Library for the MAX7219 LED display driver
Fork of MAX7219 by
Embed:
(wiki syntax)
Show/hide line numbers
max7219.cpp
Go to the documentation of this file.
00001 /******************************************************************//** 00002 * @file max7219.cpp 00003 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a 00006 * copy of this software and associated documentation files (the "Software"), 00007 * to deal in the Software without restriction, including without limitation 00008 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00009 * and/or sell copies of the Software, and to permit persons to whom the 00010 * Software is furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included 00013 * in all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00016 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00017 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00018 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00019 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00020 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00021 * OTHER DEALINGS IN THE SOFTWARE. 00022 * 00023 * Except as contained in this notice, the name of Maxim Integrated 00024 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00025 * Products, Inc. Branding Policy. 00026 * 00027 * The mere transfer of this software does not imply any licenses 00028 * of trade secrets, proprietary technology, copyrights, patents, 00029 * trademarks, maskwork rights, or any other form of intellectual 00030 * property whatsoever. Maxim Integrated Products, Inc. retains all 00031 * ownership rights. 00032 **********************************************************************/ 00033 00034 00035 #include "max7219.h" 00036 00037 00038 //********************************************************************* 00039 Max7219::Max7219(SPI *spi_bus, PinName cs): _p_spi(spi_bus) 00040 { 00041 _num_devices = 1; 00042 00043 _p_cs = new DigitalOut(cs, 1); 00044 _spi_owner = false; 00045 } 00046 00047 00048 //********************************************************************* 00049 Max7219::Max7219(PinName mosi, PinName miso, PinName sclk, PinName cs) 00050 { 00051 _num_devices = 1; 00052 00053 _p_spi = new SPI(mosi, miso, sclk); 00054 _p_cs = new DigitalOut(cs, 1); 00055 00056 _spi_owner = true; 00057 } 00058 00059 00060 //********************************************************************* 00061 Max7219::~Max7219() 00062 { 00063 delete _p_cs; 00064 00065 if(_spi_owner) 00066 { 00067 delete _p_spi; 00068 } 00069 } 00070 00071 00072 //********************************************************************* 00073 int32_t Max7219::set_num_devices(uint8_t num_devices) 00074 { 00075 int32_t rtn_val = -1; 00076 00077 if(num_devices > 0) 00078 { 00079 _num_devices = num_devices; 00080 rtn_val = _num_devices; 00081 } 00082 00083 return(rtn_val); 00084 } 00085 00086 00087 //********************************************************************* 00088 void Max7219::set_display_test(void) 00089 { 00090 uint8_t idx = 0; 00091 00092 _p_cs->write(0); 00093 for(idx = 0; idx < _num_devices; idx++) 00094 { 00095 _p_spi->write(MAX7219_DISPLAY_TEST); 00096 _p_spi->write(1); 00097 } 00098 _p_cs->write(1); 00099 } 00100 00101 00102 //********************************************************************* 00103 void Max7219::clear_display_test(void) 00104 { 00105 uint8_t idx = 0; 00106 00107 _p_cs->write(0); 00108 for(idx = 0; idx < _num_devices; idx++) 00109 { 00110 _p_spi->write(MAX7219_DISPLAY_TEST); 00111 _p_spi->write(0); 00112 } 00113 _p_cs->write(1); 00114 } 00115 00116 00117 //********************************************************************* 00118 int32_t Max7219::init_device(max7219_configuration_t config) 00119 { 00120 int32_t rtn_val = -1; 00121 uint8_t idx = 0; 00122 00123 if(config.device_number > _num_devices) 00124 { 00125 rtn_val = -1; 00126 } 00127 else if(config.device_number == 0) 00128 { 00129 //device numbering starts with index 1 00130 rtn_val = -2; 00131 } 00132 else 00133 { 00134 //write DECODE_MODE register of device 00135 _p_cs->write(0); 00136 for(idx = _num_devices; idx > 0; idx--) 00137 { 00138 if(config.device_number == idx) 00139 { 00140 _p_spi->write(MAX7219_DECODE_MODE); 00141 _p_spi->write(config.decode_mode); 00142 } 00143 else 00144 { 00145 _p_spi->write(MAX7219_NO_OP); 00146 _p_spi->write(0); 00147 } 00148 } 00149 _p_cs->write(1); 00150 00151 wait_us(1); 00152 00153 //write INTENSITY register of device 00154 _p_cs->write(0); 00155 for(idx = _num_devices; idx > 0; idx--) 00156 { 00157 if(config.device_number == idx) 00158 { 00159 _p_spi->write(MAX7219_INTENSITY); 00160 _p_spi->write(config.intensity); 00161 } 00162 else 00163 { 00164 _p_spi->write(MAX7219_NO_OP); 00165 _p_spi->write(0); 00166 } 00167 } 00168 _p_cs->write(1); 00169 00170 wait_us(1); 00171 00172 //write SCAN_LIMT register of device 00173 _p_cs->write(0); 00174 for(idx = _num_devices; idx > 0; idx--) 00175 { 00176 if(config.device_number == idx) 00177 { 00178 _p_spi->write(MAX7219_SCAN_LIMIT); 00179 _p_spi->write(config.scan_limit); 00180 } 00181 else 00182 { 00183 _p_spi->write(MAX7219_NO_OP); 00184 _p_spi->write(0); 00185 } 00186 } 00187 _p_cs->write(1); 00188 00189 wait_us(1); 00190 00191 rtn_val = 0; 00192 } 00193 00194 return(rtn_val); 00195 } 00196 00197 00198 //********************************************************************* 00199 void Max7219::init_display(max7219_configuration_t config) 00200 { 00201 uint8_t idx = 0; 00202 00203 //write DECODE_MODE register of all devices 00204 _p_cs->write(0); 00205 for(idx = 0; idx < _num_devices; idx++) 00206 { 00207 _p_spi->write(MAX7219_DECODE_MODE); 00208 _p_spi->write(config.decode_mode); 00209 } 00210 _p_cs->write(1); 00211 00212 wait_us(1); 00213 00214 //write INTENSITY register of all devices 00215 _p_cs->write(0); 00216 for(idx = 0; idx < _num_devices; idx++) 00217 { 00218 _p_spi->write(MAX7219_INTENSITY); 00219 _p_spi->write(config.intensity); 00220 } 00221 _p_cs->write(1); 00222 00223 wait_us(1); 00224 00225 //write SCAN_LIMT register of all devices 00226 _p_cs->write(0); 00227 for(idx = 0; idx < _num_devices; idx++) 00228 { 00229 _p_spi->write(MAX7219_SCAN_LIMIT); 00230 _p_spi->write(config.scan_limit); 00231 } 00232 _p_cs->write(1); 00233 00234 wait_us(1); 00235 } 00236 00237 //************************************************************* 00238 void Max7219::set_intensity(max7219_configuration_t config) 00239 { 00240 uint8_t idx = 0; 00241 wait_us(1); 00242 00243 //write INTENSITY register of device 00244 _p_cs->write(0); 00245 for(idx = _num_devices; idx > 0; idx--) 00246 { 00247 if(config.device_number == idx) 00248 { 00249 _p_spi->write(MAX7219_INTENSITY); 00250 _p_spi->write(config.intensity); 00251 } 00252 else 00253 { 00254 _p_spi->write(MAX7219_NO_OP); 00255 _p_spi->write(0); 00256 } 00257 } 00258 _p_cs->write(1); 00259 00260 wait_us(1); 00261 } 00262 00263 //********************************************************************* 00264 int32_t Max7219::enable_device(uint8_t device_number) 00265 { 00266 int32_t rtn_val = -1; 00267 uint8_t idx = 0; 00268 00269 if(device_number > _num_devices) 00270 { 00271 rtn_val = -1; 00272 } 00273 else if(device_number == 0) 00274 { 00275 //device numbering starts with index 1 00276 rtn_val = -2; 00277 } 00278 else 00279 { 00280 _p_cs->write(0); 00281 for(idx = _num_devices; idx > 0; idx--) 00282 { 00283 if(device_number == idx) 00284 { 00285 _p_spi->write(MAX7219_SHUTDOWN); 00286 _p_spi->write(1); 00287 } 00288 else 00289 { 00290 _p_spi->write(MAX7219_NO_OP); 00291 _p_spi->write(0); 00292 } 00293 } 00294 _p_cs->write(1); 00295 00296 rtn_val = 0; 00297 } 00298 00299 return(rtn_val); 00300 } 00301 00302 00303 //********************************************************************* 00304 00305 00306 00307 //********************************************************************* 00308 int32_t Max7219::disable_device(uint8_t device_number) 00309 { 00310 int32_t rtn_val = -1; 00311 uint8_t idx = 0; 00312 00313 if(device_number > _num_devices) 00314 { 00315 rtn_val = -1; 00316 } 00317 else if(device_number == 0) 00318 { 00319 //device numbering starts with index 1 00320 rtn_val = -2; 00321 } 00322 else 00323 { 00324 _p_cs->write(0); 00325 for(idx = _num_devices; idx > 0; idx--) 00326 { 00327 if(device_number == idx) 00328 { 00329 _p_spi->write(MAX7219_SHUTDOWN); 00330 _p_spi->write(0); 00331 } 00332 else 00333 { 00334 _p_spi->write(MAX7219_NO_OP); 00335 _p_spi->write(0); 00336 } 00337 } 00338 _p_cs->write(1); 00339 00340 rtn_val = 0; 00341 } 00342 00343 return(rtn_val); 00344 } 00345 00346 00347 //********************************************************************* 00348 int32_t Max7219::write_digit(uint8_t device_number, uint8_t digit, uint8_t data) 00349 { 00350 int32_t rtn_val = -1; 00351 uint8_t idx = 0; 00352 00353 if(digit > MAX7219_DIGIT_7) 00354 { 00355 rtn_val = -3; 00356 } 00357 else if(digit < MAX7219_DIGIT_0) 00358 { 00359 rtn_val = -4; 00360 } 00361 else 00362 { 00363 if(device_number > _num_devices) 00364 { 00365 rtn_val = -1; 00366 } 00367 else if(device_number == 0) 00368 { 00369 rtn_val = -2; 00370 } 00371 else 00372 { 00373 _p_cs->write(0); 00374 for(idx = _num_devices; idx > 0; idx--) 00375 { 00376 if(idx == device_number) 00377 { 00378 _p_spi->write(digit); 00379 _p_spi->write(data); 00380 } 00381 else 00382 { 00383 _p_spi->write(MAX7219_NO_OP); 00384 _p_spi->write(0); 00385 } 00386 } 00387 _p_cs->write(1); 00388 00389 rtn_val = 0; 00390 } 00391 } 00392 00393 return(rtn_val); 00394 } 00395 00396 00397 //********************************************************************* 00398 int32_t Max7219::clear_digit(uint8_t device_number, uint8_t digit) 00399 { 00400 int32_t rtn_val = -1; 00401 uint8_t idx = 0; 00402 00403 if(digit > MAX7219_DIGIT_7) 00404 { 00405 rtn_val = -3; 00406 } 00407 else if(digit < MAX7219_DIGIT_0) 00408 { 00409 rtn_val = -4; 00410 } 00411 else 00412 { 00413 if(device_number > _num_devices) 00414 { 00415 rtn_val = -1; 00416 } 00417 else if(device_number == 0) 00418 { 00419 rtn_val = -2; 00420 } 00421 else 00422 { 00423 _p_cs->write(0); 00424 for(idx = _num_devices; idx > 0; idx--) 00425 { 00426 if(idx == device_number) 00427 { 00428 _p_spi->write(digit); 00429 _p_spi->write(0); 00430 } 00431 else 00432 { 00433 _p_spi->write(MAX7219_NO_OP); 00434 _p_spi->write(0); 00435 } 00436 } 00437 _p_cs->write(1); 00438 00439 rtn_val = 0; 00440 } 00441 } 00442 00443 return(rtn_val); 00444 } 00445
Generated on Tue Jul 12 2022 19:43:24 by
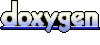