Device interface library for multiple platforms including Mbed.
Dependents: DeepCover Embedded Security in IoT MaximInterface MAXREFDES155#
DS1920.cpp
00001 /******************************************************************************* 00002 * Copyright (C) Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 *******************************************************************************/ 00032 00033 #include <MaximInterfaceCore/Error.hpp> 00034 #include <MaximInterfaceCore/OneWireMaster.hpp> 00035 #include "DS1920.hpp" 00036 00037 namespace MaximInterfaceDevices { 00038 00039 using namespace Core; 00040 00041 Result<void> DS1920::writeScratchpad(uint_least8_t th, uint_least8_t tl) { 00042 Result<void> result = selectRom(*master); 00043 if (result) { 00044 const uint_least8_t sendBlock[] = {0x4E, th, tl}; 00045 result = master->writeBlock(sendBlock); 00046 } 00047 return result; 00048 } 00049 00050 Result<DS1920::Scratchpad> DS1920::readScratchpad() const { 00051 Result<void> result = selectRom(*master); 00052 if (!result) { 00053 return result.error(); 00054 } 00055 result = master->writeByte(0xBE); 00056 if (!result) { 00057 return result.error(); 00058 } 00059 Scratchpad scratchpad; 00060 result = master->readBlock(scratchpad); 00061 if (!result) { 00062 return result.error(); 00063 } 00064 uint_least8_t receivedCrc; 00065 MaximInterfaceCore_TRY_VALUE(receivedCrc, master->readByte()); 00066 if (receivedCrc != calculateCrc8(scratchpad)) { 00067 return CrcError; 00068 } 00069 return scratchpad; 00070 } 00071 00072 Result<void> DS1920::copyScratchpad() { 00073 Result<void> result = selectRom(*master); 00074 if (result) { 00075 result = master->writeByteSetLevel(0x48, OneWireMaster::StrongLevel); 00076 if (result) { 00077 sleep->invoke(10); 00078 result = master->setLevel(OneWireMaster::NormalLevel); 00079 } 00080 } 00081 return result; 00082 } 00083 00084 Result<void> DS1920::convertTemperature() { 00085 Result<void> result = selectRom(*master); 00086 if (result) { 00087 result = master->writeByteSetLevel(0x44, OneWireMaster::StrongLevel); 00088 if (result) { 00089 sleep->invoke(750); 00090 result = master->setLevel(OneWireMaster::NormalLevel); 00091 } 00092 } 00093 return result; 00094 } 00095 00096 Result<void> DS1920::recallEeprom() { 00097 Result<void> result = selectRom(*master); 00098 if (result) { 00099 result = master->writeByte(0xB8); 00100 } 00101 return result; 00102 } 00103 00104 const error_category & DS1920::errorCategory() { 00105 static class : public error_category { 00106 public: 00107 virtual const char * name() const { return "MaximInterfaceDevices.DS1920"; } 00108 00109 virtual std::string message(int condition) const { 00110 switch (condition) { 00111 case CrcError: 00112 return "CRC Error"; 00113 00114 case DataError: 00115 return "Data Error"; 00116 } 00117 return defaultErrorMessage(condition); 00118 } 00119 } instance; 00120 return instance; 00121 } 00122 00123 Result<int> readTemperature(DS1920 & ds1920) { 00124 MaximInterfaceCore_TRY(ds1920.convertTemperature()); 00125 DS1920::Scratchpad scratchpad; 00126 MaximInterfaceCore_TRY_VALUE(scratchpad, ds1920.readScratchpad()); 00127 00128 unsigned int tempData = 00129 (static_cast<unsigned int>(scratchpad[1]) << 8) | scratchpad[0]; 00130 const unsigned int signMask = 0xFF00; 00131 int temperature; 00132 if ((tempData & signMask) == signMask) { 00133 temperature = -0x100; 00134 tempData &= ~signMask; 00135 } else if ((tempData & signMask) == 0) { 00136 temperature = 0; 00137 } else { 00138 return DS1920::DataError; 00139 } 00140 temperature += static_cast<int>(tempData); 00141 return temperature; 00142 } 00143 00144 } // namespace MaximInterfaceDevices
Generated on Tue Jul 12 2022 11:13:04 by
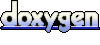