
Demo for MAXREFDES99
Embed:
(wiki syntax)
Show/hide line numbers
maxrefdes99.h
Go to the documentation of this file.
00001 /******************************************************************//** 00002 * @file maxrefdes99.h 00003 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a 00006 * copy of this software and associated documentation files (the "Software"), 00007 * to deal in the Software without restriction, including without limitation 00008 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00009 * and/or sell copies of the Software, and to permit persons to whom the 00010 * Software is furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included 00013 * in all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00016 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00017 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00018 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00019 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00020 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00021 * OTHER DEALINGS IN THE SOFTWARE. 00022 * 00023 * Except as contained in this notice, the name of Maxim Integrated 00024 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00025 * Products, Inc. Branding Policy. 00026 * 00027 * The mere transfer of this software does not imply any licenses 00028 * of trade secrets, proprietary technology, copyrights, patents, 00029 * trademarks, maskwork rights, or any other form of intellectual 00030 * property whatsoever. Maxim Integrated Products, Inc. retains all 00031 * ownership rights. 00032 **********************************************************************/ 00033 00034 #ifndef MAXREFDES99_H 00035 #define MAXREFDES99_H 00036 00037 00038 #include "mbed.h" 00039 #include "max7219.h" 00040 00041 00042 /**********************************************************//** 00043 * @brief Gets character bitmap fom memory 00044 * 00045 * @details 00046 * 00047 * On Entry: 00048 * @param[in] c - character to get bitmap for 00049 * @param[in] char_buff - pointer to buffer to store bitmap in 00050 * must be at least 6 bytes 00051 * 00052 * On Exit: 00053 * @return None 00054 **************************************************************/ 00055 void get_5x7_character(char c, uint8_t *char_buff); 00056 00057 00058 /**********************************************************//** 00059 * @brief Prints character to MAXREFDES99 at given position 00060 * 00061 * @details 00062 * 00063 * On Entry: 00064 * @param[in] p_display - pointer to Max7219 object 00065 * @param[in] position - position to print char to 00066 * posiion 1 is top left column, position 32 00067 * is bottom right column 00068 * @param[in] c - char to print 00069 * 00070 * On Exit: 00071 * @return None 00072 **************************************************************/ 00073 void print_char(Max7219 *p_display, uint8_t position, char c); 00074 00075 00076 /**********************************************************//** 00077 * @brief Prints given string to MAXREFDES99 at given position 00078 * 00079 * @details 00080 * 00081 * On Entry: 00082 * @param[in] p_display - pointer to Max7219 object 00083 * @param[in] position - position to print char to 00084 * posiion 1 is top left column, position 32 00085 * is bottom right column 00086 * @param[in] s - pointer to string to print 00087 * 00088 * On Exit: 00089 * @return None 00090 **************************************************************/ 00091 void print_string(Max7219 *p_display, uint8_t position, const char *s); 00092 00093 00094 /**********************************************************//** 00095 * @brief Shifts display right 'count' positions with given 00096 * delay between shifts 00097 * 00098 * @details 00099 * 00100 * On Entry: 00101 * @param[in] p_display - pointer to Max7219 object 00102 * @param[in] count - number of positions to shift 00103 * @param[in] delay - delay between shifts 00104 * 00105 * On Exit: 00106 * @return None 00107 **************************************************************/ 00108 void shift_display_right(Max7219 *p_display, uint8_t count, uint8_t delay); 00109 00110 00111 /**********************************************************//** 00112 * @brief Shifts display left 'count' positions with given 00113 * delay between shifts 00114 * 00115 * @details 00116 * 00117 * On Entry: 00118 * @param[in] p_display - pointer to Max7219 object 00119 * @param[in] count - number of positions to shift 00120 * @param[in] delay - delay between shifts 00121 * 00122 * On Exit: 00123 * @return None 00124 **************************************************************/ 00125 void shift_display_left(Max7219 *p_display, uint8_t count, uint8_t delay); 00126 00127 00128 /**********************************************************//** 00129 * @brief Turns on all leds for given quadrant, with quad 1 being 00130 * top left and quad 4 being bottom right 00131 * 00132 * @details 00133 * 00134 * On Entry: 00135 * @param[in] p_display - pointer to Max7219 object 00136 * @param[in] quad - see brief 00137 * 00138 * On Exit: 00139 * @return None 00140 **************************************************************/ 00141 void quad_all_on(Max7219 *p_display, uint8_t quad); 00142 00143 00144 /**********************************************************//** 00145 * @brief Turns off all leds for given quadrant, with quad 1 being 00146 * top left and quad 4 being bottom right 00147 * 00148 * @details 00149 * 00150 * On Entry: 00151 * @param[in] p_display - pointer to Max7219 object 00152 * @param[in] quad - see brief 00153 * 00154 * On Exit: 00155 * @return None 00156 **************************************************************/ 00157 void quad_all_off(Max7219 *p_display, uint8_t quad); 00158 00159 00160 /**********************************************************//** 00161 * @brief Turns all leds for whole display on 00162 * 00163 * @details 00164 * 00165 * On Entry: 00166 * @param[in] p_display - pointer to Max7219 object 00167 * 00168 * On Exit: 00169 * @return None 00170 **************************************************************/ 00171 void all_on(Max7219 *p_display); 00172 00173 00174 /**********************************************************//** 00175 * @brief Turns all leds for whole display off 00176 * 00177 * @details 00178 * 00179 * On Entry: 00180 * @param[in] p_display - pointer to Max7219 object 00181 * 00182 * On Exit: 00183 * @return None 00184 **************************************************************/ 00185 void all_off(Max7219 *p_display); 00186 00187 00188 /**********************************************************//** 00189 * @brief Demo loop for MAXREFDES99 00190 * 00191 * @details 00192 * 00193 * On Entry: 00194 * @param[in] p_display - pointer to Max7219 object 00195 * @param[in] display_config - structure holding configuration data 00196 * @param[in] endless_loop - if true run demo in endless loop 00197 * 00198 * On Exit: 00199 * @return None 00200 **************************************************************/ 00201 void demo(Max7219 *display, max7219_configuration_t display_config, bool endless_loop); 00202 00203 00204 /**********************************************************//** 00205 * @brief Shift display in given direction forever 00206 * 00207 * @details 00208 * 00209 * On Entry: 00210 * @param[in] p_display - pointer to Max7219 object 00211 * @param[in] scroll_right - if true shift right, else shift left 00212 * 00213 * On Exit: 00214 * @return None 00215 **************************************************************/ 00216 void endless_scroll_display(Max7219 *display, uint32_t scroll_right); 00217 00218 /**********************************************************//** 00219 * @brief Gets 16x16 character bitmap fom memory 00220 * 00221 * @details 00222 * 00223 * On Entry: 00224 * @param[in] c - character to get bitmap for 00225 * @param[in] char_buff - pointer to buffer to store bitmap 00226 * Buffer should be 32 bytes 00227 * @param[in] font_type - 16x16 font type used for display 00228 * On Exit: 00229 * @return None 00230 **************************************************************/ 00231 void get_16x16_character(char c, uint8_t *char_buff, uint8_t font_type); 00232 00233 00234 /**********************************************************//** 00235 * @brief Prints character to MAXREFDES99 16x16 LED display 00236 * 00237 * @details 00238 * 00239 * On Entry: 00240 * @param[in] p_display - pointer to Max7219 object 00241 @param[in] position - for single char print this value is zero, 00242 for string case it is multiple of 32 bytes as each char data is 32 bytes, 00243 * @param[in] c - char to print 00244 * @param[in] font_type - 16x16 font type used for display 00245 * 00246 * On Exit: 00247 * @return None 00248 **************************************************************/ 00249 void print_char_16x16(Max7219 *p_display, uint16_t position, char c, uint8_t font_type); 00250 00251 00252 /**********************************************************//** 00253 * @brief Prints given string to MAXREFDES99 16x16 LED display 00254 * 00255 * @details 00256 * 00257 * On Entry: 00258 * @param[in] p_display - pointer to Max7219 object 00259 * @param[in] s - pointer to string to print 00260 * @param[in] font_type - 16x16 font type used for display 00261 * 00262 * On Exit: 00263 * @return None 00264 **************************************************************/ 00265 void print_string_16x16(Max7219 *p_display, char *s, uint8_t font_type); 00266 00267 00268 /**********************************************************//** 00269 * @brief Shifts 16x16 font display right 'count' positions with given 00270 * delay between shifts 00271 * 00272 * @details 00273 * 00274 * On Entry: 00275 * @param[in] p_display - pointer to Max7219 object 00276 * @param[in] count - number of positions to shift 00277 * @param[in] delay - delay between shifts in milliseconds 00278 * 00279 * On Exit: 00280 * @return None 00281 **************************************************************/ 00282 void shift_display_right_16x16(Max7219 *p_display, uint8_t count, uint8_t delay); 00283 00284 00285 /**********************************************************//** 00286 * @brief Shifts 16x16 font display left 'count' positions with given 00287 * delay between shifts 00288 * 00289 * @details 00290 * 00291 * On Entry: 00292 * @param[in] p_display - pointer to Max7219 object 00293 * @param[in] count - number of positions to shift 00294 * @param[in] delay - delay between shifts in milliseconds 00295 * 00296 * On Exit: 00297 * @return None 00298 **************************************************************/ 00299 void shift_display_left_16x16(Max7219 *p_display, uint8_t count, uint8_t delay); 00300 00301 /**********************************************************//** 00302 * @brief Demo loop for MAXREFDES99 with 16x16 font display 00303 * 00304 * @details 00305 * 00306 * On Entry: 00307 * @param[in] p_display - pointer to Max7219 object 00308 * @param[in] display_config - structure holding configuration data 00309 * @param[in] font_type - 16x16 font type used for display 00310 * @param[in] endless_loop - if true run demo in endless loop 00311 * 00312 * On Exit: 00313 * @return None 00314 **************************************************************/ 00315 void demo_16x16(Max7219 *display, max7219_configuration_t display_config, uint8_t font_type, bool endless_loop); 00316 00317 00318 /**********************************************************//** 00319 * @brief Shift 16x16 font display in given direction forever 00320 * 00321 * @details 00322 * 00323 * On Entry: 00324 * @param[in] p_display - pointer to Max7219 object 00325 * @param[in] scroll_right - if true shift right, else shift left 00326 * 00327 * On Exit: 00328 * @return None 00329 **************************************************************/ 00330 void endless_scroll_display_16x16(Max7219 *display, uint32_t scroll_right); 00331 00332 00333 /**********************************************************//** 00334 * @brief Print demo menu 00335 * 00336 * @details 00337 * 00338 * On Entry: 00339 * 00340 * On Exit: 00341 * @return User entry 00342 **************************************************************/ 00343 uint32_t print_menu(void); 00344 00345 00346 /**********************************************************//** 00347 * @brief Get integer value from user 00348 * 00349 * @details 00350 * 00351 * On Entry: 00352 * @param[in] msg - prompt for user 00353 * @param[in] max_val - maximum allowable input 00354 * 00355 * On Exit: 00356 * @return User entry 00357 **************************************************************/ 00358 uint32_t get_user_input(char *msg, uint32_t max_val); 00359 00360 00361 /**********************************************************//** 00362 * @brief Get char from user 00363 * 00364 * @details 00365 * 00366 * On Entry: 00367 * @param[in] msg - prompt for user 00368 * 00369 * On Exit: 00370 * @return User entry 00371 **************************************************************/ 00372 char get_user_char(char *msg); 00373 00374 00375 /**********************************************************//** 00376 * @brief Get string from user 00377 * 00378 * @details 00379 * 00380 * On Entry: 00381 * @param[in] msg - prompt for user 00382 * 00383 * On Exit: 00384 * @return User entry 00385 **************************************************************/ 00386 char * get_user_string(char *msg); 00387 00388 00389 #endif /*MAXREFDES99_H*/ 00390
Generated on Tue Jul 12 2022 17:54:30 by
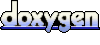