Device interface library for multiple platforms including Mbed.
Dependents: DeepCover Embedded Security in IoT MaximInterface MAXREFDES155#
DS18B20.hpp
00001 /******************************************************************************* 00002 * Copyright (C) Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 *******************************************************************************/ 00032 00033 #ifndef MaximInterfaceDevices_DS18B20_hpp 00034 #define MaximInterfaceDevices_DS18B20_hpp 00035 00036 #include <MaximInterfaceCore/array.hpp> 00037 #include <MaximInterfaceCore/SelectRom.hpp> 00038 #include <MaximInterfaceCore/Sleep.hpp> 00039 #include "Config.hpp" 00040 00041 namespace MaximInterfaceDevices { 00042 00043 /// @brief DS18B20 Programmable Resolution 1-Wire Digital Thermometer 00044 /// @details The DS18B20 digital thermometer provides 9-bit to 12-bit 00045 /// Celsius temperature measurements and has an alarm function with 00046 /// nonvolatile user-programmable upper and lower trigger points. The 00047 /// DS18B20 communicates over a 1-Wire bus that by definition requires 00048 /// only one data line (and ground) for communication with a central 00049 /// microprocessor. In addition, the DS18B20 can derive power directly 00050 /// from the data line ("parasite power"), eliminating the need for an 00051 /// external power supply. 00052 class DS18B20 { 00053 public: 00054 enum ErrorValue { CrcError = 1, DataError }; 00055 00056 static const uint_least8_t nineBitResolution = 0x1F; 00057 static const uint_least8_t tenBitResolution = 0x3F; 00058 static const uint_least8_t elevenBitResolution = 0x5F; 00059 static const uint_least8_t twelveBitResolution = 0x7F; 00060 00061 /// Holds the contents of the device scratchpad. 00062 typedef Core::array<uint_least8_t, 8> Scratchpad; 00063 00064 DS18B20(Core::Sleep & sleep, Core::OneWireMaster & master, 00065 const Core::SelectRom & selectRom) 00066 : selectRom(selectRom), master(&master), sleep(&sleep), resolution(0) {} 00067 00068 void setSleep(Core::Sleep & sleep) { this->sleep = &sleep; } 00069 00070 void setMaster(Core::OneWireMaster & master) { this->master = &master; } 00071 00072 void setSelectRom(const Core::SelectRom & selectRom) { 00073 this->selectRom = selectRom; 00074 } 00075 00076 /// Initializes the device for first time use. 00077 MaximInterfaceDevices_EXPORT Core::Result<void> initialize() const; 00078 00079 /// @brief Write Scratchpad Command 00080 /// @details If the result of a temperature measurement is higher 00081 /// than TH or lower than TL, an alarm flag inside the device is 00082 /// set. This flag is updated with every temperature measurement. 00083 /// As long as the alarm flag is set, the DS1920 will respond to 00084 /// the alarm search command. 00085 /// @param[in] th 8-bit upper temperature threshold, MSB indicates sign. 00086 /// @param[in] tl 8-bit lower temperature threshold, LSB indicates sign. 00087 /// @param[in] res Resolution of the DS18B20. 00088 MaximInterfaceDevices_EXPORT Core::Result<void> 00089 writeScratchpad(uint_least8_t th, uint_least8_t tl, uint_least8_t res); 00090 00091 /// @brief Read Scratchpad Command 00092 /// @returns scratchpad Contents of scratchpad. 00093 MaximInterfaceDevices_EXPORT Core::Result<Scratchpad> readScratchpad() const; 00094 00095 /// @brief Copy Scratchpad Command 00096 /// @details This command copies from the scratchpad into the 00097 /// EEPROM of the DS18B20, storing the temperature trigger bytes 00098 /// and resolution in nonvolatile memory. 00099 MaximInterfaceDevices_EXPORT Core::Result<void> copyScratchpad(); 00100 00101 /// @brief Read Power Supply command 00102 /// @details This command determines if the DS18B20 is parasite 00103 /// powered or has a local supply 00104 /// @returns 00105 /// True if the device is powered by a local power supply, or false if the 00106 /// device is parasitically powered. 00107 MaximInterfaceDevices_EXPORT Core::Result<bool> readPowerSupply() const; 00108 00109 /// @brief Convert Temperature Command 00110 /// @details This command begins a temperature conversion. 00111 MaximInterfaceDevices_EXPORT Core::Result<void> convertTemperature(); 00112 00113 /// @brief Recall Command 00114 /// @details This command recalls the temperature trigger values 00115 /// and resolution stored in EEPROM to the scratchpad. 00116 MaximInterfaceDevices_EXPORT Core::Result<void> recallEeprom(); 00117 00118 MaximInterfaceDevices_EXPORT static const Core::error_category & 00119 errorCategory(); 00120 00121 private: 00122 Core::SelectRom selectRom; 00123 Core::OneWireMaster * master; 00124 const Core::Sleep * sleep; 00125 mutable uint_least8_t resolution; 00126 }; 00127 00128 /// @brief Reads the current temperature as an integer value with decimal. 00129 /// @param ds18b20 Device to read. 00130 /// @returns Temperature in degrees Celsius multiplied by 16. 00131 MaximInterfaceDevices_EXPORT Core::Result<int> 00132 readTemperature(DS18B20 & ds18b20); 00133 00134 } // namespace MaximInterfaceDevices 00135 namespace MaximInterfaceCore { 00136 00137 template <> 00138 struct is_error_code_enum<MaximInterfaceDevices::DS18B20::ErrorValue> 00139 : true_type {}; 00140 00141 } // namespace MaximInterfaceCore 00142 namespace MaximInterfaceDevices { 00143 00144 inline Core::error_code make_error_code(DS18B20::ErrorValue e) { 00145 return Core::error_code(e, DS18B20::errorCategory()); 00146 } 00147 00148 } // namespace MaximInterfaceDevices 00149 00150 #endif
Generated on Tue Jul 12 2022 11:13:04 by
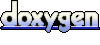