Device interface library for multiple platforms including Mbed.
Dependents: DeepCover Embedded Security in IoT MaximInterface MAXREFDES155#
DS9481P_300.hpp
00001 /******************************************************************************* 00002 * Copyright (C) Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 *******************************************************************************/ 00032 00033 #ifndef MaximInterfaceDevices_DS9481P_300_hpp 00034 #define MaximInterfaceDevices_DS9481P_300_hpp 00035 00036 #include <MaximInterfaceCore/I2CMasterDecorator.hpp> 00037 #include <MaximInterfaceCore/OneWireMasterDecorator.hpp> 00038 #include <MaximInterfaceCore/SerialPort.hpp> 00039 #include <MaximInterfaceCore/Sleep.hpp> 00040 #include "DS2480B.hpp" 00041 #include "DS9400.hpp" 00042 #include "Config.hpp" 00043 00044 namespace MaximInterfaceDevices { 00045 00046 /// DS9481P-300 USB to 1-Wire and I2C adapter. 00047 class DS9481P_300 { 00048 public: 00049 MaximInterfaceDevices_EXPORT DS9481P_300(Core::Sleep & sleep, 00050 Core::SerialPort & serialPort); 00051 00052 void setSleep(Core::Sleep & sleep) { ds2480b.setSleep(sleep); } 00053 00054 void setSerialPort(Core::SerialPort & serialPort) { 00055 this->serialPort = &serialPort; 00056 ds2480b.setUart(serialPort); 00057 ds9400.setUart(serialPort); 00058 } 00059 00060 MaximInterfaceDevices_EXPORT Core::Result<void> 00061 connect(const std::string & portName); 00062 00063 MaximInterfaceDevices_EXPORT Core::Result<void> disconnect(); 00064 00065 /// Access the 1-Wire master when connected to an adapter. 00066 Core::OneWireMaster & oneWireMaster() { return oneWireMaster_; } 00067 00068 /// Access the I2C master when connected to an adapter. 00069 Core::I2CMaster & i2cMaster() { return i2cMaster_; } 00070 00071 private: 00072 class OneWireMaster : public Core::OneWireMasterDecorator { 00073 public: 00074 explicit OneWireMaster(DS9481P_300 & parent) 00075 : OneWireMasterDecorator(parent.ds2480b), parent(&parent) {} 00076 00077 MaximInterfaceDevices_EXPORT virtual Core::Result<void> reset(); 00078 00079 MaximInterfaceDevices_EXPORT virtual Core::Result<bool> 00080 touchBitSetLevel(bool sendBit, Level afterLevel); 00081 00082 MaximInterfaceDevices_EXPORT virtual Core::Result<void> 00083 writeByteSetLevel(uint_least8_t sendByte, Level afterLevel); 00084 00085 MaximInterfaceDevices_EXPORT virtual Core::Result<uint_least8_t> 00086 readByteSetLevel(Level afterLevel); 00087 00088 MaximInterfaceDevices_EXPORT virtual Core::Result<void> 00089 writeBlock(Core::span<const uint_least8_t> sendBuf); 00090 00091 MaximInterfaceDevices_EXPORT virtual Core::Result<void> 00092 readBlock(Core::span<uint_least8_t> recvBuf); 00093 00094 MaximInterfaceDevices_EXPORT virtual Core::Result<void> 00095 setSpeed(Speed newSpeed); 00096 00097 MaximInterfaceDevices_EXPORT virtual Core::Result<void> 00098 setLevel(Level newLevel); 00099 00100 MaximInterfaceDevices_EXPORT virtual Core::Result<TripletData> 00101 triplet(bool sendBit); 00102 00103 private: 00104 DS9481P_300 * parent; 00105 }; 00106 00107 class I2CMaster : public Core::I2CMasterDecorator { 00108 public: 00109 explicit I2CMaster(DS9481P_300 & parent) 00110 : I2CMasterDecorator(parent.ds9400), parent(&parent) {} 00111 00112 MaximInterfaceDevices_EXPORT virtual Core::Result<void> 00113 start(uint_least8_t address); 00114 00115 MaximInterfaceDevices_EXPORT virtual Core::Result<void> stop(); 00116 00117 MaximInterfaceDevices_EXPORT virtual Core::Result<void> 00118 writeByte(uint_least8_t data); 00119 00120 MaximInterfaceDevices_EXPORT virtual Core::Result<void> 00121 writeBlock(Core::span<const uint_least8_t> data); 00122 00123 MaximInterfaceDevices_EXPORT virtual Core::Result<void> 00124 writePacket(uint_least8_t address, Core::span<const uint_least8_t> data, 00125 DoStop doStop); 00126 00127 MaximInterfaceDevices_EXPORT virtual Core::Result<uint_least8_t> 00128 readByte(DoAck doAck); 00129 00130 MaximInterfaceDevices_EXPORT virtual Core::Result<void> 00131 readBlock(Core::span<uint_least8_t> data, DoAck doAck); 00132 00133 MaximInterfaceDevices_EXPORT virtual Core::Result<void> 00134 readPacket(uint_least8_t address, Core::span<uint_least8_t> data, 00135 DoStop doStop); 00136 00137 private: 00138 DS9481P_300 * parent; 00139 }; 00140 00141 class DS2480BWithEscape : public DS2480B { 00142 public: 00143 DS2480BWithEscape(Core::Sleep & sleep, Core::Uart & uart) 00144 : DS2480B(sleep, uart) {} 00145 00146 Core::Result<void> escape(); 00147 }; 00148 00149 class DS9400WithEscape : public DS9400 { 00150 public: 00151 explicit DS9400WithEscape(Core::Uart & uart) : DS9400(uart) {} 00152 00153 Core::Result<void> escape(); 00154 }; 00155 00156 enum Bus { OneWire, I2C }; 00157 00158 Core::SerialPort * serialPort; 00159 Bus currentBus; 00160 DS2480BWithEscape ds2480b; 00161 OneWireMaster oneWireMaster_; 00162 DS9400WithEscape ds9400; 00163 I2CMaster i2cMaster_; 00164 00165 Core::Result<void> selectOneWire(); 00166 Core::Result<void> selectBus(Bus newBus); 00167 00168 friend class OneWireMaster; 00169 friend class I2CMaster; 00170 }; 00171 00172 } // namespace MaximInterfaceDevices 00173 00174 #endif
Generated on Tue Jul 12 2022 11:13:14 by
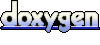