Device interface library for multiple platforms including Mbed.
Dependents: DeepCover Embedded Security in IoT MaximInterface MAXREFDES155#
RomCommands.cpp
00001 /******************************************************************************* 00002 * Copyright (C) Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 *******************************************************************************/ 00032 00033 #include "RomCommands.hpp" 00034 00035 namespace MaximInterfaceCore { 00036 00037 void skipCurrentFamily(SearchRomState & searchState) { 00038 // Set the last discrepancy to last family discrepancy. 00039 searchState.lastDiscrepancy = searchState.lastFamilyDiscrepancy; 00040 // Clear the last family discrepancy. 00041 searchState.lastFamilyDiscrepancy = 0; 00042 // Check for end of list. 00043 if (searchState.lastDiscrepancy == 0) { 00044 searchState.lastDevice = true; 00045 } 00046 } 00047 00048 Result<void> verifyRom(OneWireMaster & master, RomId::const_span romId) { 00049 SearchRomState searchState(romId); 00050 Result<void> result = searchRom(master, searchState); 00051 if (!result) { 00052 return result; 00053 } 00054 // Check if same device found. 00055 if (!equal(romId, make_span(searchState.romId))) { 00056 result = OneWireMaster::NoSlaveError; 00057 } 00058 return result; 00059 } 00060 00061 Result<RomId::array> readRom(OneWireMaster & master) { 00062 Result<void> result = master.reset(); 00063 if (!result) { 00064 return result.error(); 00065 } 00066 result = master.writeByte(0x33); 00067 if (!result) { 00068 return result.error(); 00069 } 00070 RomId::array romId; 00071 result = master.readBlock(romId); 00072 if (!result) { 00073 return result.error(); 00074 } 00075 if (!valid(romId)) { 00076 return OneWireMaster::NoSlaveError; 00077 } 00078 return romId; 00079 } 00080 00081 Result<void> skipRom(OneWireMaster & master) { 00082 Result<void> result = master.reset(); 00083 if (!result) { 00084 return result; 00085 } 00086 result = master.writeByte(0xCC); 00087 return result; 00088 } 00089 00090 Result<void> matchRom(OneWireMaster & master, RomId::const_span romId) { 00091 Result<void> result = master.reset(); 00092 if (!result) { 00093 return result; 00094 } 00095 result = master.writeByte(0x55); 00096 if (!result) { 00097 return result; 00098 } 00099 result = master.writeBlock(romId); 00100 return result; 00101 } 00102 00103 Result<void> overdriveSkipRom(OneWireMaster & master) { 00104 Result<void> result = master.reset(); 00105 if (!result) { 00106 return result; 00107 } 00108 result = master.writeByte(0x3C); 00109 if (!result) { 00110 return result; 00111 } 00112 result = master.setSpeed(OneWireMaster::OverdriveSpeed); 00113 return result; 00114 } 00115 00116 Result<void> overdriveMatchRom(OneWireMaster & master, 00117 RomId::const_span romId) { 00118 Result<void> result = master.reset(); 00119 if (!result) { 00120 return result; 00121 } 00122 result = master.writeByte(0x69); 00123 if (!result) { 00124 return result; 00125 } 00126 result = master.setSpeed(OneWireMaster::OverdriveSpeed); 00127 if (!result) { 00128 return result; 00129 } 00130 result = master.writeBlock(romId); 00131 return result; 00132 } 00133 00134 Result<void> resumeRom(OneWireMaster & master) { 00135 Result<void> result = master.reset(); 00136 if (!result) { 00137 return result; 00138 } 00139 result = master.writeByte(0xA5); 00140 return result; 00141 } 00142 00143 Result<void> searchRom(OneWireMaster & master, SearchRomState & searchState) { 00144 if (searchState.lastDevice) { 00145 searchState = SearchRomState(); 00146 } 00147 00148 Result<void> result = master.reset(); 00149 if (!result) { 00150 return result; 00151 } 00152 result = master.writeByte(0xF0); 00153 if (!result) { 00154 return result; 00155 } 00156 00157 SearchRomState newSearchState; 00158 newSearchState.lastFamilyDiscrepancy = searchState.lastFamilyDiscrepancy; 00159 for (int idBitNumber = 1; idBitNumber <= 64; ++idBitNumber) { 00160 const int idByteNumber = (idBitNumber - 1) / 8; 00161 const unsigned int idBitMask = 1 << ((idBitNumber - 1) % 8); 00162 00163 OneWireMaster::TripletData tripletData; 00164 if (idBitNumber == searchState.lastDiscrepancy) { 00165 tripletData.writeBit = 1; 00166 } else if (idBitNumber > searchState.lastDiscrepancy) { 00167 tripletData.writeBit = 0; 00168 } else { // idBitNumber < searchState.lastDiscrepancy 00169 tripletData.writeBit = 00170 (searchState.romId[idByteNumber] & idBitMask) == idBitMask; 00171 } 00172 00173 MaximInterfaceCore_TRY_VALUE(tripletData, 00174 master.triplet(tripletData.writeBit)); 00175 if (tripletData.readBit && tripletData.readBitComplement) { 00176 return OneWireMaster::NoSlaveError; 00177 } 00178 00179 if (tripletData.writeBit) { 00180 newSearchState.romId[idByteNumber] |= idBitMask; 00181 } else if (!tripletData.readBit && !tripletData.readBitComplement) { 00182 newSearchState.lastDiscrepancy = idBitNumber; 00183 if (idBitNumber <= 8) { 00184 newSearchState.lastFamilyDiscrepancy = idBitNumber; 00185 } 00186 } 00187 } 00188 00189 if (valid(newSearchState.romId)) { 00190 if (newSearchState.lastDiscrepancy == 0) { 00191 newSearchState.lastDevice = true; 00192 } 00193 searchState = newSearchState; 00194 } else { 00195 result = OneWireMaster::NoSlaveError; 00196 } 00197 return result; 00198 } 00199 00200 } // namespace MaximInterfaceCore
Generated on Tue Jul 12 2022 11:13:16 by
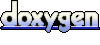