
MBED firmware for MAXREFDES117 Heart Rate / SpO2 sensor. Tested on KL25Z, K64F, and MAX32600MBED#
Dependencies: mbed
MAX30102.cpp
00001 /** \file max30102.cpp ****************************************************** 00002 * 00003 * Project: MAXREFDES117# 00004 * Filename: max30102.cpp 00005 * Description: This module is an embedded controller driver for the MAX30102 00006 * 00007 * 00008 * -------------------------------------------------------------------- 00009 * 00010 * This code follows the following naming conventions: 00011 * 00012 * char ch_pmod_value 00013 * char (array) s_pmod_s_string[16] 00014 * float f_pmod_value 00015 * int32_t n_pmod_value 00016 * int32_t (array) an_pmod_value[16] 00017 * int16_t w_pmod_value 00018 * int16_t (array) aw_pmod_value[16] 00019 * uint16_t uw_pmod_value 00020 * uint16_t (array) auw_pmod_value[16] 00021 * uint8_t uch_pmod_value 00022 * uint8_t (array) auch_pmod_buffer[16] 00023 * uint32_t un_pmod_value 00024 * int32_t * pn_pmod_value 00025 * 00026 * ------------------------------------------------------------------------- */ 00027 /******************************************************************************* 00028 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00029 * 00030 * Permission is hereby granted, free of charge, to any person obtaining a 00031 * copy of this software and associated documentation files (the "Software"), 00032 * to deal in the Software without restriction, including without limitation 00033 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00034 * and/or sell copies of the Software, and to permit persons to whom the 00035 * Software is furnished to do so, subject to the following conditions: 00036 * 00037 * The above copyright notice and this permission notice shall be included 00038 * in all copies or substantial portions of the Software. 00039 * 00040 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00041 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00042 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00043 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00044 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00045 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00046 * OTHER DEALINGS IN THE SOFTWARE. 00047 * 00048 * Except as contained in this notice, the name of Maxim Integrated 00049 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00050 * Products, Inc. Branding Policy. 00051 * 00052 * The mere transfer of this software does not imply any licenses 00053 * of trade secrets, proprietary technology, copyrights, patents, 00054 * trademarks, maskwork rights, or any other form of intellectual 00055 * property whatsoever. Maxim Integrated Products, Inc. retains all 00056 * ownership rights. 00057 ******************************************************************************* 00058 */ 00059 #include "mbed.h" 00060 #include "MAX30102.h" 00061 00062 #ifdef TARGET_MAX32600MBED 00063 I2C i2c(I2C1_SDA, I2C1_SCL); 00064 #else 00065 I2C i2c(I2C_SDA, I2C_SCL); 00066 #endif 00067 00068 bool maxim_max30102_write_reg(uint8_t uch_addr, uint8_t uch_data) 00069 /** 00070 * \brief Write a value to a MAX30102 register 00071 * \par Details 00072 * This function writes a value to a MAX30102 register 00073 * 00074 * \param[in] uch_addr - register address 00075 * \param[in] uch_data - register data 00076 * 00077 * \retval true on success 00078 */ 00079 { 00080 char ach_i2c_data[2]; 00081 ach_i2c_data[0]=uch_addr; 00082 ach_i2c_data[1]=uch_data; 00083 00084 if(i2c.write(I2C_WRITE_ADDR, ach_i2c_data, 2, false)==0) 00085 return true; 00086 else 00087 return false; 00088 } 00089 00090 bool maxim_max30102_read_reg(uint8_t uch_addr, uint8_t *puch_data) 00091 /** 00092 * \brief Read a MAX30102 register 00093 * \par Details 00094 * This function reads a MAX30102 register 00095 * 00096 * \param[in] uch_addr - register address 00097 * \param[out] puch_data - pointer that stores the register data 00098 * 00099 * \retval true on success 00100 */ 00101 { 00102 char ch_i2c_data; 00103 ch_i2c_data=uch_addr; 00104 if(i2c.write(I2C_WRITE_ADDR, &ch_i2c_data, 1, true)!=0) 00105 return false; 00106 if(i2c.read(I2C_READ_ADDR, &ch_i2c_data, 1, false)==0) 00107 { 00108 *puch_data=(uint8_t) ch_i2c_data; 00109 return true; 00110 } 00111 else 00112 return false; 00113 } 00114 00115 bool maxim_max30102_init() 00116 /** 00117 * \brief Initialize the MAX30102 00118 * \par Details 00119 * This function initializes the MAX30102 00120 * 00121 * \param None 00122 * 00123 * \retval true on success 00124 */ 00125 { 00126 if(!maxim_max30102_write_reg(REG_INTR_ENABLE_1,0xc0)) // INTR setting 00127 return false; 00128 if(!maxim_max30102_write_reg(REG_INTR_ENABLE_2,0x00)) 00129 return false; 00130 if(!maxim_max30102_write_reg(REG_FIFO_WR_PTR,0x00)) //FIFO_WR_PTR[4:0] 00131 return false; 00132 if(!maxim_max30102_write_reg(REG_OVF_COUNTER,0x00)) //OVF_COUNTER[4:0] 00133 return false; 00134 if(!maxim_max30102_write_reg(REG_FIFO_RD_PTR,0x00)) //FIFO_RD_PTR[4:0] 00135 return false; 00136 if(!maxim_max30102_write_reg(REG_FIFO_CONFIG,0x0f)) //sample avg = 1, fifo rollover=false, fifo almost full = 17 00137 return false; 00138 if(!maxim_max30102_write_reg(REG_MODE_CONFIG,0x03)) //0x02 for Red only, 0x03 for SpO2 mode 0x07 multimode LED 00139 return false; 00140 if(!maxim_max30102_write_reg(REG_SPO2_CONFIG,0x27)) // SPO2_ADC range = 4096nA, SPO2 sample rate (100 Hz), LED pulseWidth (400uS) 00141 return false; 00142 00143 if(!maxim_max30102_write_reg(REG_LED1_PA,0x24)) //Choose value for ~ 7mA for LED1 00144 return false; 00145 if(!maxim_max30102_write_reg(REG_LED2_PA,0x24)) // Choose value for ~ 7mA for LED2 00146 return false; 00147 if(!maxim_max30102_write_reg(REG_PILOT_PA,0x7f)) // Choose value for ~ 25mA for Pilot LED 00148 return false; 00149 return true; 00150 } 00151 00152 bool maxim_max30102_read_fifo(uint32_t *pun_red_led, uint32_t *pun_ir_led) 00153 /** 00154 * \brief Read a set of samples from the MAX30102 FIFO register 00155 * \par Details 00156 * This function reads a set of samples from the MAX30102 FIFO register 00157 * 00158 * \param[out] *pun_red_led - pointer that stores the red LED reading data 00159 * \param[out] *pun_ir_led - pointer that stores the IR LED reading data 00160 * 00161 * \retval true on success 00162 */ 00163 { 00164 uint32_t un_temp; 00165 unsigned char uch_temp; 00166 *pun_red_led=0; 00167 *pun_ir_led=0; 00168 char ach_i2c_data[6]; 00169 00170 //read and clear status register 00171 maxim_max30102_read_reg(REG_INTR_STATUS_1, &uch_temp); 00172 maxim_max30102_read_reg(REG_INTR_STATUS_2, &uch_temp); 00173 00174 ach_i2c_data[0]=REG_FIFO_DATA; 00175 if(i2c.write(I2C_WRITE_ADDR, ach_i2c_data, 1, true)!=0) 00176 return false; 00177 if(i2c.read(I2C_READ_ADDR, ach_i2c_data, 6, false)!=0) 00178 { 00179 return false; 00180 } 00181 un_temp=(unsigned char) ach_i2c_data[0]; 00182 un_temp<<=16; 00183 *pun_red_led+=un_temp; 00184 un_temp=(unsigned char) ach_i2c_data[1]; 00185 un_temp<<=8; 00186 *pun_red_led+=un_temp; 00187 un_temp=(unsigned char) ach_i2c_data[2]; 00188 *pun_red_led+=un_temp; 00189 00190 un_temp=(unsigned char) ach_i2c_data[3]; 00191 un_temp<<=16; 00192 *pun_ir_led+=un_temp; 00193 un_temp=(unsigned char) ach_i2c_data[4]; 00194 un_temp<<=8; 00195 *pun_ir_led+=un_temp; 00196 un_temp=(unsigned char) ach_i2c_data[5]; 00197 *pun_ir_led+=un_temp; 00198 *pun_red_led&=0x03FFFF; //Mask MSB [23:18] 00199 *pun_ir_led&=0x03FFFF; //Mask MSB [23:18] 00200 00201 00202 return true; 00203 } 00204 00205 bool maxim_max30102_reset() 00206 /** 00207 * \brief Reset the MAX30102 00208 * \par Details 00209 * This function resets the MAX30102 00210 * 00211 * \param None 00212 * 00213 * \retval true on success 00214 */ 00215 { 00216 if(!maxim_max30102_write_reg(REG_MODE_CONFIG,0x40)) 00217 return false; 00218 else 00219 return true; 00220 }
Generated on Tue Jul 12 2022 11:56:15 by
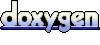