
MAXREFDES143#: DeepCover Embedded Security in IoT Authenticated Sensing & Notification
Dependencies: MaximInterface mbed
Factory.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 *******************************************************************************/ 00032 00033 #include <MaximInterface/Devices/DS2465.hpp> 00034 #include <MaximInterface/Devices/DS28E15_22_25.hpp> 00035 #include <MaximInterface/Utilities/Segment.hpp> 00036 #include "Factory.hpp" 00037 #include "SensorNode.hpp" 00038 00039 using namespace MaximInterface; 00040 00041 /// The valid master secret for the system. 00042 static const Sha256::Hash masterSecret = { 00043 0x11, 0x12, 0x13, 0x14, 0x15, 0x16, 0x17, 0x18, 0x19, 0x1A, 0x1B, 00044 0x1C, 0x1D, 0x1E, 0x1F, 0x21, 0x22, 0x23, 0x24, 0x25, 0x26, 0x27, 00045 0x28, 0x29, 0x2A, 0x2B, 0x2C, 0x2D, 0x2E, 0x2F, 0x31, 0x32}; 00046 /// An invalid master secret for example purposes. 00047 static const Sha256::Hash invalidMasterSecret = { 00048 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 00049 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 00050 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00}; 00051 00052 bool provisionCoprocessor(DS2465 & ds2465) { 00053 bool result = !ds2465.writeMasterSecret(masterSecret); 00054 if (result) { 00055 SensorNode::AuthData authData; 00056 DS28E15::Page pageData; 00057 pageData.fill(uint8_t(SensorNode::defaultPaddingByte)); 00058 const std::pair<DS28E15::Page::iterator, DS28E15::Page::iterator> 00059 pageSegment = 00060 createSegment(pageData.begin(), pageData.end(), 00061 authData.segment.size(), authData.segmentNum); 00062 if (pageSegment.first != pageData.end()) { 00063 std::copy(authData.segment.begin(), authData.segment.end(), 00064 pageSegment.first); 00065 } 00066 result = !ds2465.writePage(0, pageData); 00067 } 00068 return result; 00069 } 00070 00071 bool provisionSensorNode(SensorNode & sensorNode, bool validSecret) { 00072 const int blockNum = sensorNode.authData.pageNum / 2; 00073 const DS28E15::BlockProtection desiredProtection( 00074 false, false, false, true, blockNum); // Authentication Protection only 00075 00076 // Reset to starting defaults 00077 sensorNode.authData.reset(); 00078 00079 // Read current protection status 00080 DS28E15::BlockProtection protectionStatus; 00081 bool result = 00082 !sensorNode.ds28e15.readBlockProtection(blockNum, protectionStatus); 00083 // Check if invalid protections are set 00084 if (result) 00085 result = ((protectionStatus.statusByte() & 00086 ~(desiredProtection.statusByte())) == 0x00); 00087 // Load secret into scratchpad 00088 if (result) 00089 result = !sensorNode.ds28e15.writeScratchpad( 00090 validSecret ? masterSecret : invalidMasterSecret); 00091 // Load master secret from scratchpad without locking 00092 if (result) 00093 result = !sensorNode.ds28e15.loadSecret(false); 00094 00095 // Setup is complete if not using a valid secret 00096 if (!validSecret) 00097 return result; 00098 00099 // Create constant partial secret 00100 DS28E15::Scratchpad partialSecret; 00101 DS28E15::Page pageData; 00102 partialSecret.fill(uint8_t(SensorNode::defaultPaddingByte)); 00103 00104 // Read page data 00105 if (result) 00106 result = 00107 !sensorNode.ds28e15.readPage(sensorNode.authData.pageNum, pageData); 00108 // Load partial secret into scratchpad 00109 if (result) 00110 result = !sensorNode.ds28e15.writeScratchpad(partialSecret); 00111 // Compute secret 00112 if (result) 00113 result = 00114 !sensorNode.ds28e15.computeSecret(sensorNode.authData.pageNum, false); 00115 // Configure slave secret on DS2465 00116 if (result) { 00117 const Sha256::SlaveSecretData data = DS28E15::createSlaveSecretData( 00118 pageData, sensorNode.authData.pageNum, partialSecret, 00119 sensorNode.romId(), sensorNode.manId); 00120 result = !sensorNode.ds2465.computeSlaveSecret(data); 00121 } 00122 // Enable authentication protection if not set 00123 if (result && (protectionStatus != desiredProtection)) { 00124 const Sha256::WriteMacData data = DS28E15::createProtectionWriteMacData( 00125 desiredProtection, protectionStatus, sensorNode.romId(), 00126 sensorNode.manId); 00127 Sha256::Hash mac; 00128 result = !sensorNode.ds2465.computeWriteMac(data, mac); 00129 if (result) 00130 result = 00131 !sensorNode.ds28e15.writeAuthBlockProtection(desiredProtection, mac); 00132 } 00133 // Write initial filter life and set all other segments to default value 00134 if (result) { 00135 DS28E15::Segment blankSegment; 00136 blankSegment.fill(uint8_t(SensorNode::defaultPaddingByte)); 00137 for (int i = 0; i < DS28E15::segmentsPerPage; i++) { 00138 const DS28E15::Segment newSegment = (i == sensorNode.authData.segmentNum) 00139 ? sensorNode.authData.segment 00140 : blankSegment; 00141 const std::pair<DS28E15::Page::iterator, DS28E15::Page::iterator> 00142 pageSegment = createSegment(pageData.begin(), pageData.end(), 00143 DS28E15::Segment::size(), i); 00144 DS28E15::Segment oldSegment; 00145 std::copy(pageSegment.first, pageSegment.second, oldSegment.begin()); 00146 const Sha256::WriteMacData data = DS28E15::createSegmentWriteMacData( 00147 sensorNode.authData.pageNum, i, newSegment, oldSegment, 00148 sensorNode.romId(), sensorNode.manId); 00149 Sha256::Hash mac; 00150 result = !sensorNode.ds2465.computeWriteMac(data, mac); 00151 if (!result) 00152 break; 00153 result = !sensorNode.ds28e15.writeAuthSegment(sensorNode.authData.pageNum, 00154 i, newSegment, mac); 00155 if (!result) 00156 break; 00157 } 00158 } 00159 00160 // Reload secret with known page values 00161 // Load master secret into scratchpad 00162 if (result) 00163 result = !sensorNode.ds28e15.writeScratchpad(masterSecret); 00164 // Load master secret 00165 if (result) 00166 result = !sensorNode.ds28e15.loadSecret(false); 00167 // Read page data 00168 if (result) 00169 result = 00170 !sensorNode.ds28e15.readPage(sensorNode.authData.pageNum, pageData); 00171 // Write partial secret to scratchpad 00172 if (result) 00173 result = !sensorNode.ds28e15.writeScratchpad(partialSecret); 00174 // Compute secret 00175 if (result) 00176 result = 00177 !sensorNode.ds28e15.computeSecret(sensorNode.authData.pageNum, false); 00178 // Configure slave secret on DS2465 00179 if (result) { 00180 const Sha256::SlaveSecretData data = DS28E15::createSlaveSecretData( 00181 pageData, sensorNode.authData.pageNum, partialSecret, 00182 sensorNode.romId(), sensorNode.manId); 00183 result = !sensorNode.ds2465.computeSlaveSecret(data); 00184 } 00185 00186 return result; 00187 }
Generated on Wed Jul 13 2022 21:31:02 by
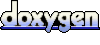