1, P5_2 SPI and DigitalInOut 01713 // void spi_init(spi_t *obj, PinName mosi, PinName miso, PinName sclk, PinName ssel) 01714 // 01715 // TODO1: NUCLEO_F446RE SPI not working; CS and MOSI data looks OK but no SCLK clock pulses. 01716 SPI spi(SPI_MOSI, SPI_MISO, SPI_SCK); // mosi, miso, sclk spi1 TARGET_NUCLEO_F446RE: Arduino 10-pin header D11 D12 D13 01717 DigitalOut spi_cs(SPI_CS); // TARGET_NUCLEO_F446RE: PB_6 Arduino 10-pin header D10 01718 // 01719 #else 01720 SPI spi(D11, D12, D13); // mosi, miso, sclk spi1 TARGET_MAX32600MBED: Arduino 10-pin header D11 D12 D13 01721 DigitalOut spi_cs(D10); // Generic: Arduino 10-pin header D10 01722 #endif 01723 01724 // example code declare GPIO interface pins 01725 // AnalogOut FB_pin(Px_x_PortName_To_Be_Determined); // Analog Input to MAX5171 device 01726 DigitalOut RS_pin(D9); // Digital Configuration Input to MAX5171 device 01727 DigitalOut PDLb_pin(D8); // Digital Configuration Input to MAX5171 device 01728 DigitalOut CLRb_pin(D7); // Digital Configuration Input to MAX5171 device 01729 DigitalOut SHDN_pin(D6); // Digital Configuration Input to MAX5171 device 01730 // AnalogIn OUT_pin(A0); // Analog Output from MAX5171 device 01731 DigitalIn UPO_pin(D5); // Digital General-Purpose Output from MAX5171 device 01732 // example code declare device instance 01733 MAX5171 g_MAX5171_device(spi, spi_cs, RS_pin, PDLb_pin, CLRb_pin, SHDN_pin, UPO_pin, MAX5171::MAX5171_IC); 01734 01735 01736 //---------------------------------------- 01737 // Global SPI options 01738 // 01739 01740 //-------------------------------------------------- 01741 // Optional Diagnostic function to print SPI transactions 01742 #ifndef MAX5171_ONSPIPRINT 01743 #define MAX5171_ONSPIPRINT 1 01744 #endif // MAX5171_ONSPIPRINT 01745 // Enable the onSPIprint diagnostic at startup (toggle with %SD menu item) 01746 #ifndef MAX5171_ONSPIPRINT_ENABLED 01747 #define MAX5171_ONSPIPRINT_ENABLED 1 01748 #endif // MAX5171_ONSPIPRINT_ENABLED 01749 01750 #define APPLICATION_ArduinoPinsMonitor 1 01751 01752 //-------------------------------------------------- 01753 // use BUTTON1 trigger some action 01754 #if defined(TARGET_MAX32630) 01755 #define HAS_BUTTON1_DEMO_INTERRUPT 1 01756 #define HAS_BUTTON2_DEMO 0 01757 #define HAS_BUTTON2_DEMO_INTERRUPT 0 01758 #elif defined(TARGET_MAX32625PICO) 01759 #warning "TARGET_MAX32625PICO not previously tested; need to define buttons..." 01760 #define HAS_BUTTON1_DEMO_INTERRUPT 1 01761 #define HAS_BUTTON2_DEMO 0 01762 #define HAS_BUTTON2_DEMO_INTERRUPT 0 01763 #elif defined(TARGET_MAX32625) 01764 #define HAS_BUTTON1_DEMO_INTERRUPT 1 01765 #define HAS_BUTTON2_DEMO_INTERRUPT 1 01766 #elif defined(TARGET_MAX32620FTHR) 01767 #warning "TARGET_MAX32620FTHR not previously tested; need to define buttons..." 01768 #define BUTTON1 SW1 01769 #define HAS_BUTTON1_DEMO_INTERRUPT 1 01770 #define HAS_BUTTON2_DEMO 0 01771 #define HAS_BUTTON2_DEMO_INTERRUPT 0 01772 #elif defined(TARGET_NUCLEO_F446RE) 01773 #define HAS_BUTTON1_DEMO_INTERRUPT 0 01774 #define HAS_BUTTON2_DEMO_INTERRUPT 0 01775 #elif defined(TARGET_NUCLEO_F401RE) 01776 #define HAS_BUTTON1_DEMO_INTERRUPT 0 01777 #define HAS_BUTTON2_DEMO_INTERRUPT 0 01778 #else 01779 #warning "target not previously tested; need to define buttons..." 01780 #endif 01781 // 01782 #ifndef HAS_BUTTON1_DEMO 01783 #define HAS_BUTTON1_DEMO 0 01784 #endif 01785 #ifndef HAS_BUTTON2_DEMO 01786 #define HAS_BUTTON2_DEMO 0 01787 #endif 01788 // 01789 // avoid runtime error on button1 press [mbed-os-5.11] 01790 // instead of using InterruptIn, use DigitalIn and poll in main while(1) 01791 #ifndef HAS_BUTTON1_DEMO_INTERRUPT_POLLING 01792 #define HAS_BUTTON1_DEMO_INTERRUPT_POLLING 1 01793 #endif 01794 // 01795 #ifndef HAS_BUTTON1_DEMO_INTERRUPT 01796 #define HAS_BUTTON1_DEMO_INTERRUPT 1 01797 #endif 01798 #ifndef HAS_BUTTON2_DEMO_INTERRUPT 01799 #define HAS_BUTTON2_DEMO_INTERRUPT 1 01800 #endif 01801 // 01802 #if HAS_BUTTON1_DEMO_INTERRUPT 01803 # if HAS_BUTTON1_DEMO_INTERRUPT_POLLING 01804 // avoid runtime error on button1 press [mbed-os-5.11] 01805 // instead of using InterruptIn, use DigitalIn and poll in main while(1) 01806 DigitalIn button1(BUTTON1); 01807 # else 01808 InterruptIn button1(BUTTON1); 01809 # endif 01810 #elif HAS_BUTTON1_DEMO 01811 DigitalIn button1(BUTTON1); 01812 #endif 01813 #if HAS_BUTTON2_DEMO_INTERRUPT 01814 # if HAS_BUTTON1_DEMO_INTERRUPT_POLLING 01815 // avoid runtime error on button1 press [mbed-os-5.11] 01816 // instead of using InterruptIn, use DigitalIn and poll in main while(1) 01817 DigitalIn button2(BUTTON2); 01818 # else 01819 InterruptIn button2(BUTTON2); 01820 # endif 01821 #elif HAS_BUTTON2_DEMO 01822 DigitalIn button2(BUTTON2); 01823 #endif 01824 01825 //-------------------------------------------------- 01826 // functions tested by SelfTest() 01827 extern uint16_t fn_MAX5171_DACCodeOfVoltage(double voltageV); 01828 extern double fn_MAX5171_VoltageOfCode(uint16_t value_u14); 01829 extern void fn_MAX5171_Init(void); 01830 extern uint8_t fn_MAX5171_CODE_LOAD(uint16_t dacCodeLsbs); 01831 extern uint8_t fn_MAX5171_UPO_HIGH(void); 01832 extern uint8_t fn_MAX5171_UPO_LOW(void); 01833 01834 //-------------------------------------------------- 01835 // optional self-test groups for self test function SelfTest() 01836 // enable by changing the #define value from 0 to 1 01837 01838 // SelfTest group DACCodeOfVoltage description: 01839 // Verify function DACCodeOfVoltage (enabled by default) 01840 #ifndef MAX5171_SELFTEST_DACCodeOfVoltage 01841 #define MAX5171_SELFTEST_DACCodeOfVoltage 1 01842 #endif 01843 01844 // SelfTest group VoltageOfCode description: 01845 // Verify function VoltageOfCode (enabled by default) 01846 #ifndef MAX5171_SELFTEST_VoltageOfCode 01847 #define MAX5171_SELFTEST_VoltageOfCode 1 01848 #endif 01849 01850 // SelfTest group CODE_LOAD description: 01851 // Verify function CODE_LOAD (enabled by default) 01852 #ifndef MAX5171_SELFTEST_CODE_LOAD 01853 #define MAX5171_SELFTEST_CODE_LOAD 1 01854 #endif 01855 01856 // SelfTest group UPO description: 01857 // Verify User Programmable Output functions UPO_HIGH and UPO_LOW (enabled by default) 01858 #ifndef MAX5171_SELFTEST_UPO 01859 #define MAX5171_SELFTEST_UPO 1 01860 #endif 01861 01862 //-------------------------------------------------- 01863 // When user presses button BUTTON1, perform self test 01864 #if HAS_BUTTON1_DEMO_INTERRUPT 01865 void onButton1FallingEdge(void) 01866 { 01867 void SelfTest(CmdLine & cmdLine); 01868 SelfTest(cmdLine_serial); 01869 } 01870 #endif // HAS_BUTTON1_DEMO_INTERRUPT 01871 01872 //-------------------------------------------------- 01873 // When user presses button BUTTON2, perform demo configuration 01874 #if HAS_BUTTON2_DEMO_INTERRUPT 01875 void onButton2FallingEdge(void) 01876 { 01877 // TBD demo configuration 01878 // TODO diagnostic LED 01879 led1 = LED_OFF; led2 = LED_OFF; led3 = LED_ON; // diagnostic rbg led BLUE 01880 } 01881 #endif // HAS_BUTTON2_DEMO_INTERRUPT 01882 01883 //-------------------------------------------------- 01884 void SelfTest(CmdLine & cmdLine) 01885 { 01886 //-------------------------------------------------- 01887 #if analogIn4_IS_HIGH_RANGE_OF_analogIn0 01888 // Platform board uses AIN4,AIN5,.. as high range of AIN0,AIN1,.. 01889 MaximTinyTester tinyTester(cmdLine, analogIn4, analogIn5, analogIn2, analogIn3, analogIn0, analogIn4, led1_RFailLED, led2_GPassLED, led3_BBusyLED); 01890 tinyTester.analogInPin_fullScaleVoltage[0] = analogInPin_fullScaleVoltage[4]; // board support 01891 tinyTester.analogInPin_fullScaleVoltage[1] = analogInPin_fullScaleVoltage[5]; // board support 01892 tinyTester.analogInPin_fullScaleVoltage[2] = analogInPin_fullScaleVoltage[2]; // board support 01893 tinyTester.analogInPin_fullScaleVoltage[3] = analogInPin_fullScaleVoltage[3]; // board support 01894 tinyTester.analogInPin_fullScaleVoltage[4] = analogInPin_fullScaleVoltage[0]; // board support 01895 tinyTester.analogInPin_fullScaleVoltage[5] = analogInPin_fullScaleVoltage[1]; // board support 01896 // low range channels AIN0, AIN1, AIN2, AIN3 01897 #else // analogIn4_IS_HIGH_RANGE_OF_analogIn0 01898 // Platform board uses simple analog inputs 01899 MaximTinyTester tinyTester(cmdLine, analogIn0, analogIn1, analogIn2, analogIn3, analogIn4, analogIn5, led1_RFailLED, led2_GPassLED, led3_BBusyLED); 01900 tinyTester.analogInPin_fullScaleVoltage[0] = analogInPin_fullScaleVoltage[0]; // board support 01901 tinyTester.analogInPin_fullScaleVoltage[1] = analogInPin_fullScaleVoltage[1]; // board support 01902 tinyTester.analogInPin_fullScaleVoltage[2] = analogInPin_fullScaleVoltage[2]; // board support 01903 tinyTester.analogInPin_fullScaleVoltage[3] = analogInPin_fullScaleVoltage[3]; // board support 01904 tinyTester.analogInPin_fullScaleVoltage[4] = analogInPin_fullScaleVoltage[4]; // board support 01905 tinyTester.analogInPin_fullScaleVoltage[5] = analogInPin_fullScaleVoltage[5]; // board support 01906 #endif 01907 tinyTester.clear(); 01908 01909 // @test group DACCodeOfVoltage // Verify function DACCodeOfVoltage (enabled by default) 01910 // @test group DACCodeOfVoltage tinyTester.blink_time_msec = 20 // quickly speed through the software verification 01911 // docTest_item['actionType'] = 'assign-propname-value' 01912 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 01913 // docTest_item['action'] = 'tinyTester.blink_time_msec = 20' 01914 // docTest_item['remarks'] = 'quickly speed through the software verification' 01915 // docTest_item['propName'] = 'tinyTester.blink_time_msec' 01916 // docTest_item['propValue'] = '20' 01917 #if MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage None quickly speed through the software verification 01918 // assign-propname-value 01919 // tinyTesterPropName = "tinyTester.blink_time_msec" 01920 // tinyTesterPropValue = "20" 01921 tinyTester.blink_time_msec = 20; 01922 #endif // MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 01923 01924 // @test group DACCodeOfVoltage tinyTester.print("VRef = 2.500 MAX5171 14-bit LSB = 0.00015V") 01925 // docTest_item['actionType'] = 'print-string' 01926 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 01927 // docTest_item['action'] = 'tinyTester.print("VRef = 2.500 MAX5171 14-bit LSB = 0.00015V")' 01928 // docTest_item['arglist'] = 'VRef = 2.500 MAX5171 14-bit LSB = 0.00015V' 01929 #if MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage None 01930 // print-string 01931 // tinyTesterFuncName = "tinyTester.print" 01932 // tinyTesterPrintStringLiteral = "VRef = 2.500 MAX5171 14-bit LSB = 0.00015V" 01933 tinyTester.print("VRef = 2.500 MAX5171 14-bit LSB = 0.00015V"); 01934 #endif // MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 01935 01936 // @test group DACCodeOfVoltage VRef = 2.500 01937 // docTest_item['actionType'] = 'assign-propname-value' 01938 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 01939 // docTest_item['action'] = 'VRef = 2.500' 01940 // docTest_item['propName'] = 'VRef' 01941 // docTest_item['propValue'] = '2.500' 01942 #if MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage None 01943 // assign-propname-value 01944 // tinyTesterPropName = "VRef" 01945 // tinyTesterPropValue = "2.500" 01946 g_MAX5171_device.VRef = 2.500; 01947 #endif // MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 01948 01949 // @test group DACCodeOfVoltage tinyTester.err_threshold = 0.00015259720441921504 // 14-bit LSB (2.500/16383) 01950 // docTest_item['actionType'] = 'assign-propname-value' 01951 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 01952 // docTest_item['action'] = 'tinyTester.err_threshold = 0.00015259720441921504' 01953 // docTest_item['remarks'] = '14-bit LSB (2.500/16383)' 01954 // docTest_item['propName'] = 'tinyTester.err_threshold' 01955 // docTest_item['propValue'] = '0.00015259720441921504' 01956 #if MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage None 14-bit LSB (2.500/16383) 01957 // assign-propname-value 01958 // tinyTesterPropName = "tinyTester.err_threshold" 01959 // tinyTesterPropValue = "0.00015259720441921504" 01960 tinyTester.err_threshold = 0.00015259720441921504; 01961 #endif // MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 01962 01963 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.499847412109375) expect 0x3FFF 01964 // docTest_item['actionType'] = 'call-function' 01965 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 01966 // docTest_item['action'] = 'DACCodeOfVoltage(2.499847412109375) expect 0x3FFF' 01967 // docTest_item['funcName'] = 'DACCodeOfVoltage' 01968 // docTest_item['arglist'] = '2.499847412109375' 01969 // docTest_item['expect-value'] = '0x3FFF' 01970 #if MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 01971 // call-function 01972 // ASSERT_EQ(g_MAX5171_device.DACCodeOfVoltage((double)2.499847412109375), (uint16_t)0x3FFF); // 01973 tinyTester.FunctionCall_u_f_Expect("MAX5171.DACCodeOfVoltage", fn_MAX5171_DACCodeOfVoltage, (double)2.499847412109375, /* expect: */ (uint16_t)0x3FFF); // 01974 #endif // MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 01975 01976 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.49969482421875) expect 0x3FFE 01977 // docTest_item['actionType'] = 'call-function' 01978 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 01979 // docTest_item['action'] = 'DACCodeOfVoltage(2.49969482421875) expect 0x3FFE' 01980 // docTest_item['funcName'] = 'DACCodeOfVoltage' 01981 // docTest_item['arglist'] = '2.49969482421875' 01982 // docTest_item['expect-value'] = '0x3FFE' 01983 #if MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 01984 // call-function 01985 // ASSERT_EQ(g_MAX5171_device.DACCodeOfVoltage((double)2.49969482421875), (uint16_t)0x3FFE); // 01986 tinyTester.FunctionCall_u_f_Expect("MAX5171.DACCodeOfVoltage", fn_MAX5171_DACCodeOfVoltage, (double)2.49969482421875, /* expect: */ (uint16_t)0x3FFE); // 01987 #endif // MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 01988 01989 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.499542236328125) expect 0x3FFD 01990 // docTest_item['actionType'] = 'call-function' 01991 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 01992 // docTest_item['action'] = 'DACCodeOfVoltage(2.499542236328125) expect 0x3FFD' 01993 // docTest_item['funcName'] = 'DACCodeOfVoltage' 01994 // docTest_item['arglist'] = '2.499542236328125' 01995 // docTest_item['expect-value'] = '0x3FFD' 01996 #if MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 01997 // call-function 01998 // ASSERT_EQ(g_MAX5171_device.DACCodeOfVoltage((double)2.499542236328125), (uint16_t)0x3FFD); // 01999 tinyTester.FunctionCall_u_f_Expect("MAX5171.DACCodeOfVoltage", fn_MAX5171_DACCodeOfVoltage, (double)2.499542236328125, /* expect: */ (uint16_t)0x3FFD); // 02000 #endif // MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 02001 02002 // @test group DACCodeOfVoltage DACCodeOfVoltage(2.4993896484375) expect 0x3FFC 02003 // docTest_item['actionType'] = 'call-function' 02004 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 02005 // docTest_item['action'] = 'DACCodeOfVoltage(2.4993896484375) expect 0x3FFC' 02006 // docTest_item['funcName'] = 'DACCodeOfVoltage' 02007 // docTest_item['arglist'] = '2.4993896484375' 02008 // docTest_item['expect-value'] = '0x3FFC' 02009 #if MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 02010 // call-function 02011 // ASSERT_EQ(g_MAX5171_device.DACCodeOfVoltage((double)2.4993896484375), (uint16_t)0x3FFC); // 02012 tinyTester.FunctionCall_u_f_Expect("MAX5171.DACCodeOfVoltage", fn_MAX5171_DACCodeOfVoltage, (double)2.4993896484375, /* expect: */ (uint16_t)0x3FFC); // 02013 #endif // MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 02014 02015 // @test group DACCodeOfVoltage DACCodeOfVoltage(1.250152587890625) expect 0x2001 02016 // docTest_item['actionType'] = 'call-function' 02017 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 02018 // docTest_item['action'] = 'DACCodeOfVoltage(1.250152587890625) expect 0x2001' 02019 // docTest_item['funcName'] = 'DACCodeOfVoltage' 02020 // docTest_item['arglist'] = '1.250152587890625' 02021 // docTest_item['expect-value'] = '0x2001' 02022 #if MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 02023 // call-function 02024 // ASSERT_EQ(g_MAX5171_device.DACCodeOfVoltage((double)1.250152587890625), (uint16_t)0x2001); // 02025 tinyTester.FunctionCall_u_f_Expect("MAX5171.DACCodeOfVoltage", fn_MAX5171_DACCodeOfVoltage, (double)1.250152587890625, /* expect: */ (uint16_t)0x2001); // 02026 #endif // MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 02027 02028 // @test group DACCodeOfVoltage DACCodeOfVoltage(1.25) expect 0x2000 02029 // docTest_item['actionType'] = 'call-function' 02030 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 02031 // docTest_item['action'] = 'DACCodeOfVoltage(1.25) expect 0x2000' 02032 // docTest_item['funcName'] = 'DACCodeOfVoltage' 02033 // docTest_item['arglist'] = '1.25' 02034 // docTest_item['expect-value'] = '0x2000' 02035 #if MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 02036 // call-function 02037 // ASSERT_EQ(g_MAX5171_device.DACCodeOfVoltage((double)1.25), (uint16_t)0x2000); // 02038 tinyTester.FunctionCall_u_f_Expect("MAX5171.DACCodeOfVoltage", fn_MAX5171_DACCodeOfVoltage, (double)1.25, /* expect: */ (uint16_t)0x2000); // 02039 #endif // MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 02040 02041 // @test group DACCodeOfVoltage DACCodeOfVoltage(1.249847412109375) expect 0x1FFF 02042 // docTest_item['actionType'] = 'call-function' 02043 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 02044 // docTest_item['action'] = 'DACCodeOfVoltage(1.249847412109375) expect 0x1FFF' 02045 // docTest_item['funcName'] = 'DACCodeOfVoltage' 02046 // docTest_item['arglist'] = '1.249847412109375' 02047 // docTest_item['expect-value'] = '0x1FFF' 02048 #if MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 02049 // call-function 02050 // ASSERT_EQ(g_MAX5171_device.DACCodeOfVoltage((double)1.249847412109375), (uint16_t)0x1FFF); // 02051 tinyTester.FunctionCall_u_f_Expect("MAX5171.DACCodeOfVoltage", fn_MAX5171_DACCodeOfVoltage, (double)1.249847412109375, /* expect: */ (uint16_t)0x1FFF); // 02052 #endif // MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 02053 02054 // @test group DACCodeOfVoltage DACCodeOfVoltage(1.24969482421875) expect 0x1FFE 02055 // docTest_item['actionType'] = 'call-function' 02056 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 02057 // docTest_item['action'] = 'DACCodeOfVoltage(1.24969482421875) expect 0x1FFE' 02058 // docTest_item['funcName'] = 'DACCodeOfVoltage' 02059 // docTest_item['arglist'] = '1.24969482421875' 02060 // docTest_item['expect-value'] = '0x1FFE' 02061 #if MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 02062 // call-function 02063 // ASSERT_EQ(g_MAX5171_device.DACCodeOfVoltage((double)1.24969482421875), (uint16_t)0x1FFE); // 02064 tinyTester.FunctionCall_u_f_Expect("MAX5171.DACCodeOfVoltage", fn_MAX5171_DACCodeOfVoltage, (double)1.24969482421875, /* expect: */ (uint16_t)0x1FFE); // 02065 #endif // MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 02066 02067 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.000457763671875) expect 0x0003 02068 // docTest_item['actionType'] = 'call-function' 02069 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 02070 // docTest_item['action'] = 'DACCodeOfVoltage(0.000457763671875) expect 0x0003' 02071 // docTest_item['funcName'] = 'DACCodeOfVoltage' 02072 // docTest_item['arglist'] = '0.000457763671875' 02073 // docTest_item['expect-value'] = '0x0003' 02074 #if MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 02075 // call-function 02076 // ASSERT_EQ(g_MAX5171_device.DACCodeOfVoltage((double)0.000457763671875), (uint16_t)0x0003); // 02077 tinyTester.FunctionCall_u_f_Expect("MAX5171.DACCodeOfVoltage", fn_MAX5171_DACCodeOfVoltage, (double)0.000457763671875, /* expect: */ (uint16_t)0x0003); // 02078 #endif // MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 02079 02080 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.00030517578125) expect 0x0002 02081 // docTest_item['actionType'] = 'call-function' 02082 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 02083 // docTest_item['action'] = 'DACCodeOfVoltage(0.00030517578125) expect 0x0002' 02084 // docTest_item['funcName'] = 'DACCodeOfVoltage' 02085 // docTest_item['arglist'] = '0.00030517578125' 02086 // docTest_item['expect-value'] = '0x0002' 02087 #if MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 02088 // call-function 02089 // ASSERT_EQ(g_MAX5171_device.DACCodeOfVoltage((double)0.00030517578125), (uint16_t)0x0002); // 02090 tinyTester.FunctionCall_u_f_Expect("MAX5171.DACCodeOfVoltage", fn_MAX5171_DACCodeOfVoltage, (double)0.00030517578125, /* expect: */ (uint16_t)0x0002); // 02091 #endif // MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 02092 02093 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.000152587890625) expect 0x0001 02094 // docTest_item['actionType'] = 'call-function' 02095 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 02096 // docTest_item['action'] = 'DACCodeOfVoltage(0.000152587890625) expect 0x0001' 02097 // docTest_item['funcName'] = 'DACCodeOfVoltage' 02098 // docTest_item['arglist'] = '0.000152587890625' 02099 // docTest_item['expect-value'] = '0x0001' 02100 #if MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 02101 // call-function 02102 // ASSERT_EQ(g_MAX5171_device.DACCodeOfVoltage((double)0.000152587890625), (uint16_t)0x0001); // 02103 tinyTester.FunctionCall_u_f_Expect("MAX5171.DACCodeOfVoltage", fn_MAX5171_DACCodeOfVoltage, (double)0.000152587890625, /* expect: */ (uint16_t)0x0001); // 02104 #endif // MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 02105 02106 // @test group DACCodeOfVoltage DACCodeOfVoltage(0.00000) expect 0x0000 02107 // docTest_item['actionType'] = 'call-function' 02108 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 02109 // docTest_item['action'] = 'DACCodeOfVoltage(0.00000) expect 0x0000' 02110 // docTest_item['funcName'] = 'DACCodeOfVoltage' 02111 // docTest_item['arglist'] = '0.00000' 02112 // docTest_item['expect-value'] = '0x0000' 02113 #if MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage DACCodeOfVoltage 02114 // call-function 02115 // ASSERT_EQ(g_MAX5171_device.DACCodeOfVoltage((double)0.00000), (uint16_t)0x0000); // 02116 tinyTester.FunctionCall_u_f_Expect("MAX5171.DACCodeOfVoltage", fn_MAX5171_DACCodeOfVoltage, (double)0.00000, /* expect: */ (uint16_t)0x0000); // 02117 #endif // MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 02118 02119 // @test group DACCodeOfVoltage tinyTester.blink_time_msec = 75 // default 75 resume hardware self test 02120 // docTest_item['actionType'] = 'assign-propname-value' 02121 // docTest_item['group-id-value'] = 'DACCodeOfVoltage' 02122 // docTest_item['action'] = 'tinyTester.blink_time_msec = 75' 02123 // docTest_item['remarks'] = 'default 75 resume hardware self test' 02124 // docTest_item['propName'] = 'tinyTester.blink_time_msec' 02125 // docTest_item['propValue'] = '75' 02126 #if MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage None default 75 resume hardware self test 02127 // assign-propname-value 02128 // tinyTesterPropName = "tinyTester.blink_time_msec" 02129 // tinyTesterPropValue = "75" 02130 tinyTester.blink_time_msec = 75; 02131 #endif // MAX5171_SELFTEST_DACCodeOfVoltage // group DACCodeOfVoltage 02132 02133 // @test group VoltageOfCode // Verify function VoltageOfCode (enabled by default) 02134 // @test group VoltageOfCode tinyTester.blink_time_msec = 20 // quickly speed through the software verification 02135 // docTest_item['actionType'] = 'assign-propname-value' 02136 // docTest_item['group-id-value'] = 'VoltageOfCode' 02137 // docTest_item['action'] = 'tinyTester.blink_time_msec = 20' 02138 // docTest_item['remarks'] = 'quickly speed through the software verification' 02139 // docTest_item['propName'] = 'tinyTester.blink_time_msec' 02140 // docTest_item['propValue'] = '20' 02141 #if MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode None quickly speed through the software verification 02142 // assign-propname-value 02143 // tinyTesterPropName = "tinyTester.blink_time_msec" 02144 // tinyTesterPropValue = "20" 02145 tinyTester.blink_time_msec = 20; 02146 #endif // MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode 02147 02148 // @test group VoltageOfCode tinyTester.print("VRef = 2.500 MAX5171 14-bit LSB = 0.00015V") 02149 // docTest_item['actionType'] = 'print-string' 02150 // docTest_item['group-id-value'] = 'VoltageOfCode' 02151 // docTest_item['action'] = 'tinyTester.print("VRef = 2.500 MAX5171 14-bit LSB = 0.00015V")' 02152 // docTest_item['arglist'] = 'VRef = 2.500 MAX5171 14-bit LSB = 0.00015V' 02153 #if MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode None 02154 // print-string 02155 // tinyTesterFuncName = "tinyTester.print" 02156 // tinyTesterPrintStringLiteral = "VRef = 2.500 MAX5171 14-bit LSB = 0.00015V" 02157 tinyTester.print("VRef = 2.500 MAX5171 14-bit LSB = 0.00015V"); 02158 #endif // MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode 02159 02160 // @test group VoltageOfCode VRef = 2.500 02161 // docTest_item['actionType'] = 'assign-propname-value' 02162 // docTest_item['group-id-value'] = 'VoltageOfCode' 02163 // docTest_item['action'] = 'VRef = 2.500' 02164 // docTest_item['propName'] = 'VRef' 02165 // docTest_item['propValue'] = '2.500' 02166 #if MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode None 02167 // assign-propname-value 02168 // tinyTesterPropName = "VRef" 02169 // tinyTesterPropValue = "2.500" 02170 g_MAX5171_device.VRef = 2.500; 02171 #endif // MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode 02172 02173 // @test group VoltageOfCode tinyTester.err_threshold = 0.00015259720441921504 // 14-bit LSB (2.500/16383) 02174 // docTest_item['actionType'] = 'assign-propname-value' 02175 // docTest_item['group-id-value'] = 'VoltageOfCode' 02176 // docTest_item['action'] = 'tinyTester.err_threshold = 0.00015259720441921504' 02177 // docTest_item['remarks'] = '14-bit LSB (2.500/16383)' 02178 // docTest_item['propName'] = 'tinyTester.err_threshold' 02179 // docTest_item['propValue'] = '0.00015259720441921504' 02180 #if MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode None 14-bit LSB (2.500/16383) 02181 // assign-propname-value 02182 // tinyTesterPropName = "tinyTester.err_threshold" 02183 // tinyTesterPropValue = "0.00015259720441921504" 02184 tinyTester.err_threshold = 0.00015259720441921504; 02185 #endif // MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode 02186 02187 // @test group VoltageOfCode VoltageOfCode(0x3FFF) expect 2.499847412109375 02188 // docTest_item['actionType'] = 'call-function' 02189 // docTest_item['group-id-value'] = 'VoltageOfCode' 02190 // docTest_item['action'] = 'VoltageOfCode(0x3FFF) expect 2.499847412109375' 02191 // docTest_item['funcName'] = 'VoltageOfCode' 02192 // docTest_item['arglist'] = '0x3FFF' 02193 // docTest_item['expect-value'] = '2.499847412109375' 02194 #if MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode VoltageOfCode 02195 // call-function 02196 // ASSERT_EQ(g_MAX5171_device.VoltageOfCode((uint16_t)0x3FFF), (double)2.499847412109375); // 02197 tinyTester.FunctionCall_f_u_Expect("MAX5171.VoltageOfCode", fn_MAX5171_VoltageOfCode, (uint16_t)0x3FFF, /* expect: */ (double)2.499847412109375); // 02198 #endif // MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode 02199 02200 // @test group VoltageOfCode VoltageOfCode(0x3FFE) expect 2.49969482421875 02201 // docTest_item['actionType'] = 'call-function' 02202 // docTest_item['group-id-value'] = 'VoltageOfCode' 02203 // docTest_item['action'] = 'VoltageOfCode(0x3FFE) expect 2.49969482421875' 02204 // docTest_item['funcName'] = 'VoltageOfCode' 02205 // docTest_item['arglist'] = '0x3FFE' 02206 // docTest_item['expect-value'] = '2.49969482421875' 02207 #if MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode VoltageOfCode 02208 // call-function 02209 // ASSERT_EQ(g_MAX5171_device.VoltageOfCode((uint16_t)0x3FFE), (double)2.49969482421875); // 02210 tinyTester.FunctionCall_f_u_Expect("MAX5171.VoltageOfCode", fn_MAX5171_VoltageOfCode, (uint16_t)0x3FFE, /* expect: */ (double)2.49969482421875); // 02211 #endif // MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode 02212 02213 // @test group VoltageOfCode VoltageOfCode(0x3FFD) expect 2.499542236328125 02214 // docTest_item['actionType'] = 'call-function' 02215 // docTest_item['group-id-value'] = 'VoltageOfCode' 02216 // docTest_item['action'] = 'VoltageOfCode(0x3FFD) expect 2.499542236328125' 02217 // docTest_item['funcName'] = 'VoltageOfCode' 02218 // docTest_item['arglist'] = '0x3FFD' 02219 // docTest_item['expect-value'] = '2.499542236328125' 02220 #if MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode VoltageOfCode 02221 // call-function 02222 // ASSERT_EQ(g_MAX5171_device.VoltageOfCode((uint16_t)0x3FFD), (double)2.499542236328125); // 02223 tinyTester.FunctionCall_f_u_Expect("MAX5171.VoltageOfCode", fn_MAX5171_VoltageOfCode, (uint16_t)0x3FFD, /* expect: */ (double)2.499542236328125); // 02224 #endif // MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode 02225 02226 // @test group VoltageOfCode VoltageOfCode(0x3FFC) expect 2.4993896484375 02227 // docTest_item['actionType'] = 'call-function' 02228 // docTest_item['group-id-value'] = 'VoltageOfCode' 02229 // docTest_item['action'] = 'VoltageOfCode(0x3FFC) expect 2.4993896484375' 02230 // docTest_item['funcName'] = 'VoltageOfCode' 02231 // docTest_item['arglist'] = '0x3FFC' 02232 // docTest_item['expect-value'] = '2.4993896484375' 02233 #if MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode VoltageOfCode 02234 // call-function 02235 // ASSERT_EQ(g_MAX5171_device.VoltageOfCode((uint16_t)0x3FFC), (double)2.4993896484375); // 02236 tinyTester.FunctionCall_f_u_Expect("MAX5171.VoltageOfCode", fn_MAX5171_VoltageOfCode, (uint16_t)0x3FFC, /* expect: */ (double)2.4993896484375); // 02237 #endif // MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode 02238 02239 // @test group VoltageOfCode VoltageOfCode(0x2001) expect 1.250152587890625 02240 // docTest_item['actionType'] = 'call-function' 02241 // docTest_item['group-id-value'] = 'VoltageOfCode' 02242 // docTest_item['action'] = 'VoltageOfCode(0x2001) expect 1.250152587890625' 02243 // docTest_item['funcName'] = 'VoltageOfCode' 02244 // docTest_item['arglist'] = '0x2001' 02245 // docTest_item['expect-value'] = '1.250152587890625' 02246 #if MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode VoltageOfCode 02247 // call-function 02248 // ASSERT_EQ(g_MAX5171_device.VoltageOfCode((uint16_t)0x2001), (double)1.250152587890625); // 02249 tinyTester.FunctionCall_f_u_Expect("MAX5171.VoltageOfCode", fn_MAX5171_VoltageOfCode, (uint16_t)0x2001, /* expect: */ (double)1.250152587890625); // 02250 #endif // MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode 02251 02252 // @test group VoltageOfCode VoltageOfCode(0x2000) expect 1.25 02253 // docTest_item['actionType'] = 'call-function' 02254 // docTest_item['group-id-value'] = 'VoltageOfCode' 02255 // docTest_item['action'] = 'VoltageOfCode(0x2000) expect 1.25' 02256 // docTest_item['funcName'] = 'VoltageOfCode' 02257 // docTest_item['arglist'] = '0x2000' 02258 // docTest_item['expect-value'] = '1.25' 02259 #if MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode VoltageOfCode 02260 // call-function 02261 // ASSERT_EQ(g_MAX5171_device.VoltageOfCode((uint16_t)0x2000), (double)1.25); // 02262 tinyTester.FunctionCall_f_u_Expect("MAX5171.VoltageOfCode", fn_MAX5171_VoltageOfCode, (uint16_t)0x2000, /* expect: */ (double)1.25); // 02263 #endif // MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode 02264 02265 // @test group VoltageOfCode VoltageOfCode(0x1FFF) expect 1.249847412109375 02266 // docTest_item['actionType'] = 'call-function' 02267 // docTest_item['group-id-value'] = 'VoltageOfCode' 02268 // docTest_item['action'] = 'VoltageOfCode(0x1FFF) expect 1.249847412109375' 02269 // docTest_item['funcName'] = 'VoltageOfCode' 02270 // docTest_item['arglist'] = '0x1FFF' 02271 // docTest_item['expect-value'] = '1.249847412109375' 02272 #if MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode VoltageOfCode 02273 // call-function 02274 // ASSERT_EQ(g_MAX5171_device.VoltageOfCode((uint16_t)0x1FFF), (double)1.249847412109375); // 02275 tinyTester.FunctionCall_f_u_Expect("MAX5171.VoltageOfCode", fn_MAX5171_VoltageOfCode, (uint16_t)0x1FFF, /* expect: */ (double)1.249847412109375); // 02276 #endif // MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode 02277 02278 // @test group VoltageOfCode VoltageOfCode(0x1FFE) expect 1.24969482421875 02279 // docTest_item['actionType'] = 'call-function' 02280 // docTest_item['group-id-value'] = 'VoltageOfCode' 02281 // docTest_item['action'] = 'VoltageOfCode(0x1FFE) expect 1.24969482421875' 02282 // docTest_item['funcName'] = 'VoltageOfCode' 02283 // docTest_item['arglist'] = '0x1FFE' 02284 // docTest_item['expect-value'] = '1.24969482421875' 02285 #if MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode VoltageOfCode 02286 // call-function 02287 // ASSERT_EQ(g_MAX5171_device.VoltageOfCode((uint16_t)0x1FFE), (double)1.24969482421875); // 02288 tinyTester.FunctionCall_f_u_Expect("MAX5171.VoltageOfCode", fn_MAX5171_VoltageOfCode, (uint16_t)0x1FFE, /* expect: */ (double)1.24969482421875); // 02289 #endif // MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode 02290 02291 // @test group VoltageOfCode VoltageOfCode(0x0003) expect 0.000457763671875 02292 // docTest_item['actionType'] = 'call-function' 02293 // docTest_item['group-id-value'] = 'VoltageOfCode' 02294 // docTest_item['action'] = 'VoltageOfCode(0x0003) expect 0.000457763671875' 02295 // docTest_item['funcName'] = 'VoltageOfCode' 02296 // docTest_item['arglist'] = '0x0003' 02297 // docTest_item['expect-value'] = '0.000457763671875' 02298 #if MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode VoltageOfCode 02299 // call-function 02300 // ASSERT_EQ(g_MAX5171_device.VoltageOfCode((uint16_t)0x0003), (double)0.000457763671875); // 02301 tinyTester.FunctionCall_f_u_Expect("MAX5171.VoltageOfCode", fn_MAX5171_VoltageOfCode, (uint16_t)0x0003, /* expect: */ (double)0.000457763671875); // 02302 #endif // MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode 02303 02304 // @test group VoltageOfCode VoltageOfCode(0x0002) expect 0.00030517578125 02305 // docTest_item['actionType'] = 'call-function' 02306 // docTest_item['group-id-value'] = 'VoltageOfCode' 02307 // docTest_item['action'] = 'VoltageOfCode(0x0002) expect 0.00030517578125' 02308 // docTest_item['funcName'] = 'VoltageOfCode' 02309 // docTest_item['arglist'] = '0x0002' 02310 // docTest_item['expect-value'] = '0.00030517578125' 02311 #if MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode VoltageOfCode 02312 // call-function 02313 // ASSERT_EQ(g_MAX5171_device.VoltageOfCode((uint16_t)0x0002), (double)0.00030517578125); // 02314 tinyTester.FunctionCall_f_u_Expect("MAX5171.VoltageOfCode", fn_MAX5171_VoltageOfCode, (uint16_t)0x0002, /* expect: */ (double)0.00030517578125); // 02315 #endif // MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode 02316 02317 // @test group VoltageOfCode VoltageOfCode(0x0001) expect 0.000152587890625 02318 // docTest_item['actionType'] = 'call-function' 02319 // docTest_item['group-id-value'] = 'VoltageOfCode' 02320 // docTest_item['action'] = 'VoltageOfCode(0x0001) expect 0.000152587890625' 02321 // docTest_item['funcName'] = 'VoltageOfCode' 02322 // docTest_item['arglist'] = '0x0001' 02323 // docTest_item['expect-value'] = '0.000152587890625' 02324 #if MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode VoltageOfCode 02325 // call-function 02326 // ASSERT_EQ(g_MAX5171_device.VoltageOfCode((uint16_t)0x0001), (double)0.000152587890625); // 02327 tinyTester.FunctionCall_f_u_Expect("MAX5171.VoltageOfCode", fn_MAX5171_VoltageOfCode, (uint16_t)0x0001, /* expect: */ (double)0.000152587890625); // 02328 #endif // MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode 02329 02330 // @test group VoltageOfCode VoltageOfCode(0x0000) expect 0.00000 02331 // docTest_item['actionType'] = 'call-function' 02332 // docTest_item['group-id-value'] = 'VoltageOfCode' 02333 // docTest_item['action'] = 'VoltageOfCode(0x0000) expect 0.00000' 02334 // docTest_item['funcName'] = 'VoltageOfCode' 02335 // docTest_item['arglist'] = '0x0000' 02336 // docTest_item['expect-value'] = '0.00000' 02337 #if MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode VoltageOfCode 02338 // call-function 02339 // ASSERT_EQ(g_MAX5171_device.VoltageOfCode((uint16_t)0x0000), (double)0.00000); // 02340 tinyTester.FunctionCall_f_u_Expect("MAX5171.VoltageOfCode", fn_MAX5171_VoltageOfCode, (uint16_t)0x0000, /* expect: */ (double)0.00000); // 02341 #endif // MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode 02342 02343 // @test group VoltageOfCode tinyTester.blink_time_msec = 75 // default 75 resume hardware self test 02344 // docTest_item['actionType'] = 'assign-propname-value' 02345 // docTest_item['group-id-value'] = 'VoltageOfCode' 02346 // docTest_item['action'] = 'tinyTester.blink_time_msec = 75' 02347 // docTest_item['remarks'] = 'default 75 resume hardware self test' 02348 // docTest_item['propName'] = 'tinyTester.blink_time_msec' 02349 // docTest_item['propValue'] = '75' 02350 #if MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode None default 75 resume hardware self test 02351 // assign-propname-value 02352 // tinyTesterPropName = "tinyTester.blink_time_msec" 02353 // tinyTesterPropValue = "75" 02354 tinyTester.blink_time_msec = 75; 02355 #endif // MAX5171_SELFTEST_VoltageOfCode // group VoltageOfCode 02356 02357 // @test group CODE_LOAD // Verify function CODE_LOAD (enabled by default) 02358 // @test group CODE_LOAD tinyTester.blink_time_msec = 75 // default 75 resume hardware self test 02359 // docTest_item['actionType'] = 'assign-propname-value' 02360 // docTest_item['group-id-value'] = 'CODE_LOAD' 02361 // docTest_item['action'] = 'tinyTester.blink_time_msec = 75' 02362 // docTest_item['remarks'] = 'default 75 resume hardware self test' 02363 // docTest_item['propName'] = 'tinyTester.blink_time_msec' 02364 // docTest_item['propValue'] = '75' 02365 #if MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD None default 75 resume hardware self test 02366 // assign-propname-value 02367 // tinyTesterPropName = "tinyTester.blink_time_msec" 02368 // tinyTesterPropValue = "75" 02369 tinyTester.blink_time_msec = 75; 02370 #endif // MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD 02371 02372 // @test group CODE_LOAD tinyTester.settle_time_msec = 500 02373 // docTest_item['actionType'] = 'assign-propname-value' 02374 // docTest_item['group-id-value'] = 'CODE_LOAD' 02375 // docTest_item['action'] = 'tinyTester.settle_time_msec = 500' 02376 // docTest_item['propName'] = 'tinyTester.settle_time_msec' 02377 // docTest_item['propValue'] = '500' 02378 #if MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD None 02379 // assign-propname-value 02380 // tinyTesterPropName = "tinyTester.settle_time_msec" 02381 // tinyTesterPropValue = "500" 02382 tinyTester.settle_time_msec = 500; 02383 #endif // MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD 02384 02385 // @test Init() 02386 // docTest_item['actionType'] = 'call-function' 02387 // docTest_item['action'] = 'Init()' 02388 // docTest_item['funcName'] = 'Init' 02389 // call-function 02390 // ASSERT_EQ(g_MAX5171_device.Init(()), (void)None); // 02391 // tinyTester.FunctionCall_Expect("MAX5171.Init", fn_MAX5171_Init, /* empty docTest_argList */ /* empty expect: */ (void)None); // 02392 g_MAX5171_device.Init(); // 02393 02394 // @test VRef expect 2.500 // Nominal Full-Scale Voltage Reference 02395 // docTest_item['actionType'] = 'test-propname-expect-value' 02396 // docTest_item['action'] = 'VRef expect 2.500' 02397 // docTest_item['remarks'] = 'Nominal Full-Scale Voltage Reference' 02398 // docTest_item['expect-value'] = '2.500' 02399 // docTest_item['propName'] = 'VRef' 02400 // test-propname-expect-value 02401 tinyTester.Expect("MAX5171.VRef", g_MAX5171_device.VRef, /* expect: */ 2.500); // Nominal Full-Scale Voltage Reference 02402 02403 // @test group CODE_LOAD tinyTester.err_threshold = 0.050 02404 // docTest_item['actionType'] = 'assign-propname-value' 02405 // docTest_item['group-id-value'] = 'CODE_LOAD' 02406 // docTest_item['action'] = 'tinyTester.err_threshold = 0.050' 02407 // docTest_item['propName'] = 'tinyTester.err_threshold' 02408 // docTest_item['propValue'] = '0.050' 02409 #if MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD None 02410 // assign-propname-value 02411 // tinyTesterPropName = "tinyTester.err_threshold" 02412 // tinyTesterPropValue = "0.050" 02413 tinyTester.err_threshold = 0.050; 02414 #endif // MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD 02415 02416 // @test group CODE_LOAD tinyTester.print("100.0% of full scale REF(2.50V) = 2.50V Jumper FB=1-2") 02417 // docTest_item['actionType'] = 'print-string' 02418 // docTest_item['group-id-value'] = 'CODE_LOAD' 02419 // docTest_item['action'] = 'tinyTester.print("100.0% of full scale REF(2.50V) = 2.50V Jumper FB=1-2")' 02420 // docTest_item['arglist'] = '100.0% of full scale REF(2.50V) = 2.50V Jumper FB=1-2' 02421 #if MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD None 02422 // print-string 02423 // tinyTesterFuncName = "tinyTester.print" 02424 // tinyTesterPrintStringLiteral = "100.0% of full scale REF(2.50V) = 2.50V Jumper FB=1-2" 02425 tinyTester.print("100.0% of full scale REF(2.50V) = 2.50V Jumper FB=1-2"); 02426 #endif // MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD 02427 02428 // @test group CODE_LOAD CODE_LOAD(0x3FFF) // 100.0% of full scale REF(2.50V) = 2.50V 02429 // docTest_item['actionType'] = 'call-function' 02430 // docTest_item['group-id-value'] = 'CODE_LOAD' 02431 // docTest_item['action'] = 'CODE_LOAD(0x3FFF)' 02432 // docTest_item['remarks'] = '100.0% of full scale REF(2.50V) = 2.50V' 02433 // docTest_item['funcName'] = 'CODE_LOAD' 02434 // docTest_item['arglist'] = '0x3FFF' 02435 #if MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD CODE_LOAD 100.0% of full scale REF(2.50V) = 2.50V 02436 // call-function 02437 // ASSERT_EQ(g_MAX5171_device.CODE_LOAD((uint16_t)0x3FFF), (uint8_t)None); // 100.0% of full scale REF(2.50V) = 2.50V 02438 // tinyTester.FunctionCall_Expect("MAX5171.CODE_LOAD", fn_MAX5171_CODE_LOAD, (uint16_t)0x3FFF, /* empty expect: */ (uint8_t)None); // 100.0% of full scale REF(2.50V) = 2.50V 02439 g_MAX5171_device.CODE_LOAD((uint16_t)0x3FFF); // 100.0% of full scale REF(2.50V) = 2.50V 02440 #endif // MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD 02441 02442 // @test group CODE_LOAD tinyTester.Wait_Output_Settling() 02443 // docTest_item['actionType'] = 'call-tinytester-function' 02444 // docTest_item['group-id-value'] = 'CODE_LOAD' 02445 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 02446 // docTest_item['propName'] = 'Wait_Output_Settling' 02447 #if MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD None 02448 // call-tinytester-function 02449 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 02450 // docTest_argList = "" 02451 tinyTester.Wait_Output_Settling(); // 02452 #endif // MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD 02453 02454 // @test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(2.500) 02455 // docTest_item['actionType'] = 'call-tinytester-function' 02456 // docTest_item['group-id-value'] = 'CODE_LOAD' 02457 // docTest_item['action'] = 'tinyTester.AnalogIn0_Read_Expect_voltageV(2.500)' 02458 // docTest_item['arglist'] = '2.500' 02459 // docTest_item['propName'] = 'AnalogIn0_Read_Expect_voltageV' 02460 #if MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD None 02461 // call-tinytester-function 02462 // tinyTesterFuncName = "tinyTester.AnalogIn0_Read_Expect_voltageV" 02463 // docTest_argList = "2.500" 02464 tinyTester.AnalogIn0_Read_Expect_voltageV(2.500); // 02465 #endif // MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD 02466 02467 // @test group CODE_LOAD tinyTester.print("0.0% of full scale REF(2.50V) = 0.000V") 02468 // docTest_item['actionType'] = 'print-string' 02469 // docTest_item['group-id-value'] = 'CODE_LOAD' 02470 // docTest_item['action'] = 'tinyTester.print("0.0% of full scale REF(2.50V) = 0.000V")' 02471 // docTest_item['arglist'] = '0.0% of full scale REF(2.50V) = 0.000V' 02472 #if MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD None 02473 // print-string 02474 // tinyTesterFuncName = "tinyTester.print" 02475 // tinyTesterPrintStringLiteral = "0.0% of full scale REF(2.50V) = 0.000V" 02476 tinyTester.print("0.0% of full scale REF(2.50V) = 0.000V"); 02477 #endif // MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD 02478 02479 // @test group CODE_LOAD CODE_LOAD(0x0000) // 0.0% of full scale REF(2.50V) = 0.000V 02480 // docTest_item['actionType'] = 'call-function' 02481 // docTest_item['group-id-value'] = 'CODE_LOAD' 02482 // docTest_item['action'] = 'CODE_LOAD(0x0000)' 02483 // docTest_item['remarks'] = '0.0% of full scale REF(2.50V) = 0.000V' 02484 // docTest_item['funcName'] = 'CODE_LOAD' 02485 // docTest_item['arglist'] = '0x0000' 02486 #if MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD CODE_LOAD 0.0% of full scale REF(2.50V) = 0.000V 02487 // call-function 02488 // ASSERT_EQ(g_MAX5171_device.CODE_LOAD((uint16_t)0x0000), (uint8_t)None); // 0.0% of full scale REF(2.50V) = 0.000V 02489 // tinyTester.FunctionCall_Expect("MAX5171.CODE_LOAD", fn_MAX5171_CODE_LOAD, (uint16_t)0x0000, /* empty expect: */ (uint8_t)None); // 0.0% of full scale REF(2.50V) = 0.000V 02490 g_MAX5171_device.CODE_LOAD((uint16_t)0x0000); // 0.0% of full scale REF(2.50V) = 0.000V 02491 #endif // MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD 02492 02493 // @test group CODE_LOAD tinyTester.Wait_Output_Settling() 02494 // docTest_item['actionType'] = 'call-tinytester-function' 02495 // docTest_item['group-id-value'] = 'CODE_LOAD' 02496 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 02497 // docTest_item['propName'] = 'Wait_Output_Settling' 02498 #if MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD None 02499 // call-tinytester-function 02500 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 02501 // docTest_argList = "" 02502 tinyTester.Wait_Output_Settling(); // 02503 #endif // MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD 02504 02505 // @test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(0.0000) 02506 // docTest_item['actionType'] = 'call-tinytester-function' 02507 // docTest_item['group-id-value'] = 'CODE_LOAD' 02508 // docTest_item['action'] = 'tinyTester.AnalogIn0_Read_Expect_voltageV(0.0000)' 02509 // docTest_item['arglist'] = '0.0000' 02510 // docTest_item['propName'] = 'AnalogIn0_Read_Expect_voltageV' 02511 #if MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD None 02512 // call-tinytester-function 02513 // tinyTesterFuncName = "tinyTester.AnalogIn0_Read_Expect_voltageV" 02514 // docTest_argList = "0.0000" 02515 tinyTester.AnalogIn0_Read_Expect_voltageV(0.0000); // 02516 #endif // MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD 02517 02518 // @test group CODE_LOAD tinyTester.print("50.0% of full scale REF(2.50V) = 1.25V") 02519 // docTest_item['actionType'] = 'print-string' 02520 // docTest_item['group-id-value'] = 'CODE_LOAD' 02521 // docTest_item['action'] = 'tinyTester.print("50.0% of full scale REF(2.50V) = 1.25V")' 02522 // docTest_item['arglist'] = '50.0% of full scale REF(2.50V) = 1.25V' 02523 #if MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD None 02524 // print-string 02525 // tinyTesterFuncName = "tinyTester.print" 02526 // tinyTesterPrintStringLiteral = "50.0% of full scale REF(2.50V) = 1.25V" 02527 tinyTester.print("50.0% of full scale REF(2.50V) = 1.25V"); 02528 #endif // MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD 02529 02530 // @test group CODE_LOAD CODE_LOAD(0x1FFF) // 50.0% of full scale REF(2.50V) = 1.25V 02531 // docTest_item['actionType'] = 'call-function' 02532 // docTest_item['group-id-value'] = 'CODE_LOAD' 02533 // docTest_item['action'] = 'CODE_LOAD(0x1FFF)' 02534 // docTest_item['remarks'] = '50.0% of full scale REF(2.50V) = 1.25V' 02535 // docTest_item['funcName'] = 'CODE_LOAD' 02536 // docTest_item['arglist'] = '0x1FFF' 02537 #if MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD CODE_LOAD 50.0% of full scale REF(2.50V) = 1.25V 02538 // call-function 02539 // ASSERT_EQ(g_MAX5171_device.CODE_LOAD((uint16_t)0x1FFF), (uint8_t)None); // 50.0% of full scale REF(2.50V) = 1.25V 02540 // tinyTester.FunctionCall_Expect("MAX5171.CODE_LOAD", fn_MAX5171_CODE_LOAD, (uint16_t)0x1FFF, /* empty expect: */ (uint8_t)None); // 50.0% of full scale REF(2.50V) = 1.25V 02541 g_MAX5171_device.CODE_LOAD((uint16_t)0x1FFF); // 50.0% of full scale REF(2.50V) = 1.25V 02542 #endif // MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD 02543 02544 // @test group CODE_LOAD tinyTester.Wait_Output_Settling() 02545 // docTest_item['actionType'] = 'call-tinytester-function' 02546 // docTest_item['group-id-value'] = 'CODE_LOAD' 02547 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 02548 // docTest_item['propName'] = 'Wait_Output_Settling' 02549 #if MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD None 02550 // call-tinytester-function 02551 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 02552 // docTest_argList = "" 02553 tinyTester.Wait_Output_Settling(); // 02554 #endif // MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD 02555 02556 // @test group CODE_LOAD tinyTester.AnalogIn0_Read_Expect_voltageV(1.2500) 02557 // docTest_item['actionType'] = 'call-tinytester-function' 02558 // docTest_item['group-id-value'] = 'CODE_LOAD' 02559 // docTest_item['action'] = 'tinyTester.AnalogIn0_Read_Expect_voltageV(1.2500)' 02560 // docTest_item['arglist'] = '1.2500' 02561 // docTest_item['propName'] = 'AnalogIn0_Read_Expect_voltageV' 02562 #if MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD None 02563 // call-tinytester-function 02564 // tinyTesterFuncName = "tinyTester.AnalogIn0_Read_Expect_voltageV" 02565 // docTest_argList = "1.2500" 02566 tinyTester.AnalogIn0_Read_Expect_voltageV(1.2500); // 02567 #endif // MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD 02568 02569 // @test group UPO // Verify User Programmable Output functions UPO_HIGH and UPO_LOW (enabled by default) 02570 // @test group UPO tinyTester.blink_time_msec = 75 // default 75 resume hardware self test 02571 // docTest_item['actionType'] = 'assign-propname-value' 02572 // docTest_item['group-id-value'] = 'UPO' 02573 // docTest_item['action'] = 'tinyTester.blink_time_msec = 75' 02574 // docTest_item['remarks'] = 'default 75 resume hardware self test' 02575 // docTest_item['propName'] = 'tinyTester.blink_time_msec' 02576 // docTest_item['propValue'] = '75' 02577 #if MAX5171_SELFTEST_UPO // group UPO None default 75 resume hardware self test 02578 // assign-propname-value 02579 // tinyTesterPropName = "tinyTester.blink_time_msec" 02580 // tinyTesterPropValue = "75" 02581 tinyTester.blink_time_msec = 75; 02582 #endif // MAX5171_SELFTEST_UPO // group UPO 02583 02584 // @test group UPO tinyTester.settle_time_msec = 500 // default 250 02585 // docTest_item['actionType'] = 'assign-propname-value' 02586 // docTest_item['group-id-value'] = 'UPO' 02587 // docTest_item['action'] = 'tinyTester.settle_time_msec = 500' 02588 // docTest_item['remarks'] = 'default 250' 02589 // docTest_item['propName'] = 'tinyTester.settle_time_msec' 02590 // docTest_item['propValue'] = '500' 02591 #if MAX5171_SELFTEST_UPO // group UPO None default 250 02592 // assign-propname-value 02593 // tinyTesterPropName = "tinyTester.settle_time_msec" 02594 // tinyTesterPropValue = "500" 02595 tinyTester.settle_time_msec = 500; 02596 #endif // MAX5171_SELFTEST_UPO // group UPO 02597 02598 // @test group UPO UPO_HIGH() 02599 // docTest_item['actionType'] = 'call-function' 02600 // docTest_item['group-id-value'] = 'UPO' 02601 // docTest_item['action'] = 'UPO_HIGH()' 02602 // docTest_item['funcName'] = 'UPO_HIGH' 02603 #if MAX5171_SELFTEST_UPO // group UPO UPO_HIGH 02604 // call-function 02605 // ASSERT_EQ(g_MAX5171_device.UPO_HIGH(()), (uint8_t)None); // 02606 // tinyTester.FunctionCall_Expect("MAX5171.UPO_HIGH", fn_MAX5171_UPO_HIGH, /* empty docTest_argList */ /* empty expect: */ (uint8_t)None); // 02607 g_MAX5171_device.UPO_HIGH(); // 02608 #endif // MAX5171_SELFTEST_UPO // group UPO 02609 02610 // @test group UPO tinyTester.Wait_Output_Settling() 02611 // docTest_item['actionType'] = 'call-tinytester-function' 02612 // docTest_item['group-id-value'] = 'UPO' 02613 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 02614 // docTest_item['propName'] = 'Wait_Output_Settling' 02615 #if MAX5171_SELFTEST_UPO // group UPO None 02616 // call-tinytester-function 02617 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 02618 // docTest_argList = "" 02619 tinyTester.Wait_Output_Settling(); // 02620 #endif // MAX5171_SELFTEST_UPO // group UPO 02621 02622 // @test group CODE_LOAD tinyTester.DigitalIn_Read_Expect_WarnOnly(UPO_pin, "UPO", 1, "UPO_pin is high after MAX5171 UPO_HIGH command") 02623 // docTest_item['actionType'] = 'call-tinytester-function' 02624 // docTest_item['group-id-value'] = 'CODE_LOAD' 02625 // docTest_item['action'] = 'tinyTester.DigitalIn_Read_Expect_WarnOnly(UPO_pin, "UPO", 1, "UPO_pin is high after MAX5171 UPO_HIGH command")' 02626 // docTest_item['arglist'] = 'UPO_pin, "UPO", 1, "UPO_pin is high after MAX5171 UPO_HIGH command"' 02627 // docTest_item['propName'] = 'DigitalIn_Read_Expect_WarnOnly' 02628 #if MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD None 02629 // call-tinytester-function 02630 // tinyTesterFuncName = "tinyTester.DigitalIn_Read_Expect_WarnOnly" 02631 // docTest_argList = "UPO_pin, "UPO", 1, "UPO_pin is high after MAX5171 UPO_HIGH command"" 02632 tinyTester.DigitalIn_Read_Expect_WarnOnly(UPO_pin, "UPO", 1, "UPO_pin is high after MAX5171 UPO_HIGH command"); // 02633 #endif // MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD 02634 02635 // @test group UPO UPO_LOW() 02636 // docTest_item['actionType'] = 'call-function' 02637 // docTest_item['group-id-value'] = 'UPO' 02638 // docTest_item['action'] = 'UPO_LOW()' 02639 // docTest_item['funcName'] = 'UPO_LOW' 02640 #if MAX5171_SELFTEST_UPO // group UPO UPO_LOW 02641 // call-function 02642 // ASSERT_EQ(g_MAX5171_device.UPO_LOW(()), (uint8_t)None); // 02643 // tinyTester.FunctionCall_Expect("MAX5171.UPO_LOW", fn_MAX5171_UPO_LOW, /* empty docTest_argList */ /* empty expect: */ (uint8_t)None); // 02644 g_MAX5171_device.UPO_LOW(); // 02645 #endif // MAX5171_SELFTEST_UPO // group UPO 02646 02647 // @test group UPO tinyTester.Wait_Output_Settling() 02648 // docTest_item['actionType'] = 'call-tinytester-function' 02649 // docTest_item['group-id-value'] = 'UPO' 02650 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 02651 // docTest_item['propName'] = 'Wait_Output_Settling' 02652 #if MAX5171_SELFTEST_UPO // group UPO None 02653 // call-tinytester-function 02654 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 02655 // docTest_argList = "" 02656 tinyTester.Wait_Output_Settling(); // 02657 #endif // MAX5171_SELFTEST_UPO // group UPO 02658 02659 // @test group CODE_LOAD tinyTester.DigitalIn_Read_Expect_WarnOnly(UPO_pin, "UPO", 0, "UPO_pin is low after MAX5171 UPO_LOW command") 02660 // docTest_item['actionType'] = 'call-tinytester-function' 02661 // docTest_item['group-id-value'] = 'CODE_LOAD' 02662 // docTest_item['action'] = 'tinyTester.DigitalIn_Read_Expect_WarnOnly(UPO_pin, "UPO", 0, "UPO_pin is low after MAX5171 UPO_LOW command")' 02663 // docTest_item['arglist'] = 'UPO_pin, "UPO", 0, "UPO_pin is low after MAX5171 UPO_LOW command"' 02664 // docTest_item['propName'] = 'DigitalIn_Read_Expect_WarnOnly' 02665 #if MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD None 02666 // call-tinytester-function 02667 // tinyTesterFuncName = "tinyTester.DigitalIn_Read_Expect_WarnOnly" 02668 // docTest_argList = "UPO_pin, "UPO", 0, "UPO_pin is low after MAX5171 UPO_LOW command"" 02669 tinyTester.DigitalIn_Read_Expect_WarnOnly(UPO_pin, "UPO", 0, "UPO_pin is low after MAX5171 UPO_LOW command"); // 02670 #endif // MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD 02671 02672 // @test group UPO UPO_HIGH() 02673 // docTest_item['actionType'] = 'call-function' 02674 // docTest_item['group-id-value'] = 'UPO' 02675 // docTest_item['action'] = 'UPO_HIGH()' 02676 // docTest_item['funcName'] = 'UPO_HIGH' 02677 #if MAX5171_SELFTEST_UPO // group UPO UPO_HIGH 02678 // call-function 02679 // ASSERT_EQ(g_MAX5171_device.UPO_HIGH(()), (uint8_t)None); // 02680 // tinyTester.FunctionCall_Expect("MAX5171.UPO_HIGH", fn_MAX5171_UPO_HIGH, /* empty docTest_argList */ /* empty expect: */ (uint8_t)None); // 02681 g_MAX5171_device.UPO_HIGH(); // 02682 #endif // MAX5171_SELFTEST_UPO // group UPO 02683 02684 // @test group UPO tinyTester.Wait_Output_Settling() 02685 // docTest_item['actionType'] = 'call-tinytester-function' 02686 // docTest_item['group-id-value'] = 'UPO' 02687 // docTest_item['action'] = 'tinyTester.Wait_Output_Settling()' 02688 // docTest_item['propName'] = 'Wait_Output_Settling' 02689 #if MAX5171_SELFTEST_UPO // group UPO None 02690 // call-tinytester-function 02691 // tinyTesterFuncName = "tinyTester.Wait_Output_Settling" 02692 // docTest_argList = "" 02693 tinyTester.Wait_Output_Settling(); // 02694 #endif // MAX5171_SELFTEST_UPO // group UPO 02695 02696 // @test group CODE_LOAD tinyTester.DigitalIn_Read_Expect_WarnOnly(UPO_pin, "UPO", 1, "UPO_pin is high after MAX5171 UPO_HIGH command") 02697 // docTest_item['actionType'] = 'call-tinytester-function' 02698 // docTest_item['group-id-value'] = 'CODE_LOAD' 02699 // docTest_item['action'] = 'tinyTester.DigitalIn_Read_Expect_WarnOnly(UPO_pin, "UPO", 1, "UPO_pin is high after MAX5171 UPO_HIGH command")' 02700 // docTest_item['arglist'] = 'UPO_pin, "UPO", 1, "UPO_pin is high after MAX5171 UPO_HIGH command"' 02701 // docTest_item['propName'] = 'DigitalIn_Read_Expect_WarnOnly' 02702 #if MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD None 02703 // call-tinytester-function 02704 // tinyTesterFuncName = "tinyTester.DigitalIn_Read_Expect_WarnOnly" 02705 // docTest_argList = "UPO_pin, "UPO", 1, "UPO_pin is high after MAX5171 UPO_HIGH command"" 02706 tinyTester.DigitalIn_Read_Expect_WarnOnly(UPO_pin, "UPO", 1, "UPO_pin is high after MAX5171 UPO_HIGH command"); // 02707 #endif // MAX5171_SELFTEST_CODE_LOAD // group CODE_LOAD 02708 02709 // 02710 #if INJECT_SELFTEST_FAIL 02711 // Test of the pass/fail report mechanism 02712 tinyTester.FAIL(); 02713 cmdLine.serial().print(F("injecting one false failure for test reporting")); 02714 #endif 02715 // 02716 // Report number of pass and number of fail test results 02717 tinyTester.Report_Summary(); 02718 } 02719 02720 //-------------------------------------------------- 02721 // selfTestFunctionClosures[functionName]['functionName'] = 'DACCodeOfVoltage' 02722 // selfTestFunctionClosures[functionName]['argListDeclaration'] = 'double voltageV' 02723 // selfTestFunctionClosures[functionName]['returnType'] = 'uint16_t' 02724 // selfTestFunctionClosures[functionName]['argNames'] = 'voltageV' 02725 // CommandParamIn_declaration = 'double voltageV' 02726 // argNames_recast_implementation = '(double)voltageV' 02727 //-------------------------------------------------- 02728 // selftest: define function under test 02729 // uint16_t MAX5171::DACCodeOfVoltage(double voltageV) 02730 uint16_t fn_MAX5171_DACCodeOfVoltage(double voltageV) 02731 { 02732 return g_MAX5171_device.DACCodeOfVoltage((double)voltageV); 02733 } 02734 02735 //-------------------------------------------------- 02736 // selfTestFunctionClosures[functionName]['functionName'] = 'VoltageOfCode' 02737 // selfTestFunctionClosures[functionName]['argListDeclaration'] = 'uint16_t value_u14' 02738 // selfTestFunctionClosures[functionName]['returnType'] = 'double' 02739 // selfTestFunctionClosures[functionName]['argNames'] = 'value_u14' 02740 // CommandParamIn_declaration = 'uint16_t value_u14' 02741 // argNames_recast_implementation = '(uint16_t)value_u14' 02742 //-------------------------------------------------- 02743 // selftest: define function under test 02744 // double MAX5171::VoltageOfCode(uint16_t value_u14) 02745 double fn_MAX5171_VoltageOfCode(uint16_t value_u14) 02746 { 02747 return g_MAX5171_device.VoltageOfCode((uint16_t)value_u14); 02748 } 02749 02750 //-------------------------------------------------- 02751 // selfTestFunctionClosures[functionName]['functionName'] = 'Init' 02752 // selfTestFunctionClosures[functionName]['argListDeclaration'] = 'void' 02753 // selfTestFunctionClosures[functionName]['returnType'] = 'void' 02754 // selfTestFunctionClosures[functionName]['argNames'] = '' 02755 // CommandParamIn_declaration = 'void' 02756 // argNames_recast_implementation = '' 02757 //-------------------------------------------------- 02758 // selftest: define function under test 02759 // void MAX5171::Init(void) 02760 void fn_MAX5171_Init(void) 02761 { 02762 return g_MAX5171_device.Init(); 02763 } 02764 02765 //-------------------------------------------------- 02766 // selfTestFunctionClosures[functionName]['functionName'] = 'CODE_LOAD' 02767 // selfTestFunctionClosures[functionName]['argListDeclaration'] = 'uint16_t dacCodeLsbs' 02768 // selfTestFunctionClosures[functionName]['returnType'] = 'uint8_t' 02769 // selfTestFunctionClosures[functionName]['argNames'] = 'dacCodeLsbs' 02770 // CommandParamIn_declaration = 'uint16_t dacCodeLsbs' 02771 // argNames_recast_implementation = '(uint16_t)dacCodeLsbs' 02772 //-------------------------------------------------- 02773 // selftest: define function under test 02774 // uint8_t MAX5171::CODE_LOAD(uint16_t dacCodeLsbs) 02775 uint8_t fn_MAX5171_CODE_LOAD(uint16_t dacCodeLsbs) 02776 { 02777 return g_MAX5171_device.CODE_LOAD((uint16_t)dacCodeLsbs); 02778 } 02779 02780 //-------------------------------------------------- 02781 // selfTestFunctionClosures[functionName]['functionName'] = 'UPO_HIGH' 02782 // selfTestFunctionClosures[functionName]['argListDeclaration'] = 'void' 02783 // selfTestFunctionClosures[functionName]['returnType'] = 'uint8_t' 02784 // selfTestFunctionClosures[functionName]['argNames'] = '' 02785 // CommandParamIn_declaration = 'void' 02786 // argNames_recast_implementation = '' 02787 //-------------------------------------------------- 02788 // selftest: define function under test 02789 // uint8_t MAX5171::UPO_HIGH(void) 02790 uint8_t fn_MAX5171_UPO_HIGH(void) 02791 { 02792 return g_MAX5171_device.UPO_HIGH(); 02793 } 02794 02795 //-------------------------------------------------- 02796 // selfTestFunctionClosures[functionName]['functionName'] = 'UPO_LOW' 02797 // selfTestFunctionClosures[functionName]['argListDeclaration'] = 'void' 02798 // selfTestFunctionClosures[functionName]['returnType'] = 'uint8_t' 02799 // selfTestFunctionClosures[functionName]['argNames'] = '' 02800 // CommandParamIn_declaration = 'void' 02801 // argNames_recast_implementation = '' 02802 //-------------------------------------------------- 02803 // selftest: define function under test 02804 // uint8_t MAX5171::UPO_LOW(void) 02805 uint8_t fn_MAX5171_UPO_LOW(void) 02806 { 02807 return g_MAX5171_device.UPO_LOW(); 02808 } 02809 02810 02811 //-------------------------------------------------- 02812 inline void print_command_prompt() 02813 { 02814 cmdLine_serial.serial().printf("\r\n> "); 02815 02816 } 02817 02818 02819 //-------------------------------------------------- 02820 void pinsMonitor_submenu_onEOLcommandParser(CmdLine& cmdLine) 02821 { 02822 // % diagnostic commands submenu 02823 // %Hpin -- digital output high 02824 // %Lpin -- digital output low 02825 // %?pin -- digital input 02826 // %A %Apin -- analog input 02827 // %Ppin df=xx -- pwm output 02828 // %Wpin -- measure high pulsewidth input in usec 02829 // %wpin -- measure low pulsewidth input in usec 02830 // %I... -- I2C diagnostics 02831 // %IP -- I2C probe 02832 // %IC scl=100khz ADDR=? -- I2C configure 02833 // %IW byte byte ... byte RD=? ADDR=0x -- write 02834 // %IR ADDR=? RD=? -- read 02835 // %I^ cmd=? -- i2c_smbus_read_word_data 02836 // %S... -- SPI diagnostics 02837 // %SC sclk=1Mhz -- SPI configure 02838 // %SW -- write (write and read) 02839 // %SR -- read (alias for %SW because SPI always write and read) 02840 // A-Z,a-z,0-9 reserved for application use 02841 // 02842 char strPinIndex[3]; 02843 strPinIndex[0] = cmdLine[2]; 02844 strPinIndex[1] = cmdLine[3]; 02845 strPinIndex[2] = '\0'; 02846 int pinIndex = strtoul(strPinIndex, NULL, 10); // strtol(str, NULL, 10): get decimal value 02847 //cmdLine.serial().printf(" pinIndex=%d ", pinIndex); 02848 // 02849 // get next character 02850 switch (cmdLine[1]) 02851 { 02852 #if HAS_digitalInOuts 02853 case 'H': case 'h': 02854 { 02855 // %Hpin -- digital output high 02856 #if ARDUINO_STYLE 02857 pinMode(pinIndex, OUTPUT); // digital pins 0, 1, 2, .. 13, analog input pins A0, A1, .. A5 02858 digitalWrite(pinIndex, HIGH); // digital pins 0, 1, 2, .. 13, analog input pins A0, A1, .. A5 02859 #else 02860 DigitalInOut& digitalInOutPin = find_digitalInOutPin(pinIndex); 02861 digitalInOutPin.output(); 02862 digitalInOutPin.write(1); 02863 #endif 02864 cmdLine.serial().printf(" digitalInOutPin %d Output High ", pinIndex); 02865 } 02866 break; 02867 case 'L': case 'l': 02868 { 02869 // %Lpin -- digital output low 02870 #if ARDUINO_STYLE 02871 pinMode(pinIndex, OUTPUT); // digital pins 0, 1, 2, .. 13, analog input pins A0, A1, .. A5 02872 digitalWrite(pinIndex, LOW); // digital pins 0, 1, 2, .. 13, analog input pins A0, A1, .. A5 02873 #else 02874 DigitalInOut& digitalInOutPin = find_digitalInOutPin(pinIndex); 02875 digitalInOutPin.output(); 02876 digitalInOutPin.write(0); 02877 #endif 02878 cmdLine.serial().printf(" digitalInOutPin %d Output Low ", pinIndex); 02879 } 02880 break; 02881 case '?': 02882 { 02883 // %?pin -- digital input 02884 #if ARDUINO_STYLE 02885 pinMode(pinIndex, INPUT); // digital pins 0, 1, 2, .. 13, analog input pins A0, A1, .. A5 02886 #else 02887 DigitalInOut& digitalInOutPin = find_digitalInOutPin(pinIndex); 02888 digitalInOutPin.input(); 02889 #endif 02890 serial.printf(" digitalInOutPin %d Input ", pinIndex); 02891 #if ARDUINO_STYLE 02892 int value = digitalRead(pinIndex); 02893 #else 02894 int value = digitalInOutPin.read(); 02895 #endif 02896 cmdLine.serial().printf("%d ", value); 02897 } 02898 break; 02899 #endif 02900 // 02901 #if HAS_analogIns 02902 case 'A': case 'a': 02903 { 02904 // %A %Apin -- analog input 02905 #if analogIn4_IS_HIGH_RANGE_OF_analogIn0 02906 // Platform board uses AIN4,AIN5,.. as high range of AIN0,AIN1,.. 02907 for (int pinIndex = 0; pinIndex < 2; pinIndex++) 02908 { 02909 int cPinIndex = '0' + pinIndex; 02910 AnalogIn& analogInPin = find_analogInPin(cPinIndex); 02911 float adc_full_scale_voltage = analogInPin_fullScaleVoltage[pinIndex]; 02912 float normValue_0_1 = analogInPin.read(); 02913 // 02914 int pinIndexH = pinIndex + 4; 02915 int cPinIndexH = '0' + pinIndexH; 02916 AnalogIn& analogInPinH = find_analogInPin(cPinIndexH); 02917 float adc_full_scale_voltageH = analogInPin_fullScaleVoltage[pinIndexH]; 02918 float normValueH_0_1 = analogInPinH.read(); 02919 // 02920 cmdLine.serial().printf("AIN%c = %7.3f%% = %1.3fV AIN%c = %7.3f%% = %1.3fV \r\n", 02921 cPinIndex, 02922 normValue_0_1 * 100.0, 02923 normValue_0_1 * adc_full_scale_voltage, 02924 cPinIndexH, 02925 normValueH_0_1 * 100.0, 02926 normValueH_0_1 * adc_full_scale_voltageH 02927 ); 02928 } 02929 for (int pinIndex = 2; pinIndex < 4; pinIndex++) 02930 { 02931 int cPinIndex = '0' + pinIndex; 02932 AnalogIn& analogInPin = find_analogInPin(cPinIndex); 02933 float adc_full_scale_voltage = analogInPin_fullScaleVoltage[pinIndex]; 02934 float normValue_0_1 = analogInPin.read(); 02935 // 02936 cmdLine.serial().printf("AIN%c = %7.3f%% = %1.3fV\r\n", 02937 cPinIndex, 02938 normValue_0_1 * 100.0, 02939 normValue_0_1 * adc_full_scale_voltage 02940 ); 02941 } 02942 #else // analogIn4_IS_HIGH_RANGE_OF_analogIn0 02943 // Platform board uses simple analog inputs 02944 // assume standard Arduino analog inputs A0-A5 02945 for (int pinIndex = 0; pinIndex < 6; pinIndex++) 02946 { 02947 int cPinIndex = '0' + pinIndex; 02948 AnalogIn& analogInPin = find_analogInPin(cPinIndex); 02949 float adc_full_scale_voltage = analogInPin_fullScaleVoltage[pinIndex]; 02950 float normValue_0_1 = analogInPin.read(); 02951 // 02952 cmdLine.serial().printf("AIN%c = %7.3f%% = %1.3fV\r\n", 02953 cPinIndex, 02954 normValue_0_1 * 100.0, 02955 normValue_0_1 * adc_full_scale_voltage 02956 ); 02957 } 02958 #endif // analogIn4_IS_HIGH_RANGE_OF_analogIn0 02959 } 02960 break; 02961 #endif 02962 // 02963 #if HAS_SPI2_MAX541 02964 case 'D': case 'd': 02965 { 02966 // %D -- DAC output MAX541 (SPI2) -- need cmdLine.parse_float(voltageV) 02967 // MAX541 max541(spi2_max541, spi2_max541_cs); 02968 float voltageV = max541.Get_Voltage(); 02969 // if (cmdLine[2] == '+') { 02970 // // %D+ 02971 // voltageV = voltageV * 1.25f; 02972 // if (voltageV >= max541.VRef) voltageV = max541.VRef; 02973 // SelfTest_MAX541_Voltage(cmdLine, max541, voltageV); 02974 // } 02975 // else if (cmdLine[2] == '-') { 02976 // // %D- 02977 // voltageV = voltageV * 0.75f; 02978 // if (voltageV < 0.1f) voltageV = 0.1f; 02979 // SelfTest_MAX541_Voltage(cmdLine, max541, voltageV); 02980 // } 02981 if (cmdLine.parse_float("V", voltageV)) 02982 { 02983 // %D V=1.234 -- set voltage 02984 max541.Set_Voltage(voltageV); 02985 } 02986 else if (cmdLine.parse_float("TEST", voltageV)) 02987 { 02988 // %D TEST=1.234 -- set voltage and compare with AIN0 02989 SelfTest_MAX541_Voltage(cmdLine, max541, voltageV); 02990 } 02991 else if (cmdLine.parse_float("CAL", voltageV)) 02992 { 02993 // %D CAL=1.234 -- calibrate VRef and compare with AIN0 02994 02995 max541.Set_Code(0x8000); // we don't know the fullscale voltage yet, so set code to midscale 02996 double max541_midscale_V = analogInPin_fullScaleVoltage[4] * analogIn4.read(); // TARGET_MAX32630 J1.5 AIN_4 = AIN0 / 5.0 fullscale is 6.0V 02997 const int average_count = 100; 02998 const double average_K = 0.25; 02999 for (int count = 0; count < average_count; count++) { 03000 double measurement_V = analogInPin_fullScaleVoltage[4] * analogIn4.read(); // TARGET_MAX32630 J1.5 AIN_4 = AIN0 / 5.0 fullscale is 6.0V 03001 max541_midscale_V = ((1 - average_K) * max541_midscale_V) + (average_K * measurement_V); 03002 } 03003 max541.VRef = 2.0 * max541_midscale_V; 03004 cmdLine.serial().printf( 03005 "\r\n MAX541 midscale = %1.3fV, so fullscale = %1.3fV", 03006 max541_midscale_V, max541.VRef); 03007 // Detect whether MAX541 is really connected to MAX32625MBED.AIN0/AIN4 03008 voltageV = 1.0f; 03009 SelfTest_MAX541_Voltage(cmdLine, max541, voltageV); 03010 } 03011 else { 03012 // %D -- print MAX541 DAC status 03013 cmdLine.serial().printf("MAX541 code=0x%4.4x = %1.3fV VRef=%1.3fV\r\n", 03014 max541.Get_Code(), max541.Get_Voltage(), max541.VRef); 03015 } 03016 } 03017 break; 03018 #endif 03019 03020 // 03021 #if HAS_I2C // SUPPORT_I2C 03022 case 'I': case 'i': 03023 // %I... -- I2C diagnostics 03024 // %IP -- I2C probe 03025 // %IC scl=100khz ADDR=? -- I2C configure 03026 // %IW byte byte ... byte RD=? ADDR=0x -- write 03027 // %IR ADDR=? RD=? -- read 03028 // %I^ cmd=? -- i2c_smbus_read_word_data 03029 // get next character 03030 // TODO: parse cmdLine arg (ADDR=\d+)? --> g_I2C_deviceAddress7 03031 cmdLine.parse_byte_hex("ADDR", g_I2C_deviceAddress7); 03032 // TODO: parse cmdLine arg (RD=\d)? --> g_I2C_read_count 03033 g_I2C_read_count = 0; // read count must be reset every command 03034 cmdLine.parse_byte_dec("RD", g_I2C_read_count); 03035 // TODO: parse cmdLine arg (CMD=\d)? --> g_I2C_command_regAddress 03036 cmdLine.parse_byte_hex("CMD", g_I2C_command_regAddress); 03037 switch (cmdLine[2]) 03038 { 03039 case 'P': case 'p': 03040 { 03041 // %IP -- I2C probe 03042 HuntAttachedI2CDevices(cmdLine, 0x03, 0x77); 03043 } 03044 break; 03045 case 'C': case 'c': 03046 { 03047 bool isUpdatedI2CConfig = false; 03048 // %IC scl=100khz ADDR=? -- I2C configure 03049 // parse cmdLine arg (SCL=\d+(kHZ|MHZ)?)? --> g_I2C_SCL_Hz 03050 if (cmdLine.parse_frequency_Hz("SCL", g_I2C_SCL_Hz)) 03051 { 03052 isUpdatedI2CConfig = true; 03053 // TODO1: validate g_I2C_SCL_Hz against system clock frequency F_CPU 03054 if (g_I2C_SCL_Hz > limit_max_I2C_SCL_Hz) 03055 { 03056 g_I2C_SCL_Hz = limit_max_I2C_SCL_Hz; 03057 } 03058 if (g_I2C_SCL_Hz < limit_min_I2C_SCL_Hz) 03059 { 03060 g_I2C_SCL_Hz = limit_min_I2C_SCL_Hz; 03061 } 03062 } 03063 if (isUpdatedI2CConfig) 03064 { 03065 // declare in narrower scope: MAX32625MBED I2C i2cMaster(...) 03066 I2C i2cMaster(I2C0_SDA, I2C0_SCL); // sda scl TARGET_MAX32635MBED: P1_6, P1_7 Arduino 10-pin header 03067 i2cMaster.frequency(g_I2C_SCL_Hz); 03068 i2cMaster.start(); 03069 i2cMaster.stop(); 03070 i2cMaster.frequency(g_I2C_SCL_Hz); 03071 cmdLine.serial().printf( 03072 "\r\n %%IC ADDR=0x%2.2x=(0x%2.2x>>1) SCL=%d=%1.3fkHz -- I2C config", 03073 g_I2C_deviceAddress7, (g_I2C_deviceAddress7 << 1), g_I2C_SCL_Hz, 03074 (g_I2C_SCL_Hz / 1000.)); 03075 i2cMaster.start(); 03076 i2cMaster.stop(); 03077 } 03078 } 03079 break; 03080 case 'W': case 'w': 03081 { 03082 // declare in narrower scope: MAX32625MBED I2C i2cMaster(...) 03083 I2C i2cMaster(I2C0_SDA, I2C0_SCL); // sda scl TARGET_MAX32635MBED: P1_6, P1_7 Arduino 10-pin header 03084 i2cMaster.frequency(g_I2C_SCL_Hz); 03085 // %IW byte byte ... byte RD=? ADDR=0x -- write 03086 // parse cmdLine byte list --> int byteCount; int mosiData[MAX_SPI_BYTE_COUNT]; 03087 #define MAX_I2C_BYTE_COUNT 32 03088 size_t byteCount = byteCount; 03089 static char mosiData[MAX_I2C_BYTE_COUNT]; 03090 static char misoData[MAX_I2C_BYTE_COUNT]; 03091 if (cmdLine.parse_byteCount_byteList_hex(byteCount, mosiData, 03092 MAX_I2C_BYTE_COUNT)) 03093 { 03094 // hex dump mosiData[0..byteCount-1] 03095 cmdLine.serial().printf( 03096 "\r\nADDR=0x%2.2x=(0x%2.2x>>1) byteCount:%d RD=%d\r\nI2C MOSI->", 03097 g_I2C_deviceAddress7, 03098 (g_I2C_deviceAddress7 << 1), byteCount, g_I2C_read_count); 03099 for (unsigned int byteIndex = 0; byteIndex < byteCount; byteIndex++) 03100 { 03101 cmdLine.serial().printf(" 0x%2.2X", mosiData[byteIndex]); 03102 } 03103 // 03104 // TODO: i2c transfer 03105 //const int addr7bit = 0x48; // 7 bit I2C address 03106 //const int addr8bit = 0x48 << 1; // 8bit I2C address, 0x90 03107 // /* int */ i2cMaster.read (int addr8bit, char *data, int length, bool repeated=false) // Read from an I2C slave. 03108 // /* int */ i2cMaster.read (int ack) // Read a single byte from the I2C bus. 03109 // /* int */ i2cMaster.write (int addr8bit, const char *data, int length, bool repeated=false) // Write to an I2C slave. 03110 // /* int */ i2cMaster.write (int data) // Write single byte out on the I2C bus. 03111 // /* void */ i2cMaster.start (void) // Creates a start condition on the I2C bus. 03112 // /* void */ i2cMaster.stop (void) // Creates a stop condition on the I2C bus. 03113 // /* int */ i2cMaster.transfer (int addr8bit, const char *tx_buffer, int tx_length, char *rx_buffer, int rx_length, const event_callback_t &callback, int event=I2C_EVENT_TRANSFER_COMPLETE, bool repeated=false) // Start nonblocking I2C transfer. More... 03114 // /* void */ i2cMaster.abort_transfer () // Abort the ongoing I2C transfer. More... 03115 const int addr8bit = g_I2C_deviceAddress7 << 1; // 8bit I2C address, 0x90 03116 unsigned int misoLength = 0; 03117 bool repeated = (g_I2C_read_count > 0); 03118 // 03119 int writeStatus = i2cMaster.write (addr8bit, mosiData, byteCount, repeated); 03120 switch (writeStatus) 03121 { 03122 case 0: cmdLine.serial().printf(" ack "); break; 03123 case 1: cmdLine.serial().printf(" nack "); break; 03124 default: cmdLine.serial().printf(" {writeStatus 0x%2.2X} ", 03125 writeStatus); 03126 } 03127 if (repeated) 03128 { 03129 int readStatus = 03130 i2cMaster.read (addr8bit, misoData, g_I2C_read_count, false); 03131 switch (readStatus) 03132 { 03133 case 1: cmdLine.serial().printf(" nack "); break; 03134 case 0: cmdLine.serial().printf(" ack "); break; 03135 default: cmdLine.serial().printf(" {readStatus 0x%2.2X} ", 03136 readStatus); 03137 } 03138 } 03139 // 03140 if (misoLength > 0) 03141 { 03142 // hex dump misoData[0..byteCount-1] 03143 cmdLine.serial().printf(" MISO<-"); 03144 for (unsigned int byteIndex = 0; byteIndex < g_I2C_read_count; 03145 byteIndex++) 03146 { 03147 cmdLine.serial().printf(" 0x%2.2X", misoData[byteIndex]); 03148 } 03149 } 03150 cmdLine.serial().printf(" "); 03151 } 03152 } 03153 break; 03154 case 'R': case 'r': 03155 { 03156 // declare in narrower scope: MAX32625MBED I2C i2cMaster(...) 03157 I2C i2cMaster(I2C0_SDA, I2C0_SCL); // sda scl TARGET_MAX32635MBED: P1_6, P1_7 Arduino 10-pin header 03158 i2cMaster.frequency(g_I2C_SCL_Hz); 03159 // %IR ADDR=? RD=? -- read 03160 // TODO: i2c transfer 03161 //const int addr7bit = 0x48; // 7 bit I2C address 03162 //const int addr8bit = 0x48 << 1; // 8bit I2C address, 0x90 03163 // /* int */ i2cMaster.read (int addr8bit, char *data, int length, bool repeated=false) // Read from an I2C slave. 03164 // /* int */ i2cMaster.read (int ack) // Read a single byte from the I2C bus. 03165 // /* int */ i2cMaster.write (int addr8bit, const char *data, int length, bool repeated=false) // Write to an I2C slave. 03166 // /* int */ i2cMaster.write (int data) // Write single byte out on the I2C bus. 03167 // /* void */ i2cMaster.start (void) // Creates a start condition on the I2C bus. 03168 // /* void */ i2cMaster.stop (void) // Creates a stop condition on the I2C bus. 03169 // /* int */ i2cMaster.transfer (int addr8bit, const char *tx_buffer, int tx_length, char *rx_buffer, int rx_length, const event_callback_t &callback, int event=I2C_EVENT_TRANSFER_COMPLETE, bool repeated=false) // Start nonblocking I2C transfer. More... 03170 // /* void */ i2cMaster.abort_transfer () // Abort the ongoing I2C transfer. More... 03171 } 03172 break; 03173 case '^': 03174 { 03175 // declare in narrower scope: MAX32625MBED I2C i2cMaster(...) 03176 I2C i2cMaster(I2C0_SDA, I2C0_SCL); // sda scl TARGET_MAX32635MBED: P1_6, P1_7 Arduino 10-pin header 03177 i2cMaster.frequency(g_I2C_SCL_Hz); 03178 // %I^ cmd=? -- i2c_smbus_read_word_data 03179 // TODO: i2c transfer 03180 //const int addr7bit = 0x48; // 7 bit I2C address 03181 //const int addr8bit = 0x48 << 1; // 8bit I2C address, 0x90 03182 // /* int */ i2cMaster.read (int addr8bit, char *data, int length, bool repeated=false) // Read from an I2C slave. 03183 // /* int */ i2cMaster.read (int ack) // Read a single byte from the I2C bus. 03184 // /* int */ i2cMaster.write (int addr8bit, const char *data, int length, bool repeated=false) // Write to an I2C slave. 03185 // /* int */ i2cMaster.write (int data) // Write single byte out on the I2C bus. 03186 // /* void */ i2cMaster.start (void) // Creates a start condition on the I2C bus. 03187 // /* void */ i2cMaster.stop (void) // Creates a stop condition on the I2C bus. 03188 // /* int */ i2cMaster.transfer (int addr8bit, const char *tx_buffer, int tx_length, char *rx_buffer, int rx_length, const event_callback_t &callback, int event=I2C_EVENT_TRANSFER_COMPLETE, bool repeated=false) // Start nonblocking I2C transfer. More... 03189 // /* void */ i2cMaster.abort_transfer () // Abort the ongoing I2C transfer. More... 03190 } 03191 break; 03192 } // switch(cmdLine[2]) 03193 break; 03194 #endif 03195 // 03196 #if HAS_SPI // SUPPORT_SPI 03197 case 'S': case 's': 03198 { 03199 // %S... -- SPI diagnostics 03200 // %SC sclk=1Mhz -- SPI configure 03201 // %SW -- write (write and read) 03202 // %SR -- read (alias for %SW because SPI always write and read) 03203 // 03204 // Process arguments SCLK=\d+(kHZ|MHZ) CPOL=\d CPHA=\d 03205 bool isUpdatedSPIConfig = false; 03206 // parse cmdLine arg (CPOL=\d)? --> g_SPI_dataMode | SPI_MODE2 03207 // parse cmdLine arg (CPHA=\d)? --> g_SPI_dataMode | SPI_MODE1 03208 if (cmdLine.parse_flag("CPOL", g_SPI_dataMode, SPI_MODE2)) 03209 { 03210 isUpdatedSPIConfig = true; 03211 } 03212 if (cmdLine.parse_flag("CPHA", g_SPI_dataMode, SPI_MODE1)) 03213 { 03214 isUpdatedSPIConfig = true; 03215 } 03216 if (cmdLine.parse_flag("CS", g_SPI_cs_state, 1)) 03217 { 03218 isUpdatedSPIConfig = true; 03219 } 03220 // parse cmdLine arg (SCLK=\d+(kHZ|MHZ)?)? --> g_SPI_SCLK_Hz 03221 if (cmdLine.parse_frequency_Hz("SCLK", g_SPI_SCLK_Hz)) 03222 { 03223 isUpdatedSPIConfig = true; 03224 // TODO1: validate g_SPI_SCLK_Hz against system clock frequency F_CPU 03225 if (g_SPI_SCLK_Hz > limit_max_SPI_SCLK_Hz) 03226 { 03227 g_SPI_SCLK_Hz = limit_max_SPI_SCLK_Hz; 03228 } 03229 if (g_SPI_SCLK_Hz < limit_min_SPI_SCLK_Hz) 03230 { 03231 g_SPI_SCLK_Hz = limit_min_SPI_SCLK_Hz; 03232 } 03233 } 03234 // Update SPI configuration 03235 if (isUpdatedSPIConfig) 03236 { 03237 // %SC sclk=1Mhz -- SPI configure 03238 spi_cs = g_SPI_cs_state; 03239 spi.format(8,g_SPI_dataMode); // int bits_must_be_8, int mode=0_3 CPOL=0,CPHA=0 03240 #if APPLICATION_MAX5715 03241 g_MAX5715_device.spi_frequency(g_SPI_SCLK_Hz); 03242 #elif APPLICATION_MAX11131 03243 g_MAX11131_device.spi_frequency(g_SPI_SCLK_Hz); 03244 #elif APPLICATION_MAX5171 03245 g_MAX5171_device.spi_frequency(g_SPI_SCLK_Hz); 03246 #elif APPLICATION_MAX11410 03247 g_MAX11410_device.spi_frequency(g_SPI_SCLK_Hz); 03248 #elif APPLICATION_MAX12345 03249 g_MAX12345_device.spi_frequency(g_SPI_SCLK_Hz); 03250 #else 03251 spi.frequency(g_SPI_SCLK_Hz); // int SCLK_Hz=1000000 = 1MHz (initial default) 03252 #endif 03253 // 03254 double ideal_divisor = ((double)SystemCoreClock) / g_SPI_SCLK_Hz; 03255 int actual_divisor = (int)(ideal_divisor + 0.0); // frequency divisor truncate 03256 double actual_SCLK_Hz = SystemCoreClock / actual_divisor; 03257 // 03258 // fixed: mbed-os-5.11: [Warning] format '%d' expects argument of type 'int', but argument 6 has type 'uint32_t {aka long unsigned int}' [-Wformat=] 03259 cmdLine.serial().printf( 03260 "\r\n %%SC CPOL=%d CPHA=%d CS=%d SCLK=%ld=%1.3fMHz (%1.1fMHz/%1.2f = actual %1.3fMHz) -- SPI config", 03261 ((g_SPI_dataMode & SPI_MODE2) ? 1 : 0), 03262 ((g_SPI_dataMode & SPI_MODE1) ? 1 : 0), 03263 g_SPI_cs_state, 03264 g_SPI_SCLK_Hz, 03265 (g_SPI_SCLK_Hz / 1000000.), 03266 ((double)(SystemCoreClock / 1000000.)), 03267 ideal_divisor, 03268 (actual_SCLK_Hz / 1000000.) 03269 ); 03270 } 03271 // get next character 03272 switch (cmdLine[2]) 03273 { 03274 case 'C': case 's': 03275 // %SC sclk=1Mhz -- SPI configure 03276 break; 03277 case 'D': case 'd': 03278 // %SD -- SPI diagnostic messages enable 03279 if (g_MAX5171_device.onSPIprint) { 03280 g_MAX5171_device.onSPIprint = NULL; 03281 // no g_MAX5171_device.loop_limit property; device_has_property(Device, 'loop_limit') != None is false 03282 } 03283 else { 03284 void onSPIprint_handler(size_t byteCount, uint8_t mosiData[], uint8_t misoData[]); 03285 g_MAX5171_device.onSPIprint = onSPIprint_handler; 03286 // no g_MAX5171_device.loop_limit property; device_has_property(Device, 'loop_limit') is false 03287 } 03288 break; 03289 case 'W': case 'R': case 'w': case 'r': 03290 { 03291 // %SW -- write (write and read) 03292 // %SR -- read (alias for %SW because SPI always write and read) 03293 // parse cmdLine byte list --> int byteCount; int mosiData[MAX_SPI_BYTE_COUNT]; 03294 #define MAX_SPI_BYTE_COUNT 32 03295 size_t byteCount = byteCount; 03296 static char mosiData[MAX_SPI_BYTE_COUNT]; 03297 static char misoData[MAX_SPI_BYTE_COUNT]; 03298 if (cmdLine.parse_byteCount_byteList_hex(byteCount, mosiData, 03299 MAX_SPI_BYTE_COUNT)) 03300 { 03301 // hex dump mosiData[0..byteCount-1] 03302 cmdLine.serial().printf("\r\nSPI"); 03303 if (byteCount > 7) { 03304 cmdLine.serial().printf(" byteCount:%d", byteCount); 03305 } 03306 cmdLine.serial().printf(" MOSI->"); 03307 for (unsigned int byteIndex = 0; byteIndex < byteCount; byteIndex++) 03308 { 03309 cmdLine.serial().printf(" 0x%2.2X", mosiData[byteIndex]); 03310 } 03311 spi_cs = 0; 03312 unsigned int numBytesTransferred = 03313 spi.write(mosiData, byteCount, misoData, byteCount); 03314 spi_cs = 1; 03315 // hex dump misoData[0..byteCount-1] 03316 cmdLine.serial().printf(" MISO<-"); 03317 for (unsigned int byteIndex = 0; byteIndex < numBytesTransferred; 03318 byteIndex++) 03319 { 03320 cmdLine.serial().printf(" 0x%2.2X", misoData[byteIndex]); 03321 } 03322 cmdLine.serial().printf(" "); 03323 } 03324 } 03325 break; 03326 } // switch(cmdLine[2]) 03327 } // case 'S': // %S... -- SPI diagnostics 03328 break; 03329 #endif 03330 // 03331 // A-Z,a-z,0-9 reserved for application use 03332 } // switch(cmdLine[1]) 03333 } // end void pinsMonitor_submenu_onEOLcommandParser(CmdLine & cmdLine) 03334 03335 03336 //-------------------------------------------------- 03337 void main_menu_status(CmdLine & cmdLine) 03338 { 03339 cmdLine.serial().printf("\r\nMain menu"); 03340 03341 cmdLine.serial().printf(" MAX5171 14-bit Force/Sense DAC"); 03342 03343 //cmdLine.serial().print(" %s", TARGET_NAME); 03344 if (cmdLine.nameStr()) 03345 { 03346 cmdLine.serial().printf(" [%s]", cmdLine.nameStr()); 03347 03348 } 03349 cmdLine.serial().printf("\r\n ? -- help"); 03350 03351 } 03352 03353 03354 //-------------------------------------------------- 03355 void main_menu_help(CmdLine & cmdLine) 03356 { 03357 // ? -- help 03358 //~ cmdLine.serial().print(F("\r\nMenu:")); 03359 cmdLine.serial().printf("\r\n # -- lines beginning with # are comments"); 03360 03361 cmdLine.serial().printf("\r\n . -- SelfTest"); 03362 03363 //cmdLine.serial().print(F("\r\n ! -- Initial Configuration")); 03364 // 03365 // % standardize diagnostic commands 03366 // %Hpin -- digital output high 03367 // %Lpin -- digital output low 03368 // %?pin -- digital input 03369 // %A %Apin -- analog input 03370 // %Ppin df=xx -- pwm output 03371 // %Wpin -- measure high pulsewidth input in usec 03372 // %wpin -- measure low pulsewidth input in usec 03373 // %I... -- I2C diagnostics 03374 // %IP -- I2C probe 03375 // %IC scl=100khz ADDR=? -- I2C configure 03376 // %IW ADDR=? cmd=? data,data,data -- write 03377 // %IR ADDR=? RD=? -- read 03378 // %I^ cmd=? -- i2c_smbus_read_word_data 03379 // %S... -- SPI diagnostics 03380 // %SC sclk=1Mhz -- SPI configure 03381 // %SW -- write (write and read) 03382 // %SR -- read (alias for %SW because SPI always write and read) 03383 // A-Z,a-z,0-9 reserved for application use 03384 // 03385 #if HAS_digitalInOuts 03386 // %Hpin -- digital output high 03387 // %Lpin -- digital output low 03388 // %?pin -- digital input 03389 cmdLine.serial().printf("\r\n %%Hn {pin:"); 03390 list_digitalInOutPins(cmdLine.serial()); 03391 cmdLine.serial().printf("} -- High Output"); 03392 cmdLine.serial().printf("\r\n %%Ln {pin:"); 03393 list_digitalInOutPins(cmdLine.serial()); 03394 cmdLine.serial().printf("} -- Low Output"); 03395 cmdLine.serial().printf("\r\n %%?n {pin:"); 03396 list_digitalInOutPins(cmdLine.serial()); 03397 cmdLine.serial().printf("} -- Input"); 03398 #endif 03399 03400 #if HAS_analogIns 03401 // Menu A) analogRead A0..7 03402 // %A %Apin -- analog input 03403 // analogRead(pinIndex) // analog input pins A0, A1, A2, A3, A4, A5; float voltage = analogRead(A0) * (5.0 / 1023.0) 03404 cmdLine.serial().printf("\r\n %%A -- analogRead"); 03405 #endif 03406 03407 #if HAS_SPI2_MAX541 03408 // TODO1: MAX541 max541(spi2_max541, spi2_max541_cs); 03409 cmdLine.serial().printf("\r\n %%D -- DAC output MAX541 (SPI2)"); 03410 #endif 03411 03412 #if HAS_I2C // SUPPORT_I2C 03413 // TODO: support I2C HAS_I2C // SUPPORT_I2C 03414 // VERIFY: I2C utility commands SUPPORT_I2C 03415 // VERIFY: report g_I2C_SCL_Hz = (F_CPU / ((TWBR * 2) + 16)) from last Wire_Sr.setClock(I2C_SCL_Hz); 03416 // %I... -- I2C diagnostics 03417 // %IP -- I2C probe 03418 // %IC scl=100khz ADDR=? -- I2C configure 03419 // %IW byte byte ... byte RD=? ADDR=0x -- write 03420 // %IR ADDR=? RD=? -- read 03421 // %I^ cmd=? -- i2c_smbus_read_word_data 03422 //g_I2C_SCL_Hz = (F_CPU / ((TWBR * 2) + 16)); // 'F_CPU' 'TWBR' not declared in this scope 03423 cmdLine.serial().printf("\r\n %%IC ADDR=0x%2.2x=(0x%2.2x>>1) SCL=%d=%1.3fkHz -- I2C config", 03424 g_I2C_deviceAddress7, (g_I2C_deviceAddress7 << 1), g_I2C_SCL_Hz, 03425 (g_I2C_SCL_Hz / 1000.)); 03426 cmdLine.serial().printf("\r\n %%IW byte byte ... byte RD=? ADDR=0x%2.2x -- I2C write/read", 03427 g_I2C_deviceAddress7); 03428 // 03429 #if SUPPORT_I2C 03430 // Menu ^ cmd=?) i2c_smbus_read_word_data 03431 cmdLine.serial().printf("\r\n %%I^ cmd=? -- i2c_smbus_read_word_data"); 03432 // test low-level I2C i2c_smbus_read_word_data 03433 #endif // SUPPORT_I2C 03434 //cmdLine.serial().printf(" H) Hunt for attached I2C devices"); 03435 cmdLine.serial().printf("\r\n %%IP -- I2C Probe for attached devices"); 03436 // cmdLine.serial().printf(" s) search i2c address"); 03437 #endif // SUPPORT_I2C 03438 03439 #if HAS_SPI // SUPPORT_SPI 03440 // TODO: support SPI HAS_SPI // SUPPORT_SPI 03441 // SPI test command S (mosiData)+ 03442 // %S... -- SPI diagnostics 03443 // %SC sclk=1Mhz -- SPI configure 03444 // %SW -- write (write and read) 03445 // %SR -- read (alias for %SW because SPI always write and read) 03446 // spi.format(8,0); // int bits_must_be_8, int mode=0_3 CPOL=0,CPHA=0 rising edge (initial default) 03447 // spi.format(8,1); // int bits_must_be_8, int mode=0_3 CPOL=0,CPHA=1 falling edge (initial default) 03448 // spi.format(8,2); // int bits_must_be_8, int mode=0_3 CPOL=1,CPHA=0 falling edge (initial default) 03449 // spi.format(8,3); // int bits_must_be_8, int mode=0_3 CPOL=1,CPHA=1 rising edge (initial default) 03450 // spi.frequency(1000000); // int SCLK_Hz=1000000 = 1MHz (initial default) 03451 // mode | POL PHA 03452 // -----+-------- 03453 // 0 | 0 0 03454 // 1 | 0 1 03455 // 2 | 1 0 03456 // 3 | 1 1 03457 //cmdLine.serial().printf(" S) SPI mosi,mosi,...mosi hex bytes SCLK=1000000 CPOL=0 CPHA=0"); 03458 // fixed: mbed-os-5.11: [Warning] format '%d' expects argument of type 'int', but argument 3 has type 'uint32_t {aka long unsigned int}' [-Wformat=] 03459 cmdLine.serial().printf("\r\n %%SC SCLK=%ld=%1.3fMHz CPOL=%d CPHA=%d -- SPI config", 03460 g_SPI_SCLK_Hz, (g_SPI_SCLK_Hz / 1000000.), 03461 ((g_SPI_dataMode & SPI_MODE2) ? 1 : 0), 03462 ((g_SPI_dataMode & SPI_MODE1) ? 1 : 0)); 03463 cmdLine.serial().printf("\r\n %%SD -- SPI diagnostic messages "); 03464 if (g_MAX5171_device.onSPIprint) { 03465 cmdLine.serial().printf("hide"); 03466 } 03467 else { 03468 cmdLine.serial().printf("show"); 03469 } 03470 cmdLine.serial().printf("\r\n %%SW mosi,mosi,...mosi -- SPI write hex bytes"); 03471 // VERIFY: parse new SPI settings parse_strCommandArgs() SCLK=1000000 CPOL=0 CPHA=0 03472 #endif // SUPPORT_SPI 03473 // 03474 // Application-specific commands (help text) here 03475 // 03476 #if APPLICATION_ArduinoPinsMonitor 03477 cmdLine.serial().printf("\r\n A-Z,a-z,0-9 -- reserved for application use"); // ArduinoPinsMonitor 03478 #endif // APPLICATION_ArduinoPinsMonitor 03479 // 03480 03481 extern void MAX5171_menu_help(CmdLine & cmdLine); // defined in Test_Menu_MAX5171.cpp\n 03482 MAX5171_menu_help(cmdLine); 03483 } 03484 03485 03486 03487 //-------------------------------------------------- 03488 // main menu command-line parser 03489 // invoked by CmdLine::append(char ch) or CmdLine::idleAppendIfReadable() 03490 void main_menu_onEOLcommandParser(CmdLine & cmdLine) 03491 { 03492 // DIAGNOSTIC: print line buffer 03493 //~ cmdLine.serial().printf("\r\nmain_menu_onEOLcommandParser: ~%s~\r\n", cmdLine.str()); 03494 // 03495 switch (cmdLine[0]) 03496 { 03497 case '?': 03498 main_menu_status(cmdLine); 03499 main_menu_help(cmdLine); 03500 // print command prompt 03501 //cmdLine.serial().printf("\r\n>"); 03502 break; 03503 case '\r': case '\n': // ignore blank line 03504 case '\0': // ignore empty line 03505 case '#': // ignore comment line 03506 // # -- lines beginning with # are comments 03507 main_menu_status(cmdLine); 03508 //~ main_menu_help(cmdLine); 03509 // print command prompt 03510 //cmdLine.serial().printf("\r\n>"); 03511 break; 03512 #if ECHO_EOF_ON_EOL 03513 case '\x04': // Unicode (U+0004) EOT END OF TRANSMISSION = CTRL+D as EOF end of file 03514 cmdLine.serial().printf("\x04"); // immediately echo EOF for test scripting 03515 diagnostic_led_EOF(); 03516 break; 03517 case '\x1a': // Unicode (U+001A) SUB SUBSTITUTE = CTRL+Z as EOF end of file 03518 cmdLine.serial().printf("\x1a"); // immediately echo EOF for test scripting 03519 diagnostic_led_EOF(); 03520 break; 03521 #endif 03522 #if APPLICATION_ArduinoPinsMonitor 03523 case '.': 03524 { 03525 // . -- SelfTest 03526 cmdLine.serial().printf("SelfTest()"); 03527 SelfTest(cmdLine); 03528 } 03529 break; 03530 case '%': 03531 { 03532 pinsMonitor_submenu_onEOLcommandParser(cmdLine); 03533 } 03534 break; // case '%' 03535 #endif // APPLICATION_ArduinoPinsMonitor 03536 // 03537 // Application-specific commands here 03538 // alphanumeric command codes A-Z,a-z,0-9 reserved for application use 03539 // 03540 #if APPLICATION_ArduinoPinsMonitor 03541 #endif // APPLICATION_ArduinoPinsMonitor 03542 03543 // 03544 // add new commands here 03545 // 03546 default: 03547 extern bool MAX5171_menu_onEOLcommandParser(CmdLine & cmdLine); // defined in Test_Menu_MAX5171.cpp 03548 if (!MAX5171_menu_onEOLcommandParser(cmdLine)) 03549 { // not_handled_by_device_submenu 03550 cmdLine.serial().printf("\r\n unknown command 0x%2.2x \"%s\"\r\n", cmdLine.str()[0], cmdLine.str()); 03551 03552 # if HAS_DAPLINK_SERIAL 03553 cmdLine_DAPLINKserial.serial().printf("\r\n unknown command 0x%2.2x \"%s\"\r\n", cmdLine.str()[0], cmdLine.str()); 03554 03555 # endif // HAS_DAPLINK_SERIAL 03556 } 03557 } // switch (cmdLine[0]) 03558 // 03559 // print command prompt 03560 cmdLine.serial().printf("\r\nMAX5171 > "); 03561 03562 } // end void main_menu_onEOLcommandParser(CmdLine & cmdLine) 03563 03564 //-------------------------------------------------- 03565 #if MAX5171_ONSPIPRINT 03566 // Optional Diagnostic function to print SPI transactions 03567 void onSPIprint_handler(size_t byteCount, uint8_t mosiData[], uint8_t misoData[]) 03568 { 03569 cmdLine_serial.serial().printf("\r\n SPI MOSI->"); 03570 for (uint8_t index = 0; index < byteCount; index++) { 03571 cmdLine_serial.serial().printf(" 0x%2.2X", mosiData[index]); 03572 } 03573 cmdLine_serial.serial().printf(" MISO<-"); 03574 for (uint8_t index = 0; index < byteCount; index++) { 03575 cmdLine_serial.serial().printf(" 0x%2.2X", misoData[index]); 03576 } 03577 cmdLine_serial.serial().printf(" "); 03578 } 03579 #endif // MAX5171_ONSPIPRINT 03580 03581 //-------------------------------------------------- 03582 void InitializeConfiguration() 03583 { 03584 // CODE GENERATOR: example code: member function Init 03585 # if HAS_DAPLINK_SERIAL 03586 cmdLine_DAPLINKserial.serial().printf("\r\nMAX5171_Init()"); 03587 03588 # endif 03589 cmdLine_serial.serial().printf("\r\nMAX5171_Init()"); 03590 03591 g_MAX5171_device.Init(); // defined in #include MAX5171.h 03592 # if MAX5171_ONSPIPRINT 03593 // Optional Diagnostic function to print SPI transactions 03594 # if MAX5171_ONSPIPRINT_ENABLED 03595 g_MAX5171_device.onSPIprint = onSPIprint_handler; 03596 # else 03597 g_MAX5171_device.onSPIprint = NULL; 03598 # endif 03599 # endif 03600 } // end of void InitializeConfiguration() 03601 03602 //-------------------------------------------------- 03603 // diagnostic rbg led GREEN 03604 void diagnostic_led_EOF() 03605 { 03606 #if USE_LEDS 03607 led1 = LED_ON; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led RED+GREEN=YELLOW 03608 // TODO1: mbed-os-5.11: [Warning] 'static osStatus rtos::Thread::wait(uint32_t)' is deprecated: Static methods only affecting current thread cause confusion. Replaced by ThisThread::sleep_for. [since mbed-os-5.10] [-Wdeprecated-declarations] 03609 ThisThread::sleep_for(250); // [since mbed-os-5.10] vs Thread::wait(250); 03610 led1 = LED_OFF; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led GREEN 03611 ThisThread::sleep_for(250); // [since mbed-os-5.10] vs Thread::wait(250); 03612 led1 = LED_ON; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led RED+GREEN=YELLOW 03613 ThisThread::sleep_for(250); // [since mbed-os-5.10] vs Thread::wait(250); 03614 led1 = LED_OFF; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led GREEN 03615 ThisThread::sleep_for(250); // [since mbed-os-5.10] vs Thread::wait(250); 03616 #endif // USE_LEDS 03617 } 03618 03619 //-------------------------------------------------- 03620 // Support commands that get handled immediately w/o waiting for EOL 03621 // handled as immediate command, do not append to buffer 03622 void on_immediate_0x21() // Unicode (U+0021) ! EXCLAMATION MARK 03623 { 03624 #if USE_LEDS 03625 led1 = LED_OFF; led2 = LED_OFF; led3 = LED_ON; // diagnostic rbg led BLUE 03626 #endif // USE_LEDS 03627 InitializeConfiguration(); 03628 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03629 } 03630 03631 //-------------------------------------------------- 03632 // Support commands that get handled immediately w/o waiting for EOL 03633 // handled as immediate command, do not append to buffer 03634 void on_immediate_0x7b() // Unicode (U+007B) { LEFT CURLY BRACKET 03635 { 03636 #if HAS_BUTTON2_DEMO_INTERRUPT 03637 onButton2FallingEdge(); 03638 #endif 03639 } 03640 03641 //-------------------------------------------------- 03642 // Support commands that get handled immediately w/o waiting for EOL 03643 // handled as immediate command, do not append to buffer 03644 void on_immediate_0x7d() // Unicode (U+007D) } RIGHT CURLY BRACKET 03645 { 03646 #if HAS_BUTTON1_DEMO_INTERRUPT 03647 onButton1FallingEdge(); 03648 #endif 03649 } 03650 03651 //---------------------------------------- 03652 // example code main function 03653 int main() 03654 { 03655 // Configure serial ports 03656 cmdLine_serial.clear(); 03657 //~ cmdLine_serial.serial().printf("\r\n cmdLine_serial.serial().printf test\r\n"); 03658 cmdLine_serial.onEOLcommandParser = main_menu_onEOLcommandParser; 03659 cmdLine_serial.diagnostic_led_EOF = diagnostic_led_EOF; 03660 /// CmdLine::set_immediate_handler(char, functionPointer_void_void_on_immediate_0x21); 03661 cmdLine_serial.on_immediate_0x21 = on_immediate_0x21; 03662 cmdLine_serial.on_immediate_0x7b = on_immediate_0x7b; 03663 cmdLine_serial.on_immediate_0x7d = on_immediate_0x7d; 03664 # if HAS_DAPLINK_SERIAL 03665 cmdLine_DAPLINKserial.clear(); 03666 //~ cmdLine_DAPLINKserial.serial().printf("\r\n cmdLine_DAPLINKserial.serial().printf test\r\n"); 03667 cmdLine_DAPLINKserial.onEOLcommandParser = main_menu_onEOLcommandParser; 03668 /// @todo CmdLine::set_immediate_handler(char, functionPointer_void_void_on_immediate_0x21); 03669 cmdLine_DAPLINKserial.on_immediate_0x21 = on_immediate_0x21; 03670 cmdLine_DAPLINKserial.on_immediate_0x7b = on_immediate_0x7b; 03671 cmdLine_DAPLINKserial.on_immediate_0x7d = on_immediate_0x7d; 03672 # endif 03673 03674 03675 //print_banner(); 03676 03677 #if HAS_I2C 03678 // i2c init 03679 // declare in narrower scope: MAX32625MBED I2C i2cMaster(...) 03680 // i2cMaster.frequency(g_I2C_SCL_Hz); 03681 #else 03682 // Ensure that the unused I2C pins do not interfere with analog inputs A4 and A5 03683 #if HAS_digitalInOut14 03684 // DigitalInOut digitalInOut14(P1_6, PIN_INPUT, PullUp, 1); // P1_6 TARGET_MAX32635MBED A4/SDA (10pin digital connector) 03685 digitalInOut14.input(); 03686 #endif 03687 #if HAS_digitalInOut15 03688 // DigitalInOut digitalInOut15(P1_7, PIN_INPUT, PullUp, 1); // P1_7 TARGET_MAX32635MBED A5/SCL (10pin digital connector) 03689 digitalInOut15.input(); 03690 #endif 03691 #if HAS_digitalInOut16 03692 // DigitalInOut mode can be one of PullUp, PullDown, PullNone, OpenDrain 03693 // PullUp-->3.4V, PullDown-->1.7V, PullNone-->3.5V, OpenDrain-->0.00V 03694 //DigitalInOut digitalInOut16(P3_4, PIN_INPUT, OpenDrain, 0); // P3_4 TARGET_MAX32635MBED A4/SDA (6pin analog connector) 03695 digitalInOut16.input(); 03696 #endif 03697 #if HAS_digitalInOut17 03698 //DigitalInOut digitalInOut17(P3_5, PIN_INPUT, OpenDrain, 0); // P3_5 TARGET_MAX32635MBED A5/SCL (6pin analog connector) 03699 digitalInOut17.input(); 03700 #endif 03701 #endif // HAS_I2C 03702 03703 03704 #if USE_LEDS 03705 #if defined(TARGET_MAX32630) 03706 led1 = LED_ON; led2 = LED_OFF; led3 = LED_OFF; // diagnostic rbg led RED 03707 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03708 led1 = LED_OFF; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led GREEN 03709 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03710 led1 = LED_OFF; led2 = LED_OFF; led3 = LED_ON; // diagnostic rbg led BLUE 03711 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03712 led1 = LED_ON; led2 = LED_ON; led3 = LED_ON; // diagnostic rbg led RED+GREEN+BLUE=WHITE 03713 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03714 led1 = LED_OFF; led2 = LED_ON; led3 = LED_ON; // diagnostic rbg led GREEN+BLUE=CYAN 03715 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03716 led1 = LED_ON; led2 = LED_OFF; led3 = LED_ON; // diagnostic rbg led RED+BLUE=MAGENTA 03717 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03718 led1 = LED_ON; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led RED+GREEN=YELLOW 03719 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03720 led1 = LED_OFF; led2 = LED_OFF; led3 = LED_OFF; // diagnostic rbg led BLACK 03721 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03722 #elif defined(TARGET_MAX32625MBED) 03723 led1 = LED_ON; led2 = LED_OFF; led3 = LED_OFF; // diagnostic rbg led RED 03724 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03725 led1 = LED_OFF; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led GREEN 03726 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03727 led1 = LED_OFF; led2 = LED_OFF; led3 = LED_ON; // diagnostic rbg led BLUE 03728 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03729 led1 = LED_ON; led2 = LED_ON; led3 = LED_ON; // diagnostic rbg led RED+GREEN+BLUE=WHITE 03730 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03731 led1 = LED_OFF; led2 = LED_ON; led3 = LED_ON; // diagnostic rbg led GREEN+BLUE=CYAN 03732 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03733 led1 = LED_ON; led2 = LED_OFF; led3 = LED_ON; // diagnostic rbg led RED+BLUE=MAGENTA 03734 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03735 led1 = LED_ON; led2 = LED_ON; led3 = LED_OFF; // diagnostic rbg led RED+GREEN=YELLOW 03736 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03737 led1 = LED_OFF; led2 = LED_OFF; led3 = LED_OFF; // diagnostic rbg led BLACK 03738 ThisThread::sleep_for(125); // [since mbed-os-5.10] vs Thread::wait(125); 03739 #else // not defined(TARGET_LPC1768 etc.) 03740 led1 = LED_ON; 03741 led2 = LED_OFF; 03742 led3 = LED_OFF; 03743 led4 = LED_OFF; 03744 ThisThread::sleep_for(75); // [since mbed-os-5.10] vs Thread::wait(75); 03745 //led1 = LED_ON; 03746 led2 = LED_ON; 03747 ThisThread::sleep_for(75); // [since mbed-os-5.10] vs Thread::wait(75); 03748 led1 = LED_OFF; 03749 //led2 = LED_ON; 03750 led3 = LED_ON; 03751 ThisThread::sleep_for(75); // [since mbed-os-5.10] vs Thread::wait(75); 03752 led2 = LED_OFF; 03753 //led3 = LED_ON; 03754 led4 = LED_ON; 03755 ThisThread::sleep_for(75); // [since mbed-os-5.10] vs Thread::wait(75); 03756 led3 = LED_OFF; 03757 led4 = LED_ON; 03758 // 03759 #endif // target definition 03760 #endif 03761 03762 // cmd_TE(); 03763 03764 // #if USE_LEDS 03765 // rgb_led.white(); // diagnostic rbg led RED+GREEN+BLUE=WHITE 03766 // #endif // USE_LEDS 03767 if (led1.is_connected()) { 03768 led1 = LED_ON; 03769 } 03770 if (led2.is_connected()) { 03771 led2 = LED_ON; 03772 } 03773 if (led3.is_connected()) { 03774 led3 = LED_ON; 03775 } 03776 03777 InitializeConfiguration(); 03778 // example code: serial port banner message 03779 #if defined(TARGET_MAX32625MBED) 03780 serial.printf("MAX32625MBED "); 03781 #elif defined(TARGET_MAX32600MBED) 03782 serial.printf("MAX32600MBED "); 03783 #elif defined(TARGET_NUCLEO_F446RE) 03784 serial.printf("NUCLEO_F446RE "); 03785 #endif 03786 serial.printf("MAX5171BOB\r\n"); 03787 03788 03789 while (1) { 03790 #if HAS_BUTTON1_DEMO_INTERRUPT_POLLING 03791 // avoid runtime error on button1 press [mbed-os-5.11] 03792 // instead of using InterruptIn, use DigitalIn and poll in main while(1) 03793 # if HAS_BUTTON1_DEMO_INTERRUPT 03794 static int button1_value_prev = 1; 03795 static int button1_value_now = 1; 03796 button1_value_prev = button1_value_now; 03797 button1_value_now = button1.read(); 03798 if ((button1_value_prev - button1_value_now) == 1) 03799 { 03800 // on button1 falling edge (button1 press) 03801 onButton1FallingEdge(); 03802 } 03803 # endif // HAS_BUTTON1_DEMO_INTERRUPT 03804 # if HAS_BUTTON2_DEMO_INTERRUPT 03805 static int button2_value_prev = 1; 03806 static int button2_value_now = 1; 03807 button2_value_prev = button2_value_now; 03808 button2_value_now = button2.read(); 03809 if ((button2_value_prev - button2_value_now) == 1) 03810 { 03811 // on button2 falling edge (button2 press) 03812 onButton2FallingEdge(); 03813 } 03814 # endif // HAS_BUTTON2_DEMO_INTERRUPT 03815 #endif 03816 # if HAS_DAPLINK_SERIAL 03817 if (DAPLINKserial.readable()) { 03818 cmdLine_DAPLINKserial.append(DAPLINKserial.getc()); 03819 } 03820 # endif // HAS_DAPLINK_SERIAL 03821 if (serial.readable()) { 03822 int c = serial.getc(); 03823 cmdLine_serial.append(c); 03824 #if IGNORE_AT_COMMANDS 03825 # if HAS_DAPLINK_SERIAL 03826 cmdLine_DAPLINKserial.serial().printf("%c", c); 03827 # endif // HAS_DAPLINK_SERIAL 03828 #endif // IGNORE_AT_COMMANDS 03829 // 03830 } 03831 } // while(1) 03832 }
Generated on Thu Jul 14 2022 04:42:56 by
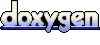