
Sample host software for the Maxim Integrated DS1775, DS75 Digital Thermometer Thermostat IC hosted on the MAX32630FTHR. The DS1775 is suitable for PCs, cell phones, and other thermally sensitive systems.
Dependencies: max32630fthr DS1775_Digitial_Thermometer_Thermostat USBDevice
main.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2019 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #include "mbed.h" 00034 #include "max32630fthr.h" 00035 #include "ds1775.h" 00036 #include "ds1775_cpp.h" 00037 #include "USBSerial.h" 00038 00039 MAX32630FTHR pegasus(MAX32630FTHR::VIO_3V3); 00040 00041 DigitalOut rLED(LED1); 00042 DigitalOut gLED(LED2); 00043 DigitalOut bLED(LED3); 00044 00045 I2C i2cBus(P3_4, P3_5); 00046 00047 Serial pc(USBTX, USBRX); /* Use USB debug probe for serial link */ 00048 Serial uart(P2_1, P2_0); 00049 uint32_t blink_cnt; /* counter for blinking the colors */ 00050 void wait_blink_colors(uint8_t dly) { 00051 uint32_t i; 00052 for (i = 0; i < dly; i++) { 00053 wait(.5); 00054 if (blink_cnt & 1) 00055 gLED = !gLED; 00056 if (blink_cnt & 2) 00057 bLED = !bLED; 00058 if (blink_cnt & 4) 00059 rLED = !rLED; 00060 blink_cnt++; 00061 wait(.5); 00062 } 00063 } 00064 00065 void wait_sec_prompt(uint8_t time) 00066 { 00067 // Ports and serial connections 00068 uint32_t i; 00069 for (i = 0; i < time; i++) { 00070 pc.printf("."); 00071 wait_blink_colors(1); 00072 } 00073 pc.printf("\r\n"); 00074 } 00075 00076 00077 // main() runs in its own thread in the OS 00078 // (note the calls to Thread::wait below for delays) 00079 /** 00080 * @brief Sample main program for DS1775 00081 * @version 1.0000.0003 00082 * 00083 * @details Sample main program for DS1775 00084 * The prints are sent to the terminal window (9600, 8n1). 00085 * The program sets the GPIOs to 3.3V and the program 00086 * configures the chip and reads temperatures. 00087 * To run the program, drag and drop the .bin file into the 00088 * DAPLINK folder. After it finishes flashing, cycle the power or 00089 * reset the Pegasus (MAX32630FTHR) after flashing by pressing the button on 00090 * the Pegasus next to the battery connector or the button 00091 * on the MAXREFDES100HDK. 00092 */ 00093 int main() 00094 { 00095 uint32_t i; 00096 float temperature; 00097 uint8_t cfg; 00098 uint8_t delay = 30; /* shutdown wait time */ 00099 DigitalOut rLED(LED1, LED_OFF); 00100 DigitalOut gLED(LED2, LED_OFF); 00101 DigitalOut bLED(LED3, LED_OFF); 00102 gLED = LED_ON; 00103 pc.baud(9600); // Baud rate = 115200 00104 pc.printf("DS1775 DS75 Digital Thermometer and " 00105 "Thermostat example source code.\r\n"); 00106 pc.printf("\r\n"); 00107 00108 DS1775 temp_sensor(i2cBus, DS1775_I2C_SLAVE_ADR_R0); 00109 i2cBus.frequency(400000); 00110 /* Configure for 9 bit resolution, fault filter 1, 00111 active low, comparator, continuous, 00112 */ 00113 temp_sensor.write_cfg_reg(uint8_t(DS1775_CFG_RESOLUTION_9BIT | 00114 DS1775_CFG_FAULT_FILTER_6 | DS1775_CFG_OS_POLARITY_ACT_LOW | 00115 DS1775_CFG_COMPARATOR_MODE | DS1775_CFG_CONTINUOUS)); 00116 for (i = 0; i < 10; i++) { 00117 wait(DS1775_WAIT_CONV_TIME_9BIT); 00118 temperature = 00119 temp_sensor.read_reg_as_temperature(DS1775_REG_TEMPERATURE); 00120 pc.printf("Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00121 temperature, temp_sensor.celsius_to_fahrenheit(temperature)); 00122 } 00123 temp_sensor.read_cfg_reg(&cfg); 00124 pc.printf("Configuration Register = 0x%02Xh \r\n", cfg); 00125 00126 #if 0 00127 temp_sensor.write_trip_low_thyst(-63.9375); 00128 temperature = 00129 temp_sensor.read_reg_as_temperature(DS1775_REG_THYST_LOW_TRIP); 00130 pc.printf("Thyst Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00131 temperature, temp_sensor.celsius_to_fahrenheit(temperature)); 00132 00133 temp_sensor.write_trip_high_tos(64.0625f); 00134 temperature = temp_sensor.read_reg_as_temperature(DS1775_REG_TOS_HIGH_TRIP); 00135 pc.printf("TOS Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00136 temperature, temp_sensor.celsius_to_fahrenheit(temperature)); 00137 pc.printf("\r\n\r\n"); 00138 #endif 00139 00140 pc.printf("\r\n"); 00141 00142 for (i = 0; i < 8; i++) { 00143 temp_sensor.write_cfg_reg(uint8_t(DS1775_CFG_RESOLUTION_12BIT | 00144 DS1775_CFG_FAULT_FILTER_6 | DS1775_CFG_OS_POLARITY_ACT_LOW | 00145 DS1775_CFG_INTERRUPT_MODE | DS1775_CFG_SHUTDOWN)); 00146 wait_sec_prompt(delay); /* leave it in shutdown mode for a while */ 00147 /* Configure for 12 bit resolution, fault filter 6, 00148 active low, interrupt, continous, 00149 */ 00150 temp_sensor.write_cfg_reg(uint8_t(DS1775_CFG_RESOLUTION_12BIT | 00151 DS1775_CFG_FAULT_FILTER_6 | DS1775_CFG_OS_POLARITY_ACT_LOW | 00152 DS1775_CFG_INTERRUPT_MODE | DS1775_CFG_CONTINUOUS)); 00153 wait(DS1775_WAIT_CONV_TIME_12BIT); 00154 temperature = 00155 temp_sensor.read_reg_as_temperature(DS1775_REG_TEMPERATURE); 00156 pc.printf("Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00157 temperature, temp_sensor.celsius_to_fahrenheit(temperature)); 00158 } 00159 temp_sensor.write_cfg_reg(uint8_t(DS1775_CFG_RESOLUTION_12BIT | 00160 DS1775_CFG_FAULT_FILTER_6 | DS1775_CFG_OS_POLARITY_ACT_LOW | 00161 DS1775_CFG_INTERRUPT_MODE | DS1775_CFG_SHUTDOWN)); 00162 temp_sensor.read_cfg_reg(&cfg); 00163 pc.printf("Configuration Register = 0x%02Xh \r\n", cfg); 00164 00165 pc.printf("\r\n\r\n"); 00166 00167 #if 0 00168 temp_sensor.write_trip_low_thyst(-55.0f); 00169 temperature = 00170 temp_sensor.read_reg_as_temperature(DS1775_REG_THYST_LOW_TRIP); 00171 pc.printf("Thyst Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00172 temperature, temp_sensor.celsius_to_fahrenheit(temperature)); 00173 00174 temp_sensor.write_trip_high_tos(125.0f); 00175 temperature = temp_sensor.read_reg_as_temperature(DS1775_REG_TOS_HIGH_TRIP); 00176 pc.printf("TOS Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00177 temperature, temp_sensor.celsius_to_fahrenheit(temperature)); 00178 pc.printf("\r\n\r\n"); 00179 #endif 00180 00181 /*************************************************************************** 00182 * Call the C code version of the driver 00183 *************************************************************************** 00184 */ 00185 #include "ds1775_c.h" 00186 pc.printf("C implementation of the code\r\n"); 00187 ds1775_init(DS1775_I2C_SLAVE_ADR_R0); 00188 /* Configure for 9 bit resolution, fault filter 1, 00189 active low, comparator, continuous, 00190 */ 00191 ds1775_write_cfg_reg(uint8_t(DS1775_CFG_RESOLUTION_9BIT | 00192 DS1775_CFG_FAULT_FILTER_6 | DS1775_CFG_OS_POLARITY_ACT_LOW | 00193 DS1775_CFG_COMPARATOR_MODE | DS1775_CFG_CONTINUOUS), i2cBus); 00194 for (i = 0; i < 10; i++) { 00195 wait(DS1775_WAIT_CONV_TIME_9BIT); 00196 temperature = ds1775_read_reg_as_temperature(DS1775_REG_TEMPERATURE, 00197 i2cBus); 00198 pc.printf("Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00199 temperature, temp_sensor.celsius_to_fahrenheit(temperature)); 00200 } 00201 00202 ds1775_read_cfg_reg(&cfg, i2cBus); 00203 pc.printf("Configuration Register = 0x%02Xh \r\n", cfg); 00204 00205 #if 0 00206 ds1775_write_trip_low_thyst(-63.9375, i2cBus); 00207 temperature = ds1775_read_reg_as_temperature(DS1775_REG_THYST_LOW_TRIP, 00208 i2cBus); 00209 pc.printf("Thyst Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00210 temperature, ds1775_celsius_to_fahrenheit(temperature)); 00211 00212 ds1775_write_trip_high_tos(64.0625f, i2cBus); 00213 temperature = ds1775_read_reg_as_temperature(DS1775_REG_TOS_HIGH_TRIP, 00214 i2cBus); 00215 pc.printf("TOS Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00216 temperature, ds1775_celsius_to_fahrenheit(temperature)); 00217 #endif 00218 00219 pc.printf("\r\n"); 00220 for (i = 0; i < 8; i++) { 00221 ds1775_write_cfg_reg(uint8_t(DS1775_CFG_RESOLUTION_12BIT | 00222 DS1775_CFG_FAULT_FILTER_6 | DS1775_CFG_OS_POLARITY_ACT_LOW | 00223 DS1775_CFG_INTERRUPT_MODE | DS1775_CFG_SHUTDOWN), i2cBus); 00224 wait_sec_prompt(delay); /* leave it in shutdown mode for a while */ 00225 /* Configure for 12 bit resolution, fault filter 6, 00226 active low, interrupt, continous, 00227 */ 00228 ds1775_write_cfg_reg(uint8_t(DS1775_CFG_RESOLUTION_12BIT | 00229 DS1775_CFG_FAULT_FILTER_6 | DS1775_CFG_OS_POLARITY_ACT_LOW | 00230 DS1775_CFG_INTERRUPT_MODE | DS1775_CFG_CONTINUOUS), 00231 i2cBus); 00232 wait(DS1775_WAIT_CONV_TIME_12BIT); 00233 temperature = ds1775_read_reg_as_temperature(DS1775_REG_TEMPERATURE, 00234 i2cBus); 00235 pc.printf("Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00236 temperature, temp_sensor.celsius_to_fahrenheit(temperature)); 00237 } 00238 ds1775_write_cfg_reg(uint8_t(DS1775_CFG_RESOLUTION_12BIT | 00239 DS1775_CFG_FAULT_FILTER_6 | DS1775_CFG_OS_POLARITY_ACT_LOW | 00240 DS1775_CFG_INTERRUPT_MODE | DS1775_CFG_SHUTDOWN), i2cBus); 00241 ds1775_read_cfg_reg(&cfg, i2cBus); 00242 pc.printf("Configuration Register = 0x%02Xh \r\n", cfg); 00243 00244 #if 0 00245 ds1775_write_trip_low_thyst(-55, i2cBus); 00246 temperature = ds1775_read_reg_as_temperature(DS1775_REG_THYST_LOW_TRIP, 00247 i2cBus); 00248 pc.printf("Thyst Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00249 temperature, ds1775_celsius_to_fahrenheit(temperature)); 00250 00251 ds1775_write_trip_high_tos(125.0f, i2cBus); 00252 temperature = ds1775_read_reg_as_temperature(DS1775_REG_TOS_HIGH_TRIP, 00253 i2cBus); 00254 pc.printf("TOS Temperature = %3.4f Celsius, %3.4f Fahrenheit\r\n", 00255 temperature, ds1775_celsius_to_fahrenheit(temperature)); 00256 #endif 00257 pc.printf("\r\n\r\n\r\n"); 00258 00259 00260 while (true) { // Blink the green LED 00261 gLED = !gLED; 00262 wait(0.5); 00263 } 00264 } 00265 00266
Generated on Fri Jul 15 2022 10:21:51 by
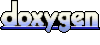