Device interface library for multiple platforms including Mbed.
Dependents: DeepCover Embedded Security in IoT MaximInterface MAXREFDES155#
DS2482_DS2484.hpp
00001 /******************************************************************************* 00002 * Copyright (C) Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 *******************************************************************************/ 00032 00033 #ifndef MaximInterfaceDevices_DS2482_DS2484_hpp 00034 #define MaximInterfaceDevices_DS2482_DS2484_hpp 00035 00036 #include <MaximInterfaceCore/I2CMaster.hpp> 00037 #include <MaximInterfaceCore/OneWireMaster.hpp> 00038 #include "Config.hpp" 00039 00040 namespace MaximInterfaceDevices { 00041 00042 /// Interface to the DS2484, DS2482-100, DS2482-101, DS2482-800 1-Wire masters. 00043 class DS2482_DS2484 : public Core::OneWireMaster { 00044 public: 00045 enum ErrorValue { HardwareError = 1, ArgumentOutOfRangeError }; 00046 00047 /// Represents a device configuration. 00048 class Config { 00049 public: 00050 /// Default construct with power-on config. 00051 explicit Config(uint_least8_t readByte = optionAPU) 00052 : readByte_(readByte & 0xF) {} 00053 00054 /// @name 1WS 00055 /// @brief 1-Wire Speed 00056 /// @{ 00057 00058 /// Get 1WS bit. 00059 bool get1WS() const { return (readByte_ & option1WS) == option1WS; } 00060 00061 /// Set 1WS bit. 00062 Config & set1WS(bool new1WS) { 00063 if (new1WS) { 00064 readByte_ |= option1WS; 00065 } else { 00066 readByte_ &= ~option1WS; 00067 } 00068 return *this; 00069 } 00070 00071 /// @} 00072 00073 /// @name SPU 00074 /// @brief Strong Pullup 00075 /// @{ 00076 00077 /// Get SPU bit. 00078 bool getSPU() const { return (readByte_ & optionSPU) == optionSPU; } 00079 00080 /// Set SPU bit. 00081 Config & setSPU(bool newSPU) { 00082 if (newSPU) { 00083 readByte_ |= optionSPU; 00084 } else { 00085 readByte_ &= ~optionSPU; 00086 } 00087 return *this; 00088 } 00089 00090 /// @} 00091 00092 /// @name PDN 00093 /// @brief 1-Wire Power Down 00094 /// @{ 00095 00096 /// Get PDN bit. 00097 bool getPDN() const { return (readByte_ & optionPDN) == optionPDN; } 00098 00099 /// Set PDN bit. 00100 Config & setPDN(bool newPDN) { 00101 if (newPDN) { 00102 readByte_ |= optionPDN; 00103 } else { 00104 readByte_ &= ~optionPDN; 00105 } 00106 return *this; 00107 } 00108 00109 /// @} 00110 00111 /// @name APU 00112 /// @brief Active Pullup 00113 /// @{ 00114 00115 /// Get APU bit. 00116 bool getAPU() const { return (readByte_ & optionAPU) == optionAPU; } 00117 00118 /// Set APU bit. 00119 Config & setAPU(bool newAPU) { 00120 if (newAPU) { 00121 readByte_ |= optionAPU; 00122 } else { 00123 readByte_ &= ~optionAPU; 00124 } 00125 return *this; 00126 } 00127 00128 /// @} 00129 00130 /// Byte representation that is read from the device. 00131 uint_least8_t readByte() const { return readByte_; } 00132 00133 private: 00134 static const unsigned int option1WS = 0x8; 00135 static const unsigned int optionSPU = 0x4; 00136 static const unsigned int optionPDN = 0x2; 00137 static const unsigned int optionAPU = 0x1; 00138 00139 uint_least8_t readByte_; 00140 }; 00141 00142 void setMaster(Core::I2CMaster & master) { this->master = &master; } 00143 00144 uint_least8_t address() const { return address_; } 00145 00146 void setAddress(uint_least8_t address) { address_ = address; } 00147 00148 /// Initialize hardware for use. 00149 MaximInterfaceDevices_EXPORT Core::Result<void> 00150 initialize(Config config = Config()); 00151 00152 /// @brief Write a new configuration to the device. 00153 /// @param[in] config New configuration to write. 00154 MaximInterfaceDevices_EXPORT Core::Result<void> writeConfig(Config config); 00155 00156 MaximInterfaceDevices_EXPORT virtual Core::Result<TripletData> 00157 triplet(bool sendBit); 00158 00159 MaximInterfaceDevices_EXPORT virtual Core::Result<void> reset(); 00160 00161 MaximInterfaceDevices_EXPORT virtual Core::Result<bool> 00162 touchBitSetLevel(bool sendBit, Level afterLevel); 00163 00164 MaximInterfaceDevices_EXPORT virtual Core::Result<uint_least8_t> 00165 readByteSetLevel(Level afterLevel); 00166 00167 MaximInterfaceDevices_EXPORT virtual Core::Result<void> 00168 writeByteSetLevel(uint_least8_t sendByte, Level afterLevel); 00169 00170 MaximInterfaceDevices_EXPORT virtual Core::Result<void> 00171 setSpeed(Speed newSpeed); 00172 00173 MaximInterfaceDevices_EXPORT virtual Core::Result<void> 00174 setLevel(Level newLevel); 00175 00176 MaximInterfaceDevices_EXPORT static const Core::error_category & 00177 errorCategory(); 00178 00179 protected: 00180 DS2482_DS2484(Core::I2CMaster & master, uint_least8_t address) 00181 : master(&master), address_(address) {} 00182 00183 /// @note Allow marking const since not public. 00184 Core::Result<void> sendCommand (uint_least8_t cmd) const; 00185 00186 /// @note Allow marking const since not public. 00187 Core::Result<void> sendCommand (uint_least8_t cmd, uint_least8_t param) const; 00188 00189 /// @brief Reads a register from the device. 00190 /// @param reg Register to read from. 00191 /// @returns Read data from register. 00192 Core::Result<uint_least8_t> readRegister(uint_least8_t reg) const; 00193 00194 /// @brief Reads the current register from the device. 00195 /// @returns Read data from register. 00196 Core::Result<uint_least8_t> readRegister() const; 00197 00198 private: 00199 /// @brief Performs a soft reset on the device. 00200 /// @note This is not a 1-Wire Reset. 00201 Core::Result<void> resetDevice(); 00202 00203 /// @brief 00204 /// Polls the device status waiting for the 1-Wire Busy bit (1WB) to be cleared. 00205 /// @returns Status byte or TimeoutError if poll limit reached. 00206 Core::Result<uint_least8_t> pollBusy(); 00207 00208 /// @brief Ensure that the desired 1-Wire level is set in the configuration. 00209 /// @param level Desired 1-Wire level. 00210 Core::Result<void> configureLevel(Level level); 00211 00212 Core::I2CMaster * master; 00213 uint_least8_t address_; 00214 Config curConfig; 00215 }; 00216 00217 } // namespace MaximInterfaceDevices 00218 namespace MaximInterfaceCore { 00219 00220 template <> 00221 struct is_error_code_enum<MaximInterfaceDevices::DS2482_DS2484::ErrorValue> 00222 : true_type {}; 00223 00224 } // namespace MaximInterfaceCore 00225 namespace MaximInterfaceDevices { 00226 00227 inline Core::error_code make_error_code(DS2482_DS2484::ErrorValue e) { 00228 return Core::error_code(e, DS2482_DS2484::errorCategory()); 00229 } 00230 00231 class DS2482_100 : public DS2482_DS2484 { 00232 public: 00233 DS2482_100(Core::I2CMaster & i2c_bus, uint_least8_t adrs) 00234 : DS2482_DS2484(i2c_bus, adrs) {} 00235 }; 00236 00237 /// DS2482-800 I2C to 1-Wire Master 00238 class DS2482_800 : public DS2482_DS2484 { 00239 public: 00240 DS2482_800(Core::I2CMaster & i2c_bus, uint_least8_t adrs) 00241 : DS2482_DS2484(i2c_bus, adrs) {} 00242 00243 /// @brief Select the active 1-Wire channel. 00244 /// @param channel Channel number to select from 0 to 7. 00245 MaximInterfaceDevices_EXPORT Core::Result<void> selectChannel(int channel); 00246 }; 00247 00248 /// DS2484 I2C to 1-Wire Master 00249 class DS2484 : public DS2482_DS2484 { 00250 public: 00251 /// @brief 1-Wire port adjustment parameters. 00252 /// @note See datasheet page 13. 00253 enum PortParameter { 00254 tRSTL = 0, 00255 tRSTL_OD, 00256 tMSP, 00257 tMSP_OD, 00258 tW0L, 00259 tW0L_OD, 00260 tREC0, // OD N/A 00261 RWPU = 8 // OD N/A 00262 }; 00263 00264 explicit DS2484(Core::I2CMaster & i2c_bus, uint_least8_t adrs = 0x30) 00265 : DS2482_DS2484(i2c_bus, adrs) {} 00266 00267 /// @brief Adjust 1-Wire port parameters. 00268 /// @param param Parameter to adjust. 00269 /// @param val New parameter value to set. Consult datasheet for value mappings. 00270 MaximInterfaceDevices_EXPORT Core::Result<void> 00271 adjustPort(PortParameter param, int val); 00272 }; 00273 00274 } // namespace MaximInterfaceDevices 00275 00276 #endif
Generated on Tue Jul 12 2022 11:13:06 by
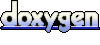