Device interface library for multiple platforms including Mbed.
Dependents: DeepCover Embedded Security in IoT MaximInterface MAXREFDES155#
LoggingI2CMaster.cpp
00001 /******************************************************************************* 00002 * Copyright (C) Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 *******************************************************************************/ 00032 00033 #include <utility> 00034 #include "HexString.hpp" 00035 #include "LoggingI2CMaster.hpp" 00036 00037 using std::string; 00038 00039 namespace MaximInterfaceCore { 00040 00041 static const char startString[] = "S "; 00042 static const char stopString[] = "P"; 00043 00044 static string formatDataString(span<const uint_least8_t> data, bool read) { 00045 string dataBuilder; 00046 for (span<const uint_least8_t>::index_type i = 0; i < data.size(); ++i) { 00047 if (read) { 00048 dataBuilder.append(1, '['); 00049 } 00050 dataBuilder.append(toHexString(data.subspan(i, 1))); 00051 if (read) { 00052 dataBuilder.append(1, ']'); 00053 } 00054 dataBuilder.append(1, ' '); 00055 } 00056 return dataBuilder; 00057 } 00058 00059 void LoggingI2CMaster::tryWriteMessage() { 00060 if (writeMessage) { 00061 writeMessage(messageBuilder); 00062 } 00063 messageBuilder.clear(); 00064 } 00065 00066 Result<void> LoggingI2CMaster::start(uint_least8_t address) { 00067 messageBuilder.append(startString); 00068 messageBuilder.append(formatDataString(make_span(&address, 1), false)); 00069 return I2CMasterDecorator::start(address); 00070 } 00071 00072 Result<void> LoggingI2CMaster::stop() { 00073 messageBuilder.append(stopString); 00074 tryWriteMessage(); 00075 return I2CMasterDecorator::stop(); 00076 } 00077 00078 Result<void> LoggingI2CMaster::writeByte(uint_least8_t data) { 00079 messageBuilder.append(formatDataString(make_span(&data, 1), false)); 00080 return I2CMasterDecorator::writeByte(data); 00081 } 00082 00083 Result<void> LoggingI2CMaster::writeBlock(span<const uint_least8_t> data) { 00084 messageBuilder.append(formatDataString(data, false)); 00085 return I2CMasterDecorator::writeBlock(data); 00086 } 00087 00088 Result<void> LoggingI2CMaster::writePacket(uint_least8_t address, 00089 span<const uint_least8_t> data, 00090 DoStop doStop) { 00091 messageBuilder.append(startString); 00092 messageBuilder.append(formatDataString(make_span(&address, 1), false)); 00093 const Result<void> result = 00094 I2CMasterDecorator::writePacket(address, data, doStop); 00095 if (result) { 00096 messageBuilder.append(formatDataString(data, false)); 00097 } 00098 if (doStop == Stop || (doStop == StopOnError && !result)) { 00099 messageBuilder.append(stopString); 00100 tryWriteMessage(); 00101 } 00102 return result; 00103 } 00104 00105 Result<uint_least8_t> LoggingI2CMaster::readByte(DoAck doAck) { 00106 const Result<uint_least8_t> data = I2CMasterDecorator::readByte(doAck); 00107 if (data) { 00108 messageBuilder.append(formatDataString(make_span(&data.value(), 1), true)); 00109 } 00110 return data; 00111 } 00112 00113 Result<void> LoggingI2CMaster::readBlock(span<uint_least8_t> data, 00114 DoAck doAck) { 00115 const Result<void> result = I2CMasterDecorator::readBlock(data, doAck); 00116 if (result) { 00117 messageBuilder.append(formatDataString(data, true)); 00118 } 00119 return result; 00120 } 00121 00122 Result<void> LoggingI2CMaster::readPacket(uint_least8_t address, 00123 span<uint_least8_t> data, 00124 DoStop doStop) { 00125 messageBuilder.append(startString); 00126 messageBuilder.append(formatDataString(make_span(&address, 1), false)); 00127 const Result<void> result = 00128 I2CMasterDecorator::readPacket(address, data, doStop); 00129 if (result) { 00130 messageBuilder.append(formatDataString(data, true)); 00131 } 00132 if (doStop == Stop || (doStop == StopOnError && !result)) { 00133 messageBuilder.append(stopString); 00134 tryWriteMessage(); 00135 } 00136 return result; 00137 } 00138 00139 } // namespace MaximInterfaceCore
Generated on Tue Jul 12 2022 11:13:15 by
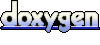