
Use the MAXREFDES99 to display the time from a DS3231 RTC. Requires the DS3231 RTC, or a MAXREFDES72 which has the rtc on it.
Dependencies: MAX7219 ds3231 mbed
main.cpp
00001 /*********************************************************************** 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 **********************************************************************/ 00032 00033 00034 #include "mbed.h" 00035 #include "ds3231.h" 00036 #include "maxrefdes99.h" 00037 #include <string> 00038 00039 00040 void set_rtc(Ds3231 & rtc); 00041 00042 00043 int main(void) 00044 { 00045 //rtc object 00046 Ds3231 rtc(D14, D15); 00047 00048 set_rtc(rtc); 00049 00050 Max7219 display(D11, D12, D13, D10); 00051 00052 //struct for holding MAX7219 configuration data 00053 max7219_configuration_t display_config; 00054 00055 //configuration data 00056 display_config.decode_mode = 0; //no BCD decode 00057 display_config.intensity = 0x0F; //max intensity 00058 display_config.scan_limit = 0x07; //scan all digits 00059 00060 //set number of MAX7219 devices being used 00061 display.set_num_devices(4); 00062 00063 //config display 00064 display.init_display(display_config); 00065 00066 //ensure all data registers are 0 00067 display.display_all_off(); 00068 00069 display.enable_display(); 00070 00071 time_t epoch_time; 00072 string str; 00073 char time_buff[32]; 00074 00075 for(;;) 00076 { 00077 //get epoch time from rtc 00078 epoch_time = rtc.get_epoch(); 00079 00080 //format time 00081 strftime(time_buff, 32, "%a %b %d %Y %I:%M %p", localtime(&epoch_time)); 00082 00083 //assign to str 00084 str.assign(time_buff); 00085 00086 //find '\n' and remove it 00087 if(str.find('\n') != std::string::npos) 00088 { 00089 str.erase(str.find('\n')); 00090 } 00091 00092 //append spaces to the end 00093 str.append(" "); 00094 00095 //print to display 00096 print_string(&display, 33, str.c_str()); 00097 00098 //shift the display the appropriate number of positions 00099 //1 char is 6 columns wide 00100 shift_display_left(&display, (str.length()*6), 150); 00101 00102 //clear the display 00103 all_off(&display); 00104 00105 //clears all data in buffer 00106 clear_buffer(); 00107 00108 wait(0.5); 00109 } 00110 } 00111 00112 00113 //********************************************************************* 00114 void set_rtc(Ds3231 & rtc) 00115 { 00116 //default, use bit masks in ds3231.h for desired operation 00117 ds3231_cntl_stat_t rtc_control_status = {0,0}; 00118 ds3231_time_t rtc_time; 00119 ds3231_calendar_t rtc_calendar; 00120 00121 rtc.set_cntl_stat_reg(rtc_control_status); 00122 00123 //get day from user 00124 rtc_calendar.day = get_user_input("\nPlease enter day of week, 1 for Sunday (1-7): ", 7); 00125 00126 //get day of month from user 00127 rtc_calendar.date = get_user_input("\nPlease enter day of month (1-31): ", 31); 00128 00129 //get month from user 00130 rtc_calendar.month = get_user_input("\nPlease enter the month, 1 for January (1-12): ", 12); 00131 00132 //get year from user 00133 rtc_calendar.year = get_user_input("\nPlease enter the year (0-99): ", 99); 00134 00135 //Get time mode 00136 rtc_time.mode = get_user_input("\nWhat time mode? 1 for 12hr 0 for 24hr: ", 1); 00137 00138 if(rtc_time.mode) 00139 { 00140 //Get AM/PM status 00141 rtc_time.am_pm = get_user_input("\nIs it AM or PM? 0 for AM 1 for PM: ", 1); 00142 //Get hour from user 00143 rtc_time.hours = get_user_input("\nPlease enter the hour (1-12): ", 12); 00144 } 00145 else 00146 { 00147 //Get hour from user 00148 rtc_time.hours = get_user_input("\nPlease enter the hour (0-23): ", 23); 00149 } 00150 00151 //Get minutes from user 00152 rtc_time.minutes = get_user_input("\nPlease enter the minute (0-59): ", 59); 00153 00154 00155 //Get seconds from user 00156 rtc_time.seconds = get_user_input("\nPlease enter the second (0-59): ", 59); 00157 00158 rtc.set_time(rtc_time); 00159 rtc.set_calendar(rtc_calendar); 00160 } 00161 00162
Generated on Thu Jul 14 2022 09:39:00 by
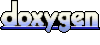